Understanding Javascript Reference Data Types
When you work with Javascript, you will often encounter various kinds of data types. There are primarily two kinds of data types in JavaScript - primitive and reference. Today, you'll gain an in-depth understanding about what Javascript reference data types are, their essential characteristics and how they work.
Simplified Definition of Javascript Reference Data Types
Javascript Reference Data Types, also known as complex data types, are essentially variables that store a reference or a pointer to the location of the value instead of storing the actual value itself. They can be any data structure like an array, object, or function. In contrast to the immutable nature of primitive data types, reference data types are mutable and can be altered once created.
Essential Characteristics of Javascript Reference Data Types
Javascript reference data types exhibit several critical characteristics:
- They are mutable.
- The variable does not hold the actual value, instead, it holds a reference to the value.
- They are compared by reference, not by actual value.
How Javascript Reference Data Types Work?
To understand how reference data types function, you need to grasp the relationship between the variable and the value it refers to. When you create a complex data type variable, the value gets stored in the heap, and a reference or pointer to this location gets saved in the variable. Any changes you make are directly applied to the value in the heap, not the variable.
For instance, consider an array - another prominent type of reference data type. When you create an array, the array's values get stored in memory and the reference to this memory location is what the variable will hold. So if you have an array like var fruit = ['apple', 'banana', 'cherry'], the variable 'fruit' does not exactly hold the array. It holds a link to the location of the array in memory. Hence, when you make changes to the array, you are directly modifying the values in the memory, not the 'fruit' variable.
A fascinating aspect of javascript reference data types is what happens when one variable is assigned to another. The new variable does not get its own copy of the value. Instead, both variables now have a reference to the same value. Thus, changes made through one variable would reflect in the other as both are merely different pointers to the same location in memory.
Primitive versus Reference Data Types in Javascript
An understanding of Primitive and Reference data types forms an essential component of your Javascript knowledge base. These two principal data types exhibit key differences that crucially affect how you write and optimise your code.
Fundamental Difference between Primitive and Reference Data Types in Javascript
The main difference between primitive and reference data types lies in how Javascript handles them. In a nutshell, primitive data types store the actual data value, while reference data types store a reference (or a pointer), not the actual data value.
In the case of primitive data types, the variable holds the actual data. These data types include data types like Boolean, null, undefined, String, and Number. Being immutable, any operation upon these types results in a new value, leaving the original untouched.
var x = 10; var y = x; y = 20; console.log(x); // Output: 10 console.log(y); // Output: 20
In the above example, when you assign the value of x to y, a fresh copy of x's value is created and assigned to y. Consequently, any changes made to y do not affect x.
On the other hand, with reference data types, the variable does not hold the actual value but a reference to the value’s location in memory. The most common reference data types are objects, arrays, and functions.
var x = {value: 10}; var y = x; y.value = 20; console.log(x.value); // Output: 20 console.log(y.value); // Output: 20
Differentiating from primitive data types, see that when you assign the value of x to y, y does not get a fresh copy of x's value. Instead, y points to the same memory location as x. This location carries the object. Therefore, a change in y.value, is directly reflected in the original object, thus both x.value and y.value output the same value (20).
Examples Illustrating Differences between Primitive and Reference Data Types in Javascript
To further comprehend these differences, let’s delve into detailed examples:
Primitive Data Type Example:
var string1 = "Hello!"; var string2 = string1; string2 = "Goodbye!"; console.log(string1); // Output: "Hello!" console.log(string2); // Output: "Goodbye!"
In this example, you'll notice that changing the value of string2 does not affect string1. This is because they both hold separate copies of the value - a specific exclusive trait to primitive data types.
Reference Data Type Example:
var array1 = [1, 2, 3]; var array2 = array1; array2.push(4); console.log(array1); // Output: [1, 2, 3, 4] console.log(array2); // Output: [1, 2, 3, 4]
Contrarily, in this example, array1 and array2 are storing a reference to the same array. Hence, modifications made via one reference (like array2.push(4)), are visible when accessed via the other reference (array1).
The stark difference in the behaviour of primitive and reference data types in Javascript, whether it is storage or handling, makes it essential for you, as a developer, to understand these fundamental Javascript concepts.
Practical Examples of Javascript Reference Data Types
Reference data types encompass arrays, functions, and objects in JavaScript. They are extremely dynamic and versatile, accommodating a plethora of programming needs. Below, you'll explore some practical examples that bolster the understanding of these reference data types.
Common Examples of Javascript Reference Data Types
The most commonly encountered Javascript reference data types are arrays, objects and functions. Their dynamism and utility make them indispensable tools for your programming pursuits.
ArraysArrays are an ordered list of values specially designed to store multiple values in a single variable. In Javascript, arrays are versatile, capable of storing different data types, including strings, numbers, objects, and even other arrays.
Here is an example of how you can create an array and manipulate it:
var colors = ["Red", "Blue", "Green"]; console.log(colors[0]); // Output: Red colors.push("Yellow"); console.log(colors); // Output: ["Red", "Blue", "Green", "Yellow"]
The beauty of arrays as a reference data type is in managing collections of values, making it a go-to data type for storing sequences or ordered lists.
ObjectsIn Javascript, practically everything can be an object. However, an object as a reference data type signifies a standalone entity with properties and types. Objects encapsulate related data and functions as properties and methods.
Consider an example where we create an object to represent a car:
var car = { brand: "Tesla", model: "Model X", year: 2021, getDetails: function() { return this.brand + " " + this.model + ", " + this.year; } }; console.log(car.getDetails()); // Output: Tesla Model X, 2021
Objects bring an added dimension of organising code in terms of real-world entities, providing a layer of abstraction that makes coding more intuitive and understandable.
FunctionsFunctions in Javascript serve as procedures, a set of statements that performs a task or calculates a value. They are certainly one of the most flexible features of Javascript, adopting the role of a reference data type in specific scenarios.
Below is an example of a function that adds two numbers:
var addNumbers = function(num1, num2) { return num1 + num2; }; console.log(addNumbers(5, 10)); // Output: 15
Functions encapsulate code for a specific task into reusable blocks, making them key players in building modular and maintainable code.
Understanding Javascript Reference Data Types Through Examples
To further illustrate how Javascript reference data types work, you can explore how these data types are manipulated in the code. Since reference data types are mutable, they can be altered even after their creation. Additionally, multiple variables can reference the same object. This behaviour contrasts sharply with primitive data types.
Let's consider an example:
var object1 = {property: "original value"}; var object2 = object1; // object2 now references the same object object2.property = "new value"; console.log(object1.property); // Output: "new value"
In the example above, object2 was assigned the value of object1, and subsequently, a change was made to object2. Contrary to primitive data types, where changes to one variable do not affect the other, here the changes made to object2.property also affected object1.property. This is because both object1 and object2 were pointing to the same object in memory.
Conclusively, an understanding of Javascript reference data types is pivotal to not just building applications in Javascript but ensuring efficiency and maintainability of code.
Techniques in Javascript for Using Reference Data Types
Once firmly grasped, Javascript reference data types can provide powerful tools for creating vibrant, interactive and dynamic web applications. Leveraging these data types effectively necessitates a comprehension of various underlying techniques. From basic manipulations to advanced operations, these techniques can guide you to formulate cleaner, more intuitive and efficient code.
Basic Techniques for Handling Javascript Reference Data Types
To utilise Javascript reference data types effectively, it's essential to familiarise yourself with some basic, yet powerful techniques. Whether it's creating and manipulating arrays, making use of key-value pairs in objects, or defining and invoking functions, these elementary techniques lay the groundwork for a solid understanding of reference data types.
Working with Arrays
Arrays in Javascript present a flexible way to store, retrieve and manipulate collections of values. Once you've initiated an array, you can push and pop values, access elements by their indices, and use in-built Javascript methods to transform the array to your liking.
Consider the following code snippet, which demonstrates creation, manipulation, and traversal of an array:
var fruits = ["Apple", "Banana", "Orange"]; console.log(fruits.length); // Output: 3 fruits.push("Mango"); console.log(fruits); // Output: ["Apple", "Banana", "Orange", "Mango"] fruits.forEach(function(item, index, array) { console.log(item, index); }); // Output: Apple 0 // Banana 1 // Orange 2 // Mango 3
Working with Objects
Javascript objects follow the concept of key-value pairs, emulating real-world entities. Basics of Javascript objects involve creating an object, defining and accessing properties, and the use of methods.
Below is an example showcasing the basic use of Javascript objects:
var student = { name: "John", age: 16, grade: "10th grade", displayDetails: function() { console.log(this.name + " is " + this.age + " years old and in " + this.grade); } }; student.displayDetails(); // Output: John is 16 years old and in 10th grade
Working with Functions
Functions in Javascript represent a set of statements designed to perform a particular task. At the basic level, it includes defining functions, passing parameters to functions, and invoking them.
The following example depicts basic usage of Javascript functions:
function greet(name, greeting) { console.log(greeting + ", " + name + "!"); } greet("John", "Hello"); // Output: Hello, John!
Advanced Techniques in Javascript for Using Reference Data Types
On progressing beyond the basics, your toolkit for handling Javascript reference data types can expand immensely. With advanced techniques, you're able to implement complex functionality, improve code maintainability and even enhance performance. Advanced techniques involve understanding concepts like higher-order functions, prototypal inheritance, and deep and shallow copying of objects and arrays.
Higher-Order Functions and Callbacks
A profound strength of JavaScript lies in the treatment of functions as first-class citizens. This empowers the concept of higher-order functions, which are functions that accept other functions as arguments and/or return functions as a result. It is a powerful tool for creating more abstract and maintainable code.
Let's consider an example where an array filter method (a higher-order function) is used to filter out even numbers from an array:
var numbers = [1, 2, 3, 4, 5, 6]; var evenNumbers = numbers.filter(function(num) { return num % 2 == 0; }); console.log(evenNumbers); // Output: [2, 4, 6]
Prototypal Inheritance
Javascript follows a prototypal inheritance model. In this model, an object can inherit properties from another object, known as the prototype. By doing so, it fosters code reuse and object-oriented programming in Javascript.
This example illustrates prototypal inheritance. Here, a Dog object inherits the properties of an Animal object:
function Animal (name) { this.name = name; } Animal.prototype.speak = function () { console.log(this.name + ' makes a noise.'); }; function Dog (name) { Animal.call(this, name); } Dog.prototype = Object.create(Animal.prototype); Dog.prototype.constructor = Dog; Dog.prototype.speak = function () { console.log(this.name + ' barks.'); }; var dog = new Dog('Rover'); dog.speak(); // Output: Rover barks.
Deep and Shallow Copy of Reference Data Types
Because of the reference nature of objects and arrays, it's often necessary to create a full independent copy of an object or an array. Shallow copies only duplicate the top-level properties, whereas a deep copy duplicates all nested levels. Deep and shallow copying techniques are crucial when you want to prevent modifications made to the copy from affecting the original data.
Here is an example using Javascript’s Object.assign() method to create a shallow copy of an object:
var obj1 = { property1: "value1", property2: "value2" }; var obj2 = Object.assign({}, obj1); console.log(obj2); // Output: { property1: 'value1', property2: 'value2' }
Mastery over these advanced techniques can greatly enhance your code structure, readability, modularity and even performance, consequently driving you toward becoming a skilled Javascript developer.
Utilisation of Javascript Reference Data Types
Mastering Javascript reference data types is an indispensable stride towards proficient programming. They facilitate dynamic data structures and functions, effortlessly accommodating diverse programming necessities. Applying them aptly guarantees cleaner, intuitive, and maintainable code, offering an edge particularly in complex applications.
Practical Use of Javascript Reference Data Types in Computer Programming
Javascript reference data types boast various applications; arrays, functions, and objects have diverse uses, each fitting distinct scenarios perfectly. Specifically, arrays are quintessential when handling sequences or ordered lists, whereas objects cater to the need for organising code in terms of real-world entities. Furthermore, the usage of functions encapsulates code for a particular task into reusable blocks, ultimately aiding the building of modular and maintainable code.
A typical application of arrays is when you need to record data over a period of time. For instance, if tracking monthly rainfall in a year, an array neatly packages this information.
var rainfall = [70, 50, 100, 75, 60, 45, 80, 90, 85, 70, 55, 100]; // Recorded rainfall for each month in a year
Objects are incredibly beneficial when representing entities. If developing a student management system, they're ideal for maintaining student records:
var student = { name: "Sam", age: 16, class: "10th", subjects: ["English", "Maths", "Science"] };
Did you know? The reference aspect of these data types comes from how they handle memory. While primitive data types like numbers and strings are stored directly in the location that the variable accesses, reference data types are stored in the heap, and the variable merely holds the address (reference) where the data is stored. It’s this reference handling that makes these data types as powerful and dynamic as they are.
Functions, being self-contained modules of code built for specific tasks, offer numerous uses. Suppose you are repeatedly calculating the area of circles of different radii throughout code; defining it as a function simplifies the process:
var calculateArea = function(radius) { return Math.PI * Math.pow(radius, 2); // Formula for calculating the area of a circle };
Benefits and Drawbacks of Using Javascript Reference Data Types
Delving into the merits and pitfalls of reference data types keeps you acquainted with their implications, fostering informed choices that endorse efficient code design. Here, we dissect some notable benefits and drawbacks of employing Javascript reference data types.
Benefits:
- Greater Flexibility: Arrays can store multiple data types, objects can encapsulate properties and methods, and functions encapsulate task-specific code.
- Modular Code: Functions, in particular, allow for a modular approach to coding, breaking down complex tasks into manageable units. Likewise, object-oriented programming features of Javascript lets developers break down complex applications into simpler objects.
- Memory Efficient: With reference types, changes to an object or array can be made in one place and reflected elsewhere where that object or array is referenced, making them memory efficient.
Did you know? Using these reference types appropriately has a significant bearing on your code’s efficiency. For instance, since objects and arrays are mutable (can be altered after creation), changes made to one variable can potentially affect other variables pointing to the same data. On the one hand, this is useful when you want changes to propagate throughout your code; on the other hand, it can lead to errors if not handled carefully.
Drawbacks:
- Unexpected Behaviour: Due to their mutable nature, changes to reference types can lead to unintended side effects if not managed correctly.
- Memory Cleanup: Accessing memory through reference types can make memory cleanup more complex, potentially leading to memory leaks.
- Debugging Difficulty: While the ability of reference types to hold different data types is a strength, it can also introduce bugs that are tricky to pinpoint and fix.
From the above exploration, it’s evident that while Javascript reference data types contribute immensely to coding flexibility, one must be aware of their drawbacks. A thorough understanding of their capabilities and limitations aids in navigating the challenges they may present.
Javascript Reference Data Types - Key takeaways
- Primitive data types in Javascript store the actual data value, while reference data types store a reference or a pointer, not the actual data value.
- Primitive data types include Boolean, null, undefined, String, and Number. When actions are performed on these types, a new value is created, and the original remains untouched.
- Javascript reference data types encompass arrays, functions, and objects. These types are mutable and are stored by the variable as a reference to the value’s location in memory-
- Techniques in Javascript for using reference data types range from fundamental manipulation to advanced operations like higher-order functions, prototypal inheritance, and deep and shallow copying of objects and arrays.
- Javascript reference data types can be utilised for creating dynamic web applications, improving code maintainability, and implementing complex functionality. Examples include, managing collections of values and building modular and maintainable code.
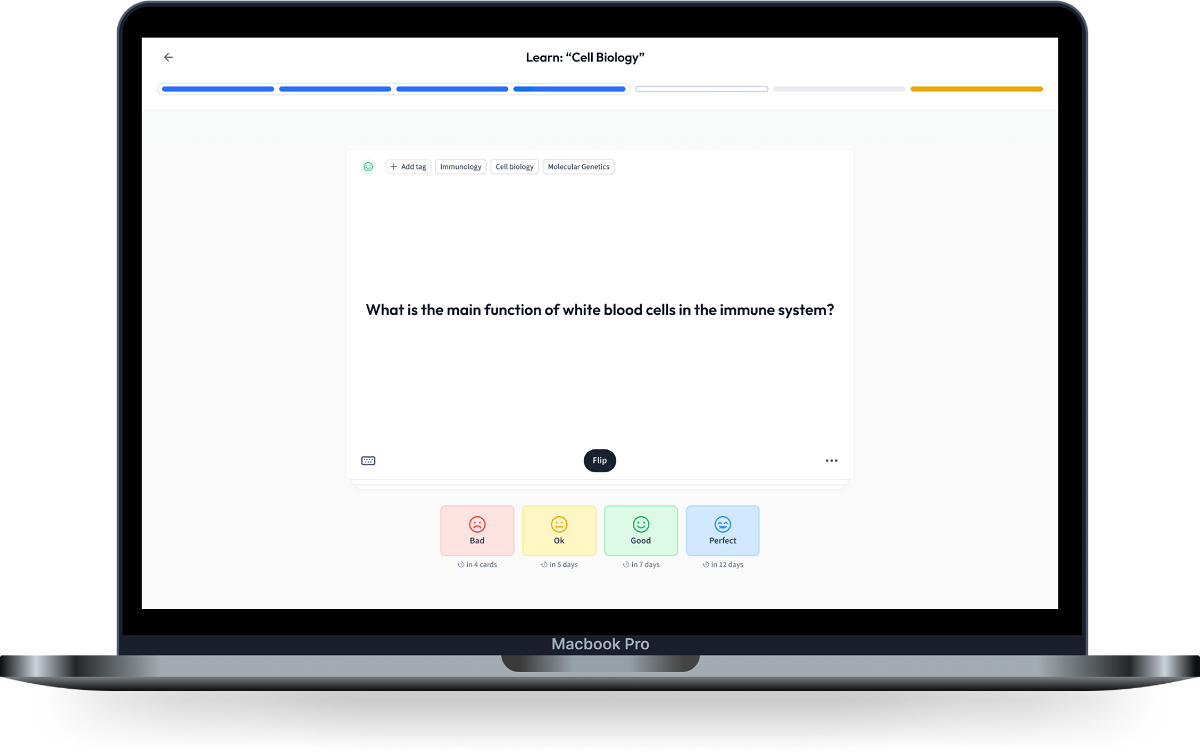
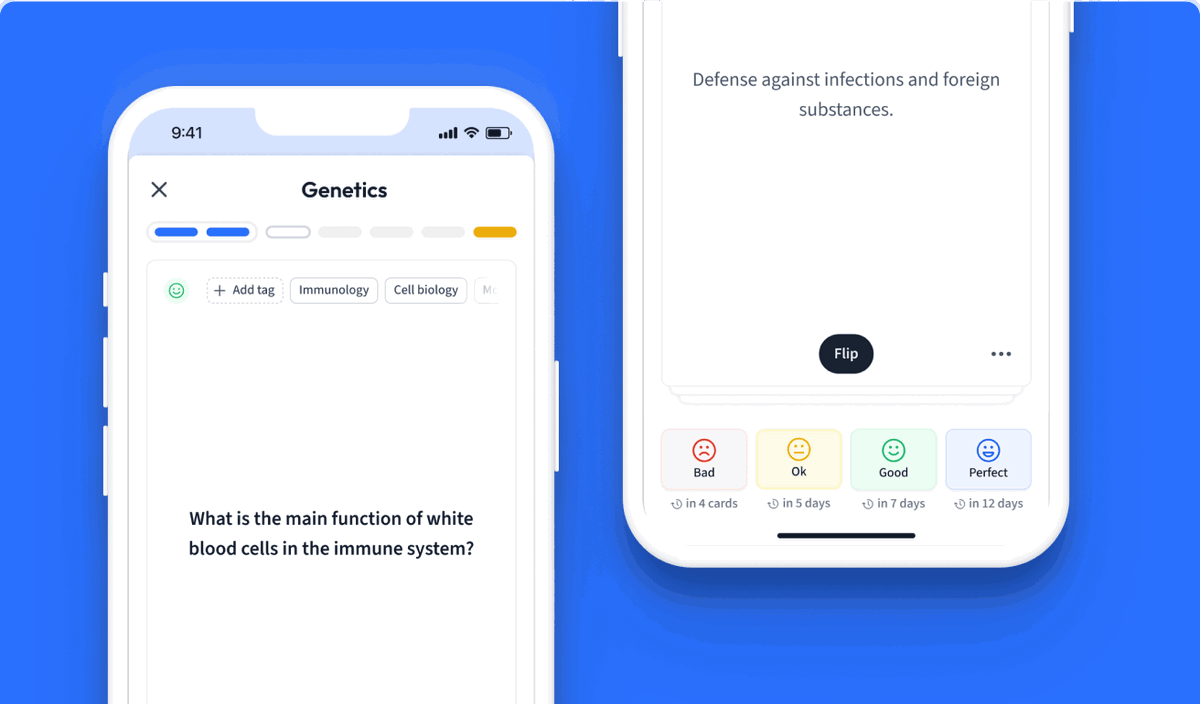
Learn with 15 Javascript Reference Data Types flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript Reference Data Types
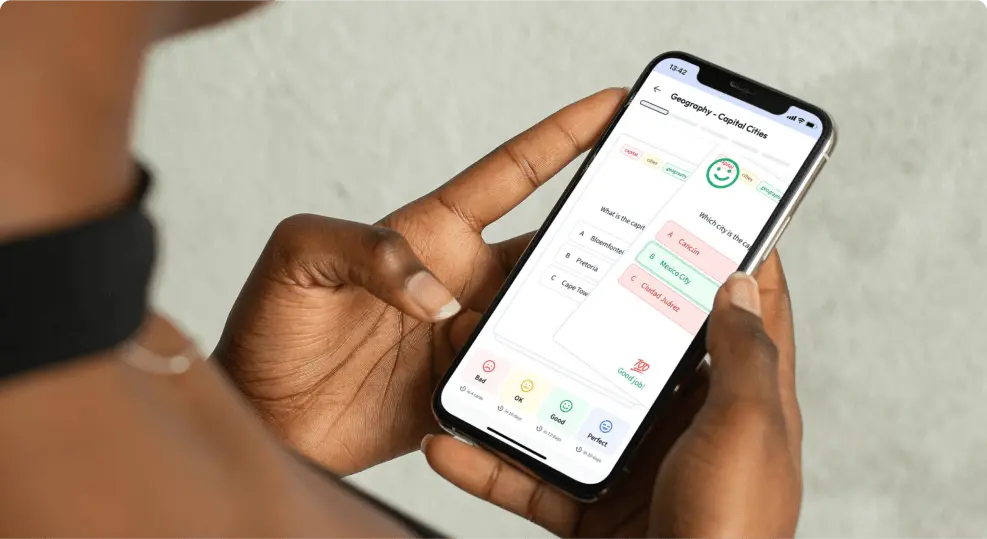
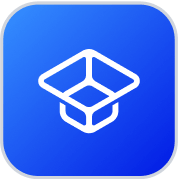
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more