About C Programming Language
The C Programming Language is a widely known, efficient, and versatile programming language that serves as the foundation for various modern programming languages. It was created by Dennis Ritchie in 1972 as a part of the development of the UNIX operating system. Today, you can find C Programming Language employed in embedded systems, operating systems, system programming, and even game development. With its solid foundation, mastering the C Programming Language is an essential skill for any programmer or aspiring computer scientist.
Core concepts of C Programming Language
Mastering the C Programming Language involves understanding its core concepts, such as data types, variables, conditional statements, loops, functions, and pointers. By gaining knowledge of these concepts, you will have the necessary foundation to learn other related languages and frameworks, build complex programs, and solve real-world problems more efficiently.
Data types and variables in C
Every programming language has data types and variables to store information. In C, data types can be divided into 3 main categories:
- Basic: int, float, double, and char
- Derived: arrays, structures, unions, and pointers
- User-defined: structures, enums, and typedefs
A variable is an identifier that represents a memory location in which data is stored, and it is always associated with a data type that dictates the type and size of data it can store.
Understanding the syntax for declaring and initializing variables in C is crucial:
// Variable declaration
data_type variable_name;
// Variable initialization
data_type variable_name = value;
For example, to declare and initialize a variable called 'age' with the value 30, you would write:
int age = 30;
Conditional statements and loops in C
Conditional statements and loops are essential control structures that allow you to execute specific blocks of code based on certain conditions and repetitions.
There are two types of conditional statements in C:
- if-else statement: Allows you to execute a block of code if a certain condition is true or execute an alternative block of code if the condition is false.
- switch statement: Permits you to choose a block of code to be executed from multiple alternatives based on a single value.
There are three types of loops in C:
- while loop: Repeats a block of code as long as a certain condition remains true.
- do-while loop: Executes a block of code at least once and then repeats it as long as a certain condition remains true.
- for loop: Iterates a block of code a specific number of times based on an initial condition, a termination condition, and a step size.
Functions and pointers in C
Functions are the building blocks of a program, allowing you to execute a group of statements to serve specific tasks and keep your code organized and reusable.
A function in C Programming Language has a name, a return type, and zero or more parameters to be passed as input. Additionally, you can declare a function with a special keyword 'void' as its return type when the function is not intended to return any value.
Functions are defined as follows:
return_type function_name(parameters) {
// Function body
}
For example, a function called 'sum' that accepts two integers as input and returns their sum could be defined like this:
int sum(int a, int b) {
return a + b;
}
Pointers are another important concept in the C Programming Language that refers to variables that store the memory address of another variable, allowing you to manipulate data directly and efficiently.
Understanding how to properly use pointers is fundamental for advanced C programming topics like dynamic memory allocation, passing arrays to functions, or even working with complex data structures like linked lists.
History of C Programming Language
The history of the C Programming Language is full of innovation and progress, with its roots in earlier languages and its influence on modern programming languages. Understanding how the C Programming Language evolved, and its development milestones, would help appreciate its impact on computer science and its continued relevance today.
Evolution and development of C
The C Programming Language has come a long way since its inception in the early 1970s. Its development has been marked by several milestones, and it has undergone numerous revisions and enhancements that shaped its current form. The path to creating C began with earlier programming languages such as assembly, ALGOL, and BCPL.
The relationship between C and earlier languages
Before the creation of C, other languages played a critical role in its inception. Some notable languages in the history leading up to C include:
- Assembly language: C was designed with low-level programming in mind, and its creators drew inspiration from assembly language when implementing features for direct interaction with hardware.
- ALGOL: The block structure and lexical scoping concepts of C were adapted from ALGOL, a programming language designed primarily for scientific purposes.
- BCPL: Developed by Martin Richards, BCPL (Basic Combined Programming Language) was the immediate predecessor of C. Its simple syntax and portability influenced the design of C, particularly in the areas of expression evaluation, control structures, and the notion of pointers.
C was developed by Dennis Ritchie at Bell Labs as part of the UNIX operating system project. At the time, Ken Thompson was also developing a language called B (based on BCPL), which was being used for the UNIX operating system. However, due to its limitations, Dennis Ritchie embarked on developing C to overcome those constraints and add more advanced features like data structures and rich operators.
Contribution of C to modern programming languages
The impact of the C Programming Language is still felt today, as it has significantly influenced the design of many modern programming languages. Some important contributions of C to modern programming languages are:
- Modular programming: C supports modular programming, promoting code organization, reusability and maintainability. This design approach has been embraced by many subsequent programming languages.
- Pointer concept: C's support for pointers has been a core foundation for working with memory. Pointers are used in various modern languages, such as C++, C#, and even interpreted languages like Python, although in a more abstracted manner.
- Low-level capabilities: C provides a way to interact with hardware and manage memory directly, making it versatile and efficient. This feature has been inherited in various systems-oriented languages like C++, Rust, and Go.
- Syntax and semantics: The C Programming Language's syntax and semantics have had a lasting impact on various programming paradigms and languages like Java, C#, JavaScript, and Python, which share many similarities in their syntax and control structures.
Overall, the evolution of the C Programming Language has played a prominent role in shaping the foundations for multiple programming languages, systems, and applications around the world. Its rich history and influence across computer science make it an essential subject for both aspiring and experienced programmers.
C Programming Language Examples
In this section, we will explore various examples of C Programming, ranging from basic to intermediate. These examples serve as a stepping stone for beginners who want to learn and practice C Programming Language and eventually tackle more advanced problems.
Basic examples for beginners in C
For beginners, it is crucial to start with fundamental and straightforward C programming examples to understand basic syntax, control structures, and functions.
"Hello, World!" program in C
The "Hello, World!" program is one of the simplest and most widely known examples to start learning any programming language. In the case of C, this program demonstrates the basic structure of a C program, the use of the printf() function to print text to the console output, and the return statement to indicate successful program execution. Here's the code for the "Hello, World!" program in C:
#include
int main() {
printf("Hello, World!\n");
return 0;
}
In this example, the #include directive tells the compiler to include a header file (stdio.h), which contains the declaration of the printf() function. The "main" function represents the entry point of the program and contains the necessary code to print "Hello, World!" to the console output.
Calculator example using C
An essential calculator program demonstrates the use of various arithmetic operations, such as addition, subtraction, multiplication, and division. It also shows how to utilize switch statements and user input. The following calculator example in C accepts two numbers and a choice of operation from the user:
#include
int main() {
float num1, num2, result;
char operator;
printf("Enter first number: ");
scanf("%f", &num1);
printf("Enter second number: ");
scanf("%f", &num2);
printf("Choose an operation (+, -, *, /): ");
scanf(" %c", &operator);
switch (operator) {
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
result = num1 / num2;
break;
default:
printf("Invalid operator\n");
return 1;
}
printf("Result: %.2f %c %.2f = %.2f\n", num1, operator, num2, result);
return 0;
}
In the above code, scanf() function is used to take input from the user for numbers and the arithmetic operation, the switch statement is used to perform a particular operation based on user's choice, and the calculated result is printed on the console output using printf() function.
Intermediate examples for C programming
Once you have a good grasp of the basic concepts of C Programming Language, it's important to explore some intermediate level examples to enhance your problem-solving skills and learn more complex algorithms and techniques.
Fibonacci sequence using C
The Fibonacci sequence is a series of numbers in which each number is the sum of the two preceding ones, usually starting with 0 and 1. An example of writing a program in C to display the first 'n' Fibonacci numbers is shown below:
#include
int main() {
int n, first = 0, second = 1, next;
printf("Enter the number of terms: ");
scanf("%d", &n);
printf("Fibonacci series: ");
for (int i = 1; i <= n; ++i) {
printf("%d ", first);
next = first + second;
first = second;
second = next;
}
printf("\n");
return 0;
}
This program demonstrates the use of for loops to iterate over a specific range and calculate the Fibonacci numbers. The variables 'first' and 'second' keep track of the previous numbers in the sequence, and 'next' stores the next number in the sequence.
Sorting algorithms in C
Sorting algorithms are fundamental techniques used to arrange data in a specific order. They play a significant role in computer science and provide an excellent opportunity to learn more complex coding patterns, such as loops, functions, and pointers. Two popular sorting algorithms are Bubble Sort and Selection Sort:
Here's a Bubble Sort algorithm implemented in C:
#include
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
int main() {
int arr[] = {64, 34, 25, 12, 22, 11, 90};
int n = sizeof(arr) / sizeof(arr[0]);
bubbleSort(arr, n);
printf("Sorted array: ");
for (int i = 0; i < n; i++)
printf("%d ", arr[i]);
printf("\n");
return 0;
}
Here's a Selection Sort algorithm implemented in C:
#include
void selectionSort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
int min_idx = i;
for (int j = i + 1; j < n; j++)
if (arr[j] < arr[min_idx])
min_idx = j;
int temp = arr[min_idx];
arr[min_idx] = arr[i];
arr[i] = temp;
}
}
int main() {
int arr[] = {64, 25, 12, 22, 11};
int n = sizeof(arr) / sizeof(arr[0]);
selectionSort(arr, n);
printf("Sorted array: ");
for (int i = 0; i < n; i++)
printf("%d ", arr[i]);
printf("\n");
return 0;
}
Both sorting algorithms utilize nested loops to iteratively compare and swap elements to sort the array. The key difference lies in how they approach this task. Bubble Sort repeatedly swaps adjacent elements if they are in the incorrect order, while Selection Sort selects the smallest (or largest) element in the unsorted part of the array and places it at the correct position.
Advantages and Disadvantages of C Programming Language
The C Programming Language has been a cornerstone of computer science for decades, and despite being an older language, it continues to provide valuable benefits to developers across various industries. However, like any programming language, C has its own set of advantages and disadvantages that have impact on its usage, depending on specific programming needs and software development goals.
Pros of using C programming language
Despite its age, the C Programming Language offers several significant advantages that continue to make it a popular choice among programmers. These benefits range from exceptional performance and efficiency to widespread compatibility and portability.
Efficiency and performance of C programs
One of the most notable advantages of C is its efficiency and performance. C programs are generally faster and require less memory compared to many other programming languages. This can be attributed to several factors, including:
- Low-level access: C provides direct access to memory and hardware resources, enabling manipulation of data and efficient memory management.
- Less overhead: C programs typically have less overhead and runtime complexities, which can result in faster execution times.
- Optimised compilation: C compilers are well-established and can produce highly optimised machine code, contributing to improved performance.
With these characteristics, C is an ideal choice for developing high-performance applications, such as operating systems, embedded systems, and game development.
Portability and compatibility of C
Another significant advantage of C is its portability and compatibility. This means that:
- C code can be easily adapted and compiled on different platforms with minimal modifications.
- Most operating systems and hardware architectures have well-established C compilers, contributing to widespread support for the language.
- C libraries are available for a vast array of tasks, simplifying cross-platform development and reducing the need for reinventing the wheel.
These factors make C a versatile programming language that is relevant across numerous platforms and systems.
Cons of using C programming language
While the C Programming Language offers several advantages, there are some disadvantages that can impact its suitability for certain projects and programming paradigms. Notably, the lack of object-oriented features, and limited debugging and error handling mechanisms can be challenging for programmers.
Lack of object-oriented features
C is a procedural programming language, and thus it lacks built-in support for object-oriented programming (OOP) features, such as classes, objects, inheritance, and polymorphism. As a result, C imposes some limitations on programmers who want to adopt OOP principles:
- OOP features must be emulated using structures, pointers, and other language constructs, which can be cumbersome and less intuitive.
- Without native OOP support, it may be harder for developers to model complex real-world problems or software components in C.
- Code written in C may be less modular, maintainable, and reusable compared to modern object-oriented programming languages like C++, Java, and Python.
Despite these limitations, if a developer is comfortable with procedural programming or the project does not require OOP principles, C remains a powerful and efficient choice.
Debugging and error handling in C
C has limited support for debugging and error handling compared to some modern programming languages. This presents challenges for developers who are working with C, such as:
- Difficulty in identifying and fixing bugs, given that C's low-level access provides more room for error, and its syntax can sometimes be ambiguous.
- Limited error checking and exception handling mechanisms, which can lead to crash-prone and unsafe software.
- Manual memory management, as C lacks garbage collection features, requires developers to handle memory allocation and deallocation, which can be error-prone and lead to memory leaks or segmentation faults.
While these disadvantages can hinder the development process, programmers with strong knowledge and practice of C can still write maintainable, robust, and efficient code, capitalizing on the advantages the language has to offer.
Application of C Programming Language
The C Programming Language has been widely used in various domains, ranging from real-world applications like operating systems and embedded systems to game development, and academic purposes in computer science education and scientific research. These versatile applications of C programming have contributed to its longevity and continued relevance in today's complex and rapidly evolving world of technology.
Real-world applications of C programs
The C Programming Language has found a wide range of applications in the real world, thanks to its efficiency, performance, and low-level capabilities. Some of the most prominent real-world applications of C programs include operating systems, embedded systems, and game development.
Operating systems and embedded systems
One of the most significant application areas of the C Programming Language is in the development of operating systems and embedded systems. Its key advantages, such as low-level access, efficiency, and performance, have made it the go-to choice for building OS kernels, firmware, and other system-level components. Some popular examples include:
- The UNIX operating system, including the Linux kernel, which has been predominantly written in C.
- Windows operating system, where C contributes significantly to the development of core components while other languages like C++ are also utilized.
- RTOS (Real-Time Operating Systems) which govern embedded devices and prioritize performance, predictability, and small memory footprint.
- Other embedded systems, such as IoT devices, automotive systems, and robotics, where C programming ensures efficient management of hardware and software resources.
Game development using C
Another area where the C Programming Language plays a significant role is in the realm of game development. Its efficient memory management, speed, and low-level capabilities make it an appealing choice for creating game engines, graphics libraries, and other game-related components. Examples of C's usage in game development include:
- Library and middleware development, such as widely used libraries like OpenGL, SDL, and OpenAL, which are implemented in C.
- Game engine development, where C is often used in conjunction with other languages like C++ and scripting languages like Lua.
- Optimisation of performance-critical sections of code, where C can provide the speed and control required for real-time graphics, physics simulations, and other computationally intensive tasks.
Academic and research purposes
Aside from its real-world applications, the C Programming Language also plays a significant role in academia and research. Its fundamental concepts, efficiency, and wide-ranging applicability make it an indispensable skill for students, educators, and researchers in computer science and other fields that involve scientific computing and simulation-based investigations.
C in computer science education
As one of the foundational languages of computer science, the C Programming Language is an essential subject in computer science curricula around the world. C programming is often taught early in the academic journey of a computer science student, providing them with essential concepts and skills that can be applied to later studies. Some of the reasons why C is highly valued in computer science education include:
- Learning the core concepts like data types, control structures, functions, and pointers, which serve as the basis for understanding more advanced languages and programming paradigms.
- Building efficient problem-solving skills through mastering algorithms, sorting techniques, and data structures in C.
- Low-level access allows students to understand the interactions between hardware and software, crucial for grasping computer organization, operating systems, and compiler design topics.
C programming in scientific research
The C Programming Language also plays a vital role in scientific research, where its efficiency, speed, and low-level capabilities make it suitable for various research purposes, such as engineering, physics, and biology simulations. Some examples of C's usage in scientific research are:
- Numerical computing applications, where the speed and precision of C enable researchers to process large datasets and perform complex calculations in domains like fluid dynamics, weather modelling and cryptography.
- Parallel and distributed computing, where the scalability of C programs allows for efficient resource management and exploitation of parallelism in high-performance computing clusters and supercomputers.
- Implementation of cutting-edge research algorithms in fields like artificial intelligence, machine learning, and computer vision, where C can be used for the development of libraries, toolkits, and low-level software.
Overall, the extensive application of the C Programming Language in various domains of the real world, academia, and research underlines its continued relevance and importance in the world of computer science and technology.
C Programming Language - Key takeaways
C Programming Language: efficient and versatile language, foundation for modern programming languages, created by Dennis Ritchie in 1972
Core concepts: data types, variables, conditional statements, loops, functions, and pointers
History: evolved from assembly, ALGOL, and BCPL, developed at Bell Labs, influenced modern programming languages
Advantages: efficiency, performance, portability, compatibility, widely used in operating systems and embedded systems
Disadvantages: lack of object-oriented features, limited debugging and error handling, manual memory management
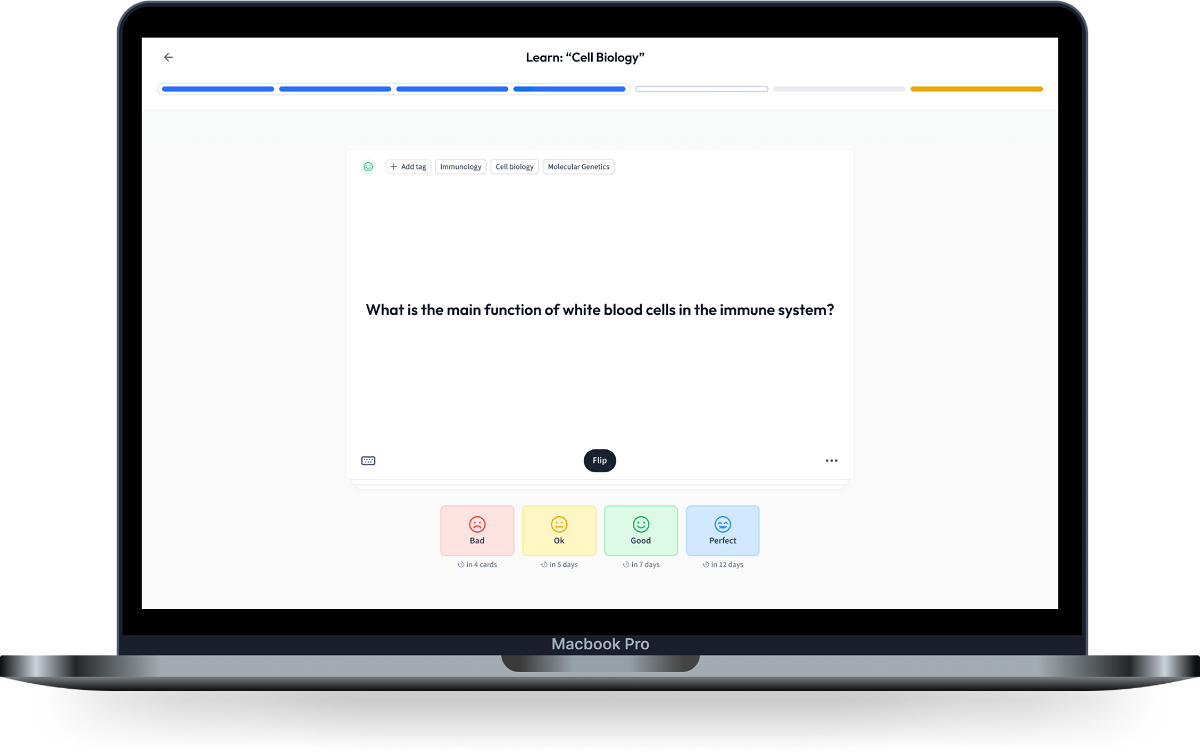
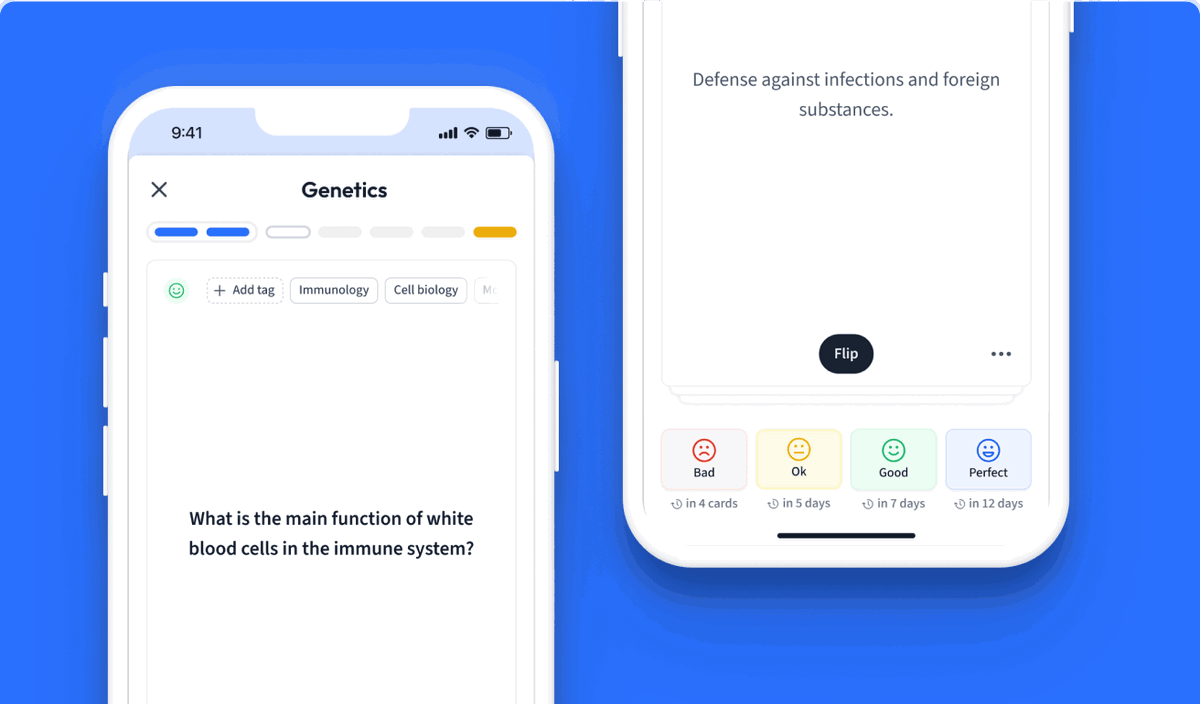
Learn with 901 C Programming Language flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about C Programming Language
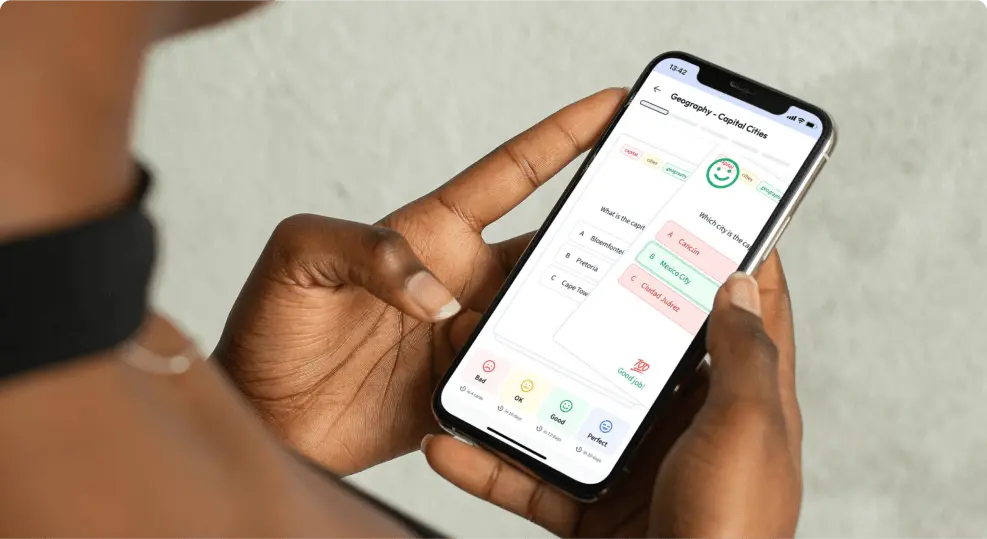
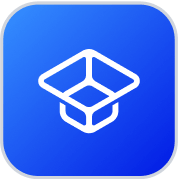
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more