Understanding Java Finally: An Introduction
In computer science, particularly in the context of the Java programming language, the term 'Java Finally' refers to a special construct that helps to manage system resources better and ensures the execution of essential code segments. It's a vital part of exception handling in Java.
The Java Finally Definition: A Deeper Insight
The magic of 'Java Finally' lies in the control it provides. Within the realms of Java, 'Finally' is a block that follows the try or catch blocks. The key feature of 'finally' is that it is always executed, irrespective of whether an exception is thrown or not. That's why it often houses essential clean-up code.
The java finally block is used to execute important code such as closing connection, stream etc. The finally block always executes whether exception is handled or not.
Imagine writing a code where you open a file and start doing some manipulations. If an exception occurs in the process, you wouldn't want the file to remain open. It's resource-consuming and leaves your program vulnerable. Here's where 'Java Finally' steps in, providing you with a safety net to ensure that the file is closed, regardless of any exceptions in the process.
Let's illustrate 'Java Finally' in action with a table:
Try Block | Block of code in which exceptions might occur, File I/O operations, etc. |
Catch Block | Provides an exception handler to manage exceptions thrown by the try block. |
Finally Block | Contains crucial code that must be executed regardless of an exception's occurrence. |
Did you know? Despite its name suggesting finality, the Java Finally block executes before the method completes- whether it's be due to a return statement, reaching the end of the method body, or even an unhandled exception!
How Do We Define Java Finally in Programming Context?
Now we know what 'Java Finally' practically does, but how do we define it more formally in a programming context? Well, 'Java Finally' is defined as a keyword in Java that forms a block of code, which follows a try and possibly multiple catch blocks. The defining characteristic of 'Java Finally' is that this block of code will be executed no matter what - whether an exception occurs or not.
Consider the following Java code snippet:
try { int x = 10 / 0; } catch (ArithmeticException e) { System.out.println("An Arithmetic Exception Occurred."); } finally { System.out.println("This is Finally Block. And, it's always executed."); }
Due to the division by zero in the "try" block, an "ArithmeticException" exception will occur. This exception is caught and handled by the "catch" block. Irrespective of this exception, the "finally" block is still executed, and thus, "This is Finally Block. And, it's always executed." will be printed on the console.
In conclusion, Java Finally is a construct designed for the specific purpose of allowing vital cleanup tasks to be carried out notwithstanding whether a particular block of code throws an exception or not. This vital feature ensures that system resources are managed effectively and eliminates potential vulnerabilities that can be exploited.
Breaking Down the Java Finally Syntax
The syntax of Java Finally is generally straightforward and easy to understand. At its most basic level, the 'finally' keyword in Java is used as part of the "try-catch-finally" construct for exception handling. It can be broken down into three vital sections namely, 'try', 'catch', and 'finally' itself.
Examining a Basic Java Finally Syntax in Detail
When diving deeper into the realms of 'Java Finally', it's important to recall the key purpose of this construct: to ensure specific segments of code always execute, regardless of whether an exception is thrown or not.
Strictly speaking, 'Java Finally' looks something like this:
try { // code that might throw an exception } catch(Exception e) { // code to handle the exception } finally { // code to be executed irrespective of an exception }
To digest this a bit, let's look at each block individually:
- Try Block: This is where you'll write the code that might potentially throw an exception. It's the origin of uncertainty in your program leading to any kind of exception.
- Catch Block: The catch block is designed to address exceptions thrown by the try block. When an exception is thrown, the program control gets transferred from the try block to the appropriate catch block that's designed to handle that specific exception.
- Finally Block: Now, here's where the 'Java Finally' magic happens. Written after the catch section(s), finally contains the code that will be executed unconditionally, irrespective of whether an exception was thrown or not. This block of code is considered vital, often including resource clean-up tasks like closing database connections, I/O streams, or reseting buffers.
Analysing the Structure of a Java Finally Block
The 'Finally' block in Java is consistent in its structure. It always follows either a 'try' block or a 'catch' block. It begins with the keyword 'finally' followed by a pair of braces {}. The essential code is placed inside these braces.
A small piece of code encapsulating this looks like:
finally { // code to be executed }
You must note that the 'finally' block doesn't necessarily have to come with both - 'try' and 'catch'. The only rule is that it should follow at least one of them. So, you may encounter code structures like 'try-finally' without a catch block involved.
However, it's beneficial to use a 'catch' block as it helps handle the exceptions that can be thrown by the 'try' block. Before going to the 'finally' block, these exceptions need to be addressed to prevent abrupt termination of the program.
While exceptions are crucial in programming, the unsung hero here is indeed the 'Java Finally' block. Despite the uncertainties posed by the 'try' and 'catch' blocks, it remains consistent and reliable in its execution. This construct allows you to write cleaner and more efficient code that effectively manages resources.
Practical Java Finally Examples and Usage
In Java, the 'Finally' keyword plays a crucial role in managing exceptions and ensuring the orderly execution of code. Complementing its theoretical understanding with practical examples is essential.
Relevant Examples of Java Finally in Use
Dive deep into how 'Java Finally' operates in a variety of scenarios by examining these comprehensive examples:
Finally Block Without Catch
In some cases, you might use a 'finally' block without a 'catch' block. This is known as a 'try-finally' block. This happens when you're not interested in handling the exception but still want to execute some code irrespective of whether an exception occurs or not.
Take a look at the following code snippet:
try { int x = 100 / 0; } finally { System.out.println("This is Finally Block, and it'll always execute."); }
In this example - even though division by zero triggers an 'ArithmeticException', no 'catch' block handles it. Still, the 'finally' block is executed and prints the message.
Finally with Catch and Multiple Exceptions
A 'try-catch-finally' block can handle multiple exceptions with different 'catch' blocks.
Take a look at the following code snippet:
try { String str = null; System.out.println(str.length()); } catch (ArithmeticException e) { System.out.println("Arithmetic Exception occurred."); } catch (NullPointerException e) { System.out.println("Null Pointer Exception occurred."); } finally { System.out.println("This is Finally Block, and it'll always execute."); }
In this example, a 'NullPointerException' is triggered due to the null value of the string. Although there's an 'ArithmeticException' catch block; it's the 'NullPointerException' catch block that handles the exception. Following this, the 'finally' block is executed.
Exceptions and Java Finally: An Interaction
The exception handling mechanism in Java is built with the inclusion of the 'Java Finally' construct in mind. Not just managing exceptions, the 'finally' construct ensures execution of vital code, regardless of whether an exception occurs, thereby preventing resource leak.
Exception in the Try Block
When an exception occurs in the 'try' block, and it's handled in a 'catch' block, the 'finally' block still gets executed. This concludes that except for abrupt termination of JVM, the 'finally' block will always run after 'try' or 'catch'.
Here's a code snippet demonstrating this:
try { int x = 10 / 0; } catch (ArithmeticException e) { System.out.println("Exception Handled."); } finally { System.out.println("Finally Block Executed."); }
Exception in the Catch Block
If an exception occurs in the 'catch' block, the 'finally' block still gets executed. This is interesting, as the exception in 'catch' is generally considered a consequence of the first exception in 'try'. This layer of exceptions doesn't deter the 'finally' block from executing.
In the following code snippet, you can see how this interaction takes place:
try { int x = 10 / 0; } catch (ArithmeticException e) { int y = 10 / 0; //Another ArithmeticException here } finally { System.out.println("Finally Block Executed."); }
This logic extends to scenarios where exceptions occur in the 'finally' block itself. The 'finally' block will complete its execution until an unhandled exception is thrown again.
To sum it up, exceptions are part and parcel of any programming process and 'Java Finally' is a construct precisely designed to handle and streamline these exceptions. It ensures that regardless of the origin or type of exception, certain segments of code are always executed, providing you with deterministic cleanup of your system resources. Understanding its interaction with various components provides high readability and robustness to your code.
The Role of the Final Keyword in Java
In the vast ecosystem of Java, the 'final' keyword plays a unique and significant role. Used in several contexts, the 'final' keyword signifies immutability and restricts further modification. It fosters consistency and can be used with variables, methods, and classes within Java.
Understanding the Final Keyword and Its Usage in Java
The 'final' keyword acts as an access modifier in Java. Also known as a "non-access modifier" due to its different context of use, it can be applied to classes, methods, and variables.
- Final Variables: When declaring a final variable, its value can be assigned only once and cannot be changed.
- Final Methods: A final method cannot be overridden by subclasses. It ensures that the original functionality defined by the method is retained and unaltered in subclass implementations.
- Final Classes: A final class cannot be subclassed or extended. This implies that no other class can inherit from a final class.
When it comes to deciding when, where, and why to use 'final', certain considerations can regulate your approach. Primarily, the 'final' keyword is used to provide an explicit safeguard against any unintentional modification. When you know that a value or method should not be changed once defined, applying the 'final' keyword ensures your code's integrity.
final int NUMBER_OF_PLANETS = 8;
In the above example, as the value of NUMBER_OF_PLANETS is final, trying to reassign a value would prompt a compile-time error.
Another appropriate usage of the final keyword in Java is when dealing with a method that provides vital core functionality that you don't want to be overridden in subclasses.
public final void performImportantOperation() { // Important code here }
Highlighting the Importance of the Final Keyword in Java
The 'final' keyword in Java performs an essential role in preserving the integrity of the system by ensuring immutability where it matters. It's pertinent in many circumstances and helps in achieving a level of rigidity in the fluid world of programming.
Beyond ensuring immutability, the 'final' keyword can also have significant implications on performance. By declaring a method as final, we give a clear indication to the Java compiler that the method need not be dynamically dispatched, and can instead be statically dispatched - a much faster operation.
The ability to declare a class as final is very valuable in certain situations. For example, the String class in Java is a final class. If the String class weren't final, it could be extended to create mutable subclasses which could lead to serious security risks.
public final class ImmutableClass { // Class contents here }
The 'final' keyword also shines in concurrent programming, where multiple threads might attempt to access and modify shared variables. A final variable can be safely shared across threads without causing data inconsistency or race conditions.
The final keyword has its roots in achieving high quality, robust, and secure Java code. From enhancing performance to preventing inheritance and maintaining thread safety, final is an absolute key player in Java's world.
Understanding the nuances of 'final' can elevate your code’s integrity and help it withstand the test of time and complexity. While the concept of the Java 'final' keyword is simple, the implications it carries cement its importance in your arsenal of Java programming tools.
Now, it’s crucial to highlight that the role of 'final' is not just limited to providing rigidity or thread safety, it dives into ensuring your system's sanity, a calm river amidst the turbulent seas of change.
Dealing with Exceptions in Java Finally
In the realm of Java programming, exceptions are both inevitable and essential. Representing abnormalities or unusual conditions encountered during program execution, exceptions need to be anticipated and managed efficiently. The use of the 'finally' block in Java proves instrumental in such cases, ensuring the execution of clean-up code regardless of whether an exception occurs or not.
How to Efficiently Manage Exceptions in Java Finally
Managing exceptions in Java essentially boils down to employing well-crafted 'try-catch-finally' blocks. The 'try' block encloses code that could potentially generate an exception. When such an exception does occur, it's immediately handed over to the corresponding 'catch' block. If there are no matching 'catch' blocks for the exception or if there were no exceptions at all, the 'finally' block still gets executed.
The primary role of a 'finally' block is to provide a mechanism to clean up after the activities of the preceding 'try' and possibly 'catch' blocks. Regardless of how the 'try' block exits - normally after all code is executed or prematurely due to an exception - the 'finally' block provides a reliable means to perform necessary clean up operations, such as closing an open file or releasing memory resources.
The finally block: A code block that follows 'try' or 'catch' blocks in Java, designed to contain clean up codes that are critical to be executed regardless of whether an exception occurred or not.
It's worth mentioning that every 'try' block should be followed by either a 'catch' block, a 'finally' block, or both. Including a 'finally' block without 'catch' is allowed, however, such a scheme is predominantly used when the clean up code needs to execute even if an exception occurs, but handling the exception itself isn’t required.
An efficient management strategy of exceptions involves anticipating potential exceptions, carefully crafting 'catch' blocks to handle them, and ensuring resource management via the 'finally' block. It's also important to understand that an exception thrown within the 'finally' block would suppress any prior exceptions that led to its execution.
Highlighting Common Exceptions You May Encounter in Java Finally
In the world of Java, there is an array of exceptions that can be encountered and effectively managed within the 'finally' construct. Some common ones include 'NullPointerException', 'ArrayIndexOutOfBoundsException', 'ArithmeticException', and 'NumberFormatException'.
- NullPointerException: This exception is thrown when an application attempts to use an object reference that has a null value. For example, calling a method on a null object.
- ArrayIndexOutOfBoundsException: As the name suggests, this exception is thrown to indicate that an array has been accessed with an illegal index. The index is either negative or greater than or equal to the size of the array.
- ArithmeticException: This is thrown when exceptional arithmetic conditions, such as division by zero, occur.
- NumberFormatException: This exception is thrown to indicate that the application has attempted to convert a string to one of the numeric types but that the string doesn’t have an appropriate format.
While these are some commonly encountered exceptions, it's crucial to understand this is not an exhaustive list, and there are many other exceptions present within Java’s extensive exception hierarchy. When working with 'finally', these exceptions can either be generated within the 'try' block or in the 'catch' block that precedes it. In either case, 'finally' ensures the execution of vital clean up code, providing much-needed consistency and predictability.
The key to managing exceptions in Java finally effectively involves recognizing potential areas of exception generation and catering to every eventuality: either by handling the exception or providing a comprehensive clean-up routine. Knowing about common Java exceptions aids in better predicting, catching, and handling these irregularities and ensuring smooth execution of your program.
Java Finally - Key takeaways
- Java Finally is a construct designed to carry out tasks irrespective of whether a particular block of code throws an exception or not.
- The 'finally' keyword in Java is used as part of the 'try-catch-finally' construct for exception handling. It includes three vital sections namely, 'try', 'catch', and 'finally'.
- The 'try' block contains code that might potentially throw an exception, the 'catch' block handles exceptions thrown by the 'try' block, and the 'finally' block contains code that will be executed unconditionally.
- The structure of a 'finally' block in Java is consistent. It always follows either a 'try' block or a 'catch' block and should follow at least one of them.
- The 'finally' block complements Java's exception handling mechanism and ensures execution of vital code, even if an exception occurs.
- The 'final' keyword in Java signifies immutability and restricts further modification. It can be used with variables, methods, and classes within Java.
- The 'final' keyword can enhance the performance of code by providing an indication to the Java compiler about a method's need for static dispatch.
- Exceptions in Java need to be anticipated and managed efficiently. The 'finally' block ensures the execution of clean-up code regardless of whether an exception occurs or not.
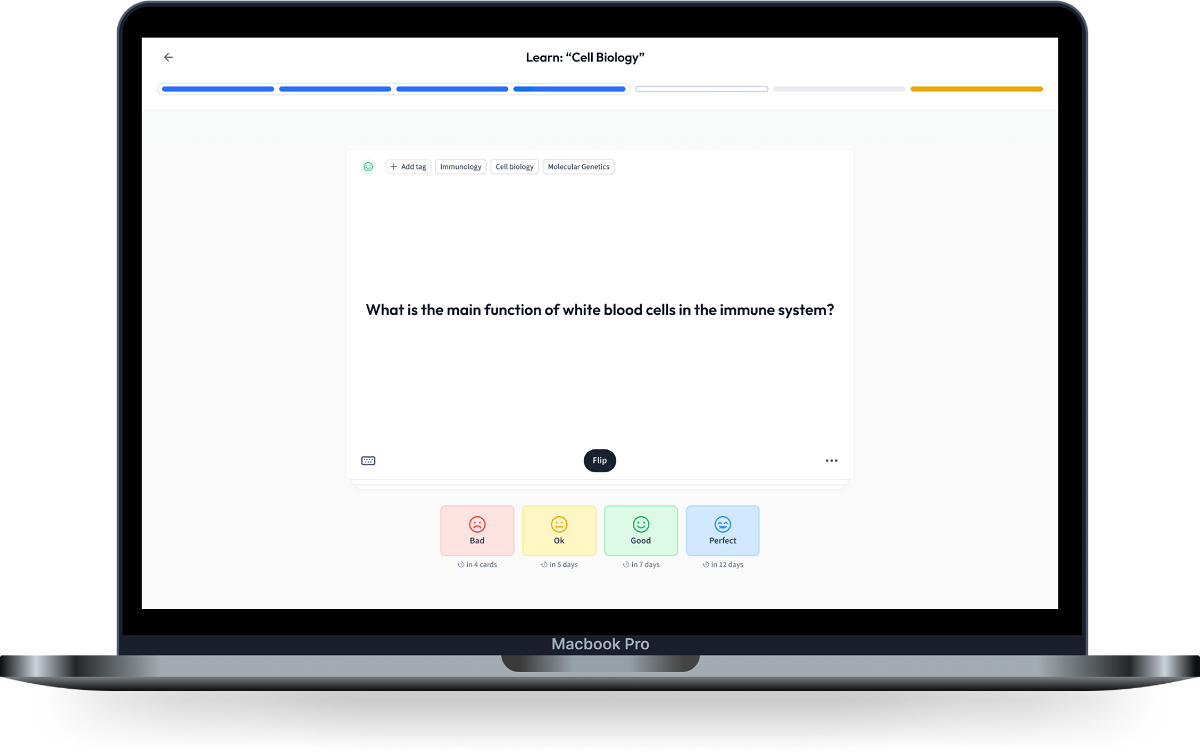
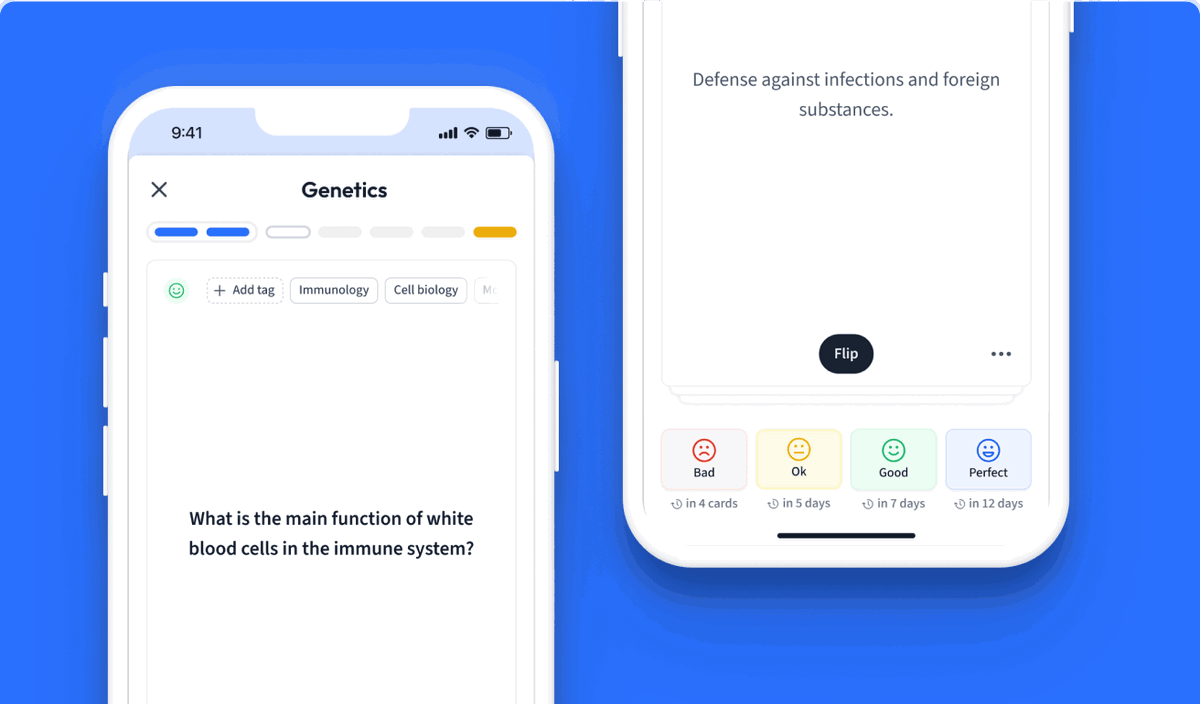
Learn with 15 Java Finally flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Java Finally
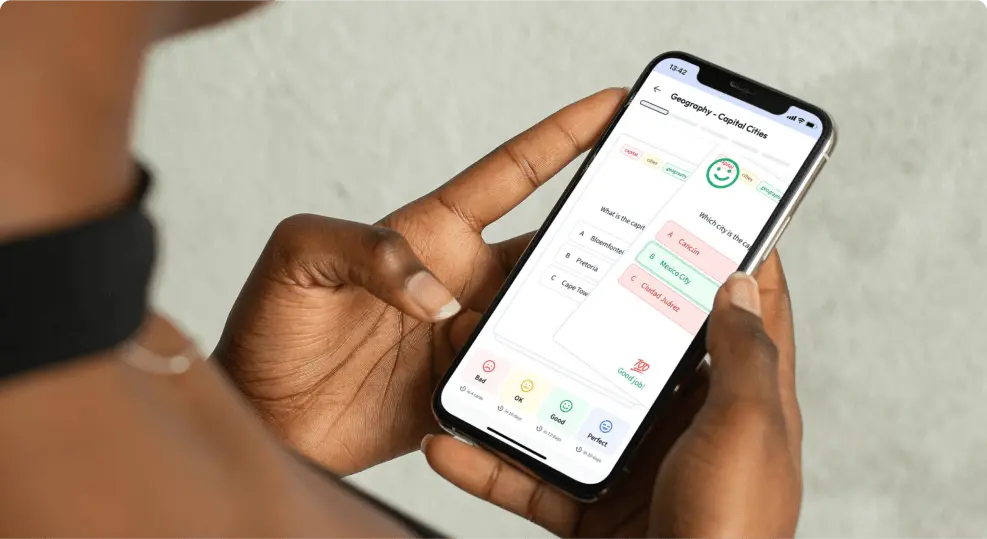
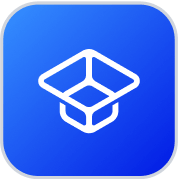
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more