Meaning of Variable Program in Computer Science
In the realm of computer science and programming, variables play a pivotal role in storing and manipulating data. As a basic building block of any programming language, understanding variables is crucial for grasping the fundamentals of coding and implementing a variable program.The concept of variable meaning in programming
A variable in programming refers to a named memory location that can store data of a specific type.
In this example, 'number' is a variable that stores the integer value 5, and 'message' is another variable containing the string "Hello, world!".
Importance of variables in coding and programs
Variables are indispensable in programming for several reasons:- Storing and manipulating data: By using variables, you can efficiently store and manage data in your programs, allowing you to perform calculations and other data manipulations easily.
- Code readability and maintainability: Variables help in making your code easier to read and understand by assigning meaningful names to the data being used in your program. This makes it easier to maintain and update the code in the future.
- Dynamic behaviour: Variables allow your program to change its behaviour based on the data stored in them. For example, you can write a program that asks users to input their age and then display a personalised message depending on the user's age.
- Reuse of code: Variables can be used to store intermediate results and reuse them in later parts of your program, reducing code redundancy and improving performance.
Different programming languages have their own syntax and rules when it comes to declaring and using variables. However, the underlying concept of variables as containers for data remains consistent across different programming languages.
Examples of Variables in Programming and Their Uses
Variables are extensively used in real-world programming applications to store and process data, enable dynamic behaviour, and enhance the readability of the code. Let's delve into some real-life examples of variables in programming: 1. User input handling: In applications where user input is required, variables can help store the user's inputs and process them accordingly. For example, a calculator application may use variables to store numbers entered by the user, as well as the results of calculations.num1 = float(input("Enter the first number: ")) num2 = float(input("Enter the second number: ")) sum = num1 + num2 print("The sum of the two numbers is: ", sum)
In this example, 'num1' and 'num2' are variables, which store the numbers entered by the user, while 'sum' is another variable used to store the result of the addition.
let productId = "P12345"; let productName = "Laptop"; let price = 999.99; let stockAvailable = 150;
Here, 'productId', 'productName', 'price', and 'stockAvailable' are variables that store different data types to represent the product's properties.
3. Gaming applications: In video games, variables can be employed to track and manipulate various aspects, such as the player's health, score, and position.
int playerHealth = 100; int playerScore = 0; Vector3 playerPosition = new Vector3(0, 0, 0);
In this example, 'playerHealth' and 'playerScore' store integer values, while 'playerPosition' is a variable of type Vector3 that represents the player's position in a 3D space.
Application of acute program variables in programming projects
In programming projects, acute program variables play a vital role in efficiently managing data, controlling the flow of the program, and adding flexibility to the project. Below are some examples of how acute program variables can be effectively utilised in programming projects: 1. Loop control: Loop constructions, like for, while, and foreach, use variables as loop control variables to count loop iterations, access elements in a collection, or cause the loop to exit under certain conditions. For example:for (int i = 0; i < 10; i++) { System.out.println("Iteration " + i); }
In this example, 'i' is the loop control variable, which stores the current loop iteration and is used to exit the loop when it reaches a specified value.
$config = array( "database" => "mysql:host=localhost;dbname=mydb", "username" => "root", "password" => "examplePassword" );
Here, the variable '$config' stores an associative array containing configuration settings for connecting to a database.
data = [1, 2, 3, 4, 5] squared_data = [] data.each do |element| squared_element = element ** 2 squared_data.push(squared_element) end
This Ruby code demonstrates the use of variables to store the initial data, the squared data, and the squared elements of each iteration, ultimately creating a new array of squared elements.
Exploring Different Types of Variables in Programming
In programming, variables can be classified based on the data types they can store. A data type can be defined as the set of values a variable can hold and the operations that can be performed on them. Different programming languages offer a variety of built-in data types to store various kinds of data. These data types can be broadly categorised into the following types:Deeper knowledge on diverse types of variables in programming
Primitive data types:
These are the fundamental data types provided by programming languages, which are used to store basic data such as integers, floating-point numbers, characters and boolean values. Examples include:
1. Integer: Variables of integer type are used to store whole numbers, both positive and negative. E.g., 3, -15, 42. ( int myInteger = 10; )
2. Floating-point: These variables store real numbers that include decimal points. E.g., 2.718, -3.14, 0.0035. ( my_float = 3.14159 )
3. Character: Character data type variables store single characters, usually represented as Unicode or ASCII values. E.g., 'A', 'z', '4', '#'. ( char myCharacter = 'G'; )
4. Boolean: A boolean variable stores a binary value, either true or false. (let myBoolean = true;)
Derived data types:
Derived data types can be created using primitive data types and are available in most programming languages. Examples include:
1. Arrays: Arrays are data structures that store a fixed number of elements of the same data type. Elements in an array can be accessed using their index position, ( int[] myArray = new int[5] {1, 2, 3, 4, 5}; )
2. Functions: Functions are blocks of code that perform specific tasks when called. Functions may have parameters that allow the passing of data in the form of arguments. Variables can also be used to store the result of a function. ( function addNumbers($num1, $num2) { $sum = $num1 + $num2; return $sum; } $result = addNumbers(3, 5); )
3. Structures: Structures are user-defined data types that group variables of different data types under a single name. In programming languages such as C++, structures are declared using the `struct` keyword. ( struct Student { int id; float gpa; char grade; }; Student myStudent; myStudent.id = 123; myStudent.gpa = 3.9; myStudent.grade = 'A'; )
Composite data types
Composite data types, also known as containers, are data structures that store collections of data, which can be of the same or different data types. Examples include lists, sets, dictionaries, and tuples.
1. Lists: Lists are ordered collections of items, which can be of different data types. Lists are mutable, meaning their elements can be modified. ( my_list = [1, 2, 'apple', 3.5] )
2. Dictionaries: Dictionaries, also known as associative arrays or hash maps, are collections of key-value pairs, where each key is associated with a value. The keys are unique, while the values can be of any data type. ( let myDictionary = { "name": "John", "age": 30, "city": "New York" }; )
Understanding the different types of variables based on their data types is essential for writing efficient and robust programs. These diverse types of variables enable you to store and manipulate a wide range of data according to your programming requirements.
Variable Program - Key takeaways
Variable Program: Named memory location to store data of a specific type.
Variable meaning in programming: Named memory location holding changeable data, e.g., integers, floating-point numbers, strings.
Examples of variables in programming: User input handling, e-commerce websites, gaming applications.
Acute program variables: Efficiently manage data, control program flow, and add flexibility to programming projects.
Different types of variables in programming: Primitive data types, derived data types, and composite data types (e.g., arrays, functions, lists, dictionaries).
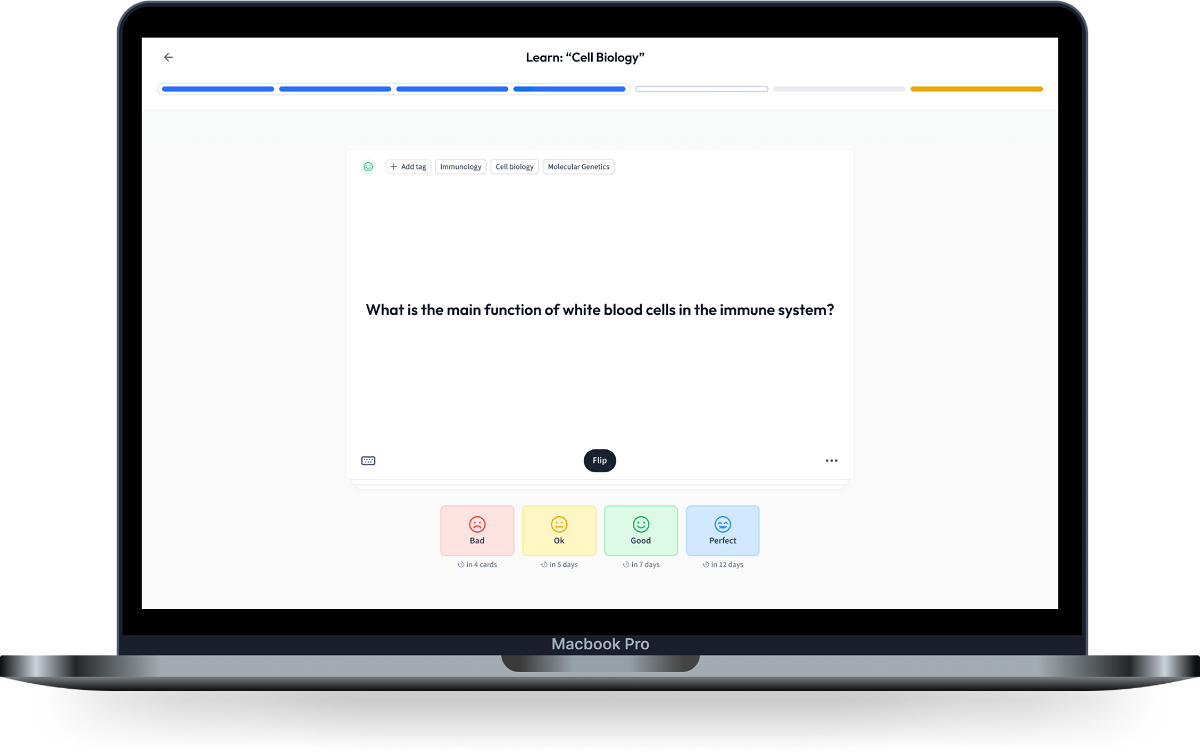
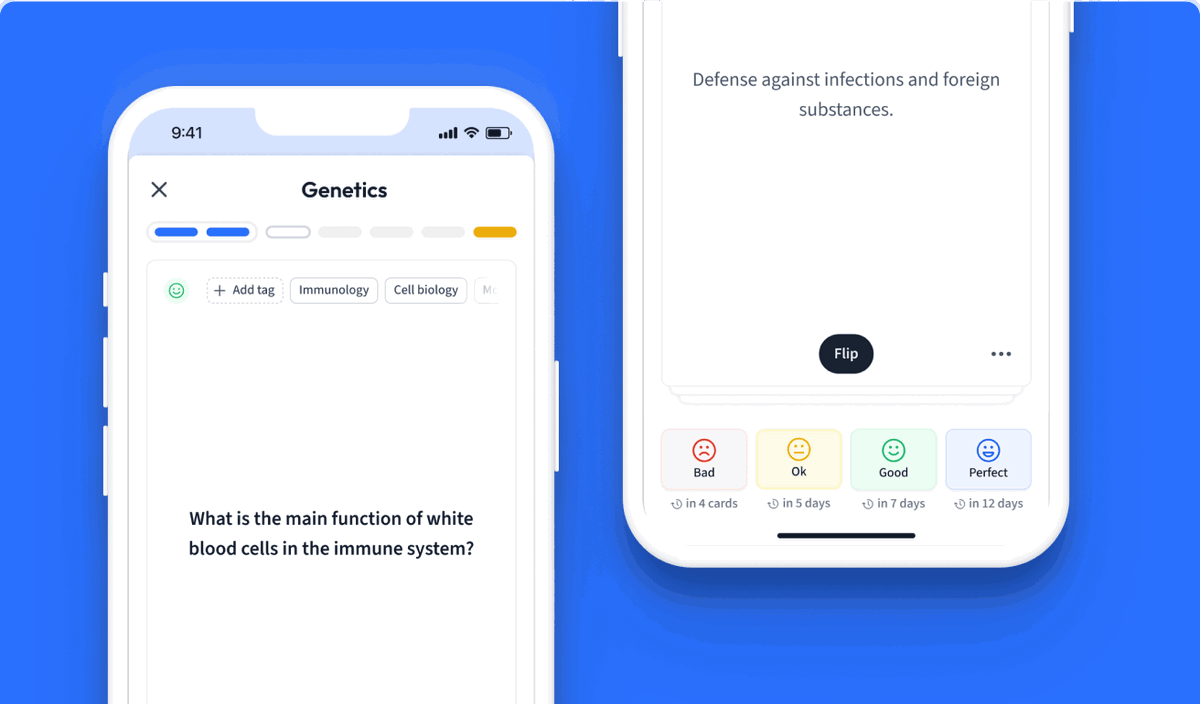
Learn with 43 Variable Program flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Variable Program
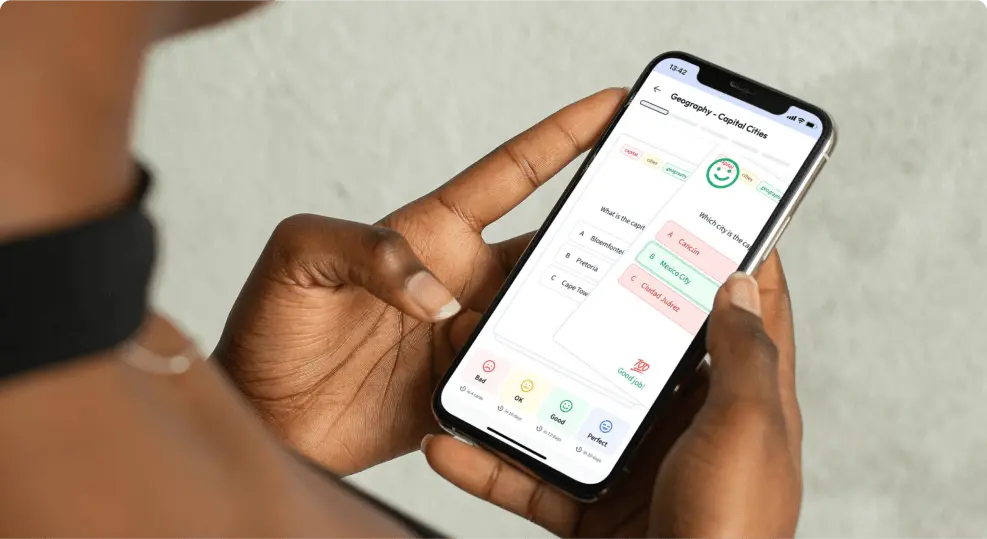
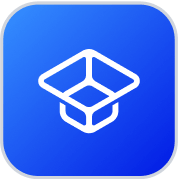
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more