Understanding the Java Map Interface Concept
An essential component of Java programming language is the Java Map Interface. This complex yet useful tool is part of the Java Collections Framework and is a crucial aspect of mastering Java.Defining the Java Map Interface
The Java Map Interface is an object that maps keys to values, thus creating key-value pairs. It operates on a principle that each key can map to at most one value. Often, you'll find it utilised when there is a need to search, update, or navigate elements based on the key. Definition-wise:A Map Interface in Java is defined as an object that maps keys to values, with each key mapped to only one value. It is part of the Java Collections Framework under java.util package.
//Creating map instance Mapmap=new HashMap ();
Key Properties of Java Map Interface
These key properties give the Java Map Interface its unique characteristics:- Null values and null keys: The Map interface allows storing of one null key and multiple null values.
- Ordering: The elements in the Map interface are not synchronized in any order.
- No Duplicates: No two mappings in a map can have the same keys. Each key is associated with a single value.
What is Map Interface in Java
In simple words, a Map Interface in Java is a powerful tool that allows you to store values based on key-value pairs. Its applications are sophisticated, and it often comes in handy whenever efficient search implementation is needed. For instance:If you need to store and retrieve Person objects against unique id, a Map Interface in Java could be extremely beneficial for fast retrievals.
//Creating Map instance Mapmap = new HashMap ();
Constructing a Map Interface in Java is a bit different from other Collection Interfaces. While others only hold a single type of objects, the Map Interface holds two objects - a Key and a Value.
Map Interface Hierarchy in Java
To truly grasp the essence of the Java Map Interface, you need to understand its relationship with other interfaces in the Java Collections Framework. The Map Interface is not purely independent, but rather forms a crucial node within a network of interfaces and classes - forming what's known as the Map Interface Hierarchy.The Structure of Map Interface Hierarchy in Java
The Java Collections Framework is made up of several interfaces and classes, with the Map Interface forming an essential part of this architectural design. At the highest level, you have the Map interface. Below it, there are three sub-interfaces you should take note of: these are SortedMap, NavigableMap, and ConcurrentMap. Further on, you'll come across several implementation classes, including HashMap, TreeMap, and LinkedHashMap among others. Here is a representation of this hierarchical relationship in tabular form: \Map interface |
- SortedMap |
-- NavigableMap |
--- TreeMap |
- HashMap |
-- LinkedHashMap |
- Hashtable |
- WeakHashMap |
- IdentityHashMap |
- ConcurrentHashMap |
How Map Interface links to other interfaces in Java
The Map Interface in Java links to many other Java interfaces. It forms a critical bridge connecting these interfaces and enabling seamless interaction within the entire Java Collections Framework. Specifically, the Map Interface provides the foundational methods used by all the map classes (like HashMap, TreeMap, Hashtable). Without going into too much detail, the aforementioned sub-interfaces - SortedMap, NavigableMap, ConcurrentMap - expand on the Map Interface base. For example, SortedMap keeps the entries sorted according to the keys' natural ordering, or a custom order defined by a Comparator. The NavigableMap extends SortedMap and introduces navigation methods for example to fetch the closest match for a given key. The ConcurrentMap extends the Map and offers concurrent access to large data sets.Detailed Exploration of Map Interface Hierarchy
When it comes to exploring the Map Interface hierarchy in Java in detail, each class and sub-interface contributes to the target function and operation of the entire Map Interface. For instance, the HashMap class implements the Map interface and permits null values and the null key. It is roughly equivalent to Hashtable, but is unsynchronized and permits nulls. The LinkedHashMap, another implementation class, inherits HashMap and implements the Map Interface like its parent class but also maintains a doubly-linked list running through all its entries, thus enabling order-specific tasks. The TreeMap class is a part of the Java Collections Framework and implements NavigableMap and SortedMap along with the Abstract Class and Map Interface. It creates a map stored in a tree structure. These classes and interfaces, along with a few others, come together to form a compact, efficient, and versatile framework that makes the Java Map Interface such a potent tool in Java programming.Map Interface Implementation Classes in Java
There's more to Java Map Interface than meets the eye. Up next, you're going to delve deeper into the Map Interface Implementation classes. These classes, as you'll see, play a crucial role in Java programming, often having the power to dictate how the Map Interface performs under different circumstances.Introduction to Map Interface Implementation classes
Map Interface Implementation classes are directly connected to the Java Map Interface. Each class takes from the core Map Interface, enhancing it with specific attributes and methods as per its purpose. For example, some classes may focus on offering ordered elements, while others may be optimised for quick searching or enable concurrent modifications by different threads. Notably, the Map Interface implementation classes offer the practical structure you'll need to interact with the Map Interface. These classes become templates you can mould and manipulate to ensure the Map Interface fits your needs. Factored into factors like search speed, ordering, duplicate allowance, and null acceptance, the different Map Interface implementation classes available in Java include:- HashMap
- LinkedHashMap
- TreeMap
- EnumMap
- WeakHashMap
- IdentityHashMap
- Hashtable
- Properties
Varieties of Map Interface Implementation Classes in Java
Different Map Interface Implementation classes serve a variety of needs in Java. Here's a closer look at some of the notable differences between these classes: A HashMap class is a hash table based implementation of the Map Interface. It does not order its elements in any way, and it allows one null key and multiple null values. It is not synchronized, implying that multiple threads can access it concurrently. A LinkedHashMap extends HashMap and maintains a doubly-linked list running through all of its entries. This specific feature makes it better suited than HashMap when one needs to iterate the map's contents in the order of their insertion. A TreeMap is a sorted map implementation. It sorts the map's contents based on the keys' natural order – or by a specified comparator during map creation. EnumMap is a specialized map implementation for use with enumeration type keys. EnumMap is ordered by the natural ordering of its keys. WeakHashMap is an implementation of the Map interface that stores only weak references to its keys. This behaviour enables a key-value pair to be garbage-collected when the key is no longer referenced outside of the WeakHashMap. The IdentityHashMap class implements mutable reference equality, instead of the normal object-oriented concept of equals. Hashtable is one of the oldest implementation classes, similar to HashMap but synchronized, meaning that Hashtable is thread-safe and can be shared without any issues between multiple threads.The Properties class, yet another Hashtable subclass, is often used to create a collection of naturally string-keyed property.
Using Map Interface Implementation Classes
Using Map Interface implementation classes is straightforward. You would decide on a suitable class based on your requirements, then sketch out a plan of interacting with the Map Interface using this class.Here is an example of how you could use the HashMap class:
//Creating a HashMap instance MapWith TreeMap, a sorted map, you might proceed differently:hashMap = new HashMap (); //Adding elements hashMap.put(1, "One"); hashMap.put(2, "Two"); //Printing the map System.out.println(hashMap);
//Creating a TreeMap Instance MapRemember, different Map Interface implementation classes offer various features. Therefore, you should always match the chosen class to the problem at hand for a smooth Java programming experience.treeMap = new TreeMap (); //Adding elements treeMap.put(2, "Two"); treeMap.put(1, "One"); //Printing the map System.out.println(treeMap); //Will print {1=One, 2=Two}
Looking into the Map.Entry Interface in Java
An integral component often overlooked in the Java Map Interface pertinence study is the Map.Entry Interface. This component forms a part of the Map Interface, providing a bridge to interact with individual key-value pairs stored in a Map.Map.Entry Interface in Java Explained
The Map.Entry Interface in Java presents you with a gateway to interact with specific elements within a Map, also referred to as 'key-value pairs'. Instead of directly dealing with the Map Interface, you can use the Map.Entry Interface to access, modify, or remove an individual key-value pair in a Map. This interface is a static nested interface, meaning it is defined right inside the Map Interface. This arrangement allows you to invoke it by calling the Map before calling Entry like shown in the syntax below:Map.EntryThe Map.Entry Interface in Java Checks if you're interested in dealing with specific key-value pairs. This way, it offers an opportunity to tap into each pair individually - a feature that can come in handy in various scenarios, like when you need to run specific operations on particular pairs within a map.
Essential Methods of the Java Map.Entry Interface
The Map.Entry interface offers a tonne of methods for you to interact directly with the key-value pairs. However, only two are especially useful, namely the getKey() and getValue() methods. These are the most widely utilised methods of the Map.Entry interface:- getKey(): As suggested by their respective names, the getKey() method allows you to retrieve the key associated with a specific key-value pair within a Map.
- getValue(): getValue(), on the other hand, enables you to fetch the value linked to a particular key-value pair.
Method | Implementation |
getKey() | for (Map.Entry me : map.entrySet()) { System.out.println(me.getKey());} |
getValue() | for (Map.Entry me : map.entrySet()) { System.out.println(me.getValue());} |
Implementing the Map.Entry Interface in Java
In order to utilise the aforementioned methods or any other method, you would first need to implement the Map.Entry interface in Java. What's interesting is that while you can directly interact with the Map.Entry interface, you do not necessarily create its individual instances. Instead, what you do is create a Map, then interact with its entries. By using the method entrySet() from the Map Interface, you can fetch all the entries then loop through the returned set, like shown in the syntax below:for(Map.Entry m : map.entrySet()){ // simulate fetching keys and values System.out.println("Key: "+m.getKey()+" Value: "+m.getValue()); }This method returns a set view of the entries or key-value pairs that the Map contains. The code iterates through this Set using an enhanced for loop. Take note of the m variable - it is an instance of Map.Entry and can, therefore, interact with the pairs directly. To conclude, understanding the Map.Entry Interface will offer you unfolded opportunities to interact with individual elements that a Map Interface contains. Give it a shot, you might find it even more convenient, depending on your unique programming needs in Java.
Map Interface Example in Java
Profound understanding of the Java Map Interface often stems from seeing it in action, interacting with the code. So, let’s dive into a simple, yet illustrative Java Map Interface example.Crafting a Java Map Interface Example
Crafting a Java Map Interface example involves a few fundamental steps. First, you need to select an implementation class - let's consider HashMap for this example. Next, you create a Map instance, and add key-value pairs to it. Let's formulate the steps:- Create a HashMap
- Use the put method to add key-value pairs to the HashMap
- Display the map's contents
import java.util.HashMap; import java.util.Map; class Main{ public static void main(String args[]){ // Creating a HashMap MapThis Java program creates a Map Interface instance (map) of type HashMap. Keys are of Integer type, while the corresponding values are of String type. Five key-value pairs are then added to the map. Upon executing the code, you'll observe that the console prints the entire map contents.map = new HashMap<>(); // Adding elements to the map map.put(1, "One"); map.put(2, "Two"); map.put(3, "Three"); map.put(4, "Four"); map.put(5, "Five"); // Displaying the map System.out.println("The Map is " + map); } }
Analysing the Map Interface Example in Java
Let's dissect the Java Map Interface example we just built. Your output from running that piece of code should look something like this:The Map is {1=One, 2=Two, 3=Three, 4=Four, 5=Five}Notice how the map contents are housed within curly braces. Each key-value pair is separated by equals signs, with commas separating different pairs. The most critical point to note in this example is the put() method's use. This method is central to adding elements to the map. You feed in the key and value as arguments, like so:
map.put(key, value);You'll observe that in output, the order of elements corresponds to the order in which they were inserted in the map. This attribute is specific to LinkedHashMap. With HashMap, as in this example, the order would usually vary, as HashMap doesn't maintain any order. Now, if you'd like to access a specific value within the map, you can use the get() method. This method requires a key input, and it returns the corresponding value.
System.out.println(map.get(3)); //Will print "Three"Subsequently, if the goal is to remove an element from the map, turn to the remove() method. Feed it the key of the element you'd like to see ousted.
map.remove(4); //Removes the element with key 4This detailed analysis provides a granular view of how to interact with the Java Map Interface. As you venture further into your Java programming journey, you'll uncover many more intriguing features and functions of this flexible interface.
Map Interface in Java Collections
In the Java Collections framework, the Map interface emerges as an essential component. Often overlooked by beginners but cherished by experts, you'll find that the Map interface is efficient, flexible, and indeed handy when dealing with data mapping. A map, put simply, is an object that holds key-value pairs, also known as associations. It allows you to map unique keys to their respective values, a duality that's fundamental to the efficient storage and retrieval of data.Implementing Map Interface in Java Collections
To use a Map interface first, envisage an empty container ready to house unique key-value pairs. Remember, each key in this container is unique and maps to a specific value. The combination of a unique key and its value forms an association or an entry – the essential building block in a map. Delving deeper into the Map interface, there aren't any direct implementations. Yet, specific classes in the Java Collection Framework execute it indirectly. These classes provide practical methods that let you interact with a map. They include HashMap, LinkedHashMap, and TreeMap. Here, both HashMap and TreeMap are members of the Java Collections Framework. Let's take a journey into the world of each of these classes:- HashMap: This is a Map-based collection class that is used for storing Key and value pairs, it is denoted as HashMap
or HashMap . This class makes no guarantees concerning the ordering of the map, and it allows one null key and multiple null values. - LinkedHashMap: The LinkedHashMap is just like HashMap with an additional feature of maintaining an order of elements inserted into it. So it has the features of both HashMap and LinkedList, thus offering the functionality of a mapping and maintaining this mapped order.
- TreeMap: The TreeMap in Java is used to implement the Map interface and NavigableMap along with the Abstract Class. It creates a collection that uses a tree for storage. Moreover, the ordering of its elements is maintained by a set using their natural ordering, whether or not an explicit comparator is provided.
Working with Map Interface within Java Collections
Now let’s take a closer look at wielding the power of Map interfaces in Java Collections effectively. Firstly, crafting a map requires selecting an appropriate implementation class as per your requirements. If you need naturally sorted pairs, look to TreeMap. If order preservation is top of your list,i.e., you need the elements to follow their input order, you'd be better served by LinkedHashMap. On the other hand, if execution speed is the overarching priority,then HashMap, with its mighty hashing technique, would come to your rescue. Once you've chosen your implementation class, the journey to creating, modifying, and interrogating a map now commences. Begin by creating a map instance, using the following code snippet:MapHaving crafted the map, next on the agenda is populating it. Make use of the 'put(Key, Value)' method, observing that the datatype of the Key and Value should correspond with the map's original declaration:mapName = new Implementation_class<>(); //Example Map exampleMap = new HashMap<>();
exampleMap.put(1, "First Entry"); exampleMap.put(2, "Second Entry"); //and so onWhile the Map interface may seem simple on the surface, remember that it forms the backbone of your data structure. Therefore, it’s prudent to master its handling. Explore all the methods provided by classes implementing the Map interface and endeavour to apply them in different use-cases. This thorough understanding of the Map interface will enable your concurrent retrieval and modification of data, thereby supercharging your programming efficiency in Java.
Deep Dive into the Methods of Map Interface in Java
Unmasking the power of the Map interface in Java is a matter of understanding its core methods. These methods can be labelled as the toolkit that assists you in efficiently interacting with mapped data. By mastering these methods, you will be well equipped to handle various operations such as adding or removing elements, accessing and modifying values, and iterating over collection objects.Important Methods of Map Interface in Java
Here's a selection of some of the crucial methods provided by the Java Map interface:void clear() | Clears the map, eliminating all of the mappings from it. |
boolean containsKey(Object key) | Probes if the map contains a specific key. |
boolean containsValue(Object value) | Inspects if the map contains a specific value. |
boolean equals(Object o) | Assesses two maps for equality. |
boolean isEmpty() | Audit if the map contains no key-value mappings. |
Set entrySet() | Fetches a set view of the mappings. |
Understanding the Use of Methods in Map Interface Java
To effectively harness these methods, it's critical to understand how they function. The clear() method, as the name suggests, vacuums the map of its contents. When invoked, it leaves the map empty, thus:map.clear();Should you question if the map contains a certain key or value, you have your veritable detectives at the ready – the containsKey(Object key) and containsValue(Object value) methods! Use these methods to investigate the presence of a key or value, deploying syntax such as:
// For Key map.containsKey("One"); // For Value map.containsValue(1);Checking two maps for equality is facilitated by the equals(Object o) method. Remember, it checks for equality, not identity. Two maps can be equal if they contain the same mappings, even though they are separate objects. The usage is as follows:
map1.equals(map2);If you're curious about whether a map is devoid of any mappings, turn to the isEmpty() method. It will return 'true' if the map is bereft of entries, and 'false' otherwise:
map.isEmpty();Lastly, the entrySet() method provides an overview of all the entries in the map. Suppose you'd like to scan through all the key-value pairs (entries). It becomes pivotal to your quest:
map.entrySet();
Practical Application of Methods of Map Interface in Java
Now, having grasped the theoretical understanding of these methods, let's apply them in a practical scenario using examples. Let's consider a map of integers and their English spelling stored inexampleMap
.
//Creating a Map MapIn the example given, you trialed several methods and their applications. Indulge further into operating with the Map interface in Java, unravel more methods, and observe how they behave in different scenarios. This exercise will not only solidify your foundational understanding of the Map interface but also gear you up for complex data operations and manipulations.exampleMap = new HashMap<>(); //Initializing the Map exampleMap.put(1, "One"); exampleMap.put(2, "Two"); exampleMap.put(3, "Three"); //Checking if map contains the Integer key 1 boolean flag = exampleMap.containsKey(1); //Returns true //Checking if map contains the value "Four" flag = exampleMap.containsValue("Four"); //Returns false //Displaying entry set Set > entries = exampleMap.entrySet();
Java Map Interface - Key takeaways
- The Java Map Interface is a potent tool in Java programming, forming a compact, efficient, and versatile framework.
- The Map Interface Implementation classes in Java include HashMap, LinkedHashMap, TreeMap, EnumMap, WeakHashMap, IdentityHashMap, Hashtable, and Properties.
- The Map.Entry Interface in Java is a component of the Map Interface, allowing interaction with individual key-value pairs in a Map. It offers getKey() and getValue() methods to retrieve the key and value of a specific pair.
- Map Interface in Java allows the creation of Map instances, addition of key-value pairs and uses the put() method to add elements to the map. Individual values can be accessed using the get() method, and elements can be removed using the remove() method.
- In the Java Collections framework, the Map interface is an essential component, effectively storing and retrieving data through unique key-value pairs. This interface is implemented by classes such as HashMap, LinkedHashMap, and TreeMap.
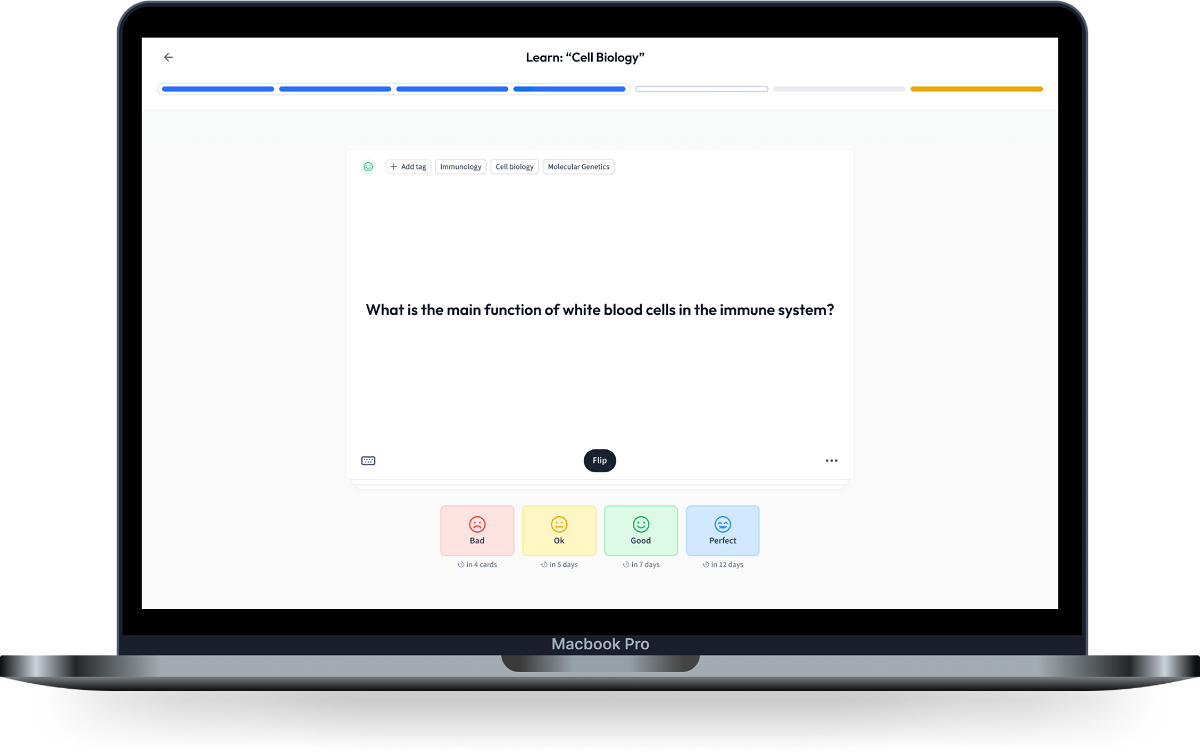
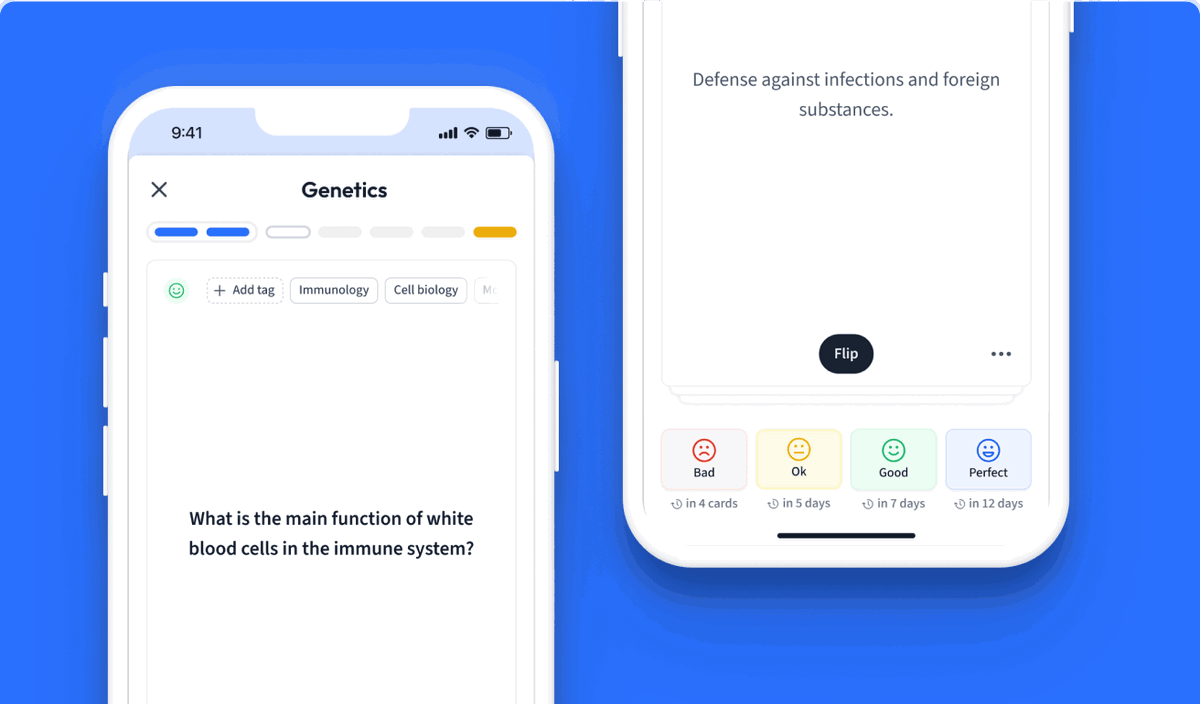
Learn with 14 Java Map Interface flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Java Map Interface
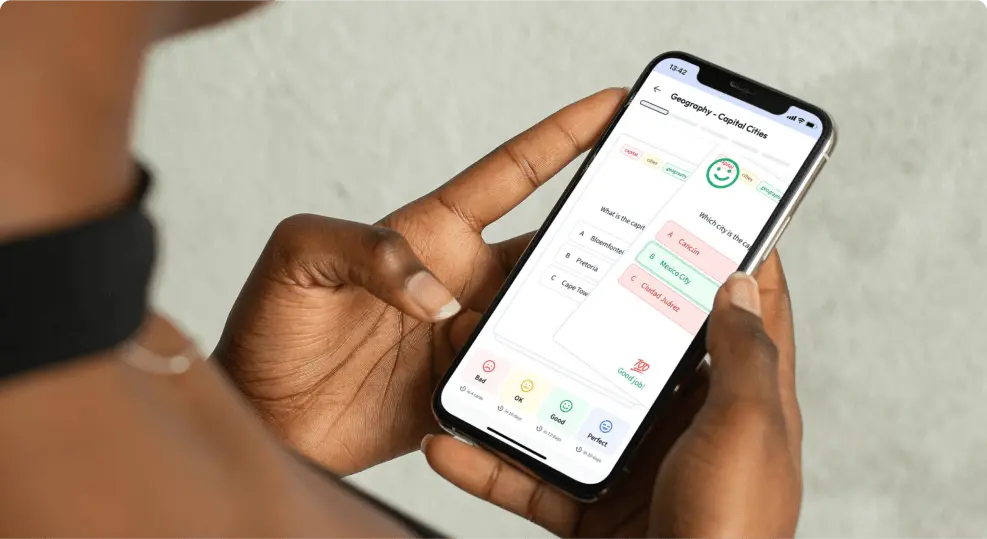
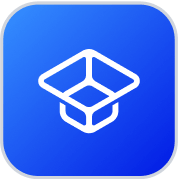
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more