Understanding JavaScript Comparison Operators
JavaScript, being one of the most robust and dynamic programming languages, employs several techniques, tools, and concepts to aid effective programming.One of such tools are the JavaScript Comparison Operators.
What are JavaScript Comparison Operators?
JavaScript comparison operators create a logical comparison between values, presenting a clear and detailed manner of investigating and comparing data. By comparing different data variables, these operators can affect control flow and outcomes in code logic. Most crucially, JavaScript comparison operators return a boolean value: either true or false. This truthy or falsy nature is what makes them invaluable for setting conditions in JavaScript code.For example, if you are writing a JavaScript code to compare the ages of students and need to know who is older, you would make use of the Greater Than and Less Than JavaScript comparison operators.
Different Types of JavaScript Comparison Operators
There are several types of JavaScript comparison operators which, when mastered, can greatly enhance the versatility of your coding. 1. Equality (==) 2. Inequality (!=) 3. Strict Equality (===) 4. Strict Inequality (!==) 5. Greater Than (>) 6. Less Than (<) 7. Greater Than or Equal To (>=) 8. Less Than or Equal To (<=)Something interesting to note is that JavaScript has both equality and strict equality operators. The former checks if two values are equivalent after type coercion while the latter checks if they are equivalent without type coercion.
Equality and Inequality in JavaScript Comparison Operators
Equality (==) and inequality (!=) are fundamental JavaScript comparison operators.Equality (==) checks whether two values are equivalent after type coercion, meaning it will consider 5 and '5' as equal because it coerces the string '5' to a number which would give us the number 5.
5 == '5' // returns true
On the contrary, inequality (!=) verifies if two values are not equivalent after type coercion.
5 != '5' // returns false
Relational: Greater than, Less than, with JavaScript Comparison Operators
Greater than (>) and less than (<) operators compare the relative size or order of two values.The greater than operator (>) returns true if the value on the left is greater than the value on the right.
6 > 5 // returns true
Conversely, the less than operator (<) returns true if the value on the left is smaller than the value on the right.
5 < 6 // returns trueAs seen from these examples, comparison operators are fundamental for directing logical flow within JavaScript code, and they enhance the functionality and versatility of the language considerably.
JavaScript Comparison and Logical Operators in Action
To fully comprehend JavaScript Comparison Operators, you need to understand how to use them efficiently. Your journey into mastering JavaScript would be incomplete without knowing how these operators interact and carry out their operations. Interestingly, you can combine these operators, increasing your control over your code's logic and flow.How to use JavaScript Comparison Operators
To use JavaScript Comparison Operators, you will need to include them in statements that perform comparisons between values. The operator resides between the two values to be compared and returns either a 'true' or 'false' boolean result depending on the outcome of their comparison. For instance, to compare if a variable 'a' is greater than 'b', you would code it as follows:var a = 10; var b = 5; console.log(a > b); // Outputs: trueHere, \(a > b\) is a comparison statement where '>' is the comparison operator checking if 'a' is greater than 'b'. The '===' operator checks for both type and value equality. It is more stringent than the '==' operator, which will only check the values after performing a type coercion if necessary.
var c = "10"; var d = 10; console.log(c === d); // Outputs: false console.log(c == d); // Outputs: trueIn the first console.log, it returned false because although the values of 'c' and 'd' are both 10, one is a string while the other is a number. The second console.log returned true because the '==' operator performed a type coercion converting the string "10" into a number before performing the comparison.
Practical examples of JavaScript Comparison Operators
Seeing the JavaScript Comparison Operators used in practical examples better clarifies their role, functionality, and impact on the overall code logic. For example, suppose you plan to write a function that checks the eligibility of a user based on their age.function checkEligibility(age){ if(age >= 18){ return "You are eligible."; } else{ return "You are not eligible."; } } console.log(checkEligibility(20)); // Outputs: You are eligible.In the above case, if age is greater than or equals 18 (the '>=' operator), it returns "You are eligible."
Combining JavaScript Comparison and Logical Operators
JavaScript Comparison Operators can be combined effectively with logical operators for complex conditions. In JavaScript, the AND operator is represented by '&&', the OR operator by '||', and the NOT operator by '!'. Here’s an example of how these operators could be used in conjunction.var e = 20; var f = 30; console.log(e > 10 && f > 10); // Outputs: trueThis '&&' operator ensures both conditions must be true for the entire statement to be true. Here, in the console.log, both 'e' and 'f' are greater than 10, so the AND operation returns true. On the other hand, the OR operator only requires one of the conditions to be true.
var g = 5; var h = 30; console.log(g > 10 || h > 10); // Outputs: true
JavaScript Comparison Operators Examples in Real-world Scenarios
Understanding JavaScript Comparison Operators are better illustrated in real-world scenarios. A common scenario could be a conditional user login based on username and password matching.function validateLogin(username, password){ var storedUsername = "user123"; var storedPassword = "pass123"; if(username === storedUsername && password === storedPassword){ return "Login successful!"; } else{ return "Invalid credentials!"; } } console.log(validateLogin("user123", "pass123")); //Outputs: Login successful!In this case, the function 'validateLogin' uses the '===' and '&&' operators to authorize a user login only when both 'username' and 'password' match the respective stored values. By understanding and implementing these operators contextually, you can create concise, efficient, and highly readable JavaScript codes.
Debunking Misconceptions about JavaScript Comparison Operators
Cracking the code and navigating through the vast sea of JavaScript can sometimes seem like a daunting task, particularly with topics that appear to hold conflicting views. But worry not, you are sailing on safe waters. Speaking of misconceptions, let's delve into JavaScript comparison operators, how they behave, and iron out any misinterpretations that may exist.Common Misunderstandings of JavaScript Comparison Operators
When it comes to JavaScript comparison operators, particularly the '==' and '===' operators, things can become a bit tricky. This section purports to bring some clarity to the looming confusion. Some predominant misunderstandings pertain to their usage, and the differences between them are often blurred.Loose equality (==): This operator, often erroneously considered exactly the same as '===', compares two values for equality, after performing type coercion if necessary. This can lead to some unexpected results for inexperienced developers.
0 == false // this returns true '0' == false // this also returns trueBoth of the above examples return 'true', even though the operands don't strictly share the same value or type. This is because the '==' operator will convert both operands to a common type before making the comparison. Similarly, there is another prevalent misunderstanding, that minor nuances with the '===' operator, commonly known as the 'strict equality operator'.
Strict equality (===): Unlike its counterpart, the strict equality operator will not perform type coercion and compares both value and type.
3 === 3 // returns true 3 === '3' // returns falseIn the above examples, the second instance returns 'false' because though the value is the same (i.e. 3), the types are different (number vs string). Hence, although '==' and '===' may seem similar, they serve different purposes, and it's important to appreciate the distinction to avoid confusion and errors.
JavaScript Comparison Operators Misconceptions: Truth vs Myth
Despite extensive resources available on the subject, there still tend to be myths and misconceptions surrounding JavaScript Comparison operators. Instead of shying away from these misconceptions, understanding them and separating fact from myth can help hone your JavaScript skills more effectively. One common misconception is that the '==' operator is always bad, while '===' is always better. The choice depends purely on context and purpose. For instance, when needing to check if a variable is either 'undefined' or 'null', using '==' could be beneficial.var myVar; if(myVar == null) { console.log("myVar is either null or undefined"); }In this case, the '==' operator handles the comparison conveniently, considering that JavaScript treats both 'undefined' and 'null' almost equivalently.
The Trap of '==' and '===' in JavaScript Comparison Operators
While the '==' and '===' operators are often at the centre of misconceptions, they are also key to understanding comparison operators in JavaScript. One pitfall to be aware of involves object comparisons. In JavaScript, when you use an equality operator to compare objects (like arrays or other non-primitive data types), it checks reference and not structural equality. This means that:[] == [] // Return false [] === [] // Also return falseThese arrays are separate instances, even though they're empty and look identical, so these comparisons return 'false'. Similarly, another trap sails around the 'NaN' value in JavaScript. The nature of 'NaN' is unique and can cause unexpected results when using comparison operators. Notably, 'NaN' is the only JavaScript type that is not equal to itself.
NaN == NaN // Returns false NaN === NaN // Returns falseIts uncanny behaviour can be mitigated by using JavaScript's global 'isNaN()' function to correctly identify 'NaN' values. While working with JavaScript comparison operators, it's worthwhile to be mindful of these nuances to keep your code free of hard-to-detect bugs and errors.
Correcting Misconceptions: JavaScript Comparison Operators
At the end of it all, the eminent takeaway is the importance of understanding JavaScript Comparison Operators, their idiosyncrasies, nuances, and correct usage. In debunking the misconceptions, remember:- '==' checks for equality after performing any necessary type coercion.
- '===' checks for equality without performing type coercion.
- All JavaScript comparison operators are essential and serve unique purposes.
- Non-primitive types, such as arrays or objects, are compared by reference, not by structure or content.
- 'NaN' compared to anything, even itself, will always return false.
Probing into Use Cases of JavaScript Comparison Operators
There are several use cases for JavaScript Comparison Operators, regardless of whether you're building a small application or working in a large, complex code base. These operators, ubiquitous in the world of coding, are integral to controlling the flow and logic of an application, and understanding their diverse use cases can deliver powerful tools to your JavaScript toolkit.JavaScript Comparison Operators and their Use Cases
Here at the core of JavaScript are Comparison Operators: powerful, versatile and crucial for a variety of tasks. They're employed everywhere from setting conditions in loops, to validating data input, to controlling if-else decisions, and much more. The simple operators==
, !=
, ===
, !==
, >
, <
, >=
and <=
turn out to be the backbone of control logic in scripting languages. Let's delve deep into the applications of these unsung heroes.
One of the most common use cases is in conditional logic – that is, directing the code to execute different instructions based on different conditions.
var studentGrade = 75; if(studentGrade >= 60) { console.log("Passed"); } else { console.log("Failed"); }In the example code above, the '
>=
' operator is used to compare the student's grade to the passing grade. If the student's grade is greater than or equal to 60, the console logs "Passed". Otherwise, it logs "Failed".
Another use case is in sorting operations. JavaScript Comparison Operators are widely used in various sorting algorithms to compare values and determine their order. Here's a quick use of the '<
' operator in a bubble sort:
function bubbleSort(array) { for(let i = 0; i < array.length; i++) { for(let j = 0; j < array.length - i - 1; j++) { if(array[j] > array[j + 1]) { let temp = array[j]; array[j] = array[j + 1]; array[j + 1] = temp; } } } return array; }
Loop Conditions and JavaScript Comparison Operators
One of the most common places where you'll encounter comparison operators is within the condition of a loop. They're especially useful in for loops, where a condition is set to determine how long the loop should continue.for(var i = 0; i < 10; i++) { console.log(i); }In the above example, the '
<
' comparison operator serves as the deciding factor for the loop's continuation. The loop ends once 'i
' is no longer less than 10.
Validation Checks with JavaScript Comparison Operators
Another prominent use case of JavaScript Comparison Operators is in form validation, a common task in virtually all web applications that require user input. For example, you could use these operators to ensure that passwords meet a certain length, or that a year of birth input values are within a specific range.function validatePassword(password) { if(password.length >= 8) { console.log("Valid Password"); } else { console.log("Password should be at least 8 characters"); } } validatePassword("myPassword");Here the '
>=
' operator checks if the length of the password is eight or more. If the condition is met, "Valid Password" is logged to the console. Otherwise, a message indicating that the password should be at least eight characters is logged.
By leveraging JavaScript comparison operators effectively in these scenarios, you can create a robust validation check that enhances user experience and ensures data integrity. From simple to complex applications, understanding how to use these operators provides a foundation for more efficient code and refined logic structures.Javascript Comparison Operators - Key takeaways
- JavaScript comparison operators include: equality (==), inequality (!=), strict equality (===), strict inequality (!==), greater than (>), less than (<), greater than or equal to (>=), less than or equal to (<=).
- The difference between equality and strict equality lies in type coercion. Equality checks if two values are equivalent after type coercion, while strict equality checks if they are equivalent without type coercion.
- Usage of JavaScript Comparison Operators involves positioning the operator between the two values to be compared, which returns either a 'true' or 'false' boolean result.
- Common misconceptions about JavaScript Comparison Operators revolve around the use of '==' and '==='. '==' checks for equality after performing any necessary type coercion, while '===' checks for equality without performing type coercion.
- Use cases of JavaScript Comparison Operators include conditional logic and sorting operations, among others. They provide the backbone for control logic in scripting languages.
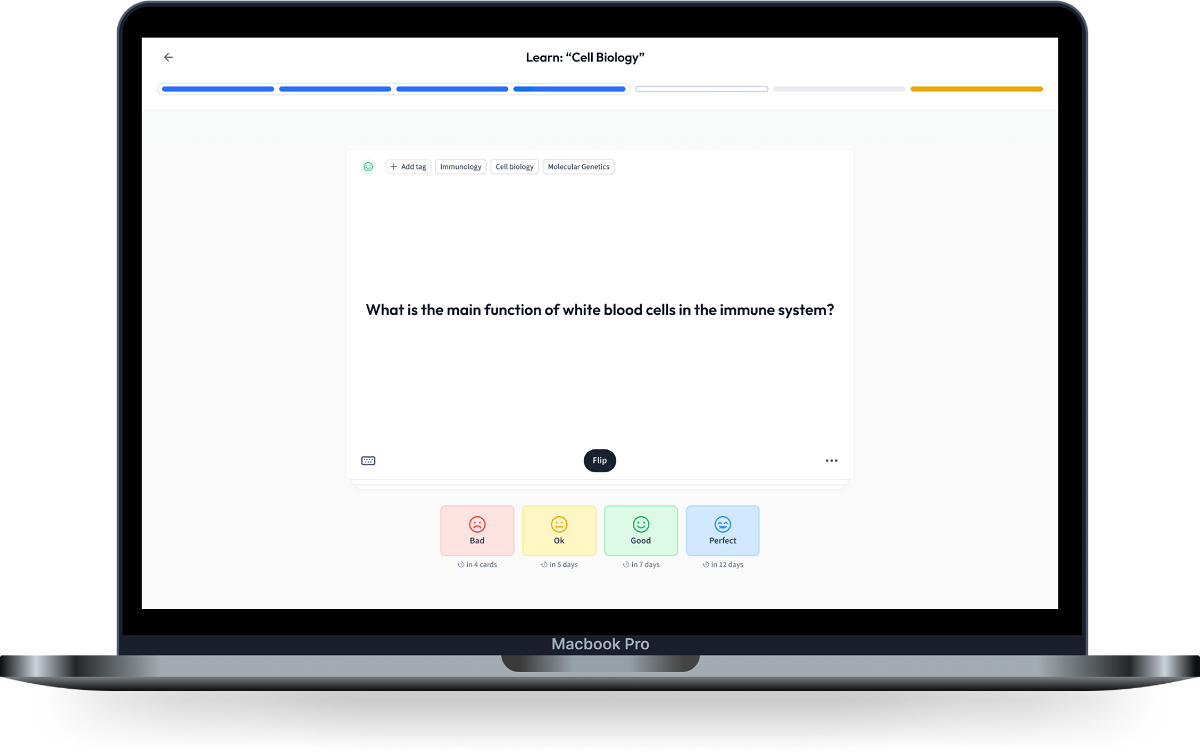
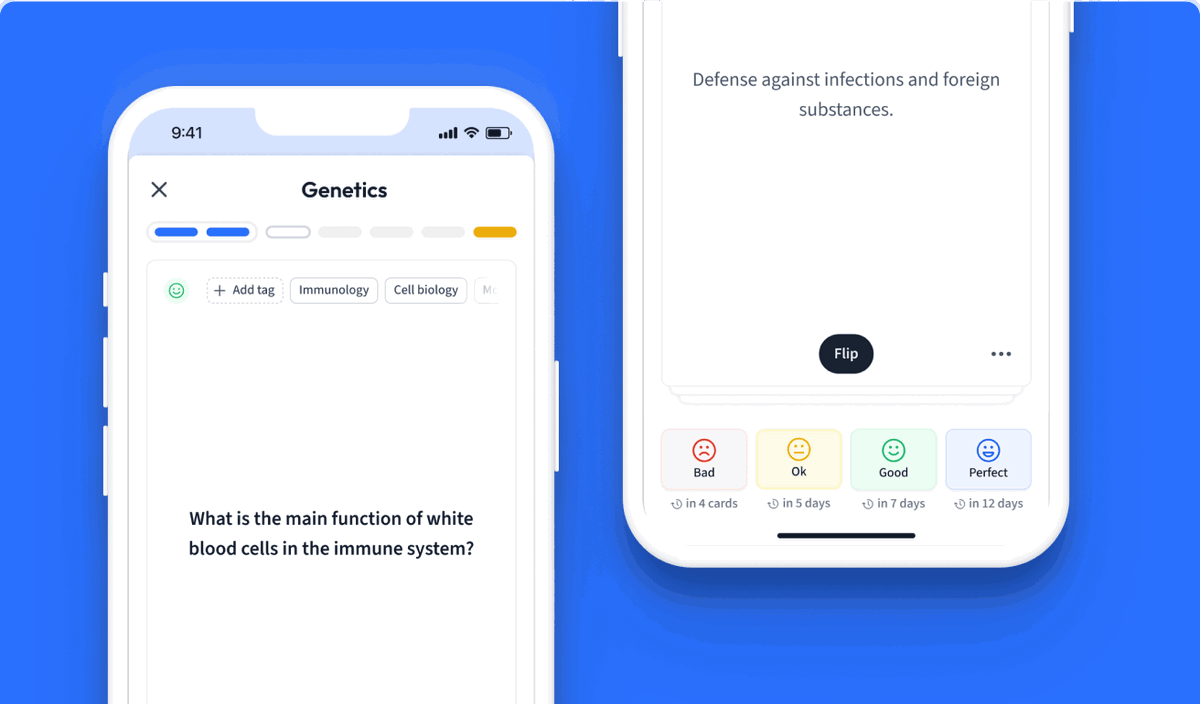
Learn with 12 Javascript Comparison Operators flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript Comparison Operators
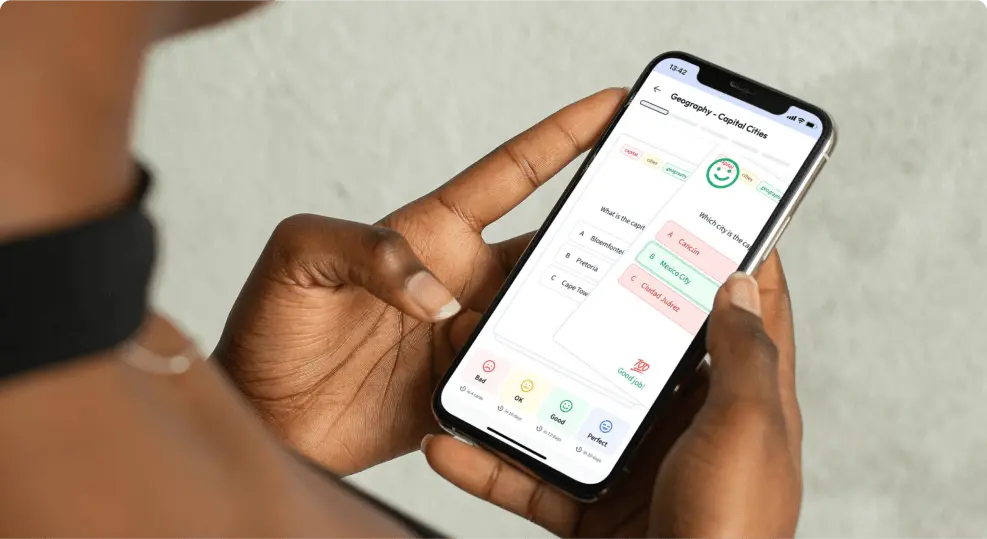
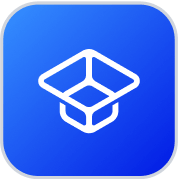
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more