Compiler: The Architect Behind Computer Programming
In any discussion about computer programming, a term you'll often come across is 'compiler'. What is this compiler you keep hearing about? In a nutshell, it's the indispensable tool that helps your computer understand and execute the programs you write.
Definition of Compiler in Computer Science
A compiler, in computer science, is a special kind of program that processes code written by programmers in high-level languages (like Python, Java or C++) and turns it into machine code that a computer's hardware can understand and execute.
Importance of Compiler in Computer Programming
The work of a compiler might seem simple, but its role in computer programming is substantial. Let's unpack some of these in bullet point form:- A compiler translates high-level languages to machine code, making it possible for computer hardware to execute the specified instructions.
- Without a compiler, programmers would have to write in assembly language or binary code — tasks which are complex, time-consuming, and highly prone to errors.
- By offering error detection, compilers help programmers fix their code. If a piece of code doesn't follow the rules of the programming language, the compiler will refuse to translate it and indicate where the error lies.
How a Compiler Works in Computer Programming
Now, you might be curious about how a compiler works. How does it turn your written code into something a machine can understand? Here's a simplified step-by-step explanation of what happens when you run your code through a compiler:Given a basic line of C++ code:
int x =10;
1. **Lexical Analysis:** The compiler breaks down your code into small chunks, called 'tokens'. For the example line of code
int x =10;The tokens would be 'int', 'x', '=', '10', and ';'. 2. **Syntax Analysis:** The compiler uses these tokens to create a parse tree, checking to see if the tokens align with the programming language's syntax rules. 3. **Semantic Analysis:** The compiler checks the code for semantic errors. An example could be assigning a string value to an integer type variable. 4. **Optimization:** The compiler tries to optimize your code to make it run more efficiently. 5. **Code Generation:** The compiler converts the optimized code into machine language, resulting in a binary file which can be executed by your computer.
Compiler vs Interpreter: Understanding the Differences
When you're diving into the world of computer programming, two fundamental concepts that you need to understand are compilers and interpreters. These are both types of translators used in the realm of software development, but they play different roles and have their respective strengths and weaknesses. Let's delve deeper into each of these and highlight their main differences.
Compiler versus Interpreter: A Comparative Study
An interpreter in computer science, is a type of translator that translates and executes code line by line. Unlike a compiler, it doesn't translate the whole program before executing it. This means that if you have a syntax error on line 100, you won't know about it until the interpreter has executed the first 99 lines of your program.
Aspect | Compiler | Interpreter |
Execution Time | A compiler takes time to analyse and translate the full program before execution, but the execution itself is faster. | An interpreter starts executing code immediately after translating the first line, but subsequent execution is slower. |
Error Detection | A compiler detects errors before running the program, which makes debugging easier. | An interpreter detects errors during the execution of the program, which can make debugging more difficult. |
Output | A compiler generates an intermediary machine code file which can be executed independently. | An interpreter does not generate an intermediary file; instead, it directly executes the instructions. |
Pros and Cons: Compiler vs Interpreter
Understanding the strengths and weaknesses of both a compiler and an interpreter can help you select the best tool for your specific programming tasks.Strengths and Weaknesses of a Compiler
Some of the benefits a compiler brings to the table include:- Better performance, as the translation and optimization process is executed in advance.
- Error-checking before execution allows for debugging and error fixing before runtime.
- Code protection, as the source code doesn't have to be shared; only the binary file is distributed.
- Compiling a program can be time-consuming, especially for large projects.
- A change in the source code necessitates a complete recompilation of the code.
Strengths and Weaknesses of an Interpreter
The advantages of using an interpreter include:- Immediate execution, as interpreters translate and execute line by line.
- Interpreted languages are ideal for scripting and automation tasks.
- Interpreted code runs slower than compiled code, as translation and execution happen simultaneously.
- While debugging, the interpretive code stops at the line containing the error, prompting a potentially incomplete execution of the program.
Code Compilation Process Explained
Delving into the core of computer science, it's crucial to explore one process that stands as a vital cornerstone of creating executables: the code compilation process.
