Understanding Run Length Encoding
Run Length Encoding (RLE) is an elementary form of data compression that can be efficiently implemented in computer systems. RLE proves particularly useful when you have large datasets with repeating values. Before we delve into understanding how RLE works, let's begin with understanding what exactly RLE is.What is Run Length Encoding?
Run Length Encoding (RLE) is a form of data compression whereby sequences of data are stored as a single count and data value.
Basic principles of Run Length Encoding
RLE is based on two primary principles:- Efficiency: It works best with repetitive data because it records the frequency of specific values, thereby eliminating the need to record each repetition separately.
- Simplicity: It uses a simplistic methodology of storing repeating values as a single set making it easy to understand and implement.
Efficiency and simplicity underpin the principle of RLE. It's efficient because it reduces storage needs for repetitive data, and it's straightforward because it is easy to code and implement.
How does Run Length Encoding work?
RLE operates by replacing sequences of the same data values within a dataset with a pair of the count number and the repeated value. It thus simplifies datasets with a lot of repetitive data.For example, consider the string "AAABBBCC". Using RLE, this would be condensed into "3A3B2C", denoting three 'A's, three 'B's and two 'C's.
Step-by-step process of Run Length Encoding
Here's a step-by-step breakdown of how RLE works:- Identify repeating data values in the dataset.
- Replace the repeating sequence with a pair comprising of the number of repetitions and the repeated value.
- Continue this process for all repeating sequences in the dataset.
char[] arr = new char[]{'A','A','A','A','B','B','B'}; for (int i = 0; i < arr.length; i++) { int runLength = 1; while (i + 1 < arr.length && arr[i] == arr[i + 1]) { i++; runLength++; } System.out.println(runLength + "" + arr[i]); }This will print: 4A3B It is important to remember that RLE is best suited for data with many runs of identical values. For data with a small number of repetitions, RLE might inadvertently increase the dataset size. Hence, the type of data is an essential factor to consider when deciding to use RLE as a compression method.
Python Run Length Encoding
In the realm of Python programming, Run Length Encoding is frequently utilised as a simple yet effective data compression technique. By virtue of Python’s easily readable syntax and extensive collection of built-in functions, implementing Run Length Encoding can be accomplished with relative ease.Implementing Run Length Encoding in Python
When you have to compress data with long runs of similar values in Python, Run Length Encoding offers a prime solution. To implement RLE, you first initiate an empty Python list or string to hold the encoded data. Beginning with the first element in a data set, you keep a count of the sequence of identical characters or values. As soon as a different element is encountered, the previous character and count are appended to your RLE list/string, and the count restarts for this new value. Repeat this process for all elements in the data set. Here's a step-by-step process for implementing RLE in Python:- Initialise an empty list or string for holding the encoded data.
- Begin the count of repeating characters with the first element.
- Append the character and count to the RLE list/string when a different value is encountered.
- Continue the process for all elements in the data set.
