Understanding Monads: The Basic
Monads constitute a fundamental concept in computer science, specifically within the paradigm of functional programming. Named after a concept in category theory, a branch of mathematics, Monads provide a framework that allows you to chain together distinct computations so that they act as one.A Monad is a design pattern that defines how functions, actions, inputs, and outputs can be used together to build robust, flexible pipelines and computational constructs.
What are Monads in Computer Science
In computer science, Monads serve as a construct which represents computations, instead of data in the domain model, which makes it distinctly different from other data types. For example, a common case for using Monads is to sequence actions which model side effects like state or I/O in a logical way. Think of it as a way to build up pipelines that process data in steps, in which each step is decorated with additional logic, for instance, error handling procedures. Here's an illustration of how you can use a Monad using the Maybe Monad in Haskell:Just "Hello, World" >>= (\str -> return (map toUpper str))This piece of code, through the use of the "bind" operator (>>=), transforms a string to uppercase, but only if the string is not Null (Nothing in Haskell), hence the Maybe Monad is frequently employed for error handling. Other common types of Monads you come across in functional programming include:
- The I/O Monad: for handling input/output actions
- The List Monad: for handling computations on lists
- The State Monad: for managing mutable state
The Role of Monads in Programming
Monads play a pivotal role in structuring programs, and managing side effects. Everything from I/O operations, exception handling, to state manipulations can be handled cleanly using Monads. In programming, a Monad takes an initial context (like a possible state of the world), and a function that takes a plain value and puts it in a context (such as a computation that can fail), and it somehow combine them to provide a new context (outcome after the computation and its contextual impact). The table below lists some common programming tasks and corresponding Monads that are typically used to handle them:Task | Monad |
Parse input | Parser monads |
Handle exceptions | Either, Error monads |
Maintain state | State monad |
Advanced flow control | Continuation monad |
The name 'monad' comes from the philosophical term, coined by Gottfried Leibniz, represents an indivisible unit. In computer science, monads can be seen as 'indivisible' too. Each monad represents a specific computation which can't be further decomposed.
Dive into Monad Operations: The Core Functions
Monads, as discussed previously, abound in functional programming. But what makes them truly unique and crucial in the world of computer science are their core operations. These operations define the behaviour of Monads and provide us with the real power behind this concept.Monad Operations: What They Are and How They Work
Within the realm of Monads, there are two primary operations - "bind" and "return". These operations, defined in the type class of the Monad, adhere to some specific laws of software composition. In Haskell, these rules are stated explicitly as part of the Monad type class definition.The bind operation, often signified as >>=, or simply 'bind', takes a Monad, applies a function that returns a Monad, and then provides a result also in the Monad context.
The return operation takes a value from a plain type and puts it into a monadic context.
The Importance of Monad Operations in Programming
The significance of these Monad operations manifests in a variety of ways in computer programming. Monads, through these operations, manage side-effects in functional programming, provide a basis for building complex sequencing computations, and enforce a form of information hiding which is of tremendous value in encapsulating the behaviour of computations. Here are some points illustrating their importance:- They help abstract the process of performing input/output operations, maintaining state and dealing with failures.
- They offer solutions for sequencing problems, allowing developers to chain together dependent computations.
- They allow a level of abstraction in which you don't need to be troubled about the underlying computation or the data being operated on.
- Through information hiding, they enhance the modularity and maintainability of the code.
Haskell Monads: A Special Case
Haskell, as a purely functional programming language, has a stringent way of dealing with side effects. This strict approach requires a comprehensive strategy to manage side-effect laced computations, a worldwide problem that Monads solve pretty elegantly. In Haskell, Monads are the cornerstone of maintaining state, error handling, parsing and I/O, among others.The Use of Monads in Haskell Programming
Haskell's philosophy towards computations with side effects is based on careful encapsulation. So, how do Monads fit in? Monads in Haskell serve as the chosen method to abstract and handle side-effects. They let you sequence operations in a linear and readable fashion, while the side-effects occurring from those operations are neatly wrapped and tucked away, keeping your code pure and unscathed. While the Monad's role in 'Linearizing' the control flow might seem trivial at first, it is quite profound. In a language without side effects like Haskell, you would typically find yourself passing around lots of intermediate state between functions if trying to emulate the classic procedural style of programming. But a Monad bypasses this, hiding the state passing behind the scenes, thus letting you organise your code as a sequence of operations, making it more readable and expressive. This is called Sequencing of computations and it's handled neatly in Haskell using the >>= (bind) operator. Here is an example of sequencing using the Maybe Monad:findPerson :: PersonId -> IO (Maybe Person) findPerson id = do res <- lookupPerson id case res of Nothing -> return Nothing Just person -> return (Just person)It starts with a person's id. The Monad action, lookupPerson, attempts to fetch the person based on the id. If successful, the person is returned within a Just Monad, otherwise, Nothing is returned signifying failure. In addition to sequencing, Haskell Monads play other pivotal roles:
- Isolated side-effects: Monads provide a mechanism to quarantine and deal with side-effects in a controlled environment, thus maintaining the functional nature of the language.
- Action chaining: Computation results can be passed through a chain of operations, where each operation subtly transforms the Monad or selects a course based on the outcome of the previous operation.
- Exception handling: Some monads like the Error Monad and the Maybe Monad can imbue a Haskell program with exception handling capabilities.
Examples of Haskell Monads in Computer Science
Haskell's Monad library comprises a diverse range of core Monads, each designed to manage specific types of computations.- Maybe Monad: This Monad encapsulates an optional value. A value of type Maybe a either contains a value of type a (represented as
Just a
), or it is empty (represented asNothing
). It is helpful in computations which can result in failure or not produce a value. - List Monad: The List Monad embodies non-deterministic computations. In this case, the bind operation generates a list of all possible outcomes.
- State Monad: This Monad encapsulates computations which manipulate state. It encapsulates a function that takes a state, manipulates it, and returns it.
- IO Monad: A key Monad in the Haskell library, the IO Monad isolates side-effect causing operations, keeping them separate from the pure part of the Haskell programs.
- Reader Monad: The Reader Monad represents a computation which can read values from a shared environment.
- Writer Monad: The Writer Monad encapsulates a computation that produces a value along with some side output.
let outcomes = [1,2] >>= \n -> ['a','b'] >>= \c -> return (n,c)In the Haskell code snippet above, the bind (>>=) operation is used to generate all possible pairs between the list of numbers [1,2] and the list of characters ['a','b'], creating a non-deterministic computation - along the lines of "for each number n in [1,2] for each character c in ['a','b'], generate a pair (n,c)" This results in a list of all possible pairs: [(1,'a'),(1,'b'),(2,'a'),(2,'b')] which is captured in the variable 'outcomes'. Understanding and harnessing the power of Monads in Haskell can exponentially increase the effectiveness of your functional programming skills and enable you to write more comprehensive and reliable code.
The Technique Behind Monads in Computer Science
When getting down to the nitty-gritty of monads in computer science, we find that they are primarily a design pattern, prevalent in functional programming, to tackle a specific type of problem – chaining operations in a context-dependent manner. They provide a standardised way of applying a function that yields a wrapped value to a wrapped value, thereby chaining these operations together. In essence, monads establish a common pattern for sequencing and combining computations and side effects.Understanding the Monads Technique: A Detailed Look
So, let’s unpack this monadic technique. At its core, it’s all about dealing with computations that are not just about crunching values but also involve some extra contextual information. Consider opening a file, reading its content, then closing it – all in a purely functional language. Each of these operations can fail – the file may not exist, its content might be inaccessible or it might just be locked. These operations are side-effecting and can break the consistency of the function world. Herein lies the problem that Monads solve. They serve as a uniform interface to chain and sequence these side-effecting operations in a way that makes them first-class citizens of the functional paradigm. How does this happen?Monadic Binding (>>=): This is the magic sauce behind the sequencing. The bind operation (commonly denoted as >>= in Haskell) takes a wrapped value and a function that can produce a new wrapped value based on the inner value, and it connects them together, producing a new wrapped value. This operation is context-aware; the context includes potential failure (Maybe), multiple choices (List) or state changes (State), etc.
listOfNumbers = [1,2,3] listOfSquares = listOfNumbers >>= \x -> return (x * x)Here, a simple list [1,2,3] is chained with a function that can square a number. The >>= operation takes each number in the list, squares it (applying the function) and adds back into the list, thereby producing a new list of squared numbers ([1,4,9]). Remember, it’s the context handling that makes the Monad – not only does the function get applied to the value but the surrounding context of the value also comes into play. For a Maybe Monad this context could be the possibility of failure that it encapsulates, for a List Monad, it's the idea of non-deterministic computation it represents. Another crucial concept in the monadic technique is monadic composition. Here, monadic values and functions are composed together to create a larger monadic action. Consider a series of database operations that need to be executed in sequence. Using Monads, these operations can be bound together to form a single monadic computation thus making it easier to manage and reason about.
The Impact of the Monads Technique on Programming
Now, you might wonder, why is understanding Monad’s technique crucial to you as a software developer? Simply put, the Monad pattern can significantly improve the way you handle side-effects in programs, manage complex control flows, and boost the modularity and readability of your code. In functional languages like Haskell that default to being pure (i.e., side-effect free and deterministic), the monadic technique can make profound impacts:Control Over Side Effects: Side effects are inherent to software programming – it’s what makes programs valuable. Being able to control and reason about these effects is what makes them manageable. Monads provide a very effective way to isolate and manage these side effects without sacrificing the purity of a function. In Haskell, the IO monad is one such example that wraps all side-effecting computations.
- Concise and Readable Code: The Monad abstraction helps avoid callback hell or deep nesting of function calls, making your code cleaner, and easier to reason about. Whether it’s async calls in JavaScript or chained computations in Haskell, Monads help linearising your code.
- Consistency: By defining a uniform way of dealing with side-effects and chaining operations, Monad’s technique enforces a level of consistency in your code. This makes it easier to learn and understand a code base.
- Increased Modularity: Monads promote function compositions which can lead to modular and reusable pieces of code.
Monads in Practice: Real World Examples
Moving beyond the theory, it is time to delve into the hands-on usage of monads. In the real-world programming sphere, the implementation of monads varies greatly depending upon the individual language and the particular problem that's being addressed. Whether it's JavaScript's Promises for asynchronous operations, Java's Optional to grapple with nulls, or Haskell's Maybe and Either Monads, practical applications are rife.Practical Examples of Monads in Computer Science
Let's explore a few examples where monads come to life practically across different programming scenarios and languages:JavaScript's Promises: A Promise in JavaScript represents a value that may not be available yet. The Promise object acts like a placeholder for the awaited value. This is a classic example of Monad, particularly in handling asynchronous operations. Think of the act of requesting information from a server and waiting for its response. The Promise Monad handles this gracefully, allowing you to chain operations or functions that are dependent on the async result via the .then construct.
const promiseExample = new Promise((resolve, reject) => { setTimeout(() => { resolve('Data received!'); }, 2000); }); promiseExample.then(data => console.log(data)); // logs 'Data received!' after 2 secondsNext, let’s look at Java's Optional – another handy monadic tool to handle nullable values and avoid the dreaded Null Pointer Exception:
Java's Optional Monad: A pervasive problem in many code bases is dealing with null variables, which can lead to the infamous Null Pointer Exception if not properly checked. Java's Optional Monad provides a robust solution to this issue. An Optional object can either hold a non-null value or Nothing (None). It lets you execute a series of operations on an object without manually checking for null at each step.
OptionalIn the example above, getSomeStringValue() can either return a String or null. The Optional Monad wraps this value allowing us to transform it (with map) into uppercase without manual null checks. If the value does exist, it will be transformed; if it's null, our orElse statement will ensure that "DEFAULT STRING" is returned.optionalValue = Optional.ofNullable(getSomeStringValue()); String finalValue = optionalValue .map(String::toUpperCase) .orElse("DEFAULT STRING");
Case Studies: How Monads Improve Programming Efficiency
Delving further into practical usage, let’s explore case studies to highlight performance efficiencies brought about by Monads in programming:Case Study 1: Error Propagation with Haskell's Either Monad |
Handling errors elegantly and effectively can make a code base robust and easier to maintain. Haskell's Either Monad is designed for this purpose. A computation that can fail is wrapped in an Either Monad, and it can either contain a valid value (encapsulated in a Right object) or an error (encapsulated in a Left object). This setup allows you to chain several operations together and the moment any operation fails, the entire chain fails, and the error can be handled at a single place. Consider a series of operations where error could potentially occur - opening a file, reading its content and then parsing the content. With Either Monad, this turns into a linear, easy-to-read chain of operations, clearly showcasing the order of operations, and presenting an error message if any step fails. |
Case Study 2: Sequence of Computations with Haskell's State Monad |
Haskell's State Monad provides an elegant way of performing a series of computations that alter a shared state. Suppose we want to generate a series of unique IDs. Using the State Monad, we can keep track of the next available ID in a series of computations and ensure the uniqueness of IDs. Again, the linearisation of computations, clear order of operations and encapsulated state manipulation is what makes this highly advantageous. Thus, using State Monad, we can keep the unique ID generation functionality completely pure, despite it being a side effect. |
Monads - Key takeaways
- Monads are data types with two primary operations - "bind" and "return". They adhere to specific laws of software composition in Haskell.
- The "bind" operation takes a Monad, applies a function that returns a Monad, and then provides a result also in Monad context.
- The "return" operation takes a value from a plain type and places it into a monadic context.
- Monads and their operations help manage side-effects in functional programming, enforce information hiding, and build complex sequencing computations.
- In Haskell, Monads serve as a method to manage state, error handling, parsing, and I/O. They allow sequencing and chaining of computations, isolating side-effects, and exception handling.
- Monads in computer science are design patterns in functional programming that chain operations in a context-dependent manner, managing computations that involve extra contextual information.
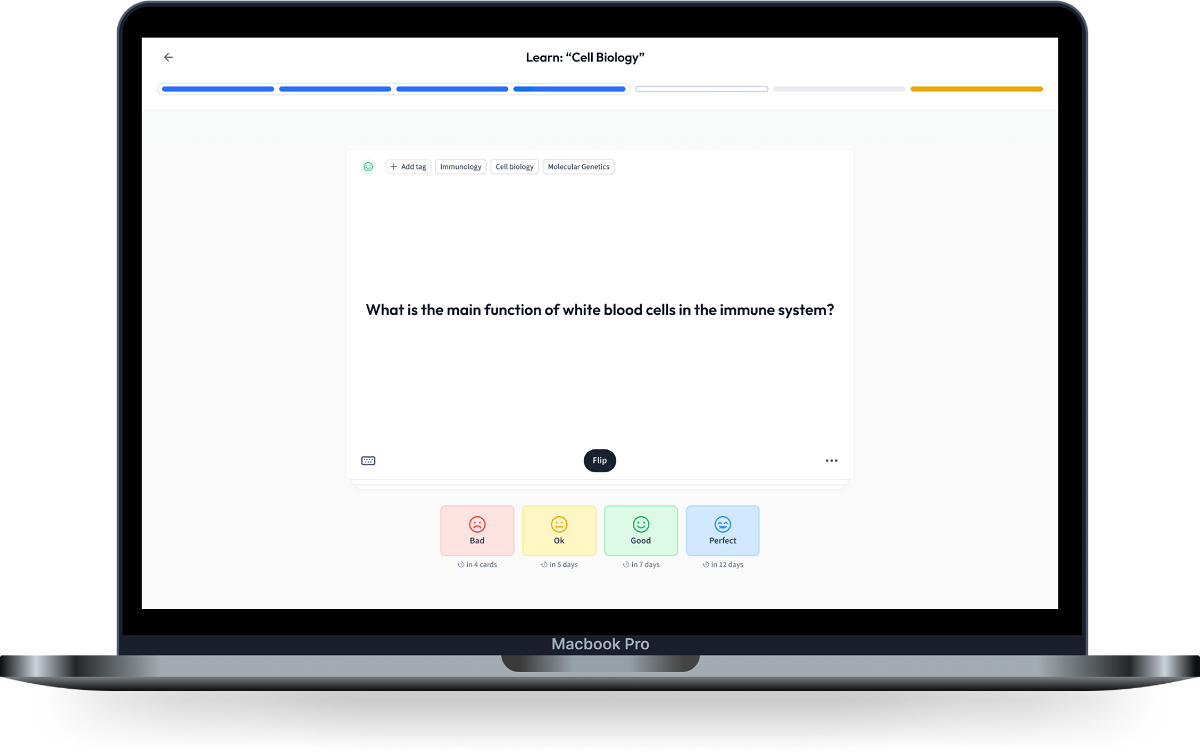
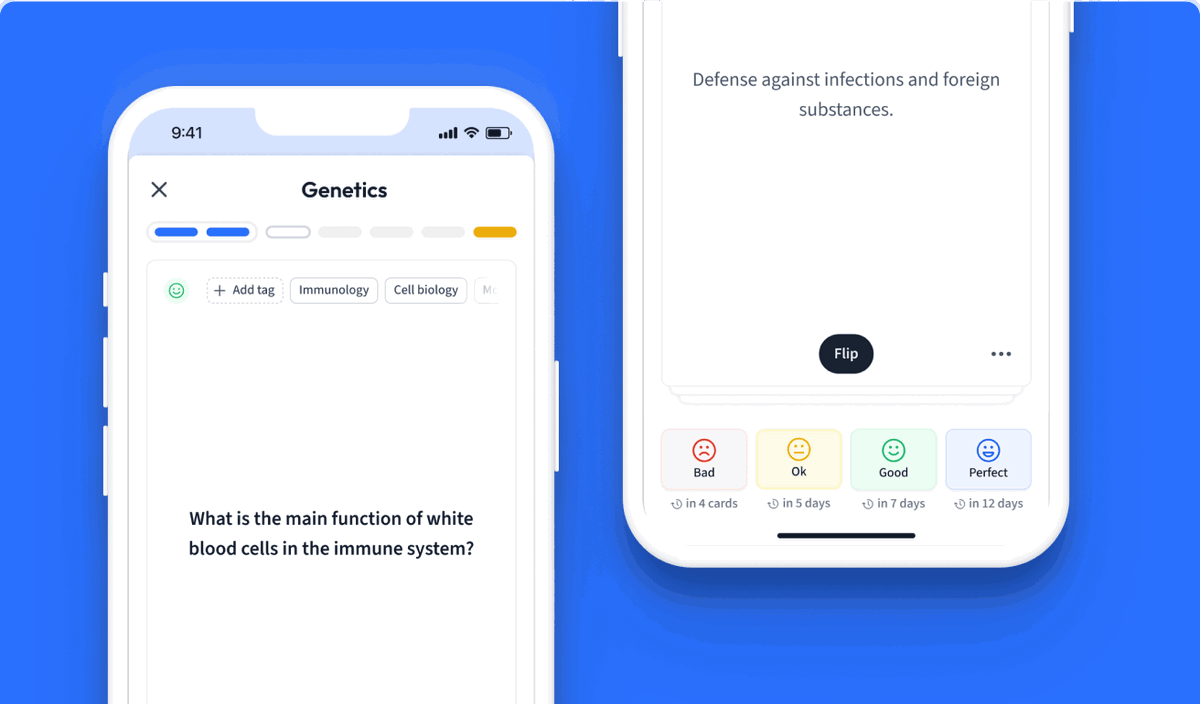
Learn with 45 Monads flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Monads
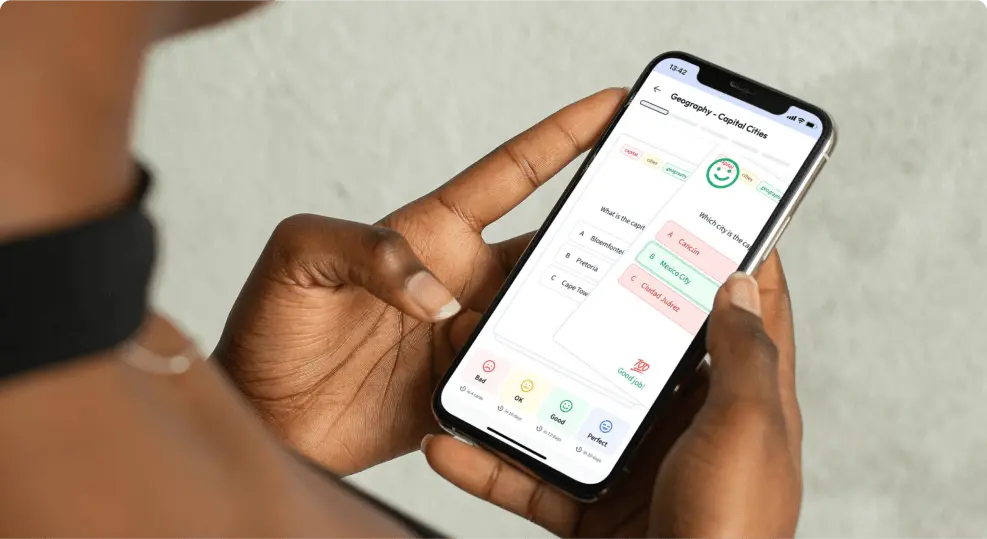
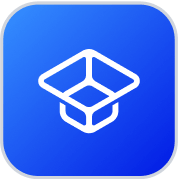
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more