An Overview of the Code Compilation Process
Code compilation is a multi-level procedure where a compiler transforms high-level programming language code, which is written by developers, into a form that can be understood and executed by a computer, typically machine code.
Stages Involved in the Code Compilation Process
The process of code compilation can generally be broken down into three critical stages: Syntax Analysis, Semantic Analysis, and Code Generation. Each of these stages solves a piece of the puzzle in converting the source code into machine code. Let's take a closer look at each of these stages.Syntax Analysis in Code Compilation
Syntax Analysis, also referred to as Parsing, is the step where the compiler looks at the structure of the programming language and checks the code against the syntactical rules of the used language. Consider this simple Python statement:x = 12The Python compiler checks that the above statement adheres to Python’s rules (syntax). If the same statement would be:
12 = xThis would result in a syntax error as it clearly violates the syntax rules of Python programming.
Semantic Analysis in Code Compilation
Semantic Analysis is where the compiler checks the source code to ensure that it not only makes syntactical sense but also has a valid meaning within the context of the language's semantics. Consider the following Python statement:x = "hello" + 12On the syntactic level, it's quite alright; there's a variable assignment with an operation conducted on two operands. But semantically, it doesn't make sense (you cannot add a string and an integer in Python). Thus, the Python compiler would throw a type error.
Code Generation in Code Compilation
Lastly, after your code has passed both syntax and semantic analysis and been optimized accordingly, it comes down to the final stage: Code Generation. This phase is where the optimizer and target code generation come into play. The compiler takes the intermediate source code and translates it into machine code (binary code: a series of 1's and 0's) that your computer hardware can execute directly. The exact nature and format of the machine code produced by a compiler depend on the target platform and the specific requirements of the project's infrastructural setup. Taking each of these stages into account, it becomes evident just how sophisticated and layered the process of code compilation really is. It's not merely a matter of translating code; it's about ensuring that the written code is both syntactically correct and semantically meaningful within the context of the programming language and then optimizing and generating efficiently executable machine code. It's an intricate sequence of steps, validation checks, and transformations, resulting in the seamless execution of code that end-users interact with every day.Insight into C++ Compiler
If you're getting started with computer programming using C++, you'll inevitably come across an important tool: the C++ compiler. This tool is integral to translating your written C++ code into a language your computer can understand and execute.
Understanding the Role and Functioning of a C++ Compiler
A C++ compiler is a critical component in the C++ programming process. It's the conversion tool that takes raw code written by programmers and transforms it into something understandable to a machine, typically machine code.
A C++ Compiler is a special program designed to turn C++ code written by programmers into machine code that a computer's hardware can understand and execute.
- A C++ compiler interprets and translates written C++ source code into executable files.
- It spots and reports coding errors making debugging and error checking easier.
- A compiler optimises and streamlines code, creating a more efficient end-product.
1. **Lexical Analysis:** The compiler scans the code and breaks it down into chunks, called tokens. For example, given the line of code
cout << "Hello, World!";The tokens are 'cout', '<<', '"Hello, World!"', and ';'. 2. **Syntax Analysis:** The compiler uses the tokens to create a parse tree, verifying they comply with the programming language's syntax rules. 3. **Semantic Analysis:** The compiler checks the code for semantic consistency. An example could be trying to perform an impossible operation, like adding a string to an integer. 4. **Intermediate Code Generation:** The source code is translated into an intermediate language, providing a layer of abstraction from machine languages. 5. **Code Optimization and Generation:** Compiler optimises intermediate code for performance improvement, then translates the optimized code into machine language, resulting in a binary file that can be executed by a computer.
Getting Started with a C++ Compiler
Starting with a C++ compiler is a straightforward process. In practical terms, this is done via the command line interface on your local computer where you type specific command strings to compile your C++ program.
To start, you must have a C++ compiler installed on your system. The GNU Compiler Collection (GCC) is a popular, open-source compiler that supports multiple languages, including C++. Once you've installed a compatible compiler, follow these steps:- Create a simple C++ file: Create a new .cpp file on your computer. The .cpp extension indicates that the file contains C++ language code. It can be something as simple as a Hello World program.
- Open a command prompt: Navigate to the directory where your C++ file is located.
- Type the compile command: If, for instance, you're using GCC, the command to compile a C++ file named helloworld.cpp would look like this:
g++ helloworld.cpp -o helloworld
- Run your program: If the compilation is successful, a new executable file will be created in your directory. You can now run your program using a simple command:
./helloworldThe terminal should show your program's output - in this case, 'Hello, world!' Remember, C++ can be complex because it's a statically-typed, free-form, multi-paradigm, compiled, general-purpose programming language. As a newcomer, don't let this intimidate you. The best way to get to grips with C++ or any language, is with practice and consistent work. Before you know it, compiling your C++ projects will become second nature.
Delving into Python and Java Compilers
In the realm of computer programming, compilers play an essential role by translating high-level programming languages into machine language that a computer can execute. The Python compiler and the Java compiler serve as perfect examples of how compilers work in conjunction with different programming languages, each bringing unique features to the table.
Decoding the Python Compiler
Known for its syntax simplicity, Python has become one of the most loved programming languages worldwide. But how does Python code become a language your computer understands? Enter the Python compiler.
A Python compiler is a unique tool that translates Python code, which humans can understand, into byte code (intermediate code) that the Python interpreter, another element of the Python execution process, can interpret.
An Introduction to Java Compiler: Get Acquainted
As a versatile and powerful language, Java is quite popular in the programming realm. Its universality stems from its 'write once, run anywhere' principle, which is made possible due to the Java compiler.
A Java compiler is a program that takes human-readable Java source code and converts it into platform-independent Java bytecode, which can then be executed by a Java Virtual Machine (JVM).
Features of the Java Compiler
The Java Compiler is packed with features that streamline the overall processing of the Java code: 1. **Cross-Platform Capability:** The Java Compiler produces bytecode, which is non-machine-specific code that can run on any device with a JVM. This leads to its primary advantage of being platform-independent. 2. **Generating Intermediate Code:** Unlike Python, which compiles and runs code almost simultaneously, the Java compiler first converts high-level code into intermediate bytecode before runtime. 3. **Robustness:** The Java Compiler rigidly applies static typing, enforcing type checking at compile time. This reduces the number of errors at runtime, increasing the robustness of the code. 4. **Javac Tool:** The javac tool, provided with the Java Development Kit (JDK), reads source files written in the Java programming language, and compiles them into bytecode which can be executed by the JVM.Working with the Python Compiler in Programming
Enhancing your skills in Python requires a good understanding of how the Python compiler works. As a programmer, your interaction with the Python compiler is typically implicit. When you execute a Python script, the conversion from the high-level Python code to byte code happens behind the scenes before execution by the Python Virtual Machine. However, if you'd like to explicitly compile Python scripts to byte code, Python provides tools to facilitate this. The compileall module in Python's standard library can compile all .py files in a directory.python -m compileall .This command will compile all .py files in the current directory to .pyc files.
Compiler Example: Analysing the Java Compiler
Now, let's focus on the Java compiler. The Java compiler is invoked using the javac command. If you've written a Java file named HelloWorld.java, you can compile it using the following command:javac HelloWorld.javaAfter running this command in your terminal, the Java compiler will take your readable Java source code and compile it into something called bytecode, which is saved in a file named HelloWorld.class.
- If any errors are found during the compilation process, they will be listed in the terminal. You will need to correct these errors before your code can be compiled successfully.
- If no errors are detected, no output is returned. However, a HelloWorld.class file will be generated in the same directory.
- The .class file contains bytecode which can be interpreted by the Java Virtual Machine, regardless of the platform it's running on.
Compiler - Key takeaways
- A compiler translates a high-level programming language code into a form that can be understood and executed by a computer, typically machine code.
- An interpreter translates and executes code line by line, unlike a compiler which analyzes and translates the whole program before executing it.
- A compiler is typically faster in execution as it translates the full program before execution. It also detects errors before running the program and generates an intermediary machine code file abstracting the encoded file from the end user. However, it can be time-consuming especialy with larger projects.
- An interpreter executes code immediately after translating the first line but subsequent execution is slower. It also detects errors during execution which can make debugging a bit difficult. It does not generate an intermediary file but directly executes instructions.
- Code Compilation Process involves Syntax Analysis for checking structural rules, Semantic Analysis to ensure the code has a valid meaning within the context of the language's semantics, and Code Generation where the code is translated into machine code.
- A C++ Compiler is a special program that translates C++ code written by programmers into machine code that a computer's hardware can understand and execute.
- Python Compiler translates Python code into byte code (intermediate code) that the Python interpreter can interpret.
- A Java Compiler transforms Java language code into byte code which a Java Virtual Machine can understand.
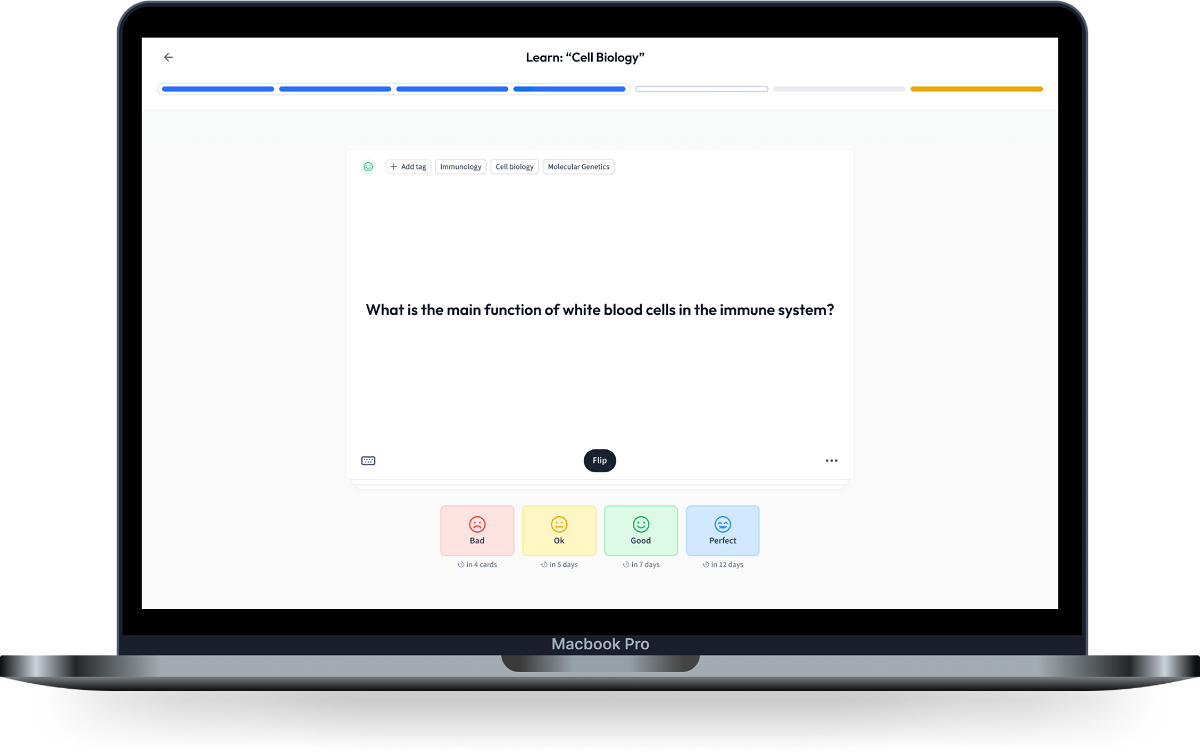
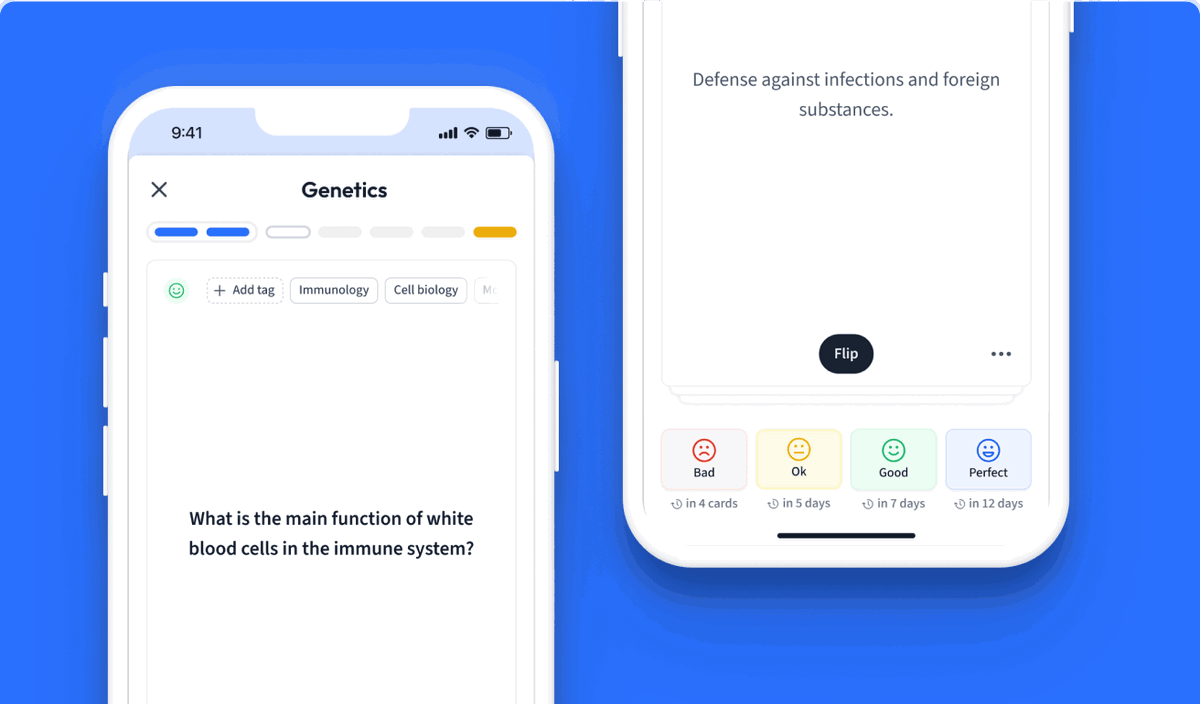
Learn with 15 Compiler flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Compiler
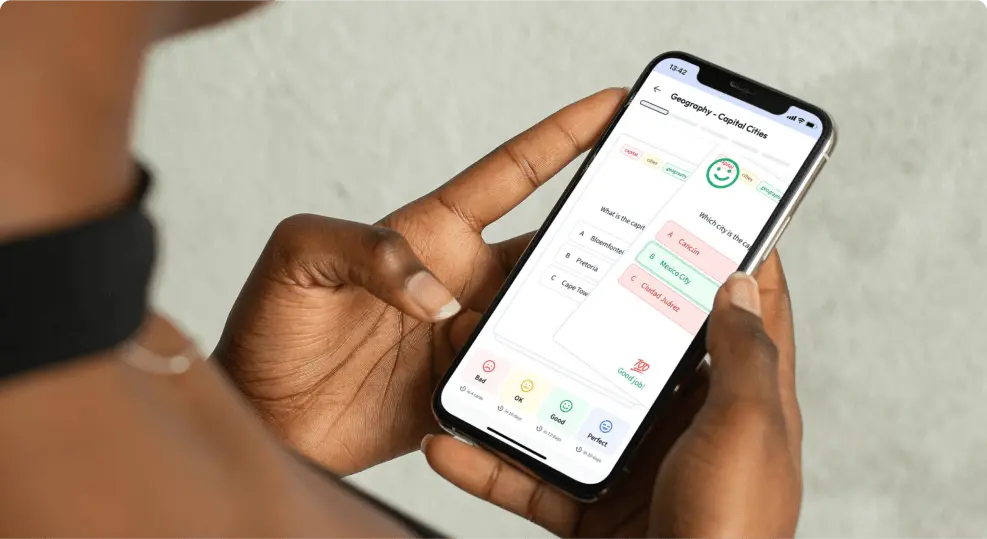
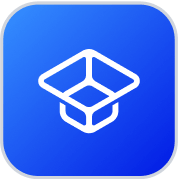
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more