A simple Python function that implements RLE on a string of characters could look like this:
def run_length_encoding(input_str): encoding = '' i = 0 while i < len(input_str): count = 1 while i + 1 < len(input_str) and input_str[i] == input_str[i+1]: i += 1 count += 1 encoding += str(count) + input_str[i] i += 1 return encoding
Python Run Length Encoding: An Easy Guide
This guide aims to simplify the process of implementing RLE in Python. First, input_str is the string that we wish to compress using RLE. The encoding of the string is stored in the variable 'encoding'. The outer while loop traverses through each character in the string. The inner while loop is only used if the current character is the same as the next character. If they are the same, the loop increments the count of the current character and increments the pointer 'i' by one. Once a different character is found, the count and the current character are appended to the 'encoding' string. The outer loop then moves on to the next character in the input string. The returned string 'encoding' contains the Run Length Encoding of the input string. The time complexity of this approach is \(O(n)\), where \(n\) is the length of the input string.Analysing the Python Run Length Encoding Example
Let's examine the Python RLE function in action. Passing 'AAABBBCCC' as the input_string into the function, you would receive '3A3B3C' as the encoded string. This encoded string depicts that 'A', 'B', and 'C' each repeat themselves 3 times in the original data. Alternately passing 'AABBCC' as the input_string yields '2A2B2C' as the encoded string, showing that 'A', 'B', and 'C' each repeat 2 times. Successful outputs confirm that the RLE function is working correctly, indicating that sequences with identical characters are being adequately encoded into a single character-frequency pair. Please note that if the input string contains characters that do not repeat, such as 'ABC', the output ('1A1B1C') is longer than the input. This highlights that RLE may not always result in data compression and is most suitably utilised on data that contains suitable patterns of repeating characters. In conclusion, Python Run Length Encoding is a helpful addition to your data compression toolkit, especially for datasets where specific values exhibit a high frequency of occurrence.Binary Run Length Encoding
When referring to data compression techniques, Run Length Encoding (RLE) plays a significant role with its simplicity and easy implementation. Binary Run Length Encoding is a specification of RLE that solely deals with binary data – sequences that include only two types of values, typically represented as 0 and 1.Using Binary Run Length Encoding for Data Compression
Binary Run Length Encoding aims to minimise the storage needs of binary sequences that contain long runs of the same values. It does this by storing each series of consecutive identical numbers as a pair. The pair contains the length of the sequence (run length) and the number being repeated (0 or 1). The algorithm scans the binary input from left to right. The encoding begins with the first number, and it counts the number of times this 'runs' or repeats consecutively, before a different number appears. The count (run length) and the binary number constitute a pair, which then forms the encoded result. This process repeats with the next different number and continues until the end of the binary input. As with standard RLE, Binary Run Length Encoding is most efficient when the data has long runs of 0's or 1's. On the other hand, alternating values such as '010101' could result in a longer encoded result than the original sequence. Consider working with a binary image data, where long runs of white or black pixels can be encoded efficiently. If the image is mostly white with few black pixels, the RLE for this binary data could considerably reduce the storage size.binary_input = '000001111100000111' i = 0 RLE = [] while(i < len(binary_input)): count = 1 while (i + 1 < len(binary_input) and binary_input[i] == binary_input[i + 1]): i += 1 count += 1 RLE.append((count, int(binary_input[i]))) i += 1 print(RLE)The script prints [(5, 0), (5, 1), (5, 0), (3, 1)], representing lengths of sequences for '0's and '1's respectively in the binary data.
Interpreting Binary Run Length Encoding
Interpreting Binary Run Length Encoding involves the conversion of compressed binary data back into its original form. This is a crucial ability, as the ultimate aim of data compression is not just to save space, but to be able to retrieve the original data when needed. Decoding Binary RLE is quite straightforward. For each pair in the encoded sequence, append the binary number (0 or 1) to the output string the number of times specified by the run length. Process each pair in the sequence this way, and you will end up with the original binary sequence.RLE = [(5, 0), (5, 1), (5, 0), (3, 1)] binary_output = '' for pair in RLE: count, value = pair binary_output += str(value) * count print(binary_output)This script will provide the output '000001111100000111' - the original binary sequence. When using Binary RLE, it's important to keep in mind:
- The original data should consist of long runs of similar values for the encoding to be efficient.
- RLE isn't effective on data with frequently alternating binary values, as it might produce a longer result than the input.
- Both the encoding and decoding process have a time complexity of \(O(n)\), making them efficient to run on large binary data.
Decompressing Run-Length Encoded Lists
After understanding the process of encoding data with Run Length Encoding, it's just as important to explore how to reverse this process. Decompressing RLE data involves understanding the pairs of run lengths and data values, and using this information to rebuild the original data set.A Deep Dive into Decompressing Run-Length Encoded Lists
Decompressing Run-Length Encoded Lists intervenes when you need to revert your encoded list back to its original. To accomplish this, we use the information contained in the RLE pairs to rebuild the data. The process is relatively straightforward: for each pair, take the second value and repeat it the number of times specified in the first place of the pair. The decompression implementation is as follows: Start by creating an empty list to store the decompressed data. Iterate over the encoded list, which should contain pairs of data in the format (run length, data value). For each pair, append the data value to the new list the number of times specified by the run length. With Python, this process becomes simpler thanks to the built-in list multiplication function. Here's a step-by-step process:- Initialise an empty list to store the decompressed data.
- Iterate over the pairs in the encoded list.
- For each pair, append the data value to the new list, repeating it as many times as the run length indicates.
encoded_list = [(4, 'A'), (3, 'B'), (2, 'C')] decompressed_list = [] for pair in encoded_list: run_length, data_value = pair decompressed_list += [data_value] * run_length print(decompressed_list)This script creates a list ['A', 'A', 'A', 'A', 'B', 'B', 'B', 'C', 'C'], which is the original data before it was run-length encoded into [(4, 'A'), (3, 'B'), (2, 'C')]. The time complexity of this implementation is still \(O(n)\), due to the iteration over the list elements. It is crucial to note that although the decompression might create large lists depending on the data and run lengths, decompression is considered a fast operation as it mostly involves repeating values in a list.
Example of Decompressing a Run-Length Encoded List
Consider the run-length encoded list [(5, 0), (3, 1), (6, 0)]. It signifies that '0' repeats five times, followed by '1' repeating thrice, and then '0' repeating six times in the original list.encoded_list = [(5, 0), (3, 1), (6, 0)] decompressed_list = [] for pair in encoded_list: run_length, data_value = pair decompressed_list += [data_value] * run_length print(decompressed_list)The script outputs the list [0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 0, 0, 0], restoring the original sequence of values. Decompressing a run-length encoded list symbolises the beneficial side of encoding data by using Run Length Encoding – the embedded quality of compressing data without losing any information. It is a reversible data compression algorithm, retaining the ability to restore the original data perfectly. When using RLE and its decompression, however, always bear in mind that this method is specifically useful when dealing with data that contains long sequences of similar values. The algorithm does not perform well on data that consist of frequent alternations between different values. The decompressed data could end up larger than the original if no suitable runs exist in the data. Finally, while decompressing, understand that large run lengths could lead to a significant increase in the amount of memory required to store the decompressed data. Thus, the ability of the system to handle increased memory needs should be factored into decisions about when and how to use run-length encoding and decoding.
Run Length Encoding in JPEG Images
JPEG format is universally recognised for its efficient handling of colour images, making it the de facto standard for photographs on the Internet. A vital feature that supports JPEG's efficiency is its use of a lossy compression algorithm, where part of the original image data is discarded to save space. This algorithm uses several stages of transformation and compression, one of which involves the use of Run Length Encoding (RLE).The role of JPEG Run Length Encoding in image compression
The application of Run Length Encoding within the JPEG process is intimately tied to the way in which the JPEG algorithm preprocesses image data. After an image is disassembled into blocks, a Discrete Cosine Transform (DCT) is applied, resulting in a matrix of frequency coefficients. Most often, high-frequency components have smaller magnitudes and can even be zero, leading to consecutive zero coefficients—a perfect scenario for RLE.In the context of JPEG, Run Length Encoding is used in combination with Huffman coding in a process known as JPEG baseline Huffman RLE. The goal is to encode sequences of zeroes that occur between non-zero DCT coefficients or at the end of the block. So, each symbol to be encoded consists of two parts:
- The size of the non-zero amplitude following a run of zeroes; and
- The length of the run of zeroes preceding this amplitude.
An Overview of the Run Length Encoding Algorithm in JPEG
Consequently, the Run Length Encoding process in JPEG is slightly different compared to standard RLE. The differences lie in the detail of how runs are defined and elements counted. JPEG Run Length Encoding identifies a run as a sequence of consecutive zero coefficients and counts them. The end of run occurs when a non-zero coefficient or the end of the block is encountered. Then, instead of coding the actual zero and its length, a two-part symbol is created. The first part is the number of zero coefficients (RUNLENGTH), and the second part is the size (SIZE) of the non-zero amplitude that ends the run. Both then encoded using Huffman codes. A critical component to understand in this process is the treatment of zero sequences at the end of a block. In JPEG, there is a special end-of-block symbol (EOB). When JPEG encounters a run of more zeros than what remains in the block (or if the rest of the block is all zeros), it does not create a new (RUNLENGTH, SIZE) pair. Instead, it outputs the EOB symbol and proceeds to the next block. This clever mechanism creates additional data compression, as all trailing zeros are compressed into one symbol.Understanding the Run Length Encoding Compression mechanism
The practical application of RLE in JPEG involves multiple critical steps. Before the RLE, the transformed coefficients are reshaped into a one-dimensional list using a step known as zig-zag ordering, which aims to put low frequency coefficients before higher ones. This process favours long runs of zeros, further leveraging the beneficial aspects of RLE. As mentioned earlier, each run and its associated non-zero amplitude are tagged into the (RUNLENGTH, SIZE) pair. However, the actual non-zero coefficient is also needed to recreate the image data during the decompression process. Therefore, the Huffman-encoded symbol is followed by a binary representation of the non-zero amplitude. For this, SIZE bits are used, and the binary representation should be the smallest positive number that keeps the sign of the amplitude.Amplitude | Size | Positive Representation | Negative Representation |
1, -1 | 1 | 1 | 0 |
2, 3, -2, -3 | 2 | 10, 11 | 00, 01 |
4, 5, 6, 7, -4, -5, -6, -7 | 3 | 100, 101, 110, 111 | 000, 001, 010, 011 |
The Run Length Encoding Application in Digital Image Processing
Digital image processing taps into the power of Run Length Encoding for fast, space-saving operations, particularly in JPEG image compression. From a broader image processing perspective, each step of the Run Length Encoding and subsequent Huffman coding works in harmony with the earlier stages of the JPEG compression algorithm. The Discrete Cosine Transform is precisely engineered to produce data where small or zero coefficients are likely, thereby preparing the data for Run Length Encoding. Then, the zero coefficients are transformed into a significantly smaller amount of encoded data, thereby saving space. Meanwhile, Huffman coding utilises the statistical frequency of symbols to further compress the data.In addition to its space-saving functionality, JPEG's Run Length Encoding process also contributes to the execution speed of the JPEG compression algorithm. For image processing tasks, computational efficiency is just as paramount as memory efficiency, which is why the multiple stages of the JPEG compression process are all necessary to perform image saving, sending, and loading operations quickly and seamlessly – all while maintaining a high-quality approximation of the original image.
Run Length Encoding Examples and Applications
Run Length Encoding (RLE) is a straightforward and effective data compression technique used in many applications where data redundancy is common. This method can compress data without loss and is best aligned with data that includes many consecutive repetitions of the same element.Practical Run Length Encoding Examples
Run Length Encoding primarily serves digital picture manipulation. It serves widely in bitmap image file formats, such as BMP, TIFF, and PCX. However, it is not limited to graphics and can also be beneficial for text data. Let's provide some simple examples of how it works. For a grey-scale image or a text file where 'A' follow by thirteen 'B's and then 'C', using ASCII representation, would be initially represented as:65 66 66 66 66 66 66 66 66 66 66 66 66 67Using Run Length Encoding, this data can be compressed by replacing consecutive repeated characters with the character itself followed by the count of repetition:
65 1 66 13 67 1While this is a basic representation of RLE, the concept may extend to more complex scenarios. One example would be modifying the RLE method for binary inputs by differentiating between runs of 0s and runs of 1s. Consequently, a binary number like '00001111000', can be compressed using RLE into two distinct binary numbers representing lengths of 0s and 1s respectively. Thus, '00001111000' is encoded to '443', implying four 0s, followed by three 1s, followed by two 0s.
The use of the Run Length Encoding Algorithm in Practice
To better understand the practical usage of Run Length Encoding, let's take a detailed look at how it functions. Consider an image with pixel values:127 127 130 130 130 131Instead of storing each pixel value separately, Run Length Encoding locates and groups identical neighbouring values and stores this as a two-part data point: the pixel's value and the length of the run. The above pixel values would thus be stored as:
(127, 2), (130, 3), (131, 1)The use of RLE in practice involves an algorithm composed of two processes: encoding and decoding. The encoding function traverses the image matrix and records the colour value that starts a run and its length. During decoding, the compressed data is processed, and for each pixel value and length pair, a series of pixels are output on the image matrix. The Run Length Encoding technique can significantly reduce data size; however, not all types of data are suitable for this method. Data similarity and regularity are key factors in successful compression.
Exploring the Benefits of Run Length Encoding Compression
One of the major advantages of Run Length Encoding lies in its simplicity. RLE provides a very straightforward encoding and decoding scheme, which can be executed rapidly on a computer, making RLE a speedy method of compression. Another benefit is its lossless nature. Data compressed using Run Length Encoding can be entirely restored during the decoding process, which is beneficial for applications that require precise data reproduction, such as medical imaging. Yet another advantage is in data storage and transmission. RLE helps manage data packets more efficiently due to reduced data sizes. This makes it an attractive option for computer graphics and digital media transmission. Finally, RLE may increase data security. While not inherently a cryptographic method, its compression process can somewhat obscure the original data.Various Applications of Run Length Encoding
Run Length Encoding finds its place in a wide range of applications:- Computer Graphics: Bitmap images and TIFF files utilise RLE for storing pixel information. These types of files comprise many runs of pixels, making them ideal for RLE.
- Medical Imaging: Many medical images, such as CT scans and MRIs, have regions of similar pixel values. RLE proves extremely useful for compressing these images for storage or transmission without losing data.
- Thematic Maps: In geographical information systems, thematic maps use RLE. Here, large regions might have the same attribute, such as land use or soil type. RLE effectively compresses this type of data.
- Fax Machines: Considering the binary nature of faxed documents (text or lines on a white background), RLE is extremely efficient in compressing this data for transmission.
Run Length Encoding - Key takeaways
- Run Length Encoding (RLE) is a simple data compression method which replaces sequences of identical characters with a single character-frequency pair. In Python, this can be represented as '3A3B3C' for the input string 'AAABBBCCC'.
- Binary Run Length Encoding focuses on binary data, storing series of consecutive identical numbers (0s or 1s) as pairs containing the sequence length and number. For example, '000001111100000111' would be encoded as [(5, 0), (5, 1), (5, 0), (3, 1)].
- Decompressing Run-Length Encoded Lists involves converting compressed data back into its original form. For a run-length encoded list like [(5, 0), (3, 1), (6, 0)], this would result in the list [0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 0, 0, 0].
- Run Length Encoding plays a significant role in JPEG image compression. JPEG uses a process called 'JPEG baseline Huffman RLE' to encode sequences of zeroes that occur between non-zero Discrete Cosine Transform (DCT) coefficients or at the end of the block. This results in significant reduction in file size.
- The Run Length Encoding algorithm in JPEG is slightly different from standard RLE, identifying runs as sequences of consecutive zero coefficients and creating two-part symbols consisting of the number of zeros (run length) and the size of the non-zero amplitude that ends the run.
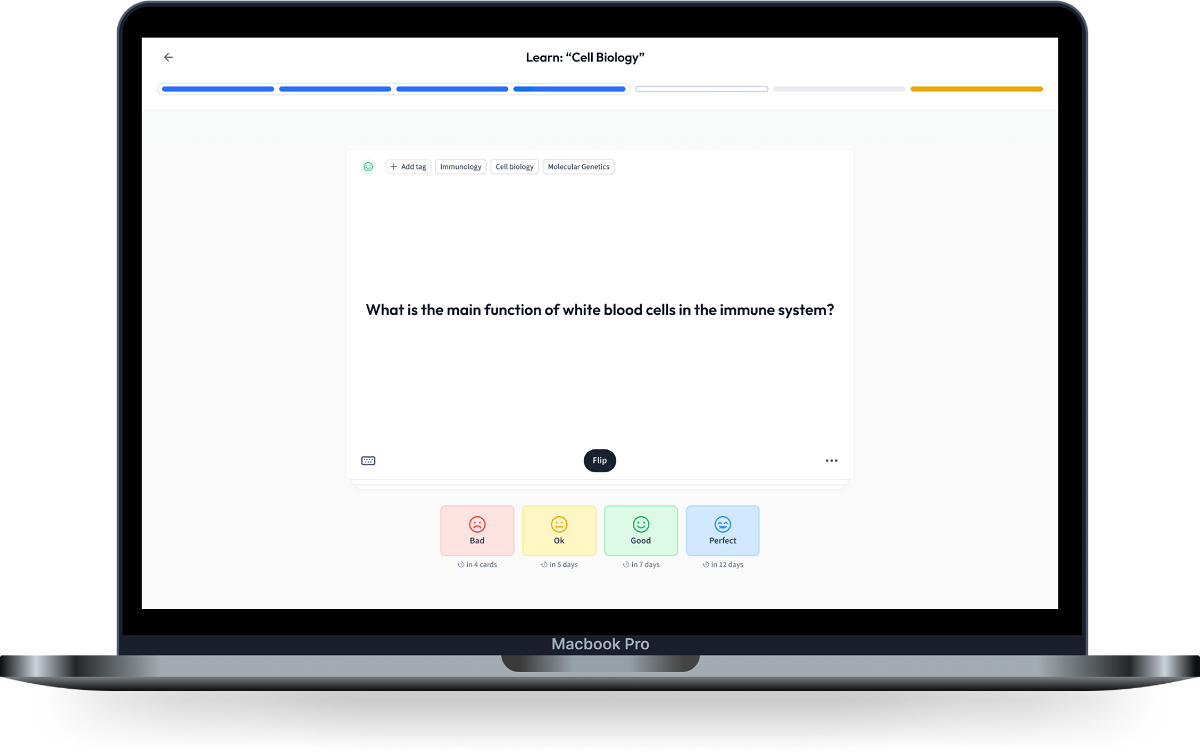
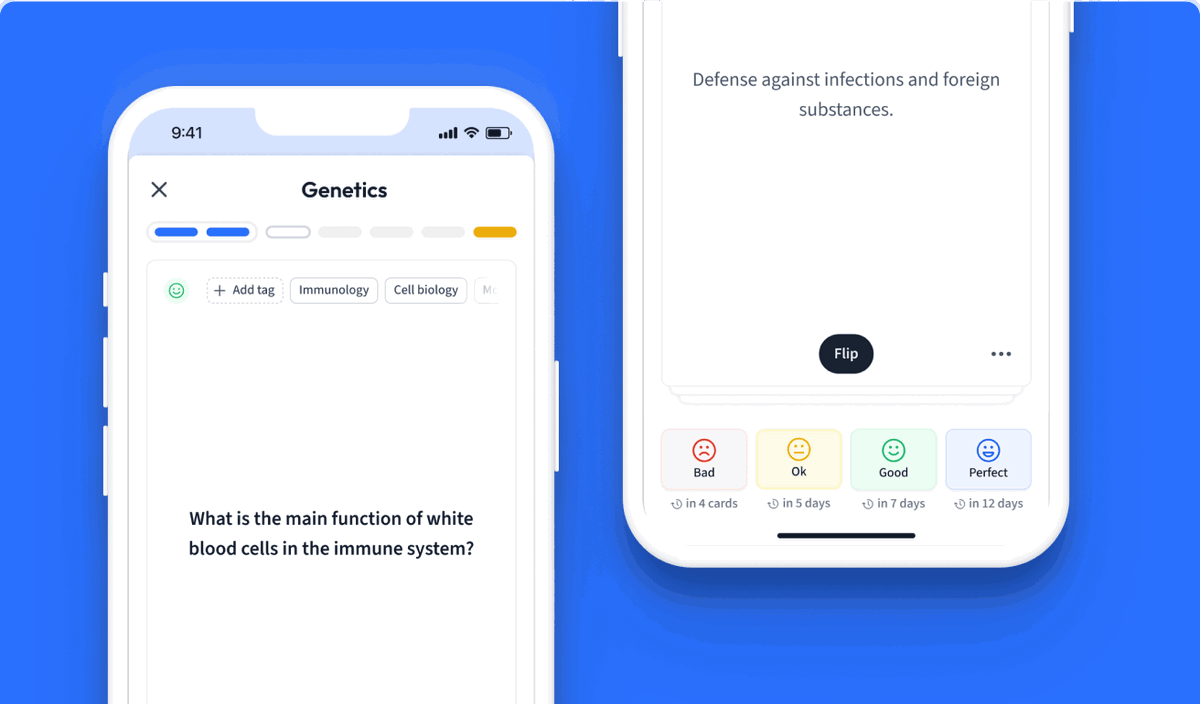
Learn with 12 Run Length Encoding flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Run Length Encoding
What is the practical application of Run Length Encoding in Computer Science?
Run Length Encoding is commonly used in data compression techniques. Its practical application includes file storing and transferring, reducing file size in graphics, text or data file compression and in fax machines where similar sequences are encoded into compact format.
How does Run Length Encoding contribute to data compression in Computer Science?
Run Length Encoding (RLE) contributes to data compression by reducing repetitive data sequences. It replaces these sequences with a code presenting the symbol and its frequency. This method significantly decreases the data size, making it efficient for storage and transmission.
What are the limitations of Run Length Encoding in data compression for Computer Science?
Run Length Encoding is ineffective in compressing types of data that don't have many runs of the same byte value. It performs poorly with high-entropy data sources and cannot represent simple repeating patterns efficiently. Additionally, RLE may increase the size of already compressed data.
What is the process of implementing Run Length Encoding in Computer Science?
Run Length Encoding (RLE) in Computer Science is implemented by replacing repetitive sequences of identical data elements, also known as "runs", with a single data value and a count. This count specifies the number of times the single data value appears consecutively, thereby compressing the data.
What are the common examples that use Run Length Encoding in the field of Computer Science?
Run Length Encoding is commonly used in computer graphics for image compression, particularly with bitmap images. It is also used in the process of telecommunication data compression, fax transmission and in file and data storage compression techniques.
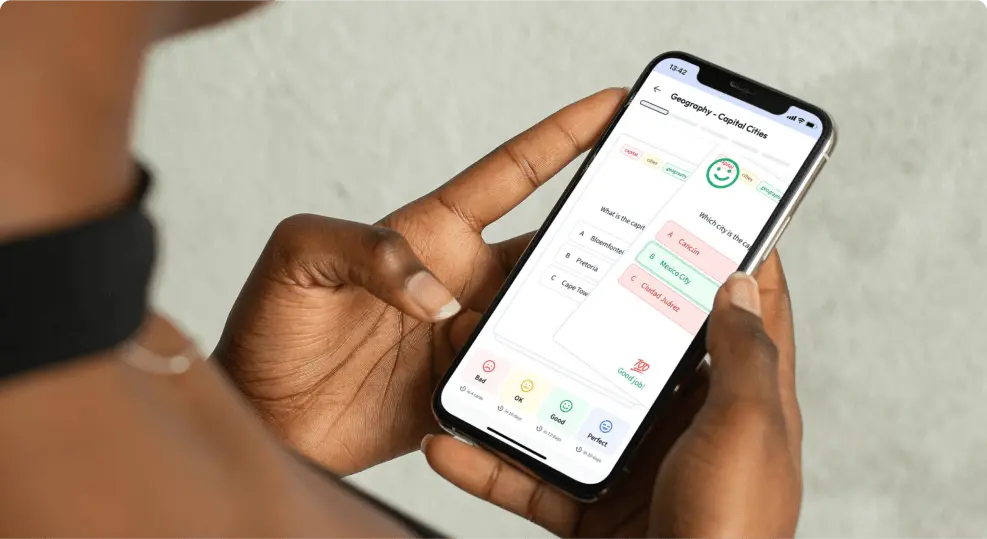
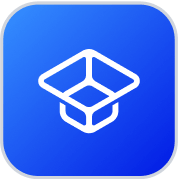
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more