Mastering Java Operators: A Comprehensive Guide
In the realm of computer programming, Java operators play a pivotal role. They act as the building blocks, permitting you to manipulate variables and values to perform a wide array of operations. Understanding these operators within Java can empower you to write functional and efficient code.
Understanding the Syntax and Function of Java Operators
Before you start utilizing Java operators, it's crucial to comprehend the syntax and function they serve in your code. Operators enable you to execute various mathematical, logical or relational operations.
At its core, a Java operator is essentially a symbol that indicates the compiler to execute a specific mathematical or logical operation.
These operators can be broadly divided into a few classes based on their functionality:
- Arithmetic operators
- Relational operators
- Logical operators
- Bitwise operators
- Assignment operators
Each of these classes serve unique purposes and aid in tackling different problems in your code. The charm of programming lies in employing these operators appropriately to build powerful and efficient code.
Class | Example Operator | Function |
Arithmetic | + | Addition |
Relational | == | Equality Checking |
Logical | && | Logical AND |
Bitwise | >> | Right shift |
Assignment | = | Assignment of value |
Now, to comprehend how these work, let's break each one of these down and understand their function in detail.
Various Examples of Java Operators for Better Comprehension
To understand Java operators better, let's look at some examples and see how they function in real code.
The most basic and widely-used operator is the addition (+) operator.
int a = 5; int b = 10; int sum = a + b; //This will give sum as 15
Similarly, we have operators for subtraction (-), multiplication (*) and division (/). For instance, let's look at multiplication.
The code snippet below executes a simple multiplication operation between two numbers.
int c = 5; int d = 10; int product = c * d; //This will give product as 50.
Moving on, when we talk about comparison or relational operators, these are the ones you will see the most: less than (<), greater than (>), equal to (==), not equal to (!=), less than or equal to (<=), and greater than or equal to (>=). As for logical operators, we usually use AND (&&), OR (||), and NOT (!).
For understanding these operators and how they work, practice and application is key. Remember, the more you code, the more familiar you become with these operators and their applications – enabling you to write more efficient and robust code.
Unravel the Complexity of Ternary Operator in Java
In the landscape of Java programming, apart from the simple operators, there are some more complex ones that can add speed and sophistication to your coding style, and one such powerful operator is the ternary operator.
Definition of Ternary Operator in Java
Serving as an efficient alternative way to express conditional statements, the ternary operator or the conditional operator in Java is a unique operator that takes three operands and is therefore named as "ternary". It is denoted by the symbols "?" and ":" and can be deemed to perform a similar task as an if-else statement.
The typical structure of a ternary operator in Java is as follows: condition ? expressionOnTrue : expressionOnFalse. It will evaluate the condition provided. If the condition holds true, it executes the expressionOnTrue else it executes the expressionOnFalse.
Let's understand this in more detail using some mathematical syntax.
Given a condition \( C \), and two expressions \( E_1 \) and \( E_2 \), the ternary operation can be expressed as:
\[ C ? E_1 : E_2 \]Now let's break it down:
- \( C \): This is a boolean expression (condition that evaluates to either true or false).
- \( E_1 \): This expression will be executed if \( C \) is true.
- \( E_2 \): This expression will be executed if \( C \) is false.
So, you can reason that the ternary operator is a precise and compact way of writing conditional statements in Java.
Real-life Examples for Effective Application of Ternary Operator in Java
Now that you understand the technical definition of a ternary operator, it will be worthwhile to see how it can be leveraged in real-world programming scenarios through some examples. Recognising the benefits of this operator in real-life situations can give you the practical knowledge needed to effectively apply it in your coding projects.
Consider a simple example where we want to decide a variable value based on a condition. Let's say we need to assign minimum value among two numbers to a variable. Using ternary operator, we can write:
int a = 10; int b = 20; int smallerNumber = (a < b) ? a : b;
In the example above, the condition checks if 'a' is less than 'b'. If it's true, the value of 'a' is assigned to 'smallerNumber', otherwise the value of 'b' is stored. The code snippet is simple, concise, and offers excellent readibility.
Let's look at another example, where we're determining if a given number is even or odd using the ternary operator.
int num = 15; String result = (num % 2 == 0) ? "even" : "odd";
In this example, the condition checks if the remainder of 'num' divided by 2 equals 0; if this is true, then "even" is assigned to 'result', otherwise "odd" is assigned. This demonstrates how the ternary operator can also be used with different types of data, in this case, with int and String.
These examples emphasise the practical usability and flexibility of the ternary operator in Java. By understanding these examples, you can start to use this operator more effectively in your code and take advantage of its benefits, such as reduced lines of code and enhanced readability.
Deep Dive into Java Bitwise Operators
In computer programming, specifically in Java, bitwise operators are a fascinating category of operators that you work with on a bit level. These operators can prove extremely handy while performing operations related to binary numbers. Diving into the world of bitwise operators can open up a new level of understanding and manipulation of data at the fundamental, binary level.
Essential Information on Java Bitwise Operators
Bitwise operators in Java are used to perform manipulation of individual bits of a number. They can be applied to integral types - characters, integers and longs. You might wonder why you would want to manipulate bits in your code. Well, really, it all comes down to efficiency. Bit-level operations are faster and use less memory.
In Java, there are six types of bitwise operators:
- Bitwise AND (&)
- Bitwise OR (|)
- Bitwise XOR (^)
- Bitwise Complement (~)
- Left Shift (<<)
- Right Shift (>>)
- Zero-fill Right Shift (>>>)
Each operator serves a different purpose and can be utilised based on the given requirement or problem statement. For instance, the Bitwise AND operator (&) compares each binary digit of the first operand with the corresponding binary digit of the second operand. If both digits are 1, the result digit is 1; otherwise, it is 0.
Consider two binary numbers – 1101 and 1011. If we perform a bitwise AND operation on these, the result is 1001 as depicted below:
1101 & 1011 ______ = 1001 (Bitwise AND) ______
Moving on to another operator, Bitwise OR (|) again compares the binary bits of the operands, but in this operation, if either of the bits or both are 1, then the result bit is 1.
For instance, the bitwise OR operation on binary numbers 1101 and 1011 results in 1111. Here is a step-by-step representation of this:
1101 |1011 ______ =1111 (Bitwise OR) ______
Other operators like Bitwise XOR (^), Bitwise Complement (~) and shift operators like Left Shift (<<), Right Shift (>>) and Zero-fill Right Shift (>>>) follow their distinct rules and principles while computing the respective results.
Practical Java Bitwise Operators Examples for Learners
Practical application of these operators in code can solidify your understanding and make concepts clearer. The following examples should help you understand how these bitwise operators work in Java.
Let's start with a simple example using Bitwise AND (&) operator. In the following code snippet, you can see how Bitwise AND operator is used to calculate the result.
public class HelloWorld{ public static void main(String []args){ int a = 9; // Binary form -> 1001 int b = 10; // Binary form -> 1010 int result = a & b; // Bitwise AND -> 1000 which is 8 System.out.println("Result = " + result); } }
Now let's take a look at how Bitwise OR (|) operator works:
public class HelloWorld{ public static void main(String []args){ int a = 9; // Binary form -> 1001 int b = 10; // Binary form -> 1010 int result = a | b; // Bitwise OR -> 1011 which is 11 System.out.println("Result = " + result); } }
As observed in these examples, a crucial requirement when working with bitwise operators is a clear understanding of the conversion between binary and decimal numbers. Understanding how these operators work can assist in creating optimised and efficient code leveraging the power of binary computing.
In conclusion, bitwise operators, though tricky at first, can offer significant insight into the inner workings of binary number manipulation. Pursuing a more profound understanding of these operators enables you to write code that can handle a variety of complex tasks efficiently.
Logical Operators in Java: An Essential Tool
If you've been delving into Java, you've probably come across logical operators. These operators are a fundamental part of Java that manage the logical connection between variables and allow complex Boolean expressions to be formed. Logical operators act as cogs in the machinery of if-then, while, and for statements, helping tailor a program's flow to the programmer's requirements.
An Insight into Logical Operators in Java
As their name suggests, logical operators in Java come into action when you need to make decisions based on particular conditions. In essence, these operators assess whether certain conditions are true or false, thereby allowing your Java application to choose between alternative paths of execution. There are five different logical operators:
- Logical AND (&&): Returns true if both expressions are true.
- Logical OR (||): Returns true if either expression is true.
- Logical NOT (!): Reverses the truth value of the operand. If it is true, it returns false. If it is false, it returns true.
- Bitwise AND (&): Similar to the logical AND but has a broader application as it can operate on primitive data types.
- Bitwise OR (|): Similar to the logical OR, it can operate on more primitive data types.
The logical AND (&&) and logical OR (||) are short-circuit operators. In other words, if the first condition makes the result apparent, the second condition isn't assessed. For instance, in an OR operation, if the first operand is true, the outcome is true without checking the second operand. This mechanism can speed up the processing time and avoid errors like divide-by-zero.
A | B | A && B | A || B |
true | true | true | true |
true | false | false | true |
false | true | false | true |
false | false | false | false |
This table demonstrates the return values when logical AND and OR operators are used with boolean values.
Useful Examples to Help You Understand Logical Operators in Java Better
Let's take a look at some concrete examples to visualise how these logical operators work in Java code.
Let's say you have two boolean variables, 'isRaining' and 'isCloudy'. You want to decide whether you should carry an umbrella. You will carry the umbrella if it's either raining already or if it's cloudy (and might rain). Here's how the 'Logical OR' operator can be used:
boolean isRaining = true; boolean isCloudy = false; boolean shouldTakeUmbrella = isRaining || isCloudy;
In the example above, the value of 'shouldTakeUmbrella' will be true, as 'isRaining' is true. Even though 'isCloudy' is false, the 'Logical OR' operator returns true if any one of the conditions is true.
Now, let's consider a situation where you only want to go for a run if it is neither raining nor cloudy. 'Logical NOT' comes into play here:
boolean isRaining = false; boolean isCloudy = false; boolean shouldGoForRun = !isRaining && !isCloudy;
In this example, the value of 'shouldGoForRun' will be true because 'isRaining' and 'isCloudy' both are false. The 'Logical NOT' operator flips the value of the specified boolean, and the 'Logical AND' operator then checks if both flip results are true.
It is worth noting that even though bitwise AND (&) and OR (|) operators can be used in place of logical AND and OR for boolean values, the former ones don't support short-circuiting. Therefore, it's generally good practice to use logical operators for boolean logic to prevent unnecessary computations and potential errors.
These examples show the power of logical operators in controlling program flow based on multiple conditions. Knowledge of how to use these operators effectively can prove to be an enormous asset in your Java programming journey.
The Importance of Java Operator Precedence
When you're programming in Java, operator precedence matters. Essentially, it represents the order in which operators are evaluated in an expression. It is especially important when an expression contains multiple operators, because the value of the expression can differ significantly based on the order in which the operations are performed.
Making Sense of Java Operator Precedence
Java operator precedence is a set of rules that determine how an expression involving several operators is evaluated. The precedence of operators can often be thought of as 'ranking' of operators. Operators with higher precedence are performed before those with lower precedence.
Consider an arithmetic expression with both multiplication and addition, like \(6 + 2 \times 3\). Would you first multiply 2 and 3 or add 6 and 2? In mathematics, you'd perform multiplication before addition due to operator precedence. This is also true in Java, where multiplication has a higher precedence than addition.
In essence, operator precedence ensures that expressions are interpreted and executed correctly by the Java compiler. Moreover, it helps avoid potential bugs and errors in your code that could be challenging to pinpoint.
In Java, operators have the following order of precedence from highest to lowest:
- Postfix operators: expression++, expression--
- Unary operators: ++expression, --expression, +expression, -expression
- Multiplicative: *, /, %
- Additive: +, -
- Shift: <<, >>, >>>
- Relational: <, >, <=, >=, instanceof
- Equality: ==, !=
- Bitwise inclusive OR: |
- Bitwise exclusive OR: ^
- Bitwise AND: &
- Logical AND: &&
- Logical OR: ||
- Conditional: ?:
- Assignment: =, +=, -=, *=, /=, %=, &=, ^=, |=, <<=, >>=, >>>=
Expressions are evaluated from left to right. However, operators with the same level of precedence are performed based on their associativity property. Most operators are left-associative (left to right), but some operators like assignment operators are right-associative.
Relevant Examples to Understand Java Operator Precedence Effectively
One of the best ways to understand Java operator precedence is to see it in action. So, let's dive into some constructive examples.
Consider a Java operation where multiple operators are involved:
int result = 3 * 2 + 4 / 2 - 2;
Due to operator precedence, multiplication and division are performed before addition and subtraction. Thus, the result is calculated as:
int result = 6 + 2 - 2; int result = 8 - 2; int result = 6;
Now consider an example where parentheses are used:
The parentheses (brackets) change the usual order of operations:
int result = 3 * (2 + 4) / 2 - 2;
Although multiplication and division usually have a higher precedence, brackets override this, so the addition is performed first. The expression is thus evaluated as follows:
int result = 3 * 6 / 2 - 2; int result = 18 / 2 - 2; int result = 9 - 2; int result = 7;
Both these examples should help you see how significant operator precedence can be when executing Java expressions. Whether you're a novice or seasoned coder, understanding operator precedence is crucial as you advance your programming skills. This understanding can lead to cleaner, more precise, and effective code.
Java Operators - Key takeaways
- Java operators perform various tasks like arithmetic operations, comparison or relational operations, and logic operations in programming. The common arithmetic operators include addition (+), subtraction (-), division (/), and multiplication (*).
- In Java, the ternary operator is a unique operator that takes three operands. It serves as an efficient alternative way to express conditional statements and is denoted by the symbols "?" and ":". It operates as: condition ? expressionOnTrue : expressionOnFalse.
- Java bitwise operators work on a bit level and perform operations related to binary numbers. They include Bitwise AND (&), Bitwise OR (|), Bitwise XOR (^), Bitwise Complement (~), Left Shift (<<), Right Shift (>>), and Zero-fill Right Shift (>>>). These operators operate on individual bits of a number and are typically used for efficiency, as bit-level operations are faster and use less memory.
- Logical operators in Java assess whether certain conditions are true or false, and include Logical AND (&&), Logical OR (||), and Logical NOT (!). Logical AND and OR are short-circuit operators, meaning if the first condition makes the result apparent, the second condition isn't assessed.
- Java operator precedence is a set of rules that determine how an expression involving several operators is evaluated. Operators with higher precedence (like multiplication and division) are performed before those with lower precedence (like addition and subtraction).
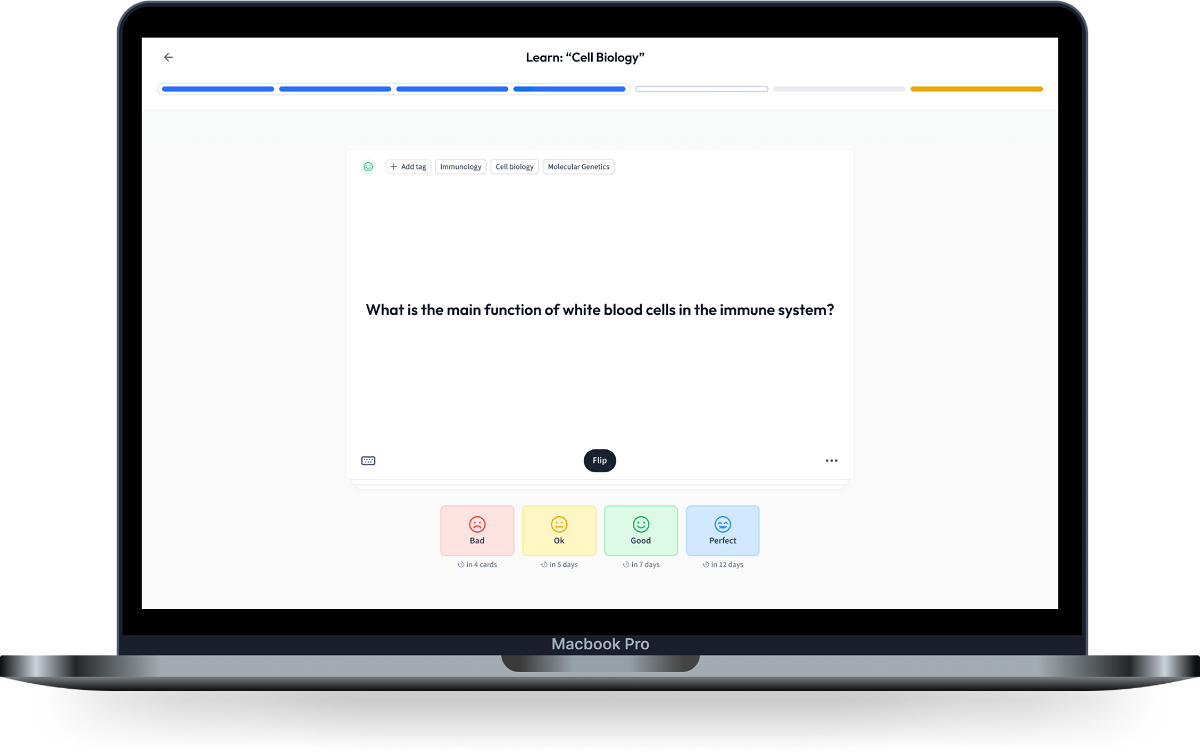
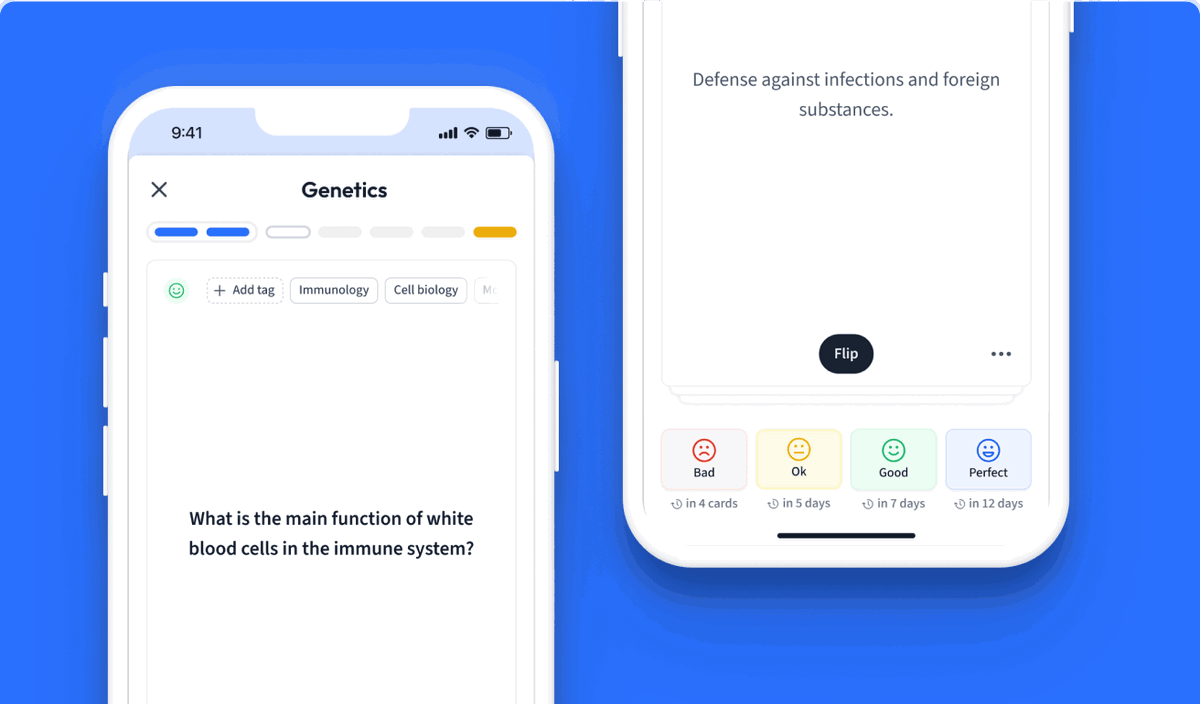
Learn with 81 Java Operators flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Java Operators
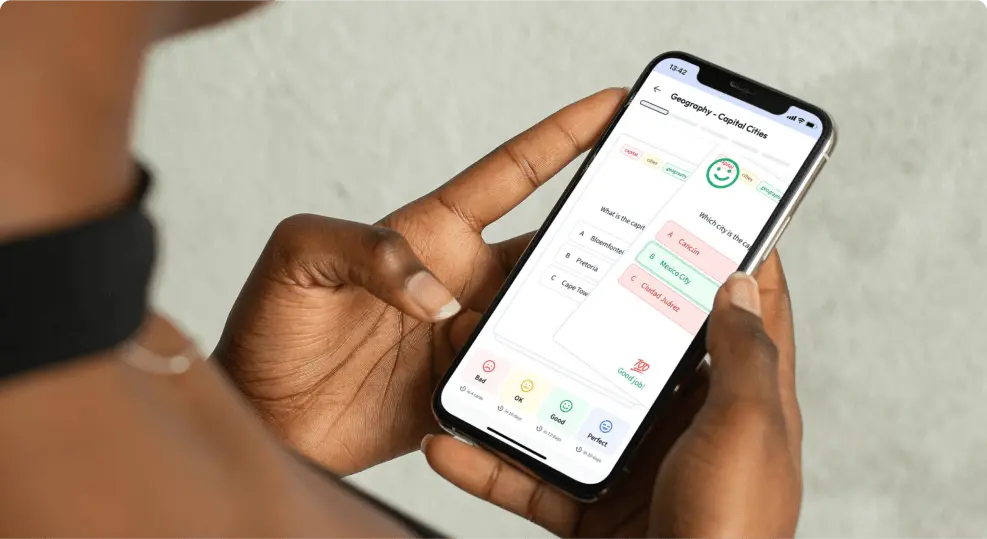
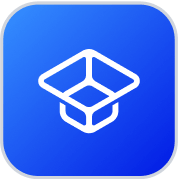
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more