Understanding the Java Switch Statement
In computer programming, you, as a coder, often find yourself in situations where you need to decide between multiple scenarios for a particular situation. The Java Switch Statement is a workaround. It offers a more readable and convenient alternative to a long list of if-then-else scenarios.A Java Switch Statement is a type of control flow statement that allows your code to branch in different ways based on the value of a variable or expression.
What is a Switch Statement in Java?
In Java, a Switch Statement works with the byte, short, char, and int primitive data types. It also works with enumerated types (including a few special classes), String and Wrapper classes. A typical syntax is as follows:switch (expression) { case x: // code block break; case y: // another code block break; default: // yet another code block }
The expression within the switch statement must return an int, char, byte, or short. Each data type allows your program to act according to different scenarios.
Digging Deeper into the Java Switch Statement Concept
Each value specified as a 'case' should be a unique, constant, and literal value. Duplicate case values aren't allowed, and Java will trigger an error in such situations.- case: This defines a constant condition that if met, executes the block of code following the case statement until a break keyword or the closing curly brace } is encountered.
- break: The break keyword signifies the end of each case and is used to take control out of the switch block.
- default: A default case is used when none of the cases is true. This case does not require a break keyword.
Java Switch Statement Logic Explained
Essentially, the flow of control in a switch statement is as follows: 1. Expression in the switch case is evaluated one time. 2. Control of the program jumps to the first matching case value. 3. If there is no match, the default code block is executed (if it exists). The following table displays this:Select Expression | Case 1 | Case 2 | Default Case |
Value 1 | execute | skip | skip |
Value 2 | skip | execute | skip |
Value 3 | skip | skip | execute |
int dayOfWeek = 3; switch (dayOfWeek) { case 1: System.out.println("Monday"); break; case 2: System.out.println("Tuesday"); break; case 3: System.out.println("Wednesday"); break; default: System.out.println("Invalid Day"); }In this example, the variable 'dayOfWeek' is evaluated, and since it matches with 'case 3', it prints 'Wednesday' and breaks out of the switch construct. If 'dayOfWeek' had been 5, it wouldn't have matched any case, resulting in the execution of the default statement.
Writing with the Java Switch Statement
Writing with the Java Switch Statement involves understanding how to structure it, the syntax to use and the step-by-step instructions in developing one. Let's take a closer look at all these elements.Switch Statement Syntax in Java
The syntax of a switch statement in Java is crucial. A switch statement begins with the keyword "switch" followed by an expression in parentheses. The expression is followed by a pair of curly braces, which contain a sequence of "case" labels and an optional "default" label:switch (expression) { case value1: // Statements break; case value2: // Statements break; . . . default: // Default Statements }
Basics of Switch Statement Syntax in Java
There are three basic parts of a switch statement in Java.Expression: This is typically a variable that will be evaluated, and its result will be compared with the values of the cases (value1, value2 ...). The expression must return a single value of type char, byte, short, int, or String.
Case: Each case identifies a value that will be compared with the result of the expression. If a match is found, the code after the case is executed.
Default: This is an optional part of the switch statement. Whenever no case matches, the code within the default case is executed.
How to Write a Switch Statement in Java
Writing a switch statement in Java requires following a set method. First, create a switch block. Then, add each case you want to handle. You'll want to include a break statement for each case, too. Finally, don't forget to include the default case to manage any unexpected values.Step-by-Step Guide on Writing Java Switch Statement
Let’s go through a step-by-step process of writing a Java Switch Statement: Step 1: Define the expression or variable that you want the switch statement to evaluate. This goes just after the "switch" keyword and inside the parentheses. The value of this variable or the result of the expression will determine which case in the switch statement to execute.String day = "Monday"; switch (day) { ... }Step 2: Declare every possible value that your expression might have as a case. After the colon, write the code that you want to execute if the case matches the result of the expression.
switch (day) { case "Monday": System.out.println("Start of the work week"); break; ... }Step 3: Repeat Step 2 for each possible value of your variable or expression. Step 4: Add the "default" clause. This clause will handle all the other situations where none of the declared cases match the value of the expression.
switch (day) { case "Monday": System.out.println("Start of the work week"); break; ... default: System.out.println("Invalid day!"); }Just remember, your switch-cases have to be exhaustive. All potential cases should be covered. Also, bear in mind that Java Switch Statement does not support every data type. You can't use booleans or longs. You can use byte, short, char, int, String, enum types and a couple of special classes like Byte, Short, Char, and Integer.
Exploring Java Switch Statement through Examples
Nothing beats learning more than throwing yourself into the practical realm. Engaging with hands-on examples often provides greater understanding, so here you will explore the Java Switch Statement through comprehensive examples.Example Switch Statement in Java
To kick things off, consider the following simple example of a Java switch case in action:int num = 3; switch (num) { case 1: System.out.println("One"); break; case 2: System.out.println("Two"); break; case 3: System.out.println("Three"); break; default: System.out.println("Invalid number"); }In this Java switch case, you are using the integer variable 'num' in the switch expression. This 'num' is evaluated once, and its value is compared with the values of each case. If the value of 'num' matches 'case 3', "Three" is printed.
Examining an Example of Java Switch Statement
Let's examine an example of a Java switch statement where the switch expression is a string:String day = "Fri"; switch (day) { case "Mon": System.out.println("Monday"); break; case "Tue": System.out.println("Tuesday"); break; case "Wed": System.out.println("Wednesday"); break; case "Thu": System.out.println("Thursday"); break; case "Fri": System.out.println("Friday"); break; case "Sat": System.out.println("Saturday"); break; case "Sun": System.out.println("Sunday"); break; default: System.out.println("Invalid day"); }In this case, the switch statement assesses the string variable 'day'. If 'day' matches 'case "Fri"', "Friday" is printed. The rest of the cases are ignored. If 'day' did not match any of the cases, 'default' would then be executed, and "Invalid day" would be outputted.
How to Use Switch Statement in Java
Using a switch statement in Java involves a few crucial steps. Once the flow is understood, it becomes straightforward to use. Firstly, you initialise your variable or evaluate your constant expression. Secondly, define your switch construct with the variable or expression to be evaluated. Proceed to describe each case you are expecting the variable to have, including a statement to execute if that case matches the variable. Each of these cases needs to be followed by a 'break'.int x = 2; switch (x) { case 1: System.out.println("One"); break; case 2: System.out.println("Two"); break; default: System.out.println("Not One or Two"); }
Practical Tips on Using Java Switch Statement
Here are a few practical tips and best practices when using Java Switch Statement:Tip | Description |
Include a default case | Always include a default case as a safety measure to handle any unexpected value of the variable or expression. |
Use the break statement properly | After each case, use the break statement. This statement stops the execution of the remaining code in the switch block and exits. |
Consider enums for multiple constants | If you have a scenario with multi constants, consider using Enum with Switch statement; it can make code neater and less error-prone. |
The Role of Java Switch Statement in Programming
In programming, particularly in Java, a Switch Statement has an essential role. Switch Statement expedites decision-making processes based on specific conditions and optimises the readability of the code. Typically, as an alternative to 'if-else' statements, it facilitates the swift execution of code based on different case values.Usage of Java Switch Statement in Programming
In programming, the usage of the Java Switch Statement is primarily seen for simplifying complex conditionals. These are instances where numerous values of a variable or an expression might require an appropriate response. By defining these expected outcomes as cases within a switch statement, the resulting output can be coded to respond optimally.char grade = 'A'; switch (grade) { case 'A': System.out.println("Excellent!"); break; case 'B': case 'C': System.out.println("Well done"); break; case 'D': System.out.println("You passed"); break; case 'F': System.out.println("Better luck next time"); break; default: System.out.println("Invalid grade"); }In this example, the variable 'grade' is defined and evaluated within the switch statement. There are several cases defined in accordance with potential grade values. Each case has specific feedback tied to it that will print if that case is assessed. The Switch Statement is not limited to only basic types like integers or characters. It can also work with String variables, which enriches the range of possibilities when working with conditionals. Additionally, the Switch Statement has a significant impact on enhancing programming efficiency. Compared with repeated 'if-else' formulations, a switch statement aids developers in writing cleaner and more concise code. One important concept to remember while using Switch Statements is the break keyword. Departing from a case once it has been successfully executed is necessary, and this is precisely what the 'break' keyword achieves. Otherwise, if 'break' is not used properly, conditions might cascade into the next case, resulting in potential logic errors or unwanted outputs.
The Impact and Importance of Java Switch Statement in Computer Science
In the realm of computer science, the Java Switch Statement is far from trivial. It is a powerful tool providing developers with a simplified approach to handling several potential input or variable values. By enabling developers to define several cases for different outcomes, it supports the construction of intricate conditional statements. This versatility significantly impacts the ability to write efficient and cleaner codes. Furthermore, the execution of the Switch Statement is usually faster than a same-sized sequence of if-else statements. This benefit arises from the fact that, in a Switch Statement, the evaluation is only performed once. In an if-else statement, each condition would be evaluated until a true condition is encountered. The Java Switch Statement has made an undeniable mark on the field of computer science and continues to be used extensively. It is integral to concepts like control flow, which is the order in which individual statements, instructions, or function calls are executed or evaluated in a script or a function. Precise controls like these greatly influence the control over the execution of the program, thereby optimising the overall program efficiency. Notability, Switch Statements are not only limited to Java but are prevalent in many other programming languages, offering similar functionalities. It shows the importance and impact that this concept has had on the computer science world. This wide adoption and the fundamental role played by switch statements in the code structure undeniably highlight its significance in the field. Remarkably, with the advancement of Java over the years, features like the enhanced switch (introduced in Java 12), have allowed for more concise code writing and improved readability. This testifies to the ongoing evolution and importance of the Java Switch Statement in the realm of computer science and programming. Therefore, having a thorough understanding of this tool is immensely beneficial, whether you're a novice coder or a seasoned software engineer.Java Switch Statement - Key takeaways
- A Java switch statement executes a block of code based on the value of an expression, which must return an int, char, byte, or short.
- In a switch statement, 'case' is a constant condition that leads to the execution of certain code, 'break' ends this execution, and 'default' is carried out when none of the cases are true.
- The switch statement syntax in Java involves the keyword 'switch' followed by an expression in parentheses, which is followed by 'case' labels and an optional 'default' label enclosed in curly braces.
- Switch statement expression should be a variable or constant that yields a single value, each case identifies a value compared with the result of the expression, and 'default' executes when no case matches.
- The creation of a Java switch statement involves setting an expression for evaluation, defining possible case values with accompanying code, and adding a 'default' clause to handle unmatched cases.
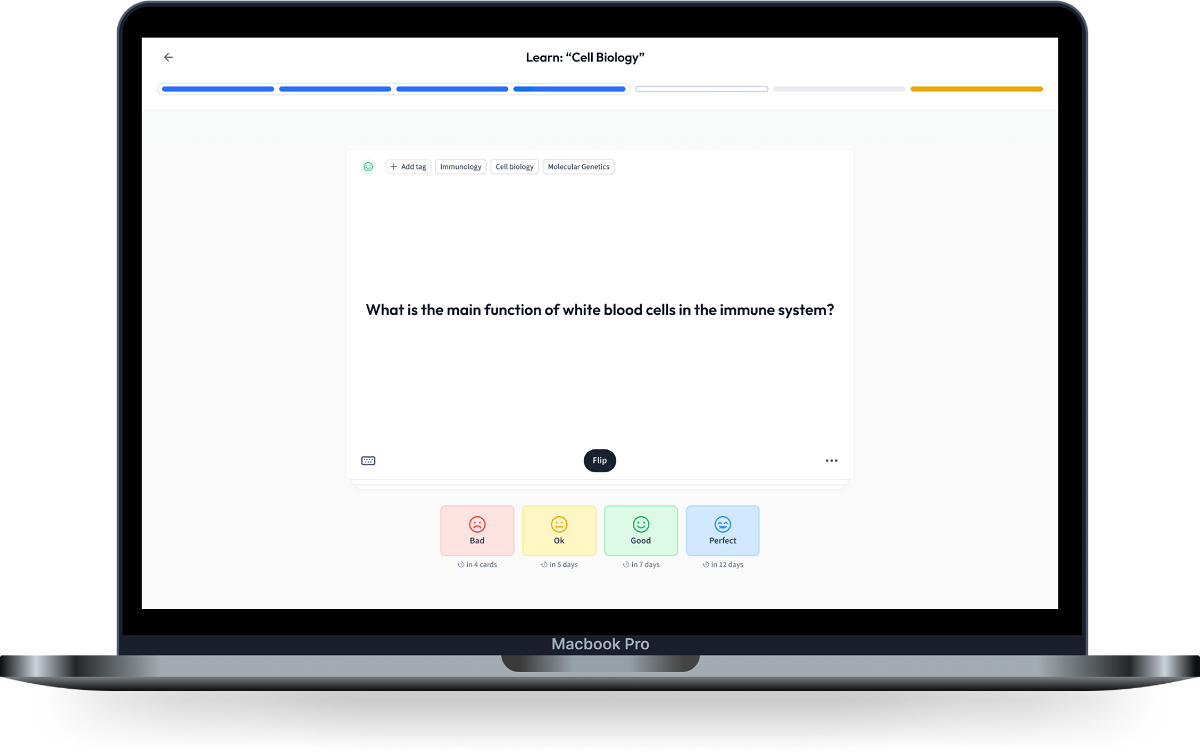
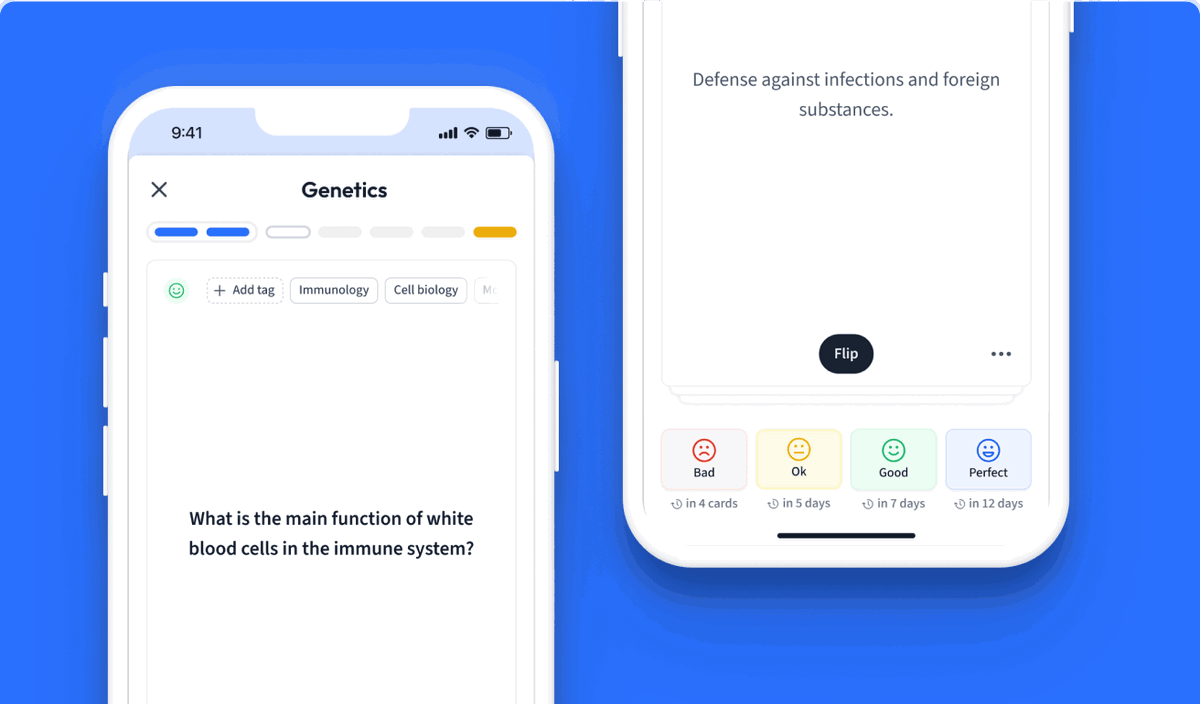
Learn with 12 Java Switch Statement flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Java Switch Statement
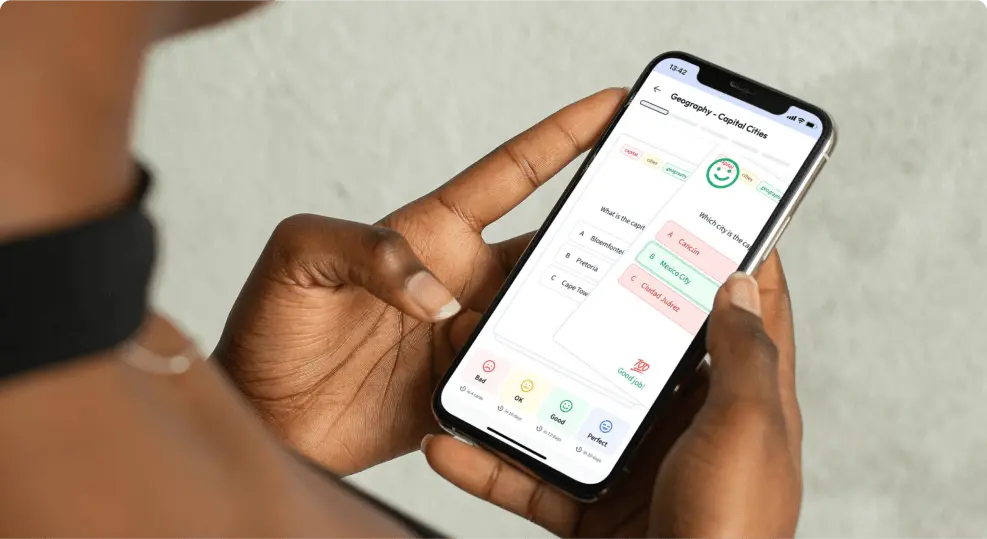
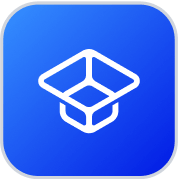
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more