Understanding Java Type Casting
In the fascinating field of computer science, specifically when delving into programming with Java, one term you will encounter is "type casting". Type casting is an essential concept to grasp when learning Java, as it aids in the smooth operation of your code.An Introduction to What is Type Casting in Java
Type Casting in Java is a process by which we change an object or variable from one data type to another. It forms a vital part of Java's internal functionality, enabling disparate types to interact by converting them to a common form.
Defining Java Type Casting and Conversion
There are two types of type casting operations in Java, namely "implicit" and "explicit" casting. Implicit casting, also known as automatic conversion, happens when the compiler automatically converts one data type to another. This occurs when converting a smaller type to a larger type, where the target type can easily accommodate all possible values of the source type.For instance, converting an 'int' value to a 'long' value. Java will automatically handle this for you.
Understanding How to Type Cast in Java
Approaching type casting in Java requires a systematic understanding of how variable types work. However, don't be alarmed - with the correct approach, type casting can become second nature.The Basic Steps in Java Type Casting
To execute Java type casting, you can follow these steps:- Identify the source and target types.
- Determine whether implicit or explicit casting is required.
- Apply the correct syntax for the type of casting.
Unraveling Java Type Conversion and Casting Techniques
Java has a specific set of rules and techniques for performing type casting. Knowledge of these rules provides the ability to handle the manipulation of data types efficiently.Implicit Conversion (Automatic) | byte -> short -> int -> long -> float -> double |
Explicit Conversion (Forced) | double -> float -> long -> int -> short -> byte |
Remember that explicit casting could lead to data loss, so use it judiciously.
boolean bool = true; String str = String.valueOf(bool);Mastering Java type casting will enable you to build far more dynamic and robust Java applications, as you'll have greater control and flexibility over the data types used in your programs. Happy coding!
Features of Java Type Casting
Delving into the sea of Java programming, one significant facet that stands out is type casting. It allows variable values to intermingle in ways that were previously impossible, and this flexibility is what offers richer depth to Java projects.Significant Characteristics of Java Type Casting
Java type casting possesses quite a few notable features that separate it from similar functionalities in other programming languages. Firstly, it is essential to note that Java type casting is a bidirectional operation meaning you can cast data types:- From smaller to larger types (implicit casting)
- From larger to smaller types (explicit casting)
In Java, type casting applies to both primitive and reference types. However, reference type casting differs from primitive type casting. For reference types, downcasting and upcasting concepts are prevalent and crucial to understand.
Another remarkable attribute of type casting in Java is that it does not allow direct casting between boolean and non-boolean types. This restriction is unique to Java and aims to prevent logical errors in programming.Benefits of Using Type Casting in Java Programming
Type Casting in Java programming has several advantages. It unlocks a wholly new dimension of flexibility and control over your code. Here are some valuable benefits:- It allows mixing of different types of data in operations, thereby broadening the scope of operations.
- Enables you to use elements interchangeably in complex programming situations, adding a layer of flexibility to your work.
- With the help of explicit casting, you can minimise memory usage by converting larger data types to smaller ones where feasible.
- Using reference type casting, it's possible to access methods of a subclass from a superclass reference, thereby supporting polymorphism.
How Java Type Conversion Differs from Other Languages
Java's type casting approach varies considerably from that of other programming languages. While most languages offer some typecasting functionality, Java's two-way, automatic, and manual casting stands out. In certain languages such as Python, type casting is freely possible between boolean and numerical values, which Java restricts. The overarching framework of Java assures that type casting only happens when it's safe or explicitly commanded by the programmer. Moreover, some languages (e.g., JavaScript) use dynamic typing, meaning type conversion occurs automatically based on context. In contrast, Java's type system is static, meaning types must match explicitly, or type casting must be used. Java strictly differentiates between integer and floating-point types, and explicit type casting must be done when fractional numbers need to be used as integers or vice versa. Some languages automatically round fractional numbers when used in an integer context. Each of these characteristics defines Java's unique approach to type conversion and casts, ensuring reliable and explicit control over your coding operations, albeit with more strictness than some alternative languages.Java Type Casting Examples
Great! You're moving forward impressively through the sceneries of Java typecasting. As you navigate this landscape, examples are excellent for cementing newly acquired knowledge. So, grab your exploratory gadgets as we unearth a substantial selection of Java typecasting implementations.Practical Examples of Type Casting in Java
In the world of Java, typecasting occurs in a variety of forms, each with its unique facets and eccentricities. By examining a few cases, you can get a sense of how to apply these principles in your projects. Firstly, the **implicit type casting** stage. Recollect that this occurs automatically when converting a smaller type to a larger type. No special syntax is required. See the example below:short shortValue = 10; int intValue = shortValue;In the above code, a short value is automatically converted into an int. Transitioning to the more complex **explicit type casting**, where the compiler will not intervene and you must instruct it to convert a larger type to a smaller one. Here's how to do it:
double doubleValue = 3.14; int intValue = (int) doubleValue;Above, a double value is forcefully cast into an int, truncating the decimal component due to the integer's inability to store decimal places. Java typecasting also includes **reference typecasting**. Reference type casting requires familiarity with object-oriented programming in Java. Here's a simple example:
Object obj = new String("Hello"); String str = (String) obj;In above code, you can see that an Object (the superclass) is being cast to a String (the subclass).
Step by Step Guide to Undertake Java Type Casting
You've already done the groundwork and familiarised yourself with the theory of typecasting, so now let's look at a step-by-step procedure to practice these models accurately: 1. **Step 1**: Identify the types of the source and target variables. 2. **Step 2**: Determine whether an implicit or explicit cast is required depending on their sizes. 3. **Step 3**: If it's an implicit cast, assign the source to the target directly. If it's an explicit cast, use the syntax shown above. In the case of reference casting, an additional step is required: 4. **Step 4**: Ensure that the object you are casting is actually an instance of the target type. You can use an 'instanceof' check for this.Common Mistakes to Avoid While Performing Java Type Conversion
In your journey of Java type conversion, it's not uncommon to stumble. Identifying these common mistakes before they happen can save hours of debugging time: - **Mistake 1**: Trying to cast incompatible types. Remember, boolean cannot be cast to any non-boolean types, or vice versa. - **Mistake 2**: Forgetting to add the cast operator in an explicit cast. A missing cast operator will lead to compilation error. - **Mistake 3**: Downcast without an 'instanceof' check. It's always a good practice to check whether an object is an instance of a subclass before casting, to prevent ClassCastException.Object obj = new Integer(10); if (obj instanceof String) { String str = (String) obj; // This will throw a ClassCastException because obj is not an instance of String. }- **Mistake 4**: Ignoring possible data loss with explicit casts. Always be cautious that explicit casting from a larger type to a smaller type may lead to data loss. Remember, as you continue your journey, mistakes are just learning points in disguise. With each misstep, you become a more experienced programmer ready to tackle greater challenges!
Java Type Casting - Key takeaways
- Java Type Casting is a process by which an object or variable is changed from one data type to another, contributing to Java's internal functionality by enabling different types to interact by converting them to a common form.
- Java type casting operations are divided into implicit and explicit casting. Implicit casting, or automatic conversion, happens when the compiler automatically converts one data type to another, usually from a smaller to larger type. Explicit casting, or forced conversion, is required when converting a larger type to a smaller type, which may mean potential data loss.
- To execute Java type casting, one must identify the source and target types, determine whether implicit or explicit casting is needed, and apply the appropriate syntax for the casting type.
- Java restricts direct conversion or casting of boolean types to any non-boolean types. A method like String.valueOf() should be used instead.
- Java type casting is bidirectional, allowing data types to be cast from smaller to larger types (implicit casting) and from larger to smaller types (explicit casting). It applies to both primitive and reference types, and it doesn't allow direct casting between boolean and non-boolean types.
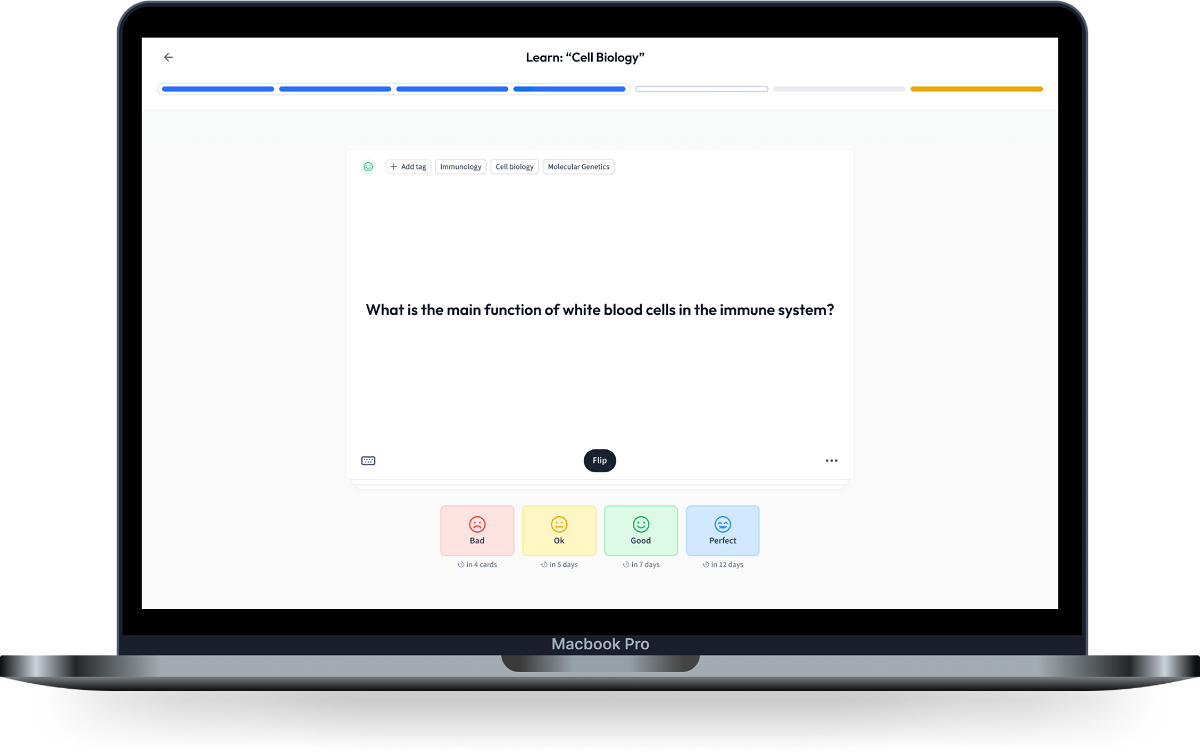
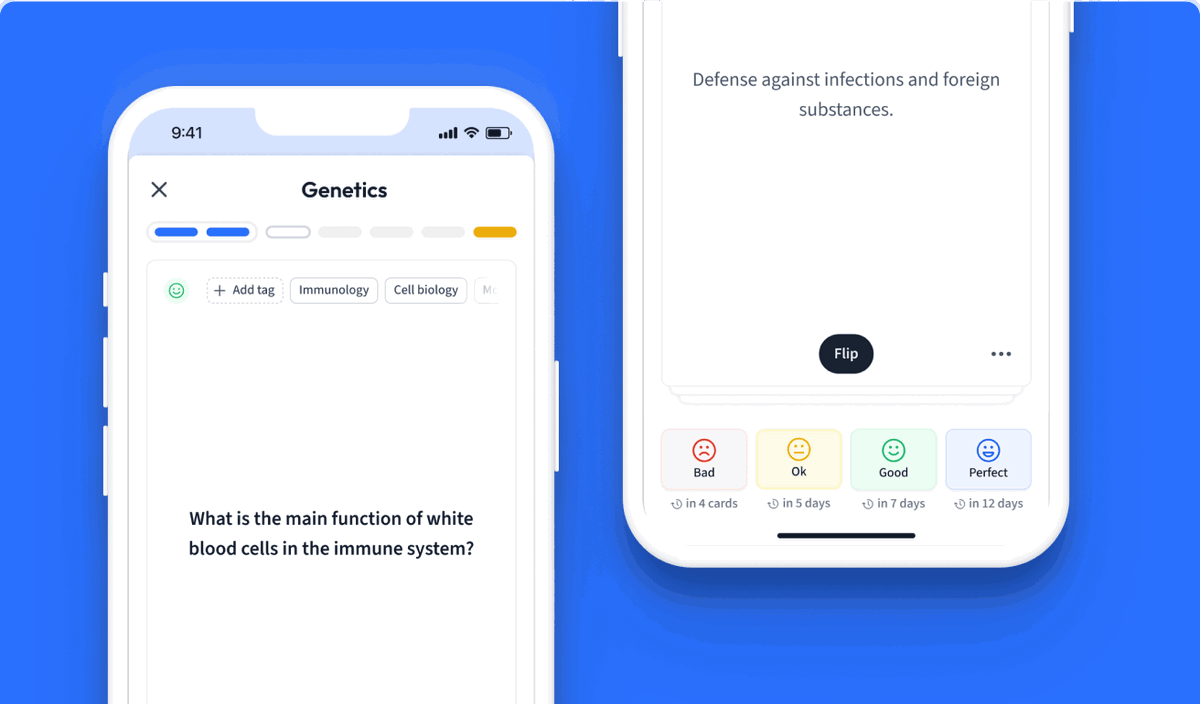
Learn with 12 Java Type Casting flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Java Type Casting
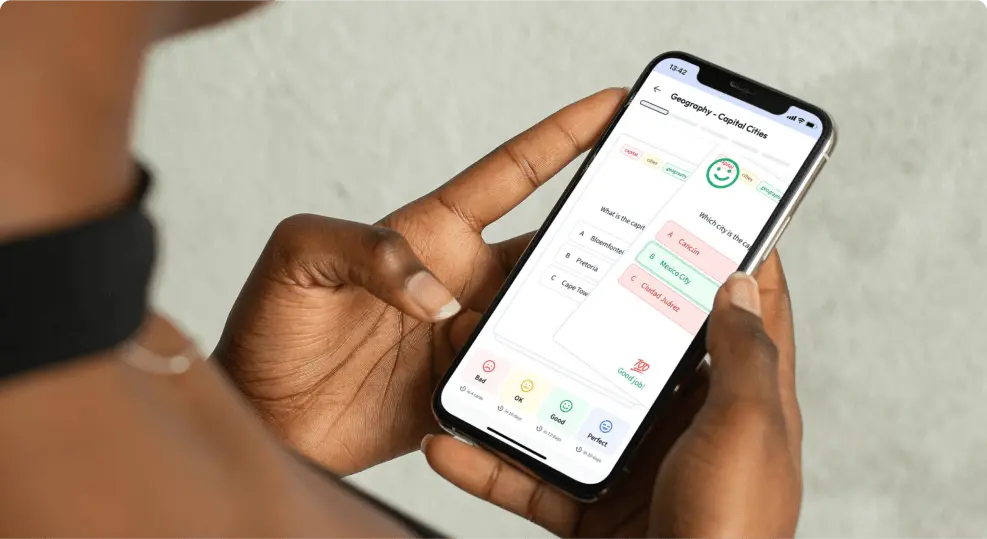
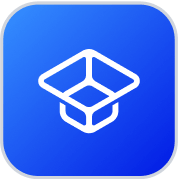
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more