Understanding Javascript DOM Manipulation for Beginners
Javascript DOM (Document Object Model) Manipulation is a foundational concept in computer science where you, a budding programmer, can modify website content, alter its structure, and change styles directly with the help of JavaScript. But before we dive deeper into the concept of DOM manipulation, let's set a solid groundwork by understanding what DOM actually is.What is DOM Manipulation Methods in JavaScript
The DOM (Document Object Model) is essentially a platform and language-neutral interface that allows scripts to dynamically access and update content, structure, and style of a document.
- Adding, modifying or deleting elements and attributes
- Changing the style or content of an element
- Reacting to an event happening on an element such as a mouse click
getElementById() | querySelector() |
createElement() | createTextNode() |
appendChild() | removeChild() |
replaceChild() | insertBefore() |
var element = document.createElement("h2"); var textNode = document.createTextNode("Hello World!"); element.appendChild(textNode); document.body.appendChild(element);
It's critical to note that modifying the DOM can become relatively expensive in terms of a page's performance. Therefore, it's a good idea to minimise direct manipulation of the DOM where possible. Virtual DOMs, such as those used by ReactJS, are a popular solution to this issue.
Fundamentals of DOM Manipulation in JavaScript
To unlock the full potential of Javascript DOM Manipulation, you must understand its two essential components: Nodes and Elements. These two are some of the building blocks of web pages and understanding them will hugely improve how you interact with websites.In the context of the Document Object Model, Nodes are any type of object within the DOM hierarchy, and Elements are a specific type of node that corresponds to the HTML tags in the markup language.
- Element nodes
- Text nodes
- Comment nodes
- getElementById
- getElementsByClassName
- getElementsByTagName
- querySelector
- querySelectorAll
tag in your HTML:
var para = document.querySelector('p'); para.textContent = "New text!";
tag it finds in the Document and then changes its contents to "New text!".
Practical Approach to Advanced DOM Manipulation JavaScript
Understanding the DOM and manipulating it are two different things, and even though having a theoretical understanding of the DOM structure is critical, you can only truly master it by diving deep into the practical aspects. Manipulating the DOM involves an intricate understanding of not just how the DOM works, but using the different methods, properties, and events to interact with the elements present on the page.Components of Advanced DOM Manipulation JavaScript
DOM Manipulation is based on some core components. Understanding these components will make DOM manipulation more comprehensible and approachable. Let's look at some fundamental components:Nodes and Elements: Almost everything in the HTML DOM is a node: the document itself is a document node, all HTML elements are element nodes, all HTML attributes are attribute nodes. Text inside HTML elements are text nodes. Comments are comment nodes.
- Click
- Load
- Mouseover
- Keypress
querySelectorAll() | createTextNode() |
querySelector() | innerText |
getElementById() | innerHTML() |
Using DOM Manipulation Techniques in JavaScript
Effective use of DOM manipulation in JavaScript involves understanding how and when to apply different methods and properties, and when to listen for certain events. Here are a few techniques: Adding or Deleting Nodes: When you have a complex web page with many elements, there may be a need to add or delete elements programmatically. There are various methods available in JavaScript to do so. Adding a node can be done with methods like createElement() and appendChild():var newEl = document.createElement('li'); var newText = document.createTextNode('Quinoa'); newEl.appendChild(newText); var position = document.getElementsByTagName('ul')[0]; position.appendChild(newEl);In this example, the code first creates a new list item and a new text node. It then appends the text node to the list item, and finally appends the list item to the unordered list in the HTML. Changing Attributes: Sometimes, the attribute of an element might need to be changed based on certain conditions or events. JavaScript allows you to do so using methods like getAttribute() and setAttribute().
var element = document.querySelector('img'); var sourceAttr = element.getAttribute('src'); element.setAttribute('src', 'newImage.png');Changing Styles: Another powerful aspect of DOM manipulation in JavaScript comes from its ability to alter the styles applied to an HTML element. This can be done using the style property.
var element = document.querySelector('p'); element.style.color = 'blue'; element.style.fontSize = '20px';In this example, we first select the paragraph element, then change its color to blue and font size to 20 pixels using the style property. Effectively manipulating the DOM is a key skill for any JavaScript developer. These techniques - adding and deleting nodes, changing attributes, and altering styles - are each vital tools you can utilise to build interactive and dynamic web pages. Practice using these methods and properties to become more comfortable with DOM manipulation and use JavaScript to its full potential!
Illuminate Your Skills with DOM Manipulation JavaScript Examples
The best way to master Javascript DOM Manipulation is by practicing and understanding real-life examples. These examples encompass various practical techniques and methods you can use to interact with websites in dynamic ways. You'll see how you can access HTML elements, modify them, add or delete elements, and change styles or attributes using the power of JavaScript.Real-life DOM Manipulation JavaScript Examples
In this section, you'll learn about three practical examples of DOM Manipulation using JavaScript. DOM Manipulation Case 1: Creating and Adding a New Element This example illustrates the use of the `createElement()`, `appendChild()`, and `createTextNode()` methods.var newElement = document.createElement("h2"); var newContent = document.createTextNode("This is the new H2 Heading"); newElement.appendChild(newContent); document.body.appendChild(newElement);In this code snippet, you first create a new `
` element using `createElement()`. A text node is then created to hold the content, which is added to the new `` element using `appendChild()` method. This newly created element is then appended to the `` element, effectively adding it to your webpage.
DOM Manipulation Case 2: Changing Styles
Changing the style of a particular element can be done by accessing the `style` property of that element. Have a look at this example.
var element = document.querySelector('p');
element.style.color = 'red';
element.style.fontSize = '18px';
In this scenario, the first paragraph found will have its text colour change to red and its font size set to 18 pixels.
DOM Manipulation Case 3: Removing an Element
The following JavaScript code is a clear example of how you can remove an existing element from your HTML document using the `removeChild()` method.
var parentElement = document.querySelector('div');
var childElement = parentElement.querySelector('p');
parentElement.removeChild(childElement);
This code selects the first `
var element = document.querySelector('p'); element.style.color = 'red'; element.style.fontSize = '18px';In this scenario, the first paragraph found will have its text colour change to red and its font size set to 18 pixels. DOM Manipulation Case 3: Removing an Element The following JavaScript code is a clear example of how you can remove an existing element from your HTML document using the `removeChild()` method.
var parentElement = document.querySelector('div'); var childElement = parentElement.querySelector('p'); parentElement.removeChild(childElement);This code selects the first `
` that is a child of the first `div` found and removes it from the HTML document. DOM Manipulation Case 4: Changing Attributes The following example shows how to change the value of an attribute using JavaScript. The `setAttribute()` function is used to change the existing attribute, or add a new attribute if it doesn't exist.
var element = document.querySelector('img'); element.setAttribute('src', 'newImage.png');The above code changes the `src` attribute of the first image found in the document to 'newImage.png'. DOM Manipulation case 5: Event Handling Event handling is an integral part of JavaScript DOM Manipulation. Events are actions that take place in the browser that can be detected by JavaScript, allowing dynamic interaction with web content. Here's a simple example of an event handler changing the content of a `
` tag when a button is clicked.
document.querySelector('button').addEventListener('click', function(e){ document.querySelector('p').textContent = 'The button was clicked!'; });In this case, an event listener is added to the button element that waits for a 'click'. When the button is clicked, the text content of the `
` tag is changed to 'The button was clicked!'. Each of these examples show you different dimensions of how you can use JavaScript to manipulate the DOM. Through them, you get hands-on practise of a variety of techniques that are regularly used in web development. Keep experimenting with these methods and you'll find yourself growing more confident and adept in your DOM manipulation skills.
Exploring DOM (Document Object Model) Manipulation Techniques in JavaScript
Delving into the world of DOM manipulation techniques in JavaScript is like setting foot on an endless journey of innovation and creativity. By mastering these techniques, you can transform static HTML files into interactive websites, providing the user with an engaging and dynamic experience. DOM manipulation involves accessing HTML elements and modifying them via JavaScript, a task that can be achieved using multiple methods, properties and events.Tools for Better DOM Manipulation Techniques in JavaScript
To maximise efficiency and streamline work in manipulating the DOM, several tools have emerged over time. Some of them include libraries and frameworks that abstract the complexities of direct DOM manipulation, providing developers with a clear and concise way of achieving their goals. jQuery: This is a robust and versatile JavaScript library, designed to simplify HTML document traversal, event handling, animation, and AJAX. It requires less code and reduces the complexity of tasks involving DOM manipulation. As an example, consider a scenario where you want to change the text of an HTML element:$(document).ready(function() { $("p").click(function() { $(this).text("Paragraph clicked"); }); });This jQuery code sets the text of `
` elements to "Paragraph clicked" when clicked. D3.js: This is a powerful JavaScript library that lets you create interactive and visually appealing data visualisations using HTML, CSS, and SVG. It provides a variety of DOM manipulation methods specially tailored for data visualisation. Here's an example, where D3.js is used to create a bar chart:
var data = [4, 8, 15, 16, 23, 42]; var scale = d3.scaleLinear() .domain([0, d3.max(data)]) .range([0, 420]); d3.select(".chart") .selectAll("div") .data(data) .enter().append("div") .style("width", function(d) { return scale(d) + "px"; }) .text(function(d) { return d; });The code first scales the data, selects the chart class, and binds the data. The bar chart is then created using `.style()` and `.text()` methods. React.js: React is a JavaScript library developed by Facebook for building interactive user interfaces. It employs a virtual DOM which significantly improves the performance of complex or large web applications. Here's an example of a React component:
class Welcome extends React.Component { render() { returnThis code snippet defines a Welcome component, which renders a heading containing a greeting to the `name` provided via props. Each tool, whether jQuery, D3.js, or React.js, has its own strengths and characteristics, making it more suitable for different specific applications. Getting to know these tools and effectively using them in your work affords you greater command and control over DOM manipulation.Hello, {this.props.name}
; } }
Tips to Enhance DOM Manipulation Techniques in JavaScript
Advancing in DOM manipulation techniques relies not just on learning them, but also on knowing how to enhance their efficiency. Here are some valuable tips to keep in mind: Use ID or Class Selectors: When you need to manipulate an element using JavaScript, it's more efficient to use ID or class selectors. This is because these selectors are implemented with JavaScript methods that are optimized for performance. Leverage Event Delegation: Event delegation is a technique whereby you assign a single event listener to a parent element in order to avoid the need to assign individual listeners to multiple child elements. This drastically improves performance when dealing with a large number of elements. Choose the Right Tool: As indicated earlier, different tools are designed for different circumstances. Understand the strengths and weaknesses of each and choose the one appropriate for your application's needs. Minimise DOM Manipulation: Although DOM manipulation is powerful, it can also be expensive in terms of performance. By finding ways to minimise manipulation, such as by manipulating an off-DOM node and then appending it to the DOM, or by using virtual DOM in React.js, you can achieve better performance. Understand Asynchronicity: JavaScript is asynchronous, which means it doesn't execute line by line, waiting for each line to finish before moving to the next. Instead, it moves on to subsequent lines even if the current operation isn't complete. Understanding how to deal with asynchronicity, using techniques like promises or async/await, can significantly facilitate DOM manipulation. Keeping these tips in mind not only helps you write code effectively, but it also optimizes your code's performance. Remember, the goal is not just about knowing how to manipulate the DOM, but also about doing it optimally and beneficially for the best user experience.A Deep Dive into DOM Manipulation Methods in JavaScript
Unleashing the full potential of JavaScript requires a deep understanding of DOM manipulation methods. In web development, these methods play a paramount role as they empower developers to dynamically alter the structure, style, and content of a webpage. When you muster the various methods and techniques related to DOM manipulation in JavaScript, you will undoubtedly find valuable ways to develop more efficient, responsive, and interactive websites.Importance of DOM Manipulation Methods in JavaScript
DOM manipulation methods form the backbone of JavaScript's interactivity. They allow you to efficiently extract, modify, and insert elements within a document, effectively making your website dynamic. First, let's understand the term 'DOM'. DOM, short for Document Object Model, is an object-oriented model that treats an XML or HTML document as a tree structure. It represents every HTML element of a page as objects, and the relationships between them can be modified, deleted, or added to using JavaScript. Therefore, knowing how to manipulate the DOM is indispensable when developing web applications. DOM manipulation methods prove beneficial in various aspects, including: Real-time Content Update: With DOM manipulation, a webpage need not be refreshed entirely to update a section or component of the page. Any part of a webpage can be changed at any time using JavaScript, enhancing the user experience with real-time content update. For instance, suppose you are developing a weather application. By implementing DOM manipulation methods proficiently, you can display weather updates without having the users refresh the page. Responsive Feedback: Interactive web applications often need to provide instant feedback to users' interactions. With DOM manipulation methods, you can handle user events such as clicks, keyboard inputs, or mouse movements, and provide immediate visual feedback. Form Validation: DOM manipulation methods allow checking and verifying user inputs in real-time. For example, it can be used to validate form entries on the client-side, giving immediate feedback on input data, enhancing usability, and reducing server load. Customised User Experience: By manipulating the DOM, you can adjust elements on the webpage to provide a personalised user experience. This could be as simple as displaying the logged user's name or as complex as altering the entire layout of a page based on user preferences. Let's take a quick look at some fundamental DOM manipulation methods:document.getElementById('id') document.getElementsByClassName('className') document.getElementsByTagName('tagName') document.querySelector('cssSelector') document.querySelectorAll('cssSelector') element.innerHTML element.setAttribute(name, value) element.getAttribute(name) element.removeAttribute(name) element.style.propertyThese methods open up a myriad of opportunities for interacting with HTML documents and creating engaging, user-friendly web applications.
Utilising DOM Manipulation Methods for an Efficient JavaScript Program
An effectively utilised DOM manipulation method can drastically help improve the efficiency and performance of a JavaScript program. When dealing with operations performed directly on the DOM, knowing when and how to manipulate the DOM while keeping your application's performance in check is crucial. Here are few tips to utilise DOM Manipulation in JavaScript more effectively: Minimise Direct DOM Manipulation: Direct DOM manipulation can be expensive in terms of performance. When possible, manipulate the DOM in a JS object, an array, or in memory before applying it to the DOM. Batch DOM Updates: Instead of making several small updates to the DOM, collect them and make all updates at once. It helps in preventing unnecessary layout recalculations and repaints. Cache DOM References: Always store references to the DOM elements in a variable if you plan to access them multiple times.var element = document.getElementById('myElement'); element.style.color = 'blue'; element.style.backgroundColor = 'black';In the code snippet above, instead of querying the DOM twice for the same element, the element is stored in a variable and accessed from there. Avoid Inline Styles: It's always a better idea to manipulate classes instead of directly tweaking inline styles. Add, remove, or toggle classes on an element to get the changes you want. These tips can help ensure that your JavaScript program is efficient and responsive. Using the DOM manipulation methods in a consistent and optimised manner will result in a significant boon for your web development projects. As you foster these techniques, you will find yourself handling even the most complex JavaScript programs with ease and proficiency.
Javascript DOM Manipulation - Key takeaways
- Javascript DOM Manipulation is the ability to change the structure, style, and content of a webpage dynamically.
- In Advanced DOM manipulation JavaScript, understanding the key components - Nodes and Elements, Events, and Methods and Properties is crucial.
- Nodes and Elements comprise all aspects in the HTML DOM where the document is a document node, all HTML elements are element nodes and attributes are attribute nodes. Text within HTML elements are text nodes and comments are comment nodes.
- Events allow interaction with nodes and JavaScript can detect such occurrences or actions in the browser.
- Methods such as querySelectorAll(), querySelector(), getElementById() and Properties like createTextNode(), innerText, innerHTML() are available in JavaScript for modifying elements within the nodes.
- Practical examples of DOM Manipulation JavaScript include creating and adding a new element, changing styles, removing elements, changing attributes and handling events.
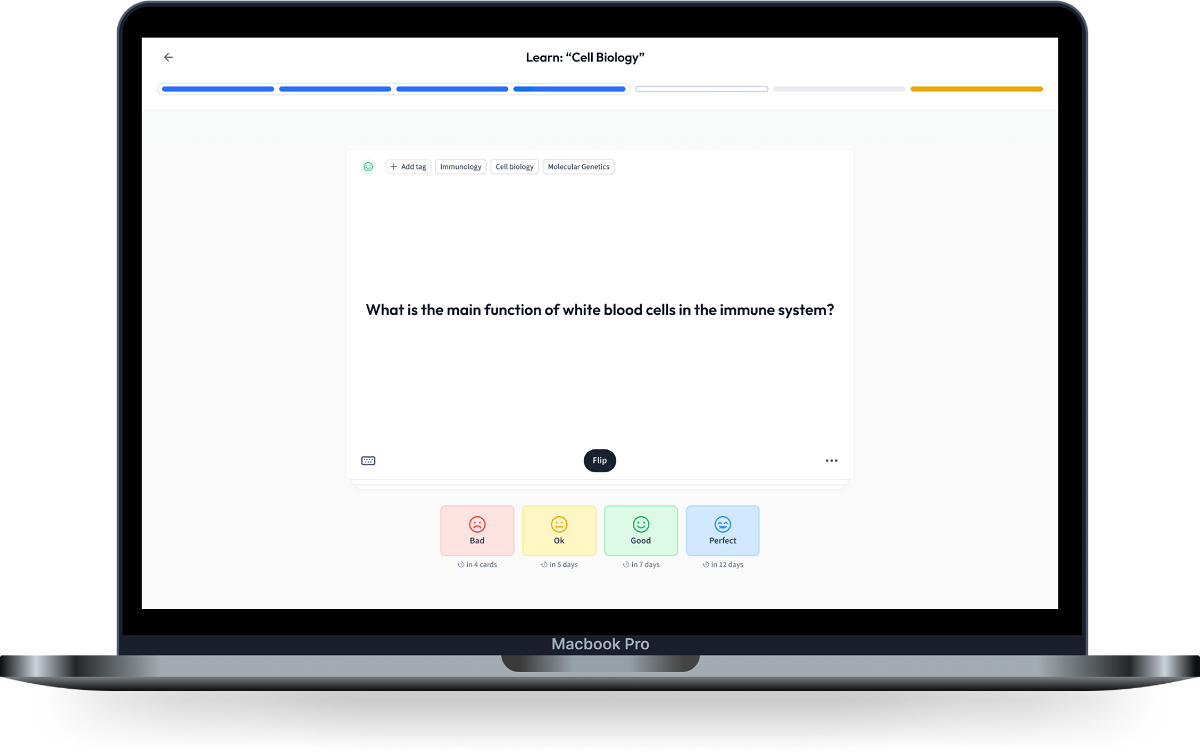
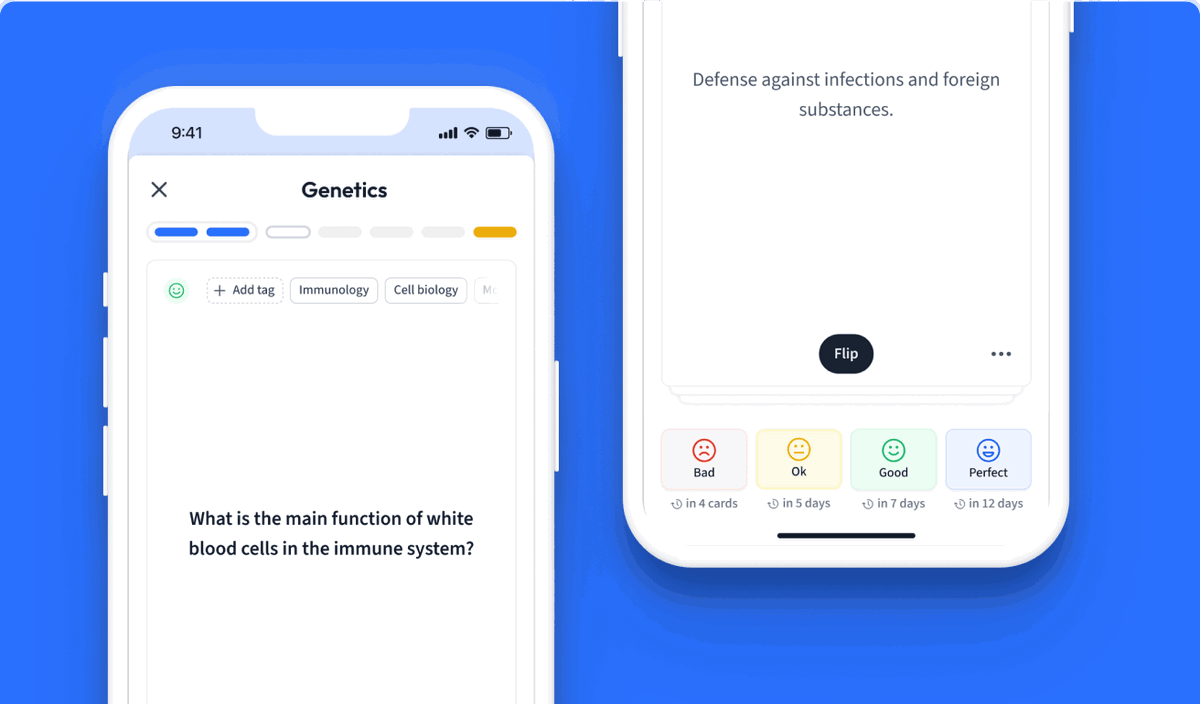
Learn with 72 Javascript DOM Manipulation flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript DOM Manipulation
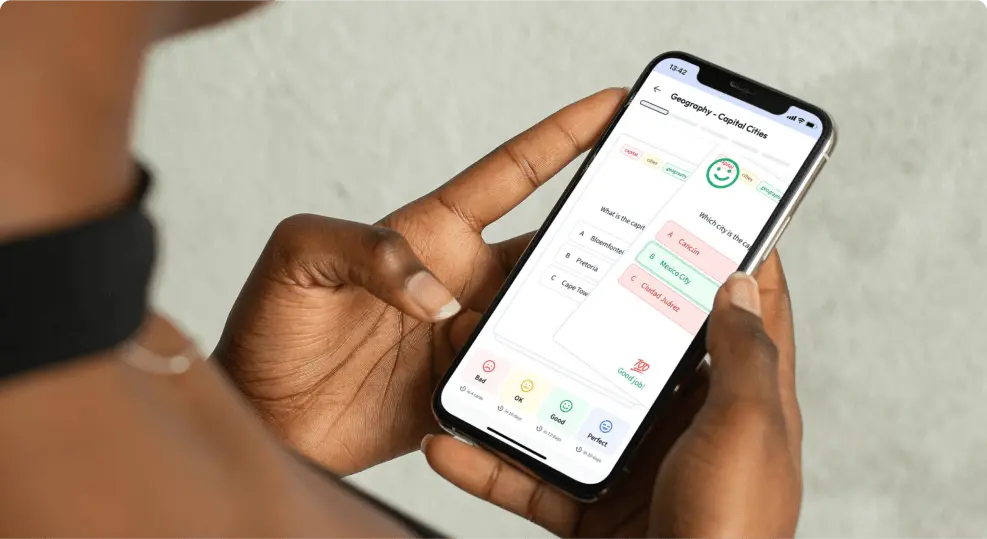
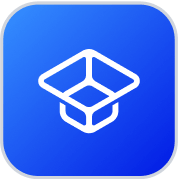
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more