Understanding the Model View Controller in Computer Science
In the realm of computer software architecture, you might have heard of the Model View Controller (MVC) design pattern on multiple occasions. It is a subject you need to have a firm grasp on as it’s a significant component of web development and many programming languages.What exactly is a Model View Controller?
A Model View Controller, commonly referred to by its acronym 'MVC', is a widely used design pattern in software engineering and web development. It aids in organising your code to ensure that it remains manageable as it scales. This architectural pattern isolates the development of an application into three interconnected components.The Model View Controller separates the manipulation of information, user interface, and control into the Model, View, and Controller components respectively.
Components involved in a Model View Controller
Understanding the components of the MVC is crucial to fully grasping the concept as a whole.Model | It embodies the application's data and business logic. It manages and maintains the data of an application. |
View | It determines what the users see—the User Interface. It presents the model’s data to the user. |
Controller | It acts as an intermediary between the Model and the View and controls the flow of information between them. |
The role of Model in Model View Controller
The Model is one of the key components of MVC and is responsible for managing applications' data. The Model classifies the core business rules and data. It communicates with the database, carries out necessary data processing and, and returns the result. Consequently, regardless of what the user sees or how they interact with it, the back-end data handling will always remain consistent.Key functionalities of the Model Component
The Model in MVC is often misunderstood as just data. However, it has many roles beyond just representing data. It performs key tasks such as:- Fetching the data from the database
- Performing necessary validations
- Processing the data
- Updating the data
For instance, consider an e-commerce application. If a user wants to check out their shopping cart, the Model will gather the relevant data (items in the cart), calculate the total cost, check inventory for stock levels, and numerous other tasks. All this happens at the Model level in MVC architecture.
Exploring the Design Pattern of Model View Controller
The essence of understanding MVC can be fruitfully delved into when you dissect its concept as a Design Pattern. Form a conceptual standpoint, this implies how the MVC organises and structures different classes and objects to collaborate and create an application.Decoding the Model View Controller Design Pattern
As a design pattern, the MVC reduces the complexities involved in the development process. It separates the concerns into different sections, an invaluable asset when dealing with large-scale applications, promoting modularity, flexibility, and maintainability. To exemplify this more vividly:- In MVC, each module is developed independently. This means each component, whether the Model, the View, or the Controller, can be updated or altered without affecting the functioning of the others.
- This level of modularity has significant implications because it allows for parallel development. For instance, the View can be designed simultaneously as the database structure is being developed within the Model, leapfrogging application development time.
- The MVC pattern is extremely flexible, making it easier to adapt to changes. If you want to change the user interface or the database of your application, you can do so without modifying the entire codebase.
- The separation of concerns also boosts the maintainability of the code. Since the code is organised into separate components, debugging becomes faster and more efficient. It also makes it easier for new developers to understand and contribute to the project.
How the Model View Controller design impacts application development
The MVC design pattern profoundly impacts how applications are developed and managed. It provides a standard that developers can follow to result in clean and organised code. Let's consider an example to see this in action. In a traditional application development process where MVC is not implemented, consider trying to add a new feature or debugging a piece of code. It could require trawling through thousands of lines of code to find exactly where the change needs to happen. This would be time-consuming and potentially lead to new errors. Now, contrast this with an application developed using MVC. To add a new feature, you'd know exactly where to place the code. If the feature is related to data management, it will go into the Model. If it's a new user interface element, it will go into the View. If it's about application flow control, it belongs to the Controller. Consequently, this dramatically reduces the time spent locating the right place for the code and minimises the risk of unintentionally introducing new bugs.Common Design Patterns vs Model View Controller Design Pattern
In Computer Science, a design pattern can be thought of as a template—a reusable solution to a common problem in software design. There are several commonly adopted design patterns other than MVC, such as Singleton, Factory, and Observer. While these patterns are beneficial in specific scenarios, MVC stands apart when it comes to developing enterprise-level applications.Design Pattern | Description | MVC Comparison |
Singleton | Used when we need to ensure that only one instance of a particular class is created and a global point of access to it is provided. | While Singleton controls access to a resource, MVC is more about structure and flow. MVC can, however, utilise Singleton pattern for parts of its functionality, like a single database connection. |
Factory | Provides an interface for creating families of related objects without specifying their concrete classes. | MVC and Factory pattern serve different purposes, MVC is structural and Factory is creational. However, MVC's Model might employ a Factory pattern to create various data objects. |
Observer | Defines a dependency between objects so that when one object changes state, all its dependents are notified and updated automatically. | The essence of MVC aligns with the Observer pattern. The View is essentially an observer of the Model. Any change in Model's state will be notified to the View for it to update reflectively. |
Key Aspects of Model View Controller Architecture
Diving deeper into the complexities of the Model View Controller architecture reveals a world that thrives on clear communication between its components and capitalises on the segregational power it bestows upon developers, giving them the freedom to individually perfect each element of an application independently.Detailed Overview of Model View Controller Architecture
The ground-breaking aspect of MVC Architecture lies not just in the division of responsibilities but also in the communication that transpires between the components. The MVC architecture runs on an interconnected navigator which creates a sinusoidal flow of data; each module talks to one or at most two other components, forming a closed feedback system. As an authoritative example, when a user interacts with the View (like clicking a button), the View communicates this event to the Controller. The Controller, understanding this input, brings about the required changes in the Model (like updating a data value). The Model, after its update, informs the View, which subsequently changes the UI for the user, adding that extra item to the shopping cart. This approach provides distinct hues to the MVC's architectural painting:- The Model is only aware of the Controller, and no information about the View reaches it.
- The View, although it receives updates from the Model, it never makes any changes to it but relies on the Controller to dictate those changes.
- The Controller maintains a dual communication channel; it receives user inputs from the View and manipulates data in the Model.
Understanding the communication process in Model View Controller Architecture
In the MVC architecture, the communication between the components can be illustrated in five significant steps in the context of a simple user request:- The user interacts with the user interface in some way (for example, by clicking a mouse button).
- The Controller handles the user input and changes the state of the Model.
- The Model notifies the Controller after it has changed its state.
- The Controller sends the updated data to the View.
- The View gets the data and updates the user interface so that the user can see the change.
class Model: services = { 'email': { 'number': 1000, 'price': 2,}, 'sms': { 'number': 1000, 'price': 10,}, 'voice': { 'number': 1000, 'price': 15,}, } class View: def list_services(self, services): for svc in services: print(svc, ' ') class Controller: def __init__(self): self.model = Model() self.view = View() def get_services(self): services = self.model.services.keys() return(self.view.list_services(services))In this code, you can follow the sequence of communication from Model to Controller and then to View, ensuring no direct interaction between Model and View.
Benefits of using Model View Controller Architecture
Amid numerous ways of building applications, the MVC stands out with its distinct advantages that lend towards more efficient programming practices. Demonstration of these benefits with practical insights can profoundly influence your understanding of its prominence.Enhancements in Code Quality and Productivity
MVC architecture, with its clear separation of responsibilities, leads to easier maintenance of code and increases overall productivity. 1. By following the MVC principles, developers can concurrently design, develop and test different components. This parallel development doesn't impose any restrictions as each component interacts with only a limited aspect of the others. Thus, it saves significant development time by avoiding a sequential wait and reducing eventual bottlenecks. 2. Subsequent changes or additions to the system are also facilitated. As each component operates independently, you can alter one without the need for modifying the others. 3. In terms of maintainability and testing, a compartmentalised and modular code structure tremendously boosts debugging. Identifying and fixing a bug in one module won't inflict any damaging ripple effects on the others. 4. Lastly, this architecture also promotes code reusability. As the components are independent, they can be easily reused in different parts of the application or even in different applications altogether, reducing redundant coding efforts and leading to cleaner, more efficient code. In essence, MVC introduces an invigorating breath of modularity, efficiency, and simplicity into your coding practices. In unison, these benefits optimise productivity and lend your codes a superior quality that stands the test of readability, scalability and maintainability with finesse.Practical Examples of Model-View-Controller
It is supremely important to relate this understanding of the Model-View-Controller architecture with real-world scenarios, bristling with all its numerous advantages and complexities. In this regard, this section will walk you through actual instances of MVC implementation and periodically analyse those examples for a more profound understanding.Implementing the Model View Controller in Real Projects
To better understand MVC, there's nothing quite like immersing oneself in a real project that employs its principles. For programming languages like Java, ASP .NET, and PHP, MVC plays a strong role in many real-world applications. Let's explore some examples. A practical example of Model-View-Controller can be seen in a basic web application developed using Java. The user requests a webpage, and the request goes to the Controller. The Controller determines the appropriate Model and invokes its functions to retrieve the required data. Once the Model has fetched the data, it returns it to the Controller, which subsequently renders the appropriate View, populating it with the data retrieved by the Model. This data is presented to the user in their browser. Consider a short example from a Java-based web application demonstrating the MVC pattern://Model public class User { private String name; public User(String name){ this.name = name; } public String getName(){ return this.name; } } //View public class UserView { public void printUserDetails(String userName){ System.out.println("User: "+userName); } } //Controller public class UserController { private User model; private UserView view; public UserController(User model, UserView view){ this.model = model; this.view = view; } public String getUserName(){ return model.getName(); } public void setUser(String name){ model.setName(name); } public void updateUserView(){ view.printUserDetails(model.getName()); } }In the above snippet, the separation of concerns is clear. The `User` class (Model) is an entity or a representation of a database based object. The `UserView` class (View) is responsible for rendering the user's details. Lastly, the `UserController` class (Controller), manipulates the data from the User class and updates UserView when required.
Analysis of Model-View-Controller Examples
Crucially, in these examples, the Model, View, and Controller all have distinct and separate roles. They don't override each other's functions. This separation allows for better organisation in your application. Adding new functionality or modifying existing code becomes less troublesome as you can direct your efforts to a specific part of the system, mitigating the risks of disturbing other unrelated segments of the code. The Controller acts as an intermediary between the Model and the View. It receives user input from the View and manipulates the Model accordingly. Though it updates the View, it doesn't interact directly with it in real-time, avoiding unnecessary tight coupling and ensuring that the parts remain de-coupled as much as possible. This relegates the logic and presentation to their respective parts, increasing the clarity of the system. Conclusively, these examples exemplify a pragmatic approach to implementing the MVC pattern, each part maintaining its defined boundaries, resulting in an effective and efficient system.Case Study: Model-View-Controller application in popular software
Numerous software and frameworks effectively utilise the MVC pattern. One of the emblematic examples is Ruby on Rails, a server-side web application framework that is written in Ruby and follows the MVC structure. In Ruby on Rails, each request made by a client is handled by a Controller. The Controller interacts with the Model, which deals with database interactions, data validations, and business rules. The ability of Ruby on Rails Model to handle complex SQL queries simplifies database interactions. Finally, the information is handed back to the Controller, which then renders a proper response using a View. This wrapper of MVC architecture conveys a strong sense of direction and structure to web developers using Ruby on Rails. Additionally, it provides them with a broad set of tools and functionalities that fit well into the MVC pattern, thereby making development swift, scalable, and efficient. Analysing such popular software further reveals that MVC is not just a theoretical concept but a real-world application blue-print that not only enhances the development efficiency but also produces robust and secure applications compliant with modern standards.Model View Controller - Key takeaways
- The Model in Model View Controller (MVC) is responsible for managing application data, it interacts with the database and handles the necessary data processing.
- The MVC design pattern allows for the reduction of complexities in development through the separation of concerns into different sections. This separation promotes modularity, flexibility, and maintainability within the system.
- Through the use of MVC, each component of the system can be updated or modified independently without affecting the functioning of the others. This allows parallel development, improving efficiency and adaptability within the software development process.
- The MVC architecture arranges the system components in such a way that allows for clear lines of communication between them, resulting in an efficient flow of data. Each component only interacts with one or two other components within the system.
- Examples of MVC in real-world applications include various web applications in which the user request is processed by the Controller, the data is managed by the Model, and the appropriate data view is created for the user by the View.
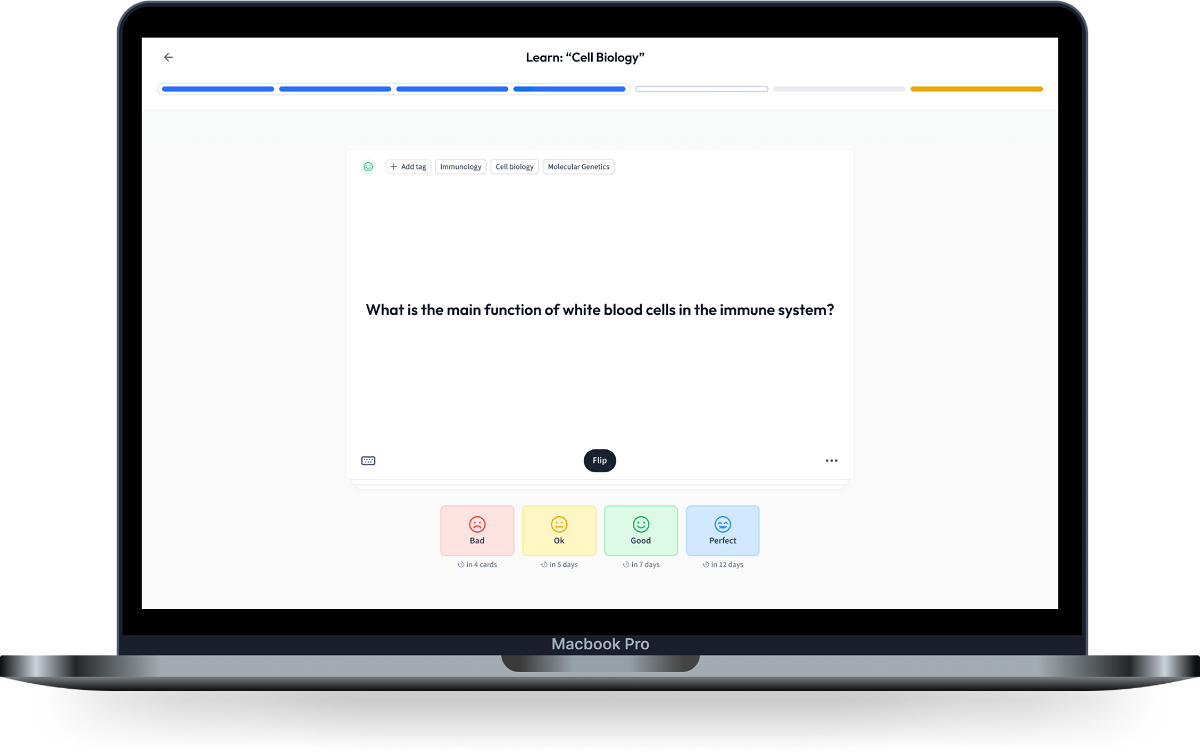
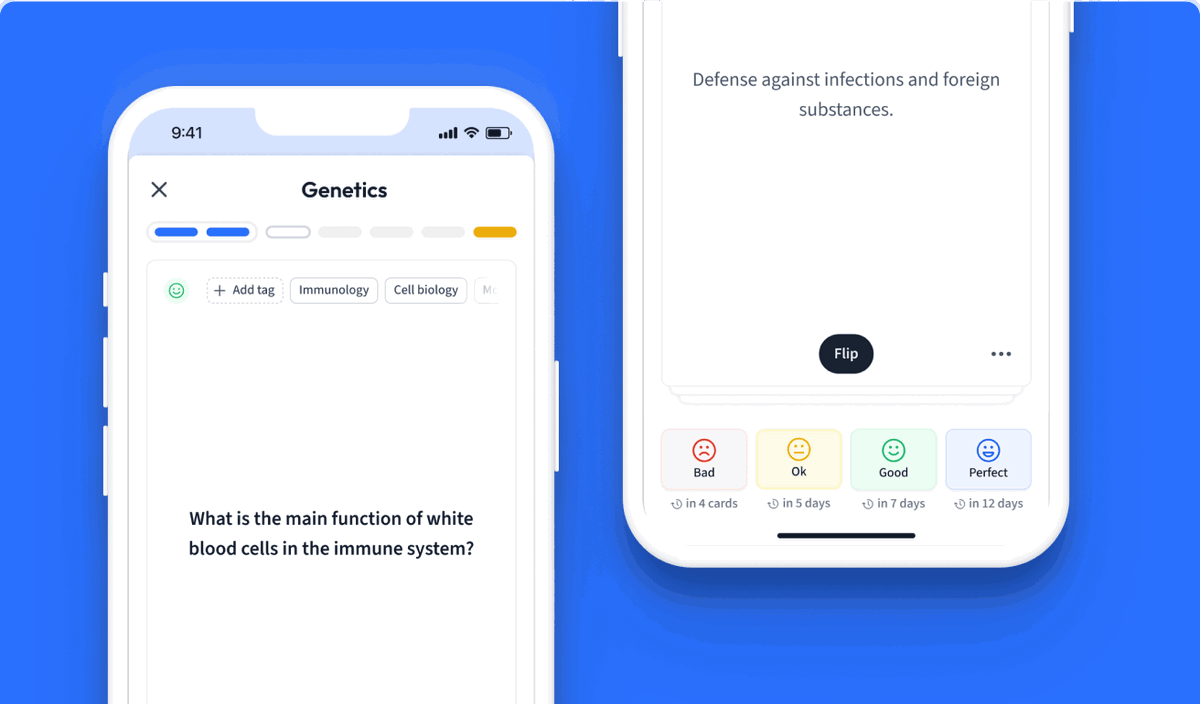
Learn with 12 Model View Controller flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Model View Controller
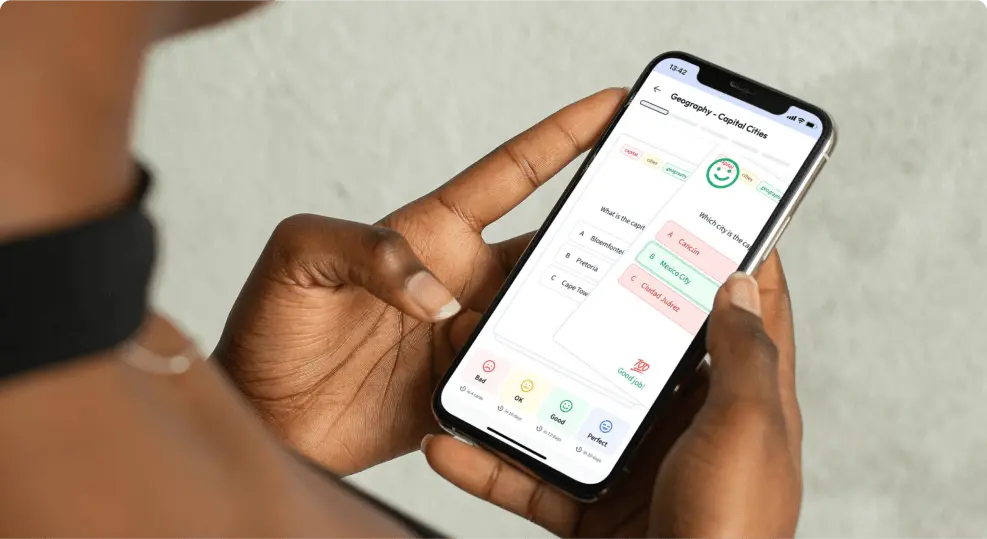
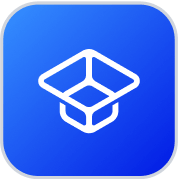
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more