Understanding Javascript Changing Elements
If you are aspiring to learn about computer science, then having a clear understanding of Javascript changing elements can unlock a new horizon of possibilities for you. Javascript is a high-level, interpreted programming language, often described as a language of the web. It's widely used for enhancing the interactivity of a website. One of the most important aspects of Javascript that you should comprehend is the ability to amend elements.
Basics of Javascript Changing Elements Definition
Javascript changing elements refers to the ability of Javascript to alter the content or attribute of HTML elements on a webpage. This process is performed by accessing the HTML elements and changing their properties using various Javascript methods.
Let's get into some important methods in Javascript that are used to modify the elements:
- getElementById: This method retrieves an element by its ID.
- getElementsByTagName: This method returns an array of elements with a specified tag name.
- getElementsByClassName: This method returns an array of elements with a specified class name.
For instance, imagine you have an HTML paragraph with an id "para1", and you want to change its content to "Hello World". First, you should access the element using its id. Here is how you do it in Javascript:
var para = document.getElementById("para1"); para.innerHTML = "Hello World";
The first line gets the element with the id "para1". The second line changes the content of that element to "Hello World".
Importance and Usage of Javascript Changing Elements in Computer Programming
Javascript changing elements holds immense significance in computer programming. Also, it empowers developers with the capability to create dynamic and interactive web pages.
Consider a website that displays the local time. The time displayed needs to be consistently updated every second to provide the correct time. This challenge can be effortlessly managed using Javascript changing elements. Javascript constantly accesses the HTML element displaying the time and updates it every second, thus displaying the correct local time.
Component | Role in Javascript Changing Elements |
The HTML element (to be changed) | Acts as the target that is going to be modified. |
Javascript | Identifies the target element, performs operations to determine new content, and manipulates the target element. |
Javascript changing elements function as the building blocks of many essential web technologies including but not limited to AJAX (Asynchronous JavaScript and XML) and JQuery, which rely hugely on the ability to manipulate HTML elements for various tasks, such as fetching data from a server and updating the data on a webpage without refreshing the entire page.
Consider an example where you have an HTML list containing several items. Using Javascript, you can easily add, modify, or remove items from this list without needing to refresh the entire webpage. This would look something like this:
// First, get the list by its id. var list = document.getElementById("myList"); // Create a new list item. var newItem = document.createElement("li"); newItem.innerHTML = "New Item"; // Add the new item to the list. list.appendChild(newItem);
The Javascript code above first accesses the list element, creates a new list item, and then adds this new item to the list.
Practical Application: Javascript Changing Elements Example
In practical scenarios, Javascript provides a highly efficient toolkit to manipulate and dynamically control the layout and style of Web elements. You're about to delve into some practical applications of Javascript changing elements.
How to Change Style of Element Javascript: Step-by-Step Guide
One common scenario when working with Javascript is changing the style of an HTML element. This can involve modifications to colour, font, size or virtually any aspect of CSS related to an object. To illustrate this, let's explore how to alter the style property of an element in a step-by-step manner.
- Firstly, you need an HTML element of which you want to modify the style. This element should have a unique id for easy targeting.
- Secondly, use Javascript's document.getElementById method to get the HTML element.
- Finally, use the style property to alter the look of the element. This can involve changing the colour, size, font, etc.
As an example, consider an HTML paragraph element with the id "text". Here's how you would modify its style:
// Access the HTML element var textElement = document.getElementById("text"); // Change style textElement.style.color = "blue"; textElement.style.fontSize = "20px";
The above code selects the HTML element with the id "text", and then changes its colour to blue and its font-size to 20 pixels.
How to Change DOM Element in Javascript: Detailed Explanation
Maintaining the momentum that you've built, let's now inspect another essential aspect of Javascript changing elements - manipulating the DOM (Document Object Model). The DOM represents the structure of HTML and XML documents and Javascript allows you to change these structures dynamically.
Changing a DOM element involves four major steps:
- Accessing the DOM element: Utilising methods like getElementById, getElementsByClassName, or getElementsByTagName to gain access to the target DOM element.
- Creating a new element: This involves using the document.createElement Javascript function to create a brand new element.
- Modifying the new element: With the help of properties like innerHTML or setAttribute, the created element can be customised.
- Replacing the old DOM element with the new: This is accomplished with the help of replaceChild function. It replaces the old DOM element with the newly generated and modified element.
For illustration, let's assume there's an old paragraph element with the id "oldElement", and you wish to replace it with a new paragraph that contains a different text:
// Access the old HTML element var oldElement = document.getElementById("oldElement"); // Create a new element var newElement = document.createElement("p"); // Modify the new element newElement.innerHTML = "This is the new element."; // Replace the old DOM element with the new oldElement.parentNode.replaceChild(newElement, oldElement);
In this code, the original paragraph ("oldElement") is replaced with the new paragraph ("This is the new element.").
Advanced Javascript Changing Elements Techniques
Once you've mastered the basics, it's time to delve deeper and uncover the more advanced techniques of Javascript changing elements. This section provides you with an expert-level train of thought on attribute manipulation, CSS modification, and element identification using id.
Change Attribute of Element Javascript: The Expert Approach
HTML elements often have attributes that provide additional information about the element. These attributes can be manipulated using Javascript, providing dynamic control over the element's behaviour and appearance.
When talking about an element attribute, it refers to additional properties of an HTML element that can be set to alter the element's default behaviour or appearance. For instance, an image element has a 'src' attribute which informs the browser where to locate and load the image from.
Changing an attribute in Javascript is a two-step process:
- You first need to access the HTML element using the previously discussed methods such as getElementById.
- Then, you use the setAttribute method to change the value of the attribute.
Suppose that you have an image with an id "myImage" and you want to change the 'src' attribute of this image. Here's how you should proceed:
// Access the HTML element var imgElement = document.getElementById("myImage"); // Change attribute imgElement.setAttribute("src", "newImage.jpg");
This script accesses the image through its id and then sets a new source for the image using setAttribute. As a result, a new image "newImage.jpg" will be displayed on the webpage.
Change CSS of Element Javascript: Advanced Guide
When it comes to polishing up web content and making it visually engaging, CSS (Cascading Style Sheets) plays an undeniably important role. But CSS is not just about static styling. With Javascript, CSS becomes a dynamic player that significantly enhances the user experience.
The CSS style of an element in Javascript can be manipulated using the style property. The 'style' property provides access to the inline style of an element and can be used to get as well as set CSS properties.
Here's how you get and set CSS styles:
- To get the value of a CSS property, use element.style.property.
- To set the value of a CSS property, use element.style.property = "value".
Imagine a situation where you have a text element and you want to change its text colour and the background colour. Here's how you could proceed:
// Access the HTML element var textElement = document.getElementById("myText"); // Change CSS style textElement.style.color = "white"; textElement.style.backgroundColor = "black";
This piece of code first gets the text element with the id "myText". It then changes the text colour to white and the background colour to black using the 'style' property.
Change Element by ID Javascript: A Comprehensive Tutorial
Getting an element by its ID is arguably one of the most basic yet most powerful ways to utilise Javascript to amend HTML elements. An ID is a unique identifier for an HTML element within a page, making it incredibly efficient to access and manipulate specific elements.
You are already familiar with the getElementById method, a prevalent and effective way to retrieve DOM elements by their unique IDs. This method allows precise targeting and manipulation of specific elements, providing a keen edge in creating sophisticated, dynamic, and interactive web pages.
For illustration, consider a scenario where you want to hide an HTML element with the id "myDiv". This could be achieved by changing the 'display' CSS property of the element to 'none', as shown in the following steps:
// Access the HTML element var divElement = document.getElementById("myDiv"); // Change CSS style to hide the element divElement.style.display = "none";
The above code collects the DIV element with id "myDiv" and alters its display style to "none", which effectively hides the element from the viewer.
Mastering the manipulation of HTML elements, whether it be changing attributes, modifying styles or working with IDs, is a vital skill in Javascript web development. It adds versatility to webpages and creates an engaging, interactive experience for users.
Working with Complex Elements in Javascript
As Javascript powers ahead in its quest to enhance web development, you're continually presented with more complex elements to manage. These elements often require expertise in handling, but as your JavaScript proficiency grows, so too does your capability to manipulate these elements effectively. Now, let's learn how to change the content of an element using JavaScript and delve into modifying elements in an array, a frequent occurrence in complex JavaScript programming.
Change Element Content Javascript: A Real-World Application
One of the many useful aspects of JavaScript involves dynamically changing or updating the content of HTML elements. This feature allows for a more interactive user experience, as web content can adapt and change based on user actions or changes in data. A common method to change an element's text content is by using the innerText property.
The innerText property in JavaScript represents the "rendered" text content of a node and its descendants. It essentially returns the visible text, excluding any HTML tags.
Let's explore the standard process used to change the content of an element using JavaScript:
- Identify the HTML element, preferably using its unique ID.
- Access the element using JavaScript's
document.getElementById
method. - Change the element content by assigning a new value to the
innerText
property of the targeted element.
Let's illustrate with an example where you'd want to change the content of a paragraph with id "demo". The original content might read "Hello World!" but you'd like to replace it with "Hello JavaScript!". Here's how you'd do so using JavaScript:
// Access the HTML element var paraElement = document.getElementById("demo"); // Change element content paraElement.innerText = "Hello JavaScript!";
This script selects the HTML element with the id "demo", and then replaces its original text "Hello World!" with "Hello JavaScript!"
Change Element in Array Javascript: A Deep Dive
Throughout your JavaScript journey, chances are that you'll frequently encounter arrays. An array is an ordered list of values that you refer to with a name and an index. Arrays in JavaScript are dynamic, meaning you can effortlessly add or remove elements, and you can change element values. Arrays provide the versatility of holding any data type, whether primitive or complex, including other arrays and objects.
An array in JavaScript is an object that allows you to store multiple values in a single variable. It can contain elements of any data type, including strings, numbers, booleans, objects and even other arrays, making it a versatile, complex data type.
Changing an element in a JavaScript array involves two basic steps:
- Access the desired element with the help of its index.
- Assign a new value to replace the old.
Let's suppose you have an array of cities, and you want to replace "London" with "Paris". Here's the JavaScript code to achieve this:
// The initial array var cities = ["New York", "London", "Tokyo"]; // Change array element cities[1] = "Paris";
The script creates an array named 'cities' with three elements "New York", "London", and "Tokyo". To replace "London" with "Paris", it targets the second element of the array (at index 1 since array indices start at 0), and assigns it the new value "Paris".
Working with complex elements in Javascript like changing the content of HTML elements or modifying elements in arrays provides you with a powerful ability to create dynamic, interactive websites. It's these essential skills that set apart proficient Javascript developers, enabling them to deliver a rewarding user experience. Always keep in mind that your Javascript skills are tools you're sharpening, and with each new line of code you write, you're getting better.
Tips, Tricks, and Best Practices for Javascript Changing Elements
Being proficient in Javascript changing elements isn't just about knowing the syntax or the basic ways to manipulate DOM elements. It's about understanding the nuances, anticipating potential pitfalls, and learning how to navigate around them to build reliable code. This section reveals several useful tips, tricks and best practices that seasoned Javascript developers adopt while working with changing elements.
Troubleshooting and Using Javascript Changing Elements Effectively
At times, despite careful planning and coding, you won't see the desired changes reflecting on the webpage. Don't fret! Debugging is an integral part of programming, and Javascript is no exception.
A recurring issue in Javascript changing elements typically unfolds as follows: you'd written your JavaScript code to change an HTML element, linked your script file correctly, but still observe no difference in the webpage. What could be wrong?
One of the primary reasons for such a non-responsive behaviour is the timing of script execution. When a browser processes an HTML document, it runs from top to bottom. If your Javascript code attempts to access and change an element before it's been loaded by the browser, the script will fail as it can't find the targeted element.
To prevent such issues, consider the following tips:
- Place your script tags just before the closing
>/body<
tag. By doing so, you're ensuring that the browser has loaded all the HTML elements before it begins processing your JavaScript code. - Alternatively, use the
window.onload
event that fires after the entire content of the web page has been loaded. This way, you can guarantee that the browser has knowledge of all the elements present in your HTML document.
Here's a demonstration of how you might use the window.onload event:
window.onload = function() { var elem = document.getElementById("myElement"); elem.innerText = "New Content"; };
This script uses the window.onload event to ensure that all HTML elements are loaded before the JavaScript code inside the function starts executing, thereby successfully altering the text of the element with id "myElement" to "New Content".
Besides script timing, other common issues involve errors in accessing elements. It's slick to remember that method names and element IDs are case-sensitive. Always double-check your code for any typos or wrong case usage.
Navigating Common Challenges in Javascript Changing Elements Execution
An understanding of potential roadblocks you might encounter while executing Javascript changing elements is crucial for effective web development. It will not only help you debug faster but also write sturdier code that's less likely to break.
Different browsers often present unique challenges in Javascript execution. This behaviour is a result of varying levels of adherence to web standards across browsers and browser versions. Such disparities may cause your Javascript changing elements code to work in one browser but fail in another.
How then can you as a developer ensure the smooth functioning of your code across all browsers?
One highly advocated practice in the Javascript community is to write 'Defensive Code'. This approach entails anticipating potential problems and implementing solutions upfront. It requires you to consider a range of scenarios and meticulously handle them to avoid errors during code execution.
To illustrate, consider an example where you attempt to change style properties of an HTML element. Without defensive programming, your code might look like the following:
var elem = document.getElementById("myElement"); elem.style.color = "blue";
If there's no element with id "myElement", this code will throw an error. To avoid such situations, a defensive coding approach would be to first check if the element exists, and then proceed with style changes:
var elem = document.getElementById("myElement"); if (elem){ // check if element exists elem.style.color = "blue"; }
This revised code prevents errors from occurring in the event of a missing element on the page.
Another common challenge in Javascript changing elements execution is dealing with differences in attribute handling across browsers. To tackle this, always refer to reliable resources like Mozilla Developer Network (MDN) to understand cross-browser support for various methods.
Your knowledge and ability to navigate these challenges is what truly showcases your expertise in Javascript changing elements. Always remember, proficiency in coding is not just about getting things done but also making them work in diverse, sometimes unpredictable, environments.
Javascript Changing Elements - Key takeaways
- Javascript changing elements is the manipulation of HTML elements using Javascript, allowing dynamic control over a webpage's layout and style.
- To change the style of an element in Javascript, first get the HTML element by its id using `document.getElementById`, then use the `style` property to modify the element's style features (e.g. color, size).
- Changing a DOM element requires accessing the target element, creating a new element using `document.createElement`, modifying the new element, and replacing the old element with the new one using `replaceChild`.
- Attributes of an HTML element can be modified in Javascript using the `setAttribute` method after accessing the element by its id.
- The CSS style of an element can be modified in Javascript using the `style` property once the element is accessed by its id.
- Change the content of an element by accessing the element with `getElementById` then alter the `innerText` property to the desired text content.
- In Javascript, elements of an array can be changed by accessing the element with its index and assigning a new value to it.
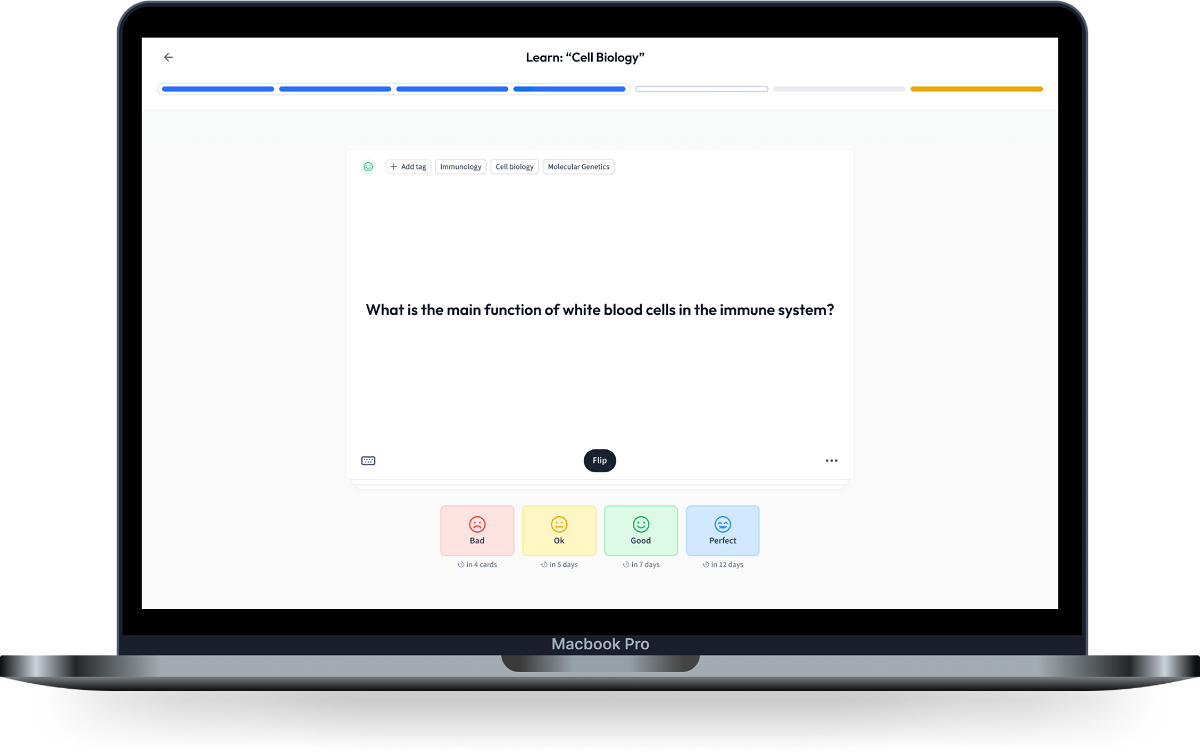
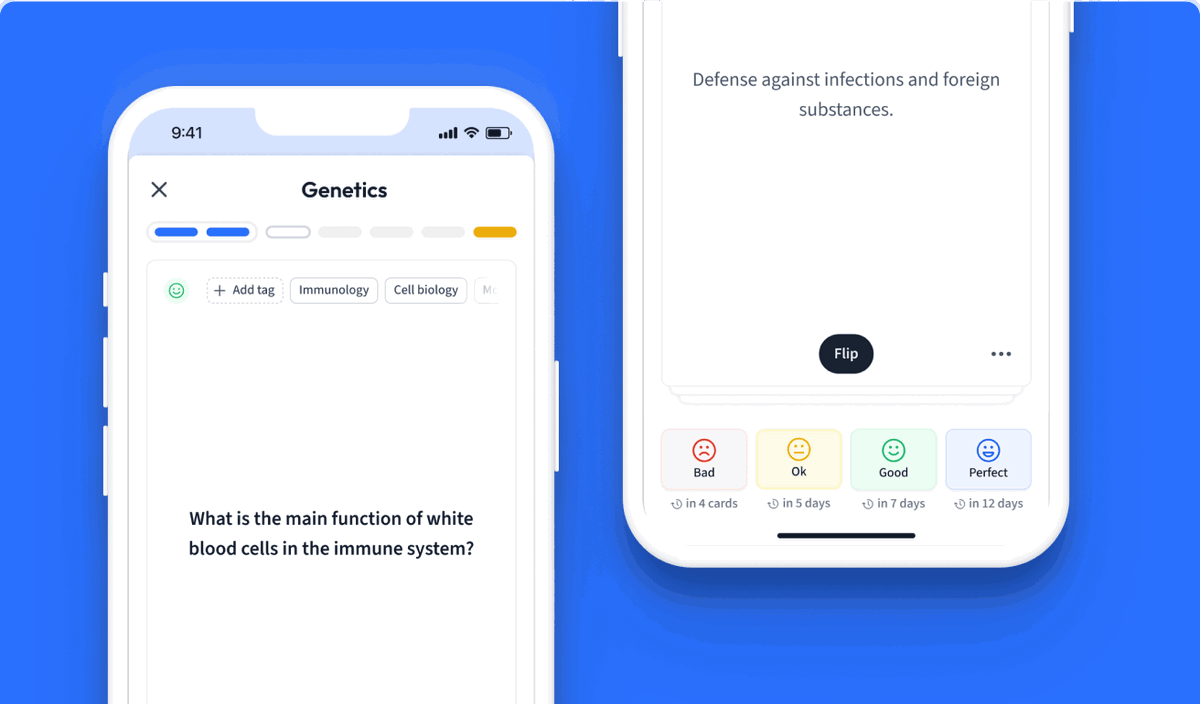
Learn with 15 Javascript Changing Elements flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript Changing Elements
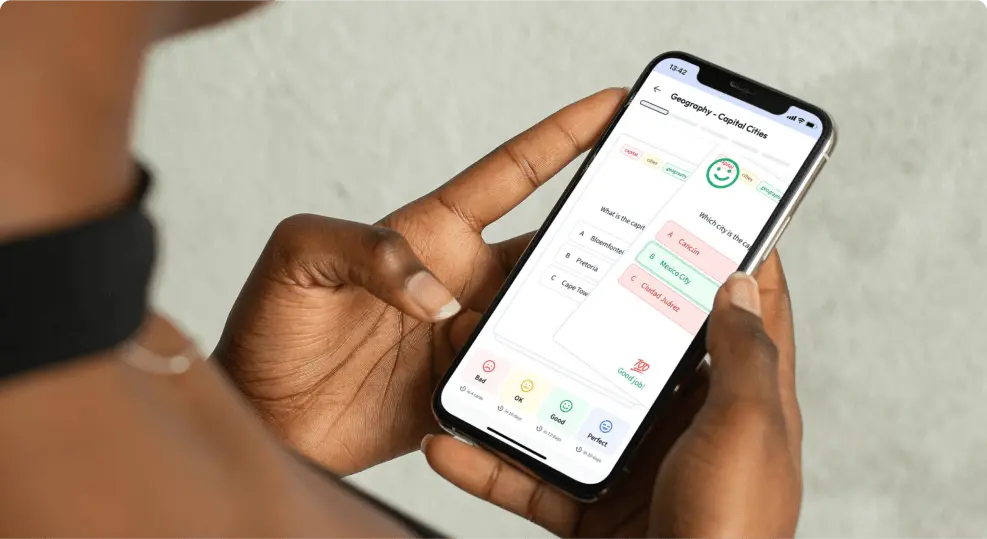
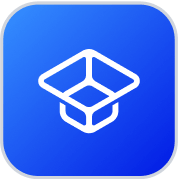
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more