Understanding the Factory Pattern in Computer Programming:
The Factory Pattern is a celebrated design pattern in object-oriented programming. To comprehend it adequately, it's crucial to decipher the essence of design patterns and their usefulness in programming. Consider design patterns as proven solutions to common programming problems. These problems arise repeatedly, and having a preset pattern to tackle them saves much time and effort. Factory Pattern is one of such patterns, and it specifically addresses issues concerning object creation in programming.Defining Factory Pattern: A Basic Overview
In its essential form, the Factory Pattern provides an interface for creating objects in a parent (super) class, but allows subclasses to alter the type of objects that will be created. The Factory Pattern’s primary goal is to define a separate method for object creation, which subclasses can then override to specify the derived type of product.In programming terms, a Factory is essentially a method or function that returns objects of a varying prototype or class.
- Defining a method in a class.
- Declaring the method “factory method” because it creates other objects.
- Allowing subclasses to redefine which class to instantiate.
Interface | An Interface to create objects |
Concrete Class | Class which provides the implementation of the interface method |
Factory Class | Class which uses the Factory method to create objects |
class Factory { createProduct (type) { let product; if (type === "Type1") { product = new ConcreteProduct1(); } else if (type === "Type2") { product = new ConcreteProduct2(); } return product; } }
The Role of Factory Pattern in Object-Oriented Programming
Factory Pattern is a fundamental aspect of Object-Oriented Programming (OOP) chiefly because it provides an approach to code for interface rather than the implementation. This pattern creates the appropriate subclasses based on the data provided to it, promoting ease in code maintenance and adding elegance to the software architecture. The Factory pattern offers several benefits in OOP:- Offers a way to encapsulate a group of individual classes with a common goal into a single unified function.
- It promotes loose-coupling by eliminating the need to bind application-specific classes into the code. The code interacts solely with the resultant interfaces.
- Provides a simple way of extending and re-utilizing the code.
By adopting the Factory Pattern, you're adhering to a principle often quoted in object-oriented design, Redirect Coding To Interfaces Not Implementations.
Real Life Factory Pattern Examples in Programming
Let's illustrate the Factory Pattern usage with JavaScript. Imagine you are creating different notifications in your application - like email notifications, SMS notifications, push notifications, etc. For this, you could employ the Factory Pattern by defining a Notification Factory that creates and returns the appropriate notification based on your input.
class NotificationFactory { createNotification(type) { if (type === 'SMS') { return new SMSNotification(); } else if (type === 'Email') { return new EmailNotification(); } else if (type === 'Push') { return new PushNotification(); } return null; } }In this code snippet, NotificationFactory is a factory for creating different types of notification objects. If we need an 'Email' notification, we provide 'Email' as an argument, and it creates and returns an instance of EmailNotification. Remember, the Factory Pattern simplifies code complexity and boosts maintainability, making it an absolute gem in OOP design. Make sure you adequately understand and utilise this pattern in your programming journey.
Digging Deep into Factory Design Pattern
Insoftware engineering, the factory design pattern, also known as the factory method pattern, primarily focuses on implementing a 'factory' or 'creator' class, responsible for creating objects rather than calling a constructor directly. This pattern promotes cleaner code, resulting in better readability and maintainability.Importance of Factory Design Pattern in Programming
The Factory Design Pattern plays a pivotal role in object-oriented programming. By using a factory method to create different objects, encapsulating the object creation process, you gain a significant advantage in terms of flexibility, and the scalability of the code. In a more complex project, you might deal with a plethora of potential objects that your code might need to instantiate. Coding each object's creation explicitly could quickly become messy and challenging to manage. Therefore, having a factory class that handles this object instantiation process based on inputs it receives, results in far cleaner and more manageable code. Furthermore, employing a factory design pattern is beneficial because:- It provides a simple way of creating objects without exposing the instantiation logic to the client.
- It provides a layer of abstraction that decouples the client code from the concrete classes.
- There is a clear separation of responsibilities. This pattern clearly separates the construction logic of complex objects from the objects themselves.
class Creator { factoryMethod() { return new Product(); } } class Product {}
Differentiating between Factory Pattern and Abstract Factory Pattern
It is important to differentiate between the Factory Pattern and the Abstract Factory Pattern as both play fundamental roles in object-oriented design and are particularly effective in circumstances where the system needs to be independent from how its objects are created, composed, and represented. While they may sound similar and both belong to the "creational patterns" group, they address different problems:- Factory Method is a creation through inheritance: object creation is deferred to subclasses that implement the factory method to create objects.
- Abstract Factory is a creation through composition: object creation is implemented in methods exposed in the factory interface.
A practical way to distinguish the two can be found in how they are instantiated. A Factory Method is usually utilized within a single class, while an Abstract Factory is often implemented with a set of Factory Methods enclosed in one larger class or interface to provide functionality for creating a suite of related objects.
Factory Design Pattern: Practical Applications
The Factory Design Pattern is adopted in an array of real-world applications. Developers utilize this pattern in scenarios where a method returns various types of classes sharing the same common super class or interface based on the provided data, or where the class is only known at runtime. One frequent use of the Factory Pattern is in the implementation of communication protocols. In Internet communication, data packets can be of various types, each requiring a different processing method. By using the Factory Pattern, we can create an object of the appropriate type based on the nature of the received packet and process it, without having the processing method worry about the underlying details of each packet type.class PacketFactory { createPacket(type) { if (type === 'DATA') { return new DataPacket(); } else if (type === 'ACK') { return new AckPacket(); } return null; } }In this code sample, `PacketFactory` is a factory for creating different types of network packet objects. By calling `createPacket`, you can get an object of the correct packet type, ready to be processed. This demonstrates but one of the many practical ways you can deploy the Factory Design Pattern in programming.
Exploring the Factory Pattern in Java
In the world of software development, design patterns serve as standardised programming templates that offer optimal solutions to common issues that can arise during software creation. The Factory Pattern is among these renowned design patterns. As part of the Creational Design Pattern group, it takes charge of object creation processes, focusing heavily on the Java programming language due to its suitability for object-oriented application development.Introduction to Factory Pattern Java
In the context of Java, the Factory Pattern is employed to build objects from a common interface or superclass. The Factory handles the instantiation process, rendering the application independent from the foundational logic of creating these objects. Java heavily emphasises object-oriented programming, and the Factory Pattern fits neatly into this paradigm by allowing for the creation of objects without exposing the specifics of their creation. This abstraction of the creation process leads to more robust, flexible, and maintainable code. The Factory Pattern's skeletal framework in Java comprises:- Super Class: An abstract class specifying object creation methods
- Sub Classes: They provide the implementation for the super class’s creation method
- Factory Class: This class interfaces with the client and provides access to the best subclass based on input parameters
In Java, the Factory is a class with a method that creates and returns instances of varying classes based on the input parameters it receives.
Factory Pattern Java: Code Examples
To better comprehend the Factory Pattern's practical use in Java, let's consider an illustrative example. Say we have an application that draws different geometric figures like circles, rectangles and squares. The Factory Pattern can be applied productively here.abstract class Shape { abstract void draw(); } class Circle extends Shape { void draw() { /* Circle drawing logic */ } } class Rectangle extends Shape { void draw() { /* Rectangle drawing logic */ } } class ShapeFactory { static Shape getShape(String type) { if (type.equals("Circle")) { return new Circle(); } else if (type.equals("Rectangle")) { return new Rectangle(); } return null; } }Here, `ShapeFactory` is a simple Factory class with a static method `getShape`. It takes a String input and returns an instance of the appropriate subclass of `Shape`. This way, the complexity of object creation is hidden from the main application code. This coding example provides a glimpse into a real-world application of the Factory Pattern in Java, demonstrating how it improves overall code structure and enables flexibility in object creation.
Tips and Tricks for Implementing Factory Pattern in Java
Effectively implementing the Factory Pattern in Java can substantially streamline the process of object creation, thereby reducing code complexity and improving maintainability. Below are some tips that can guide you:- Identify the scenarios where the application needs to create an object of different types based on some conditions.
- Define an interface or an abstract class which will be the return type of our factory method.
- Create subclasses that implement the interface or extend the abstract class, and encapsulate them in a factory class.
- Replace all explicit object creation in the application code with calls to the factory method.
Advantages and Complexity of Factory Pattern
Understanding Factory Pattern Complexity
The complexity of the Factory Pattern lies primarily in its abstraction of the object creation process. It's essential to understand this concept to properly implement the pattern and appreciate its utility in complex software development scenarios. The Factory Pattern acts as an intermediary that creates objects on behalf of the client code. Therefore, the client code doesn't directly instantiate objects using the `new` operator. Instead, the Factory takes the responsibility of determining which object to create based on the parameters it receives. From a complexity perspective, this pattern demands a good grasp of programming principles such as inheritance and abstraction. The Factory Pattern relies on a superclass or interface common to all object types the Factory can create. This superclass defines the API the factory-produced objects will adhere to, maintaining uniformity.abstract class Product { abstract void operation(); } class ConcreteProductA extends Product { void operation() { /* Logic of ConcreteProductA */ } } class ConcreteProductB extends Product { void operation() { /* Logic of ConcreteProductB */ } }Here, `Product` is a common superclass for `ConcreteProductA` and `ConcreteProductB`. `Product` abstracts the method `operation()`, which is implemented by each subclass. This enables you to call the `operation()` method on any object created by the Factory without knowing its concrete type. For designing classes within the Factory Pattern, understanding these OOP principles is crucial. Furthermore, a well-structured Factory Pattern design enables you to extend your application with new classes without modifying the client code.
Advantages of Using Factory Pattern in Programming
The Factory Pattern offers several advantages in programming, enhancing code organization, maintainability, and scalability. Firstly, it provides a clean and orderly way to initialise and use objects. Instead of scattering object creation logic throughout your code, the Factory centralises it in a single location.class Factory { createProduct(type) { switch (type) { case 'A': return new ConcreteProductA(); case 'B': return new ConcreteProductB(); } } }This concentrating of object creation results in simplified and more adaptable code. It's easier to change, upgrade, or maintain the product classes without causing problems in the application code. Secondly, the Factory Pattern adheres to the principle of 'separation of concerns'. In this construct, the Factory class solely manages object creation, while the client code just uses the objects. This segregation of responsibilities enhances code readability and testability. There's a separation between the client and the specific product classes that the Factory instantiates. The client code only needs to interact with the abstract interface common to all product classes, yielding more flexible code. Lastly, the Factory Pattern proves advantageous in cases involving complex objects. Instead of hardcoding the setup sequence for such objects, you encapsulate it inside a factory method. This approach simplifies client code and keeps the nasty creation logic hidden away inside the Factory.
Minimising Complexity with the Factory Pattern
The Factory Pattern helps manage complexity in large scale, object-oriented programs. It enables you to create new objects while hiding the complexity of their creation and initialisation from the client code. When dealing with complex or high-level modules that can create multiple types of objects, the Factory Pattern can simplify code by encapsulating the creation logic. The Factory acts as a single point of object creation, simplifying object management throughout your code. Here's how the Factory Pattern reduces complexity:- It avoids tight coupling between product classes and the client code.
- It separates the responsibilities of object creation and object usage, aiding code organization and clarity.
- It improves code reusability and makes it more straightforward to add new product types, fostering code extendibility.
Further Exploring Factory Method Design Pattern
What is the Factory Method Design Pattern?
The Factory Method Design Pattern, often referred to as a 'virtual constructor', belongs to a class of creational design patterns whose primary responsibility is object creation. It provides an interface or class with a method that creates an object, with the subclasses determining the class of object to be instantiated. Importantly, these subclasses that implement the factory method are often derived from an interface or an abstract base class. In the Factory Method Pattern, the object creation process is encapsulated within a single method. This factory method returns an instance of the object required by the calling code, invariably introducing a level of indirection and making the client code impervious to changes in the actual implementation of the object. This level of abstraction increases code flexibility and is a standard tool in the programmer's arsenal to combat the rigidity of direct object creation. Without a doubt, understanding the Factory Method pattern requires a good understanding of Object-Oriented Programming (OOP) principles, involving abstraction, encapsulation, and polymorphism.
abstract class AbstractProduct { public abstract void operation(); } abstract class Creator { public abstract AbstractProduct factoryMethod(); } class ConcreteProductA extends AbstractProduct { public void operation() { /* Implementation of the operation for ConcreteProductA. */ } } class ConcreteCreatorA extends Creator { public AbstractProduct factoryMethod() { return new ConcreteProductA(); } }
Factory Method Design Pattern vs Factory Pattern: The Difference
It's not uncommon to find the terms Factory Method Design Pattern and Factory Pattern used interchangeably or causing confusion among developers. Although both are creational design patterns and deal with object creation, the noteworthy distinctions between them draw your attention to the subtleties that exist within design patterns. The classic Factory Pattern, also known as Simple Factory, involves a single Factory class responsible for generating instances of different classes. It uses a method that, based on input parameters, creates and returns objects. The major drawback here is that the Factory class tends to become bulky and violate the Single Responsibility Principle, as adding new types would lead to modifying the Factory class. Contrastingly, the Factory Method Pattern encapsulates object creation in a method, and this method functions as a 'factory of objects'. Instead of a single Factory class, this pattern defines an interface for creating an object but allows the subclasses that implement this interface to alter the type of object that will be created. Therefore, the Factory Method Pattern is more dynamic and flexible, keeping intact the Open/Closed Principle of object-oriented design, which suggests that "software entities should be open for extension but closed for modification".Implementing the Factory Method Design Pattern: A Comprehensive Guide
Implementing the Factory Method Design pattern can be broken down into comprehensive steps. Knowing each step helps streamline the process, embedding a more in-depth understanding which, in turn, amplifies the pattern's usefulness. The first step is the identification of objects having similar behaviour but varying operations. This helps separate the common functionality, which other classes can then inherit. The second step is the creation of an abstract base class or an interface which includes an abstract factory method. This abstract factory method would typically serve as a placeholder that needs to be implemented by subclasses.
public abstract class AbstractCreator { public abstract AbstractProduct factoryMethod(); }
public class ConcreteCreatorA extends AbstractCreator { public AbstractProduct factoryMethod() { return new ConcreteProductA(); } }
AbstractCreator creator = new ConcreteCreatorA(); AbstractProduct product = creator.factoryMethod(); product.operation(); // Executes ConcreteProductA's operation.
Factory Pattern - Key takeaways
- The Factory Design Pattern is a key instrument in object-oriented programming, which facilitates flexibility, scalability and manageability through encapsulation of the object creation process.
- Differentiating the Factory Pattern and the Abstract Factory Pattern is important: Factory Method is object creation through inheritance, while Abstract Factory Pattern involves creation through composition.
- Factory Pattern proves highly useful in real-world applications, including implementation of varied communication protocols as it enables objects creation without exposing creation particulars.
- In Java, Factory Pattern builds objects from a common interface or superclass, with the Factory handling the instantiation process – a significant benefit in Java's object-oriented programming framework.
- The Factory Pattern, despite its complexity, offers multiple advantages in programming – centralising object creation logic for greater adaptability, segregating responsibilities between object creation and usage, and encapsulating setup sequences for complex objects.
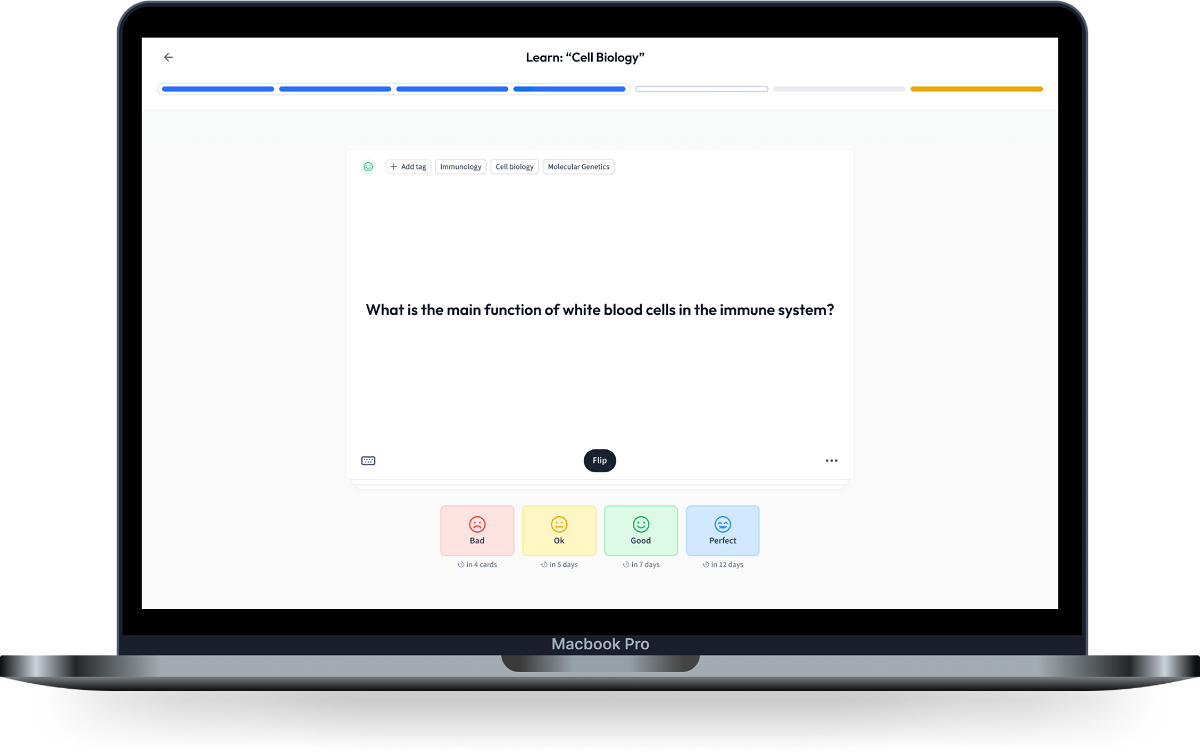
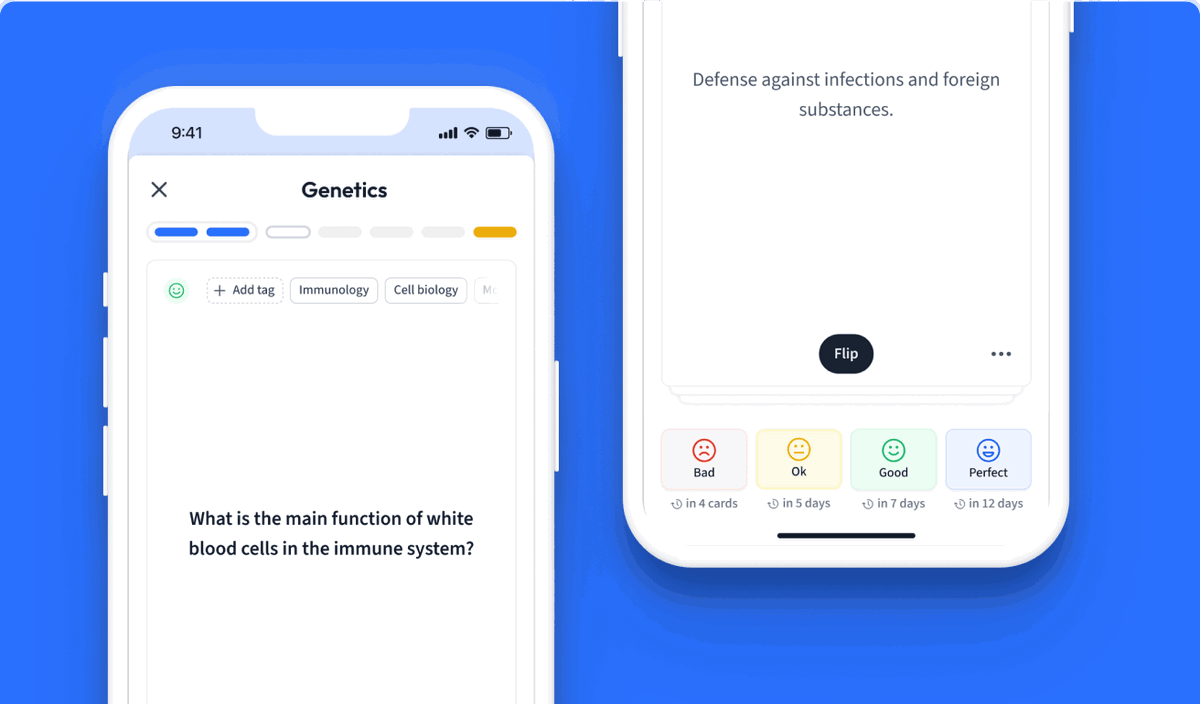
Learn with 15 Factory Pattern flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Factory Pattern
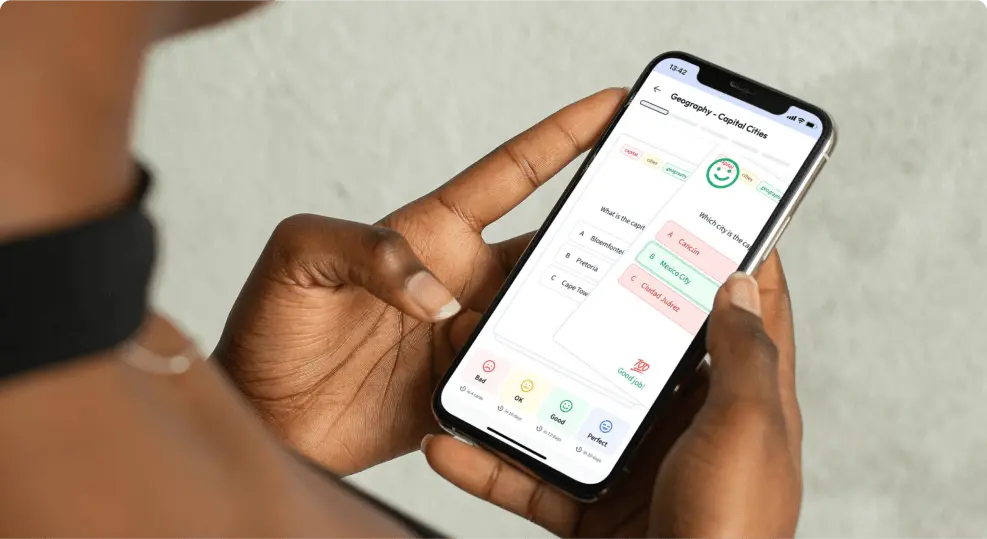
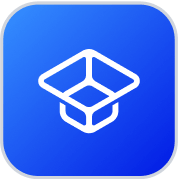
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more