One Dimensional Arrays in C: Definition and Basics
A one-dimensional array in C is a straightforward, linear data structure in which elements are stored in a continuous group. Each element of the array is given a unique index so that the value at a specific location can be accessed and modified easily. The index of the array begins with zero, and elements can be accessed by using the index enclosed within square brackets following the array name.
A one-dimensional array can be defined as:data_type array_name[array_size];
Here, data_type can be any valid C data type (such as int, float, or char), the array_name is the identifier for the array, and array_size determines the total number of elements in the array.
Since the elements of an array share a common data type and related memory, the memory consumption can be managed efficiently.
An example of declaring an integer array of size 5 would be:
int numbers[5];
In this example, integers can be added to the 'numbers' array by specifying their index positions, like so:
numbers[0] = 10; numbers[1] = 20; numbers[2] = 30; numbers[3] = 40; numbers[4] = 50;
Importance of One Dimensional Arrays in Computer Programming
One dimensional arrays are essential in computer programming because they enable the storage and manipulation of data in a more structured and scalable manner. Some advantages of one-dimensional arrays include:- Organization of data: Arrays help in organizing data into a fixed-size, ordered container. This simplifies data storage and retrieval while making code more readable and comprehensible.
- Managing large data sets: Arrays can store a large number of elements in a single variable, facilitating the processing and computation of large datasets without declaring multiple variables.
- Memory efficiency: Arrays allow programmers to allocate blocks of memory efficiently. The elements in the array are stored in adjoining memory locations, making it easy to access and process data in real-time.
- Time Complexity: Using arrays can help optimize algorithms and reduce time complexity due to their precise indexing and simplified data access.
Arrays also act as the foundation for learning and understanding more complex data structures like multidimensional arrays, lists, and linked lists.
- Sorting algorithms: Arrays are essential in implementing various sorting algorithms like bubble sort, selection sort, and insertion sort.
- Mathematical computations: Arrays are useful in mathematical computations, like matrix multiplication, where data is stored in a linear fashion.
- Searching algorithms: Arrays can store data to be searched through linear and binary search algorithms.
- String manipulation: Strings in C are stored as character arrays, which simplifies string manipulation and pattern matching.
One-Dimensional Array Example and Algorithm
Let's analyse an example of a one-dimensional array in C to illustrate its application and implementation. The example demonstrates the creation, initialization, and output of a one-dimensional integer array that holds five elements. The sum and average of the elements are then calculated and displayed.
#include
In the given example, the one-dimensional integer array 'numbers' is created and initialized with five elements. The 'sum' and 'average' variables are then used to store the sum and average of the elements within the array. Throughout the code, the following operations are performed:
- Declaration and initialization of an integer array named 'numbers' with five elements.
- Declaration of an integer variable named 'sum' and its value is initialized to 0.
- Declaration of a float variable named 'average' and its value is initialized to 0.0.
- A for loop is used to iterate through the elements of the array 'numbers'. At each element, the value is added to the 'sum' variable. The loop executes five times, once for each element in the array.
- The sum of the elements in the array is now contained in the 'sum' variable. The average is calculated by dividing the sum by the number of elements (5 in this case), and the result is type casted to a float before being stored in the 'average' variable.
- Lastly, the 'printf' function is used to print the sum and average to the console.
Steps to Implement One Dimensional Array in C Algorithm
When implementing a one-dimensional array in C, there are several steps you need to follow to ensure correct and efficient use of the data structure. The following steps outline the process of implementing a one-dimensional array:
- Declaration:Declare an array by specifying its data type, name, and size within square brackets. The size determines the number of elements that can be stored within the array. Example:
int myArray[10];
- Initialization:Assign values to the elements of the array. This can be done at the time of declaration or later on in the code using the assignment operator. Example:
int myArray[5] = {1, 2, 3, 4, 5};
- Accessing Elements:Access elements in the array using the array name and the index position within square brackets. Remember, the index starts from 0. Example:
int firstElement = myArray[0]; int thirdElement = myArray[2];
- Processing Elements:Use loops (such as for, while, or do-while) to iterate through the elements of the array and perform operations like searching, sorting, or modifying elements. Example:
for(int i = 0; i < 5; i++){ myArray[i] += 1; }
- Input and Output:Use scanf() or fgets() functions for input and printf() for output to store data into the array from the user or display the data to the user. Example:
for(int i = 0; i < 5; i++){ printf("Element %d: %d\n", i, myArray[i]); }
By following the steps outlined above, you can effectively implement and manipulate one-dimensional arrays in a C program, allowing you to manage and process data with ease and efficiency.
Initialization, Addition and Operations on One Dimensional Arrays in C
When initializing one-dimensional arrays in C, it is essential to follow best practices to ensure efficient memory allocation and accurate data representation. This not only helps in writing clean and understandable code but also enhances manageability and scalability.
Here are some best practices for initializing one-dimensional arrays in C:
- Specify the data type: Choose the appropriate data type for the array, such as int, float, or char, based on the elements you will be storing.
- Choose unique array names: Choose descriptive and unique names for your arrays to avoid confusion and naming conflicts.
- Define a constant array size:It is a good practice to declare a constant variable for the array size, which makes it easier to change the size later, if necessary. Example:
const int ARRAY_SIZE = 10;
- Dynamic or static array initialization:Choose between dynamic or static initialization depending on your requirements. Static initialization:Dynamic initialization:
int myArray[5] = {1, 2, 3, 4, 5};
int myArray[5]; myArray[0] = 1; myArray[1] = 2; myArray[2] = 3; myArray[3] = 4; myArray[4] = 5;
- Partial initialization:When initializing an array, you can also partially provide the initial values. If fewer values are provided than the array size, the remaining elements will be initialized with the default value of the data type (e.g., 0 for integers). Example:
int myArray[5] = {1, 2, 3}
- Omit the array size during declaration:When defining an array, you can omit the array size if you provide its initial values at the time of declaration. The compiler will automatically calculate the array size based on the number of initial values. Example:
int myArray[] = {1, 2, 3, 4, 5}
Following these best practices for initializing one-dimensional arrays in C ensures efficient memory allocation, makes the program easier to read, and improves scalability and manageability.
Addition of Two One Dimensional Arrays in C: A Practical Approach
In C programming, it is common to perform operations such as addition on one-dimensional arrays. The addition of two one-dimensional arrays involves adding the corresponding elements of each array and storing the results in a separate array. This section demonstrates a practical approach to add two one-dimensional arrays in C, step-by-step.
- Declare and initialize two one-dimensional arrays of the same data type and size, along with a third array to store the results. Example:
int array1[5] = {1, 2, 3, 4, 5}; int array2[5] = {6, 7, 8, 9, 10}; int resultArray[5];
- Use a loop to iterate through all the elements in the arrays, adding the corresponding elements of each array and storing the sum in the corresponding position of the result array. Example:
for (int i = 0; i < 5; i++) { resultArray[i] = array1[i] + array2[i]; }
- Use a loop to print the result array elements. Example:
for (int i = 0; i < 5; i++) { printf("resultArray[%d]: %d\n", i, resultArray[i]); }
By following these steps, you can efficiently add two one-dimensional arrays in C and store the results in a separate array.
Common Operations and Functions on One Dimensional Arrays
There are several common operations and functions performed on one-dimensional arrays in C, which are essential for processing and manipulating data within the arrays. These operations include searching, sorting, and modifying elements, as well as performing various mathematical calculations.
Some common operations and functions on one-dimensional arrays are:
- Searching:Searching for an element in an array can be performed using linear search or binary search algorithms. Example (linear search):
int searchValue = 4; int index = -1; for (int i = 0; i < 5; i++) { if (myArray[i] == searchValue) { index = i; break; } }
- Sorting:Sorting an array can be done using various algorithms, such as bubble sort, selection sort, and insertion sort. Example (bubble sort):
for (int i = 0; i < 4; i++) { for (int j = 0; j < 4 - i; j++) { if (myArray[j] > myArray[j + 1]) { int temp = myArray[j]; myArray[j] = myArray[j + 1]; myArray[j + 1] = temp; } } }
- Modifying elements:Modifying elements can be achieved by accessing the array using its index and then updating the value. Example:
myArray[2] = 7;
- Mathematical calculations:Performing mathematical calculations like the sum, average, or product of the elements in an array. Example (sum and average):
int sum = 0; float average = 0.0; for (int i = 0; i < 5; i++) { sum += myArray[i]; } average = (float)sum / 5;
These operations and functions are crucial for efficiently managing and processing data within one-dimensional arrays in C programming, allowing you to achieve various tasks and implement algorithms effectively.
One Dimensional Arrays in C - Key takeaways
One Dimensional Array in C Definition: a linear data structure where elements are stored in a continuous group, accessible via a unique index.
One-Dimensional Array Example: declaring an integer array with size 5, adding integers to the array by specifying their index positions.
One Dimensional Array in C Algorithm: steps include declaration, initialization, accessing elements, processing elements, and input/output.
Addition of Two One Dimensional Array in C: adding corresponding elements of each array and storing the results in a separate array.
Initialization of One Dimensional Array in C: best practices include specifying the data type, choosing unique array names, defining a constant array size, and choosing between dynamic or static array initialization.
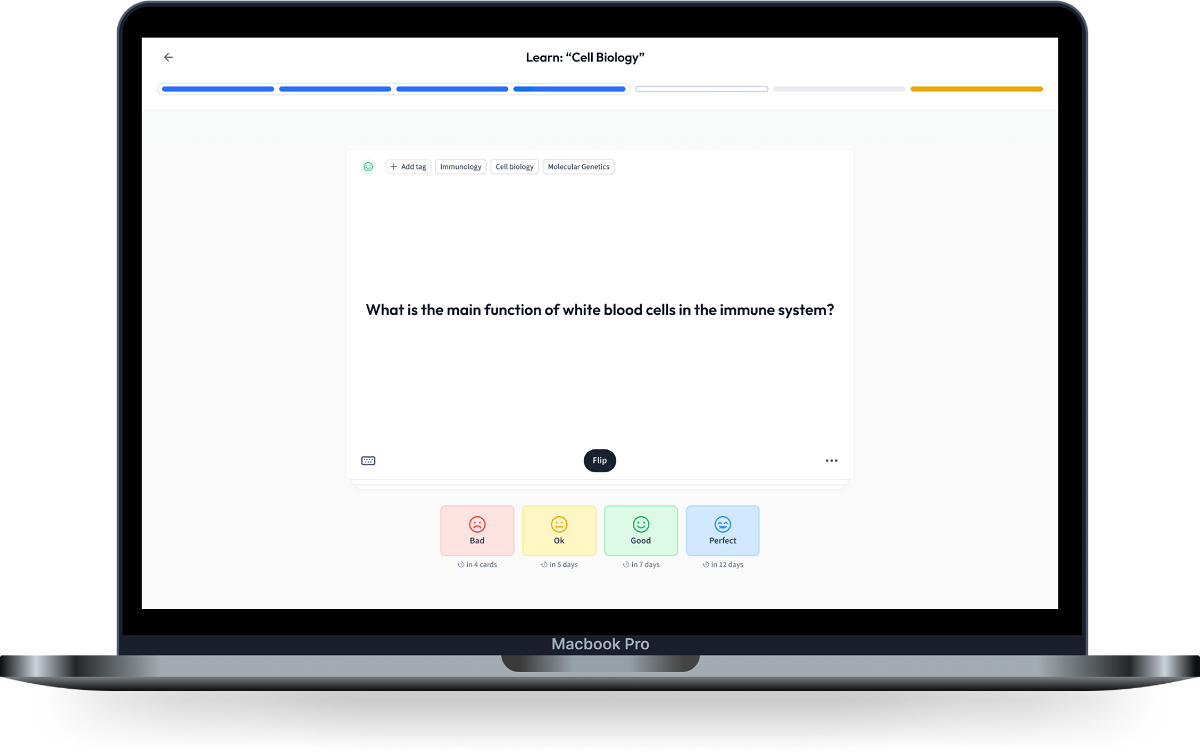
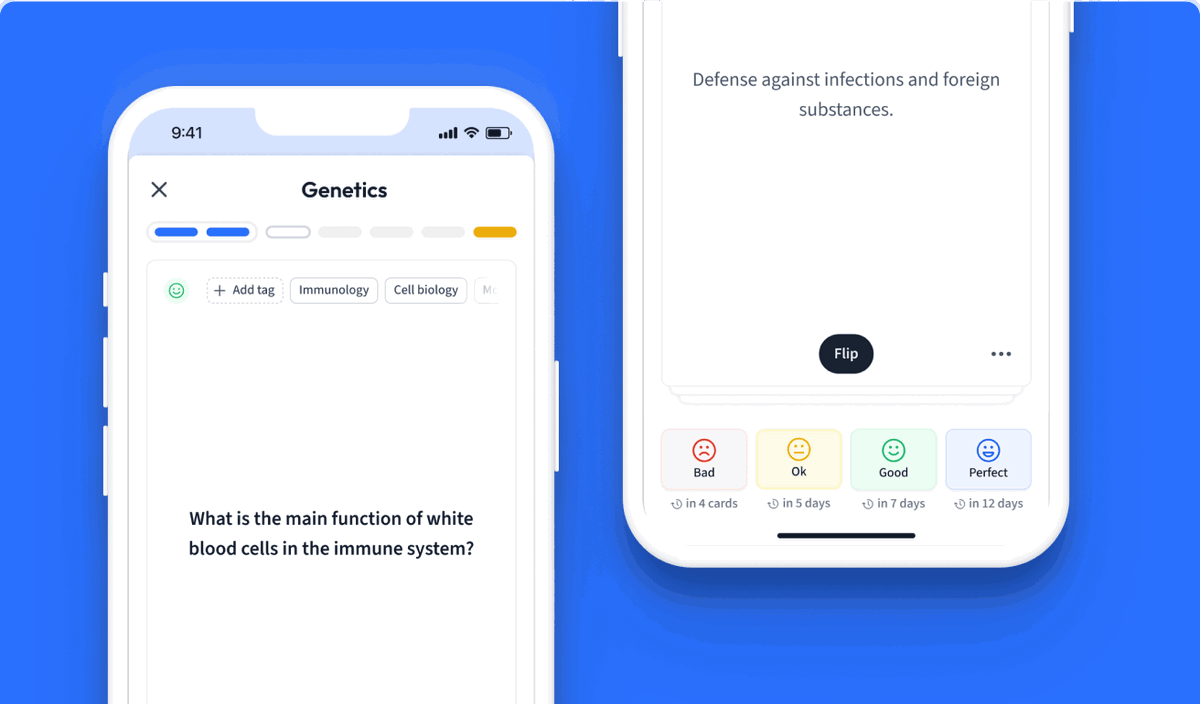
Learn with 11 One Dimensional Arrays in C flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about One Dimensional Arrays in C
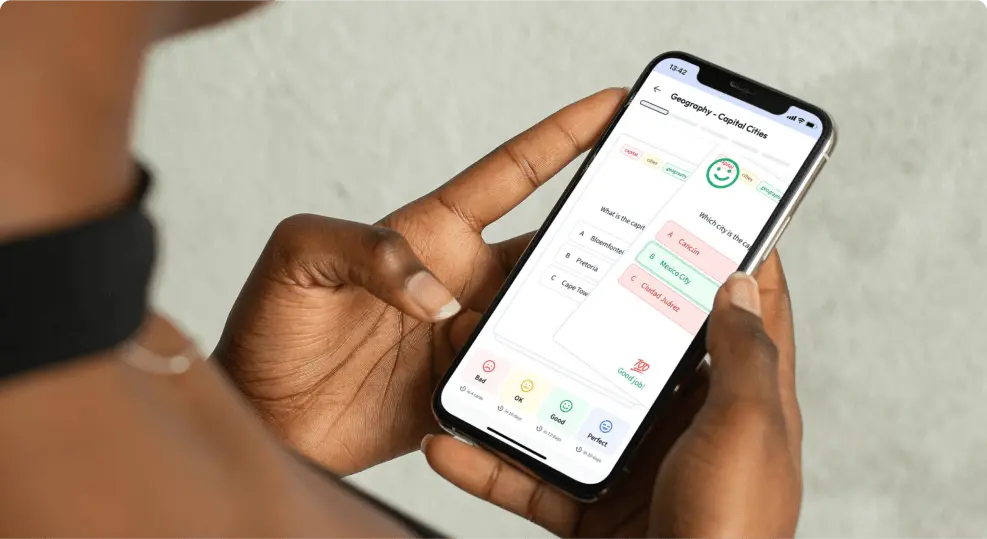
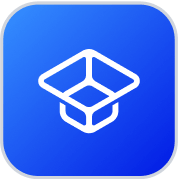
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more