Introduction to Write Functions in C
Functions play a critical role in the C programming language, allowing for modular and well-organized code. By breaking down complex tasks into smaller, more manageable pieces, functions enhance the readability and reusability of your code. This article will guide you through understanding the basics of functions, writing your own functions in C, and creating a simple algorithm employing C functions.
Understanding the Basics of Functions
Functions in C are essentially modular blocks of code that perform specific tasks. They accept input values (arguments), process them, and return an output value (result). Functions promote reusability and modularity in code, making your programs more efficient and easier to maintain. To effectively write functions in C, you should be familiar with their structure and fundamental components.
A function in C is generally defined by its name, input arguments, return type and a function body containing a set of instructions to process the input and return a result.
A typical C function has the following structure:
Return_Type Function_Name (Argument_Type Argument_Name, ...)
{
// Function Body
// ...
return value;
}
Here are some key components of a C function:
- Return_Type: Determines the type of value the function will return (e.g., int, float, char). If the function does not return a value, use
void
as the return type. - Function_Name: The unique name used to identify the function. Follows the same naming conventions as variables in C.
- Argument_Type: The types of input parameters the function accepts, such as int, float, or char.
- Argument_Name: The name of the input parameters for reference within the function body.
- Function Body: The block of code within the curly braces, which performs the required operations on the input parameters and returns the result.
Steps on How to Write a Function in C
Writing functions in C requires a systematic approach. Follow these steps to create your own custom functions:
- Determine the purpose and functionality of the function. What task will the function perform, and what will it return?
- Decide on the function's input parameters (arguments) and their types. What inputs does your function need to complete its task?
- Choose an appropriate return type for your function, indicating the type of value it will return to the calling code.
- Write the function body, which is the set of instructions needed to process the input arguments, compute the result, and return the output value using the
return
statement. - Test your function with various input values to ensure it works as intended.
For example, consider a function that calculates the area of a rectangle. The input parameters would be the length and width of the rectangle, both integer values. The function returns an integer value, the calculated area.
int calculate_area(int length, int width)
{
int area = length * width;
return area;
}
Creating a Simple Algorithm for Functions in C
Now that you understand the basics of writing a function in C, you can create a simple algorithm using multiple functions to solve a problem. To illustrate this, let's create an algorithm that calculates the area of multiple rectangles and finds the one with the largest area.
- Write a function that accepts two integers (length and width) and returns the area of the rectangle, as shown in the previous example.
- Write a second function that accepts the number of rectangles (n) and an array of length n containing the dimensions of the rectangles (length and width).
- Iterate through the array calling the first function to calculate the area of each rectangle.
- Keep track of the maximum area encountered while iterating.
- Return the maximum area found.
- Test your algorithm with different sets of rectangles and verify its correctness.
int calculate_area(int length, int width)
{
return length * width;
}
int find_maximum_area(int n, int dimensions[][2])
{
int max_area = 0;
for(int i = 0; i < n; i++)
{
int area = calculate_area(dimensions[i][0], dimensions[i][1]);
if(area > max_area)
{
max_area = area;
}
}
return max_area;
}
By following the steps outlined in this article, you'll be well on your way to writing efficient and reusable functions in the C programming language. Remember to keep your functions focused on specific tasks, as this ensures better code organization, maintainability, and readability.
Write Printf Function in C
The Printf function is a powerful and versatile tool in C programming, allowing you to format and display data to the console. This section will explore the importance of the Printf function, its various components, and how to correctly implement it in your C functions.
Importance of the Printf Function
The Printf function in C is critical for a variety of reasons, including:
- Data output: It enables you to output various types of data, such as integers, floating-point numbers, characters, and strings, to the console, making it easier to understand and analyse the program's execution process.
- Data formatting: The Printf function allows you to format output data in a specific manner, align numbers, add prefixes, and control the number of decimal places for floating-point numbers. This enhances data readability and presentation.
- Debugging: Printf is a powerful debugging tool for developers to trace the execution flow, display variable values, and identify issues within the program logic.
In summary, the Printf function is not only essential for displaying output data but also provides valuable capabilities for formatting and debugging, ensuring a smoother and more efficient development process.
How to Implement Printf in C Functions
Implementing the Printf function in C involves understanding its structure, components, and format specifiers. Let's delve into the details for effective implementation.
The Printf function is defined in the stdio.h
header file, so you need to include this header file in your C programs to use the function:
#include
The general syntax for the Printf function is as follows:
printf("Format String", Arguments);
The key components of the Printf function include the Format String and Arguments.
- Format String: A string that contains text and format specifiers, indicating how the arguments should be displayed. The format specifiers begin with a percentage sign (%) followed by a character representing the datatype.
- Arguments: The variables or values to be displayed in the formatted output. The number of arguments must match the number of format specifiers used in the Format String.
Here's a table of common format specifiers in C:
Format Specifier | Description |
%d | Integer |
%f | Floating-point number |
%c | Character |
%s | String |
%% | Percentage sign |
To implement Printf in your C functions, follow these steps:
- Include the
stdio.h
header file in your C program. - Determine the data types of the variables you want to display and select the appropriate format specifiers.
- Construct the Format String, incorporating any required formatting options and the chosen format specifiers.
- Call the Printf function with the Format String and the required arguments.
- Compile and execute your program to observe the formatted output.
Here's an example of using Printf to display a set of variables:
#include
int main()
{
int a = 10;
float b = 3.14;
char c = 'x';
char s[] = "Hello, World!";
printf("a = %d, b = %.2f, c = %c, s = %s\n", a, b, c, s);
return 0;
}
This code demonstrates the implementation of the Printf function to display a variety of data types, including integers, floating-point numbers, characters, and strings, as well as controlling the number of decimal places for a floating-point number. Implementing Printf in your C functions will greatly enhance the display, readability, and debugging of your program's output.
Difference Between Writing a Program and a Function in C
Although programs and functions in C are related, understanding their differences is vital to grasp the overall structure of C code as well as the roles of individual components. Let's explore the main differences between writing a program and a function in C.
Characteristics of a Program
A C program mainly comprises a collection of functions working together to achieve a specific goal or solve a particular problem. The key characteristics of a C program are as follows:
- Contains one or more functions, including a mandatory
main()
function, which serves as the entry point for the program execution. - Execution begins at the
main()
function and proceeds sequentially through called functions. - Functions within a program can call other functions to perform specific sub-tasks, fostering modularity and code reusability.
- Typically written in one or more source files (
.c
files) that can be compiled and linked together to create an executable file (.exe
or.out
files). - Can use global variables and constants, which are accessible throughout the program.
- Can include libraries and header files to utilise pre-built functions and data types.
Characteristics of a Function
A function in C is a self-contained block of code that performs a specific task when called by the program. Functions are the building blocks of a C program, promoting modularity and reusability. The key characteristics of a C function are:
- Consists of a unique function name, input parameters (arguments), a return type, and a function body containing the specific instructions to perform the task.
- Can return a value to the calling code using the
return
statement, or return nothing (using thevoid
return type). - Function parameters can be organised in different ways, such as call-by-value and call-by-reference, which govern how data is passed between the function and the calling code.
- Can have local variables with limited scope, only existing within the function execution.
- Defined once in a C program but called multiple times as needed, granting reusability.
Understanding Write Functions in C
To write efficient functions in C programming, it is essential to consider the following aspects:
- Purpose: Clearly define the goal of the function, ensuring that it is focused on a single, specific task. This improves readability, maintainability, and reusability of the function.
- Argument Handling: Determine the input arguments and their types required for the function to perform its purpose. Consider using pointers for passing large data structures and arrays for efficient memory usage.
- Return Values: Choose an appropriate return type for the function based on the expected output value. If the function does not return a value, use
void
as the return type. - Error Handling: Incorporate appropriate error handling within the function using constructs like conditional statements, loops, and exception handling mechanisms (such as
setjmp()
andlongjmp()
) to handle potential issues gracefully. - Documentation: Document the function using comments to explain its purpose, input parameters, return values, and any assumptions or limitations. This enhances code readability and maintainability.
- Testing and Debugging: Test the function with various input values, edge cases, and invalid inputs to ensure it works as intended and properly handles unexpected situations. Use debugging tools like GDB to identify and fix issues in the function logic.
By understanding the differences between a program and a function in C, as well as focusing on writing efficient, modular, and reusable functions, you can create well-designed C programs that effectively tackle complex problems.
Advanced Concepts in Write Functions in C
To further improve your skills in writing functions in C, it is essential to explore advanced concepts such as function types, return values, and utilising recursion. These topics enable a deeper understanding of how to create efficient and sophisticated functions that solve complex programming problems.
Function Types and Return Values
In C, function types and return values play a significant role, especially when handling more complex programming tasks. Function types are primarily defined by their return values and input parameters. Understanding how to utilise various function types and return values can optimise the flow of data and functionality within your program.
Here are some key functions types and return value considerations to keep in mind:
- Void Functions: Functions with a return type of
void
do not return any values. These functions perform actions or modify data without providing a result to the caller. To create a void function, usevoid
as the return type and omit thereturn
statement. - Scalar Functions: Functions that return a single value, such as an integer, float, or character, are referred to as scalar functions. Scalar functions are common and can be used to perform calculations, search for values, or manipulate data in specific ways.
- Array Functions: Functions that return arrays can be more complex but provide added functionality. In C, functions cannot directly return an array; instead, you can return a pointer to the first element of the array. Remember to allocate memory dynamically for the returned array and manage memory allocation and deallocation properly.
- Functions with Structs: Functions can also return structures, which are user-defined data types containing multiple elements of different data types. To return a structure, define the function with the appropriate struct type as the return value, and return an instance of the structure at the end of the function body.
Utilising Recursive Functions in C
Recursion is a powerful concept in programming that allows a function to call itself to solve complex problems. Recursive functions in C consist of a base case (or terminal condition) and a recursive case, which calls the function itself with updated input parameters.
To write a recursive function in C, follow these steps:
- Define the overall problem you want to solve using recursion.
- Break the problem into smaller sub-tasks, which can be solved by the same function with simpler input parameters.
- Determine the base case (or terminal condition), which is the simplest possible sub-task the function can handle without requiring further recursion.
- Define the recursive case within the function logic, which calls the function itself with updated input parameters.
- Ensure that the input parameters passed in the recursive call converge towards the base case to prevent infinite recursion.
- Test the recursive function for various input cases to verify correctness and efficiency.
Here's an example of a recursive function in C that calculates the factorial of a positive integer:
int factorial(int n)
{
// Base case: 0! = 1 or 1! = 1
if (n == 0 || n == 1)
{
return 1;
}
// Recursive case
return n * factorial(n - 1);
}
When utilising recursive functions in C, be cautious of potential pitfalls, such as infinite recursion, stack overflow, or redundant calculations. To mitigate these issues, use appropriate base cases, prefer iterative solutions when possible, and employ techniques like memoisation to store intermediate results and prevent redundant computations. By understanding and applying advanced function concepts like function types, return values, and recursion, you can create more efficient and versatile C programs to tackle a wide range of programming challenges.
Tips for Writing Optimised Functions in C
Writing optimised, well-documented, and error-free functions in C is crucial for creating efficient and robust programs. In this section, we'll discuss the importance of proper documentation and some techniques for debugging and error handling.
Importance of Proper Documentation
Proper documentation is a critical aspect of writing and maintaining efficient functions in C. It improves the readability and understanding of your code, making it easier for others (or even yourself) to work on the project in the future. Here are some essential tips for effective documentation:
- Comments: Include descriptive comments within your code, explaining the purpose of each function, the input parameters, the return values, their types, and any assumptions or limitations associated with each function.
- Consistency: Use a consistent commenting style throughout the code for better readability and maintainability. This can include the use of block comments or line comments with a standard format.
- Self-Explanatory Code: It's often better to write self-explanatory function names, variable names, and code structures to minimise the need for extensive comments. Choose descriptive and concise names that convey the purpose of each function and variable.
- Documentation Tools: Utilise documentation tools such as Doxygen to automatically generate code documentation from well-commented source files. This helps maintain an organised and up-to-date documentation system.
- Code Maintenance: As your project evolves, continuously review, update, and improve your documentation to keep it accurate and relevant.
Debugging and Error Handling Techniques
Debugging and error handling are essential techniques to improve performance, eliminate bugs, and ensure proper program execution. Some essential debugging and error handling techniques for writing optimised C functions include:
1. Compile-time and Run-time Debugging
To identify and fix issues in the code as early as possible, consider the following debugging methods at both compile-time and run-time:
- GDB: Utilise the GNU Debugger (GDB) to debug C programs during execution. GDB allows you to set breakpoints, inspect variable values, and execute code line by line to identify issues.
- Static Analysis: Use static code analysis tools like Splint or PVS-Studio to detect potential bugs and vulnerabilities in your code during the development process.
- Compiler Warnings: Enable and pay attention to warnings generated by the C compiler (e.g.,
-Wall
option in GCC) to identify potential issues and risks in the code.
2. Error Handling
Implement proper error handling within your C functions to ensure your program runs smoothly and can handle unexpected situations gracefully. Consider these methods for improved error handling:
- Conditional Statements: Use
if
,else
, andswitch
statements to verify and handle error conditions within your functions. - Return Values: Employ return values and error codes to communicate the outcome of a function, including any error conditions, to the calling code.
- Assertions: Utilise the C assert macro to check for conditions that should always be true and halt the program execution if they are not met. This helps identify logic errors in the code.
- Exceptions: In some cases, consider using
setjmp()
andlongjmp()
to manage and handle exceptions within C functions when traditional error handling techniques are insufficient.
By following these tips, you can significantly improve the optimisation, reliability, and maintainability of your C functions. Proper documentation, debugging, and error handling are essential practices to write high-quality and optimised functions that form the foundation of successful C programming projects.
Write Functions in C - Key takeaways
Write Functions in C: Modular blocks of code performing specific tasks, enhancing code readability and reusability.
Function structure: Return_Type Function_Name (Argument_Type Argument_Name, ...), with a function body containing instructions.
How to write a function in C: Determine purpose, input arguments, return type and write the function body, then test with various inputs.
Printf function in C: Powerful tool for formatting and displaying data, essential for data output, formatting and debugging.
Difference between writing a program and a function in C: Programs contain multiple functions, whereas functions are self-contained blocks of code performing specific tasks.
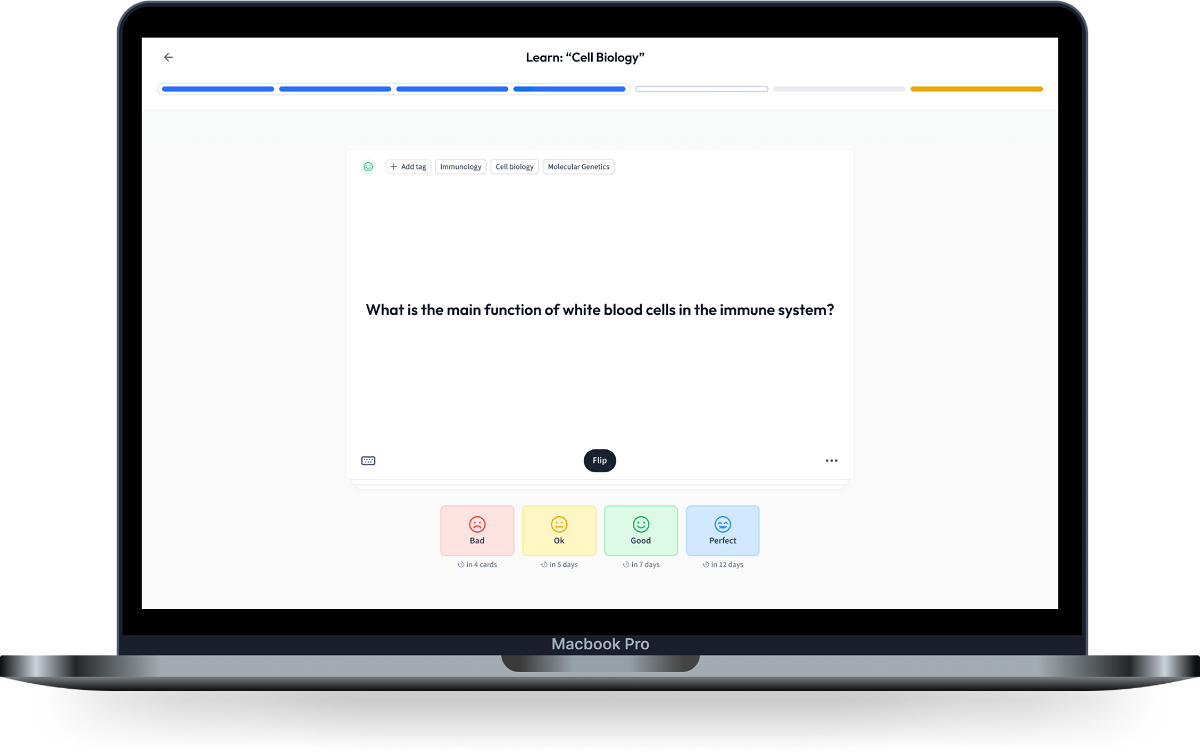
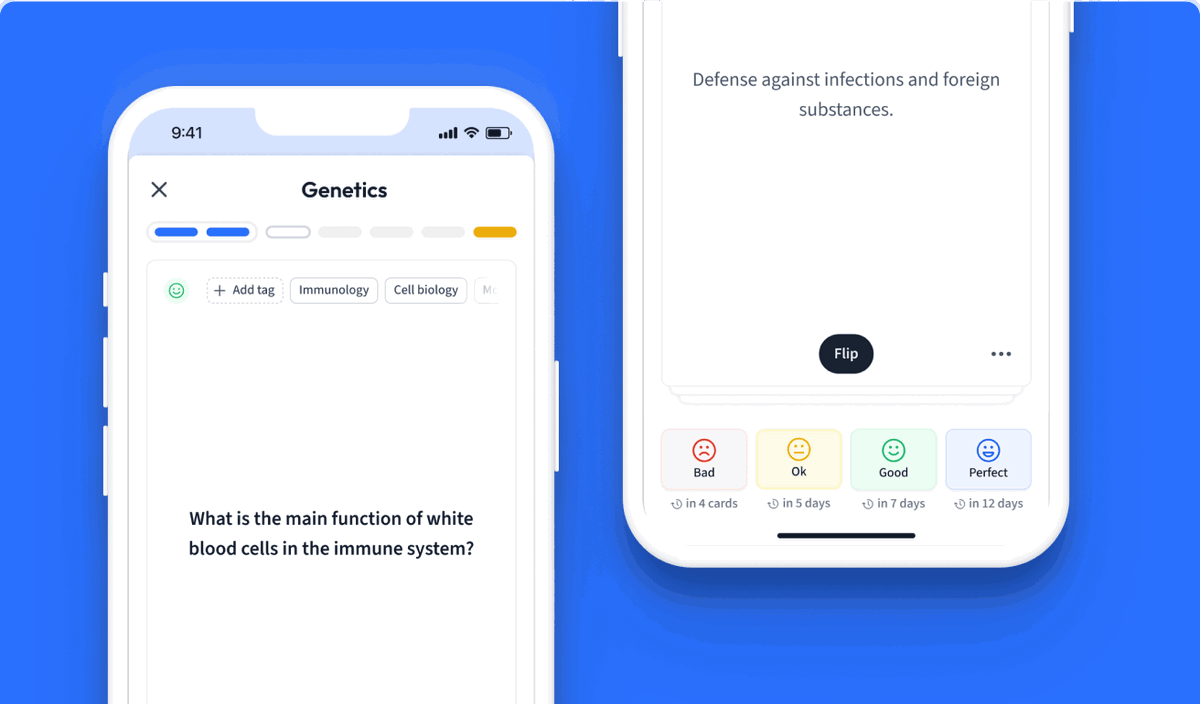
Learn with 13 Write Functions in C flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Write Functions in C
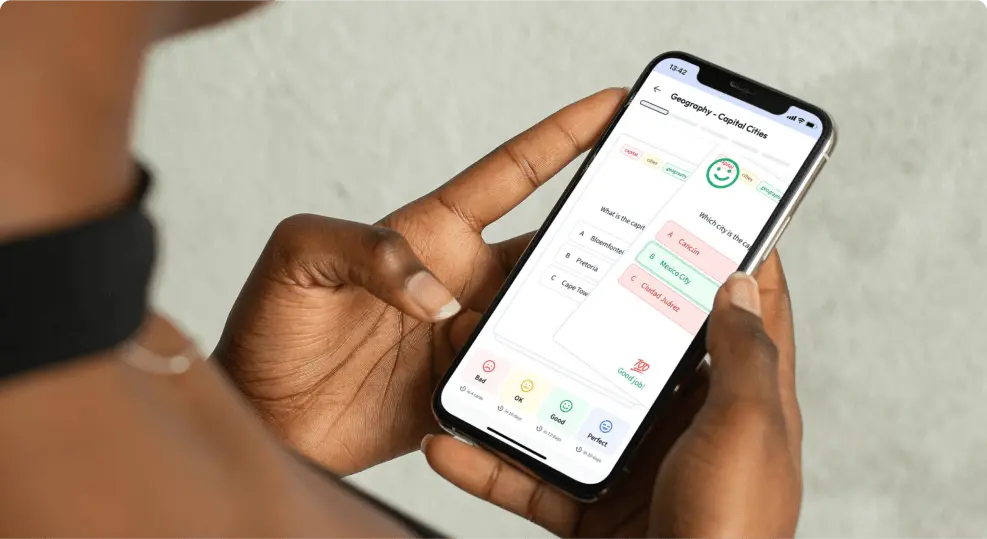
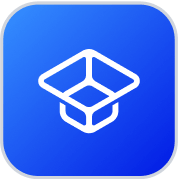
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more