Understanding Java Reflection: An Overview
In the world of computer science, particularly when you're dealing with Java, you'll encounter a term known as Java Reflection. It's a potent tool that developers have at their disposal, and you ought to have a good grasp of its concepts and utilities.Java Reflection is a language ability to inspect and modify its own structure and behaviour at runtime. It allows Java programs to manipulate internal properties of objects, methods, classes, and interfaces.
Defining Java Reflection
Let's dig deeper into understanding Java Reflection. In essence, it's a process that inspects Java classes, interfaces, constructors, and even methods at runtime, giving you an in-depth look into the components of the Java codebase. This advanced feature of Java allows you:- To instantiate new objects,
- To invoke methods,
- To get/set field values,
- To analyse class’s binary code, and
- To inspect class loading.
Consider a scenario where you need to load a class at runtime, and you aren't aware of its name during compile time. With Java Reflection, you can make this possible by retrieving and manipulating the loaded class's data.
Tracing the History of Java Reflection
Java Reflection always wasn't a part of the Java Programming language. However, having the ability to inspect and change the class's behaviour at runtime made java developers realise its importance over time.The Evolution of Reflection in Java
The inception of Java Reflection began with the introduction of introspection in JDK 1.1, which allowed developers to inspect the classes' properties. This was a significant milestone in Java's history, marking the early steps in the inception of Java Reflection. Following JDK 1.1, JDK 1.2 introduced the Reflection API, which not only enables the inspection of classes, fields, and methods but also allows their dynamic invocation. Over time, Java Reflection improved and now it is an essential part of the Java programming tool belt for creating comprehensive, complex systems.While it may seem like a powerful tool, Reflection comes with few caveats. It can break the encapsulation property of object-oriented programming and slow down the execution. Therefore, one should use it cautiously and only when necessary.
Technicalities Behind Java Reflection Technique
Diving into the technicalities of Java Reflection, it's important to remember that it operates on a runtime environment. Firstly, the ClassLoader loads the bytecode of the class. Then, JVM creates a Class object from the loaded bytecode. Here is a basic representation of Java Reflection call:Class.forName("com.example.MyClass");This command is used to dynamically load the class into memory, where 'com.example.MyClass' is the fully classified name of the class. Java Reflection Methods include: - getDeclaredMethods() to get all declared methods from the class. - getMethods() to get all public methods from the class and inherited from superclasses. - getMethod() to get a specific public method from the class or inherited from superclass. Furthermore, a refactored class can invoke methods, which typically follow the formula \(method.invoke(object, args)\). The object is the instance on which to call the method and args are the method's arguments. When used appropriately, Java Reflection can be an invaluable tool for Java developers, offering dynamic runtime modifications and detailed insights into the classes and objects being worked upon. Both experienced and budding Java developers can benefit tremendously from familiarising themselves with this essential tool in the Java toolkit.
Delving into Detail: What are Reflections in Java
In the realm of Java Programming Language, Reflection is a potent aspect that allows a program to introspect its characteristics at runtime. This means that Java programs can examine and modify their own behaviours in live mode without any compile-time limitations.Intricacies of Reflections in Java
To truly appreciate the power and utility of Reflection in Java, it helps to grasp its intricate attributes. Fundamentally, Reflection in Java revolves around the java.lang.Class class, from which all reflection operations originate. It enables a Java program to analyse and modify itself, providing impressive flexibility and dynamic functionality. Here are a few components in Reflections that aid in this process:- java.lang.Class: This serves as the entry point for all reflection operations. Every piece of data has a class corresponding to it, which can be accessed using .getClass() method.
- java.lang.reflect.Method: This holds a single method on a class or interface and can be used to understand its behaviour and invoke it.
- java.lang.reflect.Field: This represents a field of a class or interface, and it's used for the runtime inspection and alteration of class fields.
- java.lang.reflect.Constructor: This stands for a class constructor and can be used to create new instances of a class.
Java Reflection Definition: A Closer Look
The term 'Java Reflection' may seem complex, but it is fairly straightforward. It’s essentially a feature of the Java Programming Language that allows an executing Java program to examine and modify its own internal structure and behaviours at runtime. Here's an illustrative example of Java Reflection:Class myClass = MyClass.class; MyClass myClassObject = (MyClass) myClass.newInstance();This demonstrates how to create an instance of 'MyClass' using Reflection. The .newInstance() method returns an instance of the class represented by the Class object (in this case, 'MyClass').
The Function of Reflections in Java
Java Reflection serves several purposes that make it an ideal tool for dynamic programming, development environments, and even debugging. It allows an executing Java program to:- Examine the JVM’s runtime environment,
- Construct objects of arbitrary class,
- Inspect class fields and methods,
- Inspect and manipulate arrays,
- Inspect and invoke methods, and
- Inspect and manipulate objects on the field level.
The Practical Use of Reflection in Java
While Java Reflection is a powerful tool, it's fundamental to remember that with its power comes responsibility. You should avoid using it excessively because it can potentially break the design and produce code that's difficult to maintain. But in situations where you need to make your application more dynamic and responsive, Java Reflection proves to be incredibly useful. For instance, Reflection is widely used in:- IDE (like Eclipse, IntelliJ): IDEs use reflection to provide a list of methods and variables that a class declares.
- Debuggers: Debuggers use reflection to examine private members on classes.
- Test Tools (like JUnit, TestNG): Testing tools use reflection to define classes to test, load them, and then call the testing methods.
- Frameworks (like Spring, Hibernate): Frameworks use reflection to inspect the annotations defined in class files.
Advantages and Applications: Benefits of Java Reflection
Java Reflection stands as a powerful feature in Java programming, allowing computational reflections at runtime. Its potential benefits extend beyond the generic ones, thereby increasing its utilisation in software development and dynamic computing environments.The Unforeseen Perks: Benefits of Java Reflection
Java Reflection has several key benefits, many of which arise from the capability to analyse and modify the programme's behaviour during runtime. The notion of manipulating a programme from within itself is an innovative aspect of advanced computing and forms the backbone of several Java applications today. Dynamic Loading: Reflection in Java enables the dynamic loading of classes, which means you can load a class during the program's runtime. The java.lang.Class.forName() method makes this possible by loading the specified class into the JVM. This is particularly useful in scenarios where full knowledge of the classes is not available during compile time. Inspecting Classes, Fields, and Methods: Java Reflection allows you to inspect a class's structure, including its fields, constructors, and methods. You can get the modifiers, return type, and parameter list of a method or constructor, which can be particularly useful in debugging and testing. Accessing Private Members: It provides the ability to access the private members (methods or fields) of a class which can certainly prove useful in certain special use cases, though this should be done cautiously as it can lead to breaking the encapsulation concept of OOP(Object Oriented Programming). Array Manipulation: Java Reflection provides the java.lang.reflect.Array class for dynamic array manipulation. It helps in creating, inspecting, and manipulating arrays dynamically. Implementing Generic Array Types: Java Reflection helps in creating generic array types, overcoming the limitations posed by erasure of type parameters during runtime. Here's an example:Array.newInstance(Sample.class, arrayLength);Here, the Array's newInstance() method is used, and the resulting array is typecast to the type T[].
Real-World Examples Showing Benefits of Java Reflection
Java Reflection often underlies several operations in real-world scenarios, especially when it comes to frameworks and development environments. Here are a few illustrations of these practices: IDEs: Integrated Development Environments (IDEs) like Eclipse or IntelliJ IDEA use Reflection to load classes dynamically for compilation and execution, inspect class structures, and load libraries at runtime. Testing Tools: Testing tools like JUnit or TestNG use Reflection to load the classes to be tested, execute methods on these classes, and thereby determine the test's success or failure. Frameworks: Frameworks like Hibernate or Spring extensively use Reflection for loading plugins, inspecting annotations, and modifying classes during runtime for efficient object-relational mapping and dependency injection.The Wider Scope: Use of Reflection in Java
Reflection is used in Java to manage and manipulate classes, methods, and fields at runtime. The wider scope of its use includes creating objects of a class whose name is not known until runtime, invoking methods on an object associated with the class, and changing field values on an object associated with the class. Dynamic Extensibility: This feature of Java Reflection allows extensibility of systems with new user-implemented classes, which can be designed and implemented after the main system's deployment. Class Browsers and Visual Development Environments: Reflection allows software tools to analyse the user-defined classes' properties, providing more interactive and intuitive interfaces. Debugging and Testing Tools: Reflection provides sophisticated tools for checking the consistency of classes and for dynamic invocation of user-defined methods.Citing Actual Scenarios of Reflection Use in Java
Java Reflection finds extensive use in numerous practical scenarios where dynamic functioning is desired. These often involve manipulating classes and objects at runtime. Here are few real-world examples: Serialization: Serialization involves turning the state of an object into a byte stream, and resurrecting these bytes into a live object at a later time. Java's object serialization interfaces use Reflection to inspect classes, allowing objects of any class to use the standard serialization mechanics. Java Beans: A Bean is a reusable software component. Java Beans use Reflection to provide structured access to their properties, which are inferred by "getter" and "setter" methods. Code can inspect a bean to determine its properties and manipulate them, without knowing them in advance. Remote Method Invocation (RMI): Java RMI's use of stub classes can be replaced with the use of a proxy class that uses reflection to invoke methods on a remote server. A remote method can be invoked without requiring the stub class at the client side. Indeed, the use of Java Reflection comes with numerous advantages, and its applications have revolutionised and simplified complex processes within the Java programming ecosystem.Your Guide to Java Reflection: Steps in Java Reflection
Java Reflection might seem complex on the surface, but once you break down the steps involved in the process, it becomes significantly straightforward. This section guides you through the whole course of Java Reflection, spotlighting each step in detail.Familiarising with the Steps in Java Reflection
At its core, Java Reflection involves five primary steps: acquiring the Class object, gathering class information, retrieving methods, retrieving fields, and accessing constructors. Each step forms a crucial part of the Reflection process, allowing for dynamic manipulation of the program at runtime. 1. Acquiring the Class Object: The first step in using Java Reflection is obtaining a Class object. This can be achieved by using the fully qualified name of the class and the Class.forName() method.Class> c = Class.forName("com.example.MyClass");2. Gathering Class Information: Once you have the Class object, you can use its methods to gather information about the class. This includes the class name, its superclass, and the interfaces it implements.
String name = c.getName(); Class> superClass = c.getSuperclass(); Class>[] interfaces = c.getInterfaces();3. Retrieving Methods: The Class object also enables retrieval of all declared methods, including public, protected, default (package), and private methods, but not inherited ones. You can use the getMethods() method to fetch these details.
Method[] methods = c.getMethods();4. Retrieving Fields: You can retrieve all the public fields of a class, including inherited ones, using the getFields() method of the Class object.
Field[] fields = c.getFields();5. Accessing Constructors: You can also access the constructor's information of a class using getConstructors() method.
Constructor>[] constructors = c.getConstructors();
Detailed Walkthrough of Java Reflection Steps
While the primary steps offer a snapshot of working with Java Reflection, understanding each step's intricacies can greatly enhance your practical handling of this potent tool in Java. - Acquiring the Class Object: This is arguably the most crucial step in the process. Here, you are obtaining the Class object for the class you wish to inspect. Invoking the forName() method with the fully qualified class name returns a Class object. - Gathering Class Information: At this point, the Class object can be used to gather detailed information about the class it represents. Through methods attached to this object, you can retrieve its name, superclass, and all the interfaces it implements. - Retrieving Methods: This step offers a deep dive into the class's methods. The Class object enables you to retrieve all the declared methods in the class. Please note that this only retrieves the methods declared in the class, but not those in inherited classes. - Retrieving Fields: Similar to retrieving methods, retrieving fields provides an in-depth view of the class's fields. The getFields() method returns an array of Field objects, representing all the accessible public fields of the class or interface represented by the Class object. - Accessing Constructors: This step involves fetching the constructors of the class. The getConstructors() method returns an array of Constructor objects reflecting the public constructors of the class represented by the Class object. Understanding each of these steps can effectively simplify your interactions with Java Reflection, foster a deep appreciation for its dynamic nature and ideally prepare you for practical instances where Reflection might be beneficial.Java Reflection Example: Taking an In-depth Look
By examining a concrete Java Reflection example, you can see how the different steps are put into practice. This example will illustrate the entire process of using Reflection to analyse the structure of a class and manipulate its properties at runtime.public class Rectangle { public double length; public double breadth; public Rectangle(double length, double breadth) { this.length = length; this.breadth = breadth; } public double getArea() { return length * breadth; } }The sample class, `Rectangle`, has two fields (`length` and `breadth`), a constructor, and a method (`getArea`). Reflection can be used to analyse and manipulate this class at runtime.
public class ReflectionExample { public static void main(String[] args) { Class> rectangleClass = Rectangle.class; Field[] fields = rectangleClass.getFields(); for (Field field : fields) { System.out.println("Field name: " + field.getName()); System.out.println("Field type: " + field.getType()); } Method[] methods = rectangleClass.getMethods(); for (Method method : methods) { System.out.println("Method name: " + method.getName()); System.out.println("Method return type: " + method.getReturnType()); } } }Running the `ReflectionExample` main method will print out the names and types of the `Rectangle` class's fields and the names and return types of its methods.
Decoding Java Reflection with Comprehensive Examples
When it comes to using Reflection, concrete examples can be a great way to understand and apply the individual steps. In this section, we will provide comprehensive illustrations covering every step of the Reflection process, from grabbing the Class object, inspecting its details, to manipulating its fields, methods, and constructors. Consider a simple class, `Rocket`, as follows:public class Rocket { private String destination; public Rocket(String destination) { this.destination = destination; } public void launch() { System.out.println("Launching rocket to " + destination); } }Initialise an instance of `Rocket` in the traditional way:
Rocket rocket = new Rocket("Mars"); rocket.launch();Using Reflection, creating and manipulating the `Rocket` class can be done as shown below:
try { // Acquire the Class object Class> rocketClass = Class.forName("com.example.Rocket"); // Retrieving Class Information String className = rocketClass.getSimpleName(); String fullClassName = rocketClass.getName(); // Retrive Methods Method[] methods = rocketClass.getDeclaredMethods(); // Retrieving Fields Field[] fields = rocketClass.getDeclaredFields(); // Access Constructors Constructor> cons = rocketClass.getConstructor(String.class); Object rocketObject = cons.newInstance("Jupiter"); // Invoke the launch method on the rocketObject instance Method launchMethod = rocketClass.getMethod("launch"); launchMethod.invoke(rocketObject); } catch (ClassNotFoundException | NoSuchMethodException | IllegalAccessException | InstantiationException | InvocationTargetException e) { e.printStackTrace(); }This creates an instance of `Rocket` and invokes its `launch` method, all through Reflection. Such examples provide invaluable insight into the practical usage of Java Reflection. You can modify this example based on your applications, employing the steps discussed to leverage Java Reflection's immense power in your programmes.
Java Reflection - Key takeaways
- Java Reflection: A feature of Java that allows programs to examine and modify their own operations during runtime, offering developers dynamic runtime modifications and detailed insights into their classes and objects, significantly enhancing Java's flexibility and functionality.
- java.lang.Class: The main class from which all reflection operations begin, allowing programs to analyze and modify their own behaviors.
- Components of Reflection: Include java.lang.reflect.Method, java.lang.reflect.Field, and java.lang.reflect.Constructor, which respectively represent a single method, a field of a class or interface, and a class constructor. These aid in examining and altering a program's behavior.
- Java Reflection Uses: Primarily used for dynamic programming and debugging, Java Reflection allows for the inspection and manipulation of classes, interfaces, fields, and methods at runtime. Common use cases include loading new user-implemented classes dynamically, inspecting user-defined classes for software tool development, and invoking user-defined methods for debugging and testing.
- Steps in Java Reflection: Involve acquiring a Class object, gathering class information, retrieving methods and fields, and accessing constructors. These form the process of dynamically manipulating a program at runtime, starting from obtaining an object to analyzing classes and invoking methods or altering fields or constructors.
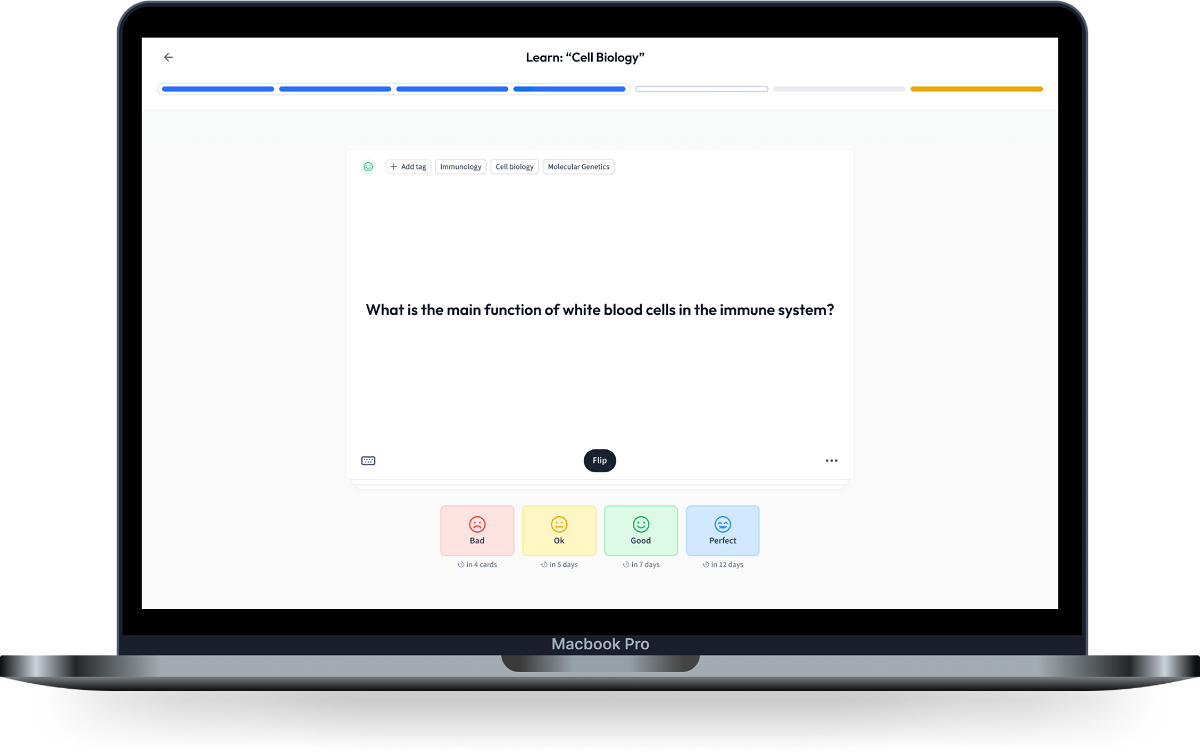
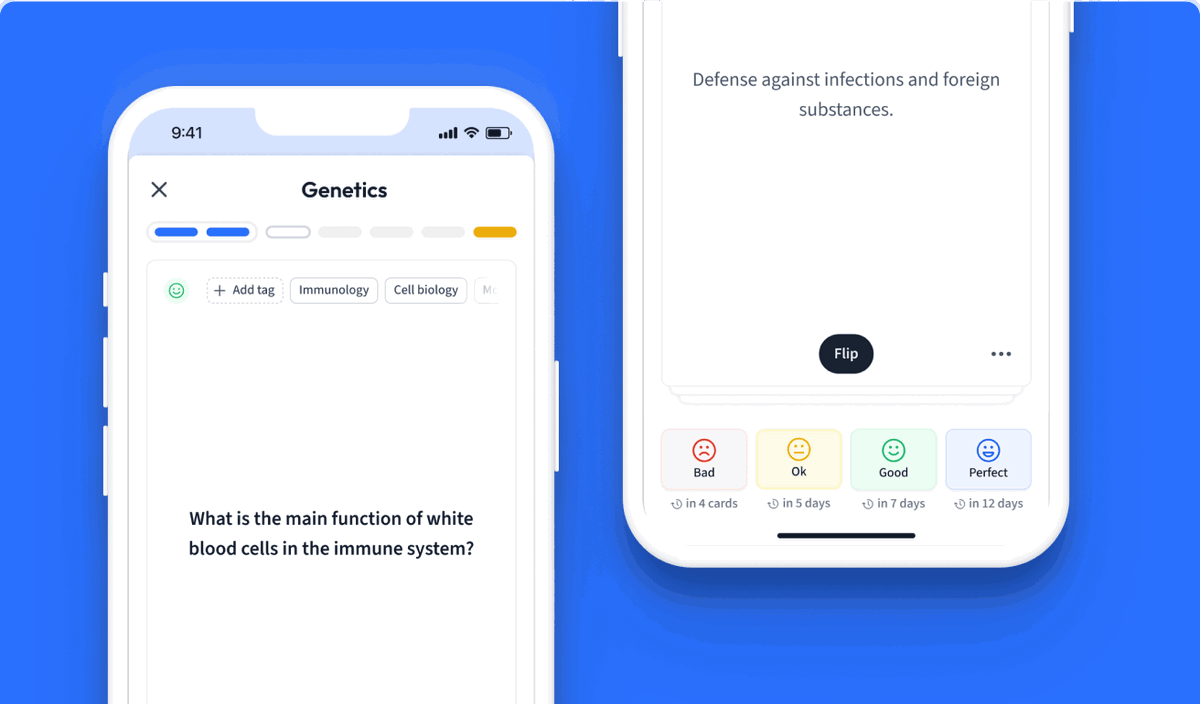
Learn with 12 Java Reflection flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Java Reflection
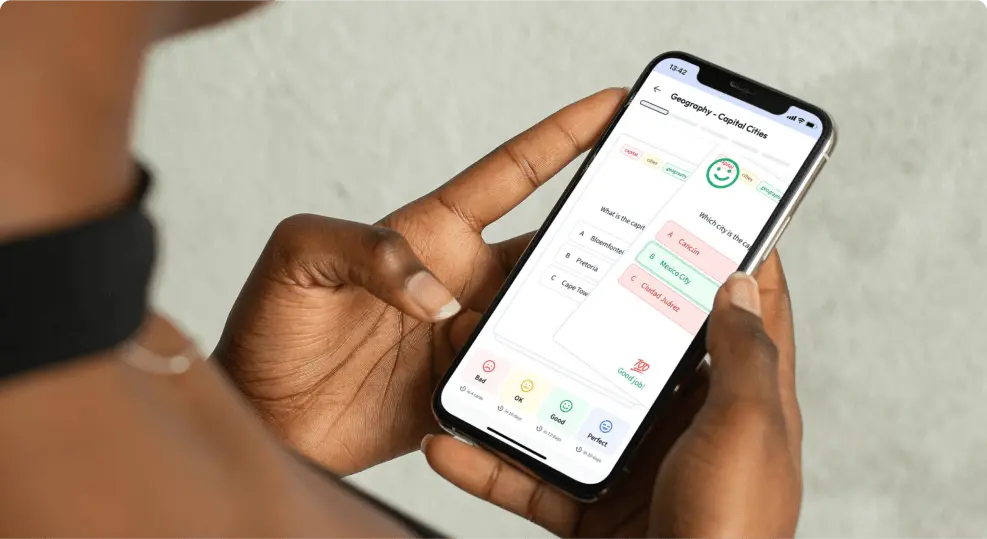
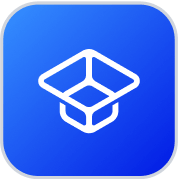
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more