Unravelling the Javascript For Loop
For you to become proficient in programming, especially in using JavaScript, understanding loops is crucial. Among the various types of loops in JavaScript, 'For Loop' is a common one and a key control structure, often used to handle repetitive tasks more efficiently. This makes getting to grips with the structure and functioning of Javascript For Loop significantly essential.Breaking Down the For Loop Syntax in Javascript
The basic syntax of a Javascript For Loop consists of three parts: initialisation, condition, and update. These can be defined as follows:Initialisation: This is where you initialise the loop counter to a starting value.
Condition: The loop continues to run until this condition is false.
Update: This part increases or decreases the loop counter.
for(initialisation; condition; update){ // code block to be executed }Let's consider an example:
for(let i=0; i < 5; i++){ console.log(i); }In this case, the loop is initialised by 'let i=0'. The loop will continue as long as 'i' is less than 5 (condition). After each iteration, 'i' will increase by 1 (update). This loop will output the numbers 0 to 4 in console.
Understanding the Use of Javascript For Loop in Computer Programming
Being proficient in usage of the Javascript For Loop enriches your programming repertoire as it aids in:
- Traversing through an array or list
- Repeating a block of code a specified number of times
- Iterating over the properties of an object (with Object.keys() or Object.entries())
let students = ['Alex', 'Maria', 'John', 'Sophia']; for(let i=0; i < students.length; i++){ console.log(students[i]); }In this case, the For Loop makes code cleaner and easier to manage, particularly when dealing with large data sets.
Familiarising with Loops in JavaScript
From the computer science perspective, loops facilitate repeated execution of a block of code. Loop concepts in JavaScript are not unique to the language and mirror other languages such as C++ or Java. Apart from For Loop, there are other types too:
- While loop: It's employed when the number of iterations is unknown. It executes until the condition becomes false.
- Do-While loop: Similar to a While loop but it processes the condition after executing the code block. Thus, the block gets executed at least once irrespective of the condition.
- ForEach loop: Specifically designed for iterating over arrays, it requires a callback function which is executed for each element.
Applying Javascript For Loop Effectively
When applied correctly, the Javascript For Loop can serve as a powerful tool in your programming arsenal, allowing you to simplify repetitive tasks and enhance code readability and maintainability.Technique for Using For Loop in Javascript
In order to utilise the Javascript For Loop effectively, it is essential to understand its working and establish a solid strategy for implementation. At the heart of the For Loop are three major components:Initialisation - Setting up a variable to act as a counter or tracking marker
Condition - Defining a condition which, as long as it remains true, allows the loop to continue
Update - Altering the counter or tracking marker in some way after each loop iteration
for (var i = 0; i < 5; i++) { //Code to be repeatedly executed goes here }In this example, 'var i = 0' is the initialisation, 'i < 5' is the condition, and 'i++' is the update. Understanding these three components is crucial to harnessing the full potential of the For Loop. For example, by manipulating the counter during the update stage, you can control the frequency of loop iterations, choosing to skip iterations or even run them in reverse order.
Mastering Looping Structures in JavaScript
Becoming skilled in using loops requires more than just a knowledge of For Loops. It involves grasping all loop varieties available in JavaScript and knowing when to use each type effectively. Besides For Loop, Javascript also offers While, Do While, and ForEach loops. While Loop usually runs while a particular condition remains true. Do While Loop, on the other hand, ensures the code block is executed at least once before checking the condition. ForEach Loop is specifically designed for iterating over arrays.Proper Usage of Array for loop in Javascript
One of the most common uses of the For Loop in Javascript is in array manipulation. By leveraging the length property of an array with the For Loop, you can iterate over each element in the array with ease. Consider the following example:var fruits = ['Apple', 'Banana', 'Cherry']; for (var i = 0; i < fruits.length; i++) { console.log(fruits[i]); }Here, 'fruits.length' represents the total number of elements in the array (three in this case). The loop will iterate over each element of the array, outputting the names of the fruits to the console.
Streamlining Programming with For loop Syntax Javascript
Using For Loop syntax can considerably streamline your Javascript code. It leads to more efficient, readable, and maintainable code, especially when dealing with repetitive tasks. Understanding its syntax allows for greater flexibility and adaptability in writing your code. You can create complex nested loops, iterate over multidimensional arrays, and even control the order and pace of iteration depending on the specifics of a project. The key to mastering For Loop syntax lies in practice and experimentation - try crafting loops with different initialisation, condition, and update parameters to see the results.Working with Real-Life Examples of Javascript For Loop
Contextualising programming concepts, particularly like Javascript For Loop, through real-life examples and case studies facilitate a deeper understanding. Such examination helps you relate theoretical knowledge with practical applications, further enhancing your programming skills. Especially for complex constructs like the For Loop, the importance of examples cannot be understated.Practical JavaScript Application: Example of For loop in Javascript
Let's review a practical example where Javascript For Loop is easily applied: creating alphanumeric codes. Often, such codes are used in generating unique references, identifiers, or even simple captcha systems. Let's assume that such an alphanumeric string requires digits and alphabets. Here's how For Loop can be applied:let alphaNumCode = ''; let possibleCharacters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789'; for (let i = 0; i < 10; i++) { alphaNumCode += possibleCharacters.charAt(Math.floor(Math.random() * possibleCharacters.length)); } console.log(alphaNumCode);In this code snippet, a For Loop is used to generate a random ten-character long alphanumeric string—this instance, particularly showcases the power and versatility of For Loop to suit specific needs.
The charAt() function returns a character at a specific index in a string, while Math.random() generates a random number between 0 and 1. Math.floor() then rounds down to the nearest whole number.
Implementing Array for loop in Javascript: Case Study
Let's consider the case where you need to process a list of students and their grades stored in an array. The task at hand might be to grade them according to some criteria. The criteria can be defined as functions that take the grade as an argument and return the result.let students = ['John', 'Maria', 'Alex', 'Sophia']; let grades = [82, 89, 91, 76]; let gradeLetters = []; function getGradeLetter(grade) { if (grade >= 90) { return 'A'; } else if (grade >= 80) { return 'B'; } else if (grade >= 70) { return 'C'; } else { return 'F'; } } for (let i = 0; i < students.length; i++) { gradeLetters[i] = getGradeLetter(grades[i]); console.log(students[i] + " : " + gradeLetters[i]); }This sequence demonstrates both the usage of array processing with For Loop and the combination of conditional logic within the loop. Each student's grade is converted to a grade letter using criteria defined in getGradeLetter function.
Hands-On with For Loop in JavaScript: A Practical Approach
For Loop, as evident from the examples above, aids in achieving a variety of tasks. However, to truly master its powerful implementation, hands-on approach with examples is invaluable. Consider the task of an HTML table creation based on arrays containing the data. For Loop can be applied in creating dynamic HTML based on data sets. For instance:var headers = ['Name', 'Age', 'City']; var data = [ ['John', 30, 'New York'], ['Maria', 28, 'Los Angeles'], ['Alex', 35, 'London'] ]; var html = '
' + headers[i] + ' | '; } html += '
---|
' + data[i][j] + ' | '; } html += '
Javascript For Loop - Key takeaways
- 'For Loop' is a common and key control structure in JavaScript, often used to handle repetitive tasks more efficiently.
- The basic syntax of a Javascript For Loop consists of three parts: initialisation (initialise the loop counter to a starting value), condition (the loop continues to run until this condition is false), and update (increases or decreases the loop counter).
- Practically, Javascript For Loop can be used for traversing through an array or list, repeating a block of code a specified number of times, and iterating over the properties of an object.
- Javascript provides other looping structures such as While loop, Do-While loop, and ForEach loop for different conditions and requirements.
- Instances of efficient For Loop usage include generating alphanumeric codes and for creating dynamic HTML based on data sets.
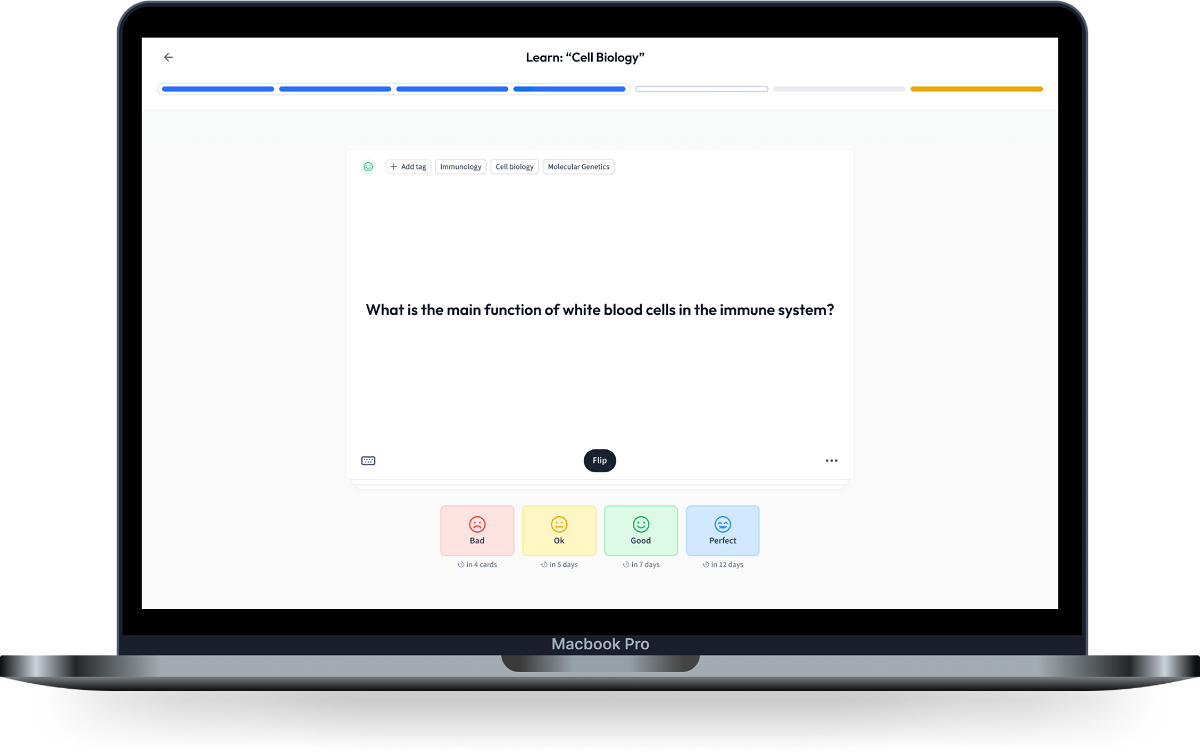
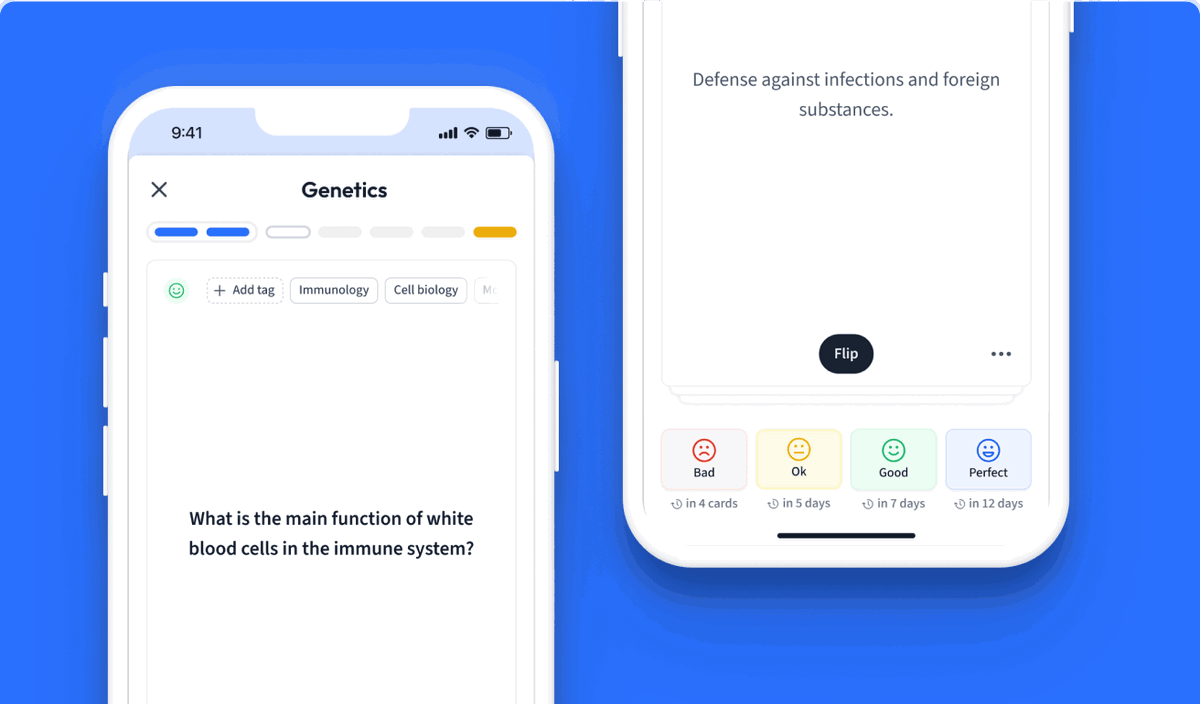
Learn with 12 Javascript For Loop flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript For Loop
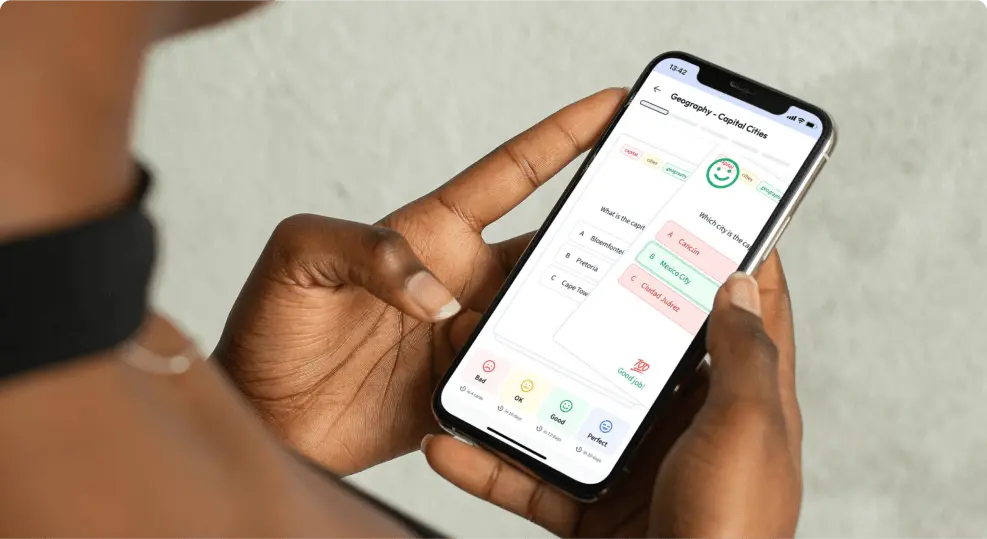
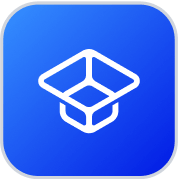
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more