Exception Handling in Computer Science
Exception Handling plays a vital role in software development, ensuring that programs run smoothly and efficiently while handling unexpected conditions, errors, and failures during program execution. Now, let's dive deeper and explore the fundamentals of Exception Handling in Java.
Key Concepts of Exception Handling in Java
Exception Handling in Java is a mechanism to handle runtime errors, allowing normal program flow to continue. The main goal is to ensure the graceful handling of errors and prevent system crashes or unexpected results. The primary concepts in Java Exception Handling are:
- Exception
- Thrown
- Try
- Catch
- Finally
- Throw
- Throws
An Exception is an unwanted event occurring during the execution of a program, which can disrupt the normal flow of control. It usually occurs due to incorrect data, user input, or external resources like network and file operations.
Types of Exceptions in Java
In Java, exceptions are primarily classified into two main types:
- Checked Exceptions
- Unchecked Exceptions
Checked Exceptions are those that the compiler checks during the compilation process. Unchecked Exceptions, on the other hand, are runtime exceptions that the compiler does not check (such as NullPointerException, ArrayIndexOutOfBoundsException, etc.).
Default Exception Handling in Java
When an exception occurs, and a proper user-defined exception handler is absent, the Java Runtime Environment (JRE) handles it by default. This process is known as Default Exception Handling. In this case, the JRE will:
- Print the exception message
- Display the class name and line number where the exception occurred
- Terminate the program abruptly
When the exception is caught using user-defined exception handlers, it allows for a more graceful and informative handling of the error.
Custom Exception Handling in Java
Custom Exception Handling refers to defining your own exception handling mechanism to suit specific program requirements. The main components of Custom Exception Handling in Java can be broken down as follows:
- Try block: The code that may produce an exception is enclosed within a try block.
- Catch block: If the exception occurs, it is caught and handled inside the catch block.
- Finally block: The code within the finally block executes regardless of whether an exception occurred or not, perfect for cleaning up resources.
Exception Handling in Java with Examples
Let's see a simple example of Custom Exception Handling in Java:
try { int[] arr = new int[5]; arr[7] = 9; // Exception will occur here } catch (ArrayIndexOutOfBoundsException e) { System.out.println("Array index out of bounds, please check your code."); } finally { System.out.println("Finally block executed."); }
In this example, we have enclosed the code prone to causing an ArrayIndexOutOfBoundsException within a try block. If the exception occurs, it is caught and custom message is printed. Finally, the code inside the finally block is executed.
Common Scenarios of Exception Handling in Computer Programming
Let's explore some common scenarios where Exception Handling comes in handy:
- File handling: Handling FileNotFoundException, IOException, etc., when working with files.
- Handling user input: Validating and checking the input provided by the user.
- Database connectivity: Handling SQLException while connecting and executing queries on a database.
- Network connections: Handling network-related exceptions, such as UnknownHostException, ConnectException, etc.
- Working with APIs: Managing unexpected responses and errors from external APIs.
Exception Handling helps improve the robustness and stability of software applications by gracefully handling errors, providing useful feedback to users, and avoiding system crashes. Understanding the key concepts and common scenarios can help you become a more proficient programmer with the ability to handle exceptions effectively.
Exception Handling - Key takeaways
Exception Handling: a critical concept in Computer Science, manages errors and unexpected events during program execution.
Types of Exceptions in Java: Checked Exceptions (compiler-checked), Unchecked Exceptions (runtime errors).
Default Exception Handling: Java Runtime Environment (JRE) handles exceptions by printing the message, displaying a class name and line number, and terminating the program.
Custom Exception Handling in Java: Includes try, catch, and finally blocks to deal with specific program requirements.
Common scenarios for Exception Handling: File handling, user input validation, database connectivity, network connections, and working with APIs.
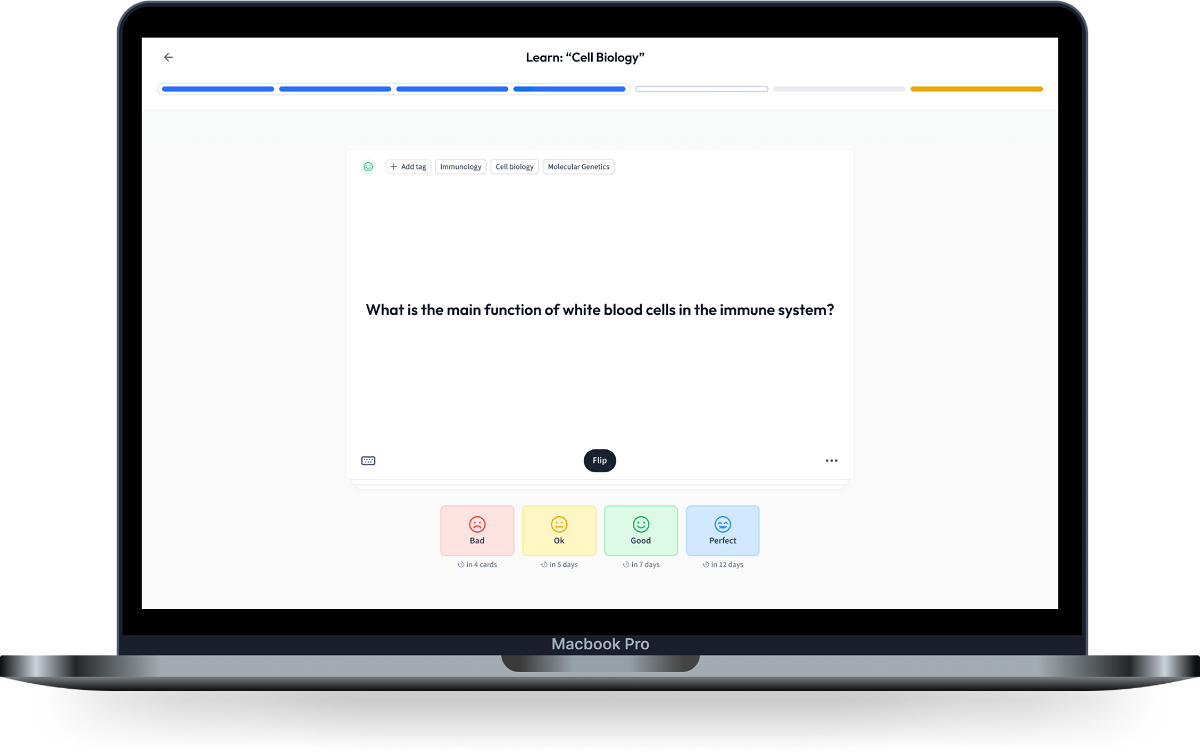
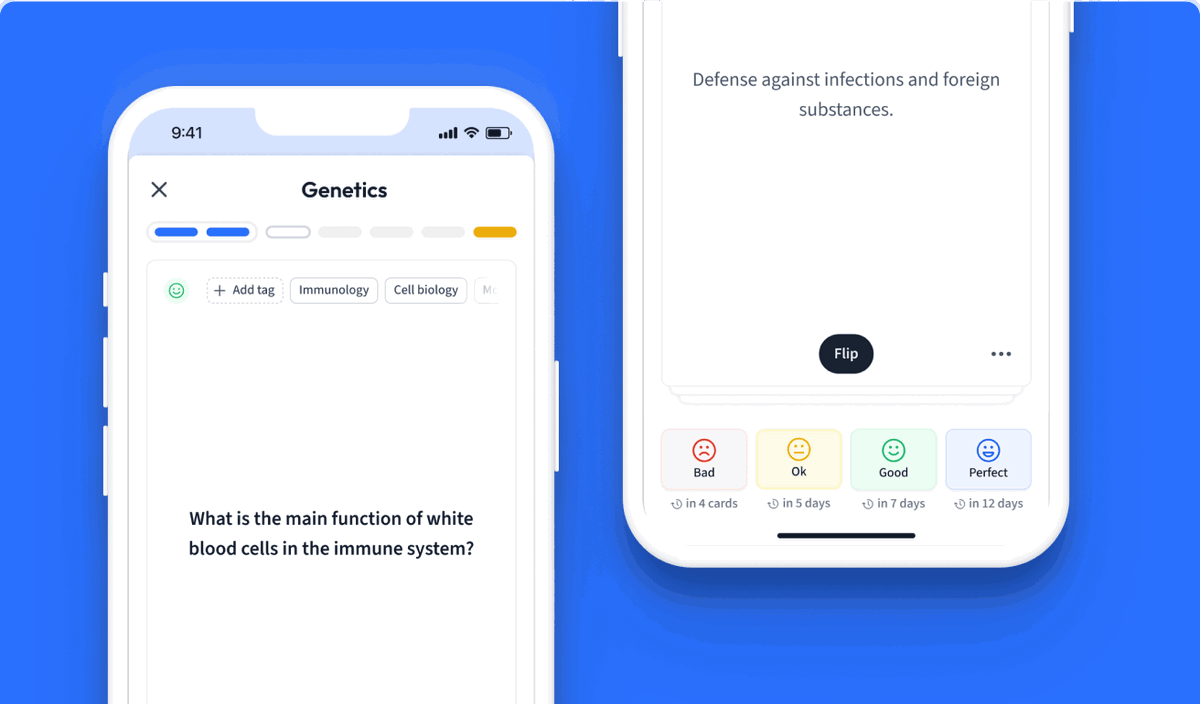
Learn with 14 Exception Handling flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Exception Handling
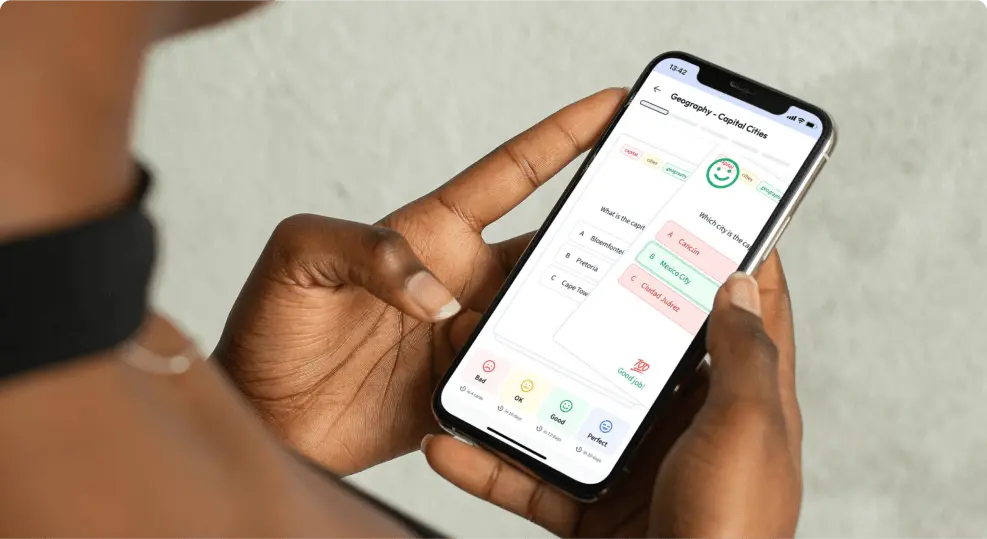
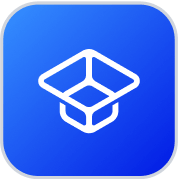
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more