Understanding the Programming Language PHP
PHP stands for Hypertext Preprocessor, a ‘server-side’ scripting language predominantly used for web development but can also be utilised for other applications. Server-side means its scripts are processed on the server as opposed to the user's browser.
What is PHP Programming Language: Definition and Overview
PHP's ability to embed right into HTML code makes it a practical and efficient programming language for web development. In a basic yet illustrative example, here's how PHP would be used in an HTML document:Write Your First PHP Script
In this code, "lt;" and "gt;" are used to replace the "<" and ">" characters commonly used in HTML and PHP scripts. This avoids any unintended formatting or execution.
The Roots and Evolution of PHP Programming Language
PHP was initially created by Rasmus Lerdorf in 1994. At that time, it was a simple set of Common Gateway Interface binaries written in C. As it evolved, it became PHP/FI (PHP/Form Interpreter). The key turning point came in 1997 when two programmers, Zeev Suraski and Andi Gutmans, rewrote the parser to create PHP 3. This significant leap pushed PHP into the programming landscape. Following the journey of PHP, let's break down some crucial versions:
PHP 4.0 | Introduced in May 2000 |
PHP 5.0 | Debuted in July 2004 with a new model for developers to use for object-oriented programming |
PHP 7.0 | Launched in December 2015, it brought significant performance enhancements compared to PHP 5 |
PHP 8.0 | Released on November 26, 2020, it brought many optimisations including named arguments, union types, attributes, etc |
PHP changed direction after version 5, focusing more on enhancing performance and fixing bugs, which resulted in the release of PHP 7. This version showed up to twice the speed of a PHP 5 application for real-world apps and up to three times for synthetic benchmarks.
Importance and Popularity of PHP in Programming Landscape
PHP still retains high popularity in the programming world due to several reasons:- It’s open-source and free, reducing development costs substantially.
- PHP boasts of a vast community of users providing a robust support network.
- Continuous updates keep PHP relevant, competitive, and highly effective at handling various web development tasks.
- It conveniently integrates with various databases like MySQL, Oracle, and MS Access, among others.
PHP Programming Language Structure
Understanding the structure of the Programming Language PHP can greatly enhance your learning experience. Grasping the intricacies of its syntax and conventions allows for a smoother navigation through the world of PHP.Introduction to PHP Language Structure
The structure of PHP is designed to be simple, flexible, and effective. It works in perfect harmony with HTML and allows for extensive server-side scripting. PHP code is enclosed within special script tags, that allow the code to be processed by a PHP interpreter. PHP can be embedded directly into an HTML file, and the server will process the PHP code before the HTML is sent to the user's browser. In essence, this means that your PHP scripts can be added right where you want them to output data. For instance, if you want to display the current date within an HTML paragraph, your PHP code would go inside the paragraph tags.In this snippet, the date function outputs the current date in 'Y-m-d' format.
PHP Syntax: Basics to Advanced Concepts
The basic structure of a PHP command includes a function, an action, and a semicolon to signify the end of a command. When you build functions, you will use parentheses and parameters. Let's consider the following code:In this case, 'echo' is a PHP function, which instructs the server to output or 'echo' back whatever follows. 'Hello, World!' is the action to be performed, and the semicolon marks the end of the function. As a PHP script grows, understanding advanced concepts such as loops, arrays, and objects becomes vital. For instance, a for loop offers a concise way to perform the same task multiple times—perfect for working with arrays.
This for loop uses initialisation (\(i=0\), run once at the start), a condition (\(i<10\), checked before every loop iteration), and an updater (\(i++\), run after every loop iteration).
PHP Coding Conventions and Standards
Consistency is key in coding, and there are certain conventions and standards developers should strive to follow to maintain understandable, clean code. Some of these conventions include:- Naming Conventions: Be clear, meaningful, and concise. Variable and function names should be in camelCase while Class names should be CapitalisedCamelCase.
- Indentation: Use an indent of 4 spaces, with no usage of tabs.
- Braces: Opening braces for classes and methods must go on the next line, and closing braces must go on the next line after the body.
- Comments: Add comments and document your code as necessary to articulate the functionality and flow to other developers and your future self.
//Correct class SampleClass { public function sampleFunction() { if (condition) { // code } } }Following these conventions and standards can help not only in team collaborations but also when you have to revisit your own code after some time. Remember, programming is an art where beauty lies in clarity and neatness.
Practical Use of PHP Programming Language: Examples and Tips
PHP has an extensive practical use in various aspects of web development. From the simplest tasks of sending emails to creating dynamic web page content, PHP is a useful tool to have. The practicality of PHP is shown in several examples that illustrate its capabilities and range.PHP Programming Language Examples with Explanations
The power of PHP is in its ability to interact with servers, create dynamic content, and accept user input. To help illustrate these capabilities, let's explore some common PHP examples and what they do. Here's a simple PHP script that echoes a string onto the page:In this script, 'echo' is the PHP command to output the string "Hello, World!". It's similar to 'printf' in C, or 'Console.WriteLine()' in C#. Another common use of PHP is sending an email:
In this script, the 'mail' function sends an email. The variables specify the email components: 'to' is the recipient's email, 'subject' is the subject line, 'message' is the message body, and 'headers' is optional additional headers.
PHP Code Snippets and What They Do
Let's dive a bit deeper and look at more complex code snippets. Here's an example of a PHP script that uses a conditional statement - an if-else statement:In this script, 'date("H")' returns the current hour. The if-else statement then outputs "Good morning!" if the current hour is less than 12, and "Good afternoon" if not.
How to Improve Your PHP Coding Skills
With a basic understanding of PHP, it's key to constantly improve your skills for better coding and handling more complex projects. Below are some tips to help you on the journey:- Practice: As with any programming language, practice is an essential part of learning. Write code daily, start projects, and solve problems.
- Read and Understand Code: Trying to understand code written by others can provide valuable insights into different coding styles and methods.
- Learn Online: There are various online platforms offering PHP courses for free or at an affordable cost. Use them to learn new concepts and enhance your PHP skills.
- Join a Coding Community: Sharing your challenges with others and learning from their experiences can be enormously beneficial. Stack Overflow and GitHub are excellent platforms to start with.
- Utilise Built-In Functions: PHP has many built-in functions, understanding them can save time and reduce the complexity of your coding.
- Debug: Debug your PHP code regularly. This will help you understand the flow of your code and identify and correct errors faster.
Exploring PHP Programming Language Applications
When delving into the world of PHP, you'll soon realise its broad applications across various domains. From creating complex web applications to simple marketing websites, PHP repeatedly proves its versatility. Let's take a closer look at its diverse applications and understand how PHP contributes to the programming world.Wide Range of PHP Programming Language Applications
PHP has a wide application spectrum, with its ability to seamlessly interact with databases and provide user input functionality being some of the key features that set it apart. One significant application is the development of Content Management Systems (CMS). CMSs lie at the heart of dynamic websites, and PHP’s extensive database interfacing abilities render it ideal for these kinds of applications. WordPress, Joomla, and Drupal are shining examples of CMSs developed in PHP. Another noteworthy use of PHP is in the development of e-commerce platforms. It can handle sessions, maintain carts, process payments, and do much more. Major e-commerce platforms like Magento utilise PHP. PHP also excels in the creation of Social Networking Interfaces. Technological giants like Facebook have made use of PHP's capabilities for this purpose. Moreover, PHP can also be employed in the creation of PDF documents on the server-side, allowing dynamic generation of printable formats and reports. Lastly, PHP is also a popular tool for updating and modifying databases. Its diverse database support and easy connectivity enhance its capabilities for backend databasing tasks.PHP in Web Development: Usage and Examples
Web development is where PHP truly shines. Its ability to generate dynamic content, access cookies, and manage databases makes PHP an integral part of many web development projects. For instance, PHP is extensively used to create dynamic web page content. Here is an example of PHP used to generate a simple introductory greeting based on the time of the day:Another prevalent use of PHP in web development is form data collection. PHP can handle data submitted by HTML forms and process the collected information securely.
In this code snippet, the '$_POST' superglobal array is used to collect form data. The array elements correspond to the 'name' attributes specified in the form's HTML.
The Role of PHP in Software Development
Beyond the realm of web development, PHP has a significant presence in traditional software development as well. PHP's command-line interface (CLI) enables developers to write standalone software applications. A comprehensive example of PHP's application in software development is its usage in creating graphical user interface (GUI) applications. It works in conjunction with PHP-GTK, a PHP extension that provides an object-oriented interface to GTK+ classes and functions. Furthermore, PHP supports database management across various platforms, making it an excellent choice for software requiring intensive database interaction. Though PHP is primarily a server-side language, its diverse applications, ranging from CMSs to standalone software applications, display its adaptability and power, making it an essential tool in any developer's toolkit.PHP and Future Developments
PHP, despite some low points, has managed to stay relevant in the fast-evolving software development landscape. Its continuous evolution to adapt to modern programming paradigms is testament to its adaptability and resilience.Upcoming Enhancements in PHP Programming Language
Looking into the future, PHP isn't settling down. Several enhancements and additions, designed to increase efficiency and interoperability, are on the horizon. One primary update is the Just-In-Time (JIT) Compiler that was introduced in PHP 8.0. The JIT compiler, in essence, converts parts of the byte code which are executed frequently into machine language instructions. Theoretically, this has the potential to make PHP significantly faster. However, the extent of these performance benefits in real-world applications is a topic of discussion and experimentation. Secondly, the introduction of Attributes (also called annotations) is another significant feature. Attributes provide a way to add meta-data to classes, methods, and functions, which wasn't natively possible in PHP before. They are commonly used in other popular programming languages such as C# and Java, and promise to bring PHP closer to these in terms of capabilities.#[Api\Controller] class MyClass { #[Api\Annotations\Route("/api/v1/my_endpoint")] #[Api\Annotations\Json] public function myFunction(){ // Function implementation } }In addition to these, several enhancements to existing functionalities such as Named Arguments, Union Types, and Match Expressions provide developers with more tools to write cleaner and more robust code. Consistent Type Errors are another small yet significant change being made to the language. In PHP 8, internal functions will throw an \(\text{Error}\) exception if a parameter of the wrong type is passed instead of emitting a warning and returning null. This makes the behaviour of built-in functions consistent with userland functions.
PHP: Paving the Way for Future Programmers
Aside from the continuous development of the language, PHP also plays a profound role in shaping the future crop of programmers. Due to its balance of simplicity and flexibility, PHP often serves as a starting point into the world of backend programming for many budding developers. The wide adoption of PHP, especially in web development, ensures that PHP skills remain in high demand, encouraging future generations of programmers to master it. As a bonus, proficiency in PHP translates well into other server-side programming languages. Another essential aspect is PHP's open-source nature. Open-source tools are inexhaustible learning resources for programmers. From understanding the language's architecture to contributing to its development, being able to access PHP's source code provides countless opportunities for learning. Equally important are PHP's vast community and extensive documentation. The supportive community provides an excellent platform for developers to learn, ask questions, and resolve their issues. Moreover, PHP's interoperability with front-end technologies like HTML, CSS, and JavaScript makes it an attractive choice for developers seeking to master the full stack. Understanding the interactions between client-side and server-side technologies is an essential skill for any web developer, and PHP is a great place to start. In conclusion, while PHP continues to evolve with new improvements and innovative features, it also provides an invaluable foundation for future programmers with its simplicity, open-source nature, and strong community support. Strong PHP skills help build a strong foundation for other server-side programming languages and aid in creating well-rounded programmers capable of tackling a broad spectrum of web development projects.Programming Language PHP - Key takeaways
- PHP 3 was a significant leap in the evolution of the PHP language which pushed PHP into the mainstream of programming.
- PHP 4.0 was introduced in May 2000, PHP 5.0 debuted in July 2004, PHP 7.0 was launched in December 2015, and PHP 8.0 was released on November 26, 2020, each with its unique features and enhancements.
- PHP is popular for its open-source nature, extensive community support, continuous updates, and easy integration with various databases.
- The structure of PHP programming language is designed to work seamlessly with HTML and allows for comprehensive server-side scripting.
- PHP programming syntax involves structures such as loops, arrays, and objects, which are essential as a PHP script grows.
- PHP language structure allows for consistent coding conventions and standards such as clear naming, proper indentation, and apt use of braces and comments for clean and understandable code.
- PHP programming language examples include scripts for outputting strings, sending emails, performing conditional statements, and more.
- PHP programming language applications involve a wide range, including the development of Content Management Systems, e-commerce platforms, Social Networking Interfaces, updating databases, and more.
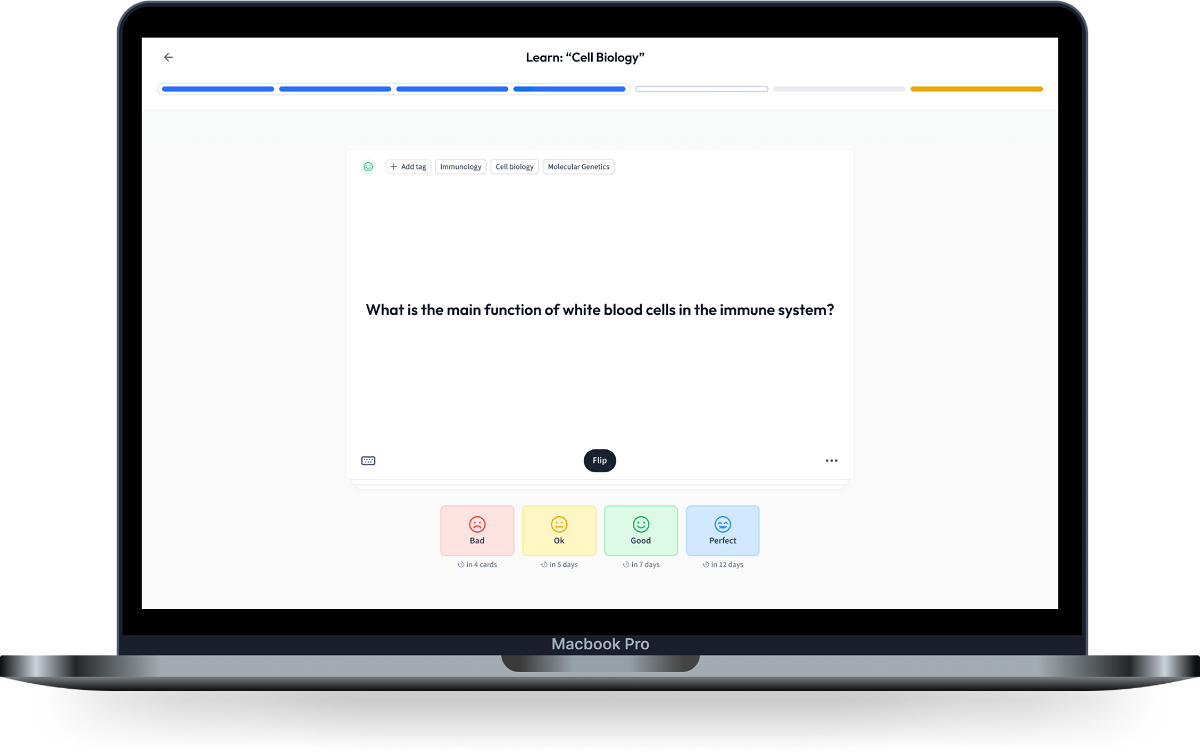
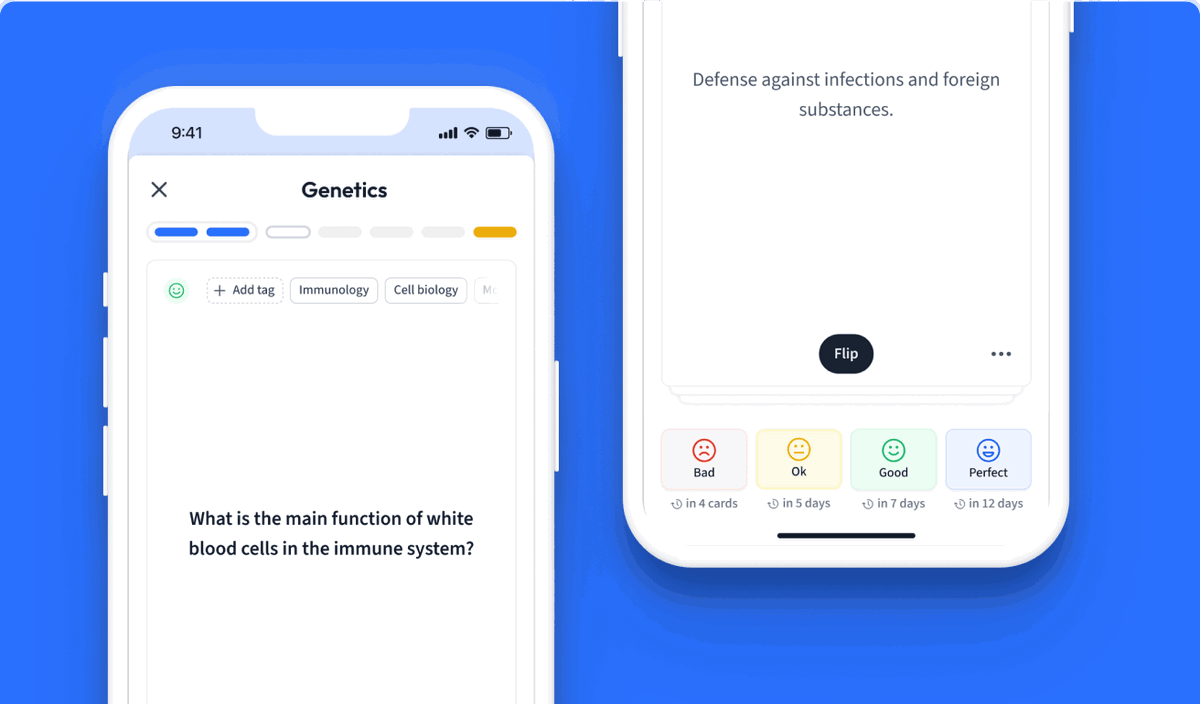
Learn with 15 Programming Language PHP flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Programming Language PHP
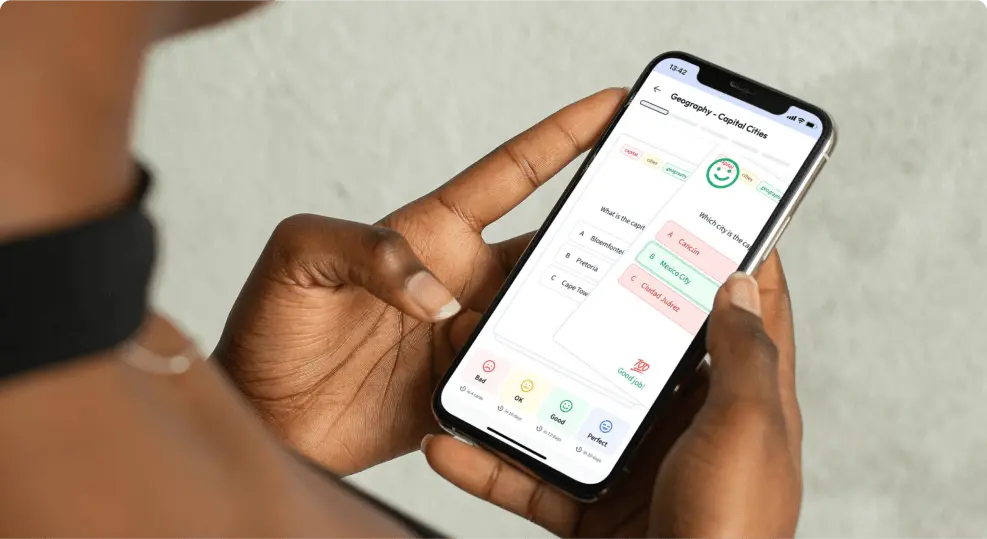
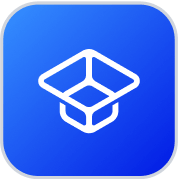
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more