Understanding Singleton Pattern in Computer Programming
Delving into the world of computer science, it's essential to grasp the concept of design patterns. Among these stands the Singleton Pattern, a remarkably powerful tool, skillfully crafted to address particular challenges in object-oriented programming. Let's embark on a journey to comprehend this intriguing pattern in detail.
Definition of Singleton Pattern
The Singleton Pattern is a design paradigm in object-oriented programming that restricts the instantiation of a class to a single object. This pattern ensures that only one instance of the class exists and provides a global point of access to it.
Picture a small town with only one Bank. This bank is where everyone goes to withdraw, deposit, or transfer funds. Here, the Bank represents a Singleton class. No matter how many customers need banking services, they all have to use that one Bank, and cannot create another one.
Importance of Singleton Design Pattern in Programming
Why should you care about the Singleton Design Pattern? There are a handful of reasons:
- Enforced control over the access to shared resources
- Reduced system complexity
- Providing a shared state among all instances
- Generation of unique global access point
Many experts consider Singleton as an anti-pattern because it interferes with the testability of code and often promotes the use of global state. Yet, used wisely, Singleton can be quite effective in cases where exactly one object is needed to coordinate system actions.
Improved Resource Access with Singleton Design Pattern
Object-oriented programming has a keen emphasis on the encapsulation of data, which can sometimes impair the accessibility of resources. Singleton pattern surfaces as a remedy by providing global access point to the resources.
Without Singleton: | Resources may be scattered, resulting in unclear communication and coordination |
With Singleton: | Establishes clear points of entry for resource access, ensuring optimal utilization |
Reducing System Complexity: A Benefit of Singleton Pattern
Singleton pattern promotes a less complicated and more manageable structure within a system.
Without Singleton: | Multiple instances could lead to conflicting system behaviour and increase complexity |
With Singleton: | The single object instance reduces conflicts, thereby simplifying system management |
Consider an organization with multiple department heads. If each head starts making independent decisions, the organization may plunge into chaos. However, if there's a single decision-making authority, say a CEO, things become coordinated and less complex. This is akin to the working of a Singleton class in a software system.
Singleton Pattern Implementation: A Deep Dive
Transcending the basic understanding of Singleton Pattern and venturing into its implementation in real-world programming can be a fascinating exploration. When applying this pattern, the primary goal is to ensure only a single instance of a class exists throughout your programme and provide a global point of access to it. Let's delve deeper into this to comprehend the nuances involved.
Successful Singleton Pattern Implementation: Key Factors to Consider
When you plan to implement the Singleton pattern successfully in your programmes, several key factors need careful consideration:
- Thread Safety: In a multithreaded program, multiple threads attempting to create an instance could potentially create many instances. Synchronisation mechanisms are needed to control the access to the Singleton object.
- Lazy Initialisation: It’s beneficial to delay the creation of the Singleton instance until it is needed. This approach is called lazy initialisation and can improve the efficiency of your programs.
- Serialization and Cloning: You need to handle serialization and cloning carefully. If not properly handled, they could lead to multiple instances of the class.
Imagine a situation where we have a printer queue. We want to manage all printing tasks from a single access point, a PrinterSpooler. However, multiple users (threads) could potentially set up their own PrinterSpooler. Here, we would have to manage the synchronisation carefully, to guarantee that only one PrinterSpooler is created and accessed by all users.
Balancing Efficiency and Simplicity in Singleton Pattern Implementation
In a quest for efficient Singleton Pattern implementation, don't overlook simplicity - an attribute that wraps complexity and empowers other developers to understand your code easily. Balancing efficiency and simplicity is all about making smart choices in your coding practices.
Efficiency: | If you create a Singleton instance right when the program starts (known as eager initialisation), it promotes faster access but at the cost of increased startup time and potential memory waste if the object is not used. |
Simplicity: | Lazy initialisation code can be more complex due to the need for thread safety, but it ensures that the Singleton instance is created only when needed, improving memory use and startup time. |
\[\text{Efficiency} + \text{Simplicity} \rightarrow \text{Successful Singleton Pattern Implementation}\]
Example of Singleton Pattern in Practice
Let's examine a simple yet illustrative example of Singleton pattern implementation in Java.
public class Singleton { private static Singleton instance; private Singleton() {} public static synchronized Singleton getInstance() { if(instance == null) { instance = new Singleton(); } return instance; } }
In this example, the class 'Singleton' has a private static instance of itself and a private constructor, ensuring no other class can instantiate it. The 'getInstance' method provides global access to the Singleton instance, and the 'synchronized' keyword ensures thread safety during the creation of the instance.
Note: Although the synchronized method achieves thread safety, it has a performance drawback as it locks the method to be accessed by only one thread at a time. A double-checked locking mechanism is a more efficient approach where synchronization is needed only during the first object creation.
Exploring Singleton Pattern in Various Programming Languages
Mastering Singleton Pattern isn't just about understanding its theory and significance. It's also about applying it skilfully across different programming languages. Each language has its unique syntax, style, and intricacies, thus the implementation of Singleton pattern varies accordingly. Let's explore it in the context of prominent programming languages- Java, C#, Python, C++, and JavaScript.
Singleton Pattern Java: An Examination
Java, being one of the most commonly used object-oriented languages, provides a robust environment to implement the Singleton pattern. It follows the principles of object-oriented programming and supports static fields and methods, which greatly facilitate the Singleton implementation.
A typical Singleton class in Java would look something like this:
public class Singleton { private static Singleton instance; private Singleton(){} public static synchronized Singleton getInstance(){ if(instance == null) { instance = new Singleton(); } return instance; } }
However, in a multithreaded environment, the synchronized getInstance() method can be a performance bottleneck. An improved version is to use "double-checked locking", which reduces the use of synchronization and increases performance.
Using Singleton Pattern C# for Optimised Coding
C# also follows an object-oriented approach and hence, Singleton pattern finds its application here too. However, it has peculiarities with regard to thread safety and object reference handling, which are crucial factors in Singleton Pattern implementation.
Key Features of Singleton Pattern in C#
C# creates Singleton pattern differently using keywords like 'static' and 'readonly', as it supports 'eager initialisation' and 'lazy initialisation'. The thread safety can be easily managed in C# using the sealed keyword that limits the inheritance and object creation.
The Singleton implementation in C# using static readonly type with lazy initialisation looks like:
public sealed class Singleton { private static readonly Singleton instance = new Singleton(); private Singleton(){} public static Singleton Instance { get { return instance; } } }
Python Singleton Pattern: An Essential Guide
Python, a high-level and highly readable programming language, due to its simplicity, has an unconventional manner of Singleton implementation. The lack of private constructors and the presence of advanced features (like metaclasses and decorators) allow for unique and strikingly simple Singleton construct.
Practical Use of Singleton Pattern in Python Projects
Despite simplicity, ensuring that the requirement of a single instance is upheld in Python necessitates careful design. The Singleton pattern is, however, employed in Python projects for logging services, database connections and configurations.
A Singleton pattern in Python, employing decorator, can be implemented as such:
def singleton(class_): instances = {} def wrapper(*args, **kwargs): if class_ not in instances: instances[class_] = class_(*args, **kwargs) return instances[class_] return wrapper @singleton class Singleton: pass
Mastering Singleton Pattern C++ for Efficient Software Development
C++, with its extensive use and support for object-oriented programming, effectively implements Singleton pattern. Despite no direct language support for Singleton pattern, C++ enables its implementation using private constructors, static fields and functions.
The Role of Singleton Pattern in C++ Programming
In projects requiring global access to some information (like configuration data) or communication among disparate parts of a project, Singleton comes in quite handy. Handling multi-threaded Singleton in C++ programming is also essential, albeit tricky.
The following is an implementation of Singleton in C++:
class Singleton { private: static Singleton* instance; Singleton(){} public: static Singleton* getInstance() { if (!instance) instance = new Singleton(); return instance; } };
Simplifying Code with Singleton Pattern Javascript
JavaScript, primarily used in web development, although not a class-based object-oriented language, supports Singleton pattern implementation too. JavaScript is a prototype-based language that uses scopes and closures. Hence, Singleton pattern can be created by using private variables and methods.
Singleton Pattern Javascript: A Hands-on Approach
Singletons are massively beneficial in JavaScript for organising code in a modular way. They allow you to group functions together with their related data and can often be replaced with a simple object literal. The pattern is also useful for caching data and maintaining a 'Single Source of Truth' in your systems.
A Singleton in JavaScript can be implemented as follows:
var Singleton = (function () { var instance; function createInstance() { return new Object("This is the instance"); } return { getInstance: function () { if (!instance) { instance = createInstance(); } return instance; } }; })();
Singleton Pattern Technique: Elevating Your Programming Skills
In the realm of programming, Singleton Pattern, a design solution belonging to the creational pattern group, emerges as a powerful technique. This design pattern ensures that only one instance of a class is created in an application or project. Implementing Singleton Pattern in your programming practices is a game-changer and could immensely elevate your coding skills. It provides a controlled mechanism for accessing a particular class, thereby preventing the issue of global variables.
Advantages of Using Singleton Pattern Technique In Your Code
Singleton Pattern comes heaving with a multitude of benefits, which underscore its favourable choice among developers. Here are some benefits that could persuade you to incorporate Singleton Pattern in your programming:
- Controlled Access: Singleton pattern provides a controlled mechanism to access a class. By encapsulating the class inside a Singleton object, others cannot directly access it. This organised access control is instrumental in maintaining code integrity.
- Resilient to Global Variable Overuse: Singleton pattern effectively saves you from the perils associated with the rampant use of global variables. It avoids data being unintentionally overwritten, a common issue with global variables.
- Only Single Instance: When adhering to Singleton, only a single instance of a class can be created. This feature is beneficial in situations when a single object is in demand, like, in database connectivity or a logger.
- Lazy Loading: Singleton allows loading on demand. The class instance is not created until a client requests it for the first time. Such lazy loading can greatly boost the memory efficiency of your programs.
Ensuring Consistency with Singleton Pattern Technique
A crucial advantage of the Singleton Pattern is the consistency it provides. Code consistency, an often overlooked aspect, is key to effective programming. A consistent code reduces complexity, enhances readability and maintainability. Singleton Pattern plays a significant role in driving such consistency.
Consistency through Singleton Pattern is maintained through its very principle— limiting the number of instances of a class to just one.
Consider a configuration settings object in an application. In this case, Singleton Pattern ensures that all parts of the app refer to the same settings object, which is crucial for keeping the configuration settings consistent across the system. Without Singleton, different parts of the app might reference their own settings objects that could potentially lead to inconsistent behaviour.
Mistakes to Avoid While Applying Singleton Pattern Technique
While Singleton Pattern offers significant advantages, a casual or misguided approach towards its implementation can lead to detrimental effects. Here are some mistakes programmers often commit while applying Singleton Pattern:
- Misusing as Global Variable: Singleton can provide access to a global instance of itself, but it should not be misused as a substitute for global variables. Singleton’s purpose is controlled access, not global exposure.
- Ignoring Thread Safety: In a multithreaded environment, it's possible that two threads gain access to the creation process, leading to two instances. Hence, developers should synchronise the instance creation process to achieve thread safety.
- Inappropriate Usage: Singleton should not be used to define classes that need multiple instances. Singleton Pattern should only be implemented when it is clearly required by program logic.
Common Pitfalls in Singleton Pattern Application
Beyond misconceptions while applying Singleton Pattern, there are some common pitfalls developers often encounter, mainly due to unawareness or inadvertency. Let's explore these major stumbling blocks:
- Serialization: Serialization creates a new instance of the class, which could potentially violate Singleton Pattern’s principle. One must implement readResolve() method to return the Singleton instance, mitigating this pitfall.
- Reflection: Java’s Reflection can access private constructor of Singleton class and create new instances, hence violating the Singleton. To resolve this, throw a run-time exception in constructor if more than one instance is created.
- Cloning: Clone method creates a copy of the Singleton class and hence breaks Singleton. To prevent this, override the clone method and throw an exception.
Let's consider the case of Serialization. If you have a Singleton class in your Java code and you serialize the Singleton's class object, upon deserialization, a new instance will be created, which contradicts the Singleton pattern. To overcome this, implement the readResolve() method in your Singleton class:
protected Object readResolve() { return getInstance(); }
This will ensure that the instance returned by the readResolve() method will replace the new object created due to deserialization, preserving the Singleton property.
Singleton Pattern - Key takeaways
- Singleton Pattern: A design solution that provides global access to resources, promoting a more manageable system structure. It helps reduce conflicts and system complexity by ensuring only a single instance of a class exists throughout a programming project.
- Singleton Pattern Implementation: Crucial factors to consider for successful implementation includes ensuring thread safety, adopting lazy initialisation, and careful handling of serialization and cloning.
- Balance of Efficiency and Simplicity: While eager initialisation promotes faster access, it might increase startup time and potential memory waste. On the other hand, lazy initialisation improves memory use and startup time but may require more complex code for thread safety.
- Singleton Pattern across Various Programming Languages: The application of Singleton Pattern varies depending on the programming language. Noteworthy examples include Singleton Pattern in Java, C#, Python, C++, and JavaScript.
- Benefits of Singleton Pattern Technique: It offers controlled access to a class, safeguards against overuse of global variables, allows only a single class instance and supports lazy loading for enhanced memory efficiency.
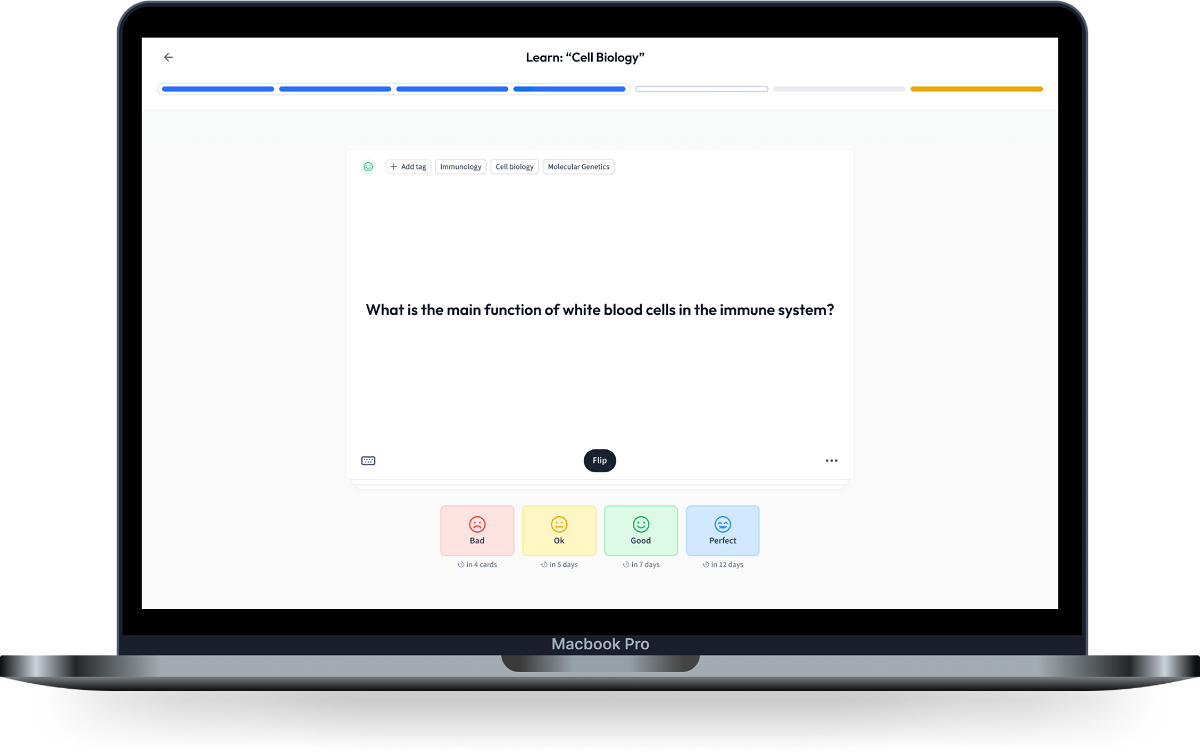
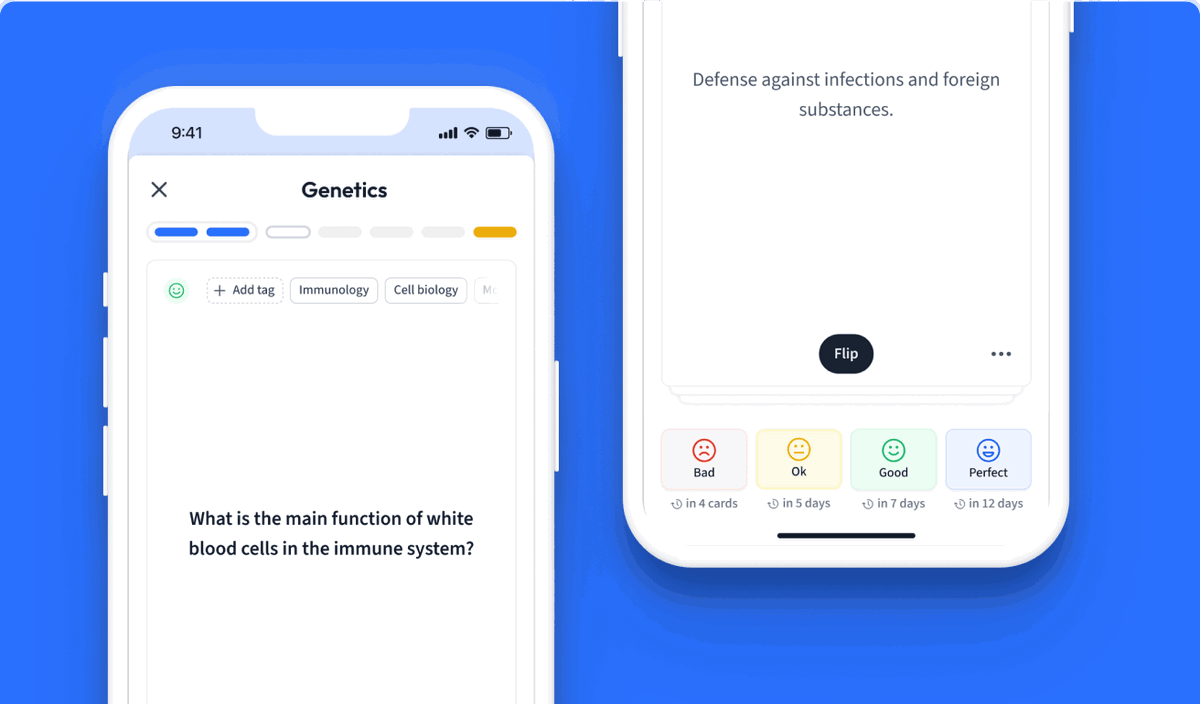
Learn with 12 Singleton Pattern flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Singleton Pattern
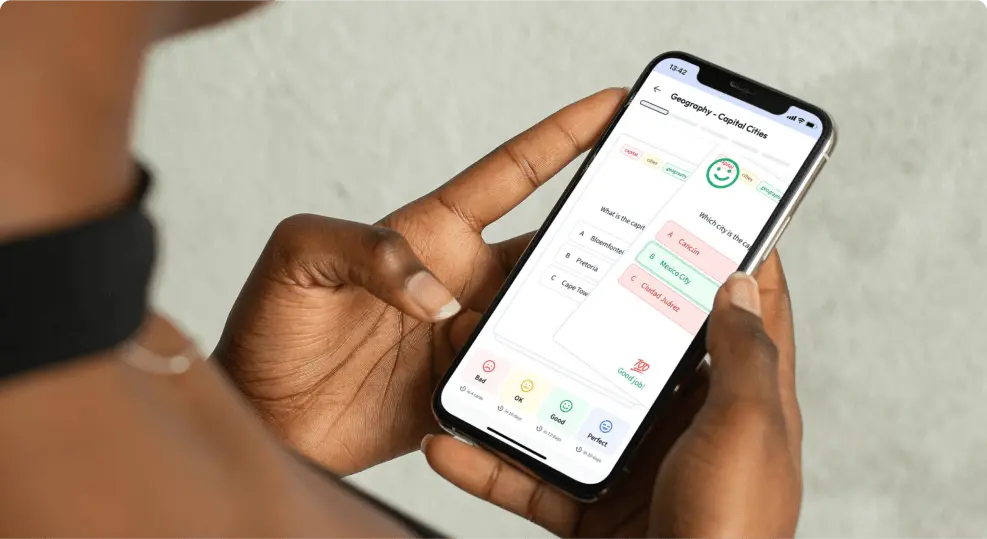
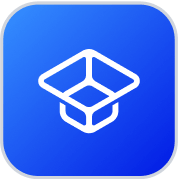
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more