Jump to a key chapter
Understanding Javascript Arithmetic Operators
Javascript arithmetic operators are invaluable tools in any developer's toolbox. These operators are used to perform mathematical calculations between variables and/or values. A firm grasp of these operators can significantly enhance your coding efficiency and broaden your problem-solving strategies.
The Basic Functions of Javascript Arithmetic Operators
Javascript comes with several arithmetic operators designed to perform various mathematical computations. These include:
- Addition (+)
- Subtraction (-)
- Multiplication (*)
- Division (/)
- Modulus (%)
- Increment (++)
- Decrement (--)
Each operator has a unique role in computation, making them indispensable in your Javascript programming.
For instance, the Modulus (%) operator is used to obtain the remainder of a division operation.
How to Use Arithmetic Operators in Javascript Effectively
To successfully employ these operators, a clear understanding of their functionality and application is necessary. Here's a practical illustration:
var x = 5; var y = 3; var sum = x + y; // Addition Operator var diff = x - y; // Subtraction Operator var prod = x * y; // Multiplication Operator var quot = x / y; // Division Operator var mod = x % y; // Modulus Operator
In the Increment and Decrement operators, the syntax format influences the operation's result. For example, using '++x' increments the value of 'x' before the remaining expressions, while 'x++' increments 'x' after evaluating the other statements in the line. This nuance is vital in controlling code execution order and outcome.
Common Misunderstandings in Javascript Arithmetic Operators Functions
It's crucial to avoid common misconceptions concerning javascript arithmetic operators. A common mistake occurs when the addition operator (+) is used with strings. In such a case, it performs string concatenation, not mathematical addition. This behaviour can lead to unexpected results if not correctly handled. Here's an example:
var str = "10"; var num = 20; var result = str + num; // result = "1020"
Going Beyond the Basic Javascript Arithmetic Operators
Once you're comfortable with using arithmetic operators for basic operations, you can incorporate more complex calculations. For instance, you can use these operators to update variables directly using combinations of assignments and arithmetic operators like +=, -=, *=, /= and %=.
Advanced Techniques using Javascript Arithmetic Operators
Consider a scenario where you want to increment a score variable by 10, instead of writing 'score = score + 10', you can shorthand it to 'score += 10'. See the example below:
var score = 50; score += 10; // score is now 60
Such techniques improve code readability and efficiency, aiding in easier debugging and code maintenance.
Real World Applications of Javascript Arithmetic Operators
Javascript arithmetic operators are essential elements in numerous real-world applications. They play a vital role in enhancing the interactivity and complexity of web applications, effectively powering the behind-the-scenes computations that enrich user experiences and functionalities.
Examples of Javascript Arithmetic Operators in Practice
Javascript arithmetic operators are at the heart of many day-to-day web computations. Whether it's tallying user scores in an online game, calculating total e-commerce shopping cart cost or determining mathematical solutions within educational apps, these operators are instrumental.
Consider an e-commerce adding items to the shopping cart functionality, the Addition (+) operator could be used to sum the total cost:
var item1 = 500; var item2 = 250; var totalCost = item1 + item2;
In an online game, the Increment (++) operator could be deployed to update the player’s score:
var score = 0; score++;
Practical Study: Javascript Program to Perform Arithmetic Operations
Let's delve into a practical study: creating a simple Javascript program to perform multiple arithmetic operations.
function performOperations(x, y) { var sum = x + y; var diff = x - y; var prod = x * y; var quot = x / y; console.log("Sum: " + sum); console.log("Difference: " + diff); console.log("Product: " + prod); console.log("Quotient: " + quot); } performOperations(10, 2);
The above function takes two parameters and performs several arithmetic operations. It's a basic example, but it lays the foundation for more complex operations within actual application development.
Arithmetic Operations in Javascript Examples: Case Studies
Let's explore a few cases where Javascript arithmetic operators shine. Understanding these real-world applications of arithmetic operators can help to grasp their potential and utility better.
// Case Study 1: Calculating Area of Circle var radius = 5; var area = Math.PI * (radius * radius); // Area is: 78.53981633974483 // Case Study 2: Calculating age from birth year var currentYear = new Date().getFullYear(); var birthYear = 1990; var age = currentYear - birthYear; // Age is: calculated based on the current year
Dissecting Real-world Examples of Javascript Arithmetic Operators
Understanding how these operators work in real-world scenarios can provide actionable insights. Let’s analyse the above case studies:
Case Study 1: Here, we calculated the area of a circle. The multiplication (*) operator was used to square the radius, then the result was multiplied by Pi (a constant in the Math object).
Case Study 2: The subtraction (-) operator is used to calculate the age from the birth year. We subtract the birth year from the current year, retrieved using the getFullYear method.
In both cases, Javascript arithmetic operators worked behind the scenes to perform the main calculations. They transform static values into dynamic, meaningful, and interactive data – underscoring their pivotally in Javascript programming.
Getting Hands-on with Javascript Arithmetic Operators
Javascript arithmetic operators are indispensable instruments for a developer. They are responsible for executing mathematical operations, fundamental to the logic and flow of a program.
Step-by-Step Guide: How to Use Arithmetic Operators in Javascript
Effectively utilising arithmetic operators in Javascript is essential. Let's break down the use of these operators into simple, manageable steps:
Step 1: First, get acquainted with the primary arithmetic operators and their functions:
- Addition (+): Adds two numbers or concatenates strings.
- Subtraction (-): Subtracts one number from another.
- Multiplication (*): Multiplies two numbers.
- Division (/): Divides one number by another.
- Modulus (%): Returns the division remainder.
- Increment (++) Increases value by one.
- Decrement (--): Decreases value by one.
Step 2: Next, understand how to implement these operators in your code. Here's an example:
var a = 10; var b = 20; var add = a + b; var sub = a - b; var mul = a * b; var div = a / b; var mod = a % b; a++; b--;
Step 3: Get well-versed with precedence rules. Arithmetic operators follow a particular order of execution, known as operator precedence. For instance, multiplication (*) and division (/) operators have higher precedence than addition (+) and subtraction(-), and they are computed left to right.
Step 4: Remember, arithmetic operators can change the operand's value, depending on the operator used. For instance, if you apply the increment operator ++ to a variable, it will increase its value by one.
Step 5: Master shorthand techniques. Productive coding involves writing efficient, concise code. Javascript offers shorthand techniques for this purpose:
var number = 10; number += 5; // equivalent to number = number + 5 number -= 3; // equivalent to number = number - 3 number *= 2; // equivalent to number = number * 2 number /= 2; // equivalent to number = number / 2 number %= 2; // equivalent to number = number % 2
Code Walkthrough: Creating a Javascript Arithmetic Operators Program
Let's create a program that implements all the arithmetic operations discussed above using Javascript:
function solveEquations(num1, num2){ var addition = num1 + num2; var subtraction = num1 - num2; var multiplication = num1 * num2; var division = num1 / num2; var modulus = num1 % num2; num1++; num2--; console.log("Addition: ", addition); console.log("Subtraction: ", subtraction); console.log("Multiplication: ", multiplication); console.log("Division: ", division); console.log("Modulus: ", modulus); console.log("Increment num1: ", num1); console.log("Decrement num2: ", num2); } solveEquations(10, 5);
In this example, the function named 'solveEquations' performs a range of arithmetic operations using Javascript's operators and logs the results in the console.
Mastering the Meaning of Arithmetic Operators in Javascript
The more comfortable you become with Javascript arithmetic operators, the more fluid your coding skills become. Indeed, these operators underlie the mathematical logic in your scripts. Let's deepen our understanding of these operators:
- Addition (+): It performs arithmetic addition if both operands are numbers. However, if one or both operands are strings, it concatenates them.
- Subtraction (-): It subtracts the right operand from the left operand. Be careful; if the operands can't be converted into numbers, it will return NaN(Not a Number).
- Multiplication (*): It multiplies the first operand with the second one. Similar to subtract, if the operands can't be converted into numbers, it will return NaN.
- Division (/): Divides left-hand operand by right-hand operand. Same as subtraction and multiplication, if the operands can't be converted into numbers, it will return NaN.
- Modulus (%): It divides left operand by the right one and returns the remainder.
- Increment (++): It adds one to its operand. If used postfix with operator after operand (for example, x++), then it increments and returns the value before incrementing.
- Decrement (--): It subtracts one from its operand. Decrement operator's behaviour is similar to the increment operator, contrasting only in that it decreases the value.
Expert Tips and Tricks for Using Javascript Arithmetic Operators Effectively
Getting the most out of Javascript arithmetic operators entails more than just a basic understanding. Follow these expert tips and tricks for effective and efficient use:
Tip 1: Use parentheses ( ) to control operator precedence in your expressions. This approach ensures that the operations are executed in the order you want.
Tip 2: The increment and decrement operators can be used in both postfix (x++) and prefix (++x) form. However, both can lead to different results in some expressions, so be aware of their use.
Tip 3: The modulus operator (%) is not just for numbers. It can also work with floating-point values. For example, 10.5 % 2 gives the result 0.5.
Tip 4: When using the addition operator with strings, if a number is first and a string second, the number is converted to a string before performing concatenation. Conversely, if the string is first, the number will be treated as a string, resulting in string concatenation, not addition.
By applying these tips, your understanding of Javascript arithmetic operators will not only be deeper, but also more practical, helping you write smarter, more efficient code.
Javascript Arithmetic Operators - Key takeaways
- Javascript arithmetic operators enable the execution of mathematical calculations between variables or values, and include addition (+), subtraction (-), multiplication (*), division (/), modulus (%), increment (++), and decrement (--), among others.
- The modulus (%) operator in Javascript is specifically used to calculate the remainder of a division operation.
- Precedence in using increment and decrement operators is highly influential in determining the operation's result. For instance, '++x' increments the value before the remaining expressions, while 'x++' increments 'x' after evaluating all other expressions.
- Understanding the meaning of arithmetic operators in Javascript is critical. For example, the addition (+) operator can perform string concatenation when used with strings, which can lead to unexpected results if not handled correctly.
- More complex calculations involve combinations of assignments and arithmetic operators, such as +=, -=, *=, /=, and %= to update variables directly. For instance, instead of writing 'score = score + 10', you can summarise it to 'score += 10'.
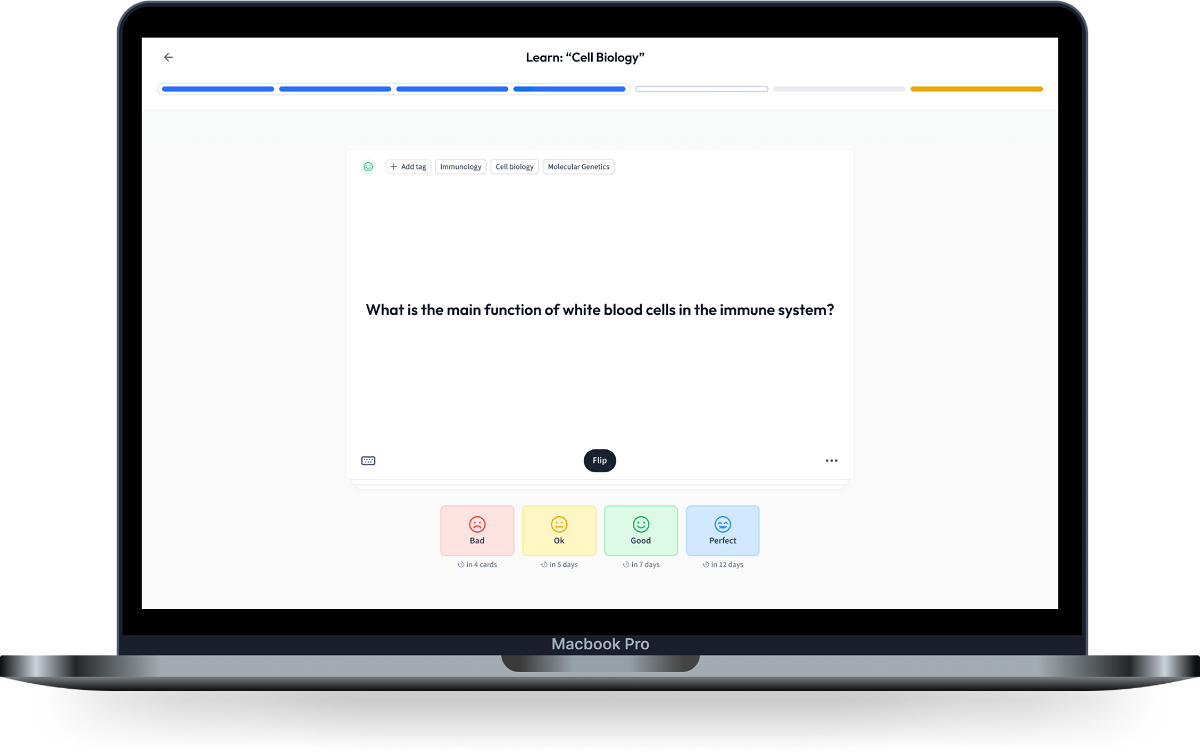
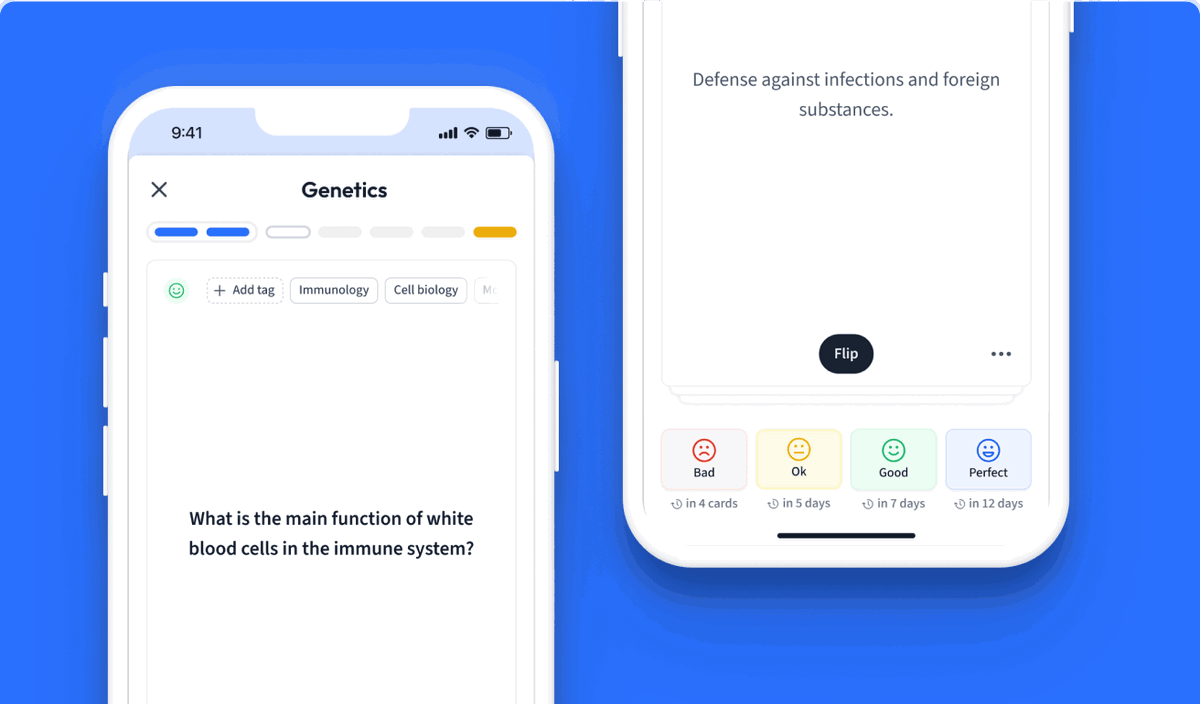
Learn with 12 Javascript Arithmetic Operators flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript Arithmetic Operators
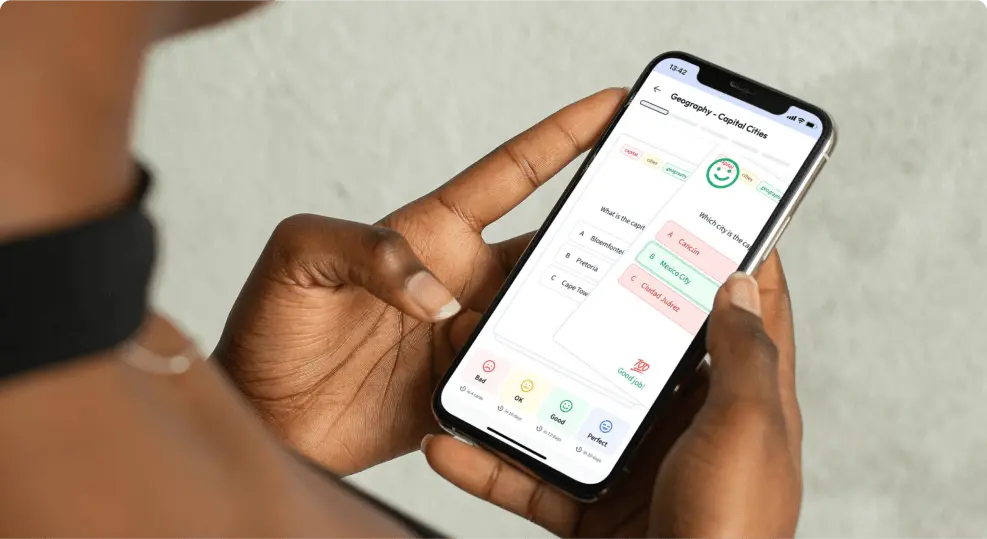
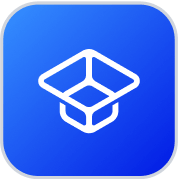
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more