Understanding Javascript Inheritance
Javascript Inheritance is a fundamental concept in programming. Although JavaScript operates differently from many other languagesthat support object-oriented programming, understanding inheritance, especially prototype inheritance, is crucial to levelling up your JavaScript programming skills.Javascript Inheritance Definition: The Basics
As you delve into JavaScript coding, you will encounter a concept known as Javascript Inheritance.Inheritance in JavaScript allows you to create objects which inherit properties from other 'parent' objects, thus reducing duplicacy and making your code more efficient.
- JavaScript uses prototypes instead of classes for inheritance.
- JavaScript Inheritance is chiefly made possible via the prototype chain.
let parent = { name: 'Parent', age: 50, greet: function() { console.log('Hello'); } }; let child = Object.create(parent);In the code snippet above, the child object inherits properties and methods from the parent object.
The role of Javascript Inheritance in Object-oriented Programming
Object-oriented Programming, also known as OOP, is a popular coding methodology that is based on the concept of 'objects'.The principle of Object-oriented Programming is to create, manipulate, and interact with objects within a system.
Given the prototype-based nature of JavaScript, Inheritance enables objects to inherit properties and functionalities from other objects, reducing code redundancy and improving program efficiency.
Insight into Javascript Prototype Inheritance
An oft-used type of inheritance in JavaScript is Prototype Inheritance, which, as the name suggests, works through prototypes.Prototype Inheritance, unique to JavaScript, involves objects inheriting directly from other objects.
- Every JavaScript object has an internal property referred to as [[Prototype]].
- JavaScript objects are equipped with a link to a 'prototype' object.
Applying Javascript Prototype Inheritance in Coding Ventures
But how does Prototype Inheritance come in handy during actual coding? Here's a telling example:Suppose there is a 'Vehicle' function that holds properties such as speed, mileage etc. If you now want to create a 'Car' function that shares the same properties but also has additional properties, you could use Prototype Inheritance. The 'Car' function would then inherit the properties of the 'Vehicle' function, and you can go ahead and add more properties to 'Car'. This is a simple yet powerful way to re-use code, reduce redundancy and enhance code readability.
function Vehicle(speed, mileage) { this.speed = speed; this.mileage = mileage; } function Car(color) { this.color = color; } Car.prototype = new Vehicle(100, 10);In the example of code above, the Car function is using the prototype property to inherit the properties of the Vehicle object.
Practical Application of Javascript Inheritance
The application of Javascript Inheritance in practical development processes is something every developer should familiarise themselves with. This involves implementing Functional Inheritance or Object-Oriented Inheritance, based on the requirements of your task.Functional Javascript Inheritance Example
Taking things forward, let's initially consider a simple example scenario to demonstrate functional inheritance in JavaScript. Consider that you have a generic object, say, a 'Vehicle'.function Vehicle(type, speed) { this.type = type; this.speed = speed; } Vehicle.prototype.describe = function() { return "This " + this.type + " moves at " + this.speed + " mph."; }A 'Car' and 'Plane' can be considered as specific variants of a 'Vehicle'. Both 'Car' and 'Plane' share certain properties with 'Vehicle' but have their own distinct properties too. Through Functional Inheritance, you can establish this relationship.
function Car(type, speed, color) { Vehicle.call(this, type, speed); this.color = color; } function Plane(type, speed, altitude) { Vehicle.call(this, type, speed); this.altitude = altitude; }As evident from the above code snippet, the Car and Plane objects inherit the properties of the Vehicle object using the `Vehicle.call(this, type, speed);` feature of Functional Inheritance.
Tips for Using Function Inheritance in Javascript Effectively
Utilising Javascript Inheritance in a practical context requires proficiency and a keen understanding of some specifications.- Always use the 'new' keyword to create a new object using a constructor function.
- Be careful when setting the prototype of an object - Javascript might use the constructor prototype by default.
- Rely on 'instanceof' if you need to check whether an object is an instance of a particular constructor.
- Remember that methods allocated via the 'prototype' keyword are shared amongst all instances of an object, which makes your code more memory efficient.
Discovering Javascript Object-Oriented Inheritance
Another form of Inheritance worth exploring is Object-Oriented Inheritance. In Javascript, this style of inheritance is facilitated mainly through the class syntax. Consider an example with a 'Parent' class and a 'Child' class, with the 'Child' class inheriting from the 'Parent' class.class Parent { constructor(name, age) { this.name = name; this.age = age; } } class Child extends Parent { constructor(name, age, grade) { super(name, age); this.grade = grade; } }In this scenario, the 'Child' class extends the 'Parent' class, thereby including the all properties of the 'Parent' in addition to its own. This is Object-Oriented Inheritance in action.
The Importance of Javascript Object-Oriented Inheritance in Web Development
While Object-Oriented Inheritance with Javascript might not seem that different from Functional Inheritance, it carries its own weight when it comes to certain aspects.- It provides clearer, more intuitive syntax and is easier to understand for developers coming from a class-based language background.
- It can help organise your code into more coherent and maintainable structures, particularly when your codebase becomes complex.
- It allows subclassing or extending classes, which opens up avenues for more flexible and robust code.
Navigating Through Javascript Multiple Inheritance
The concept of Javascript Multiple Inheritance, although not supported directly, can be a tricky terrain to manoeuvre. Let's demystify this for you.Handling Javascript Multiple Inheritance: A Guide for Students
Javascript Multiple Inheritance forms a part of the vast arrays of inheritance techniques.Multiple Inheritance refers to a feature of some object-oriented programming languages in which a class or an object can inherit characteristics and features from more than one parent class or object.
function SuperHero(name, power){ this.name = name; this.power = power; } function Flying(powerOfFlight){ SuperHero.call(this, 'Flying Hero', 'Fly'); this.powerOfFlight = powerOfFlight; } Flying.prototype = new SuperHero();In this example, you might consider the Flying SuperHero a product of multiple inheritance. But in reality, this is just Function Borrowing or Copying. The SuperHero properties are borrowed or copied to the Flying function, but this is not multiple inheritance because Flying does not have a direct link or prototype chain to the SuperHero's prototype object. However, multiple inheritance in JavaScript can be achieved through indirect ways, like using mixin or the copying methods.
The Controversies Surrounding Javascript Multiple Inheritance
Despite its compelling benefits, multiple inheritance often bears the brunt of criticism. The chief among them concerns the Diamond Problem.The Diamond problem is an ambiguity that arises when a class inherits from two classes, and those two classes, in turn, inherit from a common superclass. This often leads to ambiguity in method resolution.
Strategies for Implementing Multiple Inheritance in Javascript
There are several techniques that you can adopt to work around the initial hurdle of lack of built-in multiple inheritance support in JavaScript. Here is a comprehensive layout of two widely-used strategies: mixins and copying.Practical Roadmap: Multiple Inheritance with Mixins
Here, we discuss mixins, poring over what they are and their application to achieve multiple inheritance.A Mixin is a class which contains methods that can be used by other classes without the need for inheritance.
let flyMixin = function(obj) { obj.fly = function() { console.log("Flying!"); } }; let bird = { name: "Sparrow", speed: "10 m/s" } flyMixin(bird); bird.fly();A key advantage of mixins lies in the modular nature in which the functions are written, leading to organised and manageable code structure.
Harnessing Multiple Inheritance with Copying
Multiple inheritance in JavaScript can also be implemented by copying properties from one object to another. This typically involves two steps:- Create an instance of the parent class.
- Copy the properties of the instance to the child class.
function Dog(name, breed) { this.name = name; this.breed = breed; } function Cat(name, color) { this.name = name; this.color = color; } function CatDog(name, breed, color) { Dog.call(this, name, breed); Cat.call(this, name, color); }Here, the CatDog function is created by copying properties from both the Dog and Cat function using the `call()` method. While this might sound like a dream come true, it segues into the issue of property collision if the parent classes have a property of the same name. As such, leveraging this strategy demands careful design considerations.
Real-world cases: How Multiple inheritance in Javascript drives Complex Projects
Despite the tricky paths you have to navigate, Multiple Inheritance has an immense bearing on real-life coding ventures. From enhancing your code reusability to enriching objects with multiple behaviours, it can be a potent tool to streamline sophisticated projects. A case in point is game development, particularly in defining game characters with multiple abilities. Through multiple inheritance, you can conveniently equip a single character class with visual, audio and physics properties, inherited from their corresponding parent classes. However, you must remember that implementing multiple inheritance in your projects requires a sound understanding of JavaScript principles and astute planning to circumvent potential pitfalls.Javascript Inheritance - Key takeaways
- Javascript Inheritance: A fundamental programming concept where objects inherit properties from parent objects, essential for efficient coding and reduction of duplicacy.
- Javascript Prototype Inheritance: A particular type of inheritance in javascript involving objects inheriting directly from other objects. Each JavaScript object has an internal property ([[Prototype]]) and is equipped with a link to a 'prototype' object.
- Functional Inheritance Example: Demonstrates how objects like 'Car' and 'Plane', considered specific variants of a 'Vehicle', can inherit properties of 'Vehicle'. This process involves the use of a construct function and the 'new' keyword.
- Javascript Object-Oriented Inheritance: Another inheritance in javascript, facilitated mainly through class syntax. Here, a Child class can inherit all the properties of a Parent class, e.g., 'Child' class extends the 'Parent' class.
- Javascript Multiple Inheritance: A feature in some object-oriented programming languages allowing a class to inherit characteristics from more than one parent class. However, Javascript does not directly support this feature due to issues like the Diamond problem. Multiple inheritance in Javascript can be achieved through indirect ways like using mixins or copying methods.
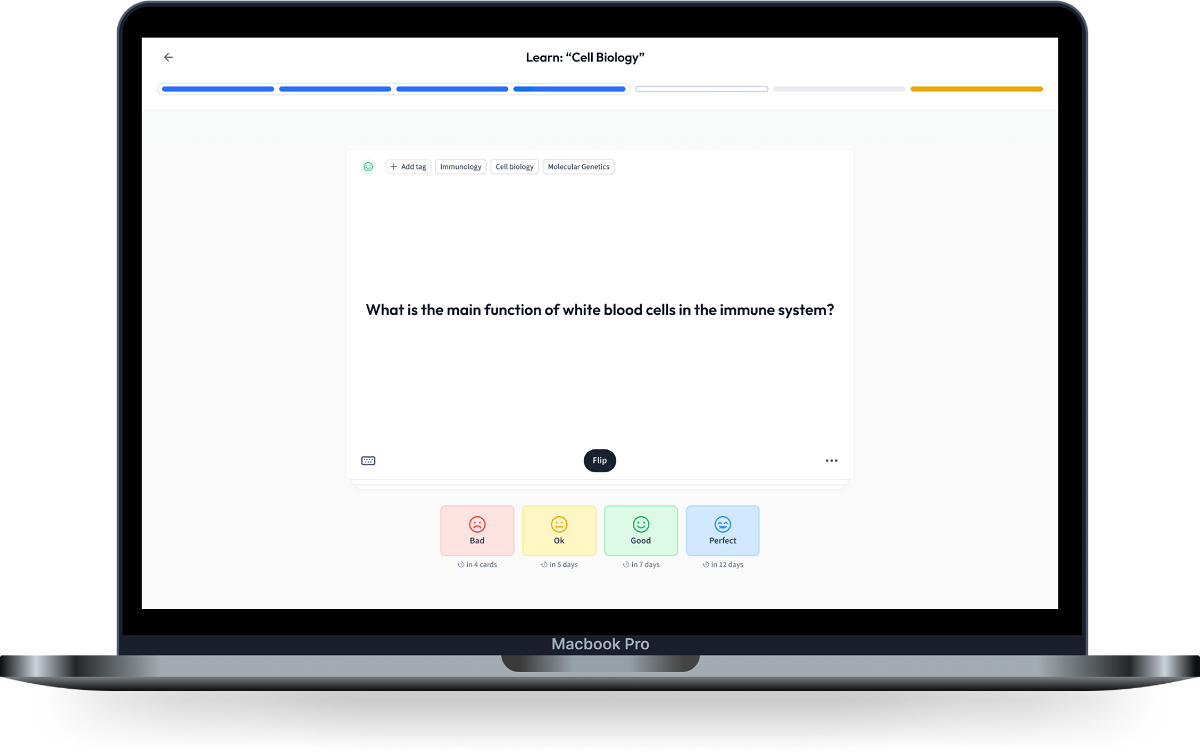
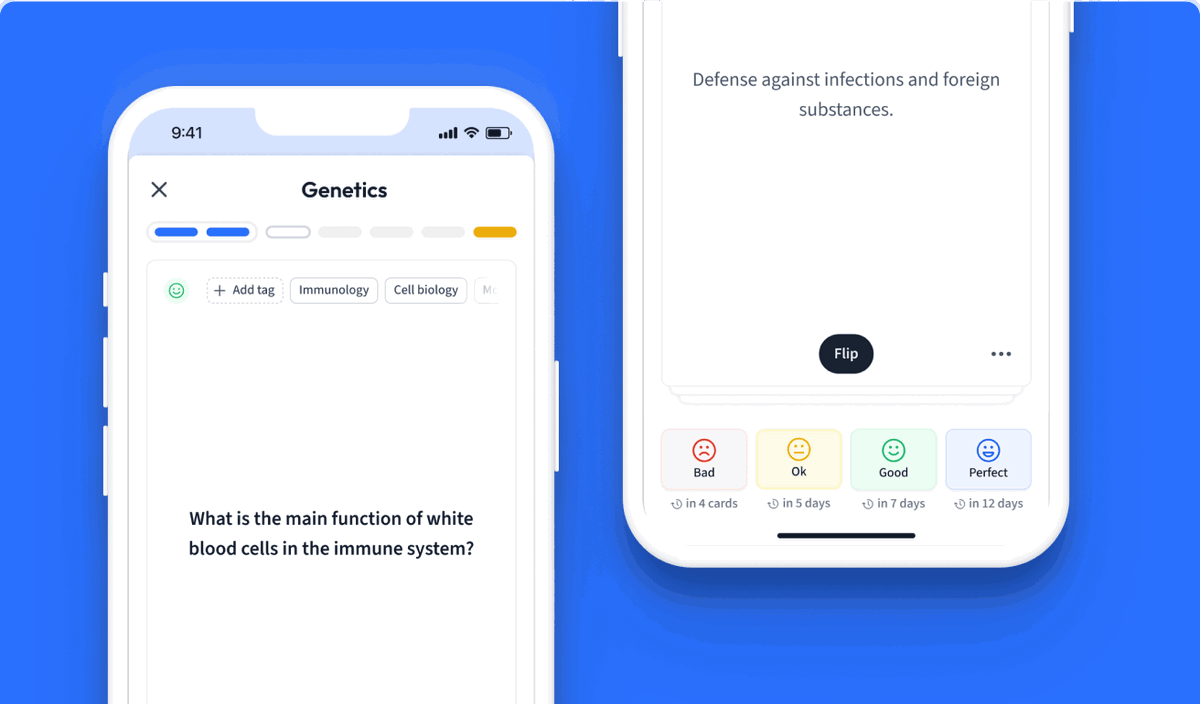
Learn with 12 Javascript Inheritance flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript Inheritance
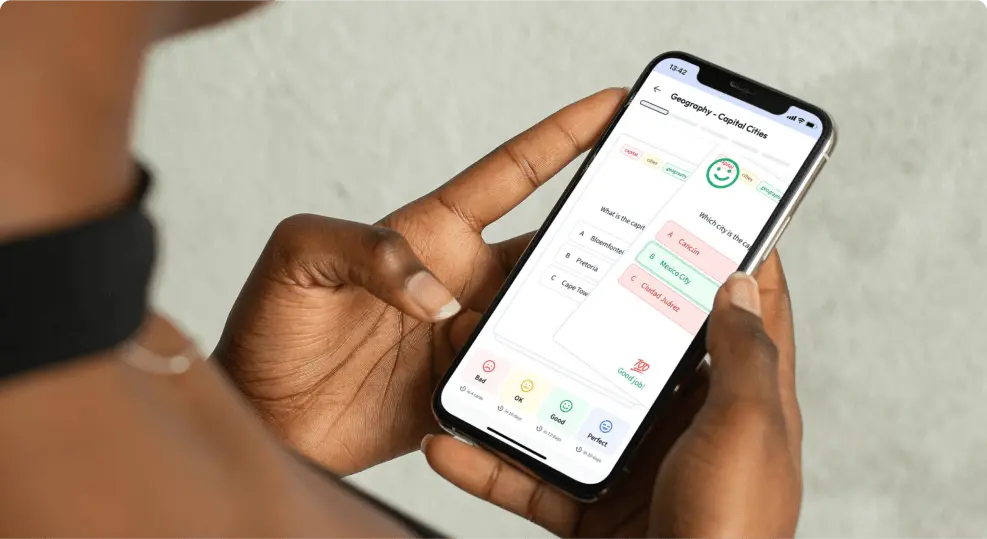
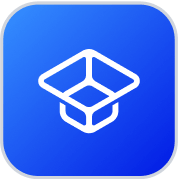
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more