Exploring Software Design Patterns in Computer Programming
In the vast and ever-evolving world of computer programming, certain repetitive problems keep surfacing. To tackle these effectively, you have proven solutions at your disposal, known as Software Design Patterns. They are essentially best practice methods applicable to the design phase of software development, eliminating the hassles of reinventing the wheel while optimising the efficiency and productivity of your codes.
Understanding the Concept of Software Design Patterns
Software Design Patterns aren't merely pieces of code but rather a concept, a generalized solution to specific problems encountered during software design. They encourage code reuse, improving the modularity and readability of your programs. The concept of design patterns emerged from the fields of civil engineering and architecture but got adopted in software engineering in the 1990s. Following are the three major types of software design patterns:- Creational patterns deal with object creation mechanisms, trying to create objects in a manner suitable to the situation.
- Structural patterns deal with the composition of classes or objects, ensuring that different parts of a system work together efficiently.
- Behavioural patterns deal with communication between objects, how they interact and distribute responsibilities.
A good example of a creational design pattern is the Singleton pattern, which restricts the instantiation of a class to a single object. This can be useful when only a single instance of a class is required to control actions.
Importance and Utilisation of Software Design Patterns in Computer Programming
The utilisation of software design patterns can drastically enhance your programming skills, optimising the speed and efficiency of your software development. Moreover, understanding and employing these patterns can hasten the process of problem-solving, as these patterns provide tested, proven development paradigms. These patterns play a paramount role in avoiding code repetition, making your code more maintainable, modular and scalable. Effective use of these patterns reduces debugging efforts too.Let's consider an example with the Observer pattern (a behavioural design pattern). It's used when there's a one-to-many dependency between objects so that when one object changes state, all its dependents are notified. The Twitter model, where a change in a person's profile (tweeting, for example) prompts notifications to their followers, exemplifies this design pattern.
An interesting fact, the application of software patterns is vast and goes beyond just software design. They are essential in various fields like game design, UI/UX design, and pretty much any field that requires solving recurring problems.
Adapter Software Design Pattern, a Key Player in Software Architecture
When you are communicating with practical aspects of software development, you'll often discover that different parts of a program might have incompatible interfaces. This is where the Adapter Software Design Pattern, also known as the Wrapper Pattern, comes into play. The Adapter pattern allows classes with incompatible interfaces to work together. It wraps the functionality of a class and creates a new interface for it, thereby making it compatible with other classes.
Introduction to Adapter Software Design Pattern and its Application
The Adapter Software Design Pattern is classified under structural design patterns, as it forms a bridge between two incompatible interfaces. This pattern involves a single class known as adapter which is responsible for linking the incompatible interfaces.
The Adapter pattern employs polymorphism and delegation principles to alter the interface of an object while keeping its functionality intact. It usually ascertains that the core functionality of an object remains the same while adding an outer wrapper that makes the object compatible with a certain API.
The term 'polymorphism' in the context of object-oriented programming refers to the ability of an object to take many forms. This means methods can perform their operations in multiple ways, depending on the object that invokes them.
It's widely used in retrofitting legacy systems to match modern requirements, when the existing interface cannot be used directly with other interfaces in an application and when the desired interface isn't available.
To create a visualisation, consider a scenario where a program uses a timer class, which has methods like addTime(). Now, if you wish to use another API which provides advanced functionality but uses a different method like addHours(), adapter pattern would be beneficial. The adapter can translate calls to addTime() into calls to addHours(), thereby bridging the incompatibility.
A noteworthy insight is that the Adapter pattern can not only adapt methods but also properties. For instance, if one component of software uses a property 'colour' while another one uses 'colourCode', an adapter could be written to bridge this discrepancy.
Advantages of Using the Adapter Software Design Pattern
Employing the Adapter software design pattern brings multiple benefits for both critical and mundane software development tasks.
- It improves reusability of old classes or interfaces.
- Adopting this pattern leads to increased transparency as complex interfaces can be adapted into simpler ones.
- Moreover, it promotes decoupling, where the client class doesn't depend directly on the adaptee class, enhancing the understandability of our code.
Apart from these, one of the most outstanding perks of this pattern is extensibility. If you need to integrate new types of functionality into your program, you can accomplish this by writing new adapters, without altering existing code, augmenting scalability. This ensures that your program remains open for extension but closed for modification, adhering to the Open-Closed Principle of SOLID architecture.
The Open-Closed Principle, one of the five principles of SOLID, states that "software entities (classes, modules, functions, etc.) should be open for extension, but closed for modification". In essence, a well-designed component of a software allows its behaviour to be extended without modifying its source code.
Though the Adapter pattern involves indirect access, necessitating an additional pointer jump and thus a little slow, the perks of reusability, transparency, decoupling, and extensibility make it an essential tool in the kit of software design patterns.
Deep Dive into Software Architecture Design Patterns
Software architecture design patterns are pre-designed, reusable solutions to common design problems that occur in software architecture. They're effective, reliable and enhance code readability, efficiency and maintainability. The software industry has identified numerous architecture design patterns, each with its unique merits and scenarios where they shine. Let’s dive deeper into these design patterns and discover the essentials of software architecture.
What are the Common Software Architecture Design Patterns and their Functions?
Among the myriad of software architecture design patterns, some of the most commonly used include the following:- Layered (n-tier) pattern
- Event-driven architecture pattern
- Microservices pattern
- Service-oriented architecture pattern
- Monolithic pattern
Aligning Software Architecture Design Patterns with Programming Objectives
Aligning the correct software architecture design pattern with the programming objectives can significantly impact your project's success. Not every project demands a cutting-edge architecture design pattern; even a simple layered architecture may serve the purpose for smaller applications. While implementing a design pattern, you must consider factors such as:- Size of the application
- Team's familiarity with the design pattern
- Type of data flow in the application
- Expected user load
- Availability of resources
Techniques and Principles in Software Design Patterns
Software Design Patterns are solutions to recurring problems, guidelines rather than rigid rules which reinforce best practices and robust plan for software designing. They come into the picture during the intermediate phase of the software development, specifically while converting the system's schema into a suitable software model. Understanding these patterns and their implementation techniques, backed by principles, can help you design better software architecture and code.
Common Techniques in Implementing Software Design Patterns
When it comes to implementing software design patterns, there are several common techniques you might employ. As they are guidelines, not hard and fast rules, these techniques serve to aid and streamline the implementation process without enforcing a rigid routine.
The first noteworthy technique is Code Reusability, which implies you don't have to rewrite the same code again and again. When you identify a pattern, you can extract it into a separate method or class, and then reuse that component whenever needed. This technique reduces redundancy and fosters maintainability. For instance, in the Singleton pattern, an instance is reused again and again rather than creating a new one each time.
public class Singleton { private static Singleton instance; private Singleton(){} public static Singleton getInstance(){ if(instance == null){ instance = new Singleton(); } return instance; } }
Besides this, harmonising Object Composition over Inheritance is another crucial technique. Design patterns encourage the use of object composition instead of inheritance to offer flexibility in code modification. It means behaviour of an object is determined more by its composition than its inheritance hierarchy — it's assembled from other objects rather than being defined statically. This is particularly valuable in the Decorator pattern as its primary aim is to add (decorate) additional features to an object dynamically.
With the composition, it's simple to change behaviour on the fly with Dependency Injection / Setting methods, whereas it's difficult to do so with inheritance.
Examples of Techniques in Creating Effective Software Design Patterns
A deep understanding of the state and behaviour of objects is a significant step towards creating effective software design patterns. In the State Pattern, for example, an object alters its behaviour when its internal state changes. The object will appear to change its class.
Another manifestation of effective techniques in software design patterns is the Observer Pattern. It specifies a "one-to-many" dependency between objects so that when one object changes state, all its dependent objects are notified and updated automatically. This pattern enhances real-time reaction to changes in object's state and encourages a decoupled interactive system.
To illustrate the Observer Pattern, consider a chat room application. When someone posts a new message, all users in the chatroom must be notified. An application can handle this by maintaining a list of observers (users) and whenever there's a new message, iterating over the list and notify (update) each user.
Core Principles of Software Design Patterns
Software design patterns are underpinned by core principles which advocate good design and effective communication between objects.
First of these principles is the Single Responsibility Principle. It holds that a class or module should have only one reason to change. This means it should encapsulate only one set of related functions.
Next is the Open-Closed Principle, which asserts that a software entity should be open for extension but closed for modification. In essence, you should design your modules so that they can be extended, without requiring modification.
Additionally, the Liskov Substitution Principle mandates that any instance of a parent class should be replaceable by its child class without affecting the correctness of the program. In this way, it ensures that a derived class doesn't alter the behaviour of the parent class.
The Interface Segregation Principle focuses on reducing the side effects and frequency of required changes by splitting the software into multiple, independent parts. It encourages many client-specific interfaces are better than one general-purpose interface.
Lastly, the Dependency Inversion Principle prescribes that high-level modules should not depend on low-level modules. Both should depend on abstractions and abstractions should not depend upon details. Details should depend upon abstractions.
Rationale behind the Principles of Software Design Patterns
The principles of software design patterns aren't arbitrary but rooted in two underlying themes— robustness and flexibility.
The Single Responsibility Principle focuses on ensuring that a class or module does only what it's supposed to do and nothing else; thereby ensuring that particular functionality will not compromise or interact with other functionalities. This fosters robustness in software design.
The Open-Closed Principle promotes code flexibility by encouraging extension over modification, ensuring that existing code remains unaffected while adding new functionality, promoting robustness and extensibility.
The Liskov Substitution Principle enforces the rule of thumb that the derived class must enhance functionality, but not alter it, fostering robustness in the software design.
The Interface Segregation Principle suggests that it's easier to update, modify, and even remove when small interface changes to a larger system rather than modifying a system-wide interface, fostering adaptability and enhancing maintainability.
Lastly, the Dependency Inversion Principle proposes that higher-level modules should not be affected by any changes in lower-level modules, reducing the risk of ripple effects across the system due to localised changes, promoting the robustness of the system and its resilience to change.
Thus, the principles of software design patterns foster a well-structured, robust, and flexible design that stands against the test of time and changes.
Learning through Examples of Software Design Patterns
A practical and memorable way to comprehend software design patterns is by examining real-world examples. These patterns, being recurring solutions to universal software problems, are often best understood when put into context. With this understanding, you can effectively address complex design challenges and devise efficient, maintainable and scalable solutions.
Exploring Examples of Commonly Used Software Design Patterns
Some commonly used software design patterns that you will encounter in most software designs are Singleton Pattern, Strategy Pattern, Composite Pattern, and Observer Pattern. By understanding their structure and applicability, you can better discern which pattern to use in a given situation.
TheSingleton Pattern is a design pattern that restricts the instantiation of a class to a single object. It is used where you need only a single instance of a class and provide a global point of access to it. A filesystem is a good example.
public class FileSystem { private static FileSystem instance; private FileSystem(){} public static FileSystem getInstance(){ if(instance == null){ instance = new FileSystem(); } return instance; } }
The Strategy Pattern is a behavioural software design pattern that enables selection of an algorithm at runtime. For example, a travel app that provides several route options (fastest, shortest, least traffic) for a trip uses the Strategy Pattern.
public interface RouteStrategy { String buildRoute(String start, String end); } public class FastestRoute implements RouteStrategy { public String buildRoute(String start, String end) { // Implementation... } } public class ShortestRoute implements RouteStrategy { public String buildRoute(String start, String end) { // Implementation... } }
The Composite Pattern is a structural software design pattern that depicts a group of objects similar to a single instance of the same type of object. This helps to create a tree structure and present it as if it were a single object. The HTML DOM (Document Object Model), where each tag inside an HTML document can contain other tags, is a great example of Composite Pattern.
The Observer Pattern is a software design pattern where an object, named the subject, maintains a list of its dependents, called observers, and notifies them automatically of any state changes. Most used in event-driven systems, like GUI libraries, where clicking a button (event) triggers reactions (state changes).
public interface Observer { void update(int value); } public class Subject { private Listobservers = new ArrayList<>(); private int value; public void setValue(int value) { this.value = value; notifyObservers(); } public void notifyObservers() { for (Observer observer : observers) { observer.update(value); } } }
A practical illustration is an Email Notification System, where adding an email to the system (subject) allows it to notify all registered clients (observers) whenever there's a new mail.
Practical Application: Case Studies of Software Design Patterns
Now moving onto case studies of software design patterns, you can gain a deeper insight into how they manifest themselves in real-world software engineering decisions and implementations.
In the development of an e-commerce website, various design patterns are utilised to manage the intricacies of the system. For example, the Facade Pattern might be used to simplify the integration with a complex payment gateway offering multiple payment options (credit card, PayPal, bank transfer). The Facade provides a unified interface to a set of interfaces in the payment gateway subsystem, hence simplifying the use of the system in a higher-level layer (like a checkout process), while still encapsulating the complex interactions behind the scenes.
public class PaymentFacade { private CreditCardPayment creditCardPayment; private PayPalPayment payPalPayment; private BankTransfer bankTransfer; public PaymentReceipt payBy(PaymentOption option) { switch (option) { case CREDIT_CARD: return creditCardPayment.pay(); case PAYPAL: return payPalPayment.pay(); case BANK_TRANSFER: return bankTransfer.pay(); default: throw new IllegalArgumentException("Invalid payment option"); } } }
Another significant design pattern illustrated in e-commerce software is the Interpreter Pattern. This pattern provides a way to evaluate language grammar or expression. This design pattern involves implementing an expression interface which tells to interpret a particular context. This pattern can be seen when implementing a complex search functionality, which becomes more effective and efficient by using this pattern.
public interface Expression { boolean interpret(String context); } public class SearchExpression implements Expression { @Override public boolean interpret(String context) { // Implementation... } }
In summary, these patterns help solve recurring challenges and add a layer of well-established practices to your code, enhancing its scalability and maintainability.
Software Design Patterns - Key takeaways
- Software Design Patterns: They are general, reusable solutions to commonly occurring problems in software design and can be used in many different applications.
- Adapter Software Design Pattern: A type of design pattern which makes two incompatible interfaces compatible without altering their existing code. It enables a class’s interface to be converted into another interface that the client code expects.
- Common Software Architecture Design Patterns: Include layered patterns, event-driven architecture patterns, microservices patterns, service-oriented architecture patterns, and monolithic patterns.
- Techniques in Software Design Patterns: Code reusability and object composition over inheritance are essential strategies when implementing software designs. This enhances code flexibility and maintainability.
- Principles of Software Design Patterns: These include the Single Responsibility Principle, the Open-Closed Principle, the Liskov Substitution Principle, the Interface Segregation Principle, and the Dependency Inversion Principle. These principles ensure robustness, flexibility and easy maintenance of software designs.
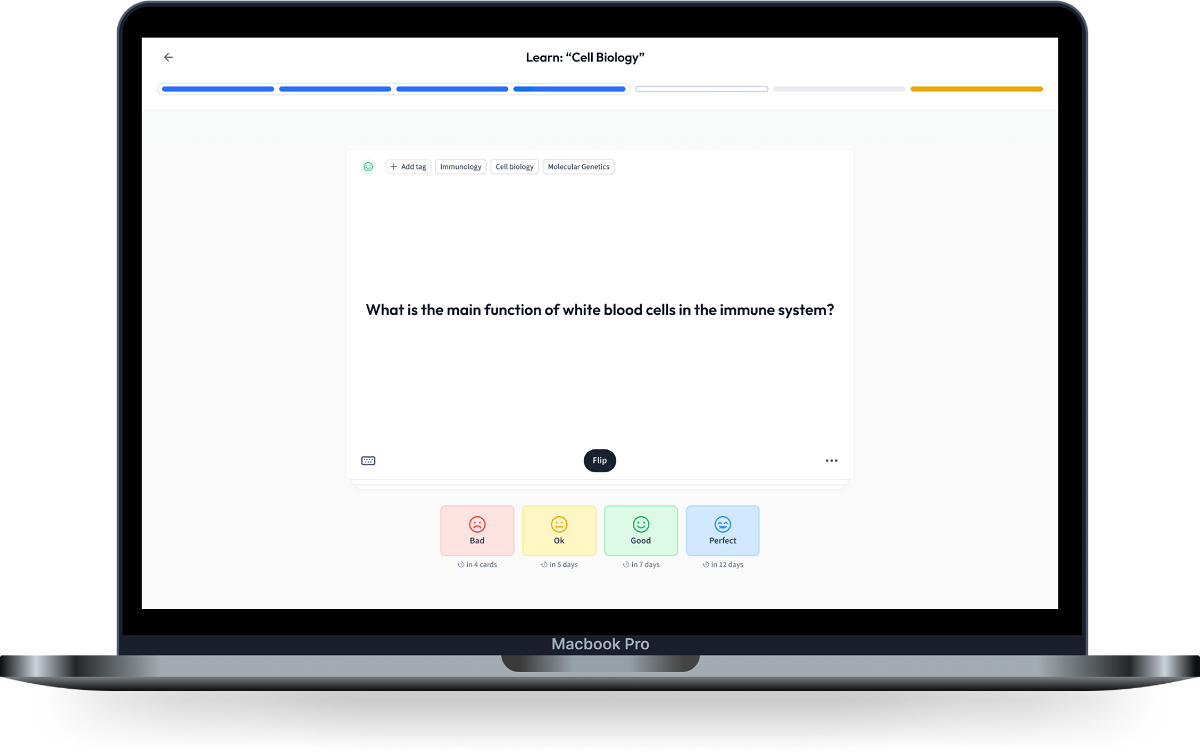
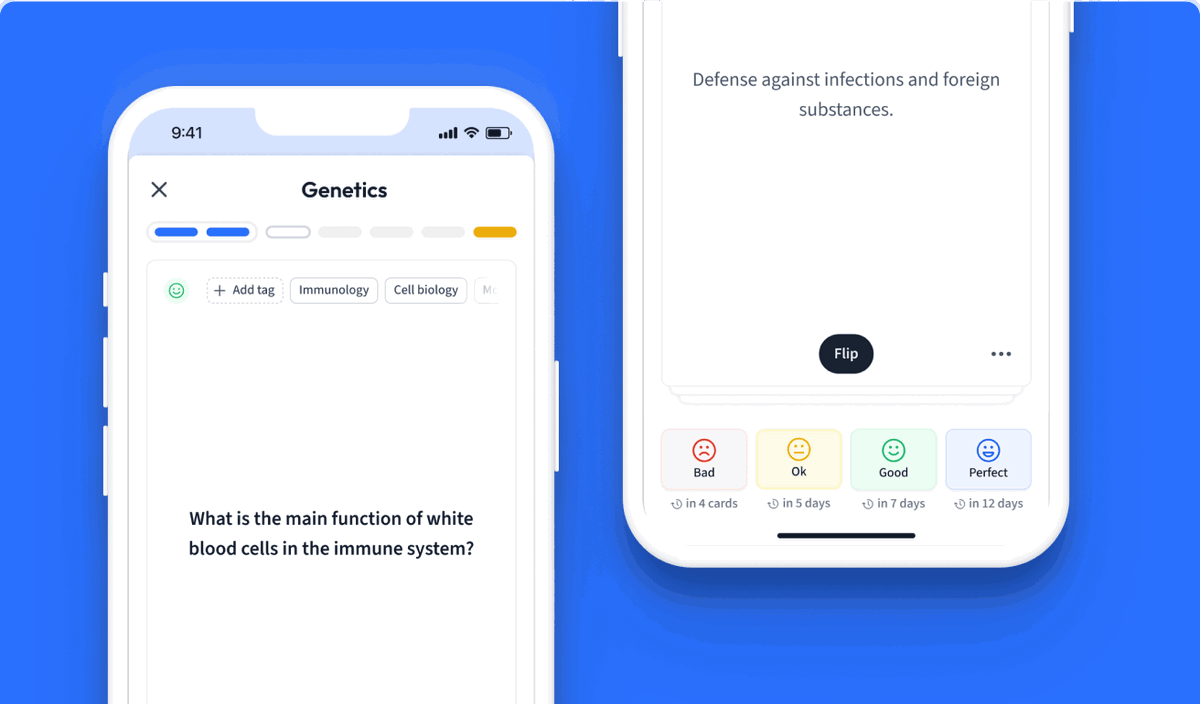
Learn with 96 Software Design Patterns flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Software Design Patterns
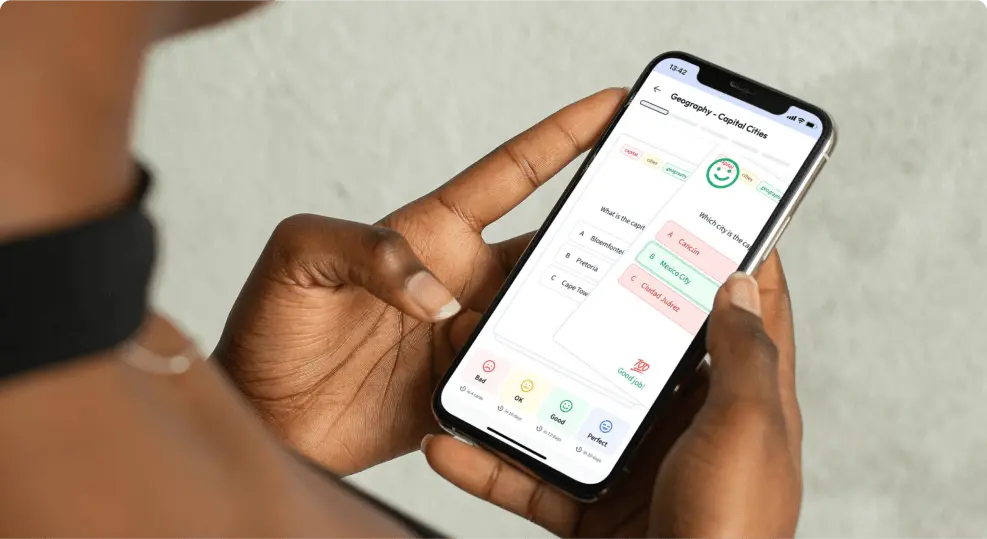
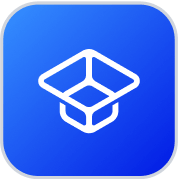
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more