Understanding Python Subplots
Python subplots are a concept in data visualization that are incredibly useful for organizing multiple graphs or plots in a systematic manner. In computer programming and data science, it's often necessary to compare different datasets, analyze trends and patterns, and gain insights from visual representations of data. Python subplots offer the advantage of displaying multiple plots on a single figure, which makes it easier for you to draw comparisons and convey important information in a concise and effective way.
Benefits of using Python Subplots in Computer Programming
There are numerous benefits of using Python subplots in your computer programming and data visualization tasks:
- Efficient use of screen space: Python subplots enable you to optimize screen space by displaying multiple plots side by side or in a grid format.
- Enhanced data comparison: Placing multiple plots in proximity allows you to easily compare and correlate trends and patterns within different datasets.
- Improved organization: Python subplots are conducive to a neat and organized presentation of visual elements, making your work more comprehensible and effective.
- Customizability: Subplots also offer a high degree of flexibility and customizability in terms of the size, layout, and arrangement of the individual plots.
- Easier sharing and exporting: Consolidating multiple plots into a single figure simplifies the process of sharing and exporting visualizations to different formats, such as image files or PDFs.
Python's popular library, Matplotlib, offers powerful functionality to create subplots, adjust their appearance, and interact with the data through various tools and resources.
Different types of Python Subplots
In Matplotlib and other data visualization libraries, there are several ways to create subplots depending on the specific requirements and desired outputs. The following techniques are commonly used to create Python subplots:
matplotlib.pyplot.subplots(nrows, ncols): This function generates a grid of subplots with a specified number of rows and columns, where nrows and ncols represent the number of rows and columns, respectively. It returns a figure object and an array of axes objects which can be used to customize individual subplots.
For example, to create a 2x2 grid of subplots:
import matplotlib.pyplot as plt
fig, ax = plt.subplots(nrows=2, ncols=2)
matplotlib.pyplot.subplot(nrows, ncols, index):
This function creates a single subplot within a grid specified by nrows and ncols and activates the subplot at the given index. Indexing starts from 1 and follows a row-wise order.
For example, to create and activate a subplot at the top-left corner of a 2x2 grid:
import matplotlib.pyplot as plt
ax1 = plt.subplot(2, 2, 1)
matplotlib.pyplot.subplot2grid(shape, loc, rowspan, colspan):
This function allows you to create subplots within a grid specified by the shape parameter (rows, columns) at a given location (loc) and with optional rowspan and colspan arguments to span multiple rows or columns. This provides more control over the layout and positioning of subplots within the grid.
To create a subplot spanning two rows and one column, starting at the top-left in a 3x2 grid of subplots:
import matplotlib.pyplot as plt
ax1 = plt.subplot2grid((3, 2), (0, 0), rowspan=2, colspan=1)
Each of these methods has its own advantages and offers a certain level of flexibility and customization for various data visualization needs. The choice of method ultimately depends on your specific requirements and the complexity of the subplots' arrangement.
How to Create Subplots in Python
In Python, creating subplots is a convenient and efficient way to display multiple plots in a single figure. You can use various methods in libraries like Matplotlib to create subplots, arrange them in a suitable structure, and customize their appearance. It is important to follow best practices during the process to ensure a high-quality and informative visualization. In this section, we will discuss how to create subplots using 'for loop' and explore some basic methods and best practices for creating subplots in Python.
Creating subplots in for loop python
One common approach to create multiple subplots is by using a 'for loop' in Python. This approach is particularly useful when you have a large number of plots or want to automate the process of creating subplots based on a given dataset. Here's how you can create subplots using a 'for loop':
- Import the required libraries, such as Matplotlib.
- Define the layout or grid structure for the subplots.
- Iterate through the dataset and create individual subplots within the loop.
- Customize and format each subplot according to your requirements.
- Show or save the resulting figure with all subplots.
For example, let's assume we have a dataset containing data for 12 different categories, and we want to create a 4x3 grid of subplots to visualize the trends for each category:
import matplotlib.pyplot as plt
# Dataset with 12 categories
categories = ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L']
# Define the grid structure
nrows, ncols = 4, 3
# Create the figure and axes objects
fig, axes = plt.subplots(nrows, ncols, figsize=(12, 16))
# Iterate through the dataset and create subplots
for i, category in enumerate(categories):
row, col = i // ncols, i % ncols
ax = axes[row, col]
# Generate example data for the plot (replace with real data)
x = range(0, 10)
y = [j * (i+1) for j in x]
ax.plot(x, y)
ax.set_title(f'Category {category}')
# Show the figure
plt.tight_layout()
plt.show()
Using a 'for loop' enables you to efficiently create and customize multiple subplots within a single figure. This approach is particularly useful when working with large datasets and complex grid structures.
Create subplots python: Basic methods and best practices
There are several basic methods to create subplots in Python that can help you achieve the desired results. By following the best practices, you can create organized and effective subplots efficiently. Here, we will discuss common methods and best practices for creating subplots in Python:
- Choose an appropriate subplot layout: The layout of the subplots, including the number of rows and columns, should be chosen based on the number of plots you wish to display and their arrangement. Make sure the grid is large enough to accommodate all subplots and their corresponding labels.
- Use the appropriate subplot creation function: Matplotlib provides various functions to create subplots, including 'subplot()', 'subplots()', and 'subplot2grid()'. Choose the function that best suits your requirements and provides the desired level of customization and control over the subplot layout.
- Customize individual subplots: Modify the appearance of individual subplots, such as the x and y-axis labels, title, legend, and plot style, to convey the intended information effectively and consistently across all subplots.
- Adjust the spacing between subplots: Use the 'tight_layout()' function or manually adjust the spacing between subplots with 'subplots_adjust()' to ensure proper spacing between subplots and improve readability.
- Export and share the resulting figure: Once you have finalized the subplots, save the resulting figure in an appropriate format for sharing or further analysis.
Following these best practices can help you create effective and informative Python subplots while ensuring efficient use of screen space and optimal readability. Adhering to these methods will ensure that your data visualization tasks are performed successfully and in line with your project requirements.
Advanced Python Subplots Techniques
In this section, we'll discuss some advanced techniques to create subplots in Python, focusing on manipulating subplot sizes, creating interactive bar charts, and adding subplot legends to enhance your data visualizations.
Python Subplots size adjustment
Adjusting the size of your subplots is crucial for improving the readability and accurate display of your data. There are several ways to customize the size of your subplots in Matplotlib, including adjusting the figure size, customizing the aspect ratio, and controlling the subplot margins.
To adjust the size of your subplots, consider the following methods:
- Adjusting the figure size: The overall size of the figure containing your subplots can greatly impact the appearance and readability of your subplots. You can control the figure size using the 'figsize' parameter in the 'plt.subplots()' function:
For example, to create a 3x3 grid of subplots with a custom figure size of (10, 10):
import matplotlib.pyplot as plt
fig, axs = plt.subplots(3, 3, figsize=(10, 10))
- Customizing the aspect ratio: The aspect ratio of your subplots – the ratio of the width to the height – can also have a significant effect on the overall appearance of your data. You can adjust the aspect ratio of your subplots by setting the 'aspect' parameter while creating each subplot:
Suppose you want to create a subplot with an aspect ratio of 2 (i.e. width is twice the height):
import matplotlib.pyplot as plt
fig, axs = plt.subplots()
axs.set_aspect(2)
- Controlling the subplot margins: The subplot spacing and margins can also impact readability and appearance, affecting the amount of space between subplots, as well as the padding around the figure boundaries. You can adjust the margins between subplots using the 'subplots_adjust()' function:
For instance, to adjust the left, right, top, and bottom margins, along with the width and height spacing between subplots:
import matplotlib.pyplot as plt
fig, axs = plt.subplots(3, 3, figsize=(10, 10))
plt.subplots_adjust(left=0.1, right=0.9, top=0.9, bottom=0.1, wspace=0.2, hspace=0.2)
By carefully adjusting the size of your subplots, modifying the aspect ratio, and controlling the subplot margins, you can create more visually appealing and informative data representations.
Subplots bar chart python: Creating interactive graphs
Crafting interactive bar charts within subplots can greatly enhance the user's experience when exploring data. An interactive graph allows users to hover over data points, pan, zoom, and display tooltips containing additional information. You can achieve this interactivity in your Python subplots using libraries like Plotly Express.
To create interactive subplot bar charts, follow these steps:
- Install the required library: To use Plotly Express, you'll need to install the library by running the command:
pip install plotly-express
- Import the library: Import Plotly Express in the script:
import plotly.express as px
- Prepare the data: Organise your data in a Pandas DataFrame with appropriate column names and indices.
- Create the subplot bar chart: Utilise the 'plotly.subplots.make_subplots()' function to create the subplot layout, specifying the number of rows and columns, as well as additional settings like shared axes and subplot titles.
- Customize the bar chart: Add the desired traces to create a complete interactive bar chart, customising the appearance and behaviour of the resulting plots.
- Show the interactive graph: Finally, display the interactive graph using the 'show()' method.
By creating interactive graphs with subplots, you can provide an engaging and informative experience for users navigating through your data visualizations.
Adding a Python Subplots legend for enhanced visualisation
A legend is an essential element for data visualization, as it helps users understand the meaning of the different data points, lines, and markers in a plot. In Python, you can add legends to your subplots using the Matplotlib library.
To add a legend to your subplots, consider the following steps:
- Create subplots: Start by creating your subplots using the 'plt.subplots()' function, specifying the grid layout (e.g., rows and columns) and any additional parameters like figure size.
- Customize your subplots: For each individual subplot, add your data, customize the plot appearance, and set appropriate labels, titles, and legends.
- Adding the legend: To add a legend to a particular subplot, use the 'legend()' function with the desired location and additional parameters, such as the font size, the number of columns, and the frameon (to specify whether to display a border around the legend).
- Displaying and exporting the figure: Lastly, adjust the spacing between subplots (using 'plt.tight_layout()' or 'plt.subplots_adjust()') and display or save the final figure.
An example of adding a legend to a 2x2 grid of subplots:
import matplotlib.pyplot as plt
fig, axs = plt.subplots(2, 2, figsize=(10, 10))
for i in range(2):
for j in range(2):
ax = axs[i, j]
ax.plot([0, 1], [0, i+j], label=f'Line {i+j+1}')
ax.legend(loc='upper left', fontsize=10)
ax.set_title(f'Subplot {i+1}-{j+1}')
ax.set_xlabel('X-axis')
ax.set_ylabel('Y-axis')
plt.tight_layout()
plt.show()
Utilizing legends in your subplots enhances visualisation by providing additional context to your data, making it easier for users to interpret and comprehend your plots.
Python Subplots - Key takeaways
Python Subplots: A concept in data visualization that enables organizing multiple plots systematically in a single figure for efficient data comparison and organization.
Create subplots in for loop python: Can automate the process of creating subplots by iterating through datasets and generating individual subplots within the loop.
Subplots bar chart python: Interactive bar charts created with libraries like Plotly Express, allowing pan, zoom and display tooltips for more engaging visualizations.
Python Subplots size: Customizable in Matplotlib through adjusting the figure size, aspect ratio, and subplot margins for better readability and visual appearance.
Python Subplots legend: Enhances visualisation by providing additional context to the data, making it easier for users to interpret and comprehend plots.
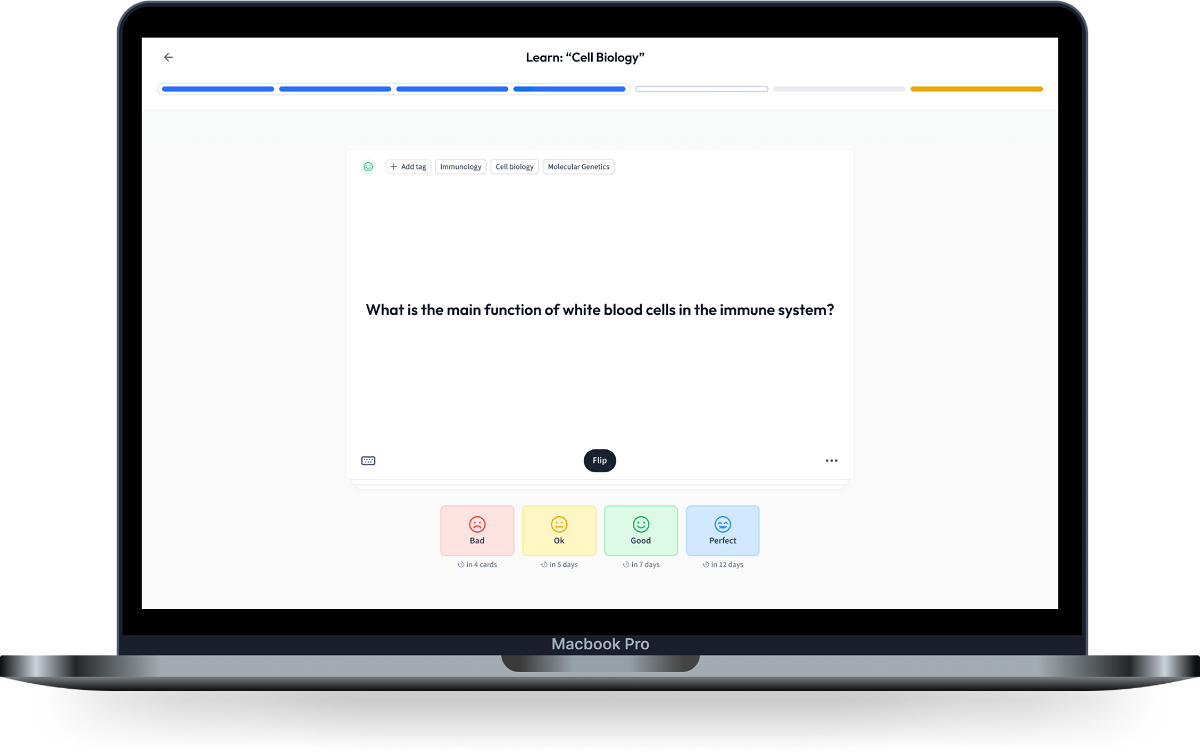
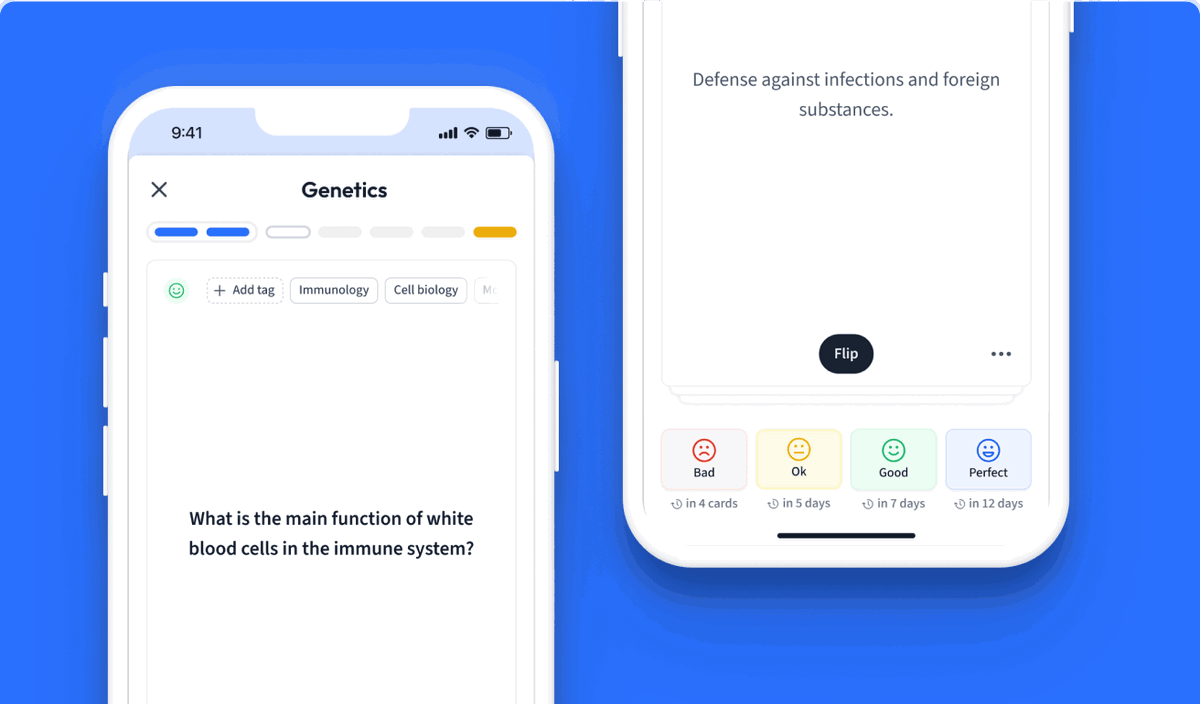
Learn with 30 Python Subplots flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Python Subplots
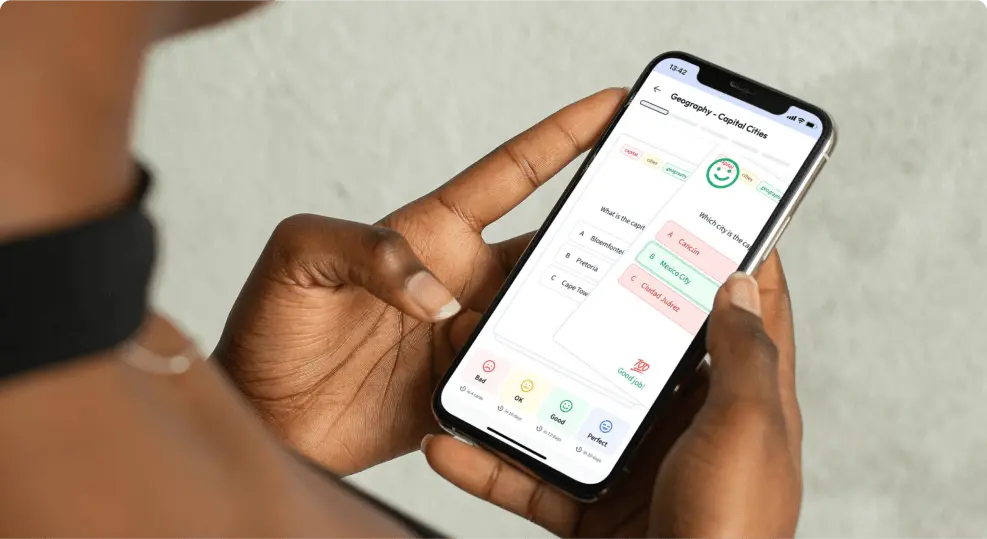
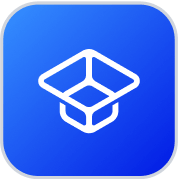
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more