What Are Javascript Object Prototypes?
Javascript object prototypes are a fundamental concept in the Javascript programming language, enabling objects to inherit properties and methods from other objects. As you delve into the world of Javascript, understanding prototypes is crucial for enhancing your coding skills and building more efficient applications.
Understanding the Basics of Javascript Object Prototypes
At the heart of Javascript, every object comes with a built-in property called prototype. This built-in property, a prototype, is essentially a reference to another object that contains attributes and methods that can be shared or 'inherited' by other objects. This relationship forms a chain, known as the prototype chain, allowing objects to access properties and methods of other objects.To understand it better, imagine Javascript object prototypes as templates from which other objects can inherit properties and methods. This mechanism is particularly useful when you want multiple objects to share the same functionality, reducing redundancy and promoting code reusability.
function Vehicle(make, model) { this.make = make; this.model = model; } Vehicle.prototype.displayInfo = function() { return this.make + ' ' + this.model; }; var car = new Vehicle('Toyota', 'Camry'); console.log(car.displayInfo()); // Outputs: Toyota Camry
The prototype property is used primarily for defining methods that should be shared by all instances of a function.
Key Characteristics of Javascript Object Prototypes
Javascript object prototypes boast several key characteristics that set them apart and make them an essential concept for any Javascript developer to grasp. Here's a look at some of these core characteristics:
- Inheritance: Prototypes serve as a means for one object to inherit properties and methods from another object, facilitating a hierarchical relationship among objects.
- Prototype Chain: Objects are linked together through prototypes, forming a chain that allows for property and method lookup along the prototype hierarchy.
- Flexibility: Prototypes provide a flexible way to extend object functionality by adding or modifying properties and methods at runtime.
- Efficiency: Through prototype inheritance, objects can share methods, significantly reducing memory usage by not duplicating methods for each instance.
One of the truly fascinating aspects of Javascript object prototypes is their dynamic nature. Unlike some other object-oriented languages where inheritance is fixed at compile-time, Javascript allows for dynamic modification of prototypes, which means you can add or change properties and methods on the prototype even after objects have been created. This degree of flexibility is a double-edged sword; it provides powerful capabilities for dynamic adaptability but also requires careful management to prevent unintended side effects. Embracing and mastering this dynamic aspect of Javascript will empower you to manipulate object behaviour in powerful ways, tailoring it to your specific needs at runtime.
Javascript Object Prototype Methods and Functions
In the realm of Javascript, understanding object prototype methods and functions is not just beneficial; it's crucial for mastering the language. These methods and functions provide a pathway for objects to inherit features from one another, promoting code reusability and efficiency.
How Javascript Object Prototype Functions Work
Javascript object prototype functions offer a unique approach to object-oriented programming, enabling objects to 'inherit' properties and methods through the prototype chain. This hierarchy comprises a series of linked prototypes, where each object can access the properties and functions of its prototype unless those properties or functions are overridden.Let's dive into the mechanics: when you attempt to access a property or method on a Javascript object, the engine first searches the object itself. If it's not found, the search moves up to the object's prototype, and this process continues up the prototype chain until the desired property or method is found or the chain ends.
function Animal(name) { this.name = name; } Animal.prototype.speak = function() { console.log(this.name + ' makes a sound.'); } var dog = new Animal('Rex'); dog.speak(); // Outputs: Rex makes a sound.
The term 'prototype' might be misleading; it serves not as a draft or a preliminary model but as a full-fledged, accessible object from which other objects can directly inherit.
Essential Methods of Javascript Object Prototypes
Object.create: This method creates a new object, using an existing object as the newly created object's prototype.
Various methods are instrumental in manipulating and leveraging prototypes in Javascript to manage and extend object functionalities. Key methods you should be familiar with include:
- Object.create(proto) - Creates a new object with the specified prototype object and properties.
- Object.getPrototypeOf(obj) - Returns the prototype (i.e., the value of the internal [[Prototype]] property) of the specified object.
- Object.setPrototypeOf(obj, prototype) - Sets a specific object's prototype (i.e., its internal [[Prototype]] property) to another object or null.
var animal = { type: 'mammal', displayType: function() { console.log(this.type); } }; // Creating a new animal type var cat = Object.create(animal); cat.type = 'cat'; cat.displayType(); // Outputs: cat
The power of Object.setPrototypeOf and Object.create lies in their ability to dynamically alter the prototype chain. This capability enables developers to implement complex inheritance structures that can be modified at runtime, providing tremendous flexibility in how objects interact and behave. For instance, changing the prototype of an object can instantly give it access to a different set of properties and methods, potentially transforming its functionality completely. However, it's essential to use these features judiciously, as altering the prototype chain can have performance implications and may introduce hard-to-track bugs in larger applications.
How to Get Prototype of An Object in Javascript
Understanding how to get the prototype of an object in Javascript is a crucial skill for many programming tasks. It allows developers to dive into the inheritance structure of their objects, providing insights on object behaviour and shared methods.Whether you're debugging or extending existing code, knowing how to access an object's prototype chain can be incredibly beneficial.
Steps to Accessing Prototypes in Javascript
Accessing the prototype of a Javascript object is straightforward, involving built-in methods designed specifically for this purpose. Following these steps ensures you can efficiently navigate and utilise the prototype chain:
- Use the Object.getPrototypeOf method to directly access the prototype of a specified object.
- Utilise the proto property (__proto__), an informal but widely supported way to access an object's prototype directly.
const animal = { type: 'mammal' }; const dog = Object.create(animal); console.log(Object.getPrototypeOf(dog) === animal); // Output: true
Remember that the __proto__ property is a historical legacy and might not be supported in all environments. It's advisable to use Object.getPrototypeOf for consistency.
Practical Examples of Retrieving Javascript Object Prototypes
Putting theoretical knowledge into practice aids in comprehending how to work with prototypes in Javascript. Below are practical examples that highlight how to retrieve the prototype of an object and the benefits of understanding this.Example 1: Accessing the Prototype to Understand Inheritance
function Car(make, model) { this.make = make; this.model = model; } Car.prototype.displayMake = function() { console.log(this.make); }; const myCar = new Car('Toyota', 'Supra'); console.log(Object.getPrototypeOf(myCar).displayMake); // function() {...}Example 2: Modifying Prototypes at Runtime
const bird = { fly: true }; const penguin = Object.create(bird); penguin.fly = false; console.log(Object.getPrototypeOf(penguin).fly); // true
Understanding and manipulating the prototype chain unlocks advanced functionality and dynamic behaviour in Javascript objects. For instance, by retrieving and modifying objects' prototypes, it's possible to implement a rich inheritance scheme without the overhead of class-based languages. This prototype-based inheritance is flexible and powerful, allowing for the creation of complex object hierarchies with minimal boilerplate code. The ability to inspect and manipulate prototypes at runtime not only aids in debugging but also encourages a more profound understanding of Javascript's object-oriented features, revealing the language's true dynamism and flexibility.
Difference Between Javascript Objects and Prototypes
In the world of Javascript, the distinction between objects and prototypes is vital for understanding the language's object-oriented capabilities. While both serve as foundational elements in Javascript programming, they cater to different aspects of the language's structure and functionality.Through this exploration, you'll gain clarity on how Javascript objects and prototypes differ in their usage, properties, and roles within the Javascript ecosystem. This knowledge is instrumental in leveraging Javascript's full potential in developing dynamic, efficient web applications.
Comparing Javascript Objects and Their Prototypes
Javascript objects and prototypes, though closely intertwined, serve distinct purposes. Objects are key constructs that hold data and functionality, encapsulating related state and behaviour. In contrast, prototypes are essentially templates that provide a mechanism for objects to inherit properties and methods.A deeper dive into their differences reveals:
- Objects are instances created from classes or constructor functions, containing unique data and methods.
- Prototypes are the mechanism through which Javascript objects inherit features from one another, residing in the prototype chain.
function Smartphone(brand, model) { this.brand = brand; this.model = model; } Smartphone.prototype.displayInfo = function() { console.log('Brand: ' + this.brand + ', Model: ' + this.model); }; var myPhone = new Smartphone('Apple', 'iPhone 13'); myPhone.displayInfo(); // Outputs: Brand: Apple, Model: iPhone 13
While objects can have their properties and methods, prototypes allow for the definition of methods that can be shared across all instances of an object, improving memory efficiency.
Javascript Objects and Prototypes Application in Real-World Scenarios
The practical applications of understanding and utilising Javascript objects and their prototypes are vast and varied across different real-world scenarios. From enhancing code reusability to streamlining the development process, the benefits are tangible.For instance, in web development, prototypes are used to extend native Javascript objects like Arrays and Strings with custom functions. This can greatly enhance functionality without affecting other instances. Additionally, objects serve as the backbone for most interactive web applications, storing user data and controlling the application logic.
// Extending the Array prototype Array.prototype.customFeature = function() { return 'This is a custom feature'; }; var arr = []; console.log(arr.customFeature()); // Outputs: This is a custom feature
One intriguing application involves leveraging prototypes for polymorphism in Javascript. Through prototype chaining, objects can inherit methods from multiple prototypes, enabling dynamic behaviour based on the object's state or type. This aspect of prototypes introduces a powerful, flexible way to manage object inheritance, mirroring concepts found in class-based programming languages. It highlights the adaptability and depth of Javascript's object-oriented features, offering developers extensive control over object behaviour and interactions.
Javascript Object Prototypes - Key takeaways
- Javascript Object Prototypes: Prototypes in Javascript allow objects to inherit properties and methods from other objects; they are a core part of the language's object-oriented capabilities.
- Prototype Chain: This is a series of linked prototypes, forming a hierarchy for property and method lookup, which substantially reduces redundancy in code and improves efficiency.
- How to Get Prototype of an Object: Use Object.getPrototypeOf(obj) to access an object's prototype, being mindful that the informal __proto__ property is not recommended for use in modern code due to compatibility concerns.
- Javascript Object Prototype Methods: Object.create(proto) creates a new object with the specified prototype; Object.getPrototypeOf(obj) retrieves an object's prototype; Object.setPrototypeOf(obj, prototype) changes an object's prototype.
- Difference Between Objects and Prototypes: Objects are instances with unique data, while prototypes serve as a mechanism for objects to inherit features, illustrating a key concept in understanding Javascript's object-oriented approach.
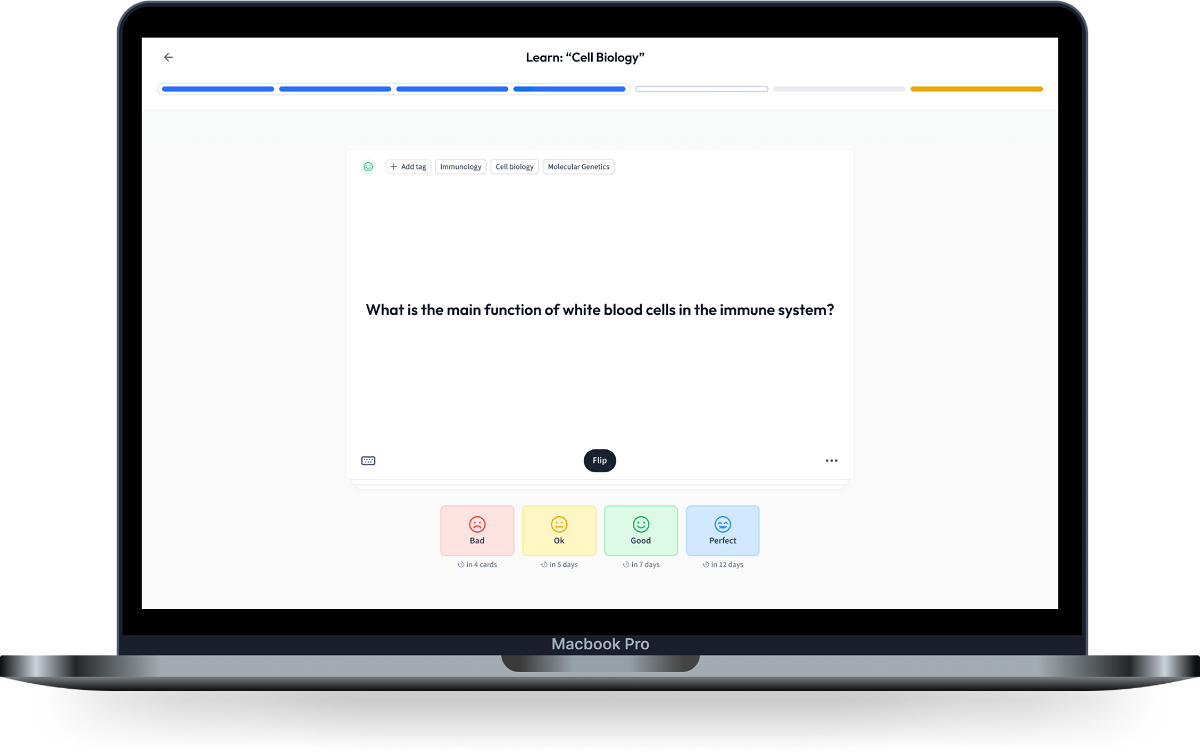
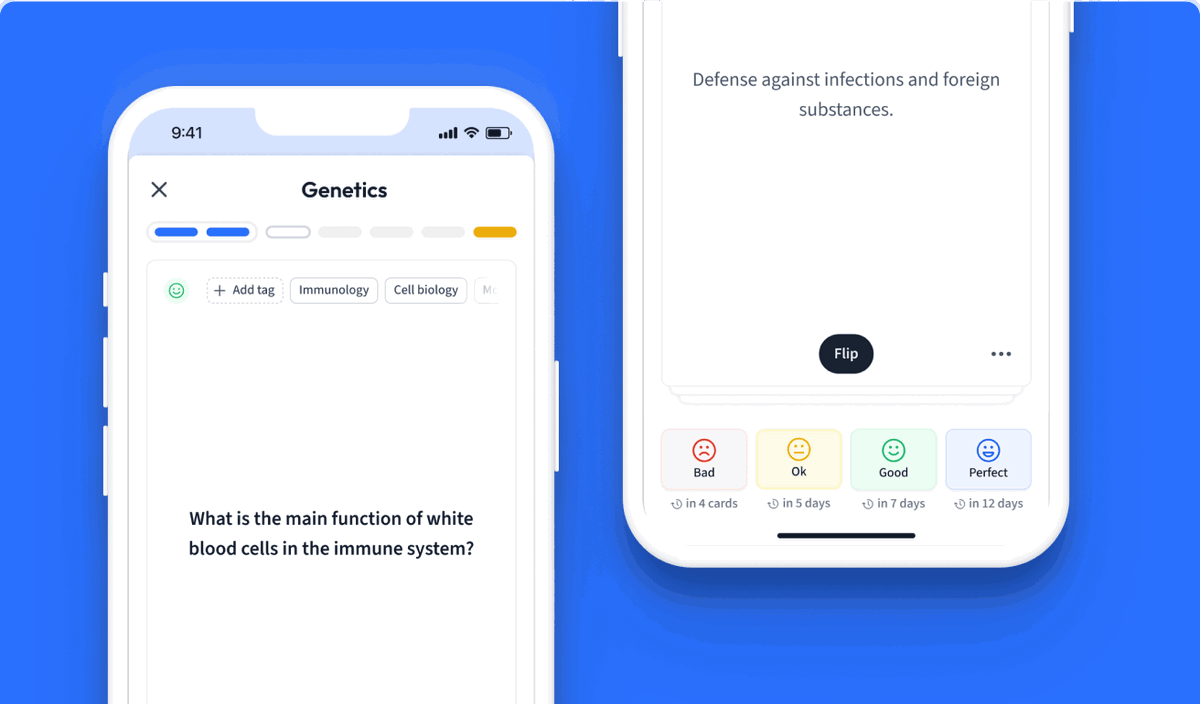
Learn with 27 Javascript Object Prototypes flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript Object Prototypes
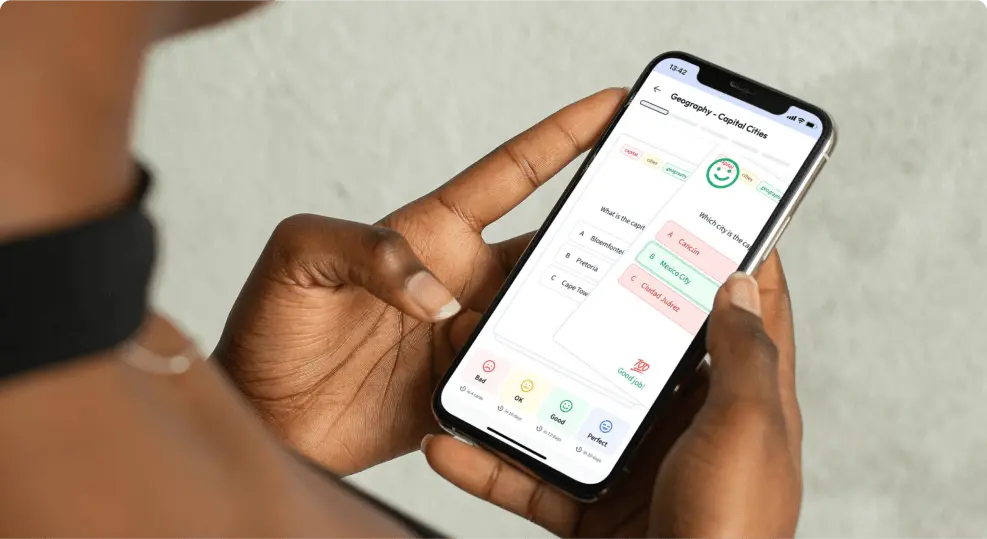
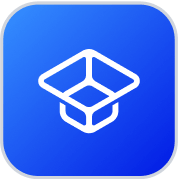
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more