Understanding Java Method Overriding
If you are delving into the depths of object-oriented programming in Java, then getting to grips with Java Method Overriding is a must. Advanced Java programming revolves around certain core concepts and Method Overriding plays a crucial role in them.Method Overriding in Java is a feature which allows a subclass or child class to provide a unique implementation of a method that is already given by its parent class or superclass.
What is Method Overriding in Java: An Introduction
Method Overriding allows a subclass to inherit the fields and methods of a superclass. But what if the method inherited from the parent class doesn't fit well with the child class? Here lies the real strength of Method Overriding; it allows you to redefine the inherited method in the child class, tailoring it to fit the needs of the subclass. A bullet-point summary of method overriding rules in Java:- The method must have the same name as in the parent class
- The method must have the same parameter as in the parent class.
- There must be an IS-A relationship (inheritance).
Basics of Java Method Overriding Technique
When overriding a method in Java, the name and signature (parameters) of the new method in the child class must match the name and signature in the parent class. As shown in the simple table:Method Name | Parameters | Overridden? |
calculateArea | int length, int breadth | Yes |
calculateArea | int radius | No (Different Parameters) |
calculateVolume | int length, int breadth | No (Different Name) |
Distinguishing Between Overloading and Overriding in Java
Java Method Overriding should not be confused with method overloading. While both concepts involve re-defining a method, there is a fundamental difference:Method Overloading involves multiple methods in the same class with the same name but different parameters, whereas Method Overriding involves two methods, one in the parent class and the other in the child class, with the same name and parameters.
Take a look at this code snippet to illustrate the difference:
class Animal { void walk() { System.out.println("The animal walks"); } } class Cat extends Animal { void walk() { System.out.println("The cat walks"); } }Here, the method 'walk()' in the Cat class is overriding the same method in the Animal class. This is a simple demonstration of Method Overriding.
In context of polymorphism, if a parent reference variable is holding the reference of the child class and we have overridden the method in the child class, it's the child class method that is invoked at runtime. This concept, also known as runtime polymorphism, is one of the most powerful features of Java Method Overriding.
How to Apply Java Method Overriding
Now that you're acquainted with the fundamental idea of Java Method Overriding, it's time to deep dive into its application. You will find it fascinating to know that this advanced feature helps architect sustainable Java applications.Fundamentals of Java Method Overriding Syntax
To override a method in Java, you need to recreate it in the subclass with precise correctness to include the same signature and return type as the method in the superclass. Following are the syntactical rules to follow when overriding a method:- Access Modifier of the overriding method (method of SubClass) can't be more restrictive than the overridden method (method of SuperClass).
- If the superclass method declares an exception, the subclass overridden method can declare the same, a subclass exception or no exception, but can't declare parent exception.
- If the superclass method doesn't declare an exception, subclass overridden method can't declare any checked exception.
- The overriding method must have the same return type (or subtype).
Here's a simple example of Method Overriding syntax:
public class Vehicle{ public void run(){ System.out.println("Vehicle is running"); } } public class Car extends Vehicle{ public void run(){ System.out.println("Car is running"); } }
Practical Java Method Overriding Examples
For understanding the power of Java Method Overriding, it is best practised with its application in real-world programming scenarios.Here is an extensive example that illustrates this:
public class Mammal { public void eat() { System.out.println("Mammal eats"); } } public class Cat extends Mammal { public void eat() { System.out.println("Cat eats rat"); } }In this example, the Cat class overrides the eat() method of the Mammal class. Hence, when you create an instance of Cat and call its eat() method, it would display 'Cat eats rat'.
Step-by-Step Guide to Java Method Overriding
Let's break down the process of applying Java Method Overriding via a step-by-step guide: 1. Define a superclass. This will be the parent class, and it should contain the method that you want to override. 2. Define a subclass that extends the superclass. This child class will override the method of the superclass. 3. In the subclass, declare the method with the same name and parameters as the one in the superclass. This replicated method should contain the new implementation that you want to introduce. 4. Create an instance of the subclass and, through this object, call the method. As the method has been overridden in the subclass, this method call will execute the new implementation in the subclass, not the one in the superclass. With these basics, you can now plunge into coding and practice Method Overriding. Being hands-on with these techniques would enhance your coding skills and make you a proficient Java developer.Exploring the Usage of Java Method Overriding
Taking a dive into Java Method Overriding further, let's explore how this programming concept is actually utilised in programming. A thorough grasp of these applications will enable you to more effectively use this technique in your own code.Common Uses of Java Method Overriding
Java Method Overriding is often used in scenarios where a developer needs to modify or enhance the functionality of a method in a subclass that has been inherited from a superclass. Here are some situations where Java Method Overriding is commonly put to use:- Logic modification: At times, you might need to implement logic in a subclass that's slightly different from the one provided in the superclass. Method overriding is a perfect fit for such scenarios where you can effectively modify the logic as per the needs of your subclass.
- Adding more functionality: Sometimes, in the subclass, you might want to extend the functionality of a method inherited from a superclass. Java Method Overriding allows you to extend the method's functionality without modifying the superclass method.
- Preventing modifications: If you want to restrict any further modifications to a method in a subclass, you can use the final keyword when declaring the method in a superclass. This effectively prevents the method from being overridden in any of its subclasses.
Java Method Overriding in Object-Oriented Programming
Java Method Overriding is a cornerstone of Object-Oriented Programming (OOP) and a key feature of Java. In OOP, the concepts of classes and objects allow you to model real-world entities. The process of inheritance allows a new class to inherit the properties of an existing class. However, there might be instances where a subclass may need to define its own behaviour that is already provided by its parent class. This is where Method Overriding comes into play. Consider a simple class hierarchy of Shape --> Circle --> Sphere. Here, the Shape class might define a method to calculate area. However, the subclass Circle might want to provide its own implementation to calculate its area, distinct from the Shape class. This demonstrates an effective use of method overriding in OOP where the subclasses provide their own tailored implementation. It's worth noting that the subclasses can call the superclass’s implementation of the overriden function with the use of super keyword. The super keyword refers to the immediate parent of a class. The keyword super is mainly used in the subclass method definition where you want to refer to the superclass’s version of the method.Here is an example code demonstrating usage of super keyword:
public class Shape { public void area() { System.out.println("Shape's area"); } } public class Circle extends Shape { public void area() { super.area(); // calling superclass's area method System.out.println("Circle's area"); } }Circle class is overriding the area() method of the Shape class, but it also makes a call to the superclass’s implementation using the super keyword.
Advantages of Java Method Overriding in Programming Projects
The benefits of method overriding become particularly apparent when working on more complex programming projects. Some key advantages of using Java Method Overriding in these contexts are:- Code Reusability: Using Java method overriding allows you to reuse code from a superclass, without needing to rewrite the entire method in the subclass. This saves effort and decreases the likelihood of errors.
- Flexibility: Method overriding provides flexible programming, allowing you to create a method in a subclass with the same name and signature as in the superclass, but with a different implementation. This allows the subclass to maintain its uniqueness.
- Support for Polymorphism: Method overriding is essential in supporting runtime polymorphism in Java. It allows an object to take many forms and enables a programmer to refer to a subclass object using a parent class reference, which is essential in dynamic method dispatch.
- Extensibility: Our program can be extended in terms of logic, sector and behaviour with the help of Java method overriding. As a result, you can extend a method's functionality without modifying the superclass method.
Java Method Overriding - Key takeaways
- Java Method Overriding is a feature in object-oriented programming that lets a subclass or child class provide a unique implementation of a method already present in its parent class or superclass.
- The rules for method overriding in Java require the method to have the same name, the same parameters as in the parent class, and an IS-A relationship (inheritance).
- Java Method Overriding shouldn't be confused with method overloading which involves multiple methods in the same class with the same name but different parameters.
- A code example of Java Method Overriding shows the method 'walk()' in the 'Cat' class overriding the same 'walk()' method in the 'Animal' class.
- Runtime polymorphism refers to the phenomenon of the method in the child class being invoked at runtime when a parent reference variable holds the reference of the child class.
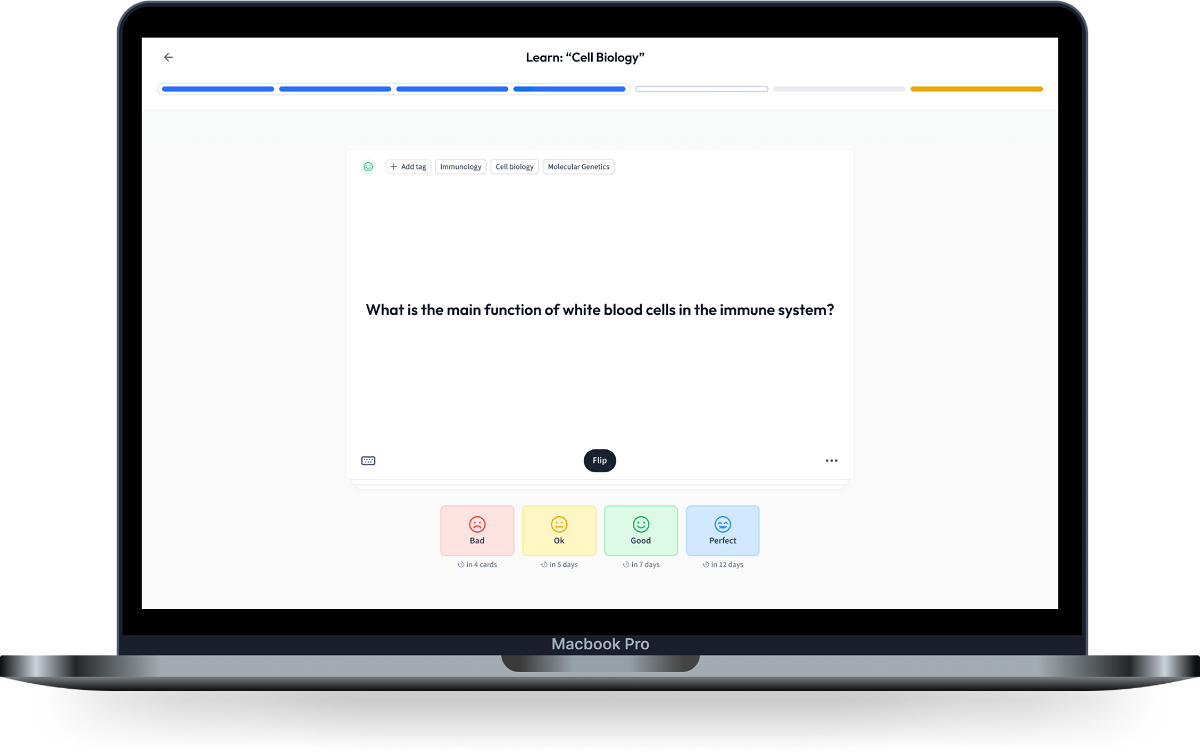
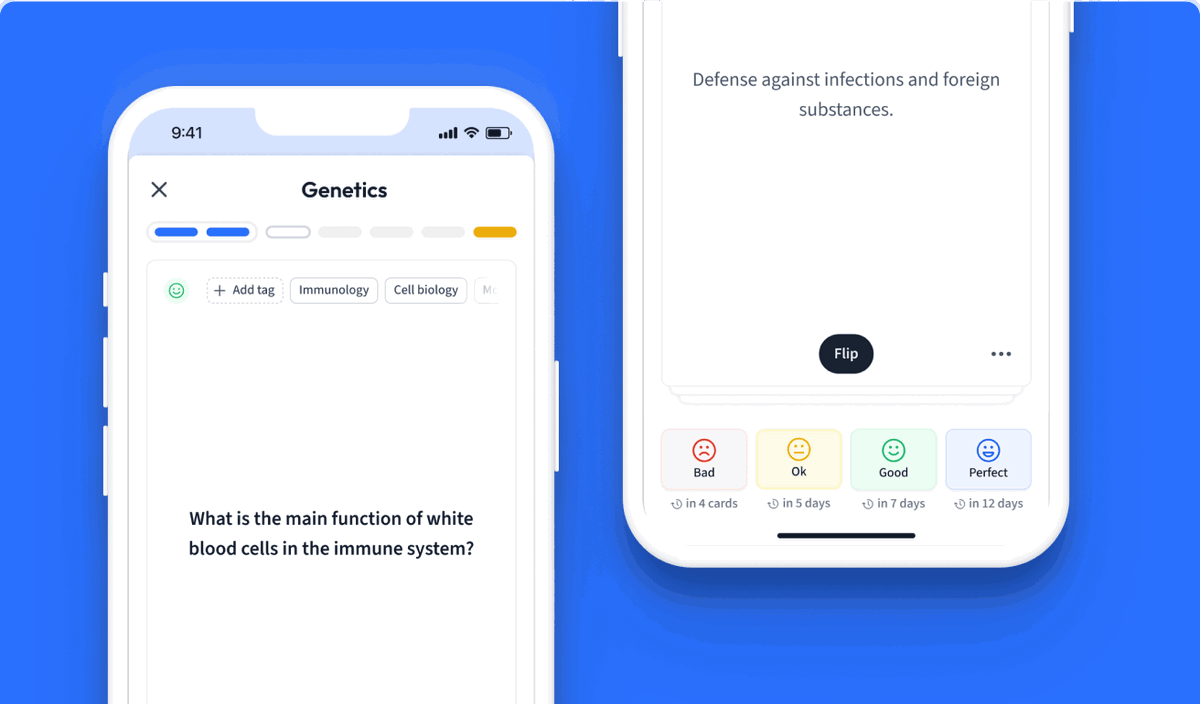
Learn with 12 Java Method Overriding flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Java Method Overriding
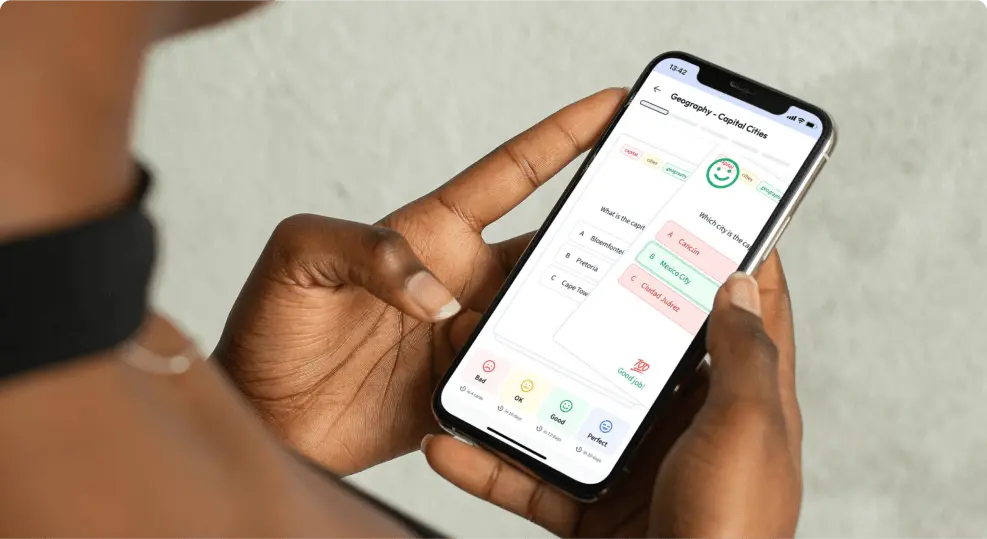
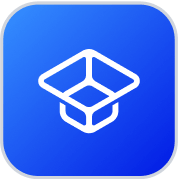
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more