Importance of Array C in computer programming
Array C is an essential concept in computer programming that every programmer should be familiar with. It provides a framework for organising and managing data, which is crucial to the smooth functioning of any programming language. Array C is widely used for storing multiple values in a single variable, which can significantly reduce the workload and complexity of code. In this section, we will discuss the importance of arrays in computer programming.An Array C is a collection of elements, each identified by at least one array index or key, where elements are the same data type.
- Organisation: Arrays help organise data in a structured and easy-to-understand manner. They can represent data in multiple dimensions, allowing programmers to easily manage and manipulate information.
- Efficient storage and retrieval: Arrays allow for efficient storage of data in memory. Since elements are stored in contiguous memory locations, it's easier and faster to access values within the array. This makes data retrieval and manipulation quite efficient and reduces the access times significantly.
- Code optimisation and readability: Using arrays can lead to shorter and simpler code. By storing multiple data values in a single variable, programmers can avoid creating multiple separate variables. This optimisation results in increased code readability.
- Flexibility: Arrays can be used in various ways to meet the needs of different programming needs. They can be resized, reshaped and used in conjunction with other data structures or algorithms.
Advantages of using Array C
There are several advantages associated with using Array C in computer programming, which can make it an essential tool for developers. Some of these benefits include:- Memory management: Arrays enable efficient allocation and deallocation of memory, which helps in managing memory resources effectively in the program.
- Time complexity: Array C data structures have a lower time complexity in the case of certain operations such as random access, making them ideal for data processing tasks.
- Better cache performance: Contiguous memory allocation in arrays can lead to better cache performance and reduced cache misses, providing faster access to the data.
- Reusability: Array C is a versatile data structure that can be easily reused in various applications and algorithms, making it an invaluable tool for programmers.
Applications of Array C in different fields
Array C is not only limited to programming languages but also finds applications in various fields and industries due to its simplicity and efficiency. Some of these applications include:- Scientific modelling: Arrays are widely used in scientific modelling and simulations to represent complex structures and data sets, enabling efficient processing of the data.
- Data mining: Array C is used in data mining algorithms to efficiently analyze large volumes of data by organising it into specific structures.
- Computer graphics: In computer graphics, arrays are utilised for managing image data and pixel values, making image processing tasks more manageable.
- Financial services: Array C finds applications in the financial services industry for tasks such as financial modelling, risk management and portfolio optimisation, among others.
- Machine learning: In machine learning, arrays are used for representing large data sets and managing complex neural networks effectively.
It's worth noting that Array C is not always the optimal data structure in all scenarios. For instance, growing the size of an array can be a time-consuming task, leading to slower performance. Alternative data structures, such as linked lists, can sometimes offer better solutions depending on the requirements of the specific task.
Basic Array Types and Operations in C
In C programming language, an array of integers is a data structure that can store a fixed number of integer values. This section will explore how to declare, initialise, access, and manipulate arrays of integers in C.Declaring and initializing an array of integers
To declare an array of integers in C, you need to specify the data type (int), the array name, and the size within square brackets. Here is the syntax for declaring an array of integers:int array_name[size];For example, to declare an array of five integers, you can use the following code:
int numbers[5];To initialise an array of integers, there are a few different methods. The most common method is to assign values during declaration:
int numbers[5] = {1, 2, 3, 4, 5};You can also assign values individually, using the index:
numbers[0] = 1; numbers[1] = 2; numbers[2] = 3; numbers[3] = 4; numbers[4] = 5;
Accessing and manipulating integers in an array
To access and manipulate elements in an integer array, use the array name and an index inside square brackets. The index starts at 0 and goes up to the size of the array minus 1. For example, to access the first element in the array, use the following syntax:int first_number = numbers[0];You can also perform operations on the elements in the array. For example, to add the first two elements of the array, use the following code:
int sum = numbers[0] + numbers[1];A common operation is to use loops to iterate and manipulate the elements within the array. For example, to print all elements in the array:
for (int i = 0; i < 5; i++) { printf("%d ", numbers[i]); }
Array string in C
An array string in C is an array of characters, grouped together to represent a sequence of characters or text. In this section, we will discuss how to declare, initialise, and work with string arrays in C.Declaring and initializing a string array
To declare a string array, you need to specify the data type (char), the array name, and the size within square brackets. Here is the syntax for declaring a string array:char array_name[size];For example, to declare a string array of 10 characters:
char greeting[10];To initialise a string array, you can either assign values during declaration or assign values individually using the index:
char greeting[10] = "Hello";Or:
greeting[0] = 'H'; greeting[1] = 'e'; greeting[2] = 'l'; greeting[3] = 'l'; greeting[4] = 'o'; greeting[5] = '\0'; // null terminator to indicate the end of the string
Working with strings in an array
Working with strings in an array involves various operations, including accessing individual characters, iterating through the characters, and manipulating them. To access a character in the string array, use the array name and an index within square brackets:char first_character = greeting[0];Iteration through the characters in a string array can be done using loops. For example, to print each character on a separate line:
for (int i = 0; greeting[i] != '\0'; i++) { printf("%c\n", greeting[i]); }Various functions can help work with strings in C, such as strcat (concatenate strings), strlen (length of the string), and strcmp (compare two strings). To use these functions, you will need to include the
#includeint len = strlen(greeting); printf("Length: %d", len);
Advanced Array Topics in C Programming
A 2D array in C, also known as a "matrix" or a "two-dimensional array", is an array of arrays. It can be visualised as a table with rows and columns, where each cell contains an element. 2D arrays are useful in representing and processing multidimensional data, such as storing data in a grid or matrix format. In this section, we will delve deep into declaring, initializing, and using 2D arrays in various programming tasks.
Declaring and initializing a 2D array
To declare a 2D array in C, you need to specify the data type, the array name, and the size of both dimensions within square brackets. Here is the syntax:
data_type array_name[row_size][column_size];
For example, to declare a 3x4 integer array, you would use the following code:
int matrix[3][4];
To initialize a 2D array, you can assign values during declaration using nested curly braces:
int matrix[3][4] = { {1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12} };
Or assign values individually using indices:
matrix[0][0] = 1; matrix[0][1] = 2; matrix[0][2] = 3; matrix[0][3] = 4; // And so on...
Common use cases of 2D arrays in programming
2D arrays find various applications in computer programming due to their ability to represent and manipulate multidimensional data. Some common use cases include:
- Image processing: A 2D array can represent and store pixel data for image processing tasks such as filtering, scaling, and transformation.
- Graphs and networks: Many graph algorithms utilise 2D arrays as adjacency matrices to represent connections between vertices in a graph or a network.
- Spatial data: In geospatial applications, 2D arrays are commonly utilised to store elevation, temperature, or other spatially distributed data.
- Matrix operations and linear algebra: 2D arrays are well-suited for representing matrices and performing operations such as addition, subtraction, multiplication, and inversion.
- Game development: Grid-based games, such as chess or tic-tac-toe, often use 2D arrays to represent the game state and manage game logic.
Array of pointers in C
An array of pointers in C is an array where each element is a pointer to a specific data type. Using arrays of pointers can provide improved efficiency and flexibility in certain situations, such as dynamically allocating memory or when working with multidimensional arrays. In this section, we will explore the concept of pointers in an array and how they can be utilised in programming tasks.
Understanding pointers in an array
To declare an array of pointers, you need to specify the data type followed by an asterisk (*), the array name, and the size within square brackets. Here is the syntax:
data_type *array_name[size];
For example, to declare an array of pointers to integers with a size of 5:
int *ptr_array[5];
Assigning values to an array of pointers can be done by using the address-of operator (&) to assign the address of a variable to each element:
int var1 = 1, var2 = 2, var3 = 3, var4 = 4, var5 = 5; ptr_array[0] = &var1 ptr_array[1] = &var2 ptr_array[2] = &var3 ptr_array[3] = &var4 ptr_array[4] = &var5
Dereferencing the pointers in an array can access the actual values:
int value1 = *ptr_array[0]; // value1 is now 1
Advantages and applications of using array pointers
Array pointers offer several advantages and can be employed in various situations, including:
- Dynamic memory allocation: Array pointers can manage memory effectively by allocating and deallocating memory as required during runtime.
- Flexible array size: Using an array of pointers enables resizing the array during runtime, providing flexibility for various programming tasks.
- Efficient memory usage: Pointers can reduce memory overhead, especially in large arrays or multidimensional arrays, by only storing the memory addresses of the data elements.
- Function parameters: Array pointers can be used as parameters in functions, enabling the passing of large arrays efficiently by passing only the memory address.
- Data structure adaptation: Arrays of pointers are commonly used to create and manipulate advanced data structures such as linked lists and trees.
Define array in C
An array in C is defined as a contiguous block of memory, containing elements of the same data type. Defining an array involves specifying the data type, array name, and size. This section will discuss various methods of defining arrays in C programming and provide tips for efficient array definition and usage.
Methods of defining arrays in C programming
There are several ways to define an array in C:
- Static allocation: The size and elements of the array are fixed at the time of declaration. Memory for the array is allocated during the compile time.
- Dynamic allocation: The size of the array can be specified during runtime, allowing for more flexibility. Memory for the array is allocated during the execution of the program.
- Initialization at declaration: Assigning values to the elements of the array during the declaration process using curly braces and a comma-separated list of values.
- Value assignment after declaration: Assigning values to the elements of the array individually or using loops after the array has been declared.
Tips for efficient array definition and usage
Here are some tips for defining and using arrays efficiently in C programming:
- Choose the appropriate array definition method based on your program's requirements. Static allocation is more suitable when you know the size and elements of the array in advance, while dynamic allocation allows for greater flexibility during runtime.
- Be mindful of array indices, which start at 0 and go up to the size of the array minus 1. Using an index outside the array's bounds can lead to undefined behaviour.
- Make use of loops for efficient value assignment and manipulation within the array. For loops and while loops are particularly useful for iterating through elements.
- Familiarise yourself with C standard library functions for array manipulation, such as memcpy(), memset(), and memmove(), which can provide efficient and high-performance solutions for common operations.
- When working with strings in arrays, always remember to include the null terminator to indicate the end of the string. Neglecting to include a null terminator can result in unpredictable outcomes.
Array C - Key takeaways
Array C: Fundamental data structure in computer programming; provides a framework for organising and managing data, essential for efficient software development.
Array types: Includes array of integers, array string (array of characters), 2D arrays, and arrays of pointers in C.
2D array in C: Also known as a matrix, it's an array of arrays that stores data in rows and columns, useful for representing multidimensional data.
Array of pointers in C: Each array element is a pointer to a specific data type, useful for dynamically allocating memory, efficient storage/retrieval, and working with multidimensional arrays.
Defining arrays in C: Can be done using static or dynamic allocation, initialization at declaration, and value assignment after declaration; efficient definition and usage are important for effective programming.
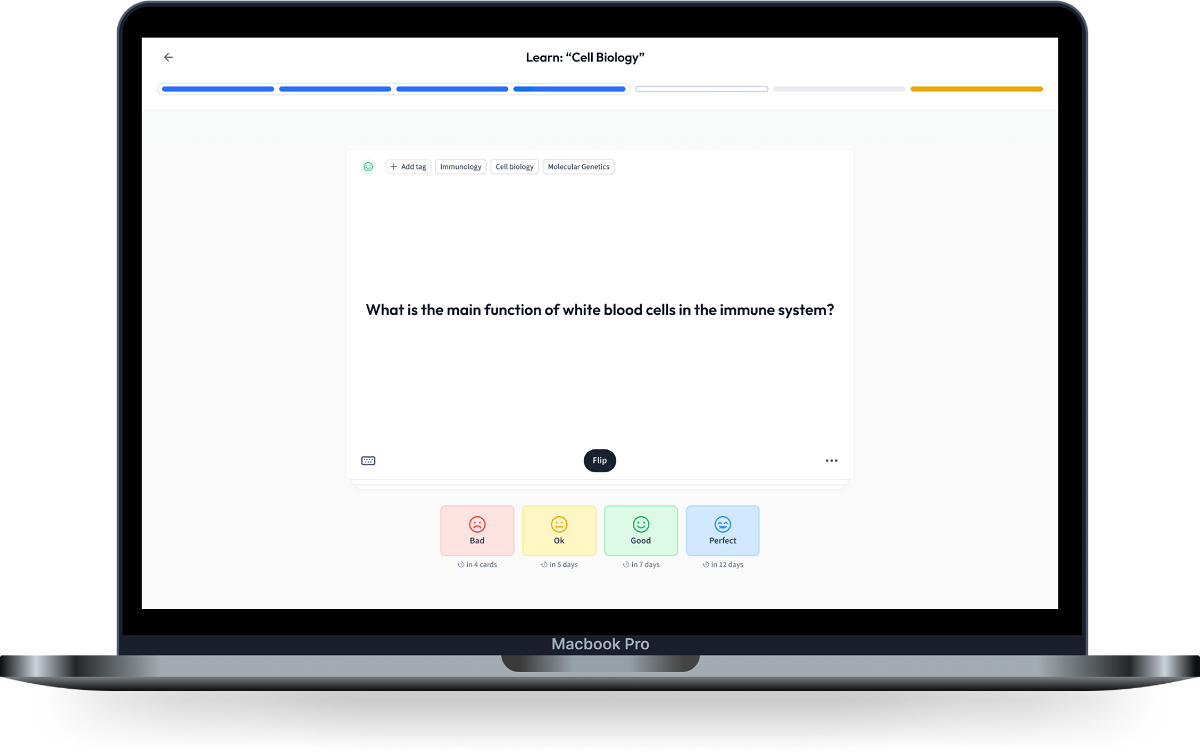
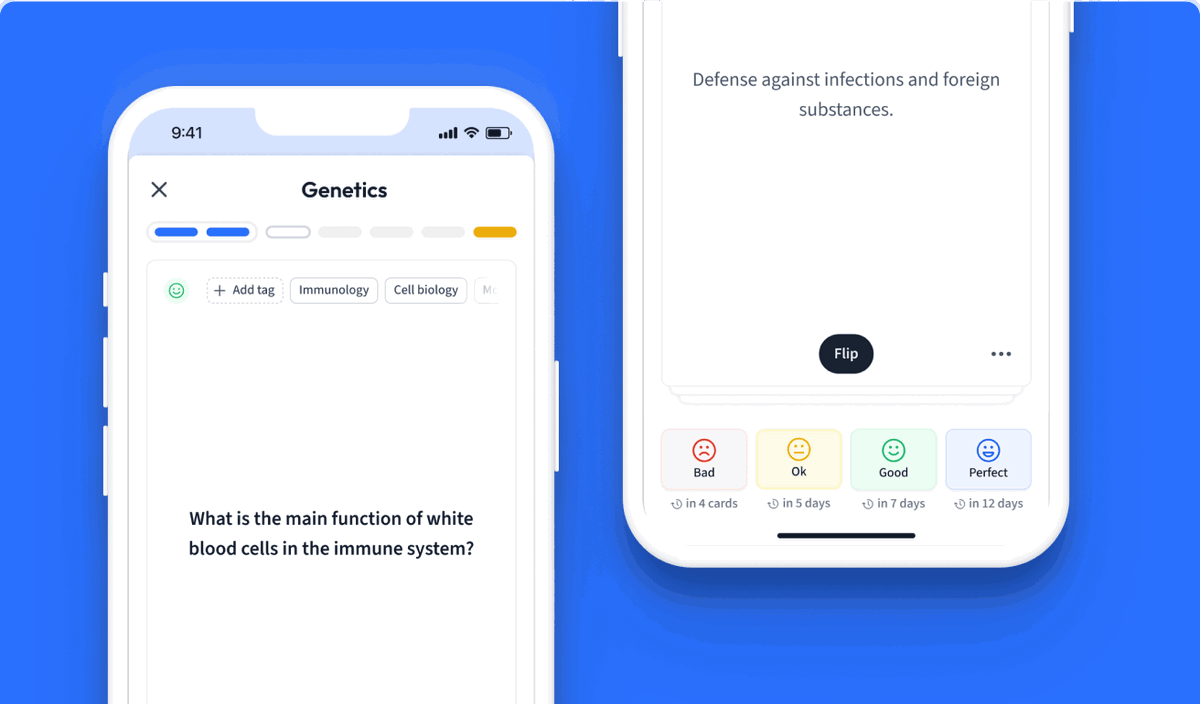
Learn with 78 Array C flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Array C
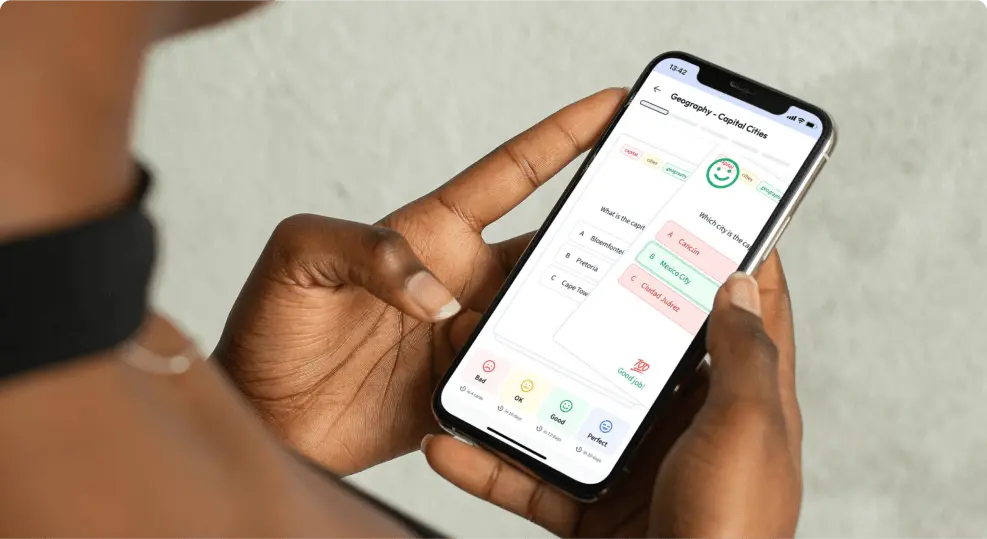
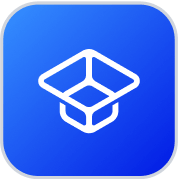
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more