Basics of C Functions Explained
In the realm of computer science, particularly in the C programming language, functions play a crucial role in increasing the efficiency and reusability of code. A C function is a block of code with a specific task that can perform an operation, such as calculating a value or processing data. Functions in C can be user-defined or built-in, owing to the versatility of the language.
A C function is defined as a named sequence of statements that takes a set of input values, processes them, and returns an output value.
Function declaration and definition
To effectively use a function in C, it must be both declared and defined. The function declaration provides information about the function's name, return type, and parameters (if any), while the function definition specifies the actual code that executes when the function is called.
The general syntax for declaring and defining a function in C is as follows:
return_type function_name(parameter_type parameter_name, ...); return_type function_name(parameter_type parameter_name, ...) { // function body // ... return value; }For instance, consider this example of a function that adds two integers and returns the result:
int add(int a, int b); int add(int a, int b) { int result; result = a + b; return result; }Calling a function in C
Once a function is declared and defined, it can be called (or invoked) anywhere within the program, as long as it is called after its declaration. A function's parameters and arguments, if any, must be specified within parentheses during the call. The general syntax for calling a function in C is:
function_name(arguments);For example, using the previously defined 'add' function:
#includeFunction arguments and return values
Functions in C may have multiple input arguments or none at all, depending on their requirements. These arguments are declared within the parentheses following the function's name, with their data types and names separated by commas. C supports several argument-passing techniques, with the most common being 'pass by value.' In this method, a copy of the argument's value is passed to the function, which means any changes made to the value within the function do not persist outside the function.
Consider the following example of a swapping function that exchanges the values of two integers:
void swap(int x, int y) { int temp; temp = x; x = y; y = temp; }As for return values, a C function typically returns a single value to its caller. This value is determined by the function's return type - such as int, float, or void (indicating no value is returned) - and the use of the 'return' statement followed by the value or variable to be returned. However, it is possible for a function to return multiple values using pointers or arrays.
Remember that a function should always have a clearly defined purpose and task. Creating too many unrelated tasks within one function can make the code more complex and difficult to understand, so it's better to break down complex tasks into multiple smaller, simpler functions for improved readability and maintenance.
C Functions Examples
In C programming, static functions have a unique role, as they are local to the source file in which they are defined. This means that a static function can only be called from within the same source file, and their scope is not visible to other files. They are primarily used when a function needs to be confined to a specific module or file, ensuring that naming conflicts and unintended calls are avoided.
Declaring and defining static functions
To declare and define a static function in C, simply use the 'static' keyword before the function's return type, as shown in the following general syntax:
static return_type function_name(parameter_type parameter_name, ...); static return_type function_name(parameter_type parameter_name, ...) { // function body // ... return value; }
For instance, a static function to compute the square of an integer might look like this:
static int square(int x); static int square(int x) { return x * x; }Attempting to call this function from another file will result in a compilation error, as its scope is limited to the file in which it is defined.
Advantages and use cases of static functions
Static functions in C offer several benefits and are suitable for specific use cases:
- Encapsulation: Static functions provide a level of encapsulation, as they are not accessible from other files. This gives the programmer control over where the function can be used, and helps to avoid naming conflicts and unintended calls.
- Code clarity: Using static functions within a module or file helps to organize code, as it separates functionality related to that specific module from the global scope and exposes only the necessary interfaces.
- Resource management: In some cases, static functions can reduce overhead and make resource allocation and de-allocation more efficient for a module or file.
When working on large-scale software projects with multiple modules or files, leveraging the advantages of static functions can lead to more maintainable code, improved resource management, and better overall software design practices.
Implementing power function in C
In this section, we will explore two methods to implement a power function in C, which calculates the result of raising a number (base) to a specified power (exponent). The two methods we will discuss are the recursive method and the iterative method.
Recursive method for power function
The recursive method for implementing the power function takes advantage of the repeated multiplication involved in exponentiation. The general idea is to repeatedly multiply the base by itself, decrementing the exponent until it reaches 0, at which point the process terminates. The process can be defined recursively as follows:
\[ power(base, exponent) = \begin{cases} 1 & \text{if }\, exponent = 0 \\ base × power(base, exponent - 1) & \text{if }\, exponent > 0 \end{cases} \]
A C implementation of the recursive power function can be seen below:
int power(int base, int exponent) { if (exponent == 0) { return 1; } else { return base * power(base, exponent - 1); } }
However, it is important to note that this recursive method can have an impact on performance due to the overhead associated with recursion, particularly for large exponent values.
Iterative method for power function
An alternative, more efficient way to implement the power function is to use an iterative method. Here, a loop is used to perform multiplication repeatedly, updating the result for each iteration until the exponent is exhausted. An example of an iterative C implementation for the power function can be seen below:
int power(int base, int exponent) { int result = 1; while (exponent > 0) { result *= base; exponent--; } return result; }
This iterative method improves performance by avoiding the overhead associated with recursion, making it a more efficient approach for calculating exponentiation in C.
As a quick illustration, here is how both the recursive and iterative power functions can be used to calculate 3 raised to the power of 4:
#includeRelationship between functions and pointers
In C programming, functions and pointers can be combined to create powerful programs by increasing the flexibility of how functions are invoked and how data is managed. This involves using pointers to refer to functions and manipulating their addresses instead of direct function calls, as well as passing pointers as function arguments to enable direct access or modification to the memory of variables.
Pointer to functions in C
A pointer to a function in C is a special pointer type that stores the address of a function instead of a regular variable. This allows for greater flexibility, as it enables functions to be assigned to variables or passed as function parameters, among other applications. To create a pointer to a function, the general syntax is as follows:
return_type (*pointer_name)(parameter_types);
For example, to create a pointer to a function with the signature 'int func(int, int)':
int (*function_pointer)(int, int);
After declaring a pointer to a function, it can be assigned the address of a suitable function, as demonstrated below:
function_pointer = &func
Or it can be used to call the function it points to:
int result = function_pointer(arg1, arg2);
Passing pointers as function arguments
One powerful application of pointers in C functions is the ability to pass pointers as function arguments. By passing a pointer to a function, the function can access and modify the memory location of a variable directly, rather than working with a copy of the variable's value. This strategy is particularly useful when working with large data structures or when multiple values need to be modified within a function.
Here's an example of a function that swaps the values of two integer variables using pointers:
void swap(int* a, int* b) { int temp = *a; *a = *b; *b = temp; }
And here's how it can be called in a program:
int main() { int x = 5, y = 7; swap(&x, &y); printf("x = %d, y = %d\n", x, y); return 0; }
Function pointers in C Programming
A function pointer is a variable that stores the address of a function in memory, allowing for a more dynamic approach to invoking functions and extending functionality in different contexts. The syntax for defining a function pointer is similar to that of declaring a regular function, but with the addition of an asterisk (*) before the pointer's name.
To define a function pointer for a given function signature, the general syntax is as follows: return_type (*function_pointer_name)(parameter_types);
Once the function pointer is defined, it can be assigned the address of a compatible function using the '&' operator or used to call the function it points to.
Here's an example that demonstrates how to define and use a function pointer to invoke a mathematical operation:
#includePractical applications of function pointers
Function pointers offer numerous practical applications in C programming, including:
- Dynamic function calls: Function pointers facilitate the dynamic selection and execution of functions, depending on runtime conditions, user input, or other factors.
- Callback functions: Function pointers can be used to pass functions as arguments to other functions, enabling callbacks. This is particularly useful when implementing event-driven systems or libraries.
- Modular programming: Function pointers aid in the creation of modular programs, allowing for implementations to be more easily extended, replaced, or maintained.
- Customizable sorting functions: By using function pointers as a comparison function, sorting functions such as qsort() and bsearch() can be customized to sort according to specific criteria or program requirements.
In each case, function pointers revolutionize the way functions are called and data is managed, offering increased flexibility and versatility in C programs.
C Functions - Key takeaways
C Functions: Named sequence of statements that process input values and return an output value.
Static Functions in C: Local to the source file they are defined in, providing encapsulation and improved organization.
Power Function in C: Can be implemented recursively or iteratively for calculating exponentiation.
Relationship between Functions and Pointers: Function pointers enable dynamic invocation and manipulation of functions and memory.
Function Pointer Applications: Include dynamic function calls, callback functions, modular programming, and customizable sorting functions.
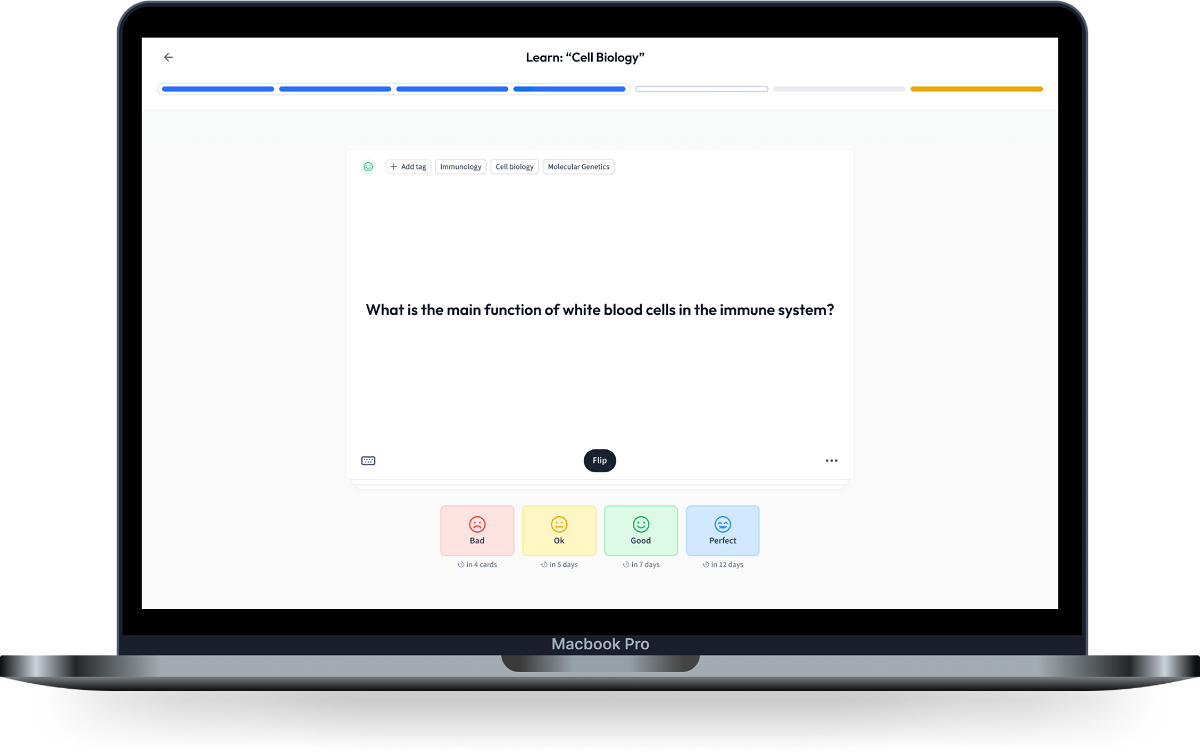
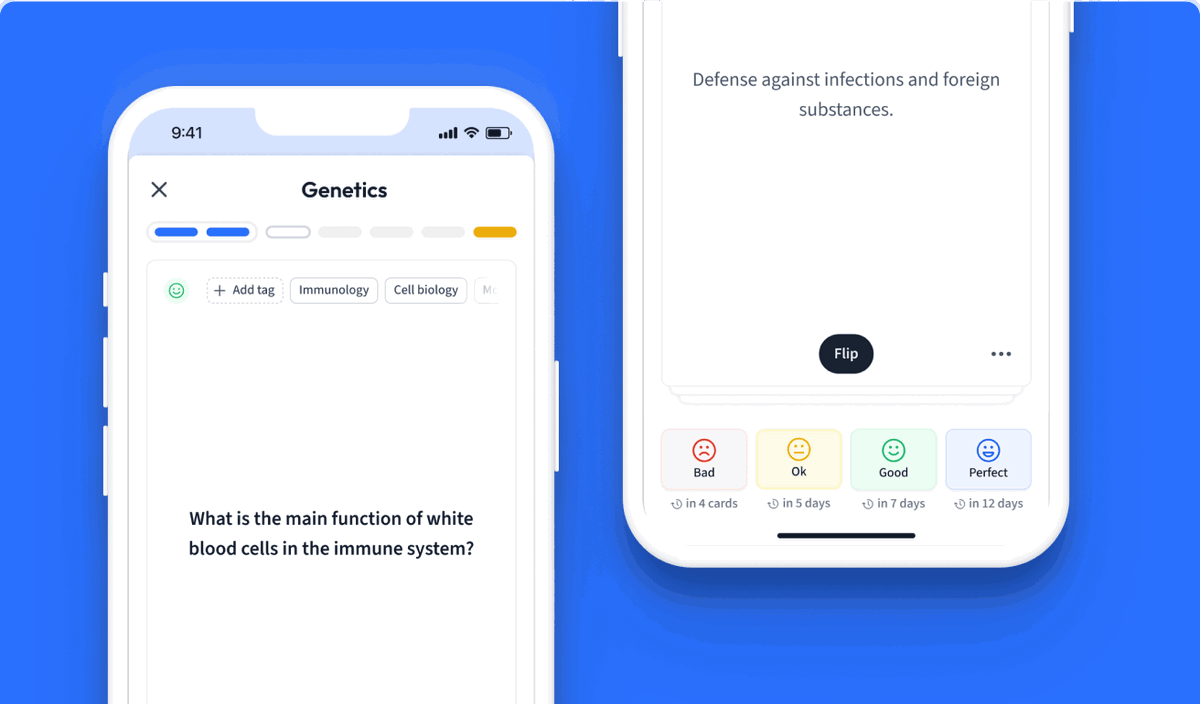
Learn with 152 C Functions flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about C Functions
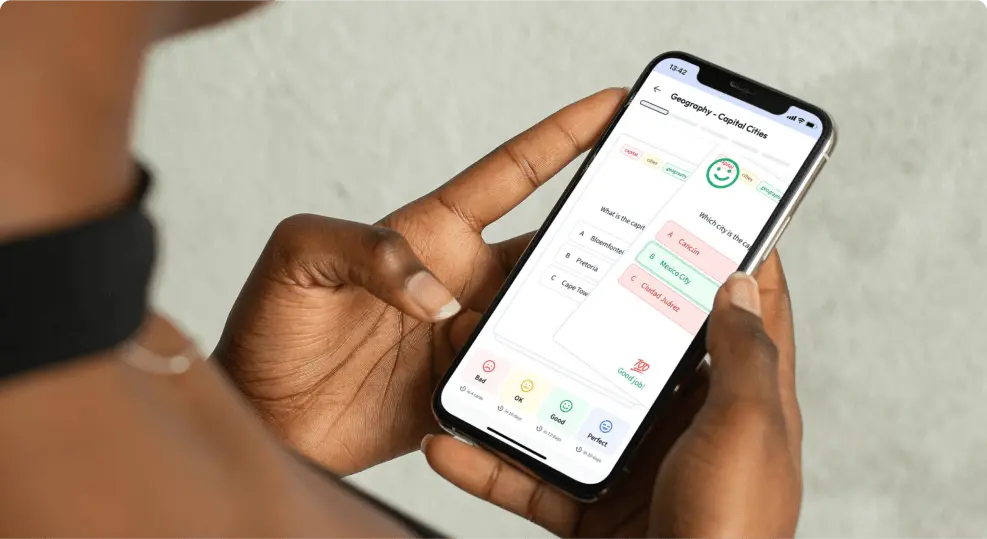
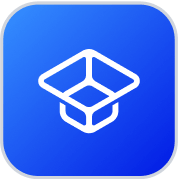
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more