Understanding Javascript Array Methods
Javascript array methods are in-built functionalities of the Javascript language which are designed to effectively manipulate arrays. Arrays are a crucial element in Javascript programming where datasets are required. They allow for the storage and access of multiple data pieces in a single variable, thus simplifying code and making it more efficient.
The Definition of Javascript Array Methods
Javascript has a wealth of array methods that help developers in various ways. These tools offer the ability to quickly transform, iterate, join, slice, reverse, and much more.A Simple Overview of Javascript Array Concepts
Arrays in Javascript, like in other languages, are variables that accommodate multiple values. You can consider it a special variable that's capable of holding more than one value at a time. Array elements are addressed by numerical indexes starting at zero. Array methods, on the other hand, are actions that can be performed on and with arrays. They provide a means to manipulate arrays. An understanding of these methods is key to effectively managing data in Javascript. Consider this array:let fruits = ["Apple", "Banana", "Mango"];Using the array method `.length()`, you can return the number of elements in an array:
let numOfFruits = fruits.length; // Returns: 3
This is a simple example showcasing the use of the `.length()` method on a Javascript array. The `.length()` method returns the number of elements in the array it is called upon. In this case, it returns `3` because there are three items in the `fruits` array.
Javascript Array Methods Explained
Methods available to Javascript arrays include `.push()`, `.pop()`, `.shift()`, `.unshift()`, `.slice()`, `.splice()`, `.concat()`, `.join()` and `.reverse()`. Each method carries out specific functions. For instance, the `push()` method adds new items to the end of an array and returns the new length. Alternatively, `.pop()` removes the last element from an array and returns that element.The `.push` method in Javascript adds one or more elements to the end of an array, and returns the new length of the array. The `.pop` method in Javascript removes the last element from an array and returns that removed element. This method changes the length of the array.
Analysing the Role of Array Methods in JavaScript
Array methods play a significant role in Javascript. They help in modifying arrays, such as adding, updating or deleting elements. Several methods can effectively search an array for a specific value or a set of values. Many of the array methods also make it easy to combine and divide arrays.Imagine having to manually index and retrieve all items in a Javascript array or having to write multiple lines of code to accomplish these tasks. Array methods make this process more efficient by enabling you to perform these operations with simple, built-in functions.
Practical Applications of Javascript Array Methods
In the broad landscape of JavaScript programming, the array methods serve as an essential toolkit. Their practical applications are numerous, ranging from simple manipulations of data within an array to complex operations.Array Methods in JavaScript with Examples
To understand the practical utilities of array methods in Javascript, it's crucial to see them in action. Here's an overview of some commonly used JavaScript array methods, alongside coding examples: push() - This method adds one or more elements to the end of an array and returns the new length of the array.let sports = ["Football", "Cricket"]; sports.push("Tennis"); console.log(sports); // Prints: ["Football", "Cricket", "Tennis"]pop() - This method removes the last element from an array and returns that element.
let sports = ["Football", "Cricket", "Tennis"]; let popped = sports.pop(); console.log(popped); // Prints: "Tennis"shift() - This method removes the first element from an array and returns that element.
let sports = ["Football", "Cricket", "Tennis"]; let shifted = sports.shift(); console.log(shifted); // Prints: "Football"unshift() - This method adds one or more elements to the front of an array and returns the new length.
let sports = ["Cricket", "Tennis"]; sports.unshift("Football"); console.log(sports); // Prints: ["Football, "Cricket", "Tennis"]
Illustrating Javascript Array Methods in Action
Let's dive deeper with some examples of JavaScript array methods in a real-world coding scenario. Use Case 1: Suppose you have a list of product prices. You could use the reduce() method to sum up all the product prices for a total cost.let prices = [10.99, 20.99, 30.99, 40.99]; let total = prices.reduce((sum, price) => sum + price, 0); console.log(total); // Prints: 103.96Use Case 2: If you have a list of movies, you could use the filter() method to get all movies released after a certain year.
let movies = [ {title: "Inception", year: 2010}, {title: "Interstellar", year: 2014}, {title: "Dunkirk", year: 2017}, ]; let recentMovies = movies.filter(movie => movie.year > 2015); console.log(recentMovies); // Prints: [ {title: "Dunkirk", year: 2017} ]
Javascript Array Methods and Their Uses
There's no doubt that the array methods in JavaScript are incredibly versatile. Knowing when and how to use them can greatly increase your efficiency when coding and help to simplify complex tasks. Among the extensive list of array methods, here are a few examples and their uses: concat(): This method is used to merge two or more arrays. It does not change the existing arrays but creates and returns a fresh array. join(): This method is employed to join all elements of an array into a string. You can specify a separator, for example, a comma or a space. reverse(): This method reverses the order of the elements in an array. It changes the original array and does not create a new one. slice(): This method is used to select part of an array and return a new array. It does not alter the original array. sort(): This method sorts the items in an array. By default, it sorts items as strings.Real World Applications of Array Methods in JavaScript
So how are these array methods used in real-world programming? Here are some examples to illustrate their practical applications: concat() could be used in a shopping website to merge arrays of different category products into a single array of all products. join() could be employed in a travel website to transform an array of destinations into a single string of 'Places to Visit.' reverse() might be used in a blog website to display the most recent posts first by reversing an array of post dates. slice() could be used in an e-learning platform to display just a part of the course outline from an array of course content. sort() could be utilized on an e-commerce website to sort products based on price, reviews, or any other user-selected criteria from low to high or vice versa. The list of real-world applications is endless. These are just a few examples to give you a glimpse of their potential. Through an understanding and effective usage of Javascript array methods, you can handle and manipulate data arrays like never before, making your programming more efficient, powerful and dynamic.Navigating the Complexities of Javascript Array Methods
As your journey into the world of JavaScript progresses, there's a good chance you'll come across situations where you need to manipulate data stored in arrays. This is where Javascript array methods come in. While typically more complex than other aspects of JavaScript, the power and flexibility these methods offer make them an indispensable part of the language.Understanding the Concept of Array Methods in JavaScript
In JavaScript, array methods refer to actions or functions that you can perform on or with arrays. They're pre-defined functions that come equipped with the language, sparing you from having to write extensive code to perform common tasks. The magic of JavaScript arrays comes with understanding the properties and methods that extend their utility. These include, but are not limited to:- push() – Adds new elements to the end of an array, and returns the new length
- pop() – Removes the last element of an array, and returns that element
- shift() – Removes the first element of an array, and returns that element
- unshift() – Adds new elements to the beginning of an array, and returns the new length
- concat() – Merges two or more arrays, and returns a copy of the joined arrays
Method | Action |
push() | Add an element to end of array |
pop() | Remove last element of array |
shift() | Remove first element of array |
unshift() | Add an element to start of array |
concat() | Merge arrays |
Breaking Down Complex Javascript Array Methods
Of course, there are other more complex array methods that JavaScript programmers frequently use. These include, but are not limited to:- splice() – Adds/Removes elements from an array
- slice() – Selects a part of an array, and returns the new array
- sort() – Sorts the elements of an array
- filter() – Creates a new array with elements that pass a test
- reduce() – Reduces the array to a single value (from left-to-right)
let fruits = ["Apple", "Orange", "Mango", "Banana"]; fruits.splice(2, 0, "Lemon", "Kiwi"); // The array fruits now becomes: ["Apple", "Orange", "Lemon", "Kiwi", "Mango", "Banana"]The slice() method, conversely, does not alter the original array. Instead, it returns a shallow copy from the original array. This can be incredibly handy when you want to preserve the data in the original array. The filter() and reduce() functions are brilliant additions to JavaScript which are a part of the pantheon of methods that accept a callback and lets us iterate over the array and perform complex tasks.
Advanced Implementation of Array Methods in JavaScript
In the real world, using these JavaScript array methods can simplify your code tremendously. They can reduce lines of code, increase readability, and make code easier to debug. Consider the scenario where you want to compute the sum of an array of numbers. The traditional approach would involve using a loop, but with the reduce method, this becomes a lot simpler:const numbers = [1, 2, 3, 4, 5]; let sum = numbers.reduce((accumulator, currentValue) => accumulator + currentValue);In this example, reduce processes each number in the array, resulting in a final value that's been "reduced" down from the multiple values in the array.
Harnessing the Power of Javascript Array Methods in Advanced Programming
In the landscape of advanced JavaScript programming, effective use of array methods can transform the way you approach and solve problems. Let's consider a complex use-case. Suppose you have an array of objects, where each object represents a book and has properties for title, author, and readers. Now, you want to find all the titles of the books read by a particular user. Here's how you can accomplish this task using the filter() and map() methods.// Array of book objects const books = [ { title: 'JavaScript: The Good Parts', author: 'Douglas Crockford', readers: ['John', 'Kate'] }, { title: 'Eloquent JavaScript', author: 'Marijn Haverbeke', readers: ['Kate', 'Sam'] }, { title: 'JavaScript Allongé', author: 'Reg Braithwaite', readers: ['John', 'Sam'] } ]; // Use the filter and map methods const booksByKate = books .filter(book => book.readers.includes('Kate')) .map(book => book.title) ;In this code snippet, the filter method extracts all book objects where 'Kate' appears in the readers array. The map method then transforms that array of book objects to an array of book titles. Thus, the booksByKate would be `['JavaScript: The Good Parts', 'Eloquent JavaScript']`. As this example shows, JavaScript array methods provide high-level, declarative ways to perform operations that would otherwise require manual iteration and temporary variables. By harnessing their power, you can streamline your JavaScript code, making it more readable and easier to debug. It's not just about understanding what each method does, but using them together to write effective JavaScript code. Harnessing these methods can significantly enhance your problem-solving capabilities in JavaScript.
Javascript Array Methods - Key takeaways
- Javascript arrays allow for the storage and access of multiple data pieces in a single variable.
- Javascript array methods, such as .push(), .pop(), .shift(), .unshift(), .slice(), .splice(), .concat(), .join(), and .reverse() aid in different actions such as transformation, iteration, joining, slicing and reversing of arrays.
- Array methods in Javascript play a significant role in managing and manipulating data, such as adding, updating or deleting elements from arrays.
- Practical applications of Javascript Array Methods can vary widely, from simple manipulations of data within an array to complex operations involving intricate computational logic.
- Understand the Concept of Array Methods in JavaScript: Array methods are predefined functions that allow programmers to perform a wide variety of tasks on arrays, from adding and removing elements to merging arrays and more.
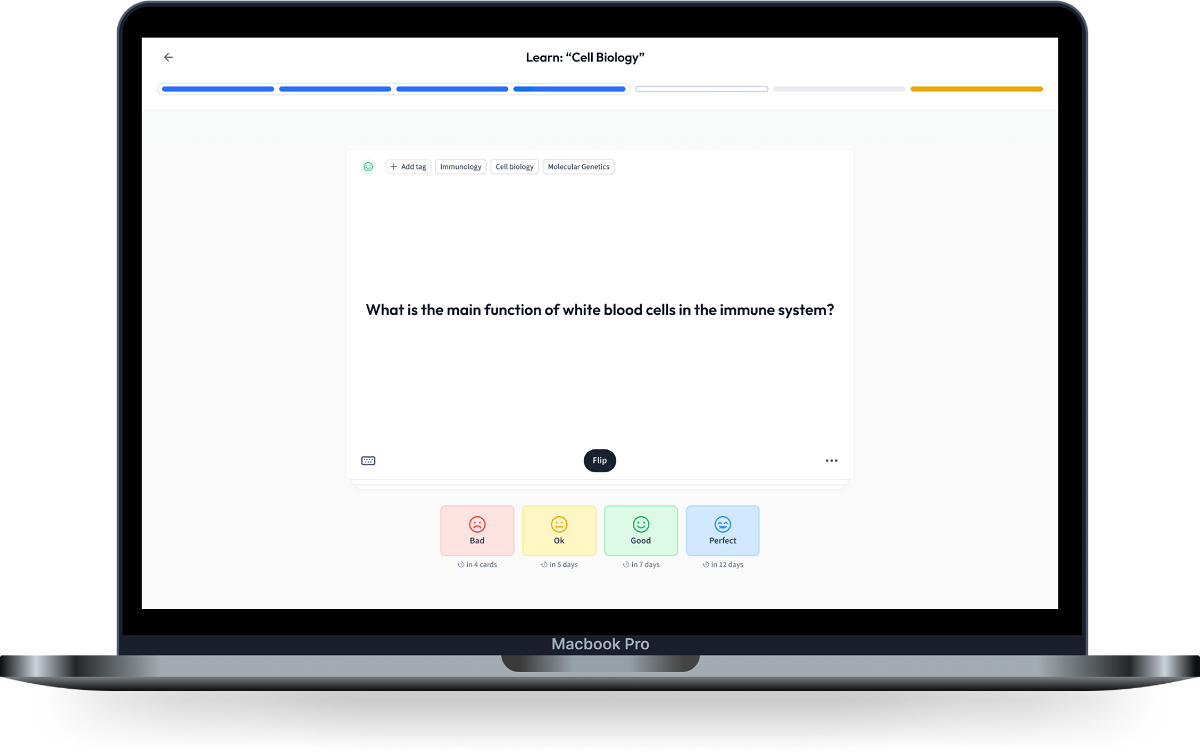
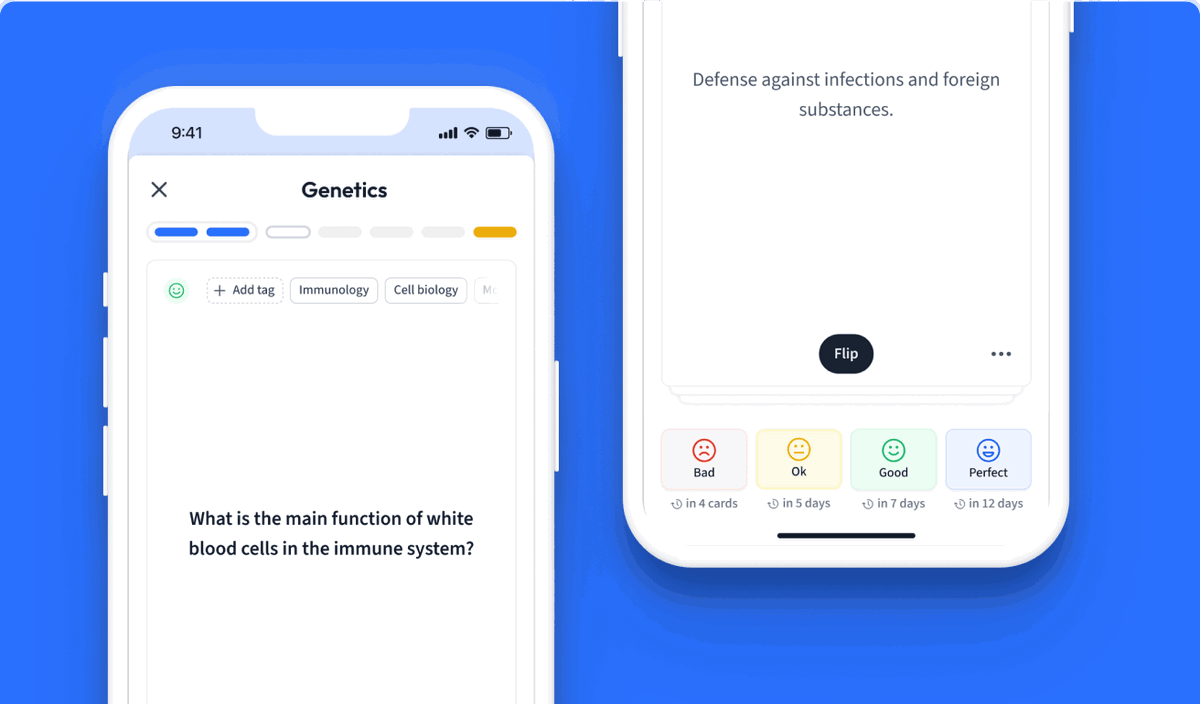
Learn with 12 Javascript Array Methods flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript Array Methods
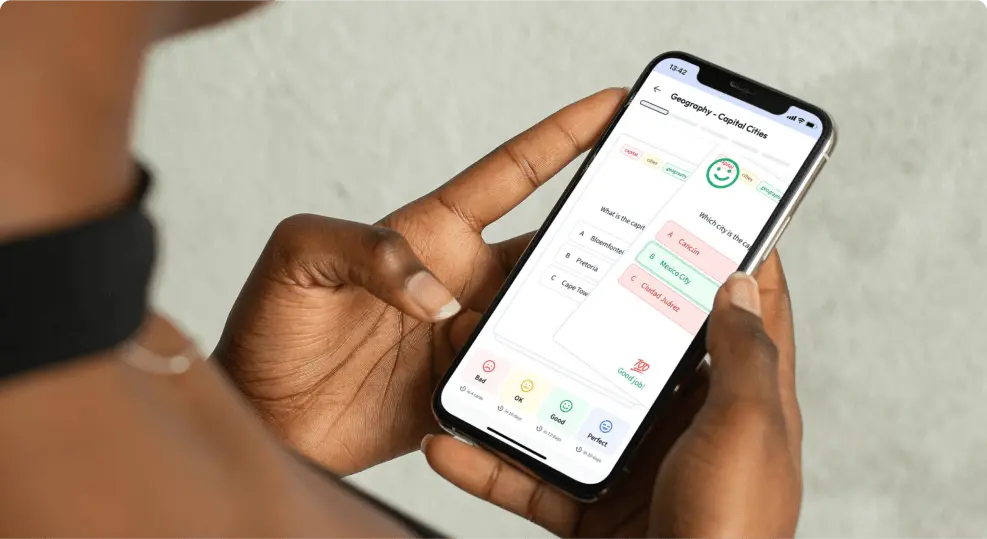
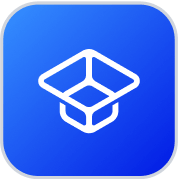
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more