Understanding Java Single Dimensional Arrays
In the realm of computer science, Java is a versatile and popular language. As a student of this subject, you will come across an important concept called Java Single Dimensional Arrays. It is a fundamental building block that allows you to manage and organize data effectively.
Definition: What is a Single Dimensional Array in Java
For understanding this, let's break it down. In Java, an array is a container object that holds a fixed number of values of a single type. The length of an array is established when the array is created. At creation, the array elements are initialized to their default values.
A single dimensional array in Java is an array that holds a sequence of elements, all of the same type, accessed through an index. For instance, if we create an array to store integer data type values, all elements of the array must be integers, and they are accessed using their index positions. The index usually starts from zero and continues to the length of the array minus one.
Primary Functions of a Single Dimensional Array in Java
Single dimensional arrays offer various functions:
- Store same type elements: Single dimensional arrays permit you to reserve memory locations to store elements of the same types.
- Access elements using indices: It's easy to access these elements using indices which start at 0.
- Code readability: They help in organizing data in a linear manner, thereby enhancing readability.
The Anatomy of a Single Dimensional Array in Java
Let's delve into the structure of a single dimensional array. It relates to the way an array is structured in memory. A single dimensional array in Java actually signifies an ordered list of elements. Think of these as slots or cells in memory, each able to contain an element and each identifiable by an index.
Index | 0 | 1 | 2 | 3 | 4 |
Element | 'a' | 'b' | 'c' | 'd' | 'e' |
As seen above, each cell corresponds to an index, which is used to access the element stored in that cell.
Constructing a Single Dimensional Array in Java: A practical guide
Now let's understand how you construct a Single Dimensional Array in Java. Creating arrays comprises two steps: declaring the array variable and allocating memory for the array values.
int [] arr; //declaring array arr = new int[5]; // allocating memory for 5 integers
{There are other ways to declare an array. For instance, you can declare and initialize an array at the same time. Here is an example:
int arr[] = new int[] {1, 2, 3, 4, 5};In the above example, we've created an array called 'arr' and added five elements to it. The first position 'arr[0]' will hold 1, 'arr[1]' will hold 2, and so on.}
You can also access elements of the array. Remember, indices always start at 0. For instance, if you'd like to get the second element from our array 'arr', use 'arr[1]'.
A great strength of arrays in Java is that they are objects. They contain methods like 'clone()', 'equals()', 'length', etc. You can use these methods in your coding journey with Java single dimensional arrays. It's also worth noting that array indices are themselves 'int' data types, so try to ensure they don't get too large. If you try to create an array with more than \(2^{31} - 1\) (or approximately 2 billion) elements, you're going to get an 'OutOfMemoryError'.
Dissecting Examples of Single Dimensional Array in Java
Applying the theory to practice, it's time to dive into some examples of a single dimensional array in Java. These examples will stimulate your appreciation of how this important aspect of the language works.
Basic Example of Single Dimensional Arrays in Java
Let's start with a basic example. Here you'll declare a single dimensional array and allocate memory for each element in the array.
double[] grades; // declaring grades = new double[5]; // allocating memory for 5 elements
This array, named 'grades', can hold five elements. Each of these elements is a double.
After declaration, you may want to initialize each element of the array. This is how you do it:
grades[0] = 85.5; // Assigning values to elements grades[1] = 79.7; grades[2] = 88.8; grades[3] = 92.9; grades[4] = 72.1;
Now, each index of the array 'grades' holds corresponding values ranging from 0 to 4. You can access the values using their indexes.
Advanced Use Cases: Single Dimensional Array Examples in Java
Moving on, let's focus on some advanced examples of single dimensional arrays in Java.
Array Initialization at the time of Declaration
int arr[] = {10, 20, 30, 40, 50};
In this case, you have declared, created and initialized the array simultaneously. The array 'arr' can hold five elements, each of which has been assigned a value at the same time.
Summing Elements of an Array
int arr[] = {10, 20, 30, 40, 50}; int sum = 0; for(int i=0; iThis example demonstrates how you can sum all the elements of an array. We used a for loop to access every element through its index and added the value to the 'sum'.(Note: 'arr.length' gives the size of array 'arr')
Finding the Largest Value in an Array
int arr[] = {10, 20, 70, 40, 50}; int max = arr[0]; for(int i=1; imax){ max = arr[i];} } This code snippet finds the largest value in the array. It initializes 'max' to the first element of the array, then compares 'max' with each element. If the array element is larger, 'max' is updated to that value.
How to Create a Single Dimensional Array in Java: A Step by Step Tutorial
Now that you've seen some examples, let's walk through the process of creating a single dimensional array step by step.
- Declaration: First, decide on the type of elements that the array will hold and declare it accordingly. This can be integer, double, character or any object.
int[] firstArray; //Declaration- Instantiation: Secondly, allocate memory to the array using the 'new' keyword. At this time, you need to specify the number of elements the array will hold.
firstArray = new int[3]; //Instantiation- Initialization: Finally, assign values to each element of the array using the indexes.
firstArray[0] = 10; //Initialization firstArray[1] = 20; firstArray[2] = 30;Combining all three steps at once, the process would look like this:
int[] firstArray = new int[]{10, 20, 30}; //Declaration, Instantiation & InitializationOnce created and initialized, you can manipulate your array, use it in operations, or display its contents by traversing the array (often using looping structures).
In conclusion, understanding how to create and manipulate single dimensional arrays in Java is a must-have skill for any budding programming enthusiast. Mastering it can significantly improve your expertise and add a useful tool to your Java toolbox.
Applying Single Dimensional Arrays in Java to Calculate Standard Deviation
Whether you're examining test scores, comparing weather patterns or discovering financial trends, the concept of standard deviation is prevalent across several fields. In Java programming, however, it holds a special place. Through single dimensional arrays, Java offers a convenient and robust way to calculate the standard deviation of a dataset.
A Practical Guide to Calculating Standard Deviation Using a Single Dimensional Array in Java
The standard deviation is a statistical measure that reveals the amount of variation or dispersion within a set of values. Intuitively, it indicates how spread out numbers are. The standard deviation of a set of values is the square root of the variance.
Variance is computed by taking the average of squared differences from the mean. Let’s represent the mean of the values as \( \mu \), variance as \( \sigma^2 \), and standard deviation as \( \sigma \). The formula for variance can be represented as:
\[ \sigma^2 = \frac{1}{n} \sum_{i=1}^{n} (x_i - \mu)^2 \]And the standard deviation is the positive square root of variance:
\[ \sigma = \sqrt{\sigma^2} \]In Java, you can store your dataset in a single dimensional array, calculate the mean value, and then compute the variance and standard deviation.
Coding Tutorials: Using Java Single Dimensional Arrays for Standard Deviation Calculation
Time to get to the code now!
- Store your dataset: Use a single dimensional array to store your data set. For this illustration, let's consider an array of 5 numbers.
double[] data = {10, 20, 30, 40, 50};- Calculate the mean: Obtain the mean (average) by summing all the numbers in the array and dividing by the count of numbers.
double total = 0; for(int i=0; i- Calculate variance: Work out the variance. For each number, subtract the mean and square the result (the squared difference). Then work out the average of those squared differences.
double variance = 0; for(int i=0; i- Calculate standard deviation: The standard deviation is just the square root of the variance.
double stdDeviation = Math.sqrt(variance);This example will calculate the standard deviation for the dataset stored in the 'data' array. Now you can use it in your algorithm for whatever computational requirement you have in your application.
Tips for improvement: While this code works perfectly fine, there's always a place for improvement. Instead of iterating over your data array twice (first to calculate the mean and then for variance), you could calculate the mean and variance in a single pass by keeping a running total of the values and their squares. This optimization is often useful when dealing with a large dataset.
In conclusion, single dimensional arrays in Java provide an efficient and convenient way to calculate statistical measures like standard deviation. Using arrays to hold your dataset, standard deviation calculation becomes a matter of applying mathematical formulas using the appropriate Java syntax and operations.
Comparing Single Dimensional and Multi-dimensional Arrays in Java
The decision to use either a single dimensional array or a multi-dimensional array in Java can impact the efficiency, simplicity, and effectiveness of your code. Both have their unique utilities and it's pivotal to comprehend the basic differences between the two, as well as their suited application.
Fundamental Differences between Single Dimensional and Multidimensional Array in Java
In Java, Arrays are a powerful data structure that can hold a fixed number of values of a single type. The length of an array is established when the array is created, which provides us with a wonderful solace: even though arrays are flexible, we always know how much memory a particular array will consume.
Yet, arrays can differ in their dimensions:
- A single dimensional array, often simply called an array, is a linear data structure with a specific number of cells that can hold data. Each cell or slot of the array can be accessed via its index.
- A multi-dimensional array can be thought of as an 'array of arrays.' This isn’t entirely accurate, but aids in understanding. In practice, multi-dimensional arrays can be visualised as tables with rows and columns.
Here's an example to showcase the difference:
A Single Dimensional Array:
int[] singleArray = new int[]{10, 20, 30, 40, 50};In this example, you're declaring, instantiating, and initializing a single dimensional array at once. The array holds five integer elements.
A Multi-dimensional Array:
int[][] multiArray = new int[][]{ {1, 2, 3}, {4, 5, 6}, {7, 8, 9} };In this snippet, you're using a two-dimensional array. This array can be visualised as a 3x3 matrix, where each row contains three elements (integers in this case). So, it's an array comprising three arrays, and you can access an element by specifying two indices instead of one.
When to Use Single Dimensional Array vs Multidimensional Array in Java
Conceptual and practical understanding of arrays is important, but equally necessary is knowing when to appropriately apply single dimensional or multi-dimensional arrays in your programming routines.
A single dimensional array is perfect for situations where you need to manage ordered data, such as:
- Storing a list of items of the same type (Such as names of students enrolled in a course).
- Keeping track of data over time (Such as temperatures recorded over a week).
- Gathering responses to survey questions (Such as yes/no responses to a survey question).
In contrast, a multi-dimensional array is most beneficial when you need to represent or navigate through grid-based data. Some appropriate examples are:
- Creating matrices for mathematical computations.
- Representing data in more than one dimension (Such as a chessboard).
- Dealing with pixel-based data in image processing.
- Picturing relational data in databases (rows and columns).
Remember, while it is possible to solve a problem with either type of array, choosing the best fit can significantly improve readability, maintainability, and efficiency of your code. Understanding the fundamental differences and optimal usage scenarios of single and multi-dimensional arrays in Java offers you a strong foundation for tackling complex data structures in the future.
Java Single Dimensional Arrays - Key takeaways
- A single dimensional array in Java is an array that holds a sequence of elements of the same type, each accessed by an index.
- Functions of a single dimensional array are storing same type elements, accessing elements using indices, and enhancing code readability.
- Creating single dimensional arrays in Java constitutes two steps: declaring the array variable and allocating memory for the array values. For instance, int[] arr; // declaring array, arr = new int[5]; // allocating memory for 5 integers.
- Arrays in Java are objects and contain methods like 'clone()', 'equals()', 'length', etc. Notably, array indices in java are 'int' data types.
- Both single dimensional and multi-dimensional arrays can be used in Java, where a single dimensional array has a linear structure and multi-dimensional array can be thought of as a 'array of arrays' or like tables with rows and columns.
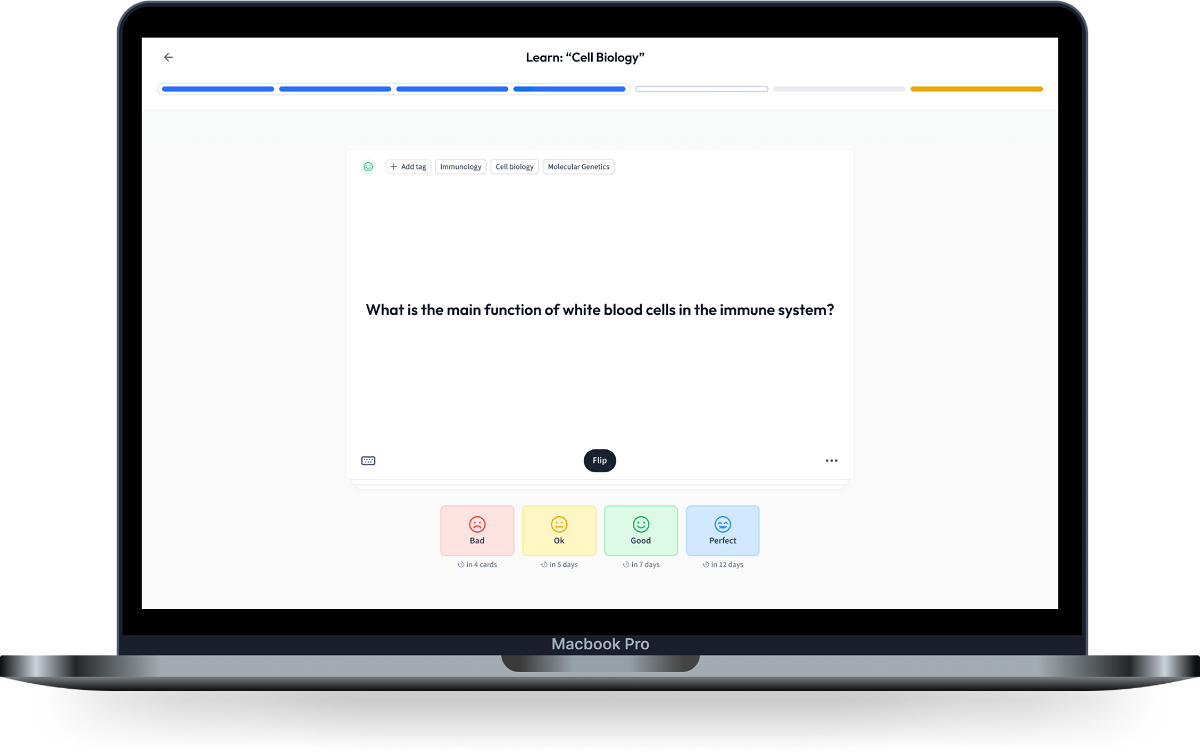
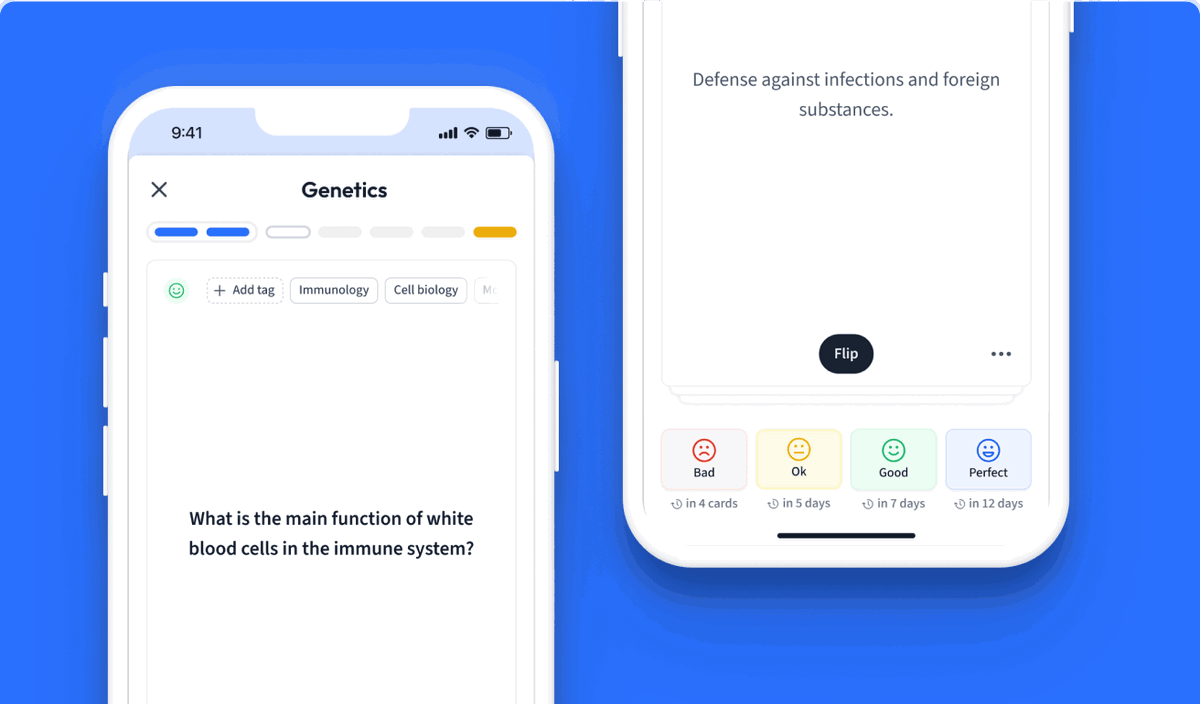
Learn with 12 Java Single Dimensional Arrays flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Java Single Dimensional Arrays
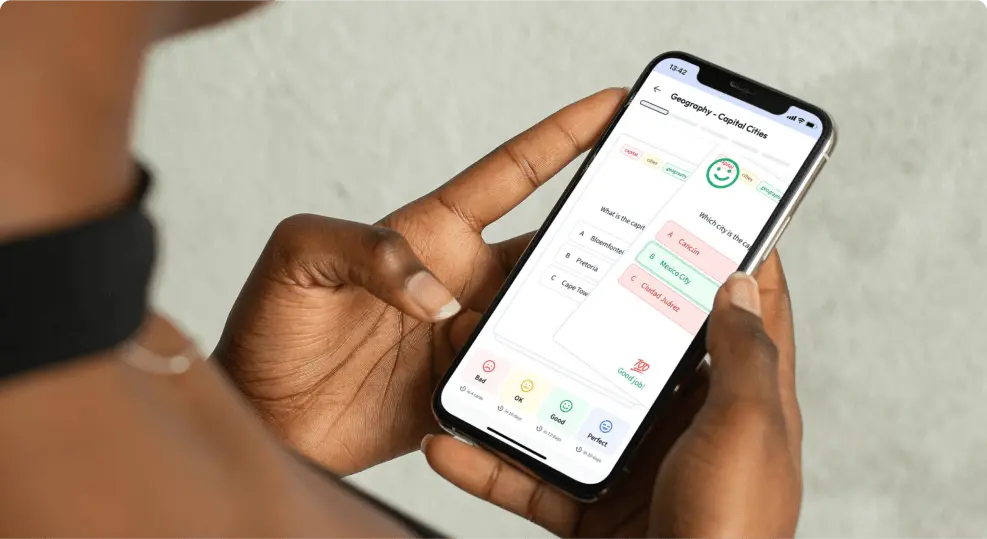
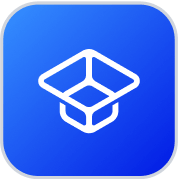
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more