Understanding the Java Queue Interface
In your journey through Computer Science and programming, you'll encounter various data structures and interfaces. One such interface in the Java programming language is the Java Queue Interface, which is a part of the Java Collections Framework. You'll find it under the Java.util.package. So, let's delve deeper into the subject and gain an understanding of how the Java Queue Interface works, and its significance.
Definition and Function: What is the Java Queue Interface?
Queue Interface in Java is defined as an ordered list of objects with its use limited to insert elements at the end of the list, known as enqueue, and to remove elements from the start of the list, known as dequeue. The Queue is a FIFO (First In First Out) data structure.
The primary purpose of the Queue interface is to hold elements in preparation for processing. Unlike Lists or Sets, Queues typically, but do not necessarily, order elements in a FIFO (first-in-first-out) manner. Except classes like PriorityQueue, that orders elements according to their natural ordering or based on a comparator provided at queue construction, most others hold elements in an order specific to the implementation.
It is interesting to note that while all queue classes in the Java Collections Framework are concurrent, not all allow null elements. It is essential to check the specification of the queue class you're using to comprehend its exact behaviour.
//Some of the common operations performed using Queue Interface are: queue.add("element"); queue.element(); queue.remove();
Core Components: Queue Interface Methods in Java
To provide efficient utilisation, Java Queue Interface provides several methods designed specifically for queue operation.
Here is a list of core methods:
- add(e): This method adds an element e at the end of the queue. Return true on success.
- element(): Retrieves, but does not remove, the head of this queue.
- remove(): Retrieves and removes the head of the queue.
Other methods include :
- offer(e)
- poll()
- peek()
Below is a table that explains the differences between these methods:
Method | Description |
offer(e) | Inserts the specified element into this queue if it is possible to do so immediately without violating capacity restrictions |
poll() | Retrieves and removes the head of this queue, or returns null if this queue is empty |
peek() | Retrieves, but does not remove, the head of this queue, or returns null if this queue is empty |
For example, using Queue methods in Java would look like:
QueueThis code segment creates a queue named cars, and adds various car names. Then it uses the peek() method to retrieve the head of the queue (BMW) and stores it in the variable firstInQueue.cars = new LinkedList<>(); cars.add("BMW"); cars.add("Audi"); cars.add("Ford"); String firstInQueue = cars.peek();
Implementing the Java Queue Interface
The Java Queue Interface is implemented using various classes like PriorityQueue, LinkedList, and ArrayBlockingQueue. The precise choice of class depends on the specific requirements of your application. Nonetheless, the basic procedure remains constant across all these classes. Let's have a look at the steps to take when implementing the Java Queue Interface.
Step-by-step Guide: How to Implement Queue Interface in Java
Implementing a Java Queue Interface involves a series of steps which you can follow to master the process. Let's break it down piece by piece:
1. Import the Queue Package:First of all, you need to import the java.util.Queue package into your Java program. You can do this by adding the following line of code at the top of your Java file:
import java.util.Queue;2. Create a Queue Object:
Next, create an object of the Queue interface. You can use any class that implements the Queue interface, like LinkedList or PriorityQueue. Here's how you can create a Queue object:
Queue3. Use the add() or offer() method to add elements:q = new LinkedList<>();
Once you have a Queue object, you can start adding elements to it. Use the add() method or offer() method to append elements to the end of the Queue. Remember, the add() method throws an exception if the element cannot be inserted, while the offer() method simply returns false:
q.add(1); q.offer(2);4. Use the remove() or poll() method to remove elements:
The remove() or poll() method can be then employed to delete elements from the queue:
q.remove(); q.poll();5. Use the element(), peek() methods to access elements:
Finally, the element() or peek() method is used to access the head of the queue, without removing it:
int x = q.peek(); int y = q.element();
Often, Queues are used when we want to maintain a list with a priority. That's when we reach for classes like PriorityQueue.
Practical Application: Java Queue Interface Implementation Examples
Now, let's take a real-life scenario to understand how the Queue Interface can be used in Java programming. Consider a printer's print queue where documents wait in line to get printed.
Consider a scenario where you need to arrange several integers in a queue and then process each one sequentially. The application for this could be, for instance, as mundane as a printer queue, where the documents wait in a queue to get printed.
Here is how you can create such a queue using Java's Queue Interface:
import java.util.Queue; import java.util.LinkedList; public class Main { public static void main(String[] args) { // Create and initialize a Queue using a LinkedList QueueIn the above example, the queue initially contains [5, 15, 25, 35]. The remove() method removes the head of this queue (5), leaving it as [15, 25, 35].waitingQueue = new LinkedList<>(); // Adding new elements to the end of the Queue waitingQueue.add(5); waitingQueue.add(15); waitingQueue.add(25); waitingQueue.add(35); System.out.println("waitingQueue : " + waitingQueue); // Remove an element from the front of the Queue int removedNumber = waitingQueue.remove(); System.out.println("Removed Element : " + removedNumber); System.out.println("waitingQueue : " + waitingQueue); } }
Now that you know how to implement the Java Queue Interface, you can try your hands at creating complex Java programs using this special data structure.
Comparing Java Queue Interface Methods
In the Java Queue Interface, different methods serve different purposes, allowing the interface to be efficiently used according to various programming needs. We'll focus on comparing, in depth, two specific methods: the element() method and the peek() method - both crucial methods used to examine the element at the head of the Queue.
Analysis: Difference between Element and Peek Method of Queue Interface Java
The element() and peek() methods in the Queue Interface receive the element at the head of the queue. While from a bird's eye perspective, both seem alike, their functionality diverges when the queue is empty.
The element() method, when called on an empty queue, throws a NoSuchElementException. This method can be useful when you wish to handle empty queues explicitly and prevent the program from continuing further operations until the queue is no longer empty.
//Throws NoSuchElementException if the Queue is empty. Queuequeue = new LinkedList<>(); queue.element();
On the other hand, the peek() method, when executed on an empty queue, returns null. Hence, you can use the peek() method if you want your program to continue running even when the queue is empty, without leading to exceptions.
//Returns null if the Queue is empty. Queuequeue = new LinkedList<>(); queue.peek();
Therefore, while both methods serve the purpose of viewing the element at the head of the queue, careful consideration of the conditions to be maintained in the queue will help determine the appropriate method to use.
Understanding the Syntax: Java Queue Interface Syntax
In order to effectively use the Java Queue Interface, it's crucial to familiarise oneself with its syntax. Understanding the structure and commands of the interface allows you to effectively create and manipulate data with it.
The declaration of a Queue interface generally takes the form of:
QueuequeueName = new <>()
'objectType' is the type of object the queue will hold, and 'queueName' is the name you choose for the queue. The 'class name' is the title of the class you want to implement.
Here is an example of a Queue of integers implemented with the LinkedList class:
QueuenumberQueue = new LinkedList<>();
With regard to the syntax of the queue's methods, here is a brief overview:
- Add elements: queueName.add(element);
- Remove elements: queueName.remove();
- Peek at the head: queueName.peek();
- Get the head element: queueName.element();
Bear in mind that all these methods (except peek()) throw an exception when called on an empty queue.
Here's a comprehensive example showcasing how a Queue, named fruitQueue, can be declared, followed by adding elements to it, viewing the head element, and finally, removing elements from the queue:
QueuefruitQueue = new LinkedList<>(); fruitQueue.add("Apple"); fruitQueue.add("Banana"); fruitQueue.add("Cherry"); String fruit = fruitQueue.element(); // Returns 'Apple' fruitQueue.remove(); // Removes 'Apple' from the Queue
Remember, mastering the syntax of the Java Queue Interface is one of the pivotal steps towards successful Java programming.
Diving into Examples of Java Queue Interface
Let's dive deeper into the practical environment of using the Java Queue Interface. This will solidify your understanding of how these implementations come about and can be put to use in the real world. Let's take a look at a step-by-step approach and blow-by-blow analyses of Java Queue Interface examples.
A Hands-On Approach: Java Queue Interface Example
So far, you've learned the basics of creating and manipulating a queue, with a simple example putting integers into a queue. Now let's deal with a more complex scenario, using an object of a custom class, rather than a primitive type like 'int'. This will enable you to see how to use the Queue Interface in more advanced contexts.
Consider a scenario where you have a company, and its staff members are objects of the class 'Employee'. Employees have a name and an ID number, and you want to create a priority queue of employees based on their ID number.
import java.util.PriorityQueue; import java.util.Queue; import java.util.Comparator; // Employee class class Employee { String name; int id; // constructor public Employee(String name, int id) { this.name = name; this.id = id; } public String getName() { return name; } public int getId() { return id; } } // Main Class public class Main { public static void main(String args[]) { // create a priority queue of employees Queueemployees = new PriorityQueue<>( Comparator.comparing(Employee::getId) ); // add employees to the queue employees.add(new Employee("John", 2)); employees.add(new Employee("Emma", 1)); employees.add(new Employee("Alex", 8)); // remove and print employees while (!employees.isEmpty()) { Employee e = employees.remove(); System.out.println("Employee ID: " + e.getId() + " Name: " + e.getName()); } } }
Here, a priority queue of 'Employee' objects is created. The 'Comparator.comparing(Employee::getId)' part ensures that employees are ordered in the queue based on their ID numbers. When the employees are removed from the queue and printed, they're listed in ascending order of their ID numbers. Thus, you can see how a priority queue can be used effectively in a practical scenario.
Additional Practical Exercises: More Examples of Java Queue Interface
The best way to familiarise yourself with the Java Queue interface is to practice with more examples! Here's an additional example to help you understand the workings of Queue Interface better.
In this example, let's create a queue of book titles that need to be returned to a library. A 'book return queue' allows us to keep track of the order books are returned in.
import java.util.LinkedList; import java.util.Queue; class Main { public static void main(String[] args) { Queuebooks = new LinkedList<>(); // Add books to the Queue books.add("Moby Dick"); books.add("War and Peace"); books.add("1984"); System.out.println("Books Queue: " + books); // Process the returned books while (!books.isEmpty()) { System.out.println("Processing returned book: " + books.poll()); } } }
In the example above, you create a Queue of Strings to represent book titles. The titles are then added to the queue using the add() method, indicating they need to be returned. Later on, you can process the books (symbolised by removing them from the queue and printing their titles) in the order they were added to the queue.
These illustrative examples help in improving your familiarity with the operational aspects of the Java Queue Interface, allowing you to facilitate their usage in more complex software projects with ease and confidence.
Beyond the Basics: Advanced Topics in Java Queue Interface
Moving beyond the fundamentals, the Java Queue Interface offers a range of advanced topics that are crucial to comprehend for a more robust and comprehensive understanding of Java programming. While honing your skills with the elementary methods and operations certainly forms a robust foundation, getting to grips with advanced topics can equip you with the tools needed to solve much more complex programming problems.
Delving Deeper: Advanced Queue Interface Methods in Java
For in-depth insights into the Java Queue Interface, let's explore some of the more advanced methods that this interface provides. Understanding their capabilities and attributes will enhance your versatility in dealing with various queue-related scenarios in Java programming.
The offer() method attempts to add an element to the queue and, unlike the add() method, returns a boolean value to signify success or failure instead of throwing an exception.
queue.offer(element); // Returns true if the element was added successfully, false otherwise.
You can use the offer() method when you want to try to add an element, but don't want a failed operation to throw an exception and stop your program.
The poll() method is another useful tool to retrieve and remove the head of the queue. Like the remove() method, it returns the head element and removes it, but differs in the way it handles an empty queue.
queue.poll(); // Returns null if the queue is empty.
By returning null instead of throwing an exception when called on an empty queue, the poll() method allows your program to keep running smoothly. This method proves especially useful when you want to continuously drain a queue without worrying about the queue being empty.
The clear() method is an efficient way to delete all elements in the queue. This method does not return any value, but upon its call, it removes all elements from the queue.
queue.clear(); // Removes all elements from the Queue.
Use the clear() method when you want to quickly empty a queue without having to retrieve and remove each element.
Expert Tips on Implementation: Advanced Java Queue Interface Implementation Topics
In terms of advanced implementation, the Java Queue Interface can be used in a wide array of applications. Whether it's priority handling in resource sharing systems, data buffering, or simulations, a proper comprehension of advanced usage scenarios is beneficial.
PriorityQueue is a priority queue implementation in Java that provides the highest priority element from the queue whenever requested. This means that rather than just first-in-first-out access, PriorityQueue sorts its elements based on a rule or comparator provided at runtime.
PriorityQueuequeue = new PriorityQueue<>(); queue.offer(3); queue.offer(1); queue.offer(2); Integer topPriorityElement = queue.peek(); // topPriorityElement now holds the value 1.
PriorityQueue becomes particularly handy when you want a subset of the elements to take precedence over the others based on a particular rule.
In multithreading scenarios, it's important to ensure that a queue is thread-safe, meaning that it can be used safely by multiple threads at the same time. Java provides two thread-safe Queue implementations: ConcurrentLinkedQueue and LinkedBlockingQueue.
QueuesafeQueue = new ConcurrentLinkedQueue<>(); // A non-blocking thread-safe queue. Queue safeBlockingQueue = new LinkedBlockingQueue<>(); // A blocking thread-safe queue.
The difference between these two lies in what happens when a thread tries to dequeue from an empty queue. As their names suggest, ConcurrentLinkedQueue continues, returning null, while LinkedBlockingQueue waits until there is an element available to dequeue. Both queue types can be used efficiently depending on the requirements of your Java multithread applications.
As you delve deeper into the Java Queue Interface, these advanced topics pave the way for you to explore the more complex and sophisticated aspects of queue handling in Java - from thread-safe queues to prioritising queue elements.
Java Queue Interface - Key takeaways
- Java Queue Interface: This Interface is implemented using various classes like PriorityQueue, LinkedList, and ArrayBlockingQueue. The choice of class depends on the specific requirements of your application.
- How to implement Queue interface in Java: First, import the java.util.Queue package into your Java program. Create an object of the Queue interface (LinkedList or PriorityQueue), then use the add() or offer() method to add elements to it, the remove() or poll() method to remove elements, and the element() or peek() methods to access elements.
- Difference between element and Peek method of Queue Interface Java: Both methods are used to access the head of the queue, however, the element() method throws a NoSuchElementException when the queue is empty while the peek() method returns null if the queue is empty.
- Java Queue Interface Syntax: To declare a Queue interface, use "Queue
queueName = new <>()", where objectType is the type of object the queue will hold, queueName is the name you choose for the queue, and the class name is the title of the class you are using to implement the Queue. - Advanced Queue Interface Methods in Java: The 'offer()' method attempts to add an element to the queue and, unlike the add() method, it returns a boolean value to signify success or failure instead of throwing an exception. The 'poll()' method retrieves and removes the head of the queue, or returns null if the queue is empty.
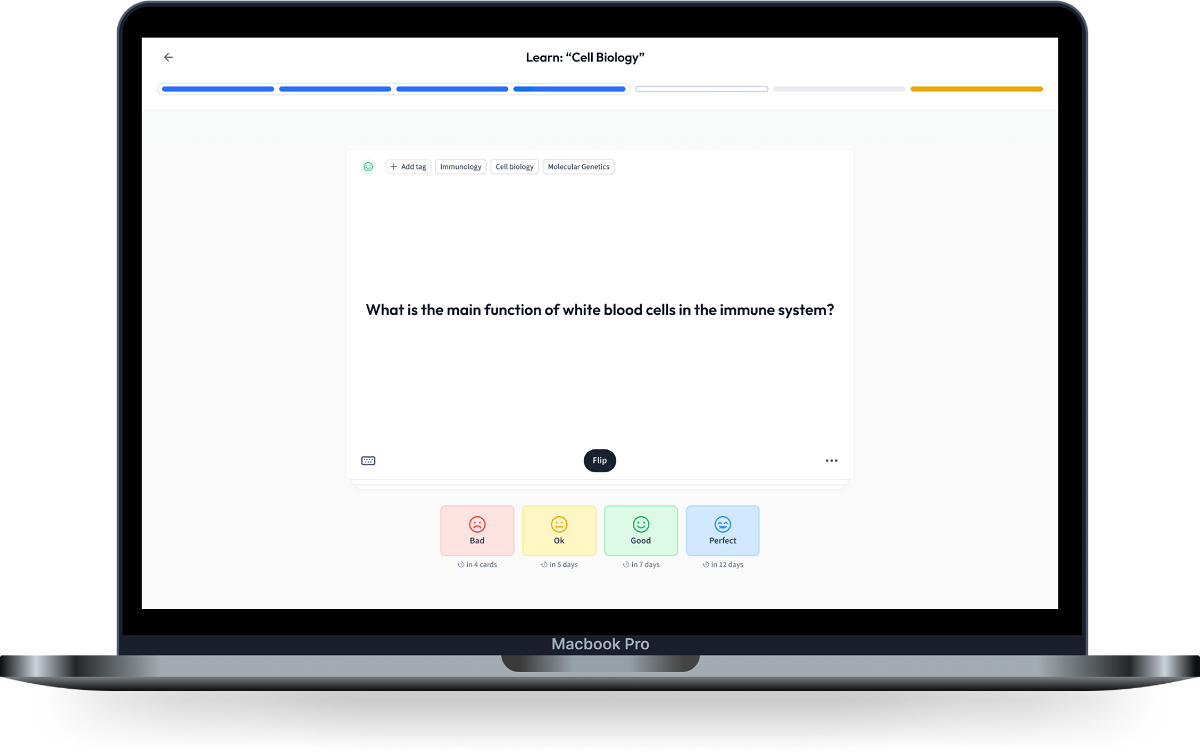
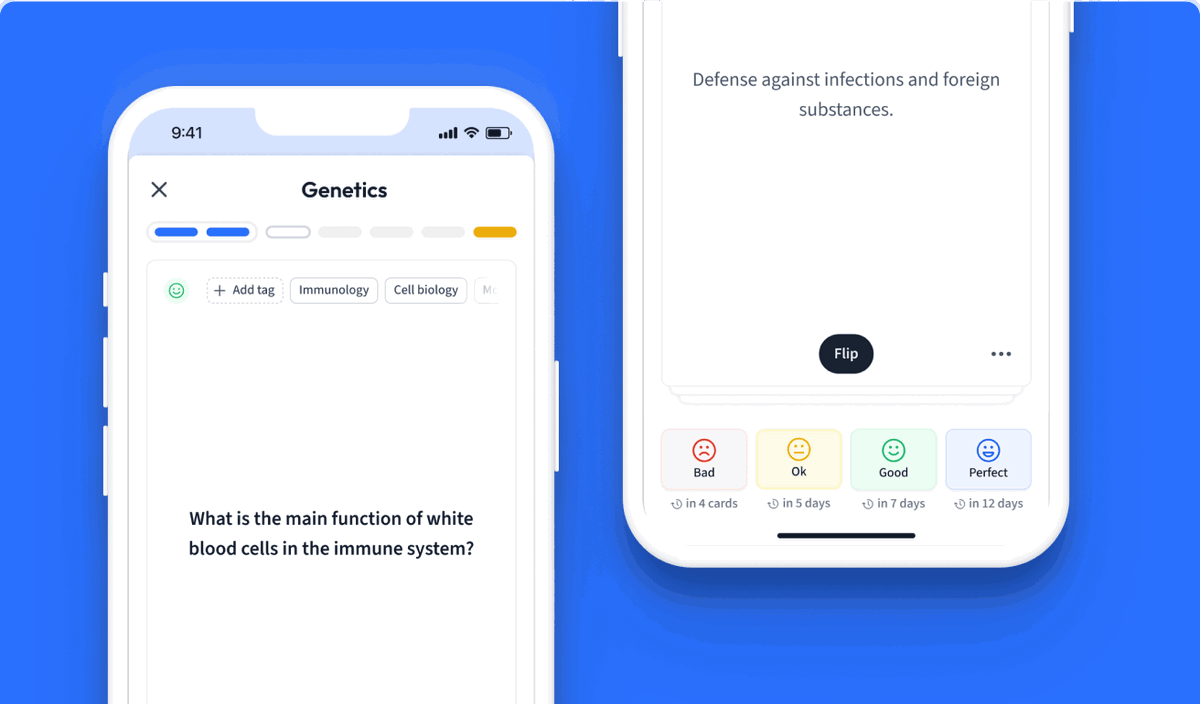
Learn with 15 Java Queue Interface flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Java Queue Interface
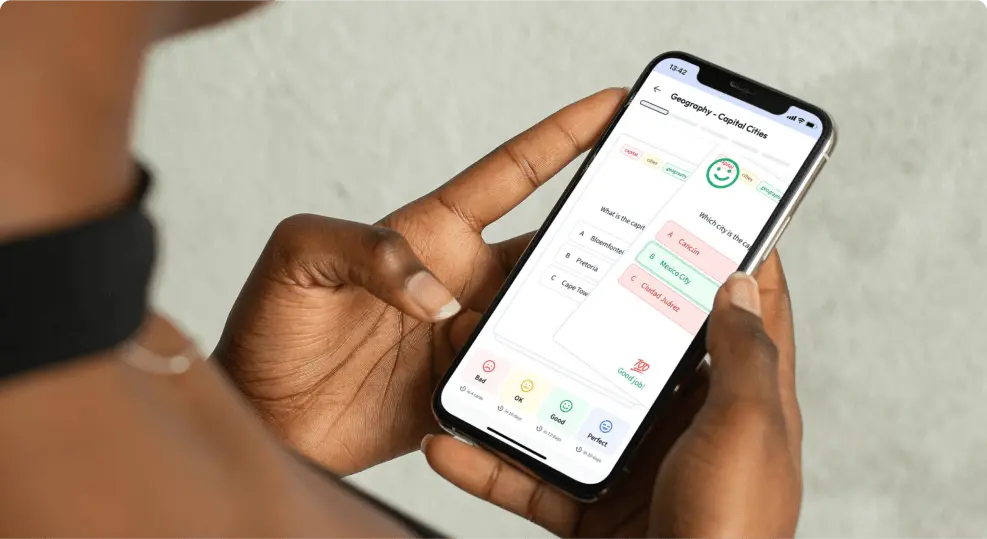
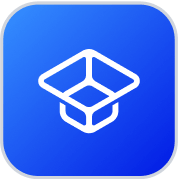
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more