Understanding Javascript Scopes
As a core concept in programming, understanding Javascript scopes is vital for efficient coding. Let's delve deeper into this crucial Computer Science topic.
What is Scope in Javascript: A Basic Introduction
Scope in Javascript, or in any other programming language, refers to the accessibility or visibility of variables, functions, and objects during a particular part of the code during run time.
Global Scope | A variable declared outside a function has a global scope and can be accessed from anywhere in your code |
Function or Local Scope | Variables declared within a function have a function or local scope and can only be accessed from within the function they are declared |
Scope is a vital concept as it manages the availability of variables without which the operations of the code could falter.
The Concept of Scope in Programming
The concept of scope in programming is integral for managing and controlling how variables and function calls are accessed throughout your code.
// Global Scope var a = 1; function example() { // Local Scope var b = 2; }
In this example, variable \(a\) is in the global scope and can be accessed anywhere in our code. Contrastingly, variable \(b\) is in the local scope of function `example` and can only be accessed within this function.
The importance of scope management in programming cannot be overstated. Properly managing scope can lead to more maintainable and bug-free code.
Javascript Scope Definition And Importance
In the context of Javascript, scopes can be classified as global, local, or block scopes.
- Global Scope: Variables or functions declared outside a function or a block have a global scope.
- Local Scope: Variables or functions defined within a function have a local scope.
- Block Scope: With the introduction of `let` and `const` in ES6, Javascript now supports block scoping. A block is any section of code surrounded by `{}` characters.
Block Scope: Features introduced in Javascript ES6 that allows variables and constants to be scoped to the block in which they were declared, rather than to the containing function.
Understanding how we declare variables in Javascript and the scope that those variables take on within our code is a crucial part of learning JavaScript. These differences in scope help keep our code clean and eliminate potential conflicts in naming.
Delving into Javascript Scope Types
As we navigate the intricate landscapes of Javascript scopes, we need to examine two fundamental types of scopes: Block Scope and Function Scope. An understanding of these two scope types will equip you to handle variables and their reach more adeptly in your code.
Block Scope vs Function Scope in Javascript: A Comparison
You can encounter two main types of scopes as a Javascript developer, namely Function Scope and Block Scope. Both scopes represent the area where you can access a variable or a function. However, they differ on the basis of their declaration and accessibility.
Function Scope : When you declare variables using the `var` keyword within a function, they are accessible only within that function. This is known as function scope.
Block Scope: With ES6, Javascript introduced new keywords like `let` and `const` for variable declarations. When you declare a variable using `let` or `const` inside a block (any code inside `{ }`), that variable is accessible only within that block. This scope is called block scope.
- In function scope, variables are hoisted to the top of its containing function.
- In block scope, variables are hoisted to the top of its containing block.
These differences in scope management play vital roles in maintaining the precedence and visibility of variables in our code.
Understanding Block Scope in Javascript
Before ES6, JavaScript didn't embrace the block-level scope, widening the potential for hoisting issues or unintentional overwriting of variables. The introduction of `let` and `const` significantly altered this scenario. Declaring a variable with `let` or `const` confines the scope of the variable to the immediate enclosing block denoted by `{ }`.
Here's an interesting feature of block-level scope. When you use a block scope variable before its declaration, the JavaScript engine does not raise a ReferenceError. Instead, it results in a Temporal Dead Zone error. This peculiar circumstance is unique to block-scope variables.
{ console.log(blockScopeVariable); // ReferenceError: blockScopeVariable is not defined let blockScopeVariable = 'Hello Block Scope'; }
What precisely makes the block scope underscore its uniqueness is the notion of temporary dead zones, which helps avert a common source of errors and bugs in our JavaScript code.
Exploring Function Scope in Javascript
Unlike block scope, function scope confines the accessibility of variables declared with the `var` keyword to the function in which they're declared. If they're defined outside of any function, they have a global scope. This means you can access them anywhere in your script.
Here's an important point worth noting. If you try to use the variable before its declaration, you won't land with the Temporal Dead Zone. Instead, it'll render `undefined`.
function exampleFunction() { console.log(functionScopeVariable); // returns undefined var functionScopeVariable = 'Hello Function Scope'; } exampleFunction();
The main reason behind this difference is the term 'Hoisting'. Hoisting is a JavaScript mechanism where variables and function declarations are moved to the top of their containing scope. For 'var' variables, this means they can appear defined (as undefined) before they're actually declared in the code.
By understanding the distinction between block and function scope in Javascript, you're better equipped to avoid common mistakes, making your code more reliable and maintainable.
Working with Javascript Scopes
Understanding and working with Javascript Scopes is a fundamental part of programming. This involves a deep comprehension of how variables and functions are accessed during different parts of the code while it's executing. In the following sections, we'll delve into the mechanics of how Javascript scopes operate and their key role in Javascript programming.
How Javascript Scopes Work: An In-depth Analysis
The Javascript engine uses scopes to look up variables. When your code is executed, the engine creates a Global Execution Context. Initially, this comes in two phases – the Creation Phase and the Execution Phase.
Creation phase: In this phase, the Javascript engine moves (hoists) all variable and function declarations to the top of their respective containing scope. In the case of variables, only the declaration is moved to the top, leaving the assignment in place. The variables initially have a value of `undefined`.
Execution phase: In this phase, the Javascript engine runs through your code line by line and assigns the values to the variables and executes the function calls.
It is essential to understand that each function invoked during the code execution creates a new Function Execution Context. They follow the same two-phase rule, adding a layer of complexity for the Javascript engine when handling function calls.
Each execution context has a reference to its outer environment. If the JavaScript engine can't find a variable within the immediate execution context, it moves to the next outer environment to look up the variable, and so on. This is known as the Scope Chain.
Scope Chain: The Scope Chain in JavaScript is a list of objects that are searched sequentially whenever a variable is referenced. The Scope Chain essentially decides the accessibility or scope of a variable.
var globalVar = 'I am a global variable'; function outerFunc() { var outerVar = 'I am an outer variable'; function innerFunc() { var innerVar = 'I am an inner variable'; console.log(innerVar); // I am an inner variable console.log(outerVar); // I am an outer variable console.log(globalVar); // I am a global variable } innerFunc(); } outerFunc();
In this example, the function innerFunc
has access to its own scope, the outer function's scope and the global scope. This is determined by the Scope Chain.
Mechanism Behind Javascript Scopes
At the heart of Javascript scopes is the mechanism of how Javascript recognises and handles each scope. This involves various actions taken by the Javascript engine upon variable or function declaration and execution.
When the Javascript engine encounters a script, it goes through the compilation phase. During this phase, the engine recognises where and how all the variables and functions are declared. And based on the variables and functions' positions and how they are declared, the engine decides the scope for these variables and functions.
- Any variable declared with
var
is function scoped. This means that if this variable is declared inside a function, that variable is only available within that function. If there is no enclosing function, then the variable has a global scope. - Variables declared with
let
and constants declared withconst
are block scoped. Meaning, these are only available within the immediate block they are declared in.
function testFunction(){ var functionVariable = 'var'; if(true){ var innerFunctionVariable = 'inner var'; let blockVariable = 'let'; const blockConst = 'const'; } console.log(functionVariable); // 'var' console.log(innerFunctionVariable); // 'inner var' console.log(blockVariable); // ReferenceError: blockVariable is not defined console.log(blockConst); // ReferenceError: blockConst is not defined } testFunction();
In the above code, notice that variables blockVariable
and blockConst
are only available within the if
statement block, and are inaccessible outside of it.
The Role of Scopes in Javascript Programming
Savvy manipulation of Javascript scopes takes programming proficiency from adequate to remarkable. From avoiding collisions with global object properties, to optimising memory usage by releasing local variables when not in use, scopes play an integral role in Javascript scripting. They also contribute to code cleanliness, structure, and security.
The scopes ensure variables' lifetime, governing when they're created, accessed, or destroyed. Especially for big codebases and applications, prudent use of scopes can significantly impact code performance.
A proper understanding of global and local scopes, and immaculate use of block-level scope (offered by let and const), can help in avoiding variable naming conflicts, and reducing the risk of overwriting variables accidentally.
- Minimising the polluting of global scope: Every variable that is not inside a function scope technically lives in the global scope. Globally scoped variables can be overwritten by any part of the app. Therefore, limiting the use of global variables is the recommended practice in scripting, to avoid inadvertent and hard-to-detect errors.
- Effectively managing memory: Javascript scopes also play an important role in memory management. Local variables exist only within their scope and are removed from memory once their function scope is exited. This automatic deallocation of memory is beneficial in memory-constrained environments.
Thus, understanding and effectively utilising scopes is a critical skill for any Javascript programmer looking to polish their coding skills and construct robust, efficient and scalable Javascript applications.
Practical Applications of Javascript Scopes
Javascript Scopes are a fundamental part of code organisation and variable management. They govern the visibility and accessibility of variables and parameters in specific blocks of code. Understanding scopes and their applications can greatly influence the structure, functionality, and performance of a program.
Examples of Javascript Scopes in Real-life Coding
In programming, your ability to utilise Javascript scopes accurately and effectively can make a difference in the code's antifragility and efficiency. To truly comprehend the function of scopes in real-world programming, it's best to look at a few examples.
To set the stage, here's a basic example without using any concepts of scope, presented alongside a similar example which utilises the scope.
// Example without using scope var total = 0; for(var i = 0; i < 3; i++) { total += 10; } console.log(total); // 30 console.log(i); // 3
// Example with using scope let total = 0; for(let i = 0; i < 3; i++) { total += 10; } console.log(total); // 30 console.log(i); // ReferenceError: i is not defined
In the first example, the variable i
is globally accessible despite being used only in the loop. By declaring i
using var
, it is available even outside the loop, making your code susceptible to potential conflicts.
In the second example, by declaring i
with let
, it's only accessible within the loop, which is all that's needed. After the loop, an attempt to access i
leads to a ReferenceError
. This is a good practice because it avoids pollution of the global scope.
Practical Example: Using Block Scope
Block scope is the area within if, switch conditions, or for and while loop statements. Variables declared with the keyword let
have block scope, they exist only within that block. Here's an illustrative example:
function someFunction() { let someVariable = "Hello, world!"; // Block scoped variable if(true) { let someVariable = "Hello, universe!"; // Another block scoped variable console.log(someVariable); // "Hello, universe!" } console.log(someVariable); // "Hello, world!" } someFunction();
Inside the function someFunction
, we define a block scoped variable someVariable
with a value of "Hello, world!". Later, within the if-statement, another block scoped variable with the same name but different value is defined. Because of block scoping in Javascript, these two variables, despite having the same name, don't overlap. So, within their respective blocks, they keep their assigned values.
Practical Example: Using Function Scope
Function-scope means the variable's scope is restricted to the boundaries of the function it's defined within. Variables declared with var
obey function scoping. Let’s look at a realistic coding example of function scope.
function calculateTotal(cartItems) { var total = 0; for (var i = 0; i < cartItems.length; i++) { total += cartItems[i].price; } return total; } console.log(calculateTotal([{ price: 50 }, { price: 100 }])); // 150 console.log(total); // ReferenceError: total is not defined console.log(i); // ReferenceError: i is not defined
In this shopping cart example, both total
and i
variables are function scoped, usable only within the calculateTotal
function. Outside calculateTotal
, both i
and total
variables are not accessible, maintaining the integrity of the code's structure and helping towards cleaner, neater code.
Each time careful use of block or function scope keeps variables contained and only accessible where necessary, your code becomes more robust and less prone to bugs. Comprehending these Javascript Scopes concepts and employing them appropriately allows for optimum code organisation and superior performance.
Javascript Scopes - Key takeaways
- Javascript Scopes: Variables or functions in Javascript can have a global, local, or block scope, depending on where they are declared.
- Global Scope: Variables or functions declared outside a function or a block have a global scope.
- Local/Function Scope: Variables or functions declared within a function have a local or function scope. If a variable declared with 'var', it is accessible only within that function.
- Block Scope: A variable declared with 'let' or 'const' inside a block (any code inside `{}`) is only accessible within that block. This scope was introduced in Javascript ES6.
- Scope Chain: If the JavaScript engine can't find a variable within the immediate execution context, it looks in the next outer environment. This sequential lookup is known as the Scope Chain.
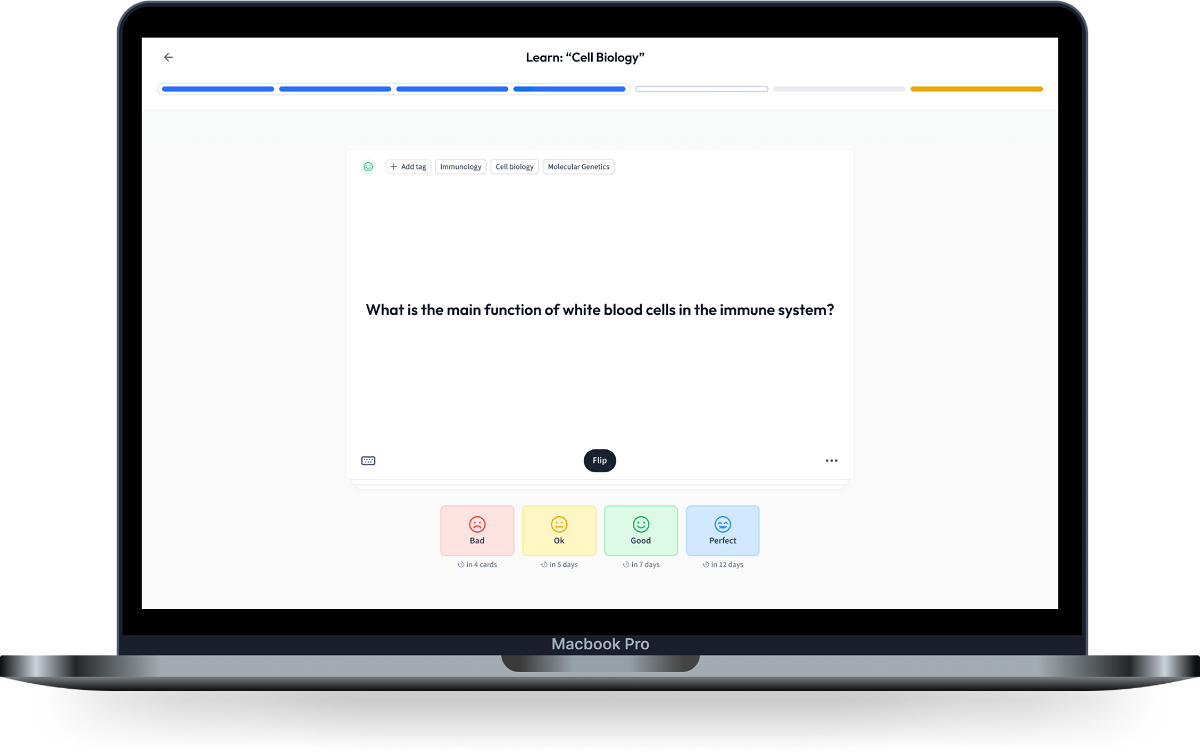
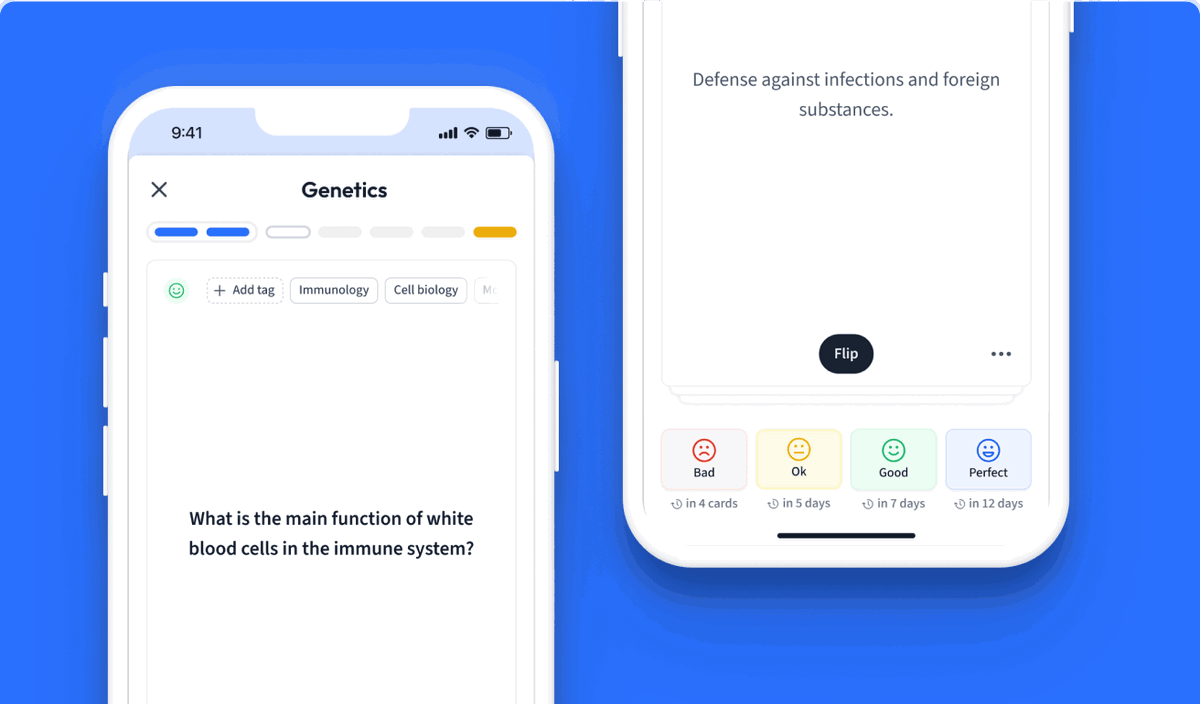
Learn with 12 Javascript Scopes flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript Scopes
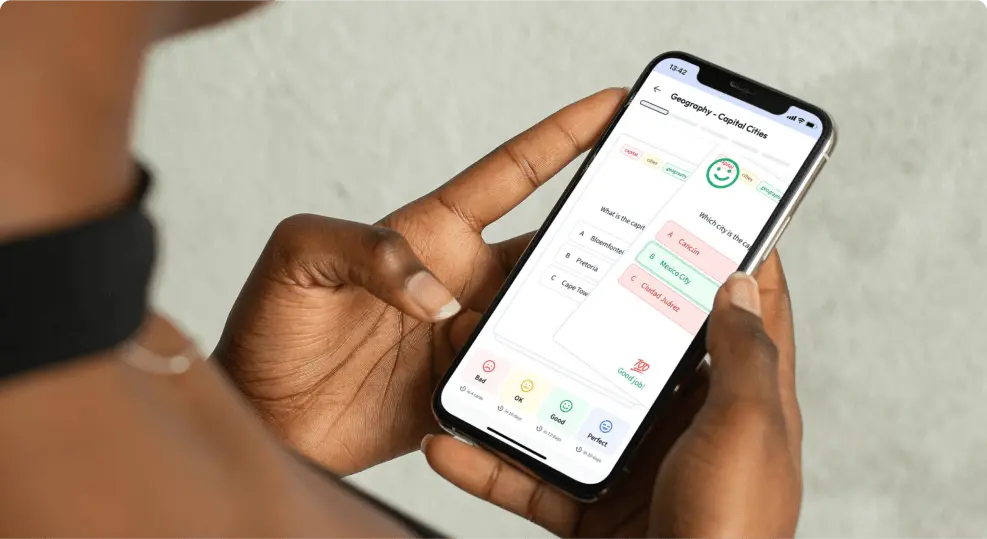
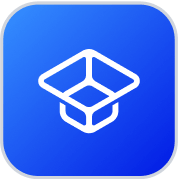
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more