Introduction to Javascript Async
Javascript Async, standing for asynchronous, refers to a design pattern which enables the non-blocking code execution. In simpler terms, it means you don't have to wait for a piece of code to complete before moving on to run the next piece of code.
Understanding the Asynchronous Concept in Computer Science
The term 'asynchronous' stems from the Greek word 'asynkhronos', which means 'not occurring at the same time'. In the realm of computer science:Asynchronous operations are those operations executed independently of the main program flow. Asynchronous actions are actions executed in a non-blocking scheme, allowing the main program flow to continue processing.
SetTimeout
XMLHttpRequest, fetch API
File reading in Node.js
How Javascript Implements Async Functionality
JavaScript implements async functionality through a few techniques:Callbacks
Promises
Async/Await
Callbacks | Functions that are slipped as arguments in another function and only run after the main function's execution is completed. |
Promises | They return a value which might be currently unknown, but will eventually be resolved. |
Async/Await | Makes asynchronous code appear and behave like synchronous code. |
For instance, loading a video is a time-consuming process. But utilizing async functions, a website can load the rest of it while the video is still loading. This results in a better user experience as the user can start interacting with rest of the website without waiting for the video to load.
While callbacks were the earliest way to work with async code, promises and async/await are modern additions to JavaScript and make working with async code much easier and readable.
Comprehending Async Await in Javascript
Understanding Async Await in JavaScript is crucial for managing asynchronous operations in your code efficiently. It's a modern approach that makes your asynchronous code cleaner, easier to read, and more manageable.Breaking Down the Async Await Construct in Javascript
Asynchronous code in JavaScript can be quite complex with traditional callbacks and even promises. With the introduction of Async/Await in ES2017, JavaScript has significantly simplified the way you write and maintain asynchronous code.'Async' and 'Await' are extensions of promises. 'Async' is a keyword that is used to declare a function as an asynchronous function that implicitly returns a promise. 'Await' is used inside an Async function to pause the execution of the function until a specific promise is resolved.
async function myFunction() {...}This code declares an asynchronous function 'myFunction()'. It returns a Promise. If the function throws an error, the Promise will be rejected. If the function finishes its execution without throwing any error, it will resolve.
let result = await someAsyncCall();'await' only works within an async function and is put in front of a call to a function that returns a Promise. It will pause the execution of the async function until the Promise is settled, and then resume the execution with the rejected or resolved value. If you want to execute functionalities alongside the promise that is waiting to be resolved, you can do so without any blocking. JavaScript, being a non-blocking language, brilliantly exploits Async Await for the same. Subsequently, no 'then' callback is required while using Async-Await. Remember, this only makes sense if the awaited Promise is independent of the lines below it in the function. If not, as is quite common, you might as well run your synchronous-looking code line-by-line on its path of asynchronous execution.
Examples of Async Await Use in Real-world Javascript Programming
Async/Await is prevalent in real-world JavaScript programming to handle asynchronous operations. Here is an example of how you can fetch data from an API using Async Await.
async function fetchUsers() { try { const response = await fetch('https://api.github.com/users'); const data = await response.json(); console.log(data); } catch (error) { console.log(error); } } fetchUsers();
Diving Deep into Async Function Javascript
Javascript Async or Asynchronous JavaScript is a methodology focused on improving user experience by allowing certain pieces of code to execute independently without affecting the overall performance of the webpage.The Technicality Behind Async Function in Javascript
Let’s further uncover the technicalities of Async functions in JavaScript. Async functions assist in writing promise-based asynchronous code, as if it were synchronous, but without blocking the execution thread. They are defined using the 'async' keyword. Async functions can contain zero or more 'await' expressions. An 'await' expression causes the async function to pause and wait for the Promise's resolution, then resumes the async function and returns the resolved value.Syntax: \[ \text{{async function name() \{ }} \] \[ \text{{// code }} \] \[ \text{{\} }} \]
async function f() { let promise = new Promise((resolve, reject) => { setTimeout(() => resolve("done!"), 1000) }); let result = await promise; // the function execution pauses here until the Promise resolves* alert(result); // "done!" } f();The async function 'f()' returns a promise. The function execution, paused at "await promise", resumes when the promise resolves, with promise's result becoming the result of 'await', and is then outputted by the alert. This function does not block the CPU, but rather allows it to perform other tasks, like responding to user actions.
Different Techniques of Async in Javascript: A Closer Look
There are different techniques for handling asynchronous programming in JavaScript. These techniques allow JavaScript, which is inherently a single-threaded language, to handle asynchronous operations. The key techniques include:- Callbacks
- Promises
- Generators
- Async/Await
Practical Examples of Async in Javascript
Understanding Async in JavaScript not only necessitates comprehension of theoretical concepts but also demands familiarity with practical applications. Async, the cornerstone of asynchronous programming in JavaScript, finds its implementation through avenues such as APIs, timers, and event handlers, to name a few.Case Studies: How Async is Implemented in Javascript
The primary objective of async in JavaScript lies in efficiently managing time-consuming tasks without blocking the main thread of execution. This affords users an uninterrupted interface, thus improving user experience. A pragmatic square-up with this would be envisioning an HTML form that collects feedback from the user. When the user submits his feedback, it might take several seconds for the server to process and store this information, if done synchronously, the user interface becomes unresponsive. Using JavaScript Async, the user interface remains active and capable of taking other inputs while the feedback is being processed. Another fitting example of asynchronous JavaScript would be accessing and manipulating APIs. Consider a Gallery Page of a website where each picture is fetched via an API. If synchronous programming is used, each image would need to be fetched and loaded completely before fetching the next image. This could lead to an excessively long loading time. Implementing JavaScript Async can handle each API call separately, allowing the images to load simultaneously, drastically improving page load times.
async function getData() { let response = await fetch('https://api.example.com/images'); let data = await response.json(); return data; } async function renderImages() { let images = await getData(); images.forEach(img => { let imgElement = document.createElement('img'); imgElement.src = img.url; document.body.appendChild(imgElement); }); } renderImages();
Unearthing the Asynchronous Nature of Javascript through Examples
An inherent part of JavaScript, Async programming exhibits its need and potential in numerous ways across varied facets of web development. Consider an online shopping platform that allows users to add items to their cart. When a user adds an item to the cart, it takes a few moments for the server to confirm the action. Here, Async JavaScript enables users to continue shopping, browsing through the item descriptions while the server processes the cart update in the background. In another common scenario, consider a news website wherein the weather forecast is displayed. Weather data generally is fetched from an external API. Using Async JavaScript, this task is handled asynchronously. This way, the user does not have to wait for weather data to load before being able to read other news. Yet another implementation of Async is observed in handling user events. Consider the example of a website having a "Load More" button to display more content to the user. Here, when a user clicks the button, JavaScript does not pause the entire website to fetch and display new content. It handles this process asynchronously, thereby keeping the website interactive while new content is loading. In each of the above scenarios, Async shines through by detaching time-consuming actions from the main execution thread, ensuring that the user interface remains responsive. This is the charm and power of asynchronicity in JavaScript. With a focus on user experience and efficiency, Async has found its application spanning across most facets of progressive web development. Ensuring one's ingenuity with Async is, therefore, essential to mastering JavaScript and venturing into high-grade web development.Techniques of Async in Javascript
Asynchronized programming in JavaScript, commonly referred to as Async, is the methodology that enables running parts of a program independently of others. Async is of paramount importance in JavaScript, a single-threaded language, where the potential risk of task stalls during waits could prove troublesome.A Comprehensive Look at Techniques in Async Programming using Javascript
A multiplicity of techniques hosts the implementation of Async in Javascript. Among these, the prominent ones include 'Callbacks', 'Promises', 'Generators', and 'Async/await'. - Callbacks: The simplest among Async techniques, a callback is essentially a function passed as an argument to another function, and it's executed after the parent function finishes its execution. Viewed as JavaScript's traditionally primary means to handle asynchronous operations, Callbacks have been superseded by more modern approaches due to their challenging maintainability with increased complexity, a conundrum often referred to as "callback hell". - Promises: Introduced in ES6, Promises are a significant upgrade to callbacks to handle asynchronous operations. A Promise signifies a probable future event, a mechanism that promises to come back with an answer in the future. Promises have two outcomes: "fulfilled", whereby the operation was successful, or "rejected", indicating an unsuccessful operation. Promises are thenable, meaning they possess a '.then' method. However, Promises, like callbacks, exhibit escalating complexity with increasing synchronised async calls – a problem popularly termed as the "then-callback hell". - Generators: Introduced in ES6, Generators are an advancement over promises. Generators can halt their execution midway and then continue from where it left off, thus making it seem synchronous in its behaviour while still being non-blocking. - Async/await: Introduced in ES8, Async/await is syntactic sugar built on promises, availing the benefits of asynchronous behaviour while writing synchronous looking code. While being the most simplified, straightforward, and widely used approach, Async/await effectively deals with asynchronous operations in JavaScript.The Impact of Asynchronous Techniques on Javascript Programming
Async's role in JavaScript programming is undeniable. It enhances user experience by facilitating uninterrupted usage and allows effective handling of multiple operations simultaneously. Equipping oneself with understanding and proficiency in deploying these techniques can notably influence the handling of varying programming scenarios. - Callbacks were the primary method to handle asynchronous operations in JavaScript but suffered from problems like inversion of control and error propagation. While companies like Node.js extensively used callbacks in their APIs, callbacks have dramatically fallen out of style due to the introduction of promises. - Promises brought along many advantages over callbacks, like better error handling and return values. They enable the chaining of asynchronous operations in a more managed and less error-prone approach than callbacks. - Generators, combined with Promises, meant that Javascript developers could now write asynchronous code that could pause and resume, making their code look and behave as if it were synchronous, yet without blocking the main thread. - With Async/await, complexity got dramatically reduced. Async/await functionality made asynchronous code seem synchronous, but with the advantages of non-blocking execution. Today, Async/await enjoys vast preference over the other techniques of asynchronous programming in Javascript due to its simplicity and intuitiveness. It's essential to understand these asynchronous techniques, as they form the basis for more complex operations in JavaScript such as network requests, access to file systems, and timers. A solid foundation in these techniques can equip you to write more efficient and user-friendly JavaScript applications.Javascript Async - Key takeaways
- Javascript Async: A Javascript methodology focused on improving user experience by allowing certain parts of a code to execute independently without affecting the overall performance of a website.
- Async/Await: Introduced in ES2017, these keywords simplify the process of dealing with asynchronous code in JavaScript.
- Promises: This term represents a value that may not be available yet but will be resolved at some point in the future, or it will be rejected. They improve the handling of complex asynchronous scenarios.
- Callbacks:: This technique involves passing a function as an argument to another function, and it's executed after the parent function finishes its execution. The approach is simple but can lead to challenging maintainability when dealing with complex asynchronous operations.
- Generators:: Introduced in ES6, these are special functions that can be exited and re-entered, with their context saved across entrances. When combined with promises, they offer a synchronous-looking technique to handle asynchronous code.
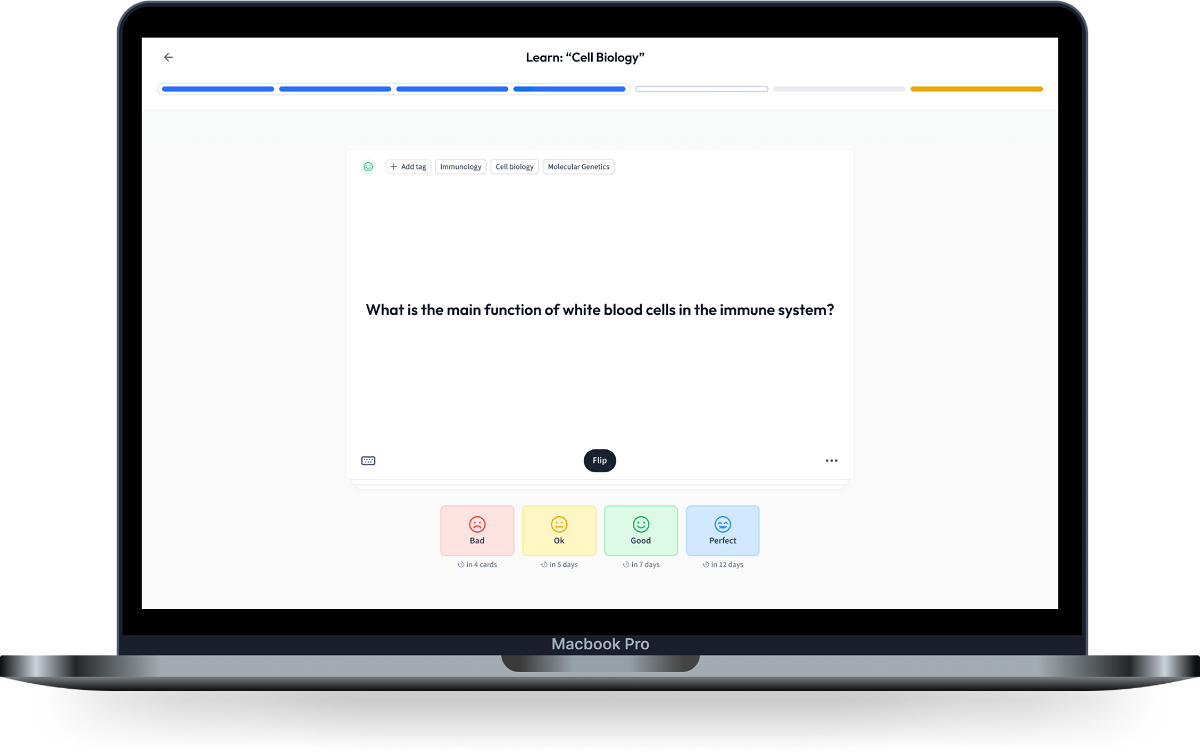
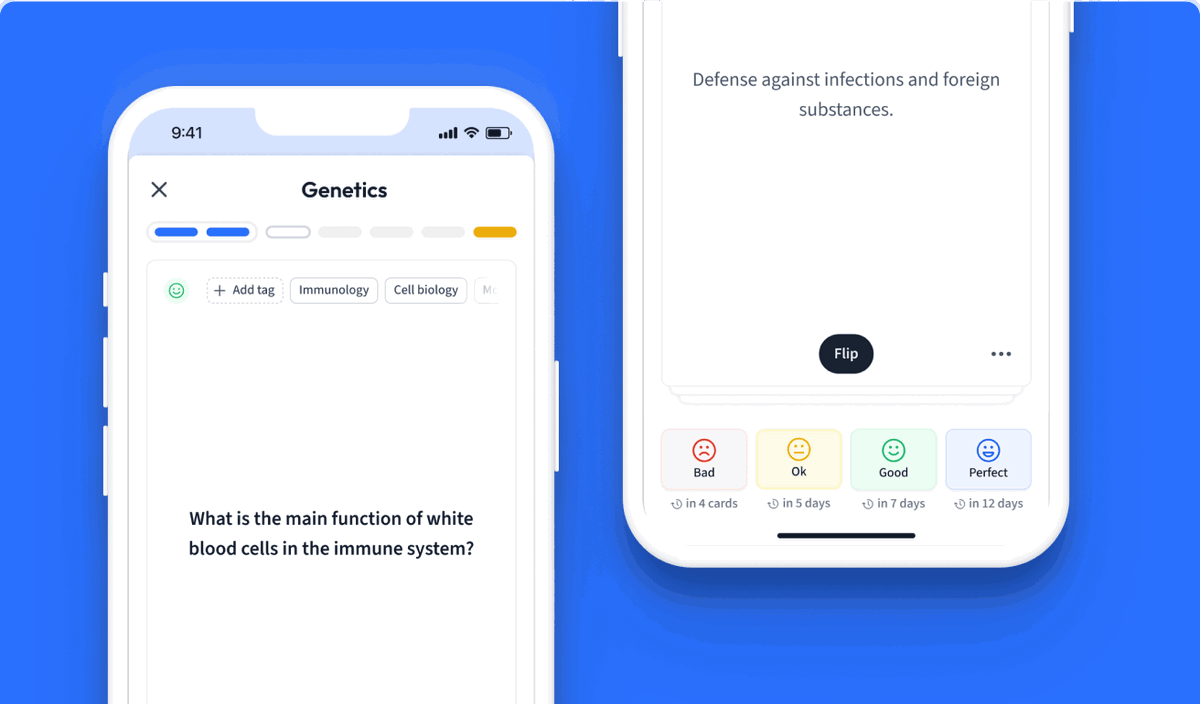
Learn with 15 Javascript Async flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript Async
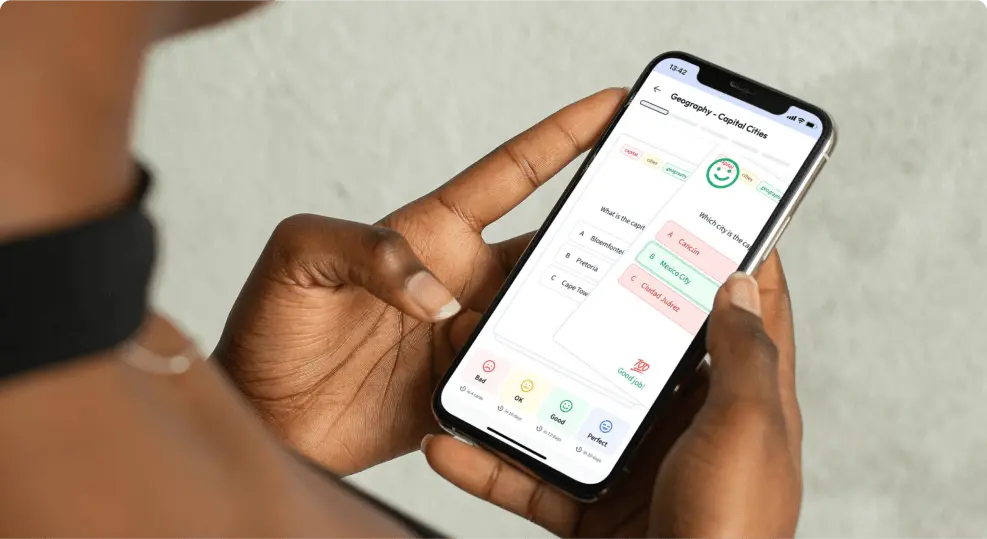
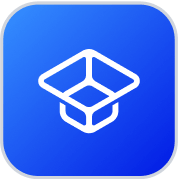
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more