What are Single Structures in C?
When embarking on the journey through the vast landscapes of computer science and programming, encountering Single Structures in C is inevitable. These structures play a vital role in organising, storing, and managing data in a more logical and accessible manner. Understanding their essence unlocks a myriad of possibilities in data manipulation and software development.
Understanding Single Structures in C definition
Single Structures in C are user-defined data types that enable the storage of different types of data under one name. They provide a method for grouping variables of diverse data types into a single logical unit, making it easier to handle complex data.
Consider the following example that demonstrates defining a simple structure in C:
typedef struct { int id; char name[50]; float salary; } Employee;
Here, the structure Employee encapsulates an integer, an array of characters, and a float into a single entity.
Key components of Single Structures in C
To appreciate the versatility and functionality of single structures in C, grasping the key components that constitute these structures is essential.
- Structure Declaration: The foundation of using structures, where the blueprint of the structure is defined.
- Defining Structure Variables: After declaring a structure, variables of that structure type can be defined, which is akin to instantiating objects in object-oriented programming.
- Accessing Structure Members: The individual components of a structure can be accessed using the dot (.) operator, enabling manipulation of specific data within the structure.
Following the Employee structure example, defining a variable and accessing its members would look like this:
Employee emp1; emp1.id = 123; strcpy(emp1.name, "John Doe"); emp1.salary = 50000.0;
This snippet effectively creates an instance of the Employee structure named emp1 and initializes its members.
Understanding the architecture and the capability of Single Structures in C provides a robust foundation for delving into more complex programming paradigms. With these structures, you can develop sophisticated data models that mirror real-world entities, laying the groundwork for more advanced topics such as linked lists, trees, and graphs in computer science.
Implementing Single Structures in C
Delving into the realms of C programming involves mastering the art of data structure implementation. Among various data structures, the single linked list stands out for its dynamic nature and flexibility. Here, you will learn the fundamental concepts and step-by-step guide on implementing single structures in C, focusing on single linked lists.
Basics of creating a Single linked list for a structure in C
A single linked list in C is a sequence of nodes, where each node contains data and a pointer to the next node in the sequence. It's a pivotal data structure because of its efficient memory utilization and ability to dynamically grow and shrink at runtime. Understanding its basics is crucial for advanced C programming.
Node: The fundamental unit of a single linked list, typically represented by a structure containing at least two members - one for storing data and another for pointing to the next node in the list.
To depict a Node in C, consider the following structure:
typedef struct Node { int data; struct Node* next; } Node;
This structure represents a node that holds an integer data and a pointer to the next node.
Remember, the pointer to the next node in a single linked list should be set to NULL if it is the last node, indicating the end of the list.
Step by Step: implementing Single Structures in C
Implementing single linked lists involves several key steps: creating nodes, linking them together, and traversing the list to access or modify data. Follow this guide to smoothly navigate through these steps.
Step 1: Creating a Node
Node* createNode(int value) { Node* newNode = (Node*)malloc(sizeof(Node)); newNode->data = value; newNode->next = NULL; return newNode; }
Step 2: Inserting a Node at the Beginning
void insertAtBeginning(Node** head, int value) { Node* newNode = createNode(value); newNode->next = *head; *head = newNode; }
Step 3: Traversing the List
void traverseList(Node* head) { Node* temp = head; while(temp != NULL) { printf("%d\n", temp->data); temp = temp->next; } }
These steps outline the basic operations on single linked lists in C. With practice, you'll be able to perform more complex operations, such as deleting a node or reversing the list.
Utilising functions like malloc
for creating nodes dynamically allows your list to grow as needed at runtime, showcasing the flexibility of single linked lists.
Functions of Single Structures in C
Single Structures in C play a pivotal role in the realm of computer science, particularly in the orchestration of complex data manipulation and organisation. Exploring their functions further provides a granular understanding of their application in programming and how they streamline data management tasks.
An overview of Single Structures in C functions
Functions associated with single structures in C extend beyond simple data storage. They facilitate a streamlined approach towards managing and utilising complex aggregated data. From simplifying code readability to enhancing memory management, understanding these functions can unlock new efficiencies in your programming projects.
Some of the core functions benefiting from single structures include:
- Data Encapsulation: Structures allow the grouping of different data types under a single name, encapsulating the data for cleaner code and easier manipulation.
- Enhanced Memory Management: By precisely defining what data is required and its structure, one can make more efficient use of memory - a crucial consideration in many programming scenarios.
- Improved Code Readability and Maintenance: Using structures, variables related to the same entity are grouped together, making the code more understandable and easier to maintain.
Mastering these functions paves the way for optimized program design, crucial for tackling increasing complexity in software development.
Practical Single Structures in C example
To illustrate the practicality and adaptability of Single Structures in C, let's delve into a real-world example demonstrating how structures can be implemented to enhance data management and processing within a program.
Consider the scenario of managing employee records within an organisation. By employing a single structure, various pieces of employee data can be conveniently grouped together:
typedef struct { int employeeID; char name[100]; float salary; char department[50]; } Employee;
This structure, Employee, encapsulates all necessary details into a single logical unit. Creating instances of this structure and manipulating them becomes profoundly simpler, allowing for an organized approach to handling employee data.
Remember, leveraging the power of functions in conjunction with structures, such as initialising, displaying, or modifying the structure's data, further amplifies the efficiency and flexibility of your programs.
Single Structures in C Best Practices
When embarking on C programming projects that involve data management and custom data types, understanding and implementing single structures effectively is crucial. Adhering to best practices not only enhances code efficiency and readability but also prevents common pitfalls that could lead to bugs or performance issues.
Writing efficient code: Single Structures in C best practices
Efficiency in code not only pertains to the speed of execution but also to how resources like memory are used. Here are key practices to follow when working with single structures in C.
- Use typedef for ease of use and readability. This makes your code cleaner and the structure easier to work with.
- Initialize structures properly to ensure that they start with a known state, avoiding any undefined behaviour.
- Consider the use of pointers efficiently to avoid unnecessary copying of data, which can lead to performance degradation especially when dealing with large structures.
- Encapsulate structure-related functions for better modularity and reusability of your code.
Here's a simple example demonstrating these practices:
typedef struct { char name[50]; int age; } Person; void initializePerson(Person *p, const char *name, int age) { strcpy(p->name, name); p->age = age; }
This snippet shows the use of typedef, how a structure is properly initialized, and the use of pointers to modify the data.
Remember to always ensure your string members in structures end with a NULL character to prevent undefined behaviors when manipulating strings.
Avoiding common mistakes with Single Structures in C
Mistakes when using single structures in C can manifest in various forms, from subtle logical errors to more blatant memory management issues. Here's how to avoid some of the most common pitfalls:
- Avoid hardcoding sizes for character arrays. Use #define or const for maximum flexibility and easy modification.
- Be mindful of structure padding and alignment. Different machines may align data differently, potentially affecting performance or causing issues when structures are used in file operations.
- Ensure dynamic memory allocated for structures is properly freed to prevent memory leaks.
- Always check for NULL pointers before dereferencing them when working with structure pointers.
Understanding the intricacies of structure padding and alignment is particularly important. Structures in C are typically aligned to certain byte boundaries for performance reasons. However, this can lead to 'padding' — unused bytes inserted between structure members to align the following member at its preferred boundary. This unused space can lead to discrepancies, especially when writing/reading structures to/from files or sending them over networks. Utilising pragma pack
is one method to control padding, but it must be used judiciously as it can affect portability and performance.
Single Structures In C - Key takeaways
- Single Structures in C definition: User-defined data types that group variables of different data types under one name.
- Structure Declaration: The process of defining the blueprint of a structure.
- Single linked list for structure in C: A sequence of nodes where each node contains data and a pointer to the next node, used for dynamic memory allocation.
- Single Structures in C functions: Offer data encapsulation, enhanced memory management, and improved code readability.
- Single Structures in C best practices: Use of typedef, proper initialization, efficient use of pointers, and encapsulation of related functions to improve modularity.
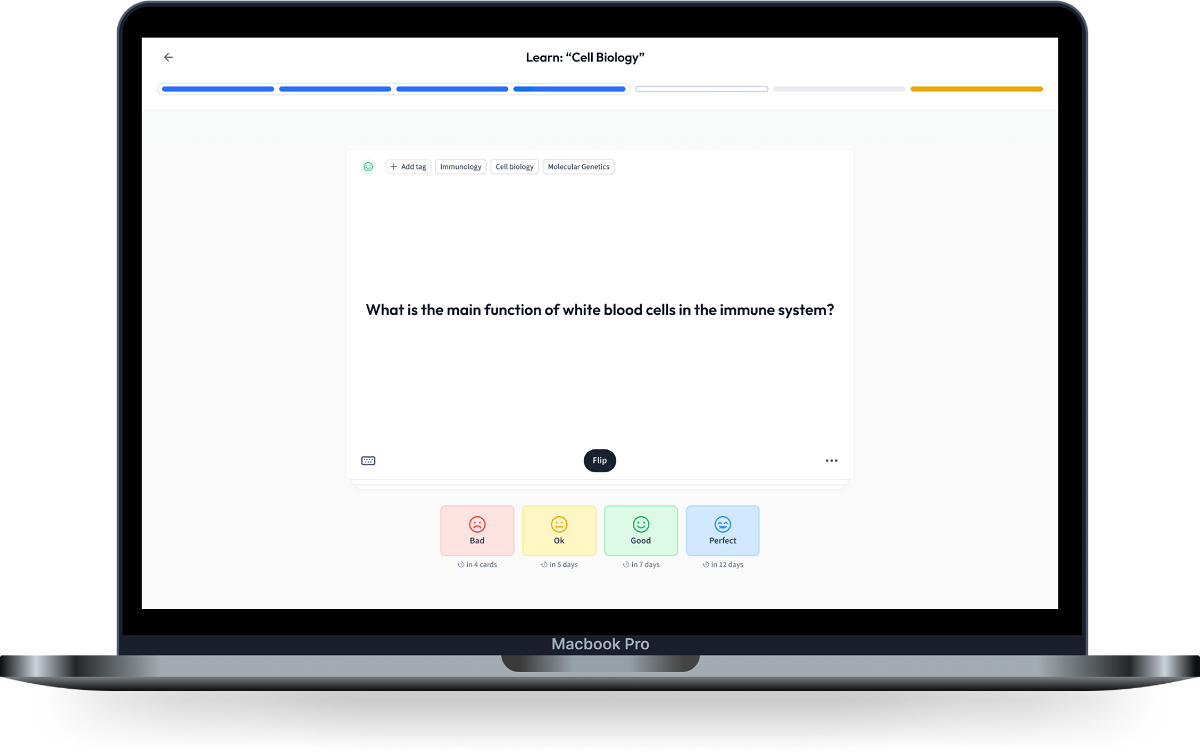
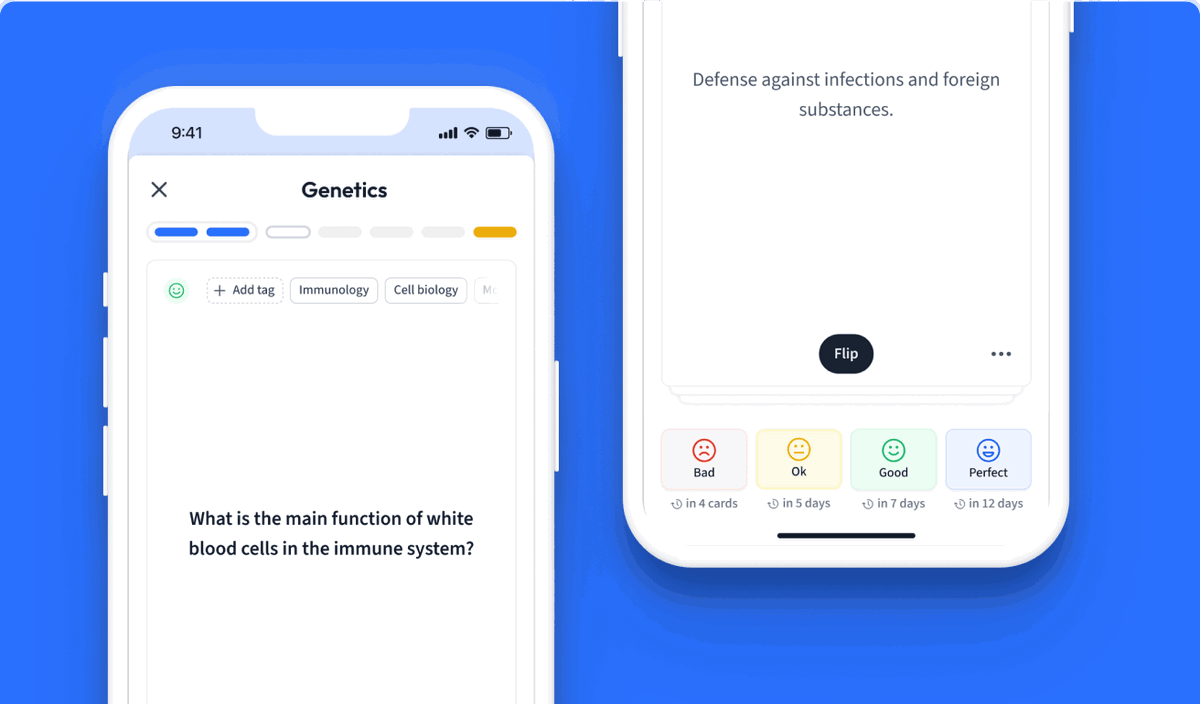
Learn with 23 Single Structures In C flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Single Structures In C
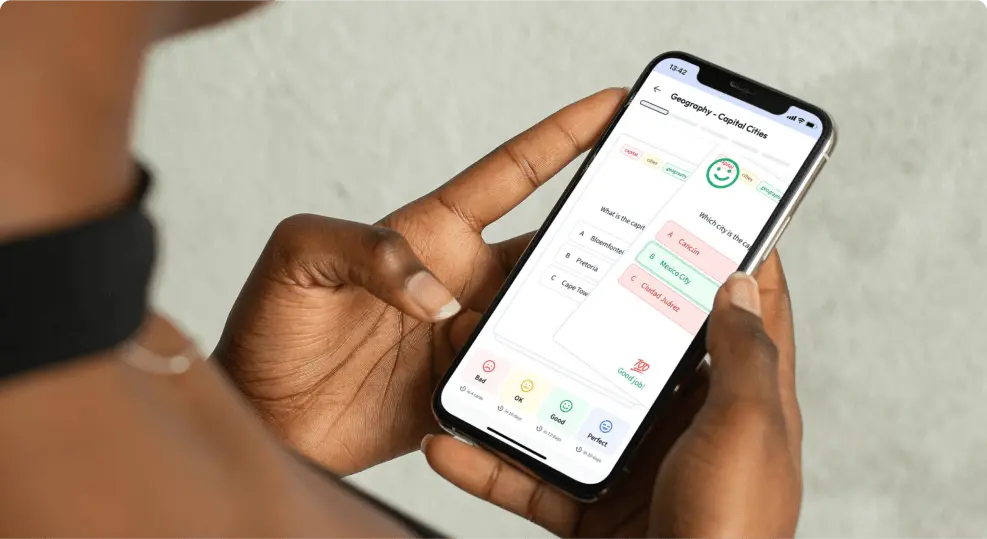
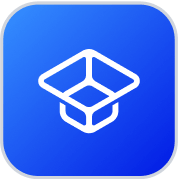
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more