Understanding the Javascript Event Loop
The JavaScript Event Loop, at its core, is a mechanism that handles the execution of multiple threads, allowing JavaScript to appear multi-threaded at the machine level even though in reality, JavaScript isn't.
What is an Event Loop in JavaScript?
JavaScript is a single-threaded language, which means it processes one task at a time while keeping others in a queue. The concept of this queue system leads us to the JavaScript Event Loop. It's a cycle of a constantly running process enabling asynchronous callbacks to be handled in a synchronised manner.Think of it like a busy restaurant. The kitchen (javascript's engine) can only handle one order (function/task) at a time, while the remaining orders get queued. The Event Loop is the restaurant's system that checks if the kitchen is ready to take another order.
The Basic Functions of Javascript Event Loop
The JavaScript Event Loop has several crucial functionalities. These are:- It maintains a task queue.
- It supervises the execution stack and checks its availability to run a new task.
- When the execution stack is empty, it allows the first task out of the task queue to be executed.
- And it repeats this process indefinitely, allowing Javascript to handle multiple tasks and callbacks seamlessly.
How Does the JavaScript Event Loop Work?
The Working of the JavaScript Event Loop is best understood in four stages:- Stack Frame creation: The main() function (or global context) is created and pushed into the current Stack Frame.
- Initial Code Execution: The browser starts reading and executing code line by line.
- Callback Queue: Asynchronous functions (like setTimeout) are sent to a Web API and pushed to the Callback Queue after they're completed.
- Event loop check: When the Stack Frame is empty, meaning that all the code has been read, the event loop checks the Callback Queue. If it's not empty, functions are dequeued and pushed onto the Stack Frame for execution.
The JavaScript runtime contains a message queue, which is a list of messages to be processed. Each message has an associated function which gets called to handle the message. At some point during the event loop, the runtime starts handling the messages on the queue, starting with the oldest one. To handle the message, the associated function is called with the message as an input parameter. As soon as the stack is again empty, a check is made to see if there is any pending timed-out delay function and if there is, that function (such as a delayed function from setTimeout) is executed, and this starts a new message execution. So, even though JavaScript is single-threaded, it can still do concurrency thanks to the event loop and the browser's or Node.js' APIs.
function main() { console.log('Hi'); setTimeout(function cb1() { console.log('cb1'); }, 5000); console.log('Bye'); } main(); // Output // "Hi" // "Bye" // "cb1" after 5 secondsIn the above code example, we first call the main function. It has three steps - first, it logs "Hi" to the console, then it sets a timeout for 5 seconds, after which it will log "cb1", and finally, it logs "Bye". Because JavaScript only has a single call stack, it handles these operations one after another. So, you'll first see "Hi" logged, then "Bye", and finally, after five seconds have elapsed, "cb1". Even though the setTimeout function was called second, it doesn't stop the rest of the code from executing - this non-blocking behaviour is thanks to the event loop.
Exploring Examples of Event Loop in JavaScript
Understanding the working of JavaScript Event Loops can sometimes be a daunting task. To help demystify the concepts, let's explore a couple of examples. We'll tackle this in two steps - a simple Event Loop example, and then a more complex one.Simple Event Loop in JavaScript Example
Imagine a very simple JavaScript code that prints out a string after a certain delay. Below is an example of such code in action:setTimeout(function() { console.log("Hello after 3 seconds"); }, 3000);When this piece of code runs, the console remains empty and waits for 3 seconds before "Hello after 3 seconds"', is printed out. This delay occurs because of the nature of the JavaScript Event Loop mechanism. Here's how JavaScript accomplishes this:
- Once the setTimeout function gets encountered in the code, since it’s a Web API, it’s sent out of the call stack to a Timer Web API.
- This Timer counts for 3000 milliseconds.
- After the Timer finishes counting, it pushes the function specified in the setTimeout into a Callback Queue.
- The Event Loop, which was running indefinitely, checks if the call stack is empty.
- Once it’s empty, it dequeues the function from the Callback Queue and pushes it onto the call stack for execution.
Complex Event Loop in JavaScript Example
Now let's consider a more complex scenario. Imagine a script that sets two timeouts - one that prints immediately and another that prints after one second:setTimeout(function() { console.log("Hello"); }, 0); setTimeout(function() { console.log("Hello after 1 second"); }, 1000);In this scenario, despite the first setTimeout function having a delay time of zero milliseconds, it will not print immediately. Instead, it gets moved into the Web API environment, and after the delay is complete, it will get pushed into the Callback Queue. In the meantime, the JavaScript engine sees the second setTimeout function and moves on to that. Once both completed setTimeout functions return from web APIs, they are sent to the Callback Queue. The function execution order in the Callback Queue is determined by their order of completion. Finally, the Event Loop continuously monitors the call stack and the Callback Queue. When the call stack is empty and the Callback Queue has executable functions, it moves them into the Stack for execution. Therefore, at first, "Hello after 1 second" will be printed, and then "Hello" will get printed. Throughout this process, the Event Loop prioritizes ensuring that the call stack gets cleared as quickly as possible before it can receive any incoming messages from the message queue. That's why, even when set to zero seconds, the first setTimeout function's callback doesn't execute immediately. It waits upon the completion of the entire script, demonstrating how the Event Loop gives higher priority to the call stack over the queued messages. This interplay between the Call Stack, Web API, Callback Queue, and Event Loop is the quintessential functioning of the JavaScript runtime environment and is essential in understanding how asynchronous code works in JavaScript.
Javascript Event Loop and Call Stack
JavaScript is a single-threaded language, but thanks to the interplay of the Event Loop and the Call Stack, it can handle multiple tasks with a high degree of efficiency.Understanding the Relationship between JavaScript Call Stack and Event Loop
The JavaScript runtime environment has two key elements: the Call Stack and the Event Loop. They are responsible for how JavaScript handles executing code. Let's delve into their relationship and functioning. The Call Stack is a data structure that records the execution context of the functions. When a JavaScript function is called, it's placed, or 'pushed', onto the Call Stack. It's then executed by the JavaScript engine. Once the function execution is complete, it's 'popped' out of the stack. If there happens to be a function within a function, the inner function is pushed onto the stack and becomes the currently executing function. On the other hand, the Event Loop is a continuously running process that checks if the Call Stack is empty. If the Call Stack is empty and the Callback Queue is not, it pushes the first callback function from the Callback Queue onto the Call Stack. The importance of the Call Stack for the Event Loop is twofold:- The Call Stack largely determines when the Event Loop pushes new callbacks onto it. That is, only when the stack is empty, the event loop moves functions from the Callback Queue to the Call Stack.
- While a function is being executed, the Call Stack is 'busy', and the Event Loop won't add more to it. That is, if a function is taking too long to execute, it can block the Call Stack and prevent other code from running.
function functionOne() { console.log('Function One'); function functionTwo() { console.log('Function Two'); } functionTwo(); } functionOne(); // Output: // Function One // Function TwoBoth functionOne and functionTwo are added to the Call Stack as they're called, and removed after they're completed. Because functionTwo was called within functionOne, it was added to the top of the stack and became the currently executing function.
How JavaScript Call Stack Influences Event Loop?
The behaviour of the JavaScript Call Stack has a significant impact on the Event Loop. Due to JavaScript's single-threaded nature, the Call Stack can contain only one function execution context at a time, influencing when and how the Event Loop processes tasks. The Event Loop is constantly checking if the Call Stack is empty. If it is, and if there are callback functions waiting in the Callback Queue, those functions get pushed onto the stack. However, if the Call Stack isn't empty, these callbacks have to wait, even if the currently executing function is taking a long time to complete. In such a scenario, we witness what's commonly known as 'blocking'. A code block that takes a significant amount of time to execute, like a large loop or a function with high computational needs, can halt the flow of functions from the Callback Queue to the Call Stack. This phenomenon, known as blocking the Event Loop, is a common performance problem that should be avoided for smooth execution of JavaScript code.for (let i = 0; i < 1000000000; i++) { console.log('Blocking Code'); } setTimeout(function() { console.log("Non-blocking Code"); }, 0);In the above example, the loop may block the call stack as it executes. The message "Non-blocking Code" won't be printed until after the for loop finishes, even though the setTimeout delay is set to 0 seconds. This shows how the call stack's 'busy' state affects the Event Loop. In conclusion, the Call Stack's behaviour influences the effectiveness of the Event Loop significantly, impacting the perceived performance of a JavaScript application. By understanding this relationship, you can write better and more effective JavaScript code.
Mechanism of Event Loop in JavaScript
The Event Loop is at the heart of every JavaScript application, responsible for managing the execution of scripts and rendering in browsers. To fully appreciate its importance and understand how JavaScript code runs asynchronously despite being single-threaded, let's delve deeper into the mechanism of the Event Loop and its timing.Breaking Down the Event Loop JavaScript Mechanism
At the core of its mechanism, the Event Loop handles the execution of multiple chunks of your JavaScript code. Each piece of this code might be a function, a script, or an event triggered by a user or a Web API. To understand the mechanism, let's unravel the components of JavaScript's concurrency model, which are essential components of the JavaScript runtime environment: Call Stack: It's the place where your JavaScript code is being executed, one operation at a time. When a line of code is ready to run, it's added (or 'pushed') onto the Call Stack. And when it finishes running, it's removed (or 'popped') from the stack. Web APIs: These are built-in libraries provided by the browser, for operations that the JavaScript language itself doesn't handle. Once a Web API operation (such as a fetch request or a timer) completes, it adds a message to the Callback Queue. Callback Queue: Once a function finishes its operation in Web APIs, it's added to this queue. Functions are queued in the order they finished their execution in Web APIs. Finally, here's what the Event Loop does: - It checks if there's an operation waiting on the Stack. If the Stack is empty, it moves the first operation from the Callback Queue to the Stack. - This process continues incessantly, making sure that every executed function gets the CPU time it needs, and ensuring that the user's interactions do not get stuck waiting in the Callback Queue for too long. Thus, the Event Loop maintains the balance and the non-blocking nature of your JavaScript code.Understanding the Timing in Event Loop JavaScript Mechanism
Understanding the timing of the Event Loop mechanism is crucial to writing efficient, non-blocking JavaScript code. Let's delve into the key timing factors:- Zero Delays: A major misconception about the JavaScript timer functions like setTimeout() is that the time parameter represents an exact wait time for its callback function's execution. However, that's not the case.
setTimeout(() => { console.log('Hello World'); }, 0);The code above does not guarantee that 'Hello World' will be printed exactly after 0 milliseconds. This is because the timing doesn’t account for the time taken by the Call Stack, the Event Loop, and the Callback Queue to process other operations. When the Event Loop takes the callback function out of the Callback Queue and onto the Call Stack, it only does so when the Stack is empty. This can create a delay.
- Order of Execution: In Event Loop, the order of execution doesn’t necessarily follow the order in the Call Stack. Instead, it's dictated by the order of operations in the Callback Queue. When operations that have been sent to Web APIs finish, they're added to the Callback Queue. The Event Loop picks operations from the Callback Queue in the order they were added.
setTimeout(() => { console.log('First'); }, 2000); setTimeout(() => { console.log('Second'); }, 1000);Although 'First' appears before 'Second' in the code and hence, into the Call Stack first, 'Second' will be printed before 'First'. This is because the Event Loop will push 'Second' to the Callback Queue before 'First', as it has a smaller time delay. As a result, 'Second' gets printed first. Understanding these timing nuances and factors can significantly enhance your JavaScript proficiency. Throughout the process, the Event Loop ensures the smooth execution of tasks, maintaining concurrency in JavaScript's single-threaded environment.
Javascript Event Loop in Asynchronous Programming
Even though JavaScript is inherently single-threaded, its ability to handle tasks asynchronously is one of its most striking features. The magic behind this feature is primarily due to the Javascript Event Loop.Role of Javascript Event Loop in Asynchronous Programming
The respect that JavaScript commands as a robust, versatile language owes much to its ability to operate seamlessly on various mediums like browsers and servers, and this is largely down to its asynchronous behaviour. The asynchronous capability is due to the Event Loop's functionality, based within the JavaScript's runtime environment, where it works alongside structures such as the Call Stack and Callback Queue. Asynchronous programming allows JavaScript to execute tasks outside of the main flow of the program without blocking the execution of subsequent code. This non-blocking nature ensures that your program doesn't get locked up, waiting for tasks like network requests or timers to complete, while there's other code that could be running. That's where the role of the Event Loop becomes crucial. The Event Loop's job is to constantly monitor the Call Stack and the Callback Queue. If the Call Stack is empty, it takes the first task from the Callback Queue and pushes it to the Call Stack for execution. This simple process is what enables asynchronous behaviour in JavaScript. Any function that needs to be run asynchronously, such as setTimeout or fetch, gets sent to a Web API module (such as the Timer or HTTP request module, built into the browser). After the Web API operation is complete, it pushes a callback function into the Callback Queue. The Event Loop then ensures that these callback functions are sent back to the Call Stack when it's ready for more tasks.Understanding Asynchronous Programming Through Javascript Event Loop Examples
Understanding the Event Loop through practical code examples can help cement the concept. Let's explore a few examples to demonstrate how the Event Loop manages asynchronous JavaScript code. In the simple example below, a timer function runs asynchronously, thereby not blocking the execution of the 'After setTimeout' log.console.log('Before setTimeout'); setTimeout(() => { console.log('Inside setTimeout'); }, 0); console.log('After setTimeout');In the output, you'll notice that 'Inside setTimeout' gets printed last, despite a delay of 0 milliseconds. This is due to the Event Loop. Although 'Inside setTimeout' is part of the setTimeout function, it gets passed to the Web API, freeing up the Call Stack. The Call Stack moves on to execute 'After setTimeout'. Only when the Call Stack is cleared and the timer has completed does the Event Loop move 'Inside setTimeout' from the Callback Queue back to the Call Stack for execution. Understanding the Event Loop's role in this scenario explains why asynchronous JavaScript works the way it does. Even though the setTimeOut function appears as though it should output 'Inside setTimeout' before 'After setTimeout', it doesn't. Let's examine another example:
console.log('Before fetch request'); fetch('https://jsonplaceholder.typicode.com/posts') .then(() => console.log('Fetch successful')) .catch(() => console.log('Fetch failed')); console.log('After fetch request');In this case, the fetch request is handled asynchronously, similar to the setTimeout function. Upon calling fetch, it's offloaded to a Web API (the HTTP requests module), letting the Call Stack move on to 'After fetch request'. The '.then()' callback only gets pushed to the Callback Queue once the fetch operation completes. Even if it takes time for the resource to be fetched from the URL, the rest of the program continues running without waiting for the fetch request to complete. The Event Loop, once again, manages this smoothly. From these examples, the evolving role of the JavaScript Event Loop becomes clear: to keep your JavaScript code non-blocking and running smoothly by efficiently managing the Call Stack and the Callback Queue around asynchronous operations.
Javascript Event Loop - Key takeaways
- Javascript Event Loop: A continuously running process that checks if the Call Stack is empty. If it is, and the Callback Queue has functions ready, it pushes these to the Call Stack for execution.
- Call Stack: A data structure that records the execution context of functions in JavaScript. Functions are 'pushed' onto the Call Stack for execution and 'popped' out once complete.
- Callback Queue: Functions that have completed their operation in Web APIs are added to the this queue where they are waiting to be executed.
- Non-blocking behaviour: Achieved through the Event Loop, which allows JavaScript to continue with other tasks without waiting for a time-consuming task (like setTimeout) to complete.
- Web APIs: Built-in libraries provided by the browser that handle operations not serviced by the JavaScript language. Once an operation finishes, it adds a message to the Callback Queue.
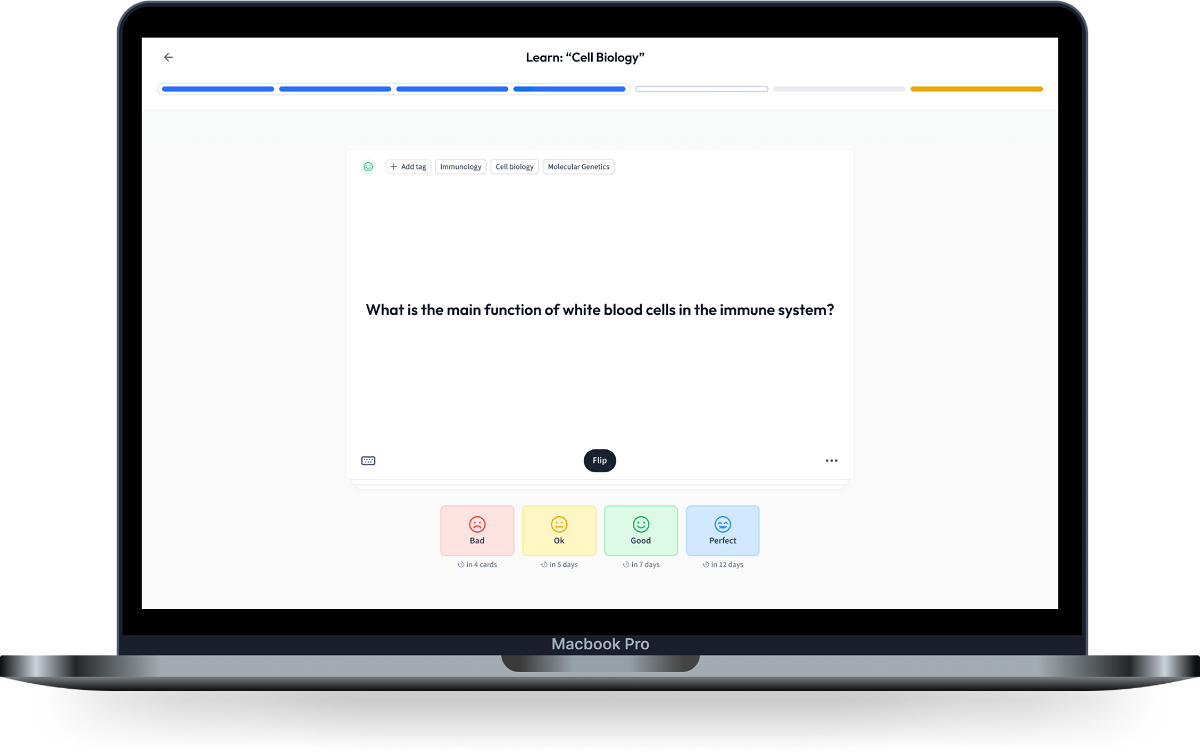
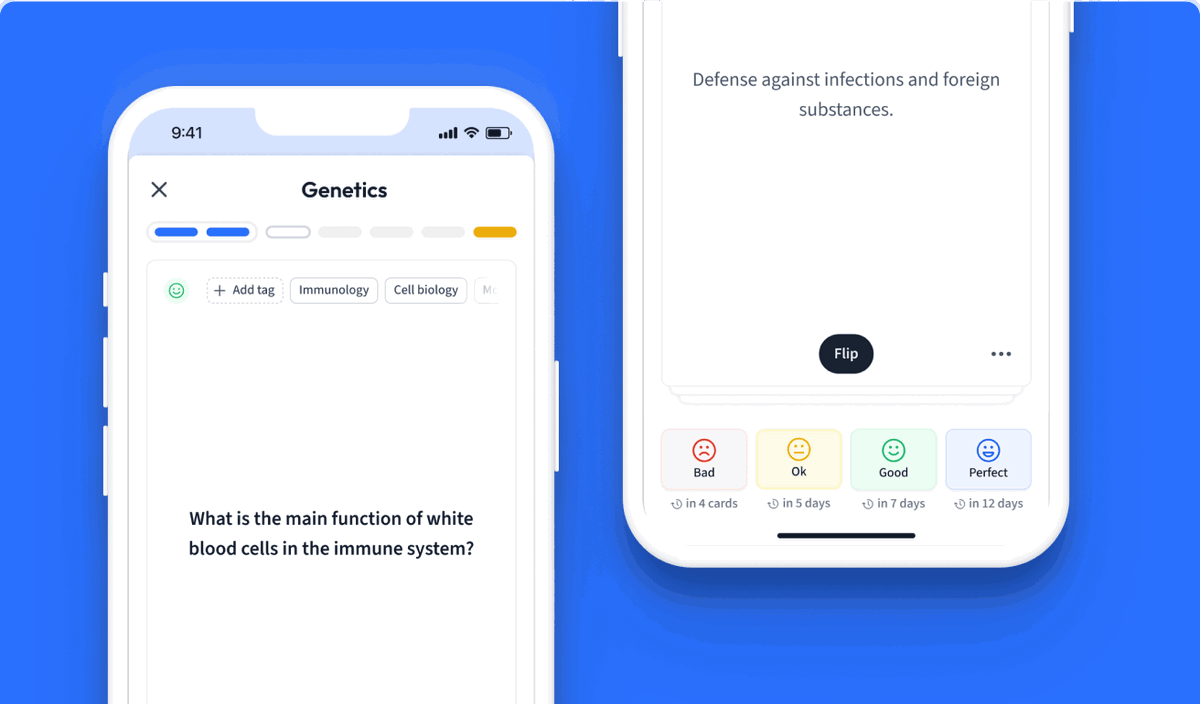
Learn with 15 Javascript Event Loop flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript Event Loop
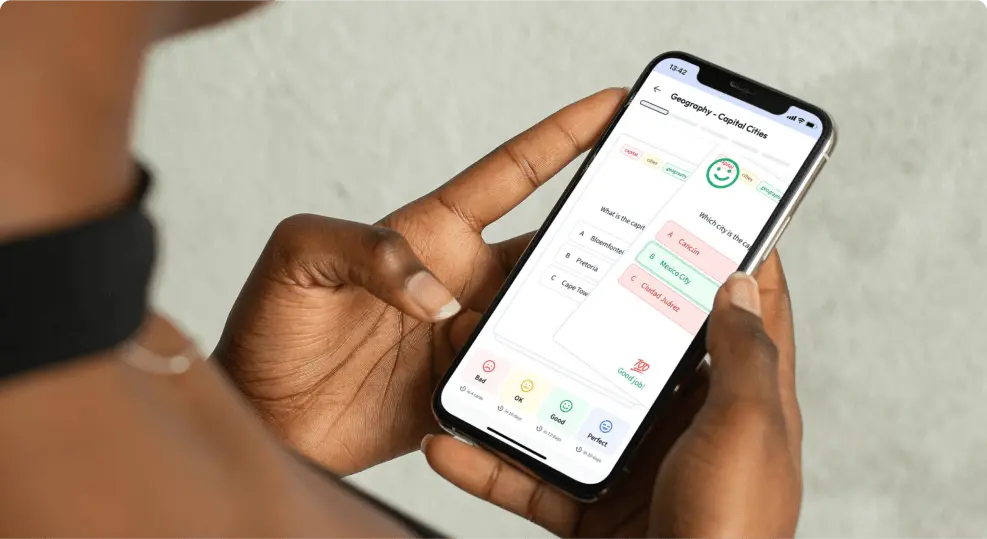
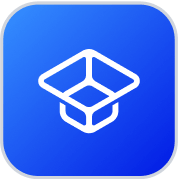
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more