Basics of While Loop in C Programming
A while loop is a control structure in C programming that enables you to execute a block of code multiple times as long as the specified condition remains true. This provides an efficient way to perform repetitive tasks without writing the same code multiple times. The basic syntax of a while loop in C is as follows:
while (condition)
{
// Code to be executed
}
In this structure, the condition must be enclosed within parentheses, and the code block to be executed must be enclosed within curly braces. The loop continues to execute the code block as long as the condition is true. Once the condition becomes false, the loop terminates, and the program execution moves to the next statement after the loop.
How while loop works in C
To better understand how a while loop works in C language, let's break down the process into the following steps:
- Evaluating the condition: When the program execution reaches the while loop, it first evaluates the condition provided within the parentheses.
- Executing the loop body: If the condition is found true, the code block enclosed within the curly braces is executed.
- Re-evaluation: The condition is re-evaluated, and if it remains true, the loop body is executed again. This process of re-evaluation and execution continues until the condition becomes false.
- Termination: Once the condition becomes false, the loop terminates, and the program execution moves to the next statement after the loop.
Here is a simple example of a while loop that prints the numbers from 1 to 5:
int main()
{
int count = 1;
while (count <= 5)
{
printf("%d\n", count);
count++;
}
return 0;
}
In this example, the initial value of the variable 'count' is set to 1. The while loop checks if the value of 'count' is less than or equal to 5. If the condition is true, the loop prints the value of 'count', then increments it by 1. The loop continues to execute until 'count' becomes greater than 5, at which point the loop terminates.
When using while loops, it's essential to ensure that the loop condition eventually becomes false; otherwise, you may encounter an infinite loop. An infinite loop occurs when the loop condition never becomes false, causing the program to run indefinitely and potentially crash or freeze the system. To avoid infinite loops, make sure to update the variables used in the loop condition and design the code logic in a way that ensures the condition will become false at some point during execution.
While Loop vs. Do While Loop in C
Both while loops and do while loops are used for executing a block of code repeatedly in C programming, but there are some key differences between them:
- Condition Evaluation: In a while loop, the condition is evaluated before the loop body is executed. In contrast, a do while loop evaluates the condition after executing the loop body. This means that a while loop may not execute its body at all if the initial condition is false, whereas a do while loop always executes its body at least once, regardless of the condition.
- Syntax: The syntax of a while loop starts with the keyword 'while', followed by the condition within parentheses, and a block of code within curly braces. On the other hand, a do while loop begins with the 'do' keyword and a block of code within curly braces, followed by the 'while' keyword and condition within parentheses, terminated by a semicolon.
The following table summarizes the differences between while loops and do while loops in C:
While Loop | Do While Loop |
Condition is evaluated before the loop body | Condition is evaluated after the loop body |
May not execute the loop body if the condition is false initially | Always executes the loop body at least once, even if the condition is false |
Keyword 'while' comes before the condition and loop body | Keyword 'do' comes before the loop body, while 'while' follows it |
Advantages and Disadvantages of While and Do While Loops
Each loop type has its own advantages and disadvantages, depending on the specific programming situation:
- While Loop Advantages:
- Suitable for situations where the condition must be true before executing the loop body.
- Can help avoid infinite loops if proper care is taken to ensure that the condition becomes false at some point.
- Easy to understand and implement, with a straightforward syntax.
- While Loop Disadvantages:
- Not suitable for situations where the loop body should be executed at least once, regardless of the initial condition.
- May accidentally lead to infinite loops if the loop condition does not become false during execution.
- Do While Loop Advantages:
- Guarantees that the loop body will be executed at least once, even if the condition is initially false.
- Suitable for situations where user input or external data is required before evaluating the loop condition.
- Do While Loop Disadvantages:
- May not be suitable for situations where the condition must be true before executing the loop body.
- Has a slightly more complex syntax and structure compared to while loops, which can be harder for beginners to understand.
In conclusion, choosing the appropriate loop type depends on your specific programming requirements, whether you need to evaluate the condition before executing the loop body or require a guaranteed execution of the loop body at least once. Both while and do while loops have their own advantages and disadvantages, so understanding their differences can help you make the right choice for your programming needs.
Infinite While Loop in C
Infinite while loops occur when the condition provided in the loop never becomes false, causing the loop to execute indefinitely. This can lead to the program becoming unresponsive or crashing the system. To spot and identify an infinite while loop, you can look for the following characteristics within the code:
- Constant conditions:If the condition in the loop is based on constant values that don't change during the execution, this will lead to an infinite loop. For example:
while (1) { // This code will run forever since the condition 1 (true) never changes }
- No update to variables: If the variables used in the loop condition don't get updated within the loop body, it can result in an infinite loop.
- Incorrect use of logical operators:Misuse or incorrect placement of logical operators in the loop condition can cause the condition to remain true regardless of variable updates. For example:
int count = 1; while (count < 5 || count > 0) // Incorrect use of || operator { printf("%d\n", count); count++; }
By closely examining your code and looking for these signs, you can spot an infinite while loop and take steps to correct the issue.
Preventing Infinite Loops and Troubleshooting
Preventing infinite while loops in C programming should be a priority for developers to ensure that the program runs smoothly and efficiently. Here are some helpful tips and techniques to prevent and troubleshoot infinite loops:
- Proper update of variables:Make sure that the variables used in the loop condition are updated within the loop body. This enables the condition to change and eventually become false, breaking the loop.
Example of updating variables:
int count = 1; while (count <= 5) { printf("%d\n", count); count++; // Properly updating the variable count }
- Use appropriate logical operators:Ensure that you use the correct logical operators in the loop condition to avoid unintentionally creating infinite loops.
AND Operator (&&) OR Operator (||) Use when multiple conditions must be true for the loop body to execute Use when at least one of the conditions must be true for the loop body to execute - Validate user input: If the loop condition depends on user input, ensure that the input is validated and checked before being incorporated into the condition. This can help prevent infinite loops based on inappropriate user input.
- Use conditional breakpoints: In debugging tools, you can set breakpoints that trigger only when specific conditions are met. This allows you to examine variables and conditions more closely, helping you identify infinite loops and their causes.
- Test your code step-by-step: Use a step-by-step debugging method to carefully examine the flow of your program and identify any potential infinite loops. This will allow you to understand how the loop behaves and make appropriate adjustments to the code.
By following these techniques and best practices, you can prevent and troubleshoot infinite loops in your C programming, ensuring that your code runs efficiently and effectively. Remember to always test your code thoroughly and pay close attention to the provided loop conditions and variable updates.
Practical Examples Using While Loop in C
In this section, let's explore a practical example using a while loop in C programming to find the factorial of a given number. A factorial of a non-negative integer n, denoted as n!, is the product of all positive integers less than or equal to n. For example, the factorial of 5 is 5! = 5 × 4 × 3 × 2 × 1 = 120.
Factorial is a non-negative integer, denoted as n!, which is the product of all positive integers less than or equal to n. Factorial can be computed using the formula: \( n! = n * (n-1) * (n-2) * ... * 1 \)
Here's a C program that calculates the factorial using a while loop:
int main()
{
int num, fact = 1;
printf("Enter a non-negative integer: ");
scanf("%d", #);
int count = num;
while (count > 0)
{
fact *= count;
count--;
}
printf("The factorial of %d is: %d\n", num, fact);
return 0;
}
Let's break down how this program works:
- First, we declare two integer variables, 'num' and 'fact', and initialise 'fact' to 1.
- Next, we prompt the user to enter a non-negative integer and store it in the 'num' variable.
- Then, we initialise the 'count' variable with the value of 'num', as we will be decrementing 'count' without affecting 'num'.
- After that, we create the while loop where the condition checks if 'count' is greater than 0.
- Inside the loop, we multiply 'fact' by 'count' and decrement 'count' in each iteration.
- Finally, we print the result, which is the calculated factorial of the input number.
By using a while loop, we can efficiently find the factorial of a given number with just a few lines of code, making this example both practical and informative.
Real-life Applications of While Loops in C
While loops are extensively employed in various real-life applications and software programs. Below are some examples of how while loops are used in practice:
- Menu-driven programs: While loops can be effectively utilised in creating menu-driven applications where users provide input to navigate different functionalities within a program. The while loop will continue running until the user selects an exit option or provides invalid input.
- Data validation: In applications that require user input or involve external data, while loops are often leveraged to ensure data validation and meet specific constraints. For instance, a while loop can be used to request input repetitively until the user provides a valid entry within the accepted boundaries.
- File processing: In programs that handle file manipulation or data handling, while loops come into play when you need to read or write data within files on a line-by-line basis, until reaching the end of the file. This ensures proper management and extraction of information.
- Sensors and hardware devices: In embedded systems and IoT-based projects, while loops are crucial for continuously monitoring and receiving data from sensors or hardware devices. They facilitate smooth communication and interaction between the microcontroller and external peripherals, enhancing overall application functionality.
- Multi-threaded applications: In multi-threaded programs, while loops can help manage the lifecycle of threads. They're used to control the thread execution, maintain responsive user interfaces, and modify thread behaviour based on external events or signals.
These real-life applications demonstrate the versatility of while loops and their importance in programming various systems and software solutions. The effectiveness of while loops lies in their simplicity, making them accessible to developers to solve a wide range of repetitive tasks and coding challenges.
Visualising While Loop with Flowchart
A flowchart is a valuable tool for visualising the flow of a while loop in C programming, as it allows you to grasp the fundamental structure and execution sequence more efficiently. A flowchart uses different shapes and symbols to represent the various components of a loop, such as conditions, loop body, and termination. Below is a step-by-step guide on how to create a flowchart for a while loop in C:
- Start: Use an oval shape to indicate the starting point of the flowchart.
- Initialisation: Place a parallelogram or rectangle, representing the initialisation of variables involved in the loop condition.
- Loop Condition: Use a diamond shape to represent the while loop condition. The condition determines whether the loop will execute or not.
- Loop Body: Place a series of rectangles or parallelograms, denoting the statements within the loop body. This includes the instructions to be executed when the loop condition is true.
- Variable Update: Inside the loop body, show the updating of variables used in the loop condition. This helps visualise the changes that lead to the loop's termination at some point.
- End: Finally, use an oval shape to indicate the ending point of the flowchart, which is reached when the loop condition becomes false.
Importance of Flowcharts in Understanding Loop Structures
Flowcharts serve as an important tool in understanding loop structures in C programming, including while loops, for several reasons:
- Visual representation: Flowcharts provide a clear and concise visual representation of the loop structure, making it easier to comprehend the logic and flow of the program.
- Algorithm clarification: A flowchart helps break down complex algorithms and simplifies the sequence of operations within a loop, ensuring a better understanding of the overall program functionality.
- Error detection: Flowcharts are effective in identifying errors, logical inconsistencies, or infinite loops within the program at an early stage before proceeding with the actual coding.
- Communication: By providing a coherent graphical representation, flowcharts enable better communication and collaboration among team members, making it simpler to discuss, review, and optimise code.
- Documentation: Flowcharts can be incorporated into project documentation to enhance readability and offer a quick reference guide for other developers or stakeholders.
In conclusion, flowcharts play a vital role in understanding and illustrating loop structures in C programming. They facilitate efficient communication, error detection, and overall comprehension of the program's control flow by visually representing the key components and sequence of operations in a while loop. By leveraging flowcharts, developers can build a better understanding of their code and enhance program design and functionality.
While Loop in C - Key takeaways
While Loop in C: Control structure that executes a block of code multiple times as long as a specified condition remains true.
Difference between while and do while loop: While loop checks the condition before executing the loop body, do while loop checks the condition after executing the loop body.
Infinite While Loop in C: Occurs when the loop condition never becomes false, causing indefinite execution and potential system crashes.
Practical Examples: While loops are used in menu-driven programs, data validation, file processing, and interaction with sensors and hardware devices.
Visualising Loop with Flowchart: Simplifies understanding of loop structure and flow, facilitates error detection, and enhances communication and collaboration in programming.
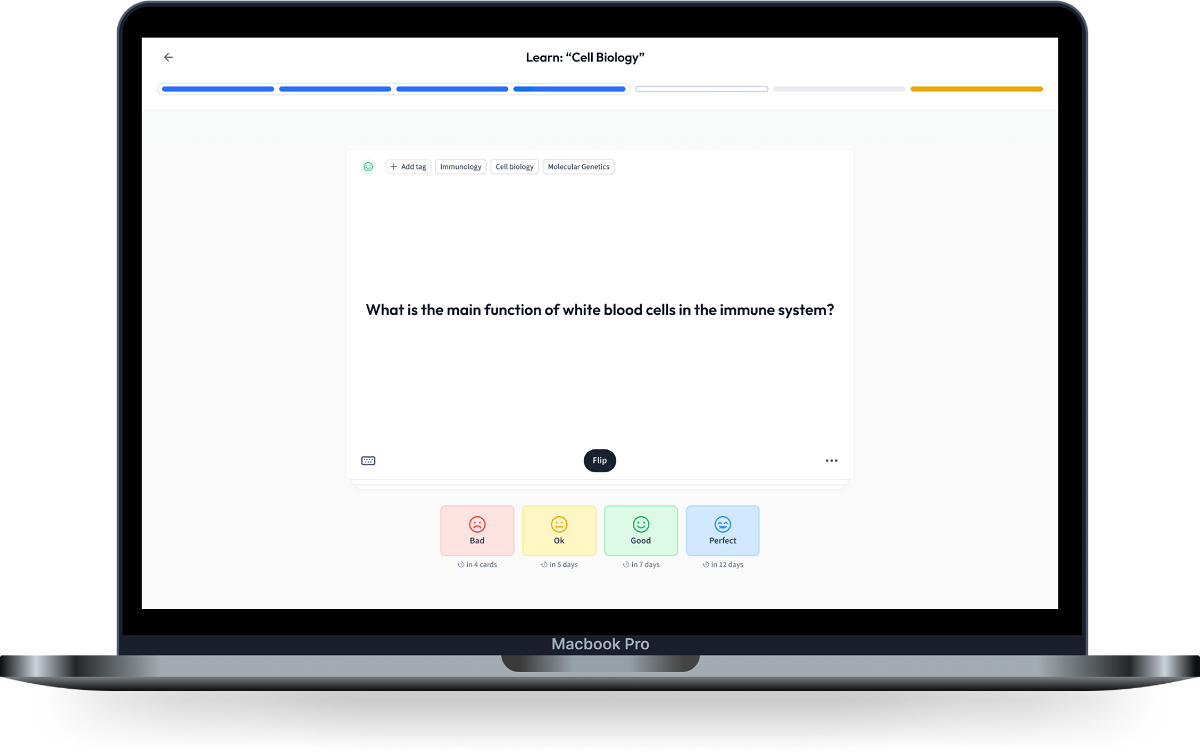
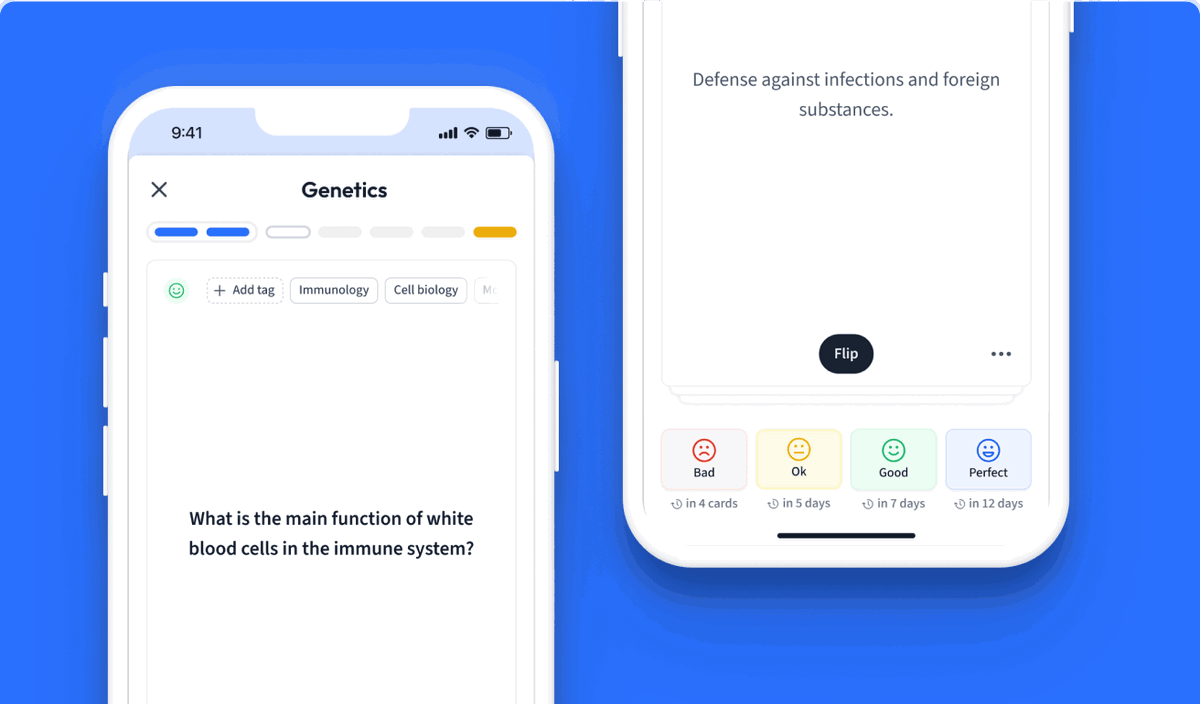
Learn with 15 While Loop in C flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about While Loop in C
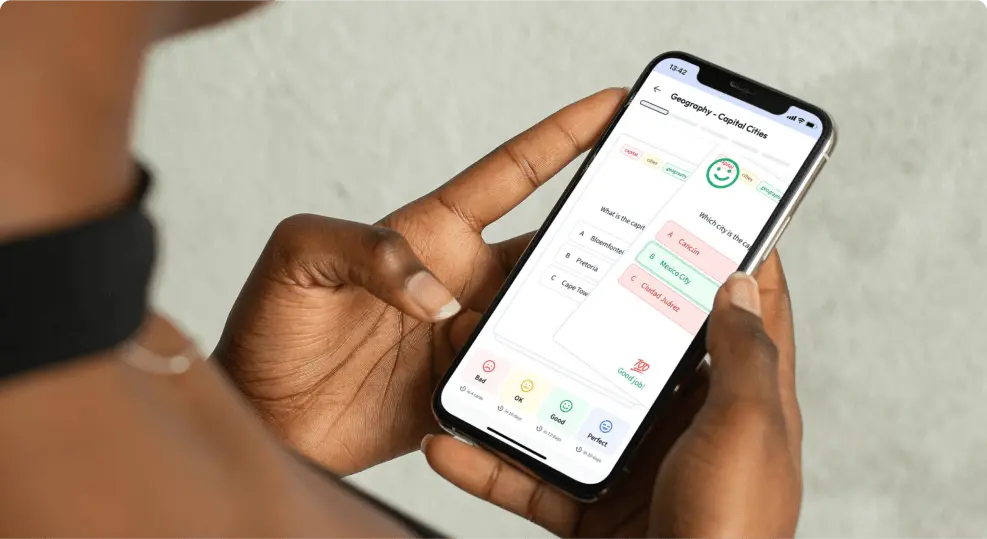
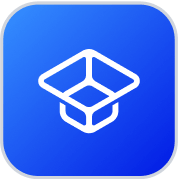
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more