Understanding Javascript Callback Basics
In your journey to learning Computer Science, especially when cruising through the ocean of JavaScript, you're likely to come across the concept of 'callback'. It's a fundamental idea, understanding which will level up your JavaScript skills significantly while opening a world of possibilities for coding strategies. Before unpacking the intricate details about Javascript Callback, let's start with its straightforward notion and dig dipper later.
Defining Javascript Callback: What is It exactly?
A JavaScript Callback is essentially a function that is passed as an argument in another function and is executed after a certain event has occurred or some task is completed. Hence, the function that takes other functions as parameters is known as a higher-order function, with the functions passed as arguments known as callback functions.
Let's explore it with this basic example:
function greet(name) { alert('Hello ' + name); } function processUserInput(callback) { var name = prompt('Please enter your name.'); callback(name); } processUserInput(greet);
In the code above, the processUserInput function accepts the greet function as its argument. The greet function is then called within the processUserInput function after the user entered their name. This is a typical example of a JavaScript Callback.
Distinct Characteristics of a Callback Function in Javascript
A Callback function in JavaScript is unique due to its two key properties:
- It could be passed as an argument, enabling functions to operate on dynamic data and behaviours.
- Callbacks can be invoked after certain events or computations have completed, thereby making the execution timeline of JavaScript programs much more flexible.
The dynamic and asynchronous nature of callback functions makes them a powerful tool in JavaScript, especially in handling tasks like event handling, timer functions, and AJAX calls. The JavaScript runtime environment employs an event-driven, non-blocking I/O model which makes it lightweight and efficient and it's powered primarily by its ability to use functions as first-class objects, i.e., callback functions.
Importance and Practical Use of Javascript Callback
Callback functions are a fundamental part of JavaScript and they play a key role in asynchronous programming.
When you write code that runs asynchronously, you can perform long network requests without blocking the main thread of execution. This is a significant advantage of JavaScript callbacks, they make sure that the Javascript engine does not wait or block other code from executing where there is a delay or operation that needs to be completed before proceeding.
Let's view this concept in action through a practical example:
function downloadImage(url, callback) { let img = new Image(); img.onload = function() { callback(null, img); }; img.onerror = function() { callback(new Error('Failed to load image at ' + url)); }; img.src = url; } downloadImage('path/to/my/image', function(err, img) { if (err) { console.error(err); } else { document.body.appendChild(img); } });
In the code above, the downloadImage function downloads an image from a certain URL. If the image is downloaded successfully, the callback function is called with null as the first argument and the image as the second argument. If the image fails to download, the callback function is called with an Error object as the first argument.
Dive into Callback Function Technique in Javascript
The callback function technique in JavaScript defines a unique mode of programming which leverages the power of asynchronous programming. It gives you the ability to structure your code in a non-linear, event-driven manner. This, in turn, allows the execution of tasks outside the traditional top-to-bottom order which is a powerful toolkit for JavaScript developers. From handling user activity events to performing complex I/O operations, this technique takes the stage front and centre in many programming scenarios.
Fundamental Concepts Regarding Callback Function Technique
In JavaScript, functions are first-class objects. This means that functions, just like any other objects can be passed as arguments into other functions, returned by other functions and can be assigned to variables. A higher-order function is a function that operates on other functions, either by taking them as arguments or by returning them.
A JavaScript Callback is a function that's passed as an argument in another function. It's set to be executed after some event has occurred or a specific task has been completed. The function that receives another function as a parameter is considered as a higher-order function, and the passed function is termed as a Callback function.
By putting this technique to use, you can create more flexible, efficient and modular code. Here are some crucial concepts to note:
- Execution clarity: Since callback functions do not run straight away, they are 'called back' (or executed) later, the order of execution in your code becomes clearer.
- Non-Blocking Code: JavaScript is single-threaded, meaning it only takes one action at a time. Callback functions can asynchronously handle heavy tasks in the background, keeping the application responsive and fast.
- Adherence to DRY principles: Callbacks are also used to make your code DRY (Don't Repeat Yourself) by reusing a function instead of repeating the same set of actions.
A Guide to Implementing a Callback Function Technique In JavaScript
If you're navigating through the seas of JavaScript, implementing callback techniques is rather a simple task. Let's look at detailed steps and ample examples to illustrate the process of implementation.
The first step is to create a higher-order function that will take another function as an argument. The second step is to create a callback function that you'll pass to the higher-order function. Look at the snippet below for clarification:
function higherOrderFunction(callback) { // some code to execute... callback(); } function callbackFunction() { // code to execute after the higherOrderFunction code has finished } higherOrderFunction(callbackFunction);
As part of the process, you can also pass parameters to the callback function, like numbers, objects or even other functions. Adding extra parameters makes your callback function more flexible and modular, as it can compute different outcomes based on the arguments you pass in.
Efficient Use of Callback Function Technique for Javascript Programming
Although JavaScript Callback technique is powerful, it can result in convoluted and hard-to-read code, leading to a pitfall known as "Callback Hell". It's where too many nested callback functions make the code look complicated and twisted.
To make efficient use of Javascript Callback methods, adhering to these recommendations can be a tremendous help:
- Modularise Functions: Each function should do one thing and do it well. Limiting your functions to perform a single task makes your code easier to understand, debug and reuse.
- Name Your Functions: Using named functions instead of anonymous ones aids in identifying what each callback function done when debugging.
- Handle Errors: Always code in ways to handle any potential errors with your callback functions. A common method is to pass the error as the first argument to your callback function.
- Avoid Deep Nesting: Deep nested callback functions can lead to complicated code. Limit the number of nested callbacks to make your code cleaner and more understandable.
Understanding and implementing callback functions is a major step towards mastering JavaScript. It allows you to harness the full power of this dynamic, event-driven language. Remember, practise is key when it comes to perfecting your use of callbacks. So, don't hesitate to roll your sleeves up and start putting this critical technique to work!
Detailed Analysis: How Javascript Callbacks Work
Javascript Callbacks represent a crucial aspect of Javascript programming. Whether working on event handling or AJAX requests, Javascript Callbacks come into play quite prominently. To use Javascript Callbacks to their fullest potential, the underlying mechanism and working principles must be understood thoroughly.
The Mechanism Behind Javascript Callback Functions
Indeed, one of the most fundamental things to grasp for understanding the Javascript language deeply is the mechanism of how callback functions work. A Javascript Callback is simply a function that is used as an argument in another function. The 'callback' name is derived from the fact that when you're passing a function as an argument, that function will be 'called back' at some point in time.
The process behind a Javascript Callback operates on the concept of higher-order functions in functional programming. As functions are first-class citizens in Javascript, they can be assigned to a variable, passed on as an argument to a function, or even returned by another function. This concept is the foundational building block for Javascript Callbacks.
Let's unravel the mechanism behind a Javascript Callback with a simplified example:
function performCalculation(x, y, operation){ return operation(x,y); } function add(a,b){ return a+b; } let result = performCalculation(5,10,add); console.log(result); // Outputs 15
In this example, the add function is passed as a callback function to the performCalculation function. The performCalculation function receives this function as an argument and computes the result by invoking (or 'calling back') the add function at a suitable point in the code, thus exhibiting how a Javascript Callback function works.
Decoding Javascript: Working Principles of Callbacks
The cardinal principle of incorporating Callbacks into Javascript is to handle the asynchronous behaviour of the language. As Javascript is single-threaded, allowing only one operation to execute at a time, callbacks are significantly employed in maintaining the tempo of the application during execution of time-consuming operations like accessing API responses or IO operations without having to pause and wait for the result.
A core premise behind a Javascript callback is the Event Queue in the Javascript execution model of Event Loop. The execution engine in Javascript utilises an Event queue where all asynchronous operations are relegated till the current execution scope (Stack) has executed all its synchronous operations. Upon completion, Javascript then checks the queue, runs the operation which first arrived in the queue and this loop continues.
This working principle sets the ground for the asynchronous behaviour of Javascript, leading to successful execution of the callback functions without disrupting the flow of the application. Proper application of this principle is crucial in handling AJAX requests or event listener functions.
Considering Performance: How Efficient are Javascript Callbacks
Javascript callbacks have an array of benefits, due to its non-blocking and asynchronous attributes contributing to improved user experience by ensuring smooth application functionality. However, understanding a JavaScript callback’s efficiency is a complex task.
A sheer advantage of using callbacks is that they keep your application from freezing up during execution of a long-running operation. For example, when downloading a large file, a callback function allows a notification to be sent after completing the download; meanwhile, the user can continue with other tasks.
However, it is not all rosy with callbacks. A usual complaint developers face while using callbacks is commonly known as Callback Hell. This term indicates the surplus occurrence of nested callbacks, diluting the code’s readability and comprehensibility. Moreover, if not maintained properly, the flow of callbacks can be confusing, making the debugging process complicated.
Another critical performance issue with callback functions in Javascript is error-handling. If an error occurs in an asynchronous operation and it is not handled properly with a callback, it will be silently lost, affecting the application's robustness.
To mitigate these issues, developers have turned towards alternatives like Promises and Async/Await which are built upon callbacks but provide cleaner syntax and better error handling. That said, it is vital to understand callbacks because they form the core of these higher-level abstractions in Javascript.
In conclusion, understanding JavaScript callbacks and its efficiency is a pivotal consideration. Despite potential pitfalls if not handled wisely, the array of benefits that Javascript Callbacks bring on debugging efficiency, non-blocking operations and user experience make them an integral part of the language.
Practical Guides: Javascript Callback Example
The real strength of understanding a topic like Javascript Callbacks lies in using practical examples. This section will provide a simplified explanation of a Callback function Javascript example, guide you to build a Javascript Callback, and then delve into an annotated example for solidifying understanding.
Simplified Explanation with a Callback Function Javascript Example
A direct and straightforward example is the best way to illustrate how a Callback function in Javascript operates. Consider the scenario where a user wants to fetch some data from a server, manipulate the data received, and finally display it to the user. The steps for the operation in Javascript would usually be asynchronous, meaning you would not want to block the execution of your code till the server responds with the data.
function getDataFromServer(callback){ // Simulating server fetch through setTimeout setTimeout(()=>{ const data = "Hello World!"; callback(data); }, 2000); } function displayData(data){ console.log(data); } getDataFromServer(displayData);
In this example, getDataFromServer is a function that simulates a fetch request to a server through setTimeout, which invokes the given callback function after 2 seconds with some data. The displayData function will be called back after the data fetch simulated by setTimeout is finished, and it displays the result "Hello World!" after 2 seconds without blocking the rest of the code.
Learn Through Practice: Building a Javascript Callback
Now, let's walk through the process of building a Javascript Callback function. This will provide a more hands-on experience of understanding how callbacks function.
Consider a scenario where you're building an application that calculates square roots of numbers. There's a server that provides you with a number and you need to print out the square of that number.
function getNumberFromServer(callback) { setTimeout(() => { const number = 16; callback(number); }, 1000); } function calculateSquareRoot(number) { console.log(Math.sqrt(number)); // Outputs 4 } getNumberFromServer(calculateSquareRoot);
In this example, the getNumberFromServer function is the asynchronous operation that mimics a server fetch. Therefore, it accepts a callback function as an argument. Here, the calculateSquareRoot function is passed as a callback which calculates the square root of a number. When the asynchronous operation of getNumberFromServer completes, it invokes the callback function with the fetched number. Then, the callback function calculateSquareRoot operates on that fetched number.
Annotated Example: Understanding Javascript Callback through Annotation
Let's examine the previous example in an annotated manner, for a more holistic understanding.
Breakdown of this Javascript Callback example:
- Firstly, define getNumberFromServer() function which accepts a callback function as an argument.
- Within this function, use setTimeout() to simulate a server fetch which takes some time.
- After 1 second (1000 milliseconds), the anonymous function inside setTimeout() gets invoked.
- This anonymous function then calls the callback function passed as an argument to getNumberFromServer(), and passes the number 16 as an argument to the callback.
- Secondly, define the calculateSquareRoot() function that console logs the square root of the number passed to it.
- Finally, invoke getNumberFromServer() and pass calculateSquareRoot() as the callback function.
// Define Function that performs server fetch and calls the callback function getNumberFromServer(callback) { // Using setTimeout to simulate server fetch setTimeout(() => { // After 1 sec, callback function is invoked with number 16 const number = 16; callback(number); }, 1000); } // Define Callback Function that computes square root function calculateSquareRoot(number) { console.log(Math.sqrt(number)); // Outputs 4 } // Fetched number from server and computed square root getNumberFromServer(calculateSquareRoot);
This example goes to show that passing functions as arguments (callback functions) enables you to dictate when those function should run in response to events or conditions.
Taking Javascript Programming Further: Advanced Callback Techniques
After gaining a solid understanding of the basic functionalities of Javascript callbacks, you can take your Javascript programming skills to the next level by mastering advanced callback techniques. This heightened proficiency can exponentially enhance your programming capabilities and contribute to producing sophisticated and highly-functional applications.
Advancing Your Skills: Master Javascript Callbacks
Becoming proficient in Javascript callbacks involves an amalgamation of several advanced techniques. These techniques play a pivotal role in enhancing the functionality and usability of your Javascript applications. It's important to master these techniques and use them appropriately in your code.
Error-First Callbacks: One of the primary techniques to consider while advancing your Javascript Callback skills is the Error-First Callback pattern. This paradigm suggests that the first parameter in any callback function should be an error object. If an error occurs during the execution of an operation, this error object should be populated; otherwise, it should be null.
function exampleErrorFirstCallback(err, data) { if (err) { console.log('Error:', err); } else { console.log('Data:', data); } }
This allows the calling function to handle errors gracefully, as the first check after executing a callback is typically whether an error has occurred. This is an integral part of the Node.js error handling mechanism and is widely used in Node.js libraries.
Inline Callbacks: Another strategy to enhance your Javascript callback skills relies on defining anonymous functions where a callback is expected, a technique known as Inline Callbacks. This enables you to easily and quickly define small one-time use functions without disrupting the code's flow or needing extra function names.
let numbers = [1, 2, 3, 4, 5]; numbers.forEach(function(value, index){ numbers[index] = value * 2; });
However, be wary of nesting too many inline callbacks, as this can lead to difficult-to-read code, a situation often referred to as 'callback hell'.
Expert Tips for Javascript Callback Implementation
Coding with Javascript Callbacks can be an exciting journey, but it requires clever strategies to alleviate issues like 'Callback Hell' and the need for more elegant, cleaner syntax. Here are some expert tips to refine your implementation of Javascript Callbacks:
- Modularise your code: Break down your code into smaller functions. This not only makes your code much more manageable and readable but also makes debugging a lot easier.
- Improve Error Handling: Always make sure you're handling errors in your callbacks. Not addressing errors adequately means that when your callbacks fail, they'll fail silently, leaving you clueless to what went wrong.
- Stay away from 'Callback Hell': Ensure that your callbacks are not overly nested. This could lead to your code becoming unreadable and difficult to debug, a phenomenon often known as 'Callback Hell'.
- Know when not to use Callbacks: Recognise when a callback is not necessary. Callbacks are perfect for handling delays, waiting for user interaction or responses from servers. However, for purely computational tasks that don't require waiting for any events, they could complicate things and reduce performance.
Debugging's Role in Javascript Callback Function Mastery
Debugging represents a critical skill for mastering Javascript Callbacks. In the asynchronous world of Javascript, debugging can be absolutely crucial for understanding how your code flows, especially when callbacks are involved. Here are some methods to improve your debugging skills when working with Javascript Callbacks:
Chrome DevTools: The Chrome browser's built-in developer tools can effectively debug Javascript code. Chrome DevTools allows you to set breakpoints in your code, step through your code line by line, inspect variables and observe the call stack.
console.log(): When dealing with asynchronous code, sometimes adding a console.log() statement can help you understand the order in which your code runs. Although this is a very rudimentary form of debugging, it can be effective at tracing the general flow of execution.
Finally, use the stack trace when an error is raised. The stack trace provides valuable information about the history of function calls that led up to the error, making it easier to identify the source of the problem. Employ these debugging tips, and you'll be on your way to mastering Javascript Callbacks.
Remember, mastering Javascript Callbacks goes beyond just understanding the syntax—it's about recognising where and when to use callbacks for efficient, elegant, and readable code. It's also about appreciating callbacks' nature and role in asynchronous Javascript, understanding the event loop, how functions are first-class citizens in Javascript and how that empowers functional programming paradigms with constructs like callbacks. This mastery can magnify your potential in Javascript programming, allowing you to craft powerful and efficient Javascript applications.
Javascript Callback - Key takeaways
- A JavaScript callback is a function passed as argument in another function. It is meant to be executed after certain event has occurred or a specific task has been completed.
- Javascript uses callbacks to create more flexible, efficient and modular code by improving execution clarity, allowing non-blocking code, and fostering adherence to DRY (Don't Repeat Yourself) principles.
- The technique to implement a callback function in JavaScript involves creating a higher order function and a callback function which is passed as an argument to the higher order function.
- Misuse of Javascript Callbacks can lead to "Callback Hell", a situation where code becomes complex and hard to read due to too many nested callback functions. Recommendations to avoid this include modularising and naming functions, handling errors, and avoiding deep nesting.
- Understanding Javascript Callbacks is important as they allow for the asynchronous behaviour of Javascript, which is single-threaded. Thus, they maintain the tempo of the application during execution of time-consuming operations.
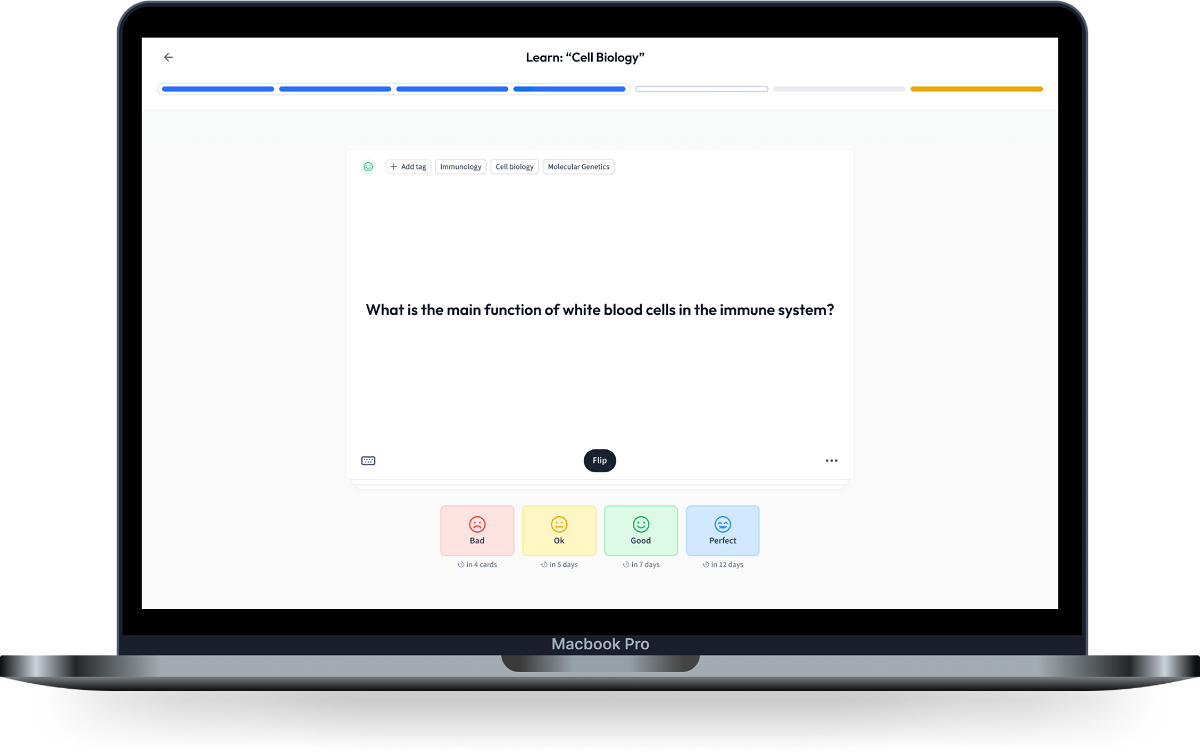
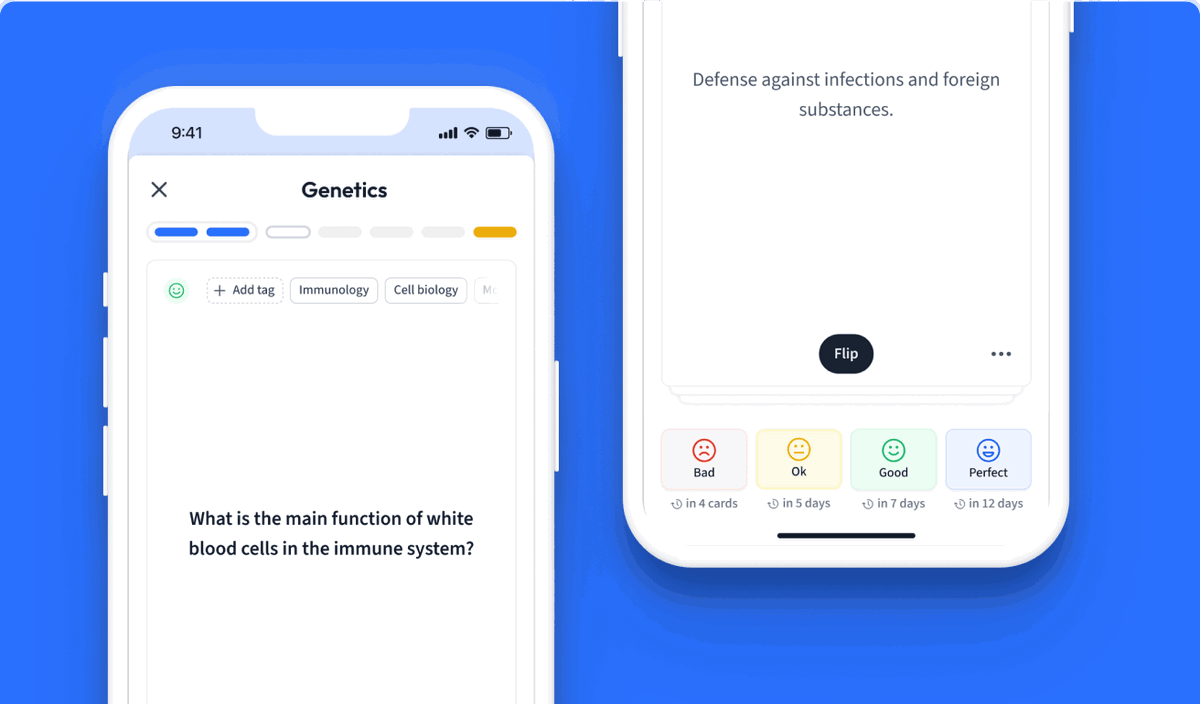
Learn with 15 Javascript Callback flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript Callback
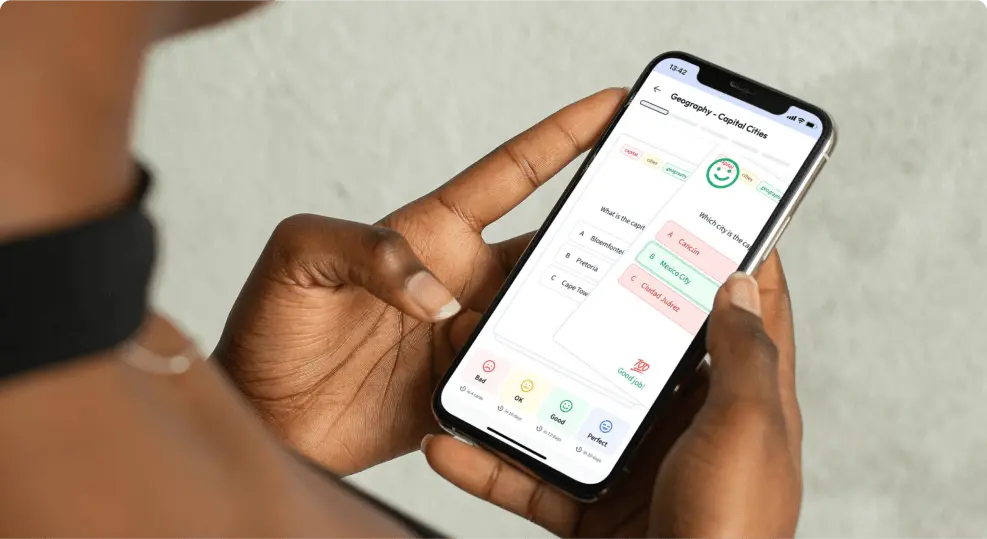
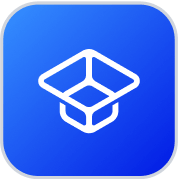
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more