Understanding Javascript Object Creation
In the realm of computer science, specifically in web development, Javascript is one indispensable language that you must get a handle on. One of the key concepts you need to understand in Javascript is Object Creation. You might be wondering why Object Creation is so vital, and how to correctly implement it, which is why we've created this web resource for you!
The Basics of Javascript Object Creation
At its core, Javascript is an object-oriented programming language. This means that almost everything you interact with in Javascript is an object. But what exactly is an object in Javascript?
An object is a standalone entity with properties and type. It is like an item lying around in the real world, like a pen, a bag, a car; each has properties like color, type, weight, and they perform different functions.
Importance of Object Creation in Javascript
Javascript Objects are paramount for several reasons:
- They help to structure and organize your code, breaking it down into manageable pieces.
- They model real-world things and systems, which make your code more intuitive.
- They allow for the reusability of code, thus saving time and improving efficiency.
Correct Syntax for Object Creation in Javascript
The basic syntax for creating an object in Javascript is via the object literal method, as shown in the example below:
var student = { firstName: "John", lastName: "Doe", age: 22 };
In this syntax, 'var' is a keyword that initializes a new variable, 'student' is the variable name, and everything within the curly braces { } is the object that you have created.
Javascript Dynamic Object Creation: A Closer Look
Moving further, Javascript Object Creation has a really interesting feature - dynamic object creation is totally possible, and can be incredibly useful.
Defining Javascript Dynamic Object Creation
Dynamic object creation in Javascript refers to the ability to create an object at runtime, assign properties to it, and even change these properties dynamically during the execution of the program.
Interesting Fact: Javascript's dynamic nature is derived from its roots in the ECMAScript language, which was designed to be used in a variety of host environments, not just web browsers. This flexibility has continued into Javascript and is a large part of what makes it such a powerful web development tool.
How to Implement Dynamic Object Creation in Javascript
You can create dynamic objects in Javascript by using the new Object() constructor or by using the bracket syntax {}. Here's a simple way to do it:
var vehicle = new Object(); vehicle.type = "Car"; vehicle.brand = "Toyota";
This will create a new object 'vehicle' with two properties, 'type' and 'brand'. Remember, in Javascript, you can always add more properties to an object later or modify existing ones, allowing for great flexibility in how your code functions.
Digging into Javascript Object Creation Patterns
The creation of objects in Javascript can be accomplished in numerous ways, each corresponding to a specific pattern. Understanding these patterns is vital to writing efficient, maintainable code. While myriad patterns exist to create objects in Javascript, here, you'll focus on the most commonly used ones. Also, you'll explore some real-world applications of these patterns.
Defining Javascript Object Creation Patterns
In Javascript, an object creation pattern is a specific way or method of creating objects. Each pattern is characterized by its syntax and the way it handles the creation of properties, functionalities, and inter-object relationships.
Commonly Used Javascript Object Creation Patterns
Despite the various ways you can create objects in Javascript, there are a few commonly used patterns mainly because of their flexibility and ease of use. These include:
- Factory Pattern
- Constructor Pattern
- Prototype Pattern
- Dynamic Prototype Pattern
The Factory Pattern creates an object and returns it, but doesn't explicitly use the new keyword. The Constructor Pattern combines object creation and initialization into a constructor function. The Prototype Pattern leverages shared properties through an object prototype. The Dynamic Prototype Pattern combines the Constructor and Prototype Patterns.
function createObject() { var obj = new Object(); // define properties and methods return obj; }
function ObjectName() { // define properties and methods } var obj = new ObjectName();
function ObjectName() { } ObjectName.prototype.someMethod = function() {}; //example method var obj = new ObjectName();
function ObjectName() { // properties if (typeof this.someMethod != 'function') { ObjectName.prototype.someMethod = function() {}; //example method } } var obj = new ObjectName();
Real World Applications of Javascript Object Creation Patterns
Considering Javascript's prevalence in web development, understanding object creation patterns is crucial. This knowledge allows customization and optimization of your code in various ways based on the project's needs. Object creation patterns find application in a myriad of scenarios including:
- Creating multiple objects sharing a similar structure
- Imitating other class-based languages
- Modularising code into maintainable, independent components
- Implementing complex design patterns like MVC (Model-View-Controller)
Javascript Object Creation Examples
T>So you've now comprehended the basic Javascript object creation patterns. Yet, there's no better way to solidify this knowledge than to practice. Programming is a practical field after all. Now, let's delve into some examples.
Exploring Examples of Javascript Object Creation Patterns
Factory Pattern
function createObject(name, age) { var obj = new Object(); obj.name = name; obj.age = age; return obj; } var student = createObject(rty, 22);
Constructor Pattern
function Person(name, age) { this.name = name; this.age = age; } var student = new Person("John", 22);
Prototype Pattern
function Person() {}; Person.prototype.name = "John"; Person.prototype.age = 22; var student = new Person();
Dynamic Pattern
function Person(name, age) { this.name = name; this.age = age; if (typeof this.sayName != "function") { Person.prototype.sayName = function() { return this.name; } } } var student = new Person("John", 22);
Step-by-Step Guide to Javascript Object Creation Practices
Let's break down the general steps to creating an object in JavaScript:
- Choose an object creation pattern that fits your needs.
- If necessary, construct an object creation function according to the chosen pattern.
- Invoke the function to create your new object.
- Add or modify properties and methods as needed.
Remember, each pattern has its strengths and weaknesses, but understanding them and knowing when to use each will enhance your Javascript mastery!
Exploring JSON Object Creation in Javascript
In the world of modern web applications, data plays a pivotal role. Hence, it becomes essential to understand data handling techniques and how to model data properly. This is where JSON, short for JavaScript Object Notation, comes into play. Chances are, you have come across this term if you've dabbled in Javascript or even looked at a modern API or web service.
Introduction to JSON Object Creation in Javascript
JSON is a data formatting standard widely accepted and used in web applications around the globe. It is language independent and is easy for humans to read and write, but also easy for machines to parse and generate. This lightweight data-interchange format is mainly based on a subset of the JavaScript Programming Language. JSON is crucial and highly beneficial as it enables the conversion and storage of complex Javascript objects into strings, making it possible to transmit these objects over the network or store them in a database.
Creating JSON objects in Javascript is quite straightforward, and Javascript provides in-built methods to turn your Javascript objects and arrays into JSON strings and vice versa. Two primary methods help us with these tasks: JSON.stringify() and JSON.parse().
Decoding the JSON Object Creation process in Javascript
Let's look deeper into these two methods:
- JSON.stringify(): This method takes a Javascript object or value and converts it into a JSON string. This method is great when you need to turn your Javascript objects into JSON prior to sending them over the network or storing them in a file or a database.
An illustrative example of JSON.stringify() is:
var student = { name: "John", age: 22, department: "Computer Science" }; var studentJSON = JSON.stringify(student);
Here, the student object is transformed into the studentJSON string via JSON.stringify().
- JSON.parse(): If JSON.stringify() helps convert Javascript objects into JSON strings, JSON.parse() does the opposite. This method parses a JSON string and creates a Javascript object or value from it. Useful when you retrieve data from a server or a local file, and it needs to be converted from a JSON string into a format Javascript can work with.
A typical example of JSON.parse() is:
var studentJSON = '{"name": "John", "age": 22, "department": "Computer Science"}'; var student = JSON.parse(studentJSON);
In this example, the JSON.parse() method is called to parse the studentJSON string and produce the student object, which you can then utilise in your code.
Practical Insights to JSON Object Creation in Javascript
Besides converting Javascript objects to JSON, it's possible to include only certain properties of an object during JSON string creation. JSON.stringify() supports a filtering mechanism that selects only certain properties for inclusion when turning your Javascript objects into JSON.
Here's how you do it:
var student = { name: "John", age: 22, department: "Computer Science" }; var studentJSON = JSON.stringify(student, ["name", "age"]);
In this example, JSON.stringify() is provided a second argument — an array listing property names to be included in the JSON string. Here, only 'name' and 'age' are included, while 'department' is left out.
Learning to create JSON objects in Javascript and understanding the processes of converting JSON into Javascript objects is critical for managing and manipulating data efficiently in your applications.
Theoretical Concepts of Javascript Object Creation
The cornerstone of Javascript, and indeed any object-oriented programming language, lies in understanding the concept of objects - their creation, manipulation, and destruction. Javascript avails variation in object creation, which will be unwrapped and highlighted in this piece.
Understanding the Theory behind Javascript Object Creation
At the root, an object in Javascript is simply a collection of key-value pairs, the so-called properties. In theory, when we talk about the creation of objects in Javascript, we are referring to creating such collections of properties, and optionally, methods - actions that can be performed on or by the objects.
Object creation patterns in Javascript play into the broader concept of 'prototypes'. In Javascript, all objects link to a prototype object from which they can inherit properties. You can think of this prototype object as a sort of 'template object', and every new object created with a certain constructor function (using the 'new' keyword) will automatically link to the constructor's prototype. And this inherent nature becomes highly relevant when discussing the Prototype pattern of object creation in Javascript.
Further, Javascript provides a first-class function, meaning that functions in Javascript are objects. This enlightening concept shapes the Constructor pattern of object creation, where each function object contains a 'prototype' property serving as the prototype for all objects created with that function when used as a constructor.
Unwrapping the Theoretical Concepts of Javascript Object Creation
Amongst the common ways to create objects in Javascript, the 'literal notation' is the simplest and most straightforward method. In such a case, you define an object using a pair of curly braces {}. A more sophisticated approach is through a constructor function, where the object is defined with a function and instantiated using the 'new' keyword.
In the Factory pattern, an object is made with a factory function that returns the object. And in the Prototype pattern, objects are cloned from a prototype, while in the Constructor/Prototype pattern (or 'classical' inheritance), a mix of constructors and prototypes are used.
Apart from these theoretical aspects, a more nuanced concept - 'closures', also manifest into the theoretical aspects of object creation. A closure in Javascript happens when an inner function refers to the variables and parameters of an outer function even after the outer function has completed its execution. This behaviour intricately interacts when objects are created using factories or constructors.
Grasping these theoretical documentation of Javascript object creation aids in apprehending the behaviour of objects when they interact with other parts of a Javascript program.
Bridging Theory and Practice in Javascript Object Creation
Understanding the underlying philosophy is rewarding, but can the theories be mapped to coding practices? Certainly! In fact, real-world programming in Javascript is a playground where these theories manifest in tangible practices.
An understanding of constructor functions allows for the efficient instantiation of multiple objects with the same properties and methods, which is excellent for code efficiency. For instance, if you're creating an e-commerce site, and you had to create hundreds of 'product' objects, understanding how to make constructor functions would save considerable time and effort.
Realising the Prototype pattern and its relationship to inheritance is the key to performing object-oriented programming (OOP) in Javascript. Often, when code bases get larger and more complicated, organising the codebase using OOP principles can lead to more manageable, readable code.
The Factory pattern comes in handy when you want to create an object, but you don't care about the type of that object. A common use case might be a log factory that generates different objects depending on where the logs are being sent. Plugging theories into practice, and thus alleviating the code quality becomes the mission.
To cement these theoretical aspects, consistent practice and structured coding endeavours are advocated.
Javascript Object Creation - Key takeaways
- Javascript objects help organize code, model real-world systems, and allow for reuse of code.
- Basic syntax for object creation in Javascript involves using the object literal method.
- Javascript allows for dynamic object creation, allowing objects to be created at runtime, and properties to be assigned or changed dynamically.
- Javascript Object Creation patterns include the Factory Pattern, Constructor Pattern, Prototype Pattern, and Dynamic Prototype Pattern.
- JSON Object Creation is a data formatting standard used for converting and storing complex Javascript objects into strings.
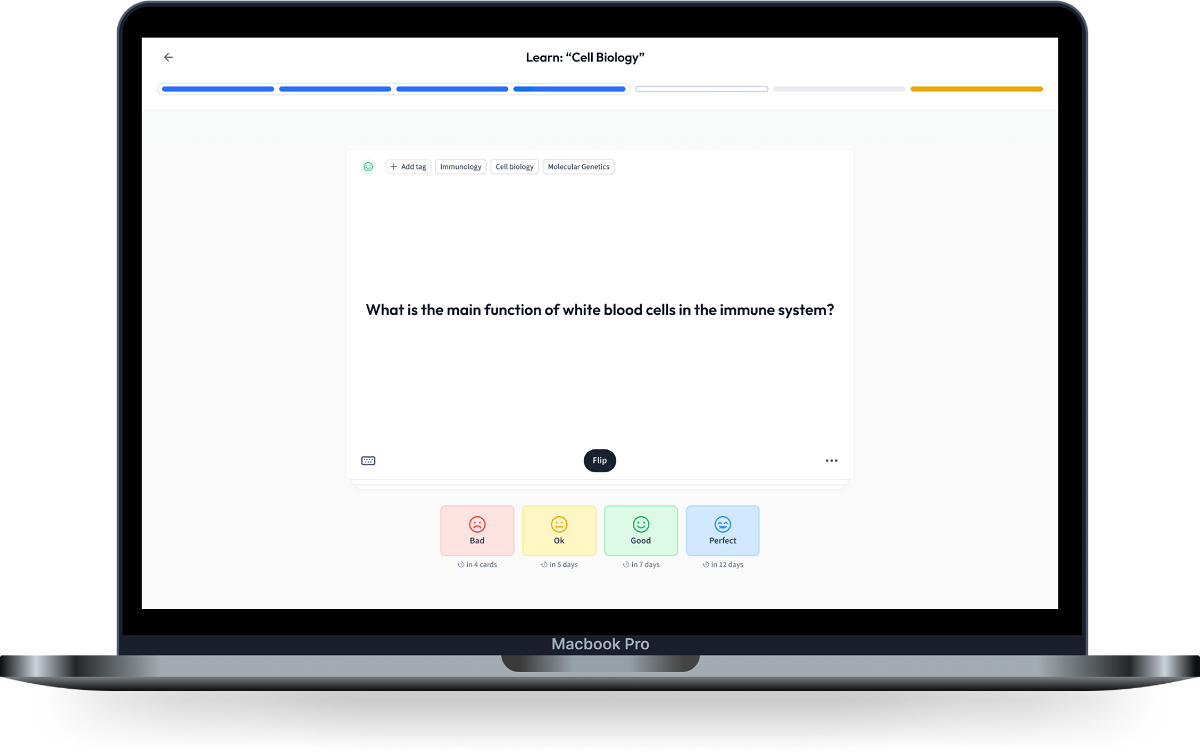
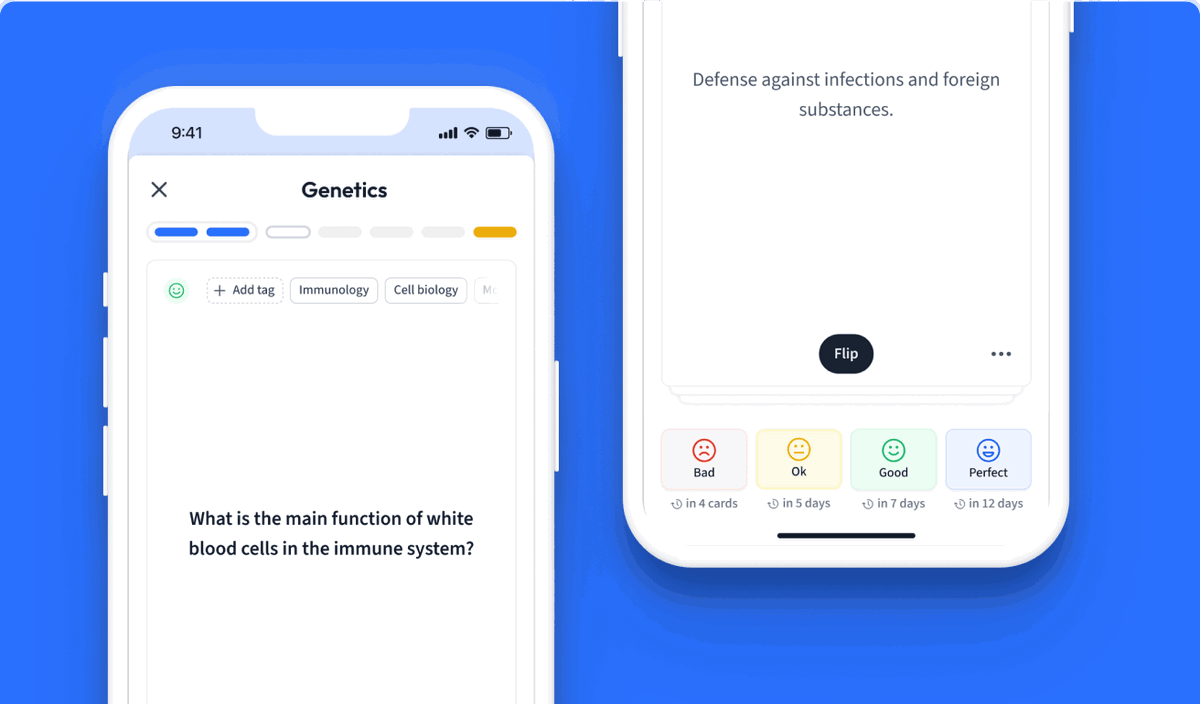
Learn with 12 Javascript Object Creation flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript Object Creation
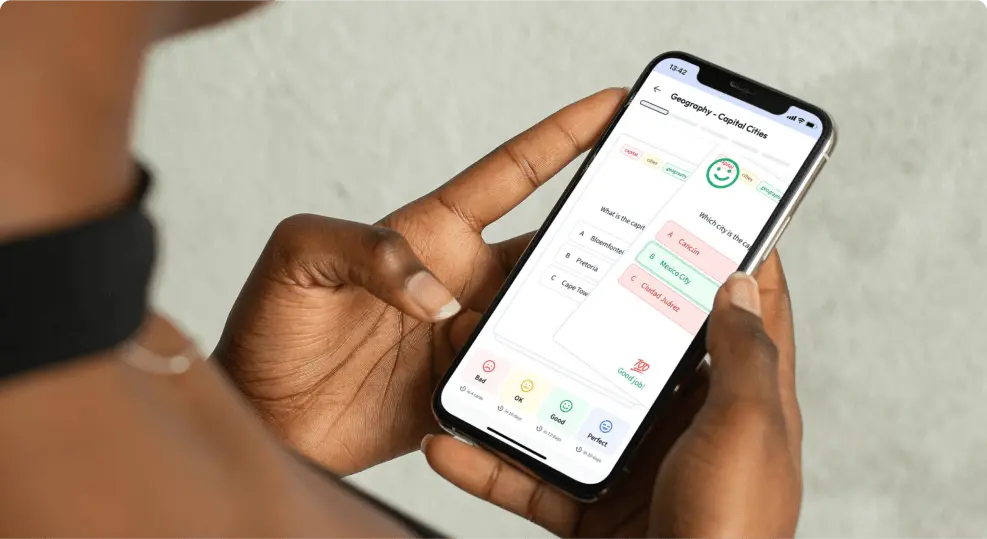
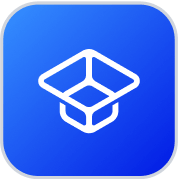
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more