Understanding Java Logical Operators
Java Logical Operators play a pivotal role in making decisions in Java programming. As a programmer, you would use these operators to build logic within your code by combining two or more boolean expressions.
Defining the Concept of Java Logical Operators
Logical operators in Java are used to manipulate boolean values directly and to make complex decisions based on multiple boolean expressions. With these operators, you can generate sophisticated logic in your applications by developing conditions that consider multiple variables.
Let's dive deeper into these operators. There are three commonly used Java logical operators:
- Logical AND (&&)
- Logical OR (||)
- Logical NOT (!)
The Logical AND operator (&&) returns true if both the boolean expressions are true.
The Logical OR operator (||) returns true if either or both of the boolean expressions are true.
The Logical NOT operator (!) returns the opposite of the boolean expression to which it is applied.
Characteristics of Java Logical Operators
The characteristics of the logical operators in Java are unique, which influences their application in intricate programming conditions.
Operator | Characteristic |
Logical AND (&&) | Returns false as soon as it finds the first false expression, hence it is referred to as a short-circuit operator. |
Logical OR(||) | Returns true as soon as it finds the first true expression, hence it is also referred to as a short-circuit operator. |
Logical NOT(!) | Is an unary operator, thus it works on a single operand. |
A unique characteristic of Java logical operators, particularly AND (&&) and OR (||), is their ability to "short-circuit". Short-circuiting implies that the Java Virtual Machine (JVM) evaluates expressions from left to right and stops as soon as the full condition is unmistakably established.
Evolution of Java Logical Operators over Time
Logical operators have remained constant in Java over time, providing the foundational logic assessments in the language since its inception. However, its implementation has drastically improved in Java's vast libraries and frameworks.
For instance, the use of logical operators has evolved into the Stream API in recent versions of Java to facilitate more advanced and concise data processing logic.
What remains most vital is for you as a programmer to understand and implement these operators skilfully within your codes in all contexts. Only with practice can you fully grasp the notion of Java Logical Operators to construct powerful and flexible logical structures in your Java programs.
Delving into Boolean Logical Operators in Java
There's no denying the considerable impact that Boolean logical operators have in Java. These operators, which revolve around the concept of true and false, create a framework for logical decision-making processes in code.
Importance of Boolean Logical Operators in Java
When it comes to Java programming, Boolean logical operators are indispensable, they serve as the gateway to decision-making in code. With the help of these operators, you can create conditions under which certain parts of your code will run. They enable you to make your Java applications more dynamic and responsive to various situations.
Below are the key reasons why Boolean logical operators are integral to Java programming:
- Conditional Execution: Boolean logical operators form the backbone of conditional statements like if, while, and for. These operators determine whether or not the associated block of code gets executed.
- Control Flow Management: They allow you to control how your application runs under different circumstances. This facet of programming, also known as control flow, is pivotal in creating versatile & adaptable software.
- Reaction to User Input: Boolean operators also contribute to how your applications respond to user inputs, allowing you to create software that adapts to the needs of its users.
To thoroughly understand their importance, we shall explore the two fundamental Boolean logical operators: true and false.
Boolean Logical Operators: True and False in Java
In Java, true and false are the fundamental Boolean operators representing the outcomes of a condition. Each operator is associated with a distinct set of output and behaviours.
Generally, true signifies that a condition has met the desired criteria. Conversely, false denotes that it has failed to do so..
For example, consider the condition "5 > 3". The outcome of this boolean expression is true because 5 is indeed greater than 3. On the other hand, the expression "2 > 3" results in false as 2 is not greater than 3.
boolean isFiveGreater = (5 > 3); // This will be true boolean isTwoGreater = (2 > 3); // This will be false
The true and false logical operators are the basis of all decision-making processes in your Java programming journey.
Unravelling the Myths about Java's Boolean Logical Operators
As key components of Java programming, Boolean Logical Operators are often surrounded by misconceptions which could limit your understanding and use of them:
Myth 1: A widely held myth is that Boolean Logical Operators are equivalent to bitwise operators. The primary difference lies in how they process their operands - while bitwise operators work on a bit level, logical operators work with entire conditions.
Myth 2: Another misconceived notion is that "false" signifies an error or abnormal program termination. However, "false" merely indicates that a particular condition was not met - it's a normal part of program flow and does not signify an error.
Debunking these myths is crucial for you to make the most out of Boolean logical operators in your Java programming.
Mastering the Java Logical AND Operator
This section focuses on one of the mainstays in any programmer's toolkit – the Java Logical AND Operator. As you immerse yourself in Java programming, the importance and prevalence of the logical AND operator becomes more apparent. Virtual everything from intricate algorithms to simple condition-checking includes this essential piece of the Java language.
Unpacking the Functionality of a Java Logical AND Operator
The logical AND operator, represented as '&&', is a binary operator requiring two operands. When both these operands or boolean expressions evaluate to true, the operator returns true. If even a single operand is false, it returns false. This operator forms the basis for developing compound conditional statements in Java.
Delving into the mechanics, it's important to recognise the short-circuit property inherent to the Java logical AND operator. When the left operand of the '&&' operator evaluates as false, the right operand is not evaluated at all. Why? Because it's certain that the overall expression will be false, regardless of the result of the right operand. This property is beneficial when the right operand might have unintended effects or cause exceptions if evaluated.
Consider the expressions detailed in this table:
Expression | Result |
true && true | true |
true && false | false |
false && true | false |
false && false | false |
The table provides a clear understanding of how the logical AND operator works in Java. Keep in mind that the order of expressions matters because of its short-circuit attribute.
Java Logical AND Operator: A Practical Example
Let's illustrate the use of the '&&' operator in a common scenario – age validation. Suppose you're building a Java program that verifies if a user is a teenager, which in this context, means they must be 13 to 19 years old inclusive.
You can accomplish this by utilising the logical AND operator by checking whether the age is greater than or equal to 13 and less than or equal to 19. If both conditions are true, your program will conclude that the user is a teenager.
int userAge = 15; boolean isTeenager = (userAge >= 13) && (userAge <= 19); // This will be true
If you run this example with an input of 15, the boolean 'isTeenager' will be set to true, testifying that a user aged 15 is indeed a teenager. If 'userAge' falls outside of the 13 to 19 range, 'isTeenager' will be set to false.
Java Logical AND Operator: Discover Hidden Facts
There is an interesting facet about the Java Logical AND Operator which is not immediately apparent. That is its associative property, essentially implying that you can chain multiple '&&' operators together without changing their order of evaluation. However, remember that operations continue from left to right due to the short-circuit property.
For instance, if you have an expression like \( (A \&\& B) \&\& C \) it is equivalent to \( A \&\& (B \&\& C) \) or simply \( A \&\& B \&\& C \).
Understanding such little-known aspects of the Java Logical AND Operator is crucial in manipulating it to serve more complex programming needs.
Discerning Bitwise Logical Operators in Java
In the expansive world of Java, understanding bitwise logical operators is key. These operators differ from general logical operators as they operate on individual bits rather than entire conditions, offering the precision required in many computational tasks. This section helps demystify these operators and shed light on their practical applications in Java.
Demystifying Bitwise Operators in Java Language
Moving beyond the veil of simple logic operators in the Java language, you step into the fascinating realm of bitwise logical operators. These powerful tools of computation allow operations at the bit level, enabling more direct manipulation of data.
First, let's talk about what a bit is. In the Java language, a bit represents the most basic unit of information, which can have a binary value of either 0 or 1. With the help of bitwise logical operators, you can compare and manipulate these bits in various ways.
Some of the most commonly used bitwise logical operators in Java are:
- The Bitwise AND Operator (&): This operator returns 1 only when both bits being compared are 1. Otherwise, it yields 0.
- The Bitwise OR Operator (|): Producing a result of 0 only when both bits are 0, this operator returns 1 in all other cases.
- The Bitwise XOR Operator (^): This operator returns 1 when the bits being compared are different, and 0 when they are the same.
- The Bitwise NOT Operator (~): This unary operator flips bits, turning 0 to 1 and 1 to 0.
Behind the Bitwise Logical Operators in Java
To grasp how these operators work in Java, let's dive into each one's individual functioning.
The Bitwise AND Operator (&) is best conceptualised by the expression \( A \text{ Bitwise AND } B = C \), where A and B represent each bit position from two numbers, and C is the corresponding bit in the result. Each bit C[i] is true only if both A[i] and B[i] are true. Here's a quick example demonstrating this:
int a = 6; int b = 4; int result = a & b;
In this example, the numbers 6 and 4 are represented in binary as 110 and 100, respectively. The Bitwise AND operation on these numbers gives 100, which is the binary representation of 4 - the resulting value of 'result'.
Next, let's delve into the Bitwise OR Operator (|). Much like the AND operator, the OR operator works on individual bits too. However, it returns 1 if either of the bits is 1. Continuing with the previous example:
result = a | b;
This code will yield a result of 6, as 110 (6 in decimal) is the result of performing a bitwise OR operation on 110 (6 in decimal) and 100 (4 in decimal).
Finally, the Bitwise XOR Operator (^) and the Bitwise NOT Operator (~) allow for more complex operations by returning 1 when the bits differ and flipping bits, respectively. The XOR operator proves extremely useful in tasks like finding missing numbers, while the NOT operator mostly finds use in turning on/off specific bits.
Working with Bitwise Logical Operators: An Example in Java
Consider a scenario where you want to check if a given number is odd or even. You could use the bitwise AND operator to accomplish this challenge efficiently, with the logic being that if the last bit in the binary representation of a number is 1, the number is odd, and if it's 0, the number is even.
int num = 17; boolean isOdd = (num & 1) == 1;
In this example, the last bit of 'num' (17 in decimal is 10001 in binary) is AND'd with 1 (which is 1 in binary). The result will be 1, and 'isOdd' will be true, indicating that 17 is indeed an odd number. Replace 'num' with an even number, and 'isOdd' will be false.
Developing an understanding of these operators broadens your computational arsenal in the Java language and brings you one step closer to becoming a versatile and proficient Java programmer.
Application of Java Logic Operators Technique
The importance of Java Logic Operators Technique finds expression in numerous programming tasks. From constructing complex evaluation systems to automating basic if-else flow control operations, this technique is fundamental to developing functional and efficient programming solutions. An in-depth understanding of these techniques is invariably beneficial for any aspiring Java developer.
Role of Java Logic Operators Technique in Programming
Java Logic Operators Technique plays a crucial role in building almost any logic-based functionality in a Java program. These operators - AND(&&), OR(||), and NOT(!) - form the nucleus of creating conditional blocks, loops and control structures, making them integral for developing complex algorithms and systems.
Operator | Description | Example |
&& | Logical AND: True if all conditions are true | (5 > 3) && (4 > 2) = true |
|| | Logical OR: True if at least one condition is true | (5 > 3) || (2 > 4) = true |
! | Logical NOT: True if the following condition is false | !(5 < 3) = true |
Furthermore, Java logic operators are heavily involved in conditional decision making. For instance, if you're building an online payment system, logical operators can be used to check if a user's account balance is sufficient and if a specific payment gateway is available, among other conditions.
Java Logic Operators Technique: An In-depth Example
Let's take a concrete example of how Java Logic Operators Technique can be applied. You're tasked with developing an access system for a secure facility where users must fulfil either of these two condition sets to gain access:
- User has 'Admin' role and knows the 'MasterPassword'.
- User has a valid fingerprint registered in the system or has a valid RFID card.
In the Java program for this system, you can use logical operators like so:
public class AccessControl { public static void main(String[] args) { String role = "Admin"; String password = "MasterPassword"; String fingerprint = "VALID"; boolean hasRFIDCard = false; boolean isAdmin = role.equals("Admin"); boolean knowsPassword = password.equals("MasterPassword"); boolean hasFingerPrint = fingerprint.equals("VALID"); if ((isAdmin && knowsPassword) || (hasFingerPrint || hasRFIDCard)) { System.out.println("Access Granted!"); } else { System.out.println("Access Denied!"); } } }
In this code, the '&&' operator checks whether the user is an admin and knows the master password. The '||' operator is used to ensure the user has either a valid fingerprint or an RFID card. The enclosing '||' operator then grants access if the user fulfils either of the two condition sets.
Perks of Mastering the Java Logic Operators Technique
Mastering the Java Logic Operators Technique offers several advantages. It helps build complex conditions effectively and fosters a more profound understanding of flow control in Java. This mastery is beneficial in crafting more efficient, readable, and maintainable code. Discussing in point form, the advantages can be marked as follows:
- Complex Conditional Logic: Logical operators enable the creation of intricate conditions.
- Improved Code Efficiency: These operators help optimise logic checks, improving the performance of your code.
- Better Code Readability: By using logical operators, you're able to make the code more intuitive and easy-to-read for other developers.
- Expanded Toolset: Adding logical operators to your programming toolset increases your capabilities as a Java developer.
Expanding your programming toolset with the Java Logic Operators technique gives you a key to unlock higher levels of Java programming efficiency and effectiveness. As you continue to explore this foundational technique, remember practice is vital in achieving mastery.
The Power of Java Logical Operators in Programming
Java logical operators stand as an integral part of programming, providing critical components to enable conditional logic and complex decision making in code. Their importance is multifaceted and offers a plethora of benefits across a variety of applications within programming.
Elucidating the Significance of Java Logical Operators in Programming
In the sphere of computer programming, Java logical operators are fundamentally significant and indispensable tools. They hold a prime position in programme routines directed towards decision-making and controlling the flow of execution. Essentially, they are gatekeepers, providing or denying access to certain code segments based on the assigned conditions.
These operators typically include AND(&&), OR(||), and NOT(!). Combining these operators in different ways allows you to create intricate conditions for if-else statements, loops, and complex algorithms, thereby enriching the fabric of code logic.
if ((condition1 && condition2) || !condition3) { // This block of code runs if either both condition1 and condition2 are true // or condition3 is false. }
In the above example, the two conditions in the brackets (condition1 && condition2) must both be true for the code within the block to execute, as the logical AND(&&) operator connects them. If either or both conditions are false, the code segment does not run. The logical OR(||) operator then connects this group with the next condition, 'not condition 3', with the code block executing if this condition doesn't hold. Thus, the whole phrase of logic presents a vivid representation of how Java logical operators offer the flexibility and manipulate the execution flow of Java code.
Java Logical Operators in Programming: Examples in Context
Java logical operators can be highly illustrative when explored through practical examples. Consider a situation where you are creating a security system that grants access to users based on different criteria. This is where the power of Java logical operators shines.
boolean hasSecurityClearance = true; boolean knowsPassword = false; if (hasSecurityClearance || knowsPassword) { System.out.println("Access Granted!"); } else { System.out.println("Access Denied!"); }
In the code snippet above, the system grants access if the user either has security clearance or knows the password. The use of the OR(||) operator here enables the code to provide access based on either of these separate conditions.
Java logical operators also prove significant when working with JavaScript Object Notation (JSON) objects, XML parsing, network programming, and multithreaded programming. From enabling precise control over the sequence of execution to allowing efficient handling of potential errors, logical operators in Java serve as fundamental building blocks in virtually every computational scenario.
Benefits of Implementing Java Logical Operators in Code Writing
Implementing Java logical operators in code fetches numerous benefits—from introducing more advanced conditional logic to rendering code more efficient and readable. Let's elaborate on a few of these:
- Advanced Conditional Logic: Logical operators enable the development of complex conditions and control structures in programming. This ability steps up the range and level of tasks that a Java program can accomplish.
- Efficiency: By enabling logical decisions, these operators contribute tremendously to optimising code performance. The execution flow of the program can be controlled effectively to prevent unnecessary operations.
- Code Readability: Using logical operators enhances the overall readability of the code, making it easier for programmers to understand the flow and logic of the program. This benefit is especially significant during team projects, where multiple developers might work on the same codebase.
Besides, Java logical operators also allow elegant solutions to several programming problems. For instance, to check if an integer is even or odd, one can simply use the AND(&&) operator for bitwise operation with the number one. The code for this operation is:
int number = 5; // or any integer boolean isOdd = (number & 1) != 0;
In this snippet, the code turns true for odd numbers and false for even ones, demonstrating how logical operators can help implement elegant and efficient solutions in Java programming.
Moving into a more advanced scenario, logical operators are immensely helpful in error-handling and debugging, too. Programmers often use them to create 'error flags' or to set, clear, and test bits in a program, thus enhancing the robustness of code and reducing the likelihood of software bugs.
Java Logical Operators - Key takeaways
- The two fundamental Boolean logical operators in Java are true and false, which represent the outcomes of a condition.
- Contrary to common myths, Boolean Logical Operators are not equivalent to bitwise operators and "false" does not signify an error or abnormal program termination.
- The Java Logical AND Operator, represented as '&&', is a binary operator that returns true when both its operands evaluate to true. Due to its short-circuit property, if the left operand evaluates as false, the right operand is not evaluated.
- Bitwise logical operators in Java operate on individual bits, allowing for direct manipulation of data. The most commonly used bitwise operators are Bitwise AND Operator (&), Bitwise OR Operator (|), Bitwise XOR Operator (^), and Bitwise NOT Operator (~).
- The Java Logic Operators Technique, utilising operators like AND (&&), OR (||), and NOT (!), is fundamental to developing complex algorithms and systems, constructing conditional blocks, loops and control structures, and making conditional decisions in Java programming.
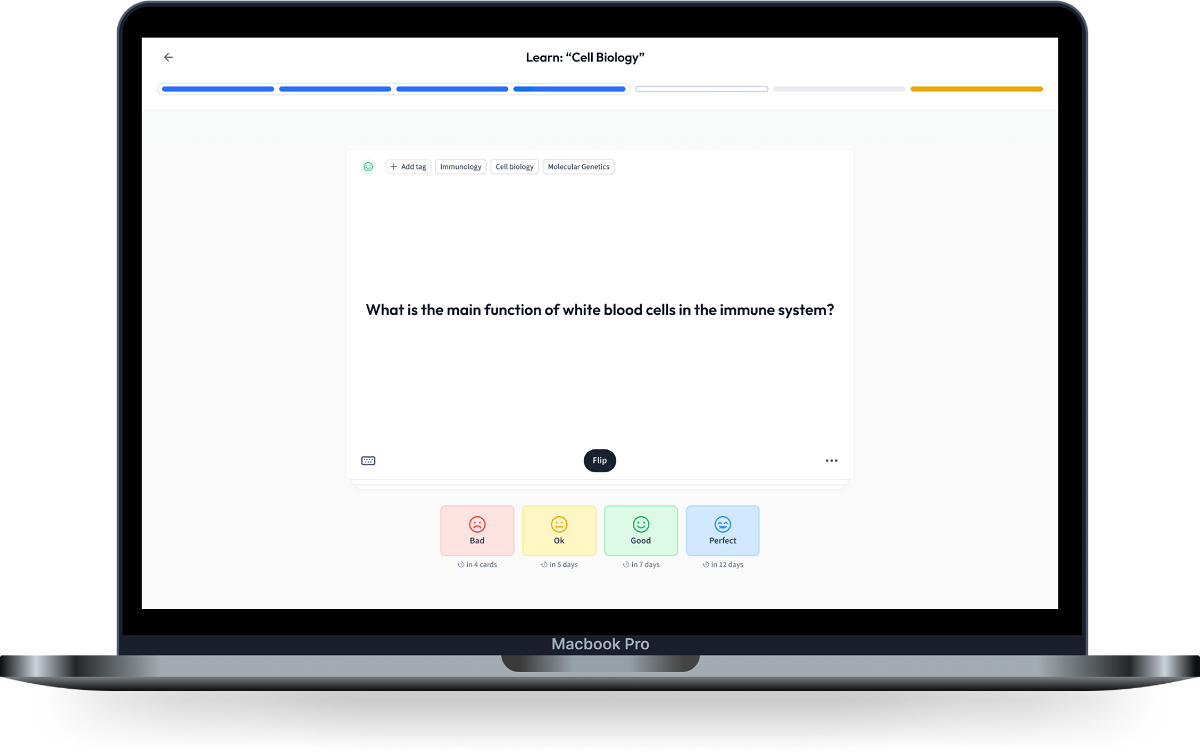
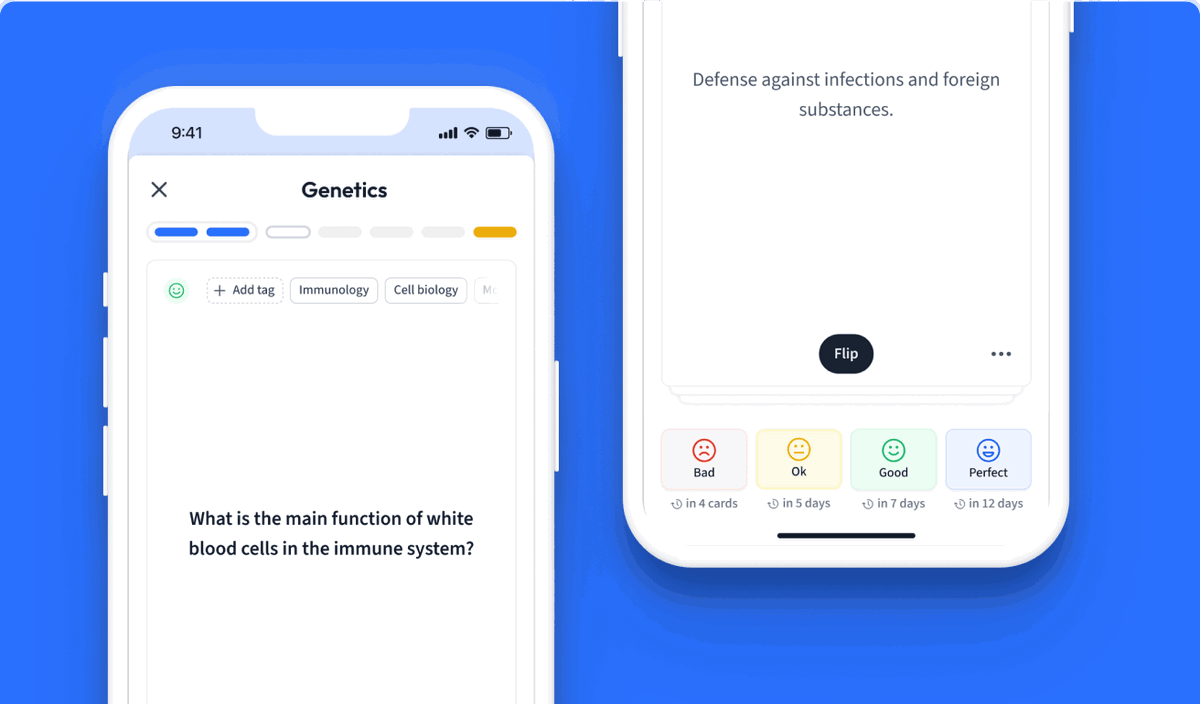
Learn with 12 Java Logical Operators flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Java Logical Operators
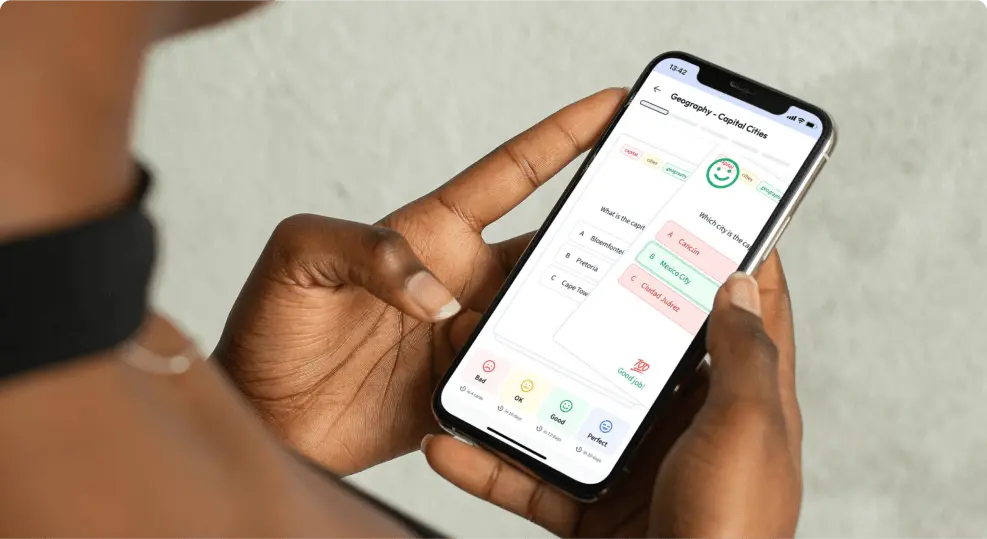
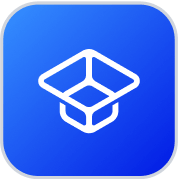
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more