Introduction to Haskell Programming
Haskell programming is a popular high-level, purely functional programming language with strong static typing and lazy evaluation. This provides you with a unique approach to solving problems and constructing software. Built around functions, in Haskell, everything you write and use is function-based.
In the context of programming, a function is a self-contained "subset" of code that performs a specific task. Functional programming is built around the concept of using these functions in a structured and standard way.
Basics of Haskell Functional Programming
In order to master Haskell programming, you need to grasp some fundamental concepts that differentiate this language from others. We'll explore some important basics for understanding how Haskell works.
- Purely Functional: In Haskell programming, a function's output is solely determined by its inputs, similar to mathematical functions. This means functions have no side-effects, dramatically reducing bugs linked to mutable state.
- Static Typing: Haskell uses static typing, which means that the type of each expression is known at compile time. This prevents many potential run-time errors.
- Lazy Evaluation: Haskell only evaluates expressions when it's necessary. This allows you to work with large data structures and infinite lists easily.
Term | Definition |
---|---|
Purely Functional | A programming paradigm where functions do not have side-effects |
Static Typing | A type system in which type checking is done at compile time |
Lazy Evaluation | An evaluation strategy which delays the evaluation of an expression until its value is actually needed |
An example of a simple function in Haskell could be a function that adds two numbers together:
addNumbers :: Int -> Int -> Int
addNumbers x y = x + y
This function called addNumbers takes two integers as input (x and y) and outputs their sum.
Understanding the Key Concepts in Haskell
To effectively navigate Haskell programming, you need to grasp crucial concepts like immutability, higher-order functions, and recursive functions. Let's break these down:
Immutability in Haskell means that once a variable is initialized, its value can't be changed. This aids in maintaining code consistency and reduces the risk of code breaking due to unexpected value changes.
- Immutability: Haskell employs immutability. Following initialization, you cannot alter the value of a variable. This contributes to reducing bugs and simplifying code comprehension.
- Higher-Order Functions: Functions in Haskell can take other functions as parameters, and functions can also return functions. This capacity to use functions as data points forms the basis for higher-order functions.
- Recursive Functions: Since you cannot employ loops like for or while in Haskell, you often use recursive functions to repeat computations.
In Haskell programming, your main source of looping is recursion—repeated application of a function to its own output. By taking advantage of Haskell's lazy evaluation, recursion can be used to handle seemingly infinite data structures efficiently.
An example of recursion in Haskell can be calculating the factorial of a number:
factorial :: Integer -> Integer
factorial 0 = 1
factorial n = n * factorial (n - 1)
Here the function, factorial, calls itself in its definition, making it a recursive function. Because of how the function is defined, the function will continue to call itself until it reaches the case where n = 0, at which point it returns 1.
Learning Haskell with Example Programs
Learning Haskell can sometimes feel like a steep ride due to its unique paradigms and syntax. However, grasping it can be made easier by breaking down the learning process into bite-sized example programs. These exercises not only familiarise you with the language but also solidify your understanding of the principles behind it.
Simple Haskell Example Program for Beginners
Starting with Haskell programming, you can begin with a straightforward example. A classic 'Hello, World!' program is a great first step. In Haskell, this can be created using the 'putStrLn' function which prints a line of text to the console.
main :: IO ()
main = putStrLn "Hello, World!"
The main function initiates the sequence of operations to be performed. Here, it calls the putStrLn function to output 'Hello, World!' to the console.
When run, this program prints 'Hello, World!' to the console, followed by a new line. But as unimpressive as this might seem, this 'Hello, World!' program reveals several essential aspects of Haskell programming: the syntax for defining functions, the putStrLn function from the Prelude library for outputting text to the console, and Haskell's use of whitespace for delimiting blocks of code.
Once you're comfortable with the basic structure and output in Haskell, you can start to experiment with incorporating variables and functions into your programs. For instance, a function that squares an input number can be defined in the following way:
square :: Int -> Int
square x = x * x
This function named 'square' accepts an integer as an input and returns the square of that input. In the context of Haskell's static typing, this function signature is significant because it states explicitly that an Integer is required as input and that an Integer will be returned.
The '::' symbol is used in Haskell for type annotations. It indicates the type of a value or function, making the code safer and easier to comprehend. It reads as "is of type". 'square :: Int -> Int' is read as "square is a function from Int to Int".
Once the square function is defined, you can call it like so:
main = print (square 5)
This would print 25 to your console, which is the result of the square function when given the value 5.
Advanced Haskell Example Program to Hone Your Skills
To further hone your Haskell programming skills, more complex examples containing higher-order functions and recursion are recommended. Let's dive into an example of such a program: calculating the Fibonacci sequence.
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones, usually starting with 0 and 1. The sequence can be defined recursively in Haskell as follows:
fibonacci :: Int -> Int
fibonacci 0 = 0
fibonacci 1 = 1
fibonacci n = fibonacci (n-1) + fibonacci (n-2)
This defines the fibonacci function, which takes an integer 'n' and calculates the nth fibonacci number. The 'fibonacci 0 = 0' and 'fibonacci 1 = 1' lines set the base cases in the recursive function. The 'fibonacci n = fibonacci (n-1) + fibonacci (n-2)' line gives the recursive rule.
Base case is a term used in recursion to stop the function from calling itself indefinitely. It is an essential component of recursive functions to prevent infinite recursion.
Although this recursive function provides the correct result, it becomes slow for large values of 'n'. This is because of the repeated calculation of the same fibonacci numbers. Here, a better approach would be to use "tail recursion" with an "accumulator".
fibonacciFast :: Int -> Int
fibonacciFast n = fibHelp 0 1 n
where
fibHelp a b 0 = a
fibHelp a b n = fibHelp b (a+b) (n-1)
This redesigned function, fibonacciFast, uses the helper function, fibHelp, that uses two accumulators a and b. Here, "a" accumulates the final result, and "b" accumulates the next number in the sequence. This helps to increase the efficiency of our function for larger inputs.
Tail recursion in functional programming refers to the phenomenon where the recursive call is the last operation in the function. By making use of tail recursion, we can improve our code's efficiency and avoid the risk of a stack overflow for large inputs.
Mastering Haskell programming entails understanding how to write and recognize both simple and complex example programs, from 'Hello, World!' all the way to recursive functions that generate the Fibonacci sequence. By working your way up from simple to more complex concepts, you will gain a thorough understanding of Haskell's unique characteristics.
Applying Dynamic Programming in Haskell
Dynamic programming is a powerful technique used in Haskell programming, which simplifies a complex problem by breaking it down into simpler subproblems and storing the results of these subproblems to avoid repeated computation. This optimisation is especially beneficial in functional programming languages like Haskell due to its pure and immutable nature.
Introduction to Haskell Dynamic Programming
Dynamic programming in Haskell can be approached differently compared to imperative languages like C++ or Java because of its unique structure and characteristics. This technique is primarily applied to optimization problems that exhibit overlapping subproblems and optimal substructure. The memoization capability of Haskell serves as the backbone of dynamic programming implementation by remembering the result of function calls, thus eliminating the need to recompute them when needed later.
Overlapping subproblems refers to a property of certain problems where optimal solutions can be constructed efficiently from optimal solutions of its subproblems. Optimal substructure describes a situation where an optimal solution to the entire problem can be constructed from the optimal solutions of its subproblems.
- Memoization: A fundamental aspect of dynamic programming in Haskell. It stores the results of expensive function calls and returns the cached result when the same inputs occur again.
- Lazy Evaluation: Haskell's inherent property of lazy evaluation aids in dynamic programming by not computing a value until it is needed.
From a mathematical perspective, dynamic programming seeks to solve complex problems using a system of equations or recursive relations. For instance, the problem of finding the nth Fibonacci number can be solved using the recursive relation \( F(n) = F(n-1) + F(n-2) \), with base values \( F(0) = 0 \) and \( F(1) = 1 \). The dynamic programming approach solves smaller instances of the problem first and stores their solutions to build up to the solution of the given problem.
In a pure functional language like Haskell, you can implement dynamic programming through higher-order functions, where a function takes one or more functions as arguments and returns a function as its result. This allows you to build abstractions over the structure of dynamic programming algorithms.
memoize :: (Int -> a) -> (Int -> a)
memoize f = (map f [0 ..] !!)
This simple solution employs the `map` function to apply function `f` to a list of integers from 0 to n. The results are stored in a list, and the `!!` operator is used to index into this list, creating a lookup table.
Higher-order functions are a core concept in Haskell and functional programming. It not only accepts other functions as input but also returns a function as output. It provides flexibility to create functional pipelines and abstractions over similar codes.
Practical Examples of Haskell Dynamic Programming
As you delve deeper into Haskell programming, it becomes essential to understand practical examples of how dynamic programming techniques can be applied. This can provide you with insights into real-life problem-solving, and how the ability to harness memoization and recursion can bring about considerable computational efficiency.
Consider the problem of computing the nth Fibonacci number again. Although our previous recursive function is correct, it's not efficient because it repeats calculations for each call (for example the (n-2)th Fibonacci number is calculated twice).
By using dynamic programming principles, we can enhance the efficiency of our function. Below is an improved Haskell program that calculates Fibonacci numbers using the memoization facility.
fibs = 0 : 1 : zipWith (+) fibs (tail fibs)
fibonacciDP :: Int -> Integer
fibonacciDP n = fibs !! n
Here, 'fibs' is an infinite list of Fibonacci numbers. The function 'zipWith (+)' adds the elements from 'fibs' and its tail, ensuring each Fibonacci number is only calculated once. The nth Fibonacci number can then be retrieved using list indexing, resulting in a highly efficient dynamic programming implementation.
To calculate the 10th Fibonacci number, simply use the `fibonacciDP` function like so:
main = print (fibonacciDP 10)
Running the program will print the value 55, which is the 10th number in the Fibonacci sequence.
Let's examine another dynamic programming problem: the coin change problem. It presents the issue of finding the minimum number of coins that add up to a certain amount, given an infinite supply of coins with different denominations.
Applying dynamic programming for such problems leads to significant enhancements in efficiency and time complexity. Haskell's list comprehension together with its memoization capability allow for an elegant and efficient solution.
change :: [Int] -> Int -> [Int]
change coins amount = dp where
dp = map (go) [0..]
go 0 = 0
go i = minimum (1 + dp !! (i - c) | c
In this program, 'change' takes a list of coin denominations and an amount and computes the minimum number of coins required to make that amount. The function uses a list 'dp' that holds the minimal combinations for all values up to 'amount'.
The list comprehension '(1 + dp !! (i - c) | c
Note: Dynamic programming in Haskell is a potent paradigm; however, it requires a firm understanding of functional programming principles like recursion, higher-order functions, and immutability. It's essential to practice different problems, ranging from easy to hard, to gain a strong foothold in dynamic programming.
Tackling Haskell Programming Challenges
As with any programming language, Haskell programming brings about certain challenges due to its distinct paradigms and features. These include understanding its functional nature, getting used to lazy evaluation, working with its advanced type system, and more. However, with knowledge and practice, you can overcome these hurdles.
Common Haskell Programming Challenges and How to Overcome Them
Learning Haskell can be an exhilarating journey. However, as a beginner or even an experienced developer transitioning from other paradigms, it's natural to encounter some roadblocks. In this section, we will discuss some prevalent challenges in Haskell programming and offer viable ways to overcome them.
- Understanding the Functional nature: Coming from an object-oriented or procedural programming background, grappling with Haskell's functional ethos can be tricky initially. To overcome this, focus on understanding how to solve problems in terms of functions and how to build your programs by composing functions.
- Mastering Lazy Evaluation: Haskell's lazy evaluation strategy, while beneficial, can be misleading during debugging. Haskell evaluates expressions only when necessary, so understanding the exact point of evaluation requires practice. Visualising code execution and studying Haskell's strictness properties can be beneficial.
- Working with Advanced Type System: Haskell's type system is powerful but its concepts like type classes, type inference, and algebraic data types can be daunting. Practice and patience help here. Strive to grasp how types interact with functions and how they enforce certain properties of your code.
- Handling IO and side effects: Haskell, being a pure language, handles I/O operations differently than you might be used to. Understanding how Haskell performs tasks with real-world effects, such as database or network operations, could be challenging. The key here is understanding the concept of monads.
Monads in Haskell encapsulate computation instead of data, allowing the sequencing of actions. Monads provide a way to handle side effects (like I/O) in a pure functional language like Haskell.
Tips and Techniques to Solve Haskell Programming Challenges
Facing Haskell programming challenges head-on becomes easier with the right set of tips and techniques. Ensure to understand the problem thoroughly and apply Haskell's functional solutions efficiently.
- Break the problem down: Learn to decompose your problem into smaller, more manageable parts. Solving these small parts will gradually lead you towards solving the entire problem more effectively.
- Develop an understanding of the syntax: Haskell's syntax differs significantly from most commonly used languages. To be proficient, spending time getting familiar with its syntax aspects such as function definition, pattern matching, list comprehension, and more is crucial.
- Master Recursion: In the absence of traditional looping constructs, recursion takes the primary role in Haskell. Develop a strong understanding of recursion and its subtleties.
A simple recursive function to calculate factorial can illustrate this:
factorial :: Integer -> Integer
factorial 0 = 1
factorial n = n * factorial (n - 1)
If we call factorial with `5` as its parameter, it will calculate `5 * factorial 4`, it continues this until it reaches `factorial 0` which returns `1` and the recursion unfolds to give the answer.
- Understand Purely Functional Programming: Since functions in Haskell don’t have side effects, every output is determined by its input which can greatly reduce bugs in code. Understanding this pureness can aid in writing better programs.
- Reasoning about Lazy Evaluation: Given Haskell’s core trait of lazy evaluation, understanding that not all expressions are evaluated at once, but only when needed is vital.
- Use Helpful Libraries and Tools: Libraries like Stack or Cabal can help you manage packages efficiently. Tools like GHCi (Glasgow Haskell Compiler) can aid in writing and debugging your code.
For debugging, Haskell offers a range of tools. The GHCi debugger lets you set breakpoints, inspect variables, and even navigate the evaluation history. Haskell programmers also swear by "printf debugging" -- strategically placed print statements can be very informative, given Haskell's lazy evaluation.
Haskell programming challenges are part of the learning journey, but don't let them hamper your progress. With comprehension and perseverance, combined with the right strategies, you can overcome these hurdles and master the art of Haskell programming.
Deepen Your Skills with Haskell Programming Exercises
When learning Haskell programming, consistent practice through exercises is an effective way to consolidate understanding and improve proficiency. Working on a range of challenges helps to strengthen your grasp of core concepts, syntax, and problem-solving skills. You can find a plethora of programming exercises, varying from beginner-level tasks to intermediate and advanced challenges, which can be instrumental in honing your Haskell programming skills.
Beginner Haskell Programming Exercises
If you're starting your journey with Haskell programming, various beginner-level exercises can ease you into the intricacies of this language. These exercises typically revolve around essential Haskell concepts, such as function declaration, list manipulation, and recursion.
- Functions and Operators: Try to create simple mathematical functions using Haskell. Start by creating a function that sums two numbers, then gradually move to more complex operations, such as finding the product or quotient of two numbers.
- List Manipulations: Haskell lists are essential and mastering them is a key part of learning Haskell programming. You could start with exercises that demand creating a list, manipulating list elements, or implementing basic list functions like `head`, `tail`, `last` and `init`.
- Recursion: Given the absence of traditional looping constructs, Haskell relies heavily on recursion. Starting with exercises that demand implementing simple recursive functions like factorial calculation or fibonnaci sequence calculator can be beneficial.
Getting familiar with basic standard library functions in Haskell is also a critical part of the learning process. For example, understanding how the 'map' function applies a particular operation on every element of a list or how the 'filter' function selects elements based on certain conditions.
Consider the following exercise that tests your understanding of function definition and list manipulations: Define a function `sumOfSquares` that takes a list of integers as an argument and returns the sum of the squares of all numbers in the list.
The solution would be:
sumOfSquares :: [Int] -> Int
sumOfSquares [] = 0
sumOfSquares (x:xs) = x*x + sumOfSquares xs
Another fundamental exercise could be creating a simple recursive function. For instance, a function called `reverseList` to reverse a list.
reverseList :: [a] -> [a]
reverseList [] = []
reverseList (x:xs) = reverseList xs ++ [x]
This function takes as input a list and uses the recursion mechanism to return the list in reverse order.
Intermediate to Advanced Haskell Programming Exercises
Once you are comfortable with the fundamental concepts of Haskell programming, it's time to challenge yourself with more complex exercises. These typically focus on advanced topics, such as higher-order functions, lazy evaluation, input/output operations, typeclasses, and monads.
- Higher-Order Functions: As a functional language, Haskell allows functions to take other functions as parameters and return functions. Try to create exercises that demand the creation of higher-order functions or the use of built-in ones like map, filter, or fold.
- Lazy Evaluation: Haskell is a lazy language which means it only evaluates expressions when it needs to. Try to create exercises that push you to think in terms of lazy evaluation, such as generating infinite lists or streams.
- Typeclasses: Haskell's strong static typing system includes a feature called typeclasses. Exercises that involve defining custom typeclasses and understanding how they work can be quite educational.
- Monads: Monads in Haskell are used to handle side-effects in a pure way. Try to create exercises that articulate the handling of I/O operations or create a simple state-machine using monads.
A practical intermediate Haskell programming exercise might include designing a simple command-line application. This can provide an understanding of how to manage side-effects in Haskell (through a concept known as Monads) and also give insights into how Haskell interfaces with the outside world (I/O operations).
One such exercise could be writing a simple text-based calculator. In this exercise, you would need to parse the user's input, perform the requested operations, and then output the result. This helps to strengthen your understanding of the IO Monad, parsing, and function composition.
main = do
putStrLn "Enter calculation: "
input String -> Float -> Float
calculate num1 "+" num2 = num1 + num2
calculate num1 "-" num2 = num1 - num2
calculate num1 "*" num2 = num1 * num2
calculate num1 "/" num2 = num1 / num2
Another interesting challenge is to implement the quicksort algorithm in Haskell. Because Haskell uses lists as its primary data structure, quicksort can be expressed concisely.
quicksort :: Ord a => [a] -> [a]
quicksort [] = []
quicksort (p:xs) =
quicksort lesser ++ [p] ++ quicksort greater
where
lesser = [y | y = p]
Building your confidence and stretching your skill set with such exercises can aid a lot in advancing your Haskell programming abilities.
Haskell programming - Key takeaways
Haskell programming is a high-level, purely functional programming language with strong static typing and lazy evaluation.
Function: In programming, it's a self-contained "subset" of code that performs a specific task. Functional programming uses these functions in a structured and standard way.
Haskell programming functions include Purely Functional where the output is determined by inputs only, Static Typing where the type of each expression is known at compile time, and Lazy Evaluation where expressions are only evaluated when necessary.
Other key Haskell programming concepts include Immutability, where you can't alter the value of a variable after initialization, Higher-Order Functions where functions can take other functions as parameters and return functions, and Recursive Functions, used instead of loops.
Key Haskell programming techniques include breaking down problems, developing an understanding of the syntax, mastering recursion, and understanding purely functional programming.
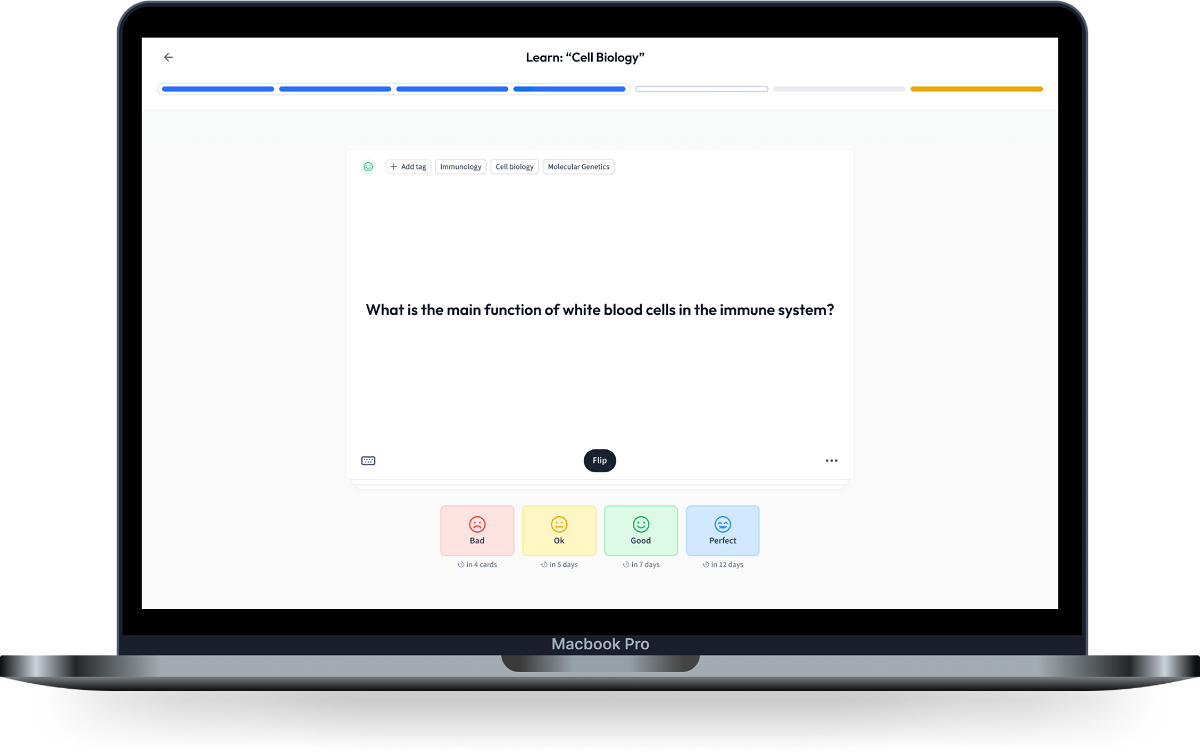
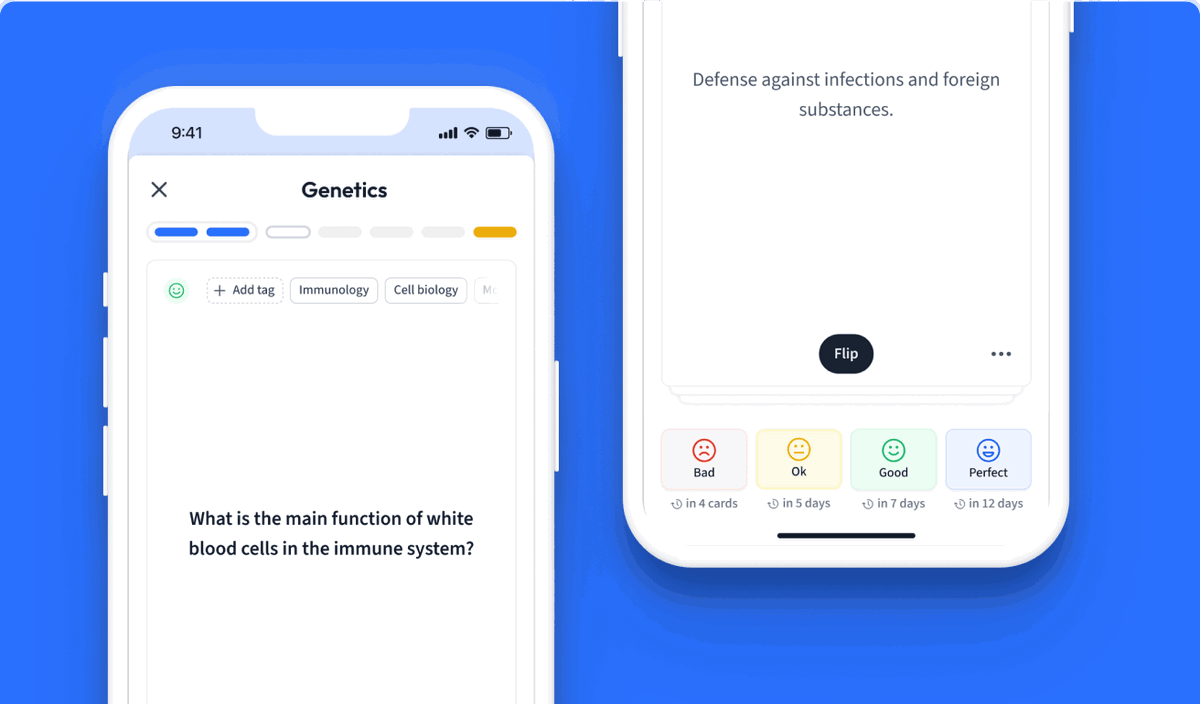
Learn with 15 Haskell Programming flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Haskell Programming
How to program in haskell?
What is haskell programming language?
What is haskell programming language used for?
How to execute haskell program?
What type of programming is haskell?
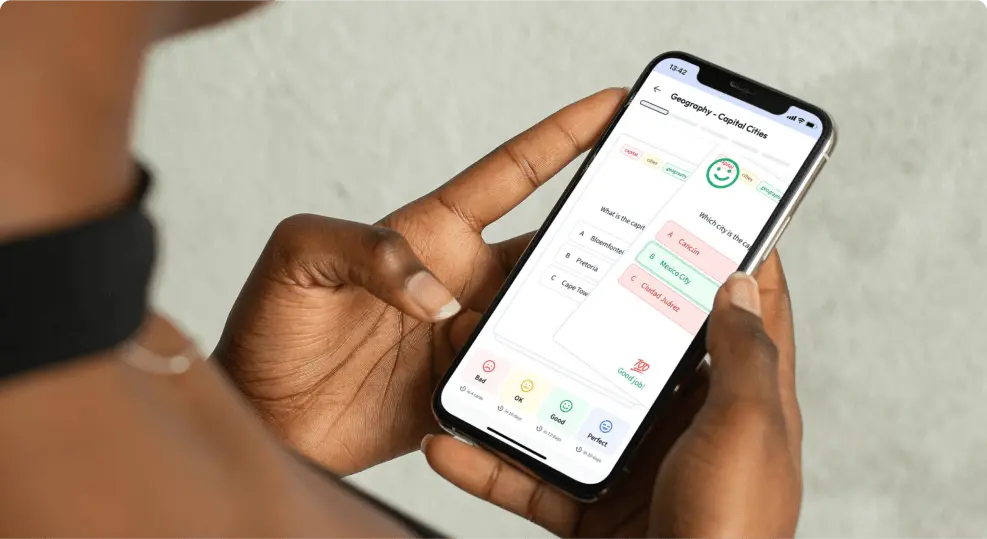
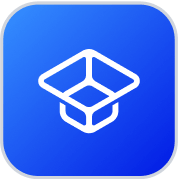
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more