Basics of Structures in C
Structures in C are a powerful tool that allows you to group variables of different data types under a single name. They can be used to store and manipulate complex data efficiently.
A structure is a user-defined data type in C, consisting of a collection of variables, which can be of different data types, grouped together under a single name.
Structures help to organize data in a meaningful way, making it easier to perform operations on specific parts of the data or the entire set. Some common applications of structures include storing details of students, employees, or geometric shapes such as points and rectangles.
Defining and Declaring Structures in C
To define a structure in C, you need to use the `struct` keyword, followed by the structure name and its members enclosed within curly braces. Each member should have a name and a data type. The general syntax of defining a structure is:
struct structure_name { data_type member1; data_type member2; ... };
For example, to define a structure for student details, you can write:
struct Student { int roll_number; char name[30]; float marks; };
After defining a structure, you can declare structure variables and start using them in your programs. To declare a structure variable, you need to use the `struct` keyword followed by the structure name and the variable name. You can optionally initialize the structure variable while declaration. Here's an example:
struct Student s1; // declaring a structure variable 's1' of type Student struct Student s2 = {101, "John", 78.5}; // declaring and initializing a structure variable 's2'
Accessing Structure Members in C
To access and manipulate the members of a structure variable, you need to use the dot (.) operator. The syntax for accessing structure members is:
structure_variable.member
Continuing with the Student structure example, if you want to assign the roll number, name, and marks to the 's1' variable, you can write:
s1.roll_number = 100; strcpy(s1.name, "Alice"); // use strcpy for copying strings s1.marks = 87.5;
Similarly, you can access the values of the structure members and perform operations on them, e.g., displaying the student details on the screen:
printf("Roll number: %d\n", s1.roll_number); printf("Name: %s\n", s1.name); printf("Marks: %.2f\n", s1.marks);
Nested Structures in C
In situations where a structure member needs to store complex information, you can use nested structures. Nested structures are structures within other structures. You can define a nested structure by including a structure variable or pointer as a member of another structure.
For example, you can define a structure called 'Address' to store address details and include it as a member of the 'Employee' structure:
struct Address { int house_number; char street[40]; char city[40]; }; struct Employee { int employee_id; char name[30]; struct Address address; };
In this example, the 'Employee' structure has an 'Address' structure as its member, which allows you to store address details for each employee. This way, you can easily access and modify individual address details through the nested structure.
To access and manipulate the members of a nested structure, you use multiple dot (.) operators. The syntax for accessing nested structure members is:
structure_variable.outer_member.inner_member
For instance, you can access and assign values to the nested Address members of the 'Employee' structure like this:
struct Employee e1; e1.employee_id = 1; strcpy(e1.name, "David"); e1.address.house_number = 10; strcpy(e1.address.street, "Baker Street"); strcpy(e1.address.city, "London");
Using structures in C can greatly improve the organization and management of your data, making it easier to work with complex and nested information.
Arrays of Structures in C
In certain situations, you might want to store a collection of structures where each structure represents an individual record with multiple fields. An array of structures is the perfect solution for such cases. It makes it simple and efficient to manage and manipulate these records by using array indexing.
Declaring and Initializing an Array of Structures in C
To declare an array of structures, you need to define the structure, then declare an array variable of that structure type. The syntax for declaring an array of structures is:
struct structure_name array_name[array_size];
For example, to declare an array of 100 'Student' structures, you can write:
struct Student students[100];
You can also initialize an array of structures while declaring it. To do this, use curly braces to define the values for each structure element in the array, separated by commas. Here's an example:
struct Student students[] = { {1, "Alice", 90}, {2, "Bob", 85}, {3, "Charlie", 78} };
Working with Arrays of Structures in C
Once you have declared an array of structures, you can easily access and manipulate its elements using array indices and the dot (.) operator. Here are some common operations you might want to perform with an array of structures:
- Assigning values to an array of structures: You can use the assignment operator (=) to set values for individual structure members within an array. For example:
students[0].roll_number = 101; strcpy(students[0].name, "David"); students[0].marks = 91;
- Accessing values of an array of structures: To access the values of individual structure members within an array and perform operations on them, use array indices and the dot (.) operator. For instance, displaying the student details for the student at index 2:
printf("Roll number: %d\n", students[2].roll_number); printf("Name: %s\n", students[2].name); printf("Marks: %.2f\n", students[2].marks);
- Looping through an array of structures: You can use loops to traverse through the array of structures and perform specific actions or operations on each element. For example, calculating the average marks of all students in the list:
int i; float total_marks = 0, average_marks; for (i = 0; i < 100; i++) { total_marks += students[i].marks; } average_marks = total_marks / 100; printf("Average marks: %.2f\n", average_marks);
Linked Lists in C
A linked list is a dynamic data structure that can grow or shrink during the program's execution. Instead of using an array, it uses pointers to connect list elements called nodes. Linked lists allow the easy insertion and deletion of elements without the need to move or resize other elements, as in the case of arrays.
Creating and Manipulating Linked Lists in C
To create a linked list, you need to define a structure representing the list's nodes and a structure pointer pointing to the next node in the list. The general syntax of defining a node type structure is:
struct Node { data_type data; struct Node* next; };
Here's an example of defining a node structure for a linked list of integers:
struct Node { int data; struct Node* next; };
Once you have defined the node structure, you can create a linked list by allocating memory dynamically for each element during runtime. To allocate memory for a new node, use the `malloc()` function and initialize the node's members:
struct Node* new_node = (struct Node*) malloc(sizeof(struct Node)); new_node->data = 42; new_node->next = NULL;
You can add, delete, or manipulate nodes in the linked list using pointers. For instance, to insert a new node at the beginning of the list, you can update the head pointer:
new_node->next = head; head = new_node;
Similarly, to traverse a linked list and perform operations on each node, you can use a loop and a temporary pointer:
struct Node* temp = head; while (temp != NULL) { printf("%d\n", temp->data); temp = temp->next; }
Stacks and Queues in C
Stacks and queues are linear data structures that follow specific rules for adding and removing elements. A stack follows the Last In First Out (LIFO) principle, where the most recently added element is removed first. A queue follows the First In First Out (FIFO) principle, where the oldest element in the list is removed before newer elements.
In C, you can implement stacks and queues using arrays or linked lists. The choice of data structure depends on your specific requirements and the trade-offs between memory usage, performance, and the ease of implementation.
Some common stack and queue operations include:
- Push/Add: Insert an element into the stack or queue.
- Pop/Remove: Remove an element from the stack or queue.
- Peek: View the top or front element without removing it from the stack or queue.
- IsEmpty: Check whether the stack or queue is empty.
When working with stacks and queues, you should carefully manage the pointers or array indices to ensure that the data structure follows the LIFO or FIFO principle, ensuring proper addition and removal of elements.
Initializing and Manipulating Structures in C
Initializing a structure is the process of setting the initial values of a structure variable's members. Proper initialization is crucial to prevent undefined behaviour due to uninitialized variables. There are various ways to initialize structures in C, and it's essential to understand each method to use them effectively in your programs.
Initializing Structure Members
There are multiple ways to initialize the members of a structure in C. The most common methods involve using curly braces, individual member assignments, or a combination of these methods:
- Using curly braces: You can initialize a structure variable using curly braces to specify the values for each member in the order of declaration. The syntax for initializing a structure with curly braces is:
struct structure_name variable_name = {value1, value2, ...};
For example, for the Student structure defined earlier:
struct Student s1 = {100, "Alice", 87.5};
- Assigning values to individual members: Another way to initialize a structure is by assigning values to individual members during or after the declaration of the structure variable. You can use the assignment operator (=) to set the initial values for each member. For example:
struct Student s2; s2.roll_number = 101; strcpy(s2.name, "Bob"); s2.marks = 82.5;
Both methods of initialization are valid and can be used depending on the specific requirements of your program.
Copying Structures in C
Copying structures in C involves duplicating the content of one structure variable to another. Since structures are user-defined data types, the assignment operator (=) can be used to copy one structure variable to another if both variables are of the same structure type. The syntax for copying a structure is:
destination_structure = source_structure;
For example, to copy the details of the 's2' Student structure to another structure variable 's3':
struct Student s3; s3 = s2;
It's important to note that this method of copying structures performs a shallow copy, meaning that if a structure member is a pointer, only the pointer's value will be copied and not the data it points to. In such cases, a deep copy would be necessary by copying the data pointed to by the pointer member manually.
Functions and Structures in C
Functions are fundamental building blocks in C, and combining them with structures allows you to create more modular, efficient, and reusable code. Functions can accept structures as parameters and return structures as results, enabling you to encapsulate your program's logic related to data manipulation effectively.
Passing Structures to Functions
The process of passing structures to functions in C can be accomplished in two ways: by value or by reference. The choice between the two methods depends on the specific requirements of your function and whether you need to modify the original structure or merely access its members.
- Passing structures by value: To pass a structure by value, you provide the structure variable as an argument to the function, and the function receives a copy of the structure. This method allows the function to access and modify the structure members without affecting the original data. The syntax for passing a structure by value is:
function_name(struct structure_name variable)
For example:
float calculate_percentage(struct Student s); // Calling the function float percentage = calculate_percentage(s1);
Keep in mind that passing structures by value can result in performance issues for large structures, as the entire structure is copied, leading to increased memory usage and processing time.
- Passing structures by reference: To pass a structure by reference, you provide a pointer to the structure variable as an argument to the function. This method allows the function to access and modify the original structure directly, but it requires careful management of pointers. The syntax for passing a structure by reference is:
function_name(struct structure_name* variable)
For example:
void update_student_marks(struct Student* s, float new_marks); // Calling the function update_student_marks(&s1, 89.5);
Passing structures by reference can result in improved performance, especially for large structures, as only the pointer is passed to the function, reducing memory usage and processing time.
Returning Structures from Functions
Functions in C can return structures as their result, allowing you to create reusable code segments for data manipulation and processing. When returning structures from functions, you can either return a structure directly or return a pointer to a structure.
Returning structures directly: To return a structure directly, you need to specify the structure type as the return type of the function and use the return statement to return a structure variable. For example:
struct Student create_student(int roll_number, char* name, float marks) { struct Student s; s.roll_number = roll_number; strcpy(s.name, name); s.marks = marks; return s; } // Calling the function struct Student s4 = create_student(102, "Eve", 94);
Returning a structure directly creates a copy of the structure, which might result in performance issues for large structures.
Returning structure pointers: Alternatively, you can return a pointer to a structure by allocating memory dynamically using the `malloc()` function. This method requires careful memory management to avoid memory leaks or undefined behaviour due to accessing uninitialized or freed memory. For example:
struct Student* create_student_pointer(int roll_number, char* name, float marks) { struct Student* s = (struct Student*) malloc(sizeof(struct Student)); s->roll_number = roll_number; strcpy(s->name, name); s->marks = marks; return s; } // Calling the function struct Student* s5 = create_student_pointer(103, "Frank", 88);
When using pointers to structures, always ensure to free the memory allocated by `malloc()` after you are done using the structure to avoid memory leaks.
Pointers and Structures in C
In C, pointers play a crucial role in managing memory and optimizing the performance of your programs, especially when used in combination with structures. This powerful combination allows you to work with large, complex data efficiently and reduce memory usage significantly.
Using Pointers with Structures
Pointers can be used to access and manipulate structures in several ways, including pointing to structures, performing structure pointer arithmetic, and dynamically allocating memory for structures. By understanding these concepts, you can take full advantage of their potential to create efficient and well-organized code.
Pointer to Structure in C
In C, you can declare a pointer to a structure by specifying the structure type, followed by an asterisk (*) and the pointer name. To assign a memory address to a structure pointer, you can use the address-of operator (&) in combination with the structure variable. Here are some essential aspects of using pointers with structures:
- Declaring a pointer to a structure: You need to declare a structure pointer by specifying the structure type followed by an asterisk (*) and the pointer name. For instance:
struct Student *ptr;
- Assigning memory address to the structure pointer: To assign the memory address of a structure variable to the structure pointer, use the address-of operator (&) like this:
struct Student s1; ptr = &s1
- Accessing structure members using pointers: To access a structure member through the structure pointer, use the arrow (->) operator. For example:
ptr->roll_number = 104; strcpy(ptr->name, "George"); ptr->marks = 95;
Structure Pointer Arithmetic in C
Unlike pointers to other data types, you cannot perform arithmetic operations (+, -, ++, --) directly on pointers to structures. This restriction exists because structure size may vary for different instances, and pointer arithmetic expects fixed-size elements. However, you can work around this limitation by using pointers to the members of a structure and performing arithmetic operations on those member pointers.
For instance, if you have an array of structures and want to move to the next or previous structure, you can create a loop that iterates through the structure members' pointers and updates them accordingly:
struct Student students[5]; int* roll_number_ptr = &students[0].roll_number; for (int i = 0; i < 5; i++) { // Access and modify the structure member using the pointer *roll_number_ptr = i + 1; // Move to the next structure in the array roll_number_ptr += sizeof(struct Student) / sizeof(int); }
Dynamic Memory Allocation
Dynamic memory allocation refers to the process of allocating memory during a program's execution, rather than specifying a fixed-size memory block at compile-time. Using pointers with dynamic memory allocation for structures allows you to create flexible and efficient programs that can handle varying amounts of data.
Allocating and Deallocating Memory for Structures in C
To allocate and deallocate memory for structures dynamically, you need to use the `malloc()` and `free()` functions, respectively. These functions enable your programs to request and release memory as needed, providing better control over memory usage and improving performance.
- Allocating memory for structures: You can allocate memory for a structure using the `malloc()` function, which accepts the size of the memory block to be allocated (typically using the `sizeof()` function) and returns a pointer to the allocated memory. For instance:
struct Student* ptr = (struct Student*) malloc(sizeof(struct Student));
When using the `malloc()` function, remember to cast the returned pointer to the appropriate structure type and check for NULL pointers, which indicate memory allocation failure.
- Deallocating memory for structures: After you have finished using a dynamically allocated structure, you should deallocate the memory using the `free()` function to avoid memory leaks. The `free()` function accepts the pointer to the memory block and returns no value. To deallocate memory for the previously allocated structure, you can write:
free(ptr); ptr = NULL;
Always ensure to set the pointer to NULL after deallocating the memory to prevent dangling pointer issues, which can cause undefined behaviour.
Advanced Topics in Structures in C
Unions in C
Unions in C are a powerful feature similar to structures that allow you to group variables of different data types under a single name. However, unlike structures, unions store all of their members in the same memory location, which means that only one member can hold a value at a time. Unions are useful when you need to store multiple data types in a single variable without wasting memory space, making them particularly valuable for saving memory in resource-constrained environments.
Differences Between Structures and Unions in C
Though structures and unions share similarities in syntax and usage, they have key differences that make them suitable for different scenarios. Here are the main differences between structures and unions:
- Memory allocation: Structures allocate separate memory locations for each member, while unions allocate a single memory location and share it among all members. As a result, structures typically require more memory than unions.
- Member access: In structures, all members can store values simultaneously, allowing access to multiple members at once. However, in unions, only one member can hold a value at a time, limiting access to a single member.
- Use cases: Structures are ideal for grouping related variables of different data types, whereas unions are suitable for creating variables that can store multiple data types interchangeably.
To declare and use a union, the syntax is similar to that of a structure. They also use the keyword 'union', followed by the union name and members enclosed within curly braces:
union union_name { data_type member1; data_type member2; ... };
For example, if you want to define a union that can store an integer, a float, or a character, you can write:
union Data { int i; float f; char c; };
When using a union, remember that only one member can hold a value at any given time and accessing other members during that period can lead to unexpected results.
Enums and Bitfields in C
Enumerations in Structures in C
Enumerations (enums) are a user-defined data type in C that allows you to assign names to integer constants, improving code readability and maintainability. Enums can be used as structure members to store discrete values efficiently.
To define an enumeration type, use the keyword 'enum' followed by the enumeration name and enumeration constants enclosed within curly braces. Each constant in the enumeration list automatically gets assigned an integer value, starting from 0:
enum enum_name { constant1, constant2, ... };
For instance, if you want to define an enumeration type to represent different grading levels, you can write:
enum Grade { A, B, C, D, E, F };
To use an enumeration as a member of a structure, define it before the structure definition and include it as a member by specifying the enumeration name:
struct Student { int roll_number; char name[30]; float marks; enum Grade grade; };
Using enumerations in structures can make your code more readable and help avoid errors related to incorrect constant values.
Using Bitfields with Structures in C
Bitfields are a powerful feature in C that allows you to specify the number of bits used for a structure member, enabling optimized memory usage for narrowly-tailored fields like flags, statuses, and small integer values.
To define a bitfield in a structure, use the 'unsigned' keyword followed by the member name and the number of bits to be allocated for the member within colons:
struct bitfield_structure { unsigned member1 : number_of_bits1; unsigned member2 : number_of_bits2; ... };
For example, you can define a structure with bitfields for storing multiple Boolean flags:
struct Flags { unsigned flag1 : 1; unsigned flag2 : 1; unsigned flag3 : 1; };
The Flags structure would only require 3 bits of memory, rather than allocating 1 byte for each unsigned integer, creating a more efficient memory usage in your programs.
Keep in mind that using bitfields can come with trade-offs in terms of performance, as manipulating individual bits might be slower than working with standard integers. However, for applications where memory is a significant constraint, bitfields offer a valuable tool for optimizing structure sizes and reducing memory usage.
Structures in C - Key takeaways
Structures in C: Group variables of different data types under a single name
Array of structure in C: Store a collection of structures for efficient management and manipulation of records
Initializing a structure in C: Set initial values of structure variable's members to prevent undefined behaviour
Data structures in C: Arrays, linked lists, stacks, and queues for more efficient programming
Declare structure in C: Use the 'struct' keyword followed by the structure name and its members
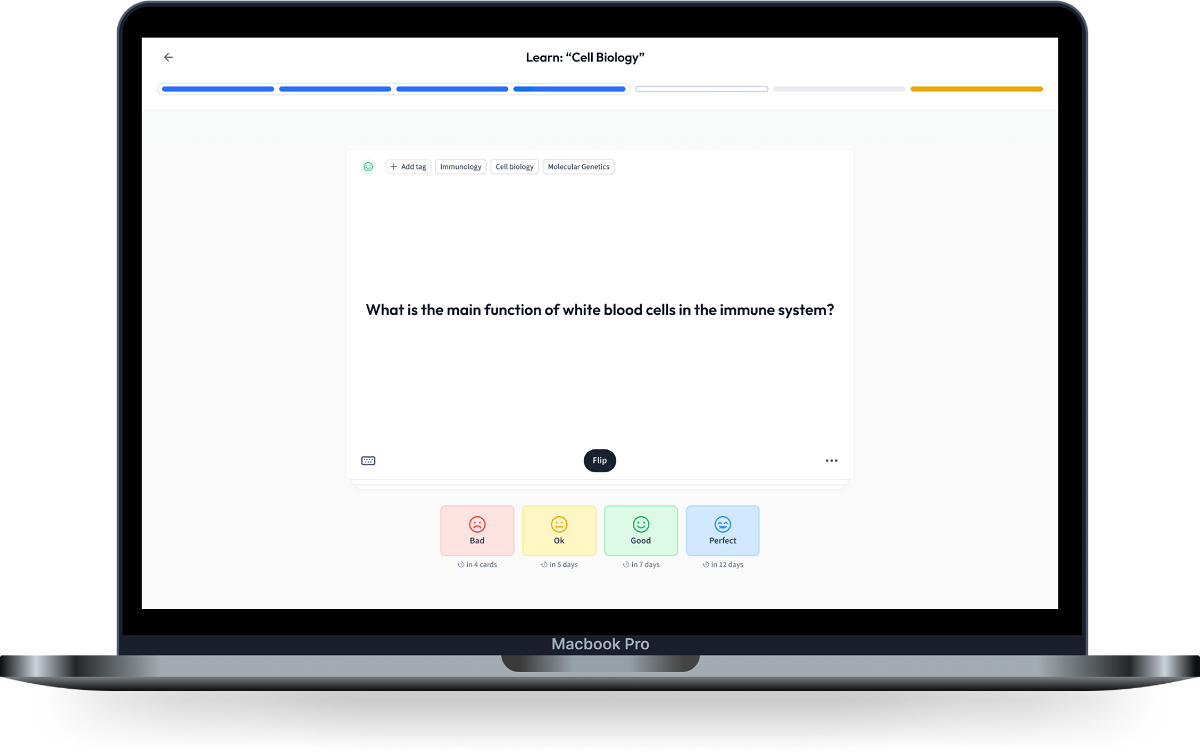
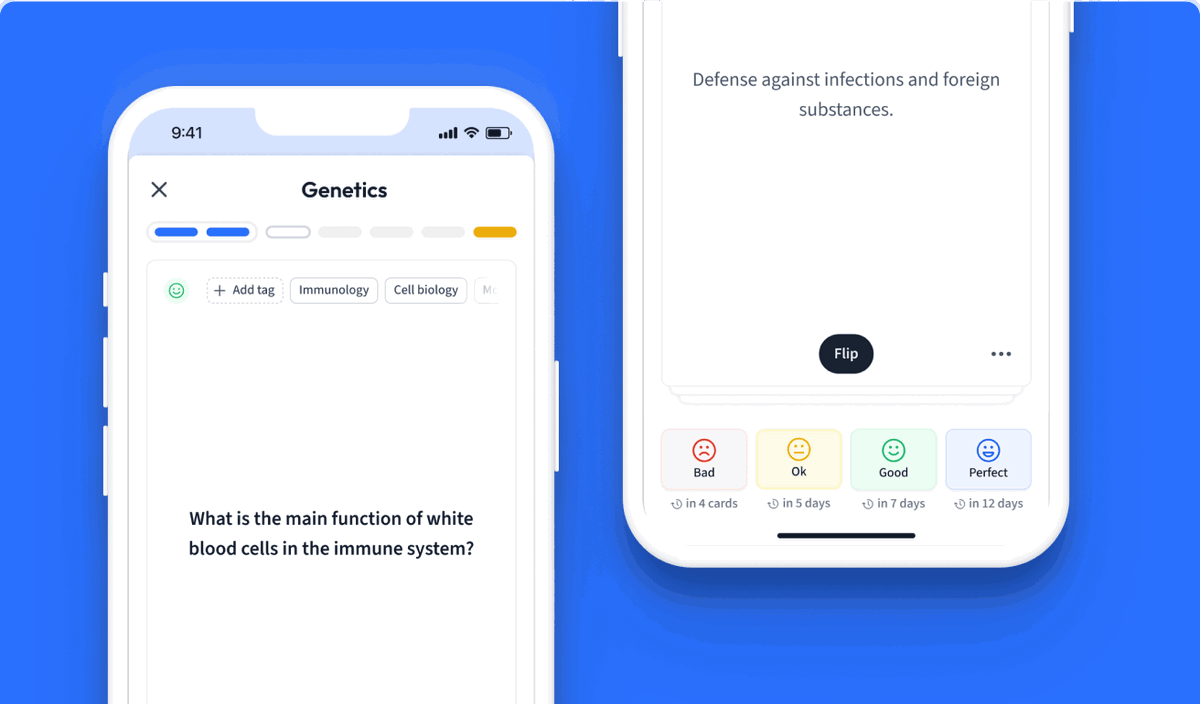
Learn with 53 Structures in C flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Structures in C
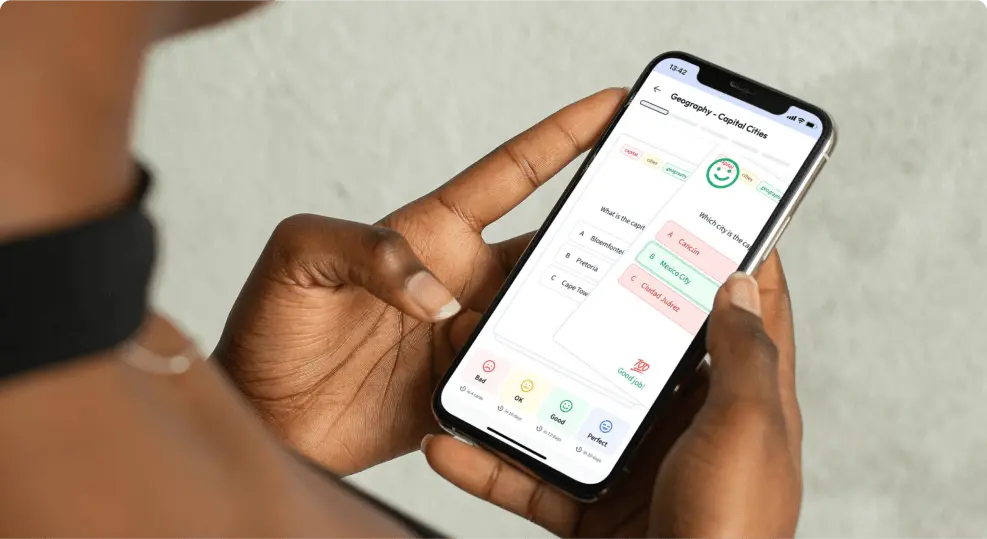
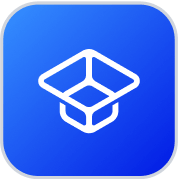
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more