Understanding the Concept: What is Critical Section?
A critical section in computer programming is a section of a multi-process program that must not be executed concurrently by more than one process. In real terms, think of it as a protective code that ensures there's no overlapping while multiple processes or threads are executed.Critical Section: this is the section of code in a multi-threaded program where a process can access shared resources. It is crucial that only one thread enters the critical section at a time to prevent a race condition.
The Role and Importance of Critical Section in Computer Programming
Managing and controlling access to shared resources is the holy grail of concurrent programming. You can think of shared resources like a printer, application data or memory space that needs to be accessed by multiple processes.Imagine running a high-traffic online newspaper. To avoid data corruption and ensure smooth interaction for every user, there must be precise control over how resources are shared amongst the different processes or threads.
- It prevents data corruption caused by multiple threads accessing shared data simultaneously.
- It enhances system performance by providing uniform access to resources.
- It helps to maintain system processing order.
Principles and Rules Governing Critical Sections
Abiding by these principles and rules is paramount for maintaining the integrity of your programs.Look at these principles as security guards that protect your data from getting corrupted by ensuring processes and threads honour access rules when they come into contact with shared resources.
- No two processes may be simultaneously inside their critical region.
- No assumptions can be made about speeds or the number of CPUs
- No process outside its critical region may block other processes.
- No process should have to wait forever to enter its critical region.
Mutual Exclusion | Only one process can execute in the critical section at any given time. |
Progress | If no process is executing in the critical section and some processes wish to enter, only those not executing in their remainder sections can participate in deciding which will enter next, and this decision cannot be postponed indefinitely. |
Bounded Waiting | There exists a bound on the number of times that other processes are allowed to enter the critical section after a process has made a request to enter its critical section and before that request is granted. |
// Critical section code example in C programming language void critical_section() { // declaration of mutex as a global variable pthread_mutex_t mutex; // lock the mutex pthread_mutex_lock(&mutex); // critical section begins here // shared data is being accessed ... // critical section ends, unlock the mutex pthread_mutex_unlock(&mutex); }
Delving Deeper into Critical Section Problem in OS
The critical section problem in operating systems is an issue that arises when shared resources are accessed by concurrent processes. The role of the operating system here is to ensure that when two or more processes require to access the shared resource concurrently, only one process gets the access at a time.Common Issues Associated with Critical Section Problem
Navigating around the critical section problem in operating systems can present several challenges. While managing the access to shared resources might sound simple, coping with these problems often forms the basis of developing more robust systems.Competition, Deadlock, and Starvation are the common issues associated with critical section problem.
How to Counter Critical Section Problem in Operating System
Solving the critical section problem involves careful synchronisation of processes. This is achieved by the implementation of various methodologies that ensure mutual exclusion. These methodologies are classified into two broad types: nonpreemptive and preemptive solutions. 1. Nonpreemptive Solutions: In these cases, a process holding a resource cannot be interrupted. Once the resource has been granted to a process, it remains with that process until voluntarily released.Mutex Lock is an example of a nonpreemptive solution, where a global Boolean variable is used to control the access to the critical section.
// Mutex lock in C #include2. Preemptive Solutions: In contrast, a process can be interrupted in preemptive solutions. A higher priority task can "take over" the resource from another task.pthread_mutex_t mutex; // Declaration of mutex void *func(void *var) { pthread_mutex_lock(&mutex); // Lock the mutex // critical section begins // critical section ends pthread_mutex_unlock(&mutex); // Release the mutex }
An example of a preemptive solution is the Semaphore mechanism, in which a value is designated to manage access to the resource.
// Semaphore in C #includeBoth nonpreemptive and preemptive solutions have their strengths and limitations and are suitable for various application scenarios. The proper selection and implementation of these solutions are key to effectively tackling the critical section problem in an operating system.sem_t semaphore; // Declaration of semaphore void *func(void *var) { sem_wait(&semaphore); // Decrement the semaphore value // critical section begins // critical section ends sem_post(&semaphore); // Increment the semaphore value }
Bounded Waiting in Critical Section Problem
In the realms of computer science, especially in regards to the critical section problem, the idea of 'bounded waiting' plays a pivotal role. Technically defining, bounded waiting refers to the condition where there is a limit or a bound on the number of times other processes can enter and leave their critical sections after a process has made a request to enter its critical section and before that request is granted. This ensures fairness and eliminates the chances of indefinite waiting or starvation.The Concept and Importance of Bounded Waiting
Known as a fundamental aspect of process synchronisation, bounded waiting is the promise that every process will eventually be able to proceed. It ensures no process has to wait infinitely for entering its critical section, thus preventing potential bottlenecks that could severely disrupt program execution.Bounded Waiting: A condition where each process trying to enter its critical section must be granted access in a finite amount of time, preventing the incidence of indefinite postponement.
- Fairness: It ensures that no process is forced to wait indefinitely, thus maintaining a fair playing ground.
- Efficiency: By limiting the waiting time, it enables faster and more efficient execution of processes.
- System Stability: Prevention of potential bottlenecks leads to overall system stability.
Bounded Waiting's Connection to Critical Section Problem
The principle of bounded waiting has significant implications for managing critical section problems. When multiple processes vie for a shared resource, a mechanism needs to be in place to decide which processes gain access and in which order. This is where bounded waiting enters the scene, acting as a decision-making rule. Consider a situation where multiple threads are attempting to enter their critical sections. Without bounded waiting, these threads might create an indecisive situation, also known as convoy effect, where freshly arriving threads continuously push back an already waiting thread. Implementing bounded waiting sets a fixed boundary, preventing this from happening. You might remember one of the computing algorithms we discussed earlier – Peterson's Algorithm. It smartly leverages the principle of bounded waiting. Likewise, the concept of Semaphore we discussed, provides a way to ensure bounded waiting.// Peterson's algorithm making use of bounded waiting int turn; // Shared variable: int turn boolean flag[2]; // Shared variable: Boolean flag[2] void enter_region(int process) { // Process numbers are 0 and 1 int other = 1 - process; // The opposite process flag[process] = true; turn = process; while (flag[other] && turn == process) ; // do nothing } void leave_region(int process) { // Process numbers are 0 and 1 flag[process] = false; }In the realm of operating systems, bounded waiting plays a crucial role in the effective management of critical section problems. By ensuring that all processes are served within a finite waiting limit, it not only allows for efficient process execution but also contributes to overall system stability and robustness. In essence, without bounded waiting, mutual exclusion solutions for critical section problems may lead to unfortunate scenarios like starvation. The concept of bounded waiting hence prevents such pitfalls, making it a key requirement in concurrent programming.
Defining the Terminology: Definition of Critical Section
The term 'Critical Section' is foundational to concurrent programming and multi-threaded systems in computer science. On a fundamental level, a critical section pertains to that segment within a set of instructions or code in multi-threading where the resource, accessible by multiple threads, is accessed and modified.Critical Section: A critical section is a code segment that requires mutual exclusion of access, implying that among several concurrent threads, only one can execute the code section at a time.
The Origin and Evolution of Critical Section Concept
Delving into the origins and evolution of the critical section concept, it's imperative to understand that multi-threaded or concurrent programmes weren't always a part of computer science. Earlier computers execute a task sequentially. However, as the demand for complex tasks, multi-tasking and latency reduction grew, the idea of executing several tasks at once, or concurrent programming, was introduced. Looking back, Edsger Dijkstra, the Dutch computer scientist, is widely recognised for formalising the concept of concurrent programming and addressing the critical section problem. In 1965, he presented a solution, also known as 'Dijkstra's Semaphore', to ensure mutual exclusion by protecting the critical section of the code. Dijkstra's pioneering work laid a foundation for later breakthroughs such as Monitors by C. A. R. Hoare and Condition variables. Over the years, as concurrency control mechanisms evolved, managing critical sections became more efficient with the introduction of lock-free and wait-free algorithms. Modern multi-core processors and complex operating systems have made effective management of critical sections a vital aspect of high-performance software engineering. When discussing the evolution of the critical section concept, concurrent programming cannot be disassociated from the broader concept of 'synchronization'. Concurrent programming's journey from the advent of Dijkstra's semaphore to the recent quantum computing advances is essentially the evolution of synchronization methods, where the critical section is an integral element.Why Critical Section is a Key Term in Computer Science
Critical sections are a cornerstone in the realm of computer science, particularly with the prominence of concurrent programming and multiprocessor systems. When delineating the importance of critical sections, the key to understanding lies in one word - 'safety'. Safety in how shared resources are accessed, safety in how processes are executed, and safety in the overall system’s functionality. Let's consider a banking system where multiple users try to access their account balances simultaneously. Without a proper critical section protocol in place, it's possible for two operations to interleave, resulting in unexpected and incorrect outcomes. Hence, the critical section acts as a control mechanism to ensure such disruptions are avoided, providing an orderly and efficient access method. Critical sections also hold strong relevance amidst the ever-evolving technology trends. In the world of multi-core processors, cloud computing and parallel processing, coordination and protection of shared resources remain a challenging task. Here, the effective management of critical sections plays a pivotal role in boosting system performance by managing the access to shared resources and preventing the hazards related to concurrent accesses. Furthermore, understanding and implementing critical sections correctly helps in avoiding issues related to multi-threading such as race conditions, deadlocks, and data inconsistencies. So, whether you're learning foundational OS concepts or working on a high-concurrency application, understanding the concept of 'Critical Section', its implications and efficient management will always hold a prominent space in your computer science journey.Practical Learning: Example of Critical Section
Grasping critical section concepts through real-world examples is an invaluable learning route. Let's take the leap from theoretical to practical learning and delve into some existing critical section examples in programming.Real-life Examples of Critical Sections in Programming
Learning how to correctly implement critical sections is a breakthrough moment for anyone studying computer science. Observing these scenarios in existing, real-world programmes helps pave the way to mastery.An everyday example of critical sections is in a banking system. Consider a scenario where two people are making a withdrawal from the same account simultaneously. Without proper control mechanisms of a critical section, one thread might read the account balance while the other thread is updating it, leading to inconsistencies.
// Example of a critical section in a banking system #includepthread_mutex_t lock; // Mutex Lock void *withdraw(void *var) { pthread_mutex_lock(&lock); // Lock the mutex // Critical section begins here balance = balance - 100; // A withdrawal is made // Critical section ends here pthread_mutex_unlock(&lock); // Unlock the mutex }
Another example is in a multi-threaded ticket booking system. If two customers try to book the last ticket at the same time, without an effectively implemented critical section, both bookings might be successful, leading to overbooking.
// Example in a ticket booking system #includeThe mutual exclusion feature of a critical section ensures only one thread performs the critical operation at a time, thus maintaining data integrity.pthread_mutex_t lock; // Mutex Lock void *book_ticket(void *var) { pthread_mutex_lock(&lock); // Lock the mutex // Critical section begins here if (available_tickets > 0) { available_tickets--; // A ticket is booked } // Critical section ends here pthread_mutex_unlock(&lock); // Unlock the mutex }
Lessons to Learn from Common Critical Section Examples
Understanding real-life critical section examples in programming provides valuable learning insights. Here are a few key lessons:- Ensuring Data Integrity: Real-life examples make it evident that critical sections are a vital tool for maintaining data integrity in multi-threading environments. They protect shared data from being manipulated by multiple threads at the same time.
- Order of Execution: Critical sections dictate the order of execution for threads. By locking resources for a single thread, they ensure operations occur in a sequential manner, avoiding unexpected outcomes.
- Resource Management: Critical sections effectively manage the usage of shared resources in a controlled manner, thus preventing potential race conditions and deadlocks.
- System Stability: Effectively implemented critical sections contribute to the overall system's stability by preventing potential bottlenecks related to shared resources.
These rules are the 'flag' array and the 'turn' variable. The flag array indicates if a process wants to enter its critical section, whilst the turn variable indicates which process's turn it is to enter the critical section.
// Peterson's Algorithm int flag[2]; // Flag array int turn; void peterson_algorithm(int process) { // Process numbers are 0 and 1 int other_process = 1 - process; flag[process] = true; turn = process; while (flag[other_process] && turn == process) ; ... // Critical section flag[process] = false; ... // Remainder section }Through studying these examples, it becomes clear that the successful implementation of critical section rules is a pivotal point in concurrent programming. Therefore, understanding real-life application examples of critical sections is a decisive step towards becoming proficient in managing concurrent processes and threads.
Critical Section - Key takeaways
- Critical Section: A code segment requiring mutual exclusion of access. Only one of several concurrent threads can execute this code section at a time.
- Critical Section Problem in OS: Issue with concurrent processes accessing shared resources. The OS must ensure that only one process accesses the shared resource at a time.
- Competition, Deadlock, Starvation: Common issues associated with the critical section problem. Competition is when multiple processes require the same resource simultaneously, deadlock is when processes hold part of a resource and wait for the rest, and starvation when processes are indefinitely unable to execute their critical sections.
- Nonpreemptive and Preemptive Solutions: Two methodologies to solve the critical section problem. Nonpreemptive solutions prevent interruption of a process holding a resource while preemptive solutions allow interruption by a higher priority task.
- Bounded Waiting: Condition where each process trying to enter its critical section must be granted access in a finite amount of time, preventing indefinite postponement.
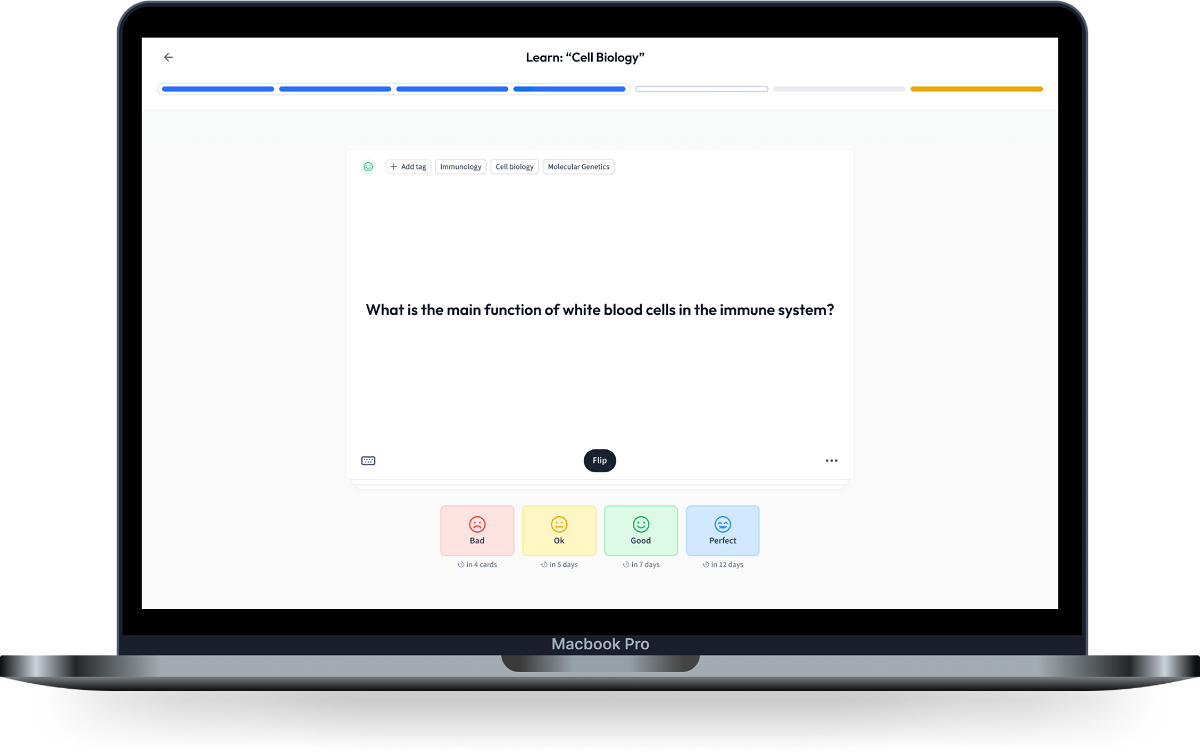
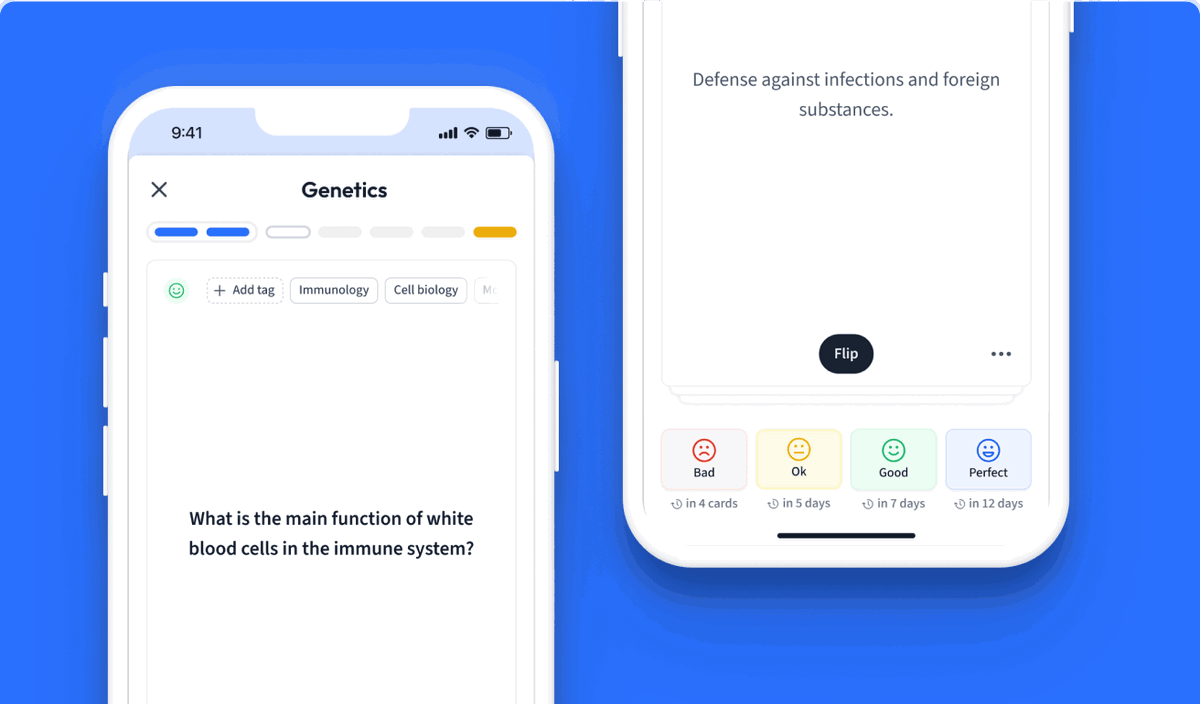
Learn with 15 Critical Section flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Critical Section
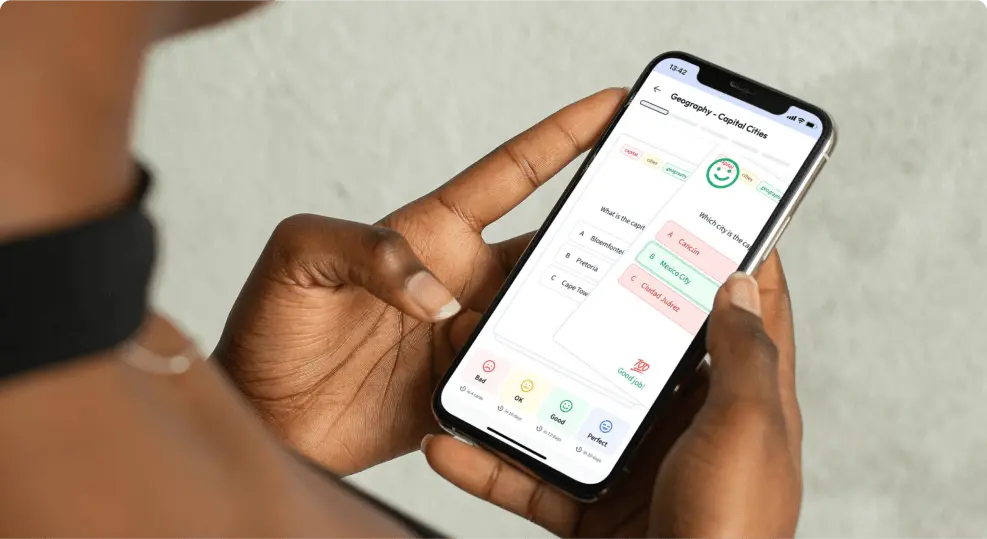
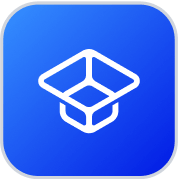
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more