Understanding Java If Else Statements
In the world of computer programming, especially when you're dabbling within the realm of Java programming, If Else Statements serve a crucial purpose. These structures are a keystone of conditional control in Java, allowing your code to make dynamic decisions based on various conditions.Basic Introduction to Java If Else Statements
Java's If-Else Statements are a form of control structures that manage the flow of execution in a program based on specified conditions. These conditions are determined by logical or comparison operations.Control Structures: These are programming block constructs that manage the execution direction of a code such as conditions and loops.
if (condition) { // Executes this block if condition is true } else { // Executes this block if condition is false }In the above example, 'condition' is a boolean expression which the If statement checks. If the condition holds true, the code within the if-block gets executed. Conversely, if the condition is false, the code within the else-block is executed. Now, let's break down a real Java If-Else Statement:
int score = 85; if (score >= 90) { System.out.println("Excellent"); } else { System.out.println("Good"); }In this case, since the score is less than 90, the code within the 'else' block is executed, and the word "Good" is printed.
Purpose and Use of Java If Else Statements in Programming
Java If Else Statements hold significant importance in programming.They often resolve real-world scenarios where certain actions are based on specific conditions. From checking user input validity, ensuring secure logins, to making decisions in game mechanics, If Else Statements are scintillatingly ubiquitous!
- Decision making based on conditions
- Creating complex algorithms and business logic
- Enhancing code maintainability and readability
- Data validation
int score = 80; if (score >= 90) { System.out.println("Excellent"); } else if (score >= 80) { System.out.println("Very Good"); } else { System.out.println("Good"); }In the above example, the condition 'score >= 90' is false, so the program jumps to the next condition 'score >= 80'. Since this condition is true, the program outputs "Very Good" and does not process further conditions. Such utilisation of Java If Else Statements makes your code powerful and flexible to handle complex logical scenarios.
Delving Into If Else Statement Example Java
Java's If Else Statements help establish control flow in programs, enabling different actions to take place based on varying conditions. By constructing conditional statements, programs become significantly more dynamic and intelligent.Simplifying Java If Else Statement with Real-Life Examples
To truly comprehend the power of If Else Statements in Java, it's always beneficial to connect it to everyday activities. The goal here is to stipulate that If Else Statements are everywhere in the surrounding world. Ever noticed how a door works? It's an amazing portrayal of a simple If Else Statement:if (door is opened) { Walk through it; } else { Open door; }This logic from our daily routines can be coded in Java as:
boolean doorIsOpen = true; if (doorIsOpen) { System.out.println("Walk through it"); } else { System.out.println("Open door"); }Relating this to a more advanced instance, consider an ATM. How does it verify a pin is correct? A basic interpretation in Java could be:
int enteredPIN = 1234; int correctPIN = 4567; if (enteredPIN == correctPIN) { System.out.println("Access Granted"); } else { System.out.println("Access Denied"); }In these examples, it can be observed how varied the usage of If Else Statements can be in Java and real life, emphasizing the flexibility and power of this vital control structure.
Applying If Else Statement Technique in Java: Case Studies
Let's consider the case of an e-commerce website. A common feature is offering discounts to customers who register for the first time. How might Java If Else Statements be used to implement this? Review the example below:boolean isFirstPurchase = true; if (isFirstPurchase) { System.out.println("Apply 10% discount"); } else { System.out.println("No discount"); }The software checks if it's the customer's first purchase. If the condition is true, it applies a 10% discount on the total price. Otherwise, there's no discount. Focus on another practical scenario: A temperature control system for a building. If it's too cold, the heating should be turned on. If it's too hot, the air conditioning should be turned on. Let's see how this is implemented:
int temperature = 22; int lowerLimit = 20; int upperLimit = 25; if (temperature < lowerLimit) { System.out.println("Turn on heating"); } else if (temperature > upperLimit) { System.out.println("Turn on air conditioning"); } else { System.out.println("Maintain current temperature"); }Here, a nested If-Else Statement is used for various conditions. It checks the current temperature and makes decisions accordingly.
Suppose the temperature is 18 degrees. The statement \( temperature < 20 \) becomes true, thus it "Turns on heating". However, if the temperature is 26 degrees, the statement \( temperature > 25 \) becomes true, leading it to "Turn on air conditioning". Finally, if the temperature is between 20-25 degrees, the system states "Maintain the current temperature".
Discovering Nested If Else Statement in Java
Peel back the numerous layers of Java programming to uncover another intricate construct - the Nested If Else Statement. A 'Nested' If Else statement is one where you find an If Else construction inside another If Else construction, akin to Russian Matryoshka dolls. Nesting them adds greater flexibility to decision making and lets programmers handle intricate logical scenarios.What Is a Nested If Else Statement in Java?
In Java, an If Else Statement can be inserted within another - hence the term 'nested'. This nesting can go as deep as required, opening doors for handling combinations of several conditions.A Nested If Else Statement is an If Else Statement with another If Else Statement positioned inside either the 'If' or 'Else' code block or potentially both.
if (condition1) { // Executes this block if condition1 is true if (condition2) { // Executes this block if condition1 and condition2 are both true } } else { // Executes this block if condition1 is false }In this example, 'condition1' is first checked. If true, it progresses to another If Statement where it then checks for 'condition2'. It's only when 'condition1' and 'condition2' are both true that the code within the nested If Statement gets executed. If 'condition1' is false, the program immediately jumps to the else-block, bypassing the nested If Statement entirely. This ability to evaluate multiple conditions sequentially is particularly useful when coding complex conditions and exceptions. Indeed, mastering such branches of logic is an important part of becoming a proficient programmer.
Practical Examples of Nested If Else Statements in Java
Let's ground ourselves into practicality with a real-life instance of Using Nested If Else Statements: The grading system of an examination.int marks = 88; if (marks >= 75) { if (marks >= 85) { System.out.println("Grade A"); } else { System.out.println("Grade B"); } } else { System.out.println("Grade C"); }In this case, the outer If Statement checks if marks are greater than or equal to 75. If true, it progresses to the nested If Statement where it checks if marks exceed or equal 85. If true once again, the program prints "Grade A". If false, it executes the nested else-block, printing "Grade B". However, if marks do not exceed or equal 75, it directly jumps to the outer else-block and prints "Grade C". To delve deeper into complex scenarios, let's consider a system that checks age and driving experience to issue driving licenses. This situation incorporates multiple nested If Else Statements.
int age = 20; int drivingExperience = 1; if (age >= 18) { if (drivingExperience >= 2) { System.out.println("License Issued"); } else { System.out.println("Experience requirement not met"); } } else { System.out.println("Age requirement not met"); }In this example, the program first checks if the applicant's age is greater than or equal to 18. If true, it then checks if the driving experience equals or exceeds 2 years. In case age is less than 18, it jumps directly to the outer else-block, stating "Age requirement not met". If experience does not reach 2 years, it states "Experience requirement not met". Only when both conditions are true does it print "License Issued".
In the provided example, the applicant's age is 20 years, which meets the age requirement as \( age \geq 18 \). However, the driving experience of 1 year does not meet the experience requirement, as \( drivingExperience < 2 \). Therefore, the program outputs "Experience requirement not met".
Structure of Java If Else Statements
Let's dissect Java's If Else Statements, capturing the essence of their structural architecture, and understand the best practices for implementing an effective structure in our programs.Unveiling the Anatomical Structure of Java If Else Statements
Diving into the anatomy, Java If Else Statements articulate a logical progression of conditions and outcomes in a program. If Else statements allow programmers to guide their program's actions based on specific conditions. A basic structure of an If Else statement is:if (condition) { // block of code to be executed if the condition is true } else { // block of code to be executed if the condition is false }In this construct:
• if : This keyword instigates the If Else construct. It determines whether a certain condition is true or false. The condition check follows this keyword within parentheses.
• (condition) : This is a Boolean expression(i.e., an expression that can be either true or false). If the condition evaluates true, the code block within the If statement gets executed. Otherwise, control is passed to the Else block.
• {...} : This is the code block, within which, the action or program segment that needs to run when the specified condition is true or false is placed.
• else : This keyword operates when the preceding If condition is false. It indicates the alternative segment of code that should run if the condition in the If statement does not hold.
if (condition1) { // block of code executed if condition1 is true } else if (condition2) { // block of code executed if condition1 is false and condition2 is true } else { // block of code executed if both condition1 and condition2 are false }Here, the 'Else If' statement adds another stair to the conditional ladder, introducing a second condition that only gets checked if the first condition is false.
Best Practices for Creating an Effective Java If Else Statement Structure
While understanding the structural templates is essential, it's equally critical to appreciate how to leverage this knowledge effectively and understand best practices for coding Java If Else Statements. Here are some points to consider:• Keep conditions simple: Avoid overly complex conditions, as they may become error-prone. If a condition is turning convoluted, try breaking it down using logical operators.
//Fit over convoluted condition if (age > 18 || age < 65){ // block of code to be executed } //Better Practice boolean isAdult = age > 18; boolean isSenior = age < 65; if (isAdult || isSenior) { // block of code to be executed }This technique enhances readability and maintainability by using Boolean variables to represent complex conditions. It's a good practice to name these variables semantically for easy comprehension.
• Refrain from Negation: Try to keep conditions positive rather than negative, as they can be more challenging to understand.
// Negated condition if (!isImportant){ // block of code to be executed } //Better Practice if (isNotImportant) { // block of code to be executed }Here, turning the negated condition 'isImportant' into 'isNotImportant' avoids confusion and increases code legibility.
• Account for all outcomes: Ensure every potential path of the program has been addressed adequately. All possible outcomes should be covered within the If-Else structure.
// The Last Else acts as a catch-all for any conditions not addressed if (grade >= 65) { System.out.println("Passed"); } else { System.out.println("Failed"); }By following these best practices whilst constructing the Java If Else Statement structures, programmers can ensure efficient functionality, excellent readability, and an error-free, optimised code.
Mastering If Else Statement Techniques in Java
Delving deeper into the realms of Java programming, special attention falls upon the If Else statement - a defining element in the decision-making prowess of a program. Mastery of the If Else technique is like being equipped with a powerful tool for controlling your program's flow, as these structures provide critical paths that accomplish different tasks depending on specific conditions.Why If Else Statement Technique in Java is Important
If Else statements lie at the intersection of conditional logic and efficient control flow, making them vital for virtually every Java program written. These conditional statements serve as essential conduits through which control is passed, based on whether a specific condition is met. They underpin the decision-making capability of a program, allowing actions to be made in response to prevailing conditions. This is critical because many real-world scenarios cannot be simulated without branching possibilities based on different factors. For instance, a weather application that suggests activities based on the weather will need an If Else structure to decide what to suggest depending on whether it's sunny, raining, snowing, or anything else. Furthermore, nested If Else Statements contribute a dynamic perspective to a program's control structure, offering a neat way to handle numerous conditions and exceptions. In essence, mastering If Else Statement techniques provides the ability to construct robust, intelligent applications that emulate the complexity and sensitivity of real-world decision-making processes, making them a cornerstone of any serious programming.Strategies to Perfect If Else Statement Technique in Java
Java If Else statements, while merely imposing a seemingly simple conditional check, can grow complex with nested structures and multiple conditions. Here are some strategies to effectively wield this powerful tool:Embrace simplicity: Despite the ability to handle intricate conditions by nesting, keeping conditions simple aids comprehensibility and decreases the chance of errors. Practice clear, concise coding by sketching out the logic before diving into code, and avoid unnecessary nesting when a logical operator can do the same job.
// Version 1 - Nested If Statement if (x > 10) { if (y > 10) { System.out.println("Both x and y are greater than 10."); } } // Version 2 - Logical Operator if (x > 10 && y > 10) { System.out.println("Both x and y are greater than 10."); }
Use descriptive variable names: It's tempting to dismiss the importance of variable names in the grand scheme, but they play a vital role in code readability. Descriptive names make the code easy to understand and maintain, reducing the mental effort required to discern a variable's purpose in a sea of code.
// Version 1 if (a > b) { ... } // Version 2 if (temperature > freezingPoint) { ... }
Utilize Code Reviews: Even experienced software engineers appreciate the benefit of an extra pair of eyes on their code. Code reviews highlight potential bottlenecks, style discrepancies, potential failures, and places where better practices or approaches could be utilised. Regularly incorporating code reviews into your practice routine fosters learning, reinforces good habits, and helps to perfect your If Else Statement technique in Java.
Java If Else Statements - Key takeaways
- Java If Else Statements is a control flow statement that enables different actions to be performed based on different conditions.
- If Else Statements in Java can be closely related to everyday decision-making activities, amplifying their application in real-life programming situations.
- Nested If Else Statement in Java is an If Else Statement within another If Else Statement, this is useful for handling complex conditions and increases decision making flexibility.
- Structure of Java If Else Statements is crucial for efficient programming, it includes the 'If' keyword that instigates the If-Else construct, the 'condition' that tests whether an expression is true or false, and the 'else' keyword that indicates alternative actions when the preceding 'If' condition is false.
- Mastery of If Else statement techniques in Java form a fundamental component for decision-making in programming and aids in efficient control flow within the program.
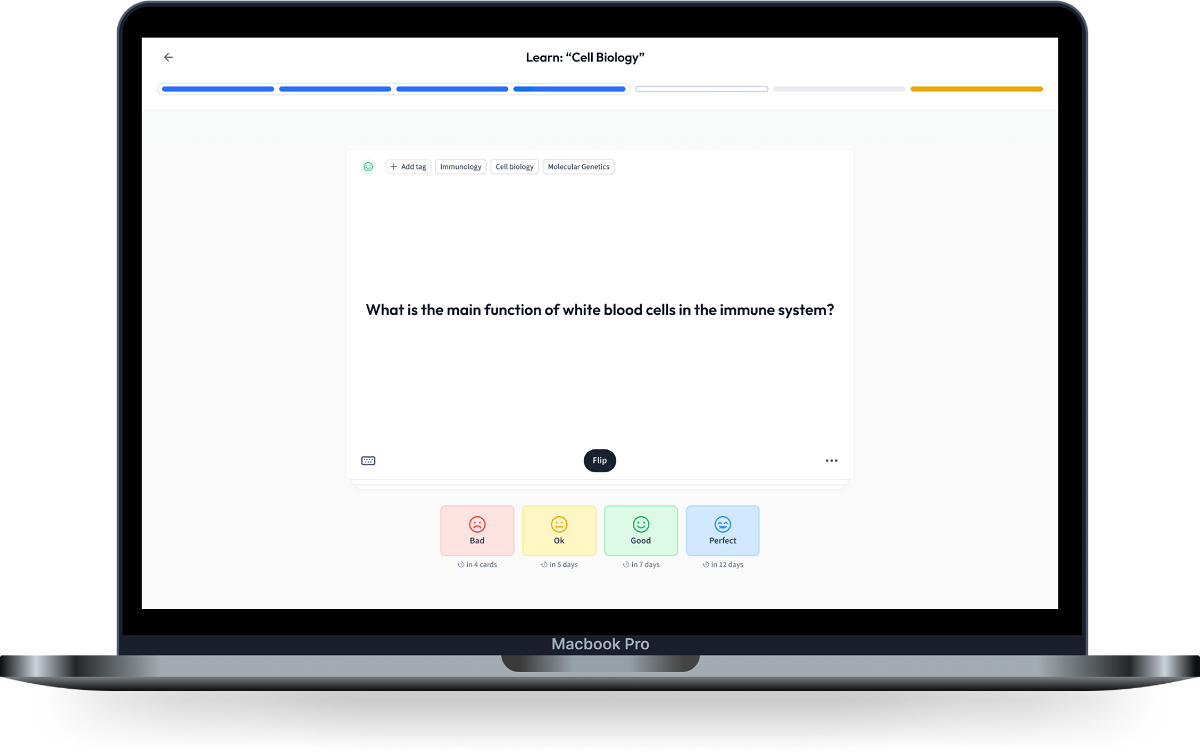
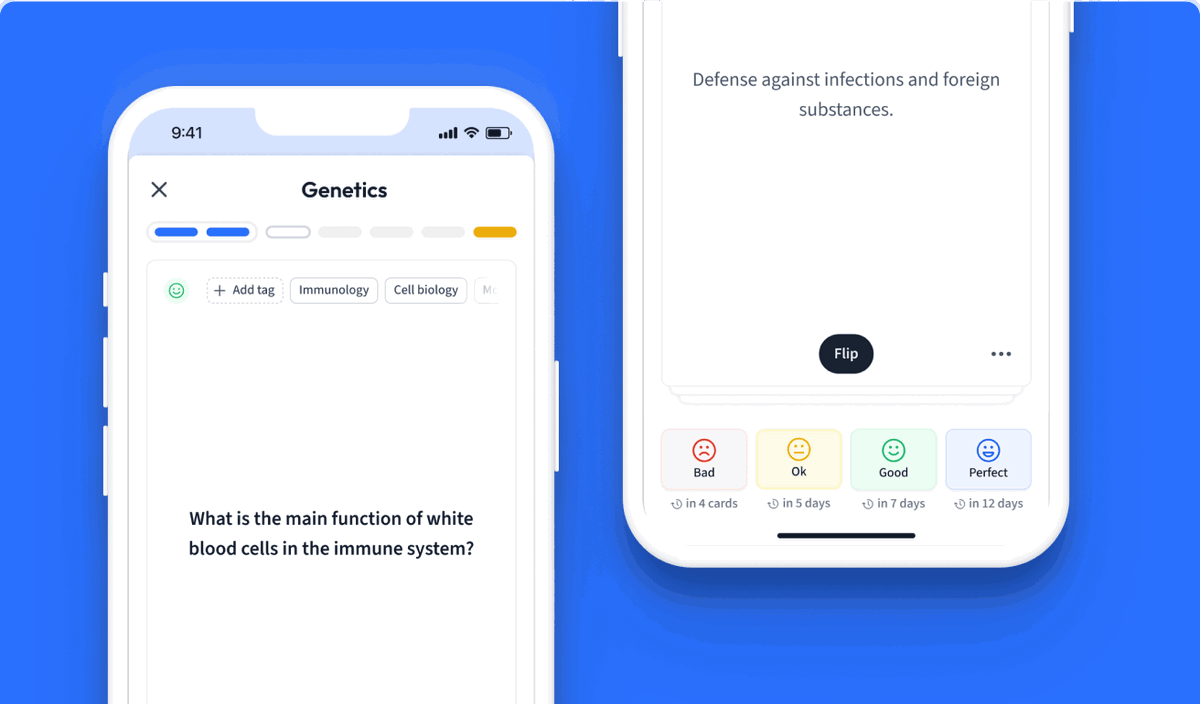
Learn with 15 Java If Else Statements flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Java If Else Statements
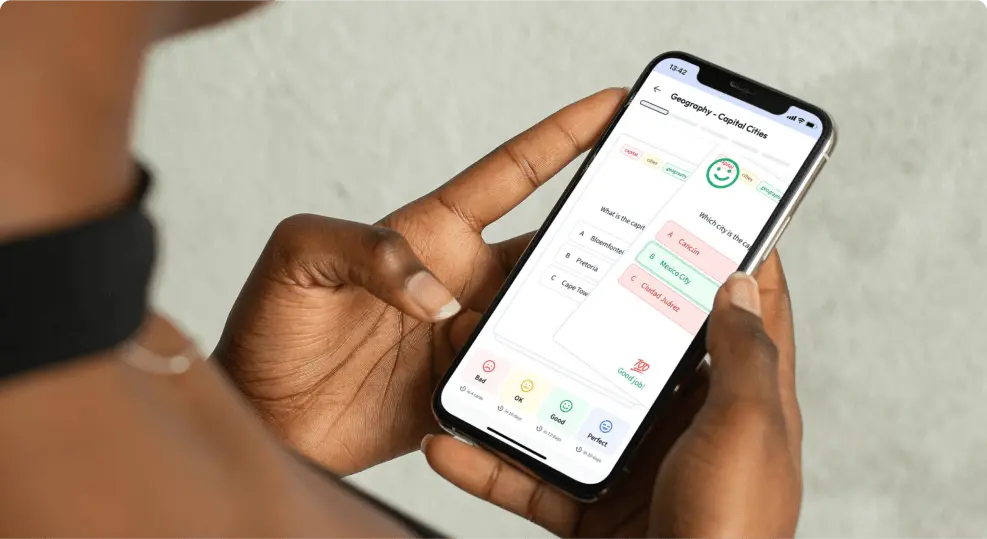
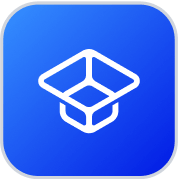
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more