Understanding Recursion Programming
Recursion programming is a significant concept in the world of computer science, transforming difficult problems into simpler tasks. Recursion can be applied to various programming languages such as Python, Java, C++, and more.Recursion in programming is a method where the solution to a problem depends on solutions to smaller instances of the same problem. It involves a function calling itself while a termination condition is defined to prevent infinite loops.
Define Recursion in Programming
Let's take a closer look at what recursion entails:- In the realm of computer science, a recursive function is a function that solves a problem by solving smaller versions of the same problem.
- The recursive function calls itself, passing a modified version of the original problem into the call.
- This process continues until a solution is found, or it hits a base case - a case for which the solution is defined explicitly, stopping the process of self-calling.
Meaning of Recursion in Programming
In computer science, recursion can imply a couple of related concepts.Apart from describing functions where a function calls itself, recursion may also refer to the process of a data structure using smaller instances of the very same type of data structure in its representation. This type of data structure design is referred to as recursive data structure.
Recursive data structures can dynamically grow to a theoretically infinite size in response to runtime requirements; they are a fundamental part of many efficient and powerful programming algorithms and techniques.
Practical Recursion Programming Examples
With all these theoretical insights, let's consider some tangible examples of recursion programming.One of the most classic examples of recursion is the Fibonacci sequence. In the Fibonacci sequence, the next number is found by adding up the two numbers before it. A recursive function to calculate the Fibonacci number could look like this in Python:
def fibonacci(n):
if n <= 1:
return n
else:
return (fibonacci(n-1) + fibonacci(n-2)
In this function, the base case is when n is less than or equal to 1. If this condition is met, the function will stop the recursion and return n. If the base case is not met, the function calls itself, hence the recursion, to perform the operation for (n-1) and (n-2) until the base case is met.
Levels in Recursion Programming
In the journey of understanding recursion in programming, the learning roadmap typically spans two broad levels: a beginner's guide focusing on basic recursion problems, and advanced challenges that deal with complex and multi-dimensional recursion problems. These levels help shape your knowledge and skills in recursive programming progressively.Beginner's Guide to Recursion Programming Problems
As a novice programmer, comprehending recursion can initially be complex. Fairly so, considering the shift in approach from iterative methods. It's imperative to start with simple problems, gradually advancing into complex clusters.
- Familiarise yourself with the concept of recursive functions: this involves understanding that recursive functions call themselves to solve a smaller version of the same problem.
- Understand base cases: a crucial concept in recursion. The base case acts as an exit ticket out of the recursion seeming loop. This case is typically something that can be solved without further recursion.
A simple recursive function to calculate the factorial of a number in Python can be as follows:
def factorial(n):
if n==1:
return 1
else:
return n * factorial(n-1)
In this function 'if n==1' is your base case that returns 1, and 'else' is your recursion that calls the function itself again, until the base case is met.
Advanced Recursive Programming Challenges
Advanced Recursive Programming Challenges pushes the boundaries of your recursive problem-solving ability. You are introduced to more complex problems that involve multiple recursive calls per iteration, deep recursion trees, or both.In contrast to simple recursive problems, advanced level problems often involve exploring multiple branches of recursion. A single problem may spiral into several smaller problems of the same type. This is commonly seen in backtracking problems or divide-and-conquer algorithms.
Consider a classic advanced problem - The Tower of Hanoi. In this problem, there are three pegs, and multiple disks of different sizes which can slide onto any peg. The puzzle starts with the disks in a stack on one peg, with the smallest at the top. The objective is to move the entire stack to another peg, obeying the rules that only one disk can be moved at a time, and no disk may be placed on top of a smaller disk.
This problem is solved using recursive approach as follows:
def TowerOfHanoi(n , source, destination, auxiliary):
if n==1:
print ("Move disk 1 from source",source,"to destination",destination)
return
TowerOfHanoi(n-1, source, auxiliary, destination)
print ("Move disk",n,"from source",source,"to destination",destination)
TowerOfHanoi(n-1, auxiliary, destination, source)
This recursive solution involves multiple recursive calls per function call, demonstrating the complexity involved at advanced recursion levels.
Exploring Recursion Strategies
In the field of computer science, there are several strategies and methodologies you can observe for crafting efficient and manageable recursive programs. The two impactful strategies that you often come across are Recursion and Dynamic Programming. Both methodologies have their specific advantages and preferred scenarios of use. Hence, understanding the comparison and contrast between these two is essential to take an informed decision.Dynamic Programming vs Recursion
Dynamic Programming and Recursion are two distinct approaches to solving problems. Both handle complex, multi-step challenges but approach them in different ways.Dynamic Programming is a problem-solving method in the field of computer science where the main problem is divided into simpler, manageable sub-problems. These sub-problems are not independent but overlapping. The solutions to these overlapping sub-problems are stored (memoised) for future reference to avoid repetition, thereby improving efficiency.
On the other hand, Recursion is a concept where a function calls itself to solve smaller instances of the same problem. However, it doesn't explicitly manage overlapping sub-problems and hence can lead to repetition and inefficiency in certain scenarios. Consider the classic example of finding the nth Fibonacci number. Using recursion, the time complexity is \(O(2^{n})\). This is due to the fact that the function computes the same subproblems, again and again, leading to exponential time complexity. Here is how a recursive code for Fibonacci would look like in Python:
def fibonacci(n):
if n <= 1:
return n
else:
return(fibonacci(n-1) + fibonacci(n-2))
However, when you solve the same Fibonacci problem with Dynamic Programming, it reduces the time complexity to \(O(n)\). This efficiency is achieved by storing the results of the overlapping sub-problems in an array and reusing them when required. Here's how you would implement Fibonacci using Dynamic Programming in Python:
def fibonacci(n):
fib = [0, 1] + [0]*(n-1)
for i in range(2, n+1):
fib[i] = fib[i-1] + fib[i-2]
return fib[n]
In the above function, the array 'fib' stores the Fibonacci numbers as they are calculated and this values are reused in future computations. Although it's clear that Dynamic Programming is more efficient in cases of overlapping sub-problems, it's slightly more involved and a bit complex to understand compared to Recursion.Effective Use of Recursion in Programming
While recursion can be a powerful approach, it must be used judiciously. Understanding how and where to effectively apply recursion can enhance your problem-solving skills and optimise your code. Here are some key pointers on the effective use of recursion in programming:- Base Case: Always define a base case for recursion. The base case is the simplest version of the problem, which can be solved directly. If the base case isn't defined, your function can recurse infinitely leading to a stack overflow.
- Recursive Case: This part should break down the problem into simpler versions and make a recursive call. The recursive case must modify the problem each time, so you come closer to reaching the base case.
- Efficiency: Recursion can be less efficient due to overhead function calls and repetition of same computations, as noted in recursive Fibonacci calculation. Use recursion intelligently, where multiple overlapping sub-problems aren't involved. If the problem involves overlapping sub-problems, consider using dynamic programming instead.
- Readability: A well-written recursive function can often be easier to understand and debug compared to its iterative counterpart. Recursive solutions are clean and elegant. If readability is a priority, recursion can be a good choice.
Recursion programming - Key takeaways
Recursion programming is a significant concept in computer science that solves complex problems by breaking them down into simpler tasks.
Recursion in programming is a method where the solution to a problem depends on solutions to smaller instances of the same problem. It involves a function calling itself with a termination condition defined to prevent infinite loops.
A recursive function is a function that solves a problem by solving smaller versions of the same problem.
Recursion in a recursive function involves the function calling itself, passing a modified version of the original problem into the call until it reaches a base case. The base case is a case for which a solution is explicitly defined.
Recursive data structures, which use smaller instances of the same type of data structure in their representation, are another aspect of recursion. Examples of recursive data structures include the binary tree.
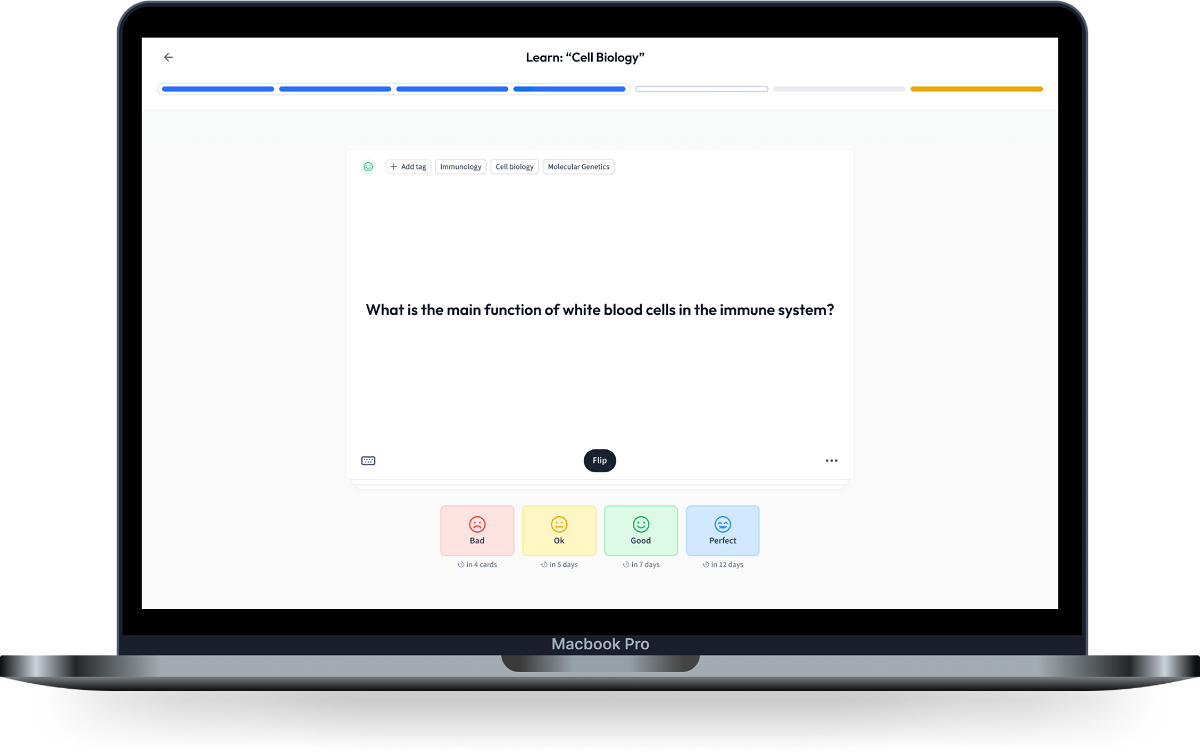
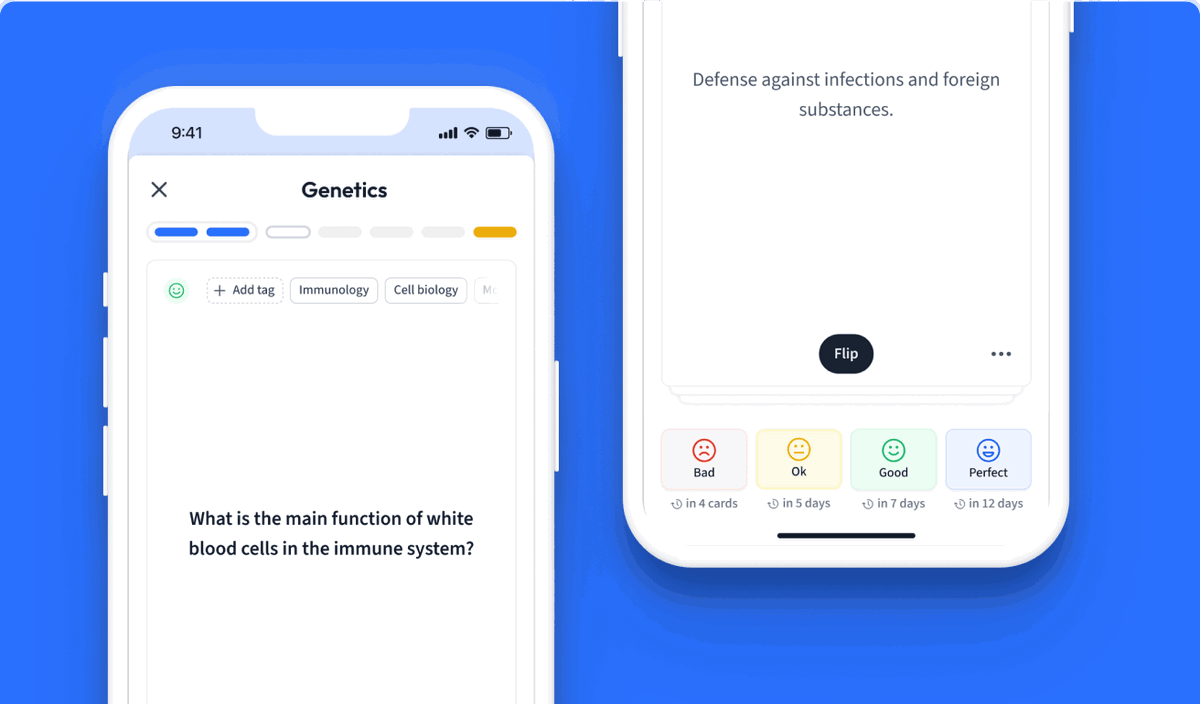
Learn with 15 Recursion Programming flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Recursion Programming
What is recursion in programming?
Is dynamic programming recursive?
How are recursion programs stopped?
What is a use of recursion in programming?
Why use recursion technique in a computer program?
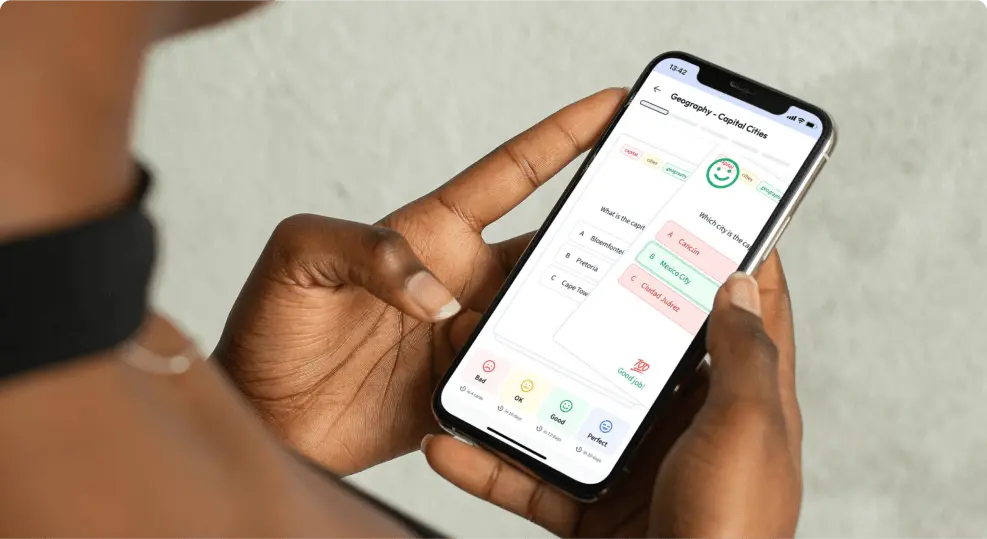
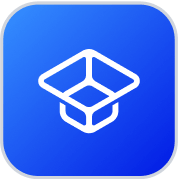
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more