Understanding Oops Concepts
Object-oriented programming, or OOP, is a programming paradigm that focuses on the use of objects and their relations to one another. Oops concepts are the fundamental principles that underpin this approach to programming, allowing developers to build efficient, robust, and scalable software.
Key Features of Oops Concepts in Computer Programming
There are several key features of Oops concepts that make this programming paradigm stand out. These features help to structure and organise code, making it easier to understand and maintain:
- Encapsulation
- Inheritance
- Polymorphism
- Abstraction
Encapsulation is the process of bundling data and methods that operate on that data within an object. This allows for greater control over what can access and modify the object's data and improves modularity.
Inheritance is the ability of one class to inherit properties and methods from a parent class. This promotes code reusability, as you can create new classes that only need to define or override the specific behaviours that are different from the parent class.
Polymorphism is the ability of a single function or method to operate on different types of data. This allows for a more intuitive and flexible way to structure code, as it permits a single interface for multiple data types.
Abstraction is the process of simplifying complex systems by breaking them down into smaller, more manageable components. By focusing on essential features and hiding implementation details, abstraction makes it easier to reason about the system as a whole.
Importance of Abstraction Oops Concept
Abstraction is a crucial aspect of the Oops concepts, as it enables developers to manage complexity more effectively. By allowing programmers to break down complex systems into simpler parts, abstraction facilitates:
- Better understanding of complex systems
- Improved maintainability of code
- Easier debugging and testing
- Efficient collaboration between team members
- Simplified integration of new features
For example, consider a software system managing a vehicle rental service. Instead of creating a single, complex class to handle all actions related to vehicles, customers, and rentals, abstraction enables you to create separate classes for each domain with specialised methods and properties. This approach makes the code easier to read and maintain, and allows for more effective collaboration between team members working on different parts of the system.
Advanced Oops Concepts and their Applications
In addition to the core Oops concepts already discussed, there are many advanced concepts that provide additional benefits and lead to more sophisticated software solutions:
- Interfaces
- Composition
- Aggregation
- Singleton pattern
- Factory pattern
Interfaces are contracts that define a set of methods a class must implement. They provide a way of ensuring consistency and interoperability between objects. In a sense, interfaces can be viewed as a more advanced form of polymorphism, as they enable multiple classes to provide the same functionality with a predefined structure.
Composition and aggregation are two related techniques for assembling objects from simpler components or building blocks. Composition represents a strong relationship between the components, with the assembled object owning or controlling the lifetime of its parts. Aggregation, on the other hand, represents a weaker relationship, with the parts having their own lifetimes independently of the 'parent' object. Both techniques allow for greater modularity and easier code maintenance.
Singleton and factory patterns are common object-oriented design patterns that facilitate the creation and management of objects in certain scenarios. The singleton pattern ensures that a class has only one instance and provides a simple way to access this instance globally. The factory pattern, meanwhile, provides a way to create instances of objects without specifying their exact class. This allows for greater flexibility and decoupling, as it makes code less reliant on specific implementations.
Oops Concepts in Python and Java
Object-oriented programming is a widely-used programming paradigm that can be found in both Python and Java languages. Understanding and mastering Oops concepts in both languages enable developers to create efficient, maintainable programs while enhancing their skill sets in object-oriented programming.
Python: Implementing Oops Concepts
Python is a versatile, high-level language that supports both object-oriented programming and procedural paradigms. Implementing Oops concepts in Python can be achieved by understanding its key components such as the class and object creation, inheritance, encapsulation, polymorphism, and abstraction.
Exploring Oops Concepts in Python for Beginners
For those new to Python or object-oriented programming, there are a few fundamental Oops concepts to understand:
- Classes and Objects: In Python, classes are blueprints that define the layout and behaviour of objects, which are instances of those classes. Object creation involves defining a class and creating instances of that class. Instances have properties (attributes) and behaviours (methods).
- Inheritance: One of the key pillars of Oops, inheritance allows a new class (subclass) to inherit characteristics and functionality from an existing class (parent class or superclass). This promotes code reusability and modularity, as the subclasses only need to add or override specific behaviours and properties.
- Encapsulation: This concept bundles data and methods that operate on that data within an object. Encapsulation helps to restrict access to and modification of the object's internal data. To achieve encapsulation, use private and protected access modifiers for attributes and use public methods to interact with the private/protected attributes.
- Polymorphism: Python supports polymorphism, which enables a single method to work with multiple types of data. In Python, polymorphism is mainly achieved through duck typing, which allows objects to be treated as if they belong to a certain type, as long as they implement the required methods.
- Abstraction: Python supports abstraction through abstract base classes (ABC) and interfaces. An abstract class provides an interface for concrete classes, which implement their methods and properties. Abstract methods enforce their implementation in subclasses, ensuring that each derived class must provide a specific functionality.
Advanced Oops Concepts in Python: Dive Deeper
For those looking to enhance their understanding of Oops concepts in Python, several advanced topics can be explored:
- Composition and Aggregation: These techniques combine objects to create more complex objects. Composition involves strong relationships between objects, with the parent object controlling the lifetime of its parts. Aggregation represents a weaker relationship where objects have their own lifetimes independent of their parent object.
- Multiple Inheritance: Python supports multiple inheritance, allowing a class to inherit features from more than one parent class. This enables greater flexibility in creating complex class hierarchies but requires careful consideration to avoid potential pitfalls such as the diamond problem.
- Operator Overloading: Python allows developers to redefine the behaviour of built-in operators for user-defined classes. This enhances code readability and allows instances of custom classes to use familiar Python syntax for performing operations.
- Decorators: Python decorators provide a powerful concept that enables the modification of the behaviour of functions or methods. Decorators can be used to implement aspects such as memoization, access control, or logging without altering the original function's code.
Java: Mastering Oops Concepts
Java is an object-oriented language that revolves around Oops concepts. By grasping these concepts in Java, developers can build powerful, maintainable, and scalable applications. Java's key components include class and object creation, inheritance, encapsulation, polymorphism, and abstraction.
Introduction to Oops Concepts in Java
For those beginning their Java journey, there are essential Oops concepts to learn:
- Classes and Objects: Java uses classes and objects to define software components. Classes provide structure and behaviour for objects, while objects are instances of classes. Java supports instance variables for storing data and instance methods for implementing behaviour.
- Inheritance: Java allows classes to inherit properties and methods from parent classes through the use of the 'extends' keyword. Inheritance promotes code reuse, as subclasses can inherit common code from the superclass, reducing the need to rewrite similar code.
- Encapsulation: By bundling data, methods, and access control mechanisms within objects, encapsulation offers a high level of code protection and organization. In Java, this is achieved through the use of private/protected instance variables and public accessor and mutator methods.
- Polymorphism: Java supports polymorphism by allowing methods to operate on different data types. Polymorphism in Java can be achieved through method overloading (static polymorphism) or method overriding (dynamic polymorphism), enabling greater flexibility and code management.
- Abstraction: Java allows class abstraction through abstract classes and interfaces. Abstract classes can implement partial functionality and enforce method declarations on subclasses without providing an implementation. Interfaces define method signatures that implementing classes must adhere to, ensuring consistent functionality across different classes.
Advanced Java Programming using Oops Concepts
For those aiming to expand their Java knowledge, several advanced Oops concepts can be studied:
- Composition and Aggregation: These techniques assemble objects to form more complex entities. Composition represents a strong relationship between objects, with the parent object owning and controlling its parts. Aggregation signifies a weaker relationship where objects can exist independently of the parent.
- Interfaces and Multiple Inheritance: Java supports multiple inheritance through interfaces, allowing classes to implement features from several interfaces concurrently. This enables greater flexibility in creating complex class hierarchies.
- Generics: Java generics allow for generic programming, providing parameterised types for collections and other classes. Generics improve type safety and code maintainability by allowing you to define classes, interfaces, and methods that work with different data types.
- Design Patterns: Design patterns in Java are reusable solutions to common problems encountered in software design. These patterns provide tried and tested solutions, enabling developers to build robust, scalable, and efficient software systems. Examples of design patterns include the Singleton, Factory, and Observer patterns.
The 4 Pillars of OOP
The four pillars of Object-Oriented Programming (OOP) are fundamental principles that guide the practice and structure of object-oriented code. These core concepts lead to maintainable, robust, and modular software designs that aid programmers in effectively managing complex systems.
Understanding the 4 Concepts of OOP
Object-Oriented Programming (OOP) offers a unique approach to programming by allowing developers to organise code around objects and their interactions. To truly grasp OOP, you must understand the four core concepts: Encapsulation, Inheritance, Polymorphism, and Abstraction. These concepts serve as guiding principles, enabling a smooth coding experience and better systems organisation.
Encapsulation: Organising Data and Functions
Encapsulation is a crucial concept in OOP, responsible for reducing complexity and improving code maintenance. It refers to the bundling of data (attributes) and functions (methods) that operate on the data within an object, controlling access to object properties. Through encapsulation:
- Code becomes more modular and maintainable
- Data integrity is maintained by restricting direct access to object attributes
- Implementation details are hidden to reduce dependencies and coupling
- Public interfaces (APIs) are defined for interacting with objects
Encapsulation is achieved by employing access modifiers, such as private and protected, to restrict direct access to attributes. Public methods, known as getters and setters, are used to manage the access and modification of private data, ensuring that all interactions with the object are controlled and secure.
Inheritance: Creating New Classes from Existing Ones
Inheritance is another essential concept in OOP, promoting code reusability and modularity by enabling new classes (subclasses) to derive properties, attributes, and behaviour from existing classes (base or parent classes). Inheritance provides several benefits:
- Eliminates code redundancy by reusing functionality from parent classes
- Enhances code organisation by forming class hierarchies
- Facilitates easy updates and code maintenance
- Increases productivity by reducing time spent rewriting common attributes and methods
To exploit inheritance effectively, the base class should be carefully designed with well-defined interfaces, clean and reusable code, and preferably be tested for robustness. Subclasses can inherit the base class's features, while extending, modifying, or overriding its behaviour based on their specific requirements.
Polymorphism: Many Forms of a Single Function
Polymorphism is the OOP concept that allows a single function or method to operate on different types of data or objects, leading to more flexible and intuitive code designs. Polymorphism can be achieved in various ways, including:
- Method Overloading: Having multiple methods with the same name but different parameter lists enables static polymorphism. The correct method is selected at compile time based on the number and types of arguments passed.
- Method Overriding: Involves redefining a method inherited from a parent class in a derived class, facilitating dynamic polymorphism. The appropriate method is selected at runtime depending on the object's actual type.
- Interfaces and Abstract Classes: Ensuring the consistent implementation of functionalities across objects by defining method signatures. Different types of objects can be processed using the same code as long as they implement the required methods.
Polymorphism contributes to efficient code management as it eliminates the need for lengthy if-else or switch-case structures to handle different object types. Instead, you can rely on well-defined interfaces and method implementations to achieve desired behaviours.
Abstraction: Simplifying Complex Systems
Abstraction is the process of simplifying complex systems through the creation of smaller, more manageable components. It involves focusing on essential features while hiding implementation details, making it easier to reason and collaborate on systems. Key aspects of abstraction include:
- Providing a high-level view of a system without going into low-level implementation complexities
- Defining interfaces and abstract classes to establish contracts between components
- Improving code maintainability by breaking systems into smaller, well-defined modules
- Enabling developers to build upon existing functionality without understanding all the underlying intricacies
Abstraction can be achieved through designing modular code and utilising abstract classes or interfaces. Abstract classes serve as a template for derived classes, providing partial implementation and enforcing that specific methods should be implemented. Interfaces, on the other hand, only define method signatures, ensuring that all implementing classes adhere to a standard protocol. Both approaches facilitate a cleaner code design and promote collaboration between teams by abstracting away complexity.
Learning the Abstraction Oops Concept
Abstraction is a fundamental principle in object-oriented programming. It revolves around the process of simplifying complex systems into smaller, more manageable components by focusing on essential features and hiding unnecessary implementation details. Understanding and mastering abstraction is vital for building efficient and maintainable software systems in languages such as Python and Java.
The Role of Abstraction in Oops Concepts
In the context of Oops concepts, abstraction plays a crucial role in organising and simplifying codebase. It helps programmers to:
- Break down complex systems into smaller components, improving readability and maintainability
- Hide implementation details, allowing developers to understand and interact with the system without being overwhelmed by complexity
- Establish contracts between components through the use of interfaces and abstract classes, ensuring consistent behaviour across different classes
- Enable efficient code reuse through inheritance and composition
- Enhance collaboration among team members by providing high-level, easily understandable classes and interfaces
Benefits and Importance of Abstraction
Abstraction brings numerous benefits to software development, making it an indispensable concept in Oops:
- Code Organization and Simplicity: Abstraction enables the structuring of code into smaller components, making it more modular and straightforward to manage.
- Improve Comprehension: By hiding low-level details and focusing on essential features, abstraction allows developers to understand the system's high-level view, improving overall comprehension.
- Reduce Dependencies: Hiding implementation details and exposing only essential interfaces help reduce dependencies between components, leading to more flexible and maintainable code.
- Code Reusability: By providing a clear structure and promoting inheritance, abstraction allows developers to reuse existing code more efficiently, reducing the need for duplicating efforts.
- Team Collaboration: By creating clean and understandable interfaces, abstraction fosters better collaboration among team members who can work on different parts of the system simultaneously.
Implementing Abstraction in Python and Java
Abstraction can be implemented in various programming languages, specifically in Python and Java, through different mechanisms:
- Python:
- Abstract Base Classes (ABCs) in Python are created by inheriting the
abc.ABC
class and using the@abstractmethod
decorator for declaring abstract methods. - Abstract methods in ABC must be implemented in any concrete class that inherits the abstract class.
- Interfaces can be created in Python by defining methods without implementations and using the ABC mechanism.
- Abstract Base Classes (ABCs) in Python are created by inheriting the
- Java:
abstract
keyword in Java is used to create an abstract class. Abstract methods are declared using the same keyword, but without any implementation.- Subclasses of the abstract class must provide an implementation for all abstract methods.
- Interfaces in Java are declared using the
interface
keyword. They contain abstract methods that must be implemented by the classes that implement the interface.
Using abstraction in both Python and Java leads to clearer, more maintainable, and scalable codebases while enabling better collaboration among development teams and promoting code reuse. Embracing abstraction as a fundamental principle of Oops concepts will aid developers in designing and building robust software systems.
Advanced Oops Concepts for Expert Programmers
As an expert programmer, taking your knowledge of object-oriented programming to an advanced level will help you create more sophisticated and efficient software solutions for complex real-world applications. Delving into advanced techniques, design patterns, and best practices in languages such as Python and Java allows you to effectively implement advanced Oops concepts, maximising your potential in this programming paradigm.
Building Expertise in Advanced Oops Concepts
Developing expertise in advanced Oops concepts involves exploring various techniques and practices that improve code organization, modularity, and reusability. From mastering advanced inheritance mechanisms to implementing design patterns, expert programmers can tackle complex challenges and create efficient solutions by leveraging advanced Object-Oriented Programming (OOP) concepts:
- Mixins and Multiple Inheritance
- Composition and Aggregation
- Generics and Type Parameterisation
- Design Patterns
- Software Architecture and Design Principles
Advanced Techniques in Python and Java
To implement advanced Oops concepts in Python and Java programming languages, expert programmers should explore a variety of techniques and strategies:
- Mixins and Multiple Inheritance: Mixins in Python are a way to reuse a class's functionality and combine it with that of multiple parent classes. Similarly, Java's interface allows for multiple inheritance, enabling more versatile combinations of class behaviours.
- Composition and Aggregation: In both Python and Java, composition and aggregation are techniques to assemble objects into more complex entities. Composition involves strong relationships between objects, whereas aggregation uses weaker relationships where objects maintain independent lifetimes.
- Generics and Type Parameterisation: Python allows for generic programming using type hints, whereas Java has its own powerful generics system. These features allow you to define classes and methods with variable type parameters, enhancing code reuse and type safety.
- Design Patterns: Expert programmers can utilise design patterns, which are reusable solutions for common problems in software design. Examples of popular design patterns include Adapter, Factory, Singleton, Observer, and Decorator patterns.
- Software Architecture and Design Principles: Good software architecture is vital for building maintainable and reusable software solutions, and expert programmers must strive to adhere to fundamental OOP design principles such as SOLID, GRASP, and DRY to achieve this.
Real-World Applications of Advanced Oops Concepts
Mastery of advanced Oops concepts empowers expert programmers to tackle real-world application challenges. These advanced concepts play a pivotal role in a range of industries, including:
- Enterprise Software Solutions: Advanced Oops concepts facilitate the development of robust and scalable business software such as Enterprise Resource Planning (ERP) systems, Customer Relationship Management (CRM) systems, and e-commerce platforms.
- Game Development: Object-oriented programming can improve the structure and organization of game code. Advanced concepts such as design patterns, composition, and aggregation help create complex game components, manage object hierarchies, and allow better collaboration among development teams.
- Machine Learning and Data Science: Oops concepts play a crucial role in streamlining machine learning and data science code. Advanced techniques such as generic programming help create reusable algorithms and data structures that can operate on a variety of data types and formats.
- Web and Mobile Applications: With a multitude of libraries and frameworks for web and mobile development, mastering advanced Oops concepts ensures that expert programmers can create efficient, maintainable, and scalable applications with improved performance and user experience.
Undoubtedly, advanced Oops concepts have significant real-world implications, enabling expert programmers to tackle complex challenges across diverse industries and build efficient, maintainable, and reusable software solutions. Increase your potential as an expert programmer by mastering these advanced concepts and putting them into practice in different programming languages and applications.
Oops concepts - Key takeaways
Object-oriented programming (OOP) and key principles (Oops concepts)
Four pillars of OOP: Encapsulation, Inheritance, Polymorphism, and Abstraction
Abstraction: Simplifying complex systems, interfaces, and abstract classes
Advanced Oops concepts: Mixins, Composition, Aggregation, Generics, and Design Patterns
Implementing Oops concepts in Python and Java, including key components and techniques
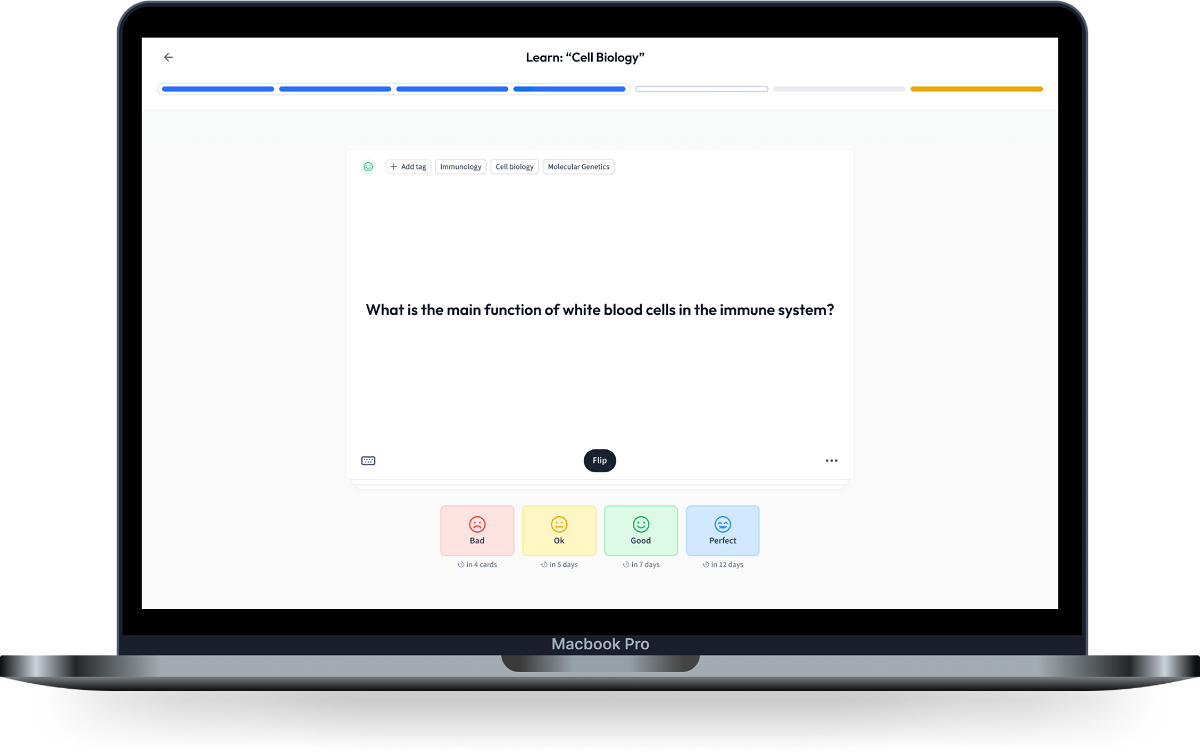
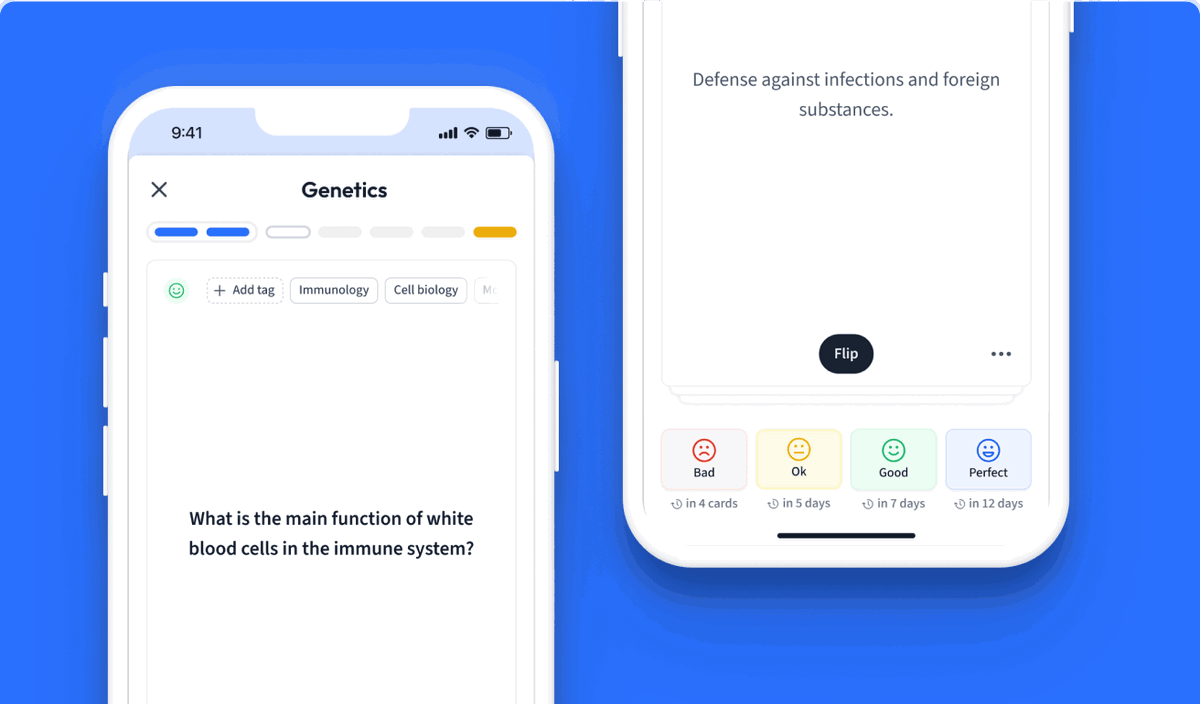
Learn with 11 Oops concepts flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Oops concepts
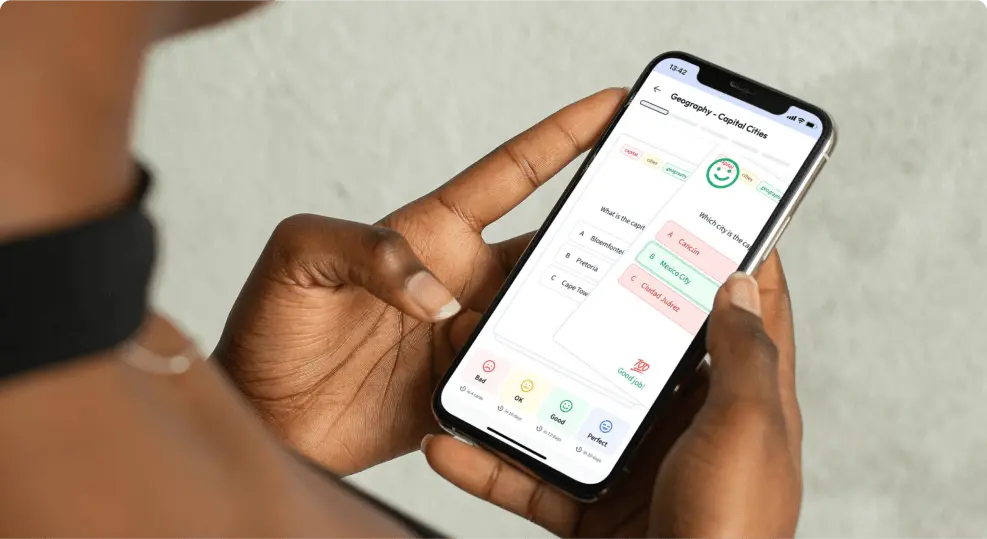
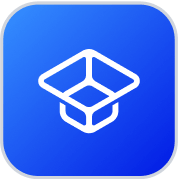
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more