Understanding Functional Programming
Functional Programming is a programming paradigm which forms the backbone of many modern technologies. In order to fully comprehend this system of writing code, it's essential to first grasp its core concepts and understand its evolution.
The Definition of Functional Programming
Functional Programming (FP) is a style of programming which models computations as the evaluation of expressions. In functional programming, functions are first-class entities, meaning they can be passed as arguments to other functions, returned as values from other functions, and even assigned to variables. The primary focus of FP is the application of functions, rather than the manipulation of data.
Functions, in the general mathematical sense, take in input and produce output. They don't have any internal state that affects the output.
For example, in mathematics, the function to calculate the square of a number is \(f(x) = x^2\). Regardless of how many times and when the function is called, provided the same input 'x', it will always produce the same output.
This is true in functional programming as well: regardless of when and how many times a function is called, if the same inputs are given, the same output will always be returned. This property is called referential transparency.
Core Concepts in Functional Programming
Several core concepts make Functional Programming what it is. Grasping these will make understanding this paradigm a breeze.
- Immutability: Once a data value is created, it cannot be changed. Any 'modification' would result in a new data value.
- Pure Functions: These functions always produce the same output given the same input, and they have no side-effects.
- First-Class and Higher Order Functions: Functions are treated as values that can be passed around. Higher-order functions are functions that can take other functions as input, or output.
Let's take an example to better understand these core concepts.
Consider a simple task - we have a list of numbers and we want to double every number in the list. In an imperative programming style, where data is mutable, we would create a loop to iterate over the list and change each number.
In Functional Programming, we would apply a function, say 'double', to every element in the list. The original list would remain unchanged, and a new list would be created as a result. This way, we avoid changing the state of our program. This is just a glimpse of how Functional Programming creates more reliable and less error-prone code.
The Evolution of Functional Programming
Functional programming has a long and rich history, tracing its roots back to the inception of computer science itself. Early stages of functional programming languages started in the 1950s with the arrival of the LISP language, followed by many others like Scheme and Haskell.
Year | Language |
---|---|
1958 | LISP |
1975 | Scheme |
1990 | Haskell |
From the 1990s to today, functional programming concepts have been increasingly adopted by a wide range of languages, including those that are not exclusively functional such as Python, JavaScript, C++, and even Java.
This surge is not without reason. As developers are handling larger and more complex systems, having a way to reason about how actions and data flow through software becomes increasingly important. By emphasizing immutability and avoiding changes in state within the system, functional programming helps maintain a high degree of control over the behavior of software, making programs easier to understand, debug, and test.
Functional Programming Languages
Functional Programming languages are designed around the concept of mathematical functions, manipulating data using pure functions without changing its state. Examples of such languages include Scala, Haskell, Clojure, and Erlang. Each of these languages applies the principles and practices of functional programming, albeit in different ways.
Analysis of Most Popular Functional Programming Languages
When it comes to popularity, several functional programming languages consistently stand out. Below is a detailed analysis of these languages:
- Haskell: Regarded as one of the most 'pure' functional programming languages, Haskell's design is based on mathematical logic, and it promotes a strong static type system and lazy evaluation. Its syntax is concise and expressive.
- Scala: Scala is a hybrid object-functional programming language which seamlessly integrates features of object-oriented and functional languages, enabling Java and other programmers to be more productive.
- Clojure: A modern functional programming language from the Lisp family, Clojure excels at handling concurrency and processing data. It is predominantly used for web development.
- Erlang: Known for being concurrent and fault-tolerant, Erlang was originally designed for telecom applications but has since found popularity in various domains, including web development and e-commerce.
Exploring Practical Examples with Functional Programming Languages
To better grasp the practicality of functional programming principles, let's walk through some examples using Scala and Haskell.
Scala, as a hybrid language, makes it easy to utilise both functional programming and object-oriented principles. In the example below, a simple function is defined to calculate the factorial of a given number:
Scala def factorial(x: BigInt): BigInt = if (x == 0) 1 else x * factorial(x - 1)
This function leverages recursion to calculate factorials, an often-used pattern in functional programming languages.
Haskell, being a 'pure' functional language, has some differences. The equivalent factorial function in Haskell would be written as:
Haskell factorial :: Integer -> Integer factorial 0 = 1 factorial n = n * factorial (n - 1)
This example underlines Haskell's heavy reliance on recursion and pattern matching.
Another key example showcasing the power of functional programming is the 'map' function. Map applies a given function to every item of a list or collection, returning a new list with the results.
In Scala, the 'map' function can be used to double each item in a list like this:
Scala val numbers = List(1, 2, 3, 4) val doubled = numbers.map(_ * 2)
In Haskell, the 'map' function can achieve the same result:
Haskell let numbers = [1, 2, 3, 4] let doubled = map (*2) numbers
These examples underpin the beautiful simplicity, conciseness, and power of functional programming, and its potential for unlocking efficient and reliable software development.
Functional Programming Examples
In order to understand functional programming in depth, examples provide a practical perspective that augments your theoretical learning. Depending on your experience level, it can be beneficial to start with simple examples and gradually progress to more complex ones. This will allow a more natural and smooth learning transition, enabling you to solidify your understanding of functional programming.
Simple Functional Programming Examples for Beginners
As a beginner, it's crucial to start with some fundamental examples to understand the basic principles of functional programming. Here are some simple examples in various functional programming languages. Let's start with a basic example of a function in Python, a language that supports functional programming style:
Python def add(a, b): return a + b
In this example, `add` is a pure function - it always returns the same result given the same arguments.
Another key feature of functional programming is the ability to pass functions as parameters. For instance, a function that applies another function to a list of numbers in Python could look like this:
Python def apply_to_list(func, numbers): return [func(num) for num in numbers]
This function takes a function and a list of numbers as arguments, and applies the function to each number – a common pattern in functional programming.
Clojure, being a Lisp dialect, has a unique syntax that might take some getting used to. Here is the equivalent function for adding two numbers in Clojure:
Clojure (defn add [a b] (+ a b))
Recall that functional programming supports higher-order functions.
Let's create an equivalent apply_to_list function in JavaScript:
Javascript function applyToNumbers(func, numbers) { return numbers.map(func); }
Taking a closer look, you may notice that the order of parameters in functions can make a significant difference in functional programming. It's conventional in functional programming to take the data that will be operated on as the last parameter. This convention facilitates a technique called 'currying', where a function is partially applied to its arguments. This results in another function which can be called later with the remaining arguments.
Advanced Functional Programming Examples for Experienced Individuals
As you become more accustomed with the principles of functional programming, it becomes essential to delve into more advanced scenarios. This is where you start realising the potential of functional programming in crafting elegant solutions.
Let's start with a classic example of recursion - calculating Fibonacci series in Haskell:
Haskell fibo :: Integer -> Integer fibo 0 = 0 fibo 1 = 1 fibo n = fibo (n-1) + fibo (n-2)
In this example, the function uses recursive calls to calculate the \(n^{th}\) Fibonacci number. Notice how there are no loops used here – functional programming often favours recursion over iteration. After understanding recursion, let's head over to monads.
Monads, a concept in Haskell, are used to handle side-effects. Here's an example showcasing a simple monadic operation:
Haskell main = do putStrLn "What's your name?" name <- getLine putStrLn ("Hello, " ++ name)
This is an interesting example showcasing usage of monads to carry values along with information about how they were computed, in addition to allowing a sequence of actions to depend on the results of prior actions.
Next, exploring the concept of immutable data structures is quintessential. Immute.js is a JavaScript library that creates persistent data structures.
Below is a simple usage:
Javascript var { Map } = require('immutable'); var map1 = Map({ a: 1, b: 2, c: 3 }); var map2 = map1.set('b', 50); map1.get('b'); // 2 map2.get('b'); // 50
As seen in the code above, after altering 'b' in map1 to create map2, map1 remains unchanged. This is an essential facet of immutable data structures, offering ease in tracking changes, and simplifying complex state management.
Advantages of Functional Programming for Computer Science
The functional programming paradigm is highly beneficial not just for individual coders, but for the field of computer science as a whole. It promotes cleaner, more efficient coding practices, and opens the gate to a multitude of advanced structures and operations. The inherent advantages of immutability, first-class functions, pure functions, and automatic memorisation make it an attractive paradigm for software creators.
Why You Should Consider Functional Programming
Despite coming to mainstream prominence more recently, functional programming has increasingly become the go-to for many developer communities. The reasons for choosing the functional programming way vary from better productivity to simplifying concurrency.
Concurrency is essentially the ability to deal with multiple things at once. In coding, concurrent execution means that multiple computations are progressing simultaneously. An increase in concurrent tasks escalates the challenges of managing state and controlling execution, and this is where functional programming steps in.
In functional programming, since data is immutable, there is no chance of data being changed by other parts of the program. This eliminates a major headache faced in concurrent programming, where shared mutable state can lead to indeterminate behaviour.
With no mutable state, it's much easier to run things concurrently or distribute them across multiple cores or servers.
Functional programming also inherently discourages monolithic structures that could impede the application's modularity. Modular code is easier to understand, test, reuse, and maintain.
Furthermore, a significant advantage of Functional Programming is its capability to handle 'Big Data'. With the explosion of data in today's digital world, processing large quantities of data has become essential. Functional languages like Apache Spark's Scala and Apache Flink's Clojure are specifically devised for such purposes.
These languages are capable of processing large amounts of data in an efficient and fault-tolerant manner. This facet alone can be reason enough to delve into functional programming.
Finally, adopting functional programming can be beneficial in learning more about the fundamentals of computing and programming design. Tracing its roots to mathematical logic, functional programming encourages you to think more abstractly and precisely about the programs you write.
Practical Benefits of Functional Programming
The practical advantages that functional programming offers are numerous and often depend on the specific language being used. However, some benefits are shared among all functional languages:
- Purity and Referential Transparency: Pure functions return the same result for the same input, irrespective of when and how many times they are called. This results in code that is easier to debug, test, and reason about.
- Modularity: With the absence of side effects, functional programming naturally promotes modular code which is easier to understand, reuse, and maintain.
- Higher-Order Functions: Being able to pass functions as arguments, return them as values, and assign them to variables does not just make code concise, but also allows powerful general-purpose functions to be created.
- Immutable Data: By default, data in a functional programming paradigm is immutable which eliminates many potential bugs related to data change and state management.
- List Processing: Functional programming languages often have powerful and efficient techniques for processing lists, exploiting recursion, and higher order functions.
To truly understand these benefits, let's consider the task of implementing a function for determining whether a string is a palindrome, i.e., it reads the same forwards and backwards. Let's take Clojure as our functional programming language.
In Clojure, this function could look like this:
Clojure (defn palindrome? [s] (= (seq s) (reverse (seq s))))
The function palindrome? checks if the sequence of characters in the string s equals its reverse. If it does, it returns true, otherwise, it returns false. This function is pure, it does not change the state of the program or the string that it is given. It directly showcases the simplicity, predictability, and the ability to work with lists and strings that functional programming offers.
The benefits of functional programming cannot be overstated. As software systems continue to grow, the immutable, stateless, and declarative model of functional programming will undoubtedly become the paradigm of choice for more and more developers.
Functional Programming vs OOP
When choosing a programming paradigm, both Functional Programming and Object-Oriented Programming offer unique advantages depending on the context and programming needs. The decision often boils down to the features or characteristics each paradigm can offer.
Understanding the Differences between Functional Programming and OOP
At a fundamental level, Object-Oriented Programming (OOP) and Functional Programming (FP) diverge in how they handle data and how they organise code.
Object-Oriented Programming (OOP) is a programming paradigm based on the concept of 'objects'. Objects contain data in the form of attributes and code in the form of methods. This style organises software design around data, or objects, and a set of interfaces to that data.
On the other hand, Functional Programming (FP) handles data and behaviour differently. It rejects the idea of a shared mutable state and object identity. Instead, its focus is on achieving computation through the evaluation of mathematical functions and avoiding changing-state and mutable data. Some notable differences between the two are:
- State: OOP allows for object states to be changed, known as mutable state. FP on the other hand, prefers immutability where object states cannot be changed after they have been created.
- Components: The main building block of OOP is the class, from which objects are instantiated. On the contrary, FP uses functions as its main component.
- Data and Behaviour: OOP bundles data and behaviours that operate on that data into objects. But in FP, data and behaviour are distinctly separated.
It's also worth noting the morphing landscapes of programming languages over time. Several modern languages like Python and JavaScript support both paradigms, allowing developers to choose the best approach based on the specific problem at hand.
Weighing the Pros and Cons: Functional Programming vs OOP
Assessing the merits and drawbacks of both paradigms can give us a better understanding of their application and help decide when to use one over the other. Let's explore the advantages of Functional Programming:
- Pure functions: One of the main selling points of FP is its use of pure functions. These promote safer code, where given the same input will always return the same output.
- Concurrency: With no mutable state and data, FP makes writing concurrent and multi-threaded code easier and inherently safer.
- Bug reduction: The characteristic of immutability in FP helps to prevent many bugs that are common in OOP.
- Efficient testing and debugging: Due to referential transparency, where functions have no side effects, testing and debugging become simpler.
However, the learning curve is significant because understanding abstract concepts like currying and partial application requires a shift in thinking for developers familiar with the OOP paradigm. Object-Oriented Programming also has a substantial list of advantages:
- Modelling: OOP provides a clear structure for the programs. OOP makes it easy to correlate the software with real-world objects, making the design process more intuitive.
- Reusability: Code can be created once and used again in other parts of the program or in other programs, reducing code redundancy.
- Information hiding: OOP provides the feature of data hiding, ensuring secure code.
On the flip side, OOP can also lead to superfluous hierarchies or relationships that don't always reflect well when the program scales. It also may also result in unintentional side-effects.
So, how do the two compare? It's clear that both programming paradigms offer distinctive benefits and drawbacks. What matters is choosing the right tool for the job, understanding the problem at hand, and having a good grasp of the programming paradigm that you intend to use.
As modern programming languages are increasingly multi-paradigm, having a sound understanding of both OOP and FP will undoubtedly mould you into a versatile and effective developer, well-equipped for any coding task.
Introduction to Functional Reactive Programming
Diving deeper into the landscape of functional programming, a noteworthy concept that deserves attention is Functional Reactive Programming (FRP). FRP is a programming paradigm that marries the robustness and predictability of functional programming with the adaptability of reactive programming.
Fundamental Aspects of Functional Reactive Programming
Functional Reactive Programming is principled around the concept of data flows and the propagation of change. In simple terms, it is all about handling streams of data over time. It employs several vital concepts derived from functional programming, such as immutability, pure functions, and using functions as first-class citizens.
A 'stream' is a sequence of ongoing events ordered in time. It can emit three different things: a value (of some type), an error, or a 'completed' signal. Consider a click on a webpage. If we think of every click as an event, the stream of clicks is a sequence of those events.
Where FRP starts to stand out is when these streams start being used like any other variable with functions applied to them. These functions are pure, they do not mutate any shared state or produce any side effects. This results in stream operations that are predictable, testable, and easier to comprehend. FRP provides operators to filter, select, transform, combine, and compose these streams.
The output of these operations is a new stream, ensuring the immutability principle of functional programming.
Here are some fundamental operators:
Map: applies a function to each event in the stream and outputs a new stream.
Filter: only lets events through that pass a certain condition.
Scan: applies a function to the first item emitted by a stream and the previous output of the scan function, similar to 'reduce' in JavaScript.
Suppose you have a stream of mouse events on a webpage. You could create a new stream of coordinates by mapping each mouse event to its coordinates. Then, you could further transform this stream by filtering for coordinates within a specific region of the webpage. Now, even if the mouse events stream fires a hundred events per second, you'll only react to the handful that pass your filter.
Functional Reactive Programming Enchancing Functional Programming
Functional Reactive Programming extends the basic concepts of functional programming to better deal with asynchronous, event-driven operations, where time is a crucial factor. This is achieved by integrating the 'reactivity' involved in dealing with real-time event streams.
Reactivity in this sense relates to a program's capability to react to changes over time and is critical in the dynamic, data-driven applications of today. From auto-saving a document, to server responses, or even simple animations, most applications respond to a multitude of asynchronous sources.
Combining reactivity with the principles of functional programming, FRP provides a powerful abstraction for dealing with these complex asynchronous operations. Let's focus on three significant enhancements:
Time-management: FRP encapsulates time management within its model, abstracting time as continuous and discrete events, allowing you to shift focus to the logic behind the functionality of your code.
Event-management: The event management capabilities of FRP are outstanding. With the option to listen, react, and propagate changes through streams of events, event management becomes organised and collaborative instead of disjointed.
Asynchronous behaviour: Managing asynchronous behaviour is often challenging in standard functional programming. But with FRP, it becomes straightforward. By treating asynchronous data flows as first-class entities, handling complex, interdependent, asynchronous operations become more understandable.
It's notable that while Functional Reactive Programming enhances Functional Programming, it also diversifies use cases for reactive programming by separating side-effects from your code and enforcing immutability. This dual-faceted augmentation stands testament to its powerful model and adoption in modern programming.
Functional Reactive Programming lays the groundwork for more organised, maintainable, and readable code, especially in an event-heavy environment. It not only enhances functional programming but also enriches your toolbox to tackle problems in an efficient and elegant manner.
Functional Programming - Key takeaways
- Functional Programming (FP) is programming style that models computations as the evaluation of expressions; functions are first-class entities and the focus is on the application of functions, not data manipulation.
- Functional programming originated in the 1950s with LISP followed by other languages such as Scheme and Haskell.
- Advantages of Functional Programming for Computer Science'
- Promotes cleaner, more efficient coding practices
- Features immutability, first-class functions, pure functions, automatic memorisation
- Allows advanced structures and operations.
- Object-Oriented Programming- a programming paradigm that organises software design around data or 'objects' and their interfaces to that data.
- Functional Reactive Programming (FRP): A programming paradigm combining functional programming's robustness and predictability with reactive programming's adaptability.
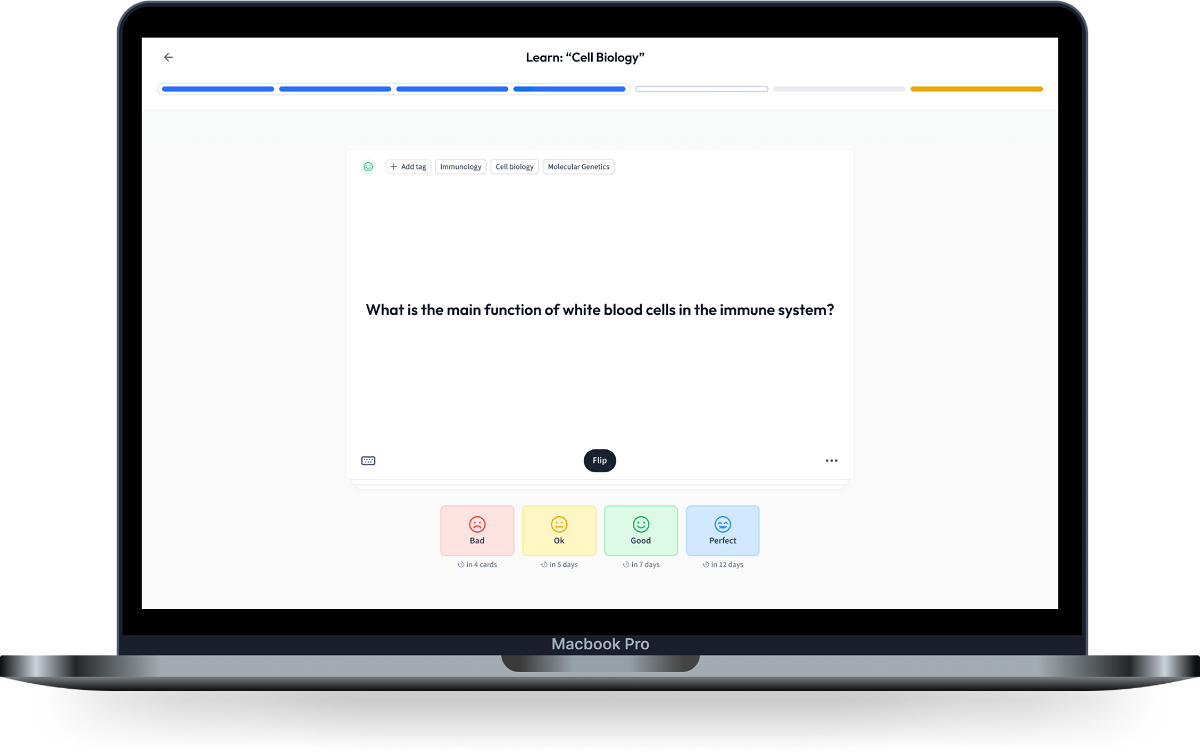
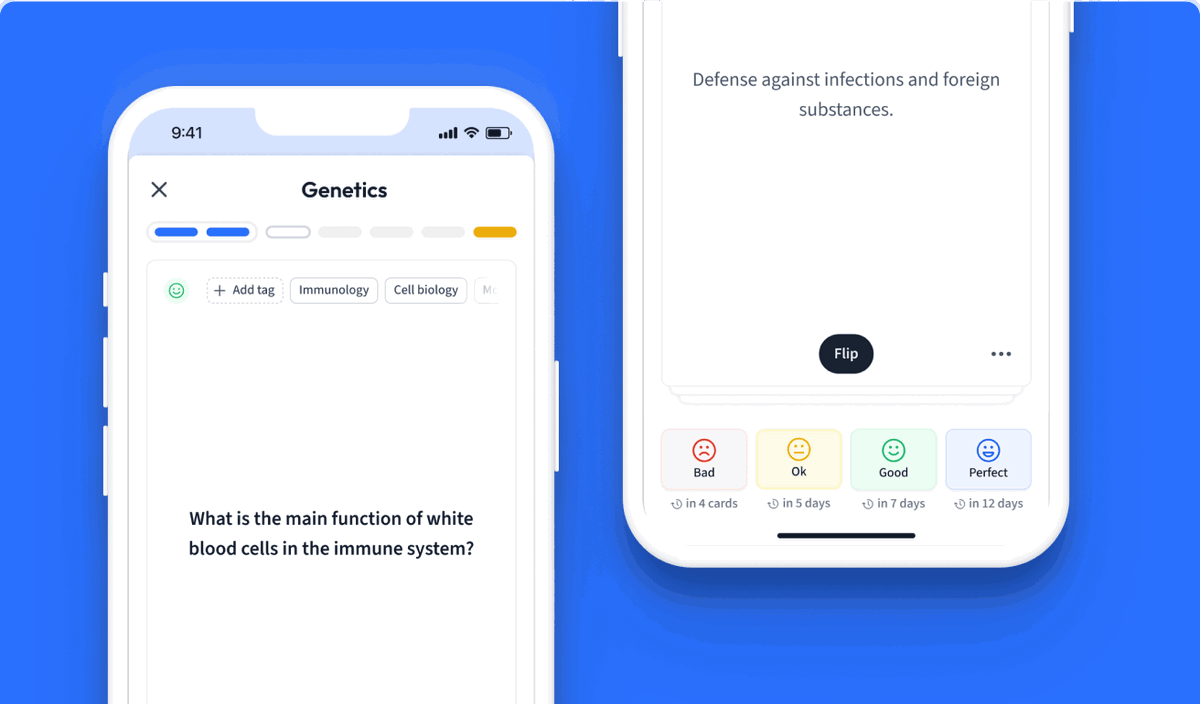
Learn with 308 Functional Programming flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Functional Programming
What is functional programming?
Functional programming is a style of programming where the primary method of computation is the evaluation of functions. These functions are based on mathematical functions and avoid changing-state and mutable data. It's a declarative type of programming rooted in mathematics. In functional programming, the outputs depend only on the input arguments, so it provides a predictable and less prone to bugs coding style.
What are functional programming languages?
Functional programming languages are a type of programming language that uses functions to create and manipulate data. They emphasise the evaluation of mathematical functions and avoid changing-state and mutable data. Examples of functional programming languages include Haskell, Lisp, Erlang, and Scala. They are used in scenarios where concurrency and ease of debugging are important.
What is object-oriented programming vs functional programming?
Object-oriented programming (OOP) is a programming paradigm where programs are organised around data or objects, which can contain data and code. Its key concepts include class, object, inheritance, encapsulation and polymorphism. However, functional programming (FP) is a style of programming where computations are treated as mathematical functions and avoids changing the state and mutable data. In FP, programs are composed using pure functions, avoiding shared state, mutable data, and side-effects.
How does functional programming work?
Functional programming works by treating computations as mathematical functions and avoids changing state or mutable data. It is a declarative programming paradigm, which means programming is done with expressions. In functional programming, functions are first-class citizens, which means they can be passed as arguments to other functions, returned as values from other functions, and assigned to variables. This all helps to make the code more predictable and easier to test and debug.
How to learn functional programming?
To learn functional programming, you should firstly familiarize yourself with the basics through online resources, textbooks, or courses. You can try platforms like Coursera, Udemy or freeCodeCamp. Pick a functional language such as Haskell, Erlang, or Scala and practise by working on projects. Get deeper into the subject by reading books like "Learn You a Haskell for Great Good!" and "Programming Erlang".
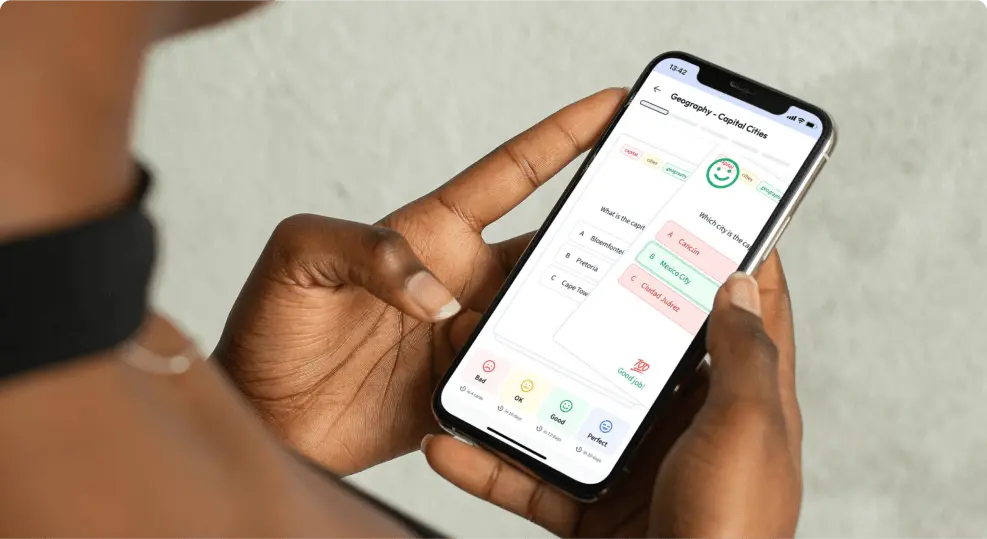
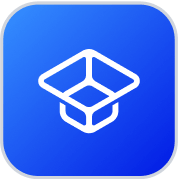
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more