Understanding Java Data Types: An Introduction
You might be wondering why Java data types play a significant role in programming. Simply put, Java Data Types help to identify the type of data your variable holds. By declaring a data type, you can manage the operations performed on your variables effectively. Your understanding of Java data types will upgrade your efficiency in managing values and operations in your program.
Basics of Java Data Types: What is data type in Java?
At a fundamental level, a Java data type is a classification of data that describes the possible values that can be assigned to it and the operations that can be done on it. Java offers two types of data types: Primitive Data Types and Non-primitive Data Types, also known as Reference or Object Data Types.
Primitive Data Types refer to the most basic data types that Java supports natively. They include byte, short, int, long, float, double, char, and boolean.
Non-primitive Data Types, on the other hand, are not defined by Java language, but by the programmer. They include Classes, Interfaces, and Arrays.
Importance of Data Types in Java Programming
Understanding data types in Java is crucial for several reasons. For starters, it helps to minimize errors during the coding process, especially those linked to the performance of operations on incompatible data types. Secondly, knowing the exact data type can help ensure memory is used efficiently. Additionally, data types allow explicit declaration of the operations allowed on the variables and prevent misuse of data.
Differentiating Primitive and Non-primitive Java Data Types
While both are essential, understanding the difference between Primitive and Non-primitive data types is crucial in mastering Java programming. Here is a comparison:
Primitive Data Types | Non-Primitive data types |
These data types work faster because they correspond to a single value. | These data types are slower because they correspond to multiple coordinated values. |
They always have a value. | They can be null. |
They are less flexible and offer fewer methods. | They are more flexible and provide numerous methods. |
Closer Look into Abstract Data Type Java
Beyond Primitive and Non-Primitive Data types, an essential aspect to explore in Java is the Abstract Data Type (ADT). An ADT is a type for objects whose behaviour is defined by a set of value and operations and not by an implementation specification. Therefore, the process how these operations are implemented is unspecified. Examples of ADT include List, Stack and Queue.
How Abstract Data Type Functions in Java Programming
ADTs in Java provide a level of abstraction that hides the implementation details. Although it may sound complex, it simply implies that as programmers, you need not worry about how the operations are performed. Your focus should be on using the right operations as the programming language takes care of the implementation.
For example, consider an ADT of a List in Java. You need only to know how to add, remove, or get items from the list. How these operations are performed by the List is irrelevant from your perspective. This abstraction makes it possible for you to use the List functionality without understanding the complexities of its implementation.
Practical Uses of Abstract Data Type in Java
The use of ADTs in Java gives you greater control over your code. ADTs allow you to encapsulate the data and its operations within a data type, thus promoting cleaner and more manageable code. They offer flexibility in changing the implementation of the data type without affecting the rest of the code, and also make debugging easier, as you can test the ADT independently of other code.
Detailed Insights into Long and Double Data Types in Java
Let's divert your attention onto two fundamental Java data types: long and double. Understanding these data types and when to use them in your code will significantly assist your efficiency and consistency when programming in Java.
Exploring Data type long in Java
In Java, the long data type is a primitive data type which is used when an int data type is not large enough to hold the value. It's principal use is for numbers that exceed the range of the int data type. The size of a long data type is 64 bits, and its value range is -9,223,372,036,854,775,808 (-263) to 9,223,372,036,854,775,807 (263-1). This immense range makes it the prime choice for use in applications that require a wide expanse of numerals.
Remember, when designating a numeric value to a long variable, you should append the letter 'L' or 'l' at the end. However, to avoid confusion with the number '1,' it's typically best to use an upper-case 'L.'
Below is an example illustrating defining a long variable, assigning a value, and manipulating the value:
long myLongValue = 15000000000L; long newLongValue = myLongValue * 3; System.out.println("Your new long value is: " + newLongValue);
Working with long Data type in Java: An Example
Now that you've familiarised yourself with the basics of using long data type, let's delve deeper into a more intricate use case.
Consider writing an application to calculate the distance between two cities, given their coordinates in latitude and longitude. Such a calculation may result in a considerable numeric value, which can be efficiently stored in a long data type:
long latitude = 51000000000L; long longitude = 94000000000L; long distance = 2 * (longitude - latitude); System.out.println("The distance between the two cities is: " + distance);
In the code snippet above, the calculated distance between the two cities exceeds the maximum value range of an int. Consequently, the use of a long integer proves paramount in effective execution.
Understanding Double Data Type in Java
Progressing to the double data type, this primitive type is utilised when floating-point numbers are required. The double data type offers more precision and a wider range of potential values because of its size, which is 64 bits.
A double type is recommended when the numerical value includes a decimal or requires precise calculations. Comparable to the long data type, a double is also efficient in memory usage, assuring optimal utilisation of system resources.
Note that the default type for decimal values is double in Java. However, you can attach 'd' or 'D' if you wish to specify the data type explicitly.
Practical Example of Double Data Type Usage in Java
To get a better understanding of how to use the double data type effectively, consider the following example where precision is vital:
Suppose you need to calculate the average grades for a class of students, considering the decimal fractions.
double sumGrades = 237.5; double numberOfStudents = 10; double avgGrades = sumGrades / numberOfStudents; System.out.println("The average grade is: " + avgGrades);
In the above example, the average can have a decimal value. Thus, the use of double allows for the fractional part to be stored as well, providing a more accurate and precise result.
Comprehensive Guide to Java Programming Data Types
Java data types form the building blocks of any Java program. Whether you're new to Java or an experienced developer, understanding these data types is crucial. They shape your program's logic, acting as the determinants for the values that can be stored and the operations that can be performed.
Summarising Java Data Types and Their Uses
Java data types are categorised into two: primitive and non-primitive (also known as reference or object) data types.
- Primitive Data Types: These are the basic types supported directly by Java and include byte, short, int, long, float, double, char, and boolean.
- Non-Primitive Data Types: These are more complex data types. They include Classes, Interfaces, and Arrays.
Each data type serves a different purpose and has specific properties which influence how it's used. For instance, if you require a data type for whole numbers within a small range, the int data type suffices. However, for larger number ranges, long is more appropriate.
Float and double are similarly used for decimal numbers, with double offering more precision due to its larger size. Char signifies characters, whilst boolean is used for logical values like true or false.
Non-primitive data types come into play when you need to create complex data structures. This includes creating a class (Class), implementing an interface (Interface), or storing multiple values of the same type in a list (Array).
The choice between primitive and non-primitive types isn't solely dependent on the data you intend to represent. It also hinges on factors like memory constraints, potential nullability, performance requirements, and the need for object-oriented features.
How Various Java Data Types Affect Program Output
Understanding how different data types can affect your program's output is essential. The choice of data type has an immediate effect on the precision, scale and memory usage of the program. For instance, using int or float for financial calculations can lead to inaccuracies due to rounding errors, whereas double or BigDecimal can offer more precision.
The range of the data type can also influence the operation results. If a computation's result exceeds the size limit of the data type, it could lead to overflows or erroneous results.
Consider the long data type, which accommodates a larger value. It offers a smoother execution compared to the int data type for calculations that yield high-value results. As a result, your program output remains accurate, facilitating efficient data handling.
Consider the following:
int value1 = 2000000000; int value2 = 2000000000; long result = value1 * value2; // erroneous output long value3 = 2000000000L; long value4 = 2000000000L; long correctResult = value3 * value4; // correct output
In this example, the first calculation will result in an incorrect output due to integer overflow, while the second calculation using the long data type will provide the correct result.
Essential Tips for Choice and Usage of Data Types in Java
Optimal usage of data types is instrumental in crafting a robust and efficient Java program. An insightful guideline to remember is that each data type should fit the requirement exactly with maximum efficiency. Also, it's pivotal to minimise the usage of larger data types due to more significant memory consumption and decreased performance.
- Profit from Numeric Data Types: Utilise int for whole numbers, unless their value exceeds its limit. Use long for larger numbers, but remember to suffix it with 'L' or 'l'. For decimals, prefer double over float for more precision.
- Character and Boolean Data Types: Use char for storing single characters and boolean for logical values. Be aware that boolean cannot be converted to or from any other data type.
- Nullability: Use object data types when you need the option to assign 'null' to a variable.
- Precision is Key: Complex equations and financial calculations mandate BigIntegers and BigDecimals to avert rounding errors.
- Memory Consideration: Choose your data types wisely, considering the memory requirements and limitations.
Your comprehensive understanding of Java data types and their appropriate use cases will strengthen your ability to create effective and efficient programs.
Java Data Types - Key takeaways
- Java data types are a classification of data that describes possible values that can be assigned to it and the operations that can be performed on them.
- There are two main categories of Java data types: Primitive data types (including 'long' and 'double') and Non-primitive data types.
- Primitive data types in Java are the most basic data types that Java supports natively, including byte, short, int, long, float, double, char, and boolean.
- Non-primitive data types are not defined by Java language, but by the programmer and include Classes, Interfaces, and Arrays.
- Abstract Data Types (ADTs) in Java are a type for objects whose behaviour is defined by a set of values and operations, not by an implementation specification, providing a level of abstraction that hides the implementation details.
- The 'long' data type in Java is used when int data type is not large enough to hold the value, particularly for larger number ranges.
- The 'double' data type in Java is utilised when floating-point numbers are required, offering more precision and a broader range of potential values.
- Understanding different data types in Java is critical for program functionality, as the choice influences the program's precision, scale, and memory usage.
- Appropriate use of Java data types provides advantages such as minimizing errors during the coding process, efficient memory usage, and preventing misuse of data.
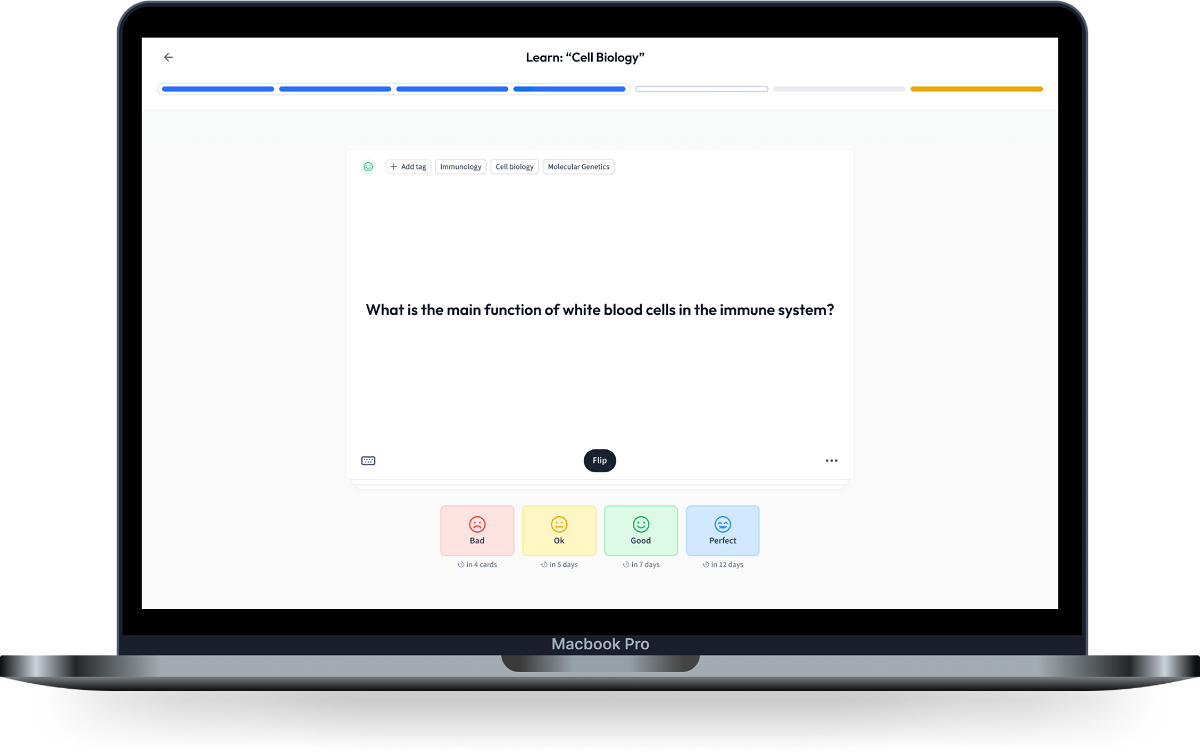
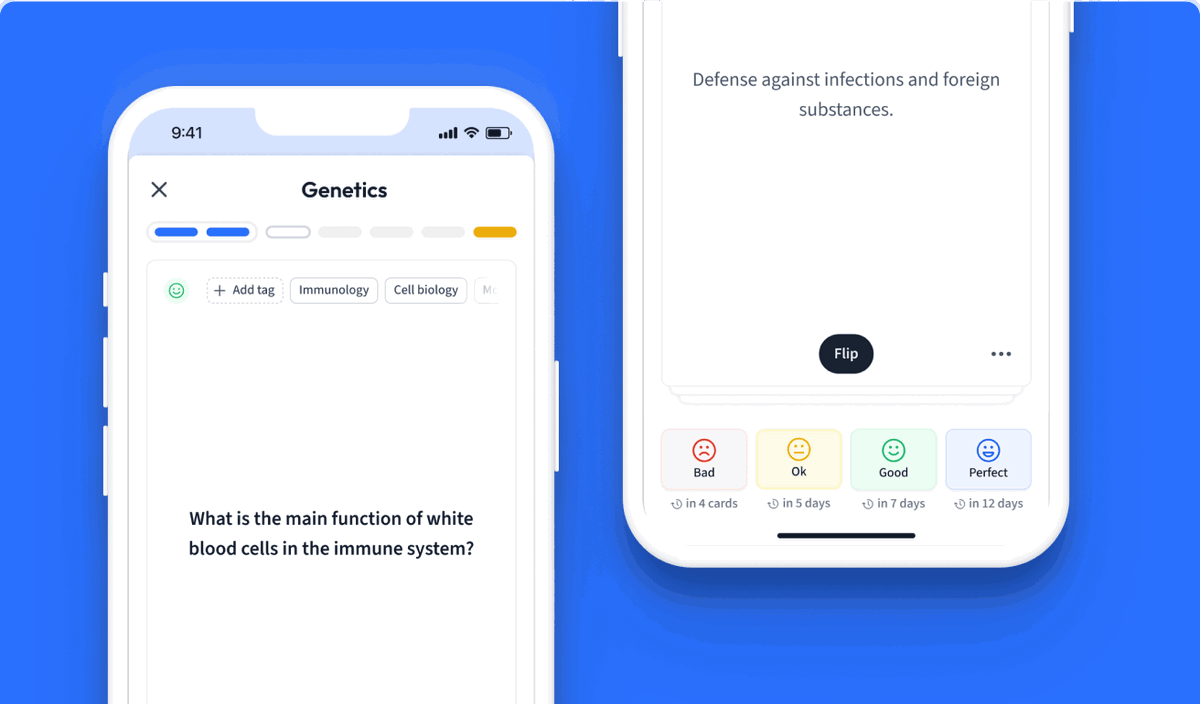
Learn with 48 Java Data Types flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Java Data Types
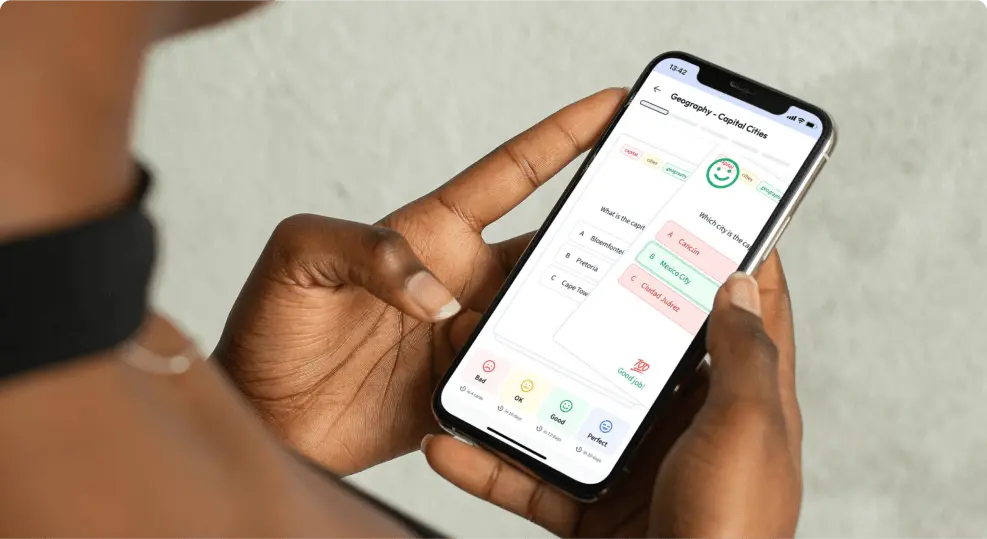
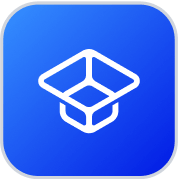
